C++ Operator Overloading Guidelines
One of the nice features of C++ is that you can give special meanings to operators, when they are used with user-defined classes. This is called operator overloading . You can implement C++ operator overloads by providing special member-functions on your classes that follow a particular naming convention. For example, to overload the + operator for your class, you would provide a member-function named operator+ on your class.
- = (assignment operator)
- + - * (binary arithmetic operators)
- += -= *= (compound assignment operators)
- == != (comparison operators)
Here are some guidelines for implementing these operators. These guidelines are very important to follow, so definitely get in the habit early.
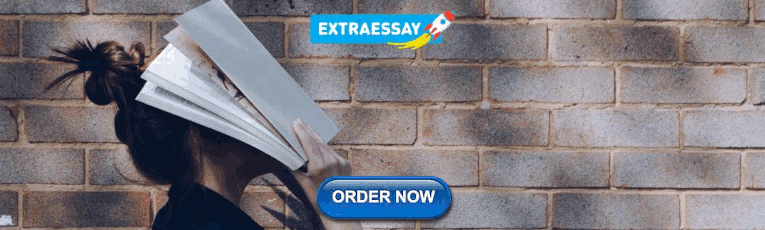
Assignment Operator =
The assignment operator has a signature like this: class MyClass { public: ... MyClass & operator=(const MyClass &rhs); ... } MyClass a, b; ... b = a; // Same as b.operator=(a);
Notice that the = operator takes a const-reference to the right hand side of the assignment. The reason for this should be obvious, since we don't want to change that value; we only want to change what's on the left hand side.
- e = 42 assigns 42 to e , then returns e as the result
- The value of e is then assigned to d , and then d is returned as the result
- The value of d is then assigned to c , and then c is returned as the result
Now, in order to support operator chaining, the assignment operator must return some value. The value that should be returned is a reference to the left-hand side of the assignment.
Notice that the returned reference is not declared const . This can be a bit confusing, because it allows you to write crazy stuff like this: MyClass a, b, c; ... (a = b) = c; // What?? At first glance, you might want to prevent situations like this, by having operator= return a const reference. However, statements like this will work with primitive types. And, even worse, some tools actually rely on this behavior. Therefore, it is important to return a non- const reference from your operator= . The rule of thumb is, "If it's good enough for int s, it's good enough for user-defined data-types."
So, for the hypothetical MyClass assignment operator, you would do something like this: // Take a const-reference to the right-hand side of the assignment. // Return a non-const reference to the left-hand side. MyClass& MyClass::operator=(const MyClass &rhs) { ... // Do the assignment operation! return *this; // Return a reference to myself. } Remember, this is a pointer to the object that the member function is being called on. Since a = b is treated as a.operator=(b) , you can see why it makes sense to return the object that the function is called on; object a is the left-hand side.
But, the member function needs to return a reference to the object, not a pointer to the object. So, it returns *this , which returns what this points at (i.e. the object), not the pointer itself. (In C++, instances are turned into references, and vice versa, pretty much automatically, so even though *this is an instance, C++ implicitly converts it into a reference to the instance.)
Now, one more very important point about the assignment operator:
YOU MUST CHECK FOR SELF-ASSIGNMENT!
This is especially important when your class does its own memory allocation. Here is why: The typical sequence of operations within an assignment operator is usually something like this: MyClass& MyClass::operator=(const MyClass &rhs) { // 1. Deallocate any memory that MyClass is using internally // 2. Allocate some memory to hold the contents of rhs // 3. Copy the values from rhs into this instance // 4. Return *this } Now, what happens when you do something like this: MyClass mc; ... mc = mc; // BLAMMO. You can hopefully see that this would wreak havoc on your program. Because mc is on the left-hand side and on the right-hand side, the first thing that happens is that mc releases any memory it holds internally. But, this is where the values were going to be copied from, since mc is also on the right-hand side! So, you can see that this completely messes up the rest of the assignment operator's internals.
The easy way to avoid this is to CHECK FOR SELF-ASSIGNMENT. There are many ways to answer the question, "Are these two instances the same?" But, for our purposes, just compare the two objects' addresses. If they are the same, then don't do assignment. If they are different, then do the assignment.
So, the correct and safe version of the MyClass assignment operator would be this: MyClass& MyClass::operator=(const MyClass &rhs) { // Check for self-assignment! if (this == &rhs) // Same object? return *this; // Yes, so skip assignment, and just return *this. ... // Deallocate, allocate new space, copy values... return *this; } Or, you can simplify this a bit by doing: MyClass& MyClass::operator=(const MyClass &rhs) { // Only do assignment if RHS is a different object from this. if (this != &rhs) { ... // Deallocate, allocate new space, copy values... } return *this; } Remember that in the comparison, this is a pointer to the object being called, and &rhs is a pointer to the object being passed in as the argument. So, you can see that we avoid the dangers of self-assignment with this check.
- Take a const-reference for the argument (the right-hand side of the assignment).
- Return a reference to the left-hand side, to support safe and reasonable operator chaining. (Do this by returning *this .)
- Check for self-assignment, by comparing the pointers ( this to &rhs ).
Compound Assignment Operators += -= *=
I discuss these before the arithmetic operators for a very specific reason, but we will get to that in a moment. The important point is that these are destructive operators, because they update or replace the values on the left-hand side of the assignment. So, you write: MyClass a, b; ... a += b; // Same as a.operator+=(b) In this case, the values within a are modified by the += operator.
How those values are modified isn't very important - obviously, what MyClass represents will dictate what these operators mean.
The member function signature for such an operator should be like this: MyClass & MyClass::operator+=(const MyClass &rhs) { ... } We have already covered the reason why rhs is a const-reference. And, the implementation of such an operation should also be straightforward.
But, you will notice that the operator returns a MyClass -reference, and a non-const one at that. This is so you can do things like this: MyClass mc; ... (mc += 5) += 3;
Don't ask me why somebody would want to do this, but just like the normal assignment operator, this is allowed by the primitive data types. Our user-defined datatypes should match the same general characteristics of the primitive data types when it comes to operators, to make sure that everything works as expected.
This is very straightforward to do. Just write your compound assignment operator implementation, and return *this at the end, just like for the regular assignment operator. So, you would end up with something like this: MyClass & MyClass::operator+=(const MyClass &rhs) { ... // Do the compound assignment work. return *this; }
As one last note, in general you should beware of self-assignment with compound assignment operators as well. Fortunately, none of the C++ track's labs require you to worry about this, but you should always give it some thought when you are working on your own classes.
Binary Arithmetic Operators + - *
The binary arithmetic operators are interesting because they don't modify either operand - they actually return a new value from the two arguments. You might think this is going to be an annoying bit of extra work, but here is the secret:
Define your binary arithmetic operators using your compound assignment operators.
There, I just saved you a bunch of time on your homeworks.
So, you have implemented your += operator, and now you want to implement the + operator. The function signature should be like this: // Add this instance's value to other, and return a new instance // with the result. const MyClass MyClass::operator+(const MyClass &other) const { MyClass result = *this; // Make a copy of myself. Same as MyClass result(*this); result += other; // Use += to add other to the copy. return result; // All done! } Simple!
Actually, this explicitly spells out all of the steps, and if you want, you can combine them all into a single statement, like so: // Add this instance's value to other, and return a new instance // with the result. const MyClass MyClass::operator+(const MyClass &other) const { return MyClass(*this) += other; } This creates an unnamed instance of MyClass , which is a copy of *this . Then, the += operator is called on the temporary value, and then returns it.
If that last statement doesn't make sense to you yet, then stick with the other way, which spells out all of the steps. But, if you understand exactly what is going on, then you can use that approach.
You will notice that the + operator returns a const instance, not a const reference. This is so that people can't write strange statements like this: MyClass a, b, c; ... (a + b) = c; // Wuh...? This statement would basically do nothing, but if the + operator returns a non- const value, it will compile! So, we want to return a const instance, so that such madness will not even be allowed to compile.
- Implement the compound assignment operators from scratch, and then define the binary arithmetic operators in terms of the corresponding compound assignment operators.
- Return a const instance, to prevent worthless and confusing assignment operations that shouldn't be allowed.
Comparison Operators == and !=
The comparison operators are very simple. Define == first, using a function signature like this: bool MyClass::operator==(const MyClass &other) const { ... // Compare the values, and return a bool result. } The internals are very obvious and straightforward, and the bool return-value is also very obvious.
The important point here is that the != operator can also be defined in terms of the == operator, and you should do this to save effort. You can do something like this: bool MyClass::operator!=(const MyClass &other) const { return !(*this == other); } That way you get to reuse the hard work you did on implementing your == operator. Also, your code is far less likely to exhibit inconsistencies between == and != , since one is implemented in terms of the other.
- Sign In / Suggest an Article
Current ISO C++ status
Upcoming ISO C++ meetings
Upcoming C++ conferences
Compiler conformance status
ISO C++ committee meeting
June 24-29, St. Louis, MO, USA
July 2-5, Folkestone, Kent, UK
assignment operators
Assignment operators, what is “self assignment”.
Self assignment is when someone assigns an object to itself. For example,
Obviously no one ever explicitly does a self assignment like the above, but since more than one pointer or reference can point to the same object (aliasing), it is possible to have self assignment without knowing it:
This is only valid for copy assignment. Self-assignment is not valid for move assignment.
Why should I worry about “self assignment”?
If you don’t worry about self assignment , you’ll expose your users to some very subtle bugs that have very subtle and often disastrous symptoms. For example, the following class will cause a complete disaster in the case of self-assignment:
If someone assigns a Fred object to itself, line #1 deletes both this->p_ and f.p_ since *this and f are the same object. But line #2 uses *f.p_ , which is no longer a valid object. This will likely cause a major disaster.
The bottom line is that you the author of class Fred are responsible to make sure self-assignment on a Fred object is innocuous . Do not assume that users won’t ever do that to your objects. It is your fault if your object crashes when it gets a self-assignment.
Aside: the above Fred::operator= (const Fred&) has a second problem: If an exception is thrown while evaluating new Wilma(*f.p_) (e.g., an out-of-memory exception or an exception in Wilma ’s copy constructor ), this->p_ will be a dangling pointer — it will point to memory that is no longer valid. This can be solved by allocating the new objects before deleting the old objects.
Okay, okay, already; I’ll handle self-assignment. How do I do it?
You should worry about self assignment every time you create a class . This does not mean that you need to add extra code to all your classes: as long as your objects gracefully handle self assignment, it doesn’t matter whether you had to add extra code or not.
We will illustrate the two cases using the assignment operator in the previous FAQ :
If self-assignment can be handled without any extra code, don’t add any extra code. But do add a comment so others will know that your assignment operator gracefully handles self-assignment:
Example 1a:
Example 1b:
If you need to add extra code to your assignment operator, here’s a simple and effective technique:
Or equivalently:
By the way: the goal is not to make self-assignment fast. If you don’t need to explicitly test for self-assignment, for example, if your code works correctly (even if slowly) in the case of self-assignment, then do not put an if test in your assignment operator just to make the self-assignment case fast. The reason is simple: self-assignment is almost always rare, so it merely needs to be correct - it does not need to be efficient. Adding the unnecessary if statement would make a rare case faster by adding an extra conditional-branch to the normal case, punishing the many to benefit the few.
In this case, however, you should add a comment at the top of your assignment operator indicating that the rest of the code makes self-assignment is benign, and that is why you didn’t explicitly test for it. That way future maintainers will know to make sure self-assignment stays benign, or if not, they will need to add the if test.
I’m creating a derived class; should my assignment operators call my base class’s assignment operators?
Yes (if you need to define assignment operators in the first place).
If you define your own assignment operators, the compiler will not automatically call your base class’s assignment operators for you. Unless your base class’s assignment operators themselves are broken, you should call them explicitly from your derived class’s assignment operators (again, assuming you create them in the first place).
However if you do not create your own assignment operators, the ones that the compiler create for you will automatically call your base class’s assignment operators.
Please Login to submit a recommendation.
If you don’t have an account, you can register for free.
C++ overloading: operators to overload as methods of a class
Become a Software Engineer in Months, Not Years
From your first line of code, to your first day on the job — Educative has you covered. Join 2M+ developers learning in-demand programming skills.
Operator overloading is an interesting topic of object-oriented programming using C++. Operator overloading enables the objects of a class to be used in expressions. Some operators must be overloaded as non-static member functions of the class. This blog discusses these operators with coding examples.
Let’s dive right in!
We’ll cover:
Introduction
- Assignment operators
Single overload
Two ways to overload, noninteger index, function call operator, operators that are preferred as members, wrapping up and next steps.
Operators are overloaded as functions in C++. They can be overloaded in various ways: as member functions only, as nonmembers only, and as both members and nonmembers. Here, we discuss the operators that can be overloaded as non-static member functions only. The first operand or the caller of these operators is always an object of the class. Other aspects related to operator overloading are discussed in Operator Overloading in C++ and Various Ways of Overloading an Operator in C++ .
From this point onward, we’ll keep using and extending this minimal implementation of the Time class.
Lines 6–29: We define a Time class as described before the code. Additionally, it defines the subscript operator ( lines 21–28 ).
- Line 21: This receives a string parameter to mention the specific attribute that we want to access for read/write. It accepts only "hour" , "minute" , or "second" as an argument. It throws an exception if it receives any other string.
Lines 31–43: We define the main() function that creates an object noon of type Time .
Line 36: We call the subscript operator to read the value of "hour" from noon .
Line 39: We call the subscript operator to write the value of "second" to noon .
Zero to Hero in C++
Learn in-demand tech skills in half the time
Mock Interview
Skill Paths
Assessments
Learn to Code
Tech Interview Prep
Generative AI
Data Science
Machine Learning
GitHub Students Scholarship
Early Access Courses
For Individuals
Try for Free
Gift a Subscription
Become an Author
Become an Affiliate
Earn Referral Credits
Cheatsheets
Frequently Asked Questions
Privacy Policy
Cookie Policy
Terms of Service
Business Terms of Service
Data Processing Agreement
Copyright © 2024 Educative, Inc. All rights reserved.
How to Implement Assignment Operator Overloading in C++
- How to Implement Assignment Operator …
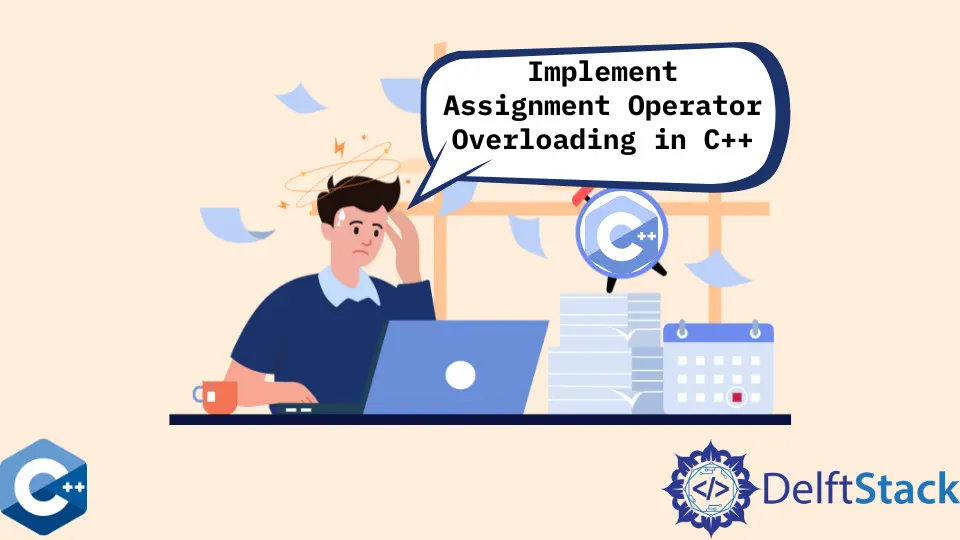
This article will explain several methods of how to implement assignment operator overloading in C++.
Use copy-assignment operator to Implement Overloaded Assignment Operator in C++
C++ provides the feature to overload operators, a common way to call custom functions when a built-in operator is called on specific classes. These functions should have a special name starting with operator followed by the specific operator symbol itself. E.g., a custom assignment operator can be implemented with the function named operator= . Assignment operator generally should return a reference to its left-hand operand. Note that if the user does not explicitly define the copy assignment operator, the compiler generates one automatically. The generated version is quite capable when the class does not contain any data members manually allocated on the heap memory. It can even handle the array members by assigning each element to the corresponding object members. Although, it has shortcomings when dealing with dynamic memory data members, as shown in the following example code.
The above code defines only copy-constructor explicitly, which results in incorrect behavior when P1 object contents are assigned to the P3 object. Note that the second call to the P1.renamePerson function should not have modified the P3 object’s data members, but it did. The solution to this is to define an overloaded assignment operator i.e., copy-assignment operator. The next code snippet implements the version of the Person class that can copy assign the two objects of the same class correctly. Notice, though, the if statement in the copy-assignment function guarantees that the operator works correctly even when the object is assigned to itself.
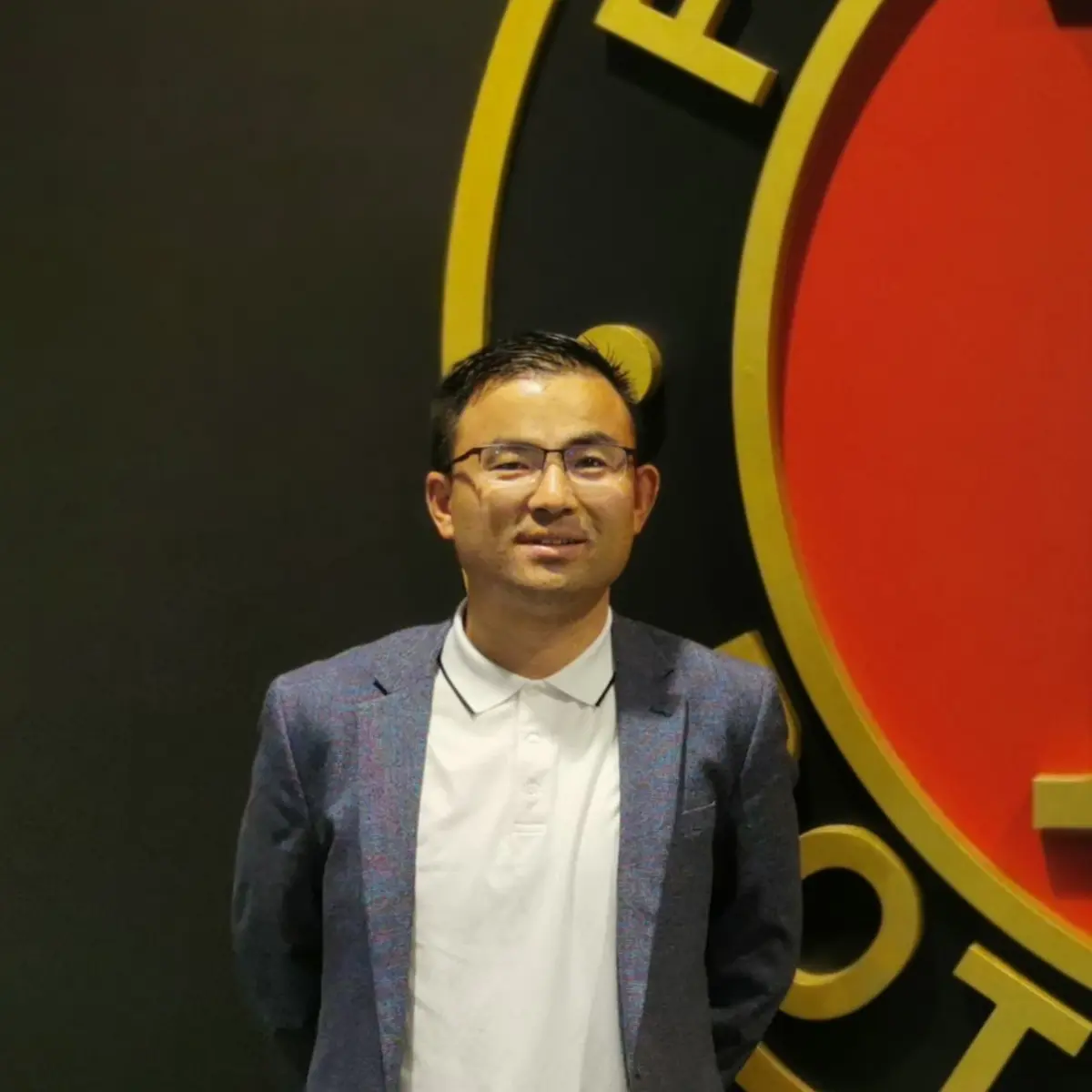
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
Related Article - C++ Class
- How to Initialize Static Variables in C++ Class
- How to Get Class Name in C++
- Point and Line Class in C++
- Class Template Inheritance in C++
- Difference Between Structure and Class in C++
- Wrapper Class in C++

25.4 — Virtual destructors, virtual assignment, and overriding virtualization
Virtual destructors
Although C++ provides a default destructor for your classes if you do not provide one yourself, it is sometimes the case that you will want to provide your own destructor (particularly if the class needs to deallocate memory). You should always make your destructors virtual if you’re dealing with inheritance. Consider the following example:
Note: If you compile the above example, your compiler may warn you about the non-virtual destructor (which is intentional for this example). You may need to disable the compiler flag that treats warnings as errors to proceed.
Because base is a Base pointer, when base is deleted, the program looks to see if the Base destructor is virtual. It’s not, so it assumes it only needs to call the Base destructor. We can see this in the fact that the above example prints:
However, we really want the delete function to call Derived’s destructor (which will call Base’s destructor in turn), otherwise m_array will not be deleted. We do this by making Base’s destructor virtual:
Now this program produces the following result:
Whenever you are dealing with inheritance, you should make any explicit destructors virtual.
As with normal virtual member functions, if a base class function is virtual, all derived overrides will be considered virtual regardless of whether they are specified as such. It is not necessary to create an empty derived class destructor just to mark it as virtual.
Note that if you want your base class to have a virtual destructor that is otherwise empty, you can define your destructor this way:
Virtual assignment
It is possible to make the assignment operator virtual. However, unlike the destructor case where virtualization is always a good idea, virtualizing the assignment operator really opens up a bag full of worms and gets into some advanced topics outside of the scope of this tutorial. Consequently, we are going to recommend you leave your assignments non-virtual for now, in the interest of simplicity.
Ignoring virtualization
Very rarely you may want to ignore the virtualization of a function. For example, consider the following code:
There may be cases where you want a Base pointer to a Derived object to call Base::getName() instead of Derived::getName(). To do so, simply use the scope resolution operator:
You probably won’t use this very often, but it’s good to know it’s at least possible.
Should we make all destructors virtual?
This is a common question asked by new programmers. As noted in the top example, if the base class destructor isn’t marked as virtual, then the program is at risk for leaking memory if a programmer later deletes a base class pointer that is pointing to a derived object. One way to avoid this is to mark all your destructors as virtual. But should you?
It’s easy to say yes, so that way you can later use any class as a base class -- but there’s a performance penalty for doing so (a virtual pointer added to every instance of your class). So you have to balance that cost, as well as your intent.
Conventional wisdom (as initially put forth by Herb Sutter, a highly regarded C++ guru) has suggested avoiding the non-virtual destructor memory leak situation as follows, “A base class destructor should be either public and virtual, or protected and nonvirtual.” A class with a protected destructor can’t be deleted via a pointer, thus preventing the accidental deleting of a derived class through a base pointer when the base class has a non-virtual destructor. Unfortunately, this also means the base class can’t be deleted through a base class pointer, which essentially means the class can’t be dynamically allocated or deleted except by a derived class. This also precludes using smart pointers (such as std::unique_ptr and std::shared_ptr) for such classes, which limits the usefulness of that rule. It also means the base class can’t be allocated on the stack. That’s a pretty heavy set of penalties.
Now that the final specifier has been introduced into the language, our recommendations are as follows:
- If you intend your class to be inherited from, make sure your destructor is virtual.
- If you do not intend your class to be inherited from, mark your class as final. This will prevent other classes from inheriting from it in the first place, without imposing any other use restrictions on the class itself.
cppreference.com
Using-declaration.
Introduces a name that is defined elsewhere into the declarative region where this using-declaration appears. See using enum and (since C++20) using namespace for other related declarations.
[ edit ] Explanation
Using-declarations can be used to introduce namespace members into other namespaces and block scopes, or to introduce base class members into derived class definitions , or to introduce enumerators into namespaces, block, and class scopes (since C++20) .
[ edit ] In namespace and block scope
Using-declarations introduce a member of another namespace into the current namespace or block scope.
See namespace for details.
[ edit ] In class definition
Using-declaration introduces a member of a base class into the derived class definition, such as to expose a protected member of base as public member of derived. In this case, nested-name-specifier must name a base class of the one being defined. If the name is the name of an overloaded member function of the base class, all base class member functions with that name are introduced. If the derived class already has a member with the same name, parameter list, and qualifications, the derived class member hides or overrides (doesn't conflict with) the member that is introduced from the base class.
[ edit ] Notes
Only the name explicitly mentioned in the using-declaration is transferred into the declarative scope: in particular, enumerators are not transferred when the enumeration type name is using-declared.
A using-declaration cannot refer to a namespace , to a scoped enumerator (until C++20) , to a destructor of a base class or to a specialization of a member template for a user-defined conversion function.
A using-declaration cannot name a member template specialization ( template-id is not permitted by the grammar):
A using-declaration also can't be used to introduce the name of a dependent member template as a template-name (the template disambiguator for dependent names is not permitted).
If a using-declaration brings the base class assignment operator into derived class, whose signature happens to match the derived class's copy-assignment or move-assignment operator, that operator is hidden by the implicitly-declared copy/move assignment operator of the derived class. Same applies to a using-declaration that inherits a base class constructor that happens to match the derived class copy/move constructor (since C++11) .
[ edit ] Defect reports
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
- Pages with unreviewed CWG DR marker
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 29 January 2024, at 04:58.
- This page has been accessed 910,304 times.
- Privacy policy
- About cppreference.com
- Disclaimers

- C++ Data Types
C++ Input/Output
- C++ Pointers
C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
C++ Exception Handling
- C++ Memory Management
- C++ Programming Language
C++ Overview
- Introduction to C++ Programming Language
- Features of C++
- History of C++
- Interesting Facts about C++
- Setting up C++ Development Environment
- Difference between C and C++
- Writing First C++ Program - Hello World Example
- C++ Basic Syntax
- C++ Comments
- Tokens in C
- C++ Keywords
- Difference between Keyword and Identifier
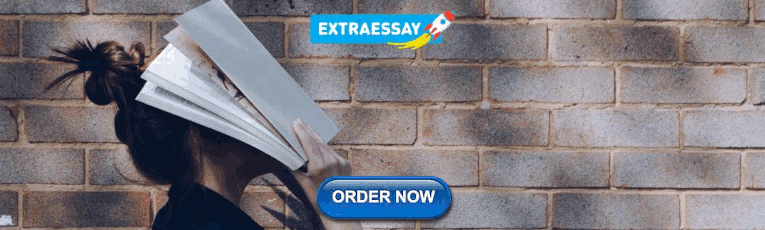
C++ Variables and Constants
- C++ Variables
- Constants in C
- Scope of Variables in C++
- Storage Classes in C++ with Examples
- Static Keyword in C++
C++ Data Types and Literals
- Literals in C
- Derived Data Types in C++
- User Defined Data Types in C++
- Data Type Ranges and their macros in C++
- C++ Type Modifiers
- Type Conversion in C++
- Casting Operators in C++
C++ Operators
- Operators in C++
- C++ Arithmetic Operators
- Unary operators in C
- Bitwise Operators in C
- Assignment Operators in C
- C++ sizeof Operator
- Scope resolution operator in C++
- Basic Input / Output in C++
- cout in C++
- cerr - Standard Error Stream Object in C++
- Manipulators in C++ with Examples
C++ Control Statements
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C++ if Statement
- C++ if else Statement
- C++ if else if Ladder
- Switch Statement in C++
- Jump statements in C++
- for Loop in C++
- Range-based for loop in C++
- C++ While Loop
- C++ Do/While Loop
C++ Functions
- Functions in C++
- return statement in C++ with Examples
- Parameter Passing Techniques in C
- Difference Between Call by Value and Call by Reference in C
- Default Arguments in C++
- Inline Functions in C++
- Lambda expression in C++
C++ Pointers and References
- Pointers and References in C++
- Dangling, Void , Null and Wild Pointers in C
- Applications of Pointers in C
- Understanding nullptr in C++
- References in C++
- Can References Refer to Invalid Location in C++?
- Pointers vs References in C++
- Passing By Pointer vs Passing By Reference in C++
- When do we pass arguments by pointer?
- Variable Length Arrays (VLAs) in C
- Pointer to an Array | Array Pointer
- How to print size of array parameter in C++?
- Pass Array to Functions in C
- What is Array Decay in C++? How can it be prevented?
C++ Strings
- Strings in C++
- std::string class in C++
- Array of Strings in C++ - 5 Different Ways to Create
- String Concatenation in C++
- Tokenizing a string in C++
- Substring in C++
C++ Structures and Unions
- Structures, Unions and Enumerations in C++
- Structures in C++
- C++ - Pointer to Structure
- Self Referential Structures
- Difference Between C Structures and C++ Structures
- Enumeration in C++
- typedef in C++
- Array of Structures vs Array within a Structure in C
C++ Dynamic Memory Management
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- new and delete Operators in C++ For Dynamic Memory
- new vs malloc() and free() vs delete in C++
- What is Memory Leak? How can we avoid?
- Difference between Static and Dynamic Memory Allocation in C
C++ Object-Oriented Programming
- Object Oriented Programming in C++
- C++ Classes and Objects
- Access Modifiers in C++
- Friend Class and Function in C++
- Constructors in C++
- Default Constructors in C++
- Copy Constructor in C++
- Destructors in C++
- Private Destructor in C++
- When is a Copy Constructor Called in C++?
- Shallow Copy and Deep Copy in C++
- When Should We Write Our Own Copy Constructor in C++?
- Does C++ compiler create default constructor when we write our own?
- C++ Static Data Members
- Static Member Function in C++
- 'this' pointer in C++
- Scope Resolution Operator vs this pointer in C++
- Local Classes in C++
- Nested Classes in C++
- Enum Classes in C++ and Their Advantage over Enum DataType
- Difference Between Structure and Class in C++
- Why C++ is partially Object Oriented Language?
C++ Encapsulation and Abstraction
- Encapsulation in C++
- Abstraction in C++
- Difference between Abstraction and Encapsulation in C++
- C++ Polymorphism
- Function Overriding in C++
- Virtual Functions and Runtime Polymorphism in C++
- Difference between Inheritance and Polymorphism
C++ Function Overloading
- Function Overloading in C++
- Constructor Overloading in C++
- Functions that cannot be overloaded in C++
- Function overloading and const keyword
- Function Overloading and Return Type in C++
- Function Overloading and float in C++
- C++ Function Overloading and Default Arguments
- Can main() be overloaded in C++?
- Function Overloading vs Function Overriding in C++
- Advantages and Disadvantages of Function Overloading in C++
C++ Operator Overloading
- Operator Overloading in C++
- Types of Operator Overloading in C++
- Functors in C++
- What are the Operators that Can be and Cannot be Overloaded in C++?
C++ Inheritance
Inheritance in c++.
- C++ Inheritance Access
- Multiple Inheritance in C++
- C++ Hierarchical Inheritance
- C++ Multilevel Inheritance
- Constructor in Multiple Inheritance in C++
- Inheritance and Friendship in C++
- Does overloading work with Inheritance?
C++ Virtual Functions
- Virtual Function in C++
- Virtual Functions in Derived Classes in C++
- Default Arguments and Virtual Function in C++
- Can Virtual Functions be Inlined in C++?
- Virtual Destructor
- Advanced C++ | Virtual Constructor
- Advanced C++ | Virtual Copy Constructor
- Pure Virtual Functions and Abstract Classes in C++
- Pure Virtual Destructor in C++
- Can Static Functions Be Virtual in C++?
- RTTI (Run-Time Type Information) in C++
- Can Virtual Functions be Private in C++?
- Exception Handling in C++
- Exception Handling using classes in C++
- Stack Unwinding in C++
- User-defined Custom Exception with class in C++
C++ Files and Streams
- File Handling through C++ Classes
- I/O Redirection in C++
C++ Templates
- Templates in C++ with Examples
- Template Specialization in C++
- Using Keyword in C++ STL
C++ Standard Template Library (STL)
- The C++ Standard Template Library (STL)
- Containers in C++ STL (Standard Template Library)
- Introduction to Iterators in C++
- Algorithm Library | C++ Magicians STL Algorithm
C++ Preprocessors
- C Preprocessors
- C Preprocessor Directives
- #include in C
- Difference between Preprocessor Directives and Function Templates in C++
C++ Namespace
- Namespace in C++ | Set 1 (Introduction)
- namespace in C++ | Set 2 (Extending namespace and Unnamed namespace)
- Namespace in C++ | Set 3 (Accessing, creating header, nesting and aliasing)
- C++ Inline Namespaces and Usage of the "using" Directive Inside Namespaces
Advanced C++
- Multithreading in C++
- Smart Pointers in C++
- auto_ptr vs unique_ptr vs shared_ptr vs weak_ptr in C++
- Type of 'this' Pointer in C++
- "delete this" in C++
- Passing a Function as a Parameter in C++
- Signal Handling in C++
- Generics in C++
- Difference between C++ and Objective C
- Write a C program that won't compile in C++
- Write a program that produces different results in C and C++
- How does 'void*' differ in C and C++?
- Type Difference of Character Literals in C and C++
- Cin-Cout vs Scanf-Printf
C++ vs Java
- Similarities and Difference between Java and C++
- Comparison of Inheritance in C++ and Java
- How Does Default Virtual Behavior Differ in C++ and Java?
- Comparison of Exception Handling in C++ and Java
- Foreach in C++ and Java
- Templates in C++ vs Generics in Java
- Floating Point Operations & Associativity in C, C++ and Java
Competitive Programming in C++
- Competitive Programming - A Complete Guide
- C++ tricks for competitive programming (for C++ 11)
- Writing C/C++ code efficiently in Competitive programming
- Why C++ is best for Competitive Programming?
- Test Case Generation | Set 1 (Random Numbers, Arrays and Matrices)
- Fast I/O for Competitive Programming
- Setting up Sublime Text for C++ Competitive Programming Environment
- How to setup Competitive Programming in Visual Studio Code for C++
- Which C++ libraries are useful for competitive programming?
- Common mistakes to be avoided in Competitive Programming in C++ | Beginners
- C++ Interview Questions and Answers (2024)
- Top C++ STL Interview Questions and Answers
- 30 OOPs Interview Questions and Answers (2024)
- Top C++ Exception Handling Interview Questions and Answers
- C++ Programming Examples
The capability of a class to derive properties and characteristics from another class is called Inheritance . Inheritance is one of the most important features of Object-Oriented Programming.
Inheritance is a feature or a process in which, new classes are created from the existing classes. The new class created is called “derived class” or “child class” and the existing class is known as the “base class” or “parent class”. The derived class now is said to be inherited from the base class.
When we say derived class inherits the base class, it means, the derived class inherits all the properties of the base class, without changing the properties of base class and may add new features to its own. These new features in the derived class will not affect the base class. The derived class is the specialized class for the base class.
- Sub Class: The class that inherits properties from another class is called Subclass or Derived Class.
- Super Class: The class whose properties are inherited by a subclass is called Base Class or Superclass.
The article is divided into the following subtopics:
Why and when to use inheritance?
- Modes of Inheritance
- Types of Inheritance
Consider a group of vehicles. You need to create classes for Bus, Car, and Truck. The methods fuelAmount(), capacity(), applyBrakes() will be the same for all three classes. If we create these classes avoiding inheritance then we have to write all of these functions in each of the three classes as shown below figure:
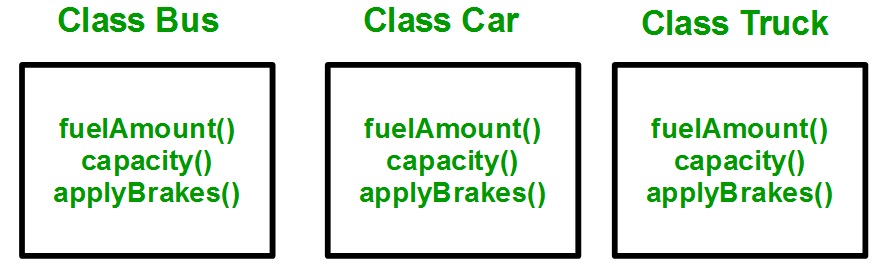
You can clearly see that the above process results in duplication of the same code 3 times. This increases the chances of error and data redundancy. To avoid this type of situation, inheritance is used. If we create a class Vehicle and write these three functions in it and inherit the rest of the classes from the vehicle class, then we can simply avoid the duplication of data and increase re-usability. Look at the below diagram in which the three classes are inherited from vehicle class:
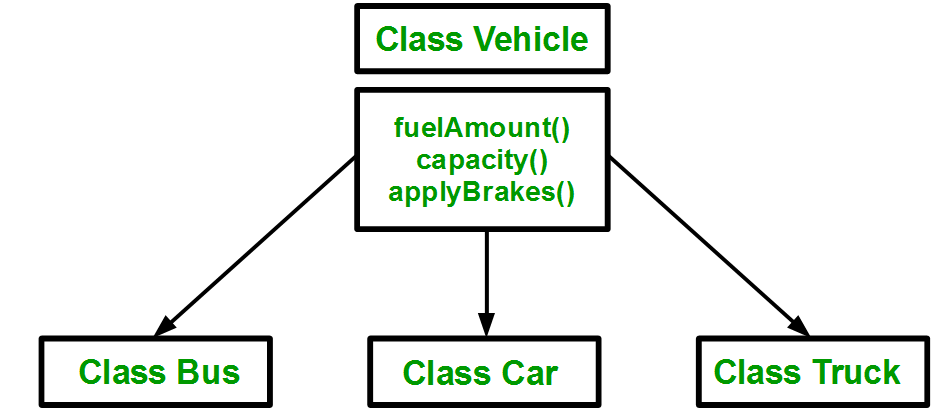
Using inheritance, we have to write the functions only one time instead of three times as we have inherited the rest of the three classes from the base class (Vehicle). Implementing inheritance in C++ : For creating a sub-class that is inherited from the base class we have to follow the below syntax.
Derived Classes: A Derived class is defined as the class derived from the base class. Syntax :
Where class — keyword to create a new class derived_class_name — name of the new class, which will inherit the base class access-specifier — either of private, public or protected. If neither is specified, PRIVATE is taken as default base-class-name — name of the base class Note : A derived class doesn’t inherit access to private data members. However, it does inherit a full parent object, which contains any private members which that class declares.
Example: 1. class ABC : private XYZ //private derivation { } 2. class ABC : public XYZ //public derivation { } 3. class ABC : protected XYZ //protected derivation { } 4. class ABC: XYZ //private derivation by default { }
o When a base class is privately inherited by the derived class, public members of the base class becomes the private members of the derived class and therefore, the public members of the base class can only be accessed by the member functions of the derived class. They are inaccessible to the objects of the derived class. o On the other hand, when the base class is publicly inherited by the derived class, public members of the base class also become the public members of the derived class. Therefore, the public members of the base class are accessible by the objects of the derived class as well as by the member functions of the derived class.
In the above program, the ‘Child’ class is publicly inherited from the ‘Parent’ class so the public data members of the class ‘Parent’ will also be inherited by the class ‘Child’. Modes of Inheritance: There are 3 modes of inheritance.
- Public Mode : If we derive a subclass from a public base class. Then the public member of the base class will become public in the derived class and protected members of the base class will become protected in the derived class.
- Protected Mode : If we derive a subclass from a Protected base class. Then both public members and protected members of the base class will become protected in the derived class.
- Private Mode : If we derive a subclass from a Private base class. Then both public members and protected members of the base class will become Private in the derived class.
Note: The private members in the base class cannot be directly accessed in the derived class, while protected members can be directly accessed. For example, Classes B, C, and D all contain the variables x, y, and z in the below example. It is just a question of access.
The below table summarizes the above three modes and shows the access specifier of the members of the base class in the subclass when derived in public, protected and private modes:
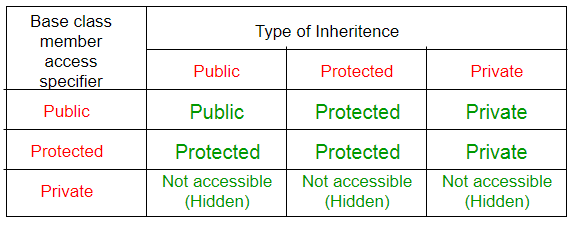
Types Of Inheritance:-
- Single inheritance
- Multilevel inheritance
- Multiple inheritance
- Hierarchical inheritance
- Hybrid inheritance
Types of Inheritance in C++
1. Single Inheritance : In single inheritance, a class is allowed to inherit from only one class. i.e. one subclass is inherited by one base class only.
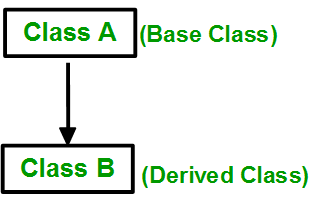
Output:- Enter the Value of A= 3 3 Enter the Value of B= 5 5 Product of 3 * 5 = 15
2. Multiple Inheritance: Multiple Inheritance is a feature of C++ where a class can inherit from more than one class. i.e one subclass is inherited from more than one base class .
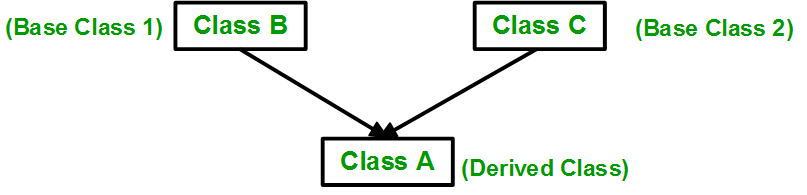
Here, the number of base classes will be separated by a comma (‘, ‘) and the access mode for every base class must be specified.
To know more about it, please refer to the article Multiple Inheritances .
3. Multilevel Inheritance : In this type of inheritance, a derived class is created from another derived class.
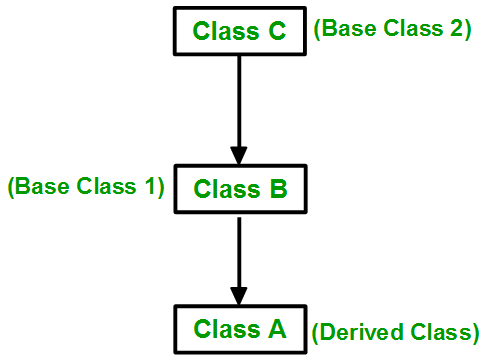
4. Hierarchical Inheritance : In this type of inheritance, more than one subclass is inherited from a single base class. i.e. more than one derived class is created from a single base class.
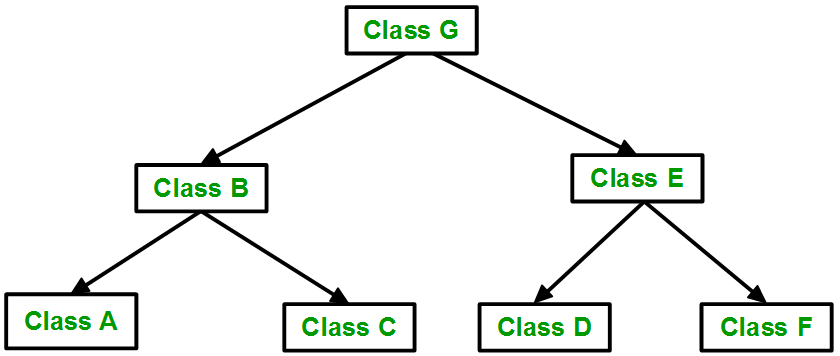
5. Hybrid (Virtual) Inheritance : Hybrid Inheritance is implemented by combining more than one type of inheritance. For example: Combining Hierarchical inheritance and Multiple Inheritance. Below image shows the combination of hierarchical and multiple inheritances:
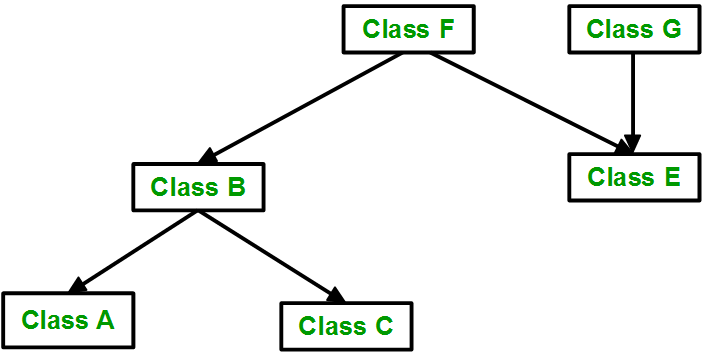
6. A special case of hybrid inheritance: Multipath inheritance : A derived class with two base classes and these two base classes have one common base class is called multipath inheritance. Ambiguity can arise in this type of inheritance. Example:
In the above example, both ClassB and ClassC inherit ClassA, they both have a single copy of ClassA. However Class-D inherits both ClassB and ClassC, therefore Class-D has two copies of ClassA, one from ClassB and another from ClassC. If we need to access the data member of ClassA through the object of Class-D, we must specify the path from which a will be accessed, whether it is from ClassB or ClassC, bcoz compiler can’t differentiate between two copies of ClassA in Class-D.
There are 2 Ways to Avoid this Ambiguity:
1) Avoiding ambiguity using the scope resolution operator: Using the scope resolution operator we can manually specify the path from which data member a will be accessed, as shown in statements 3 and 4, in the above example.
Note: Still, there are two copies of ClassA in Class-D. 2) Avoiding ambiguity using the virtual base class:
According to the above example, Class-D has only one copy of ClassA, therefore, statement 4 will overwrite the value of a, given in statement 3.
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?
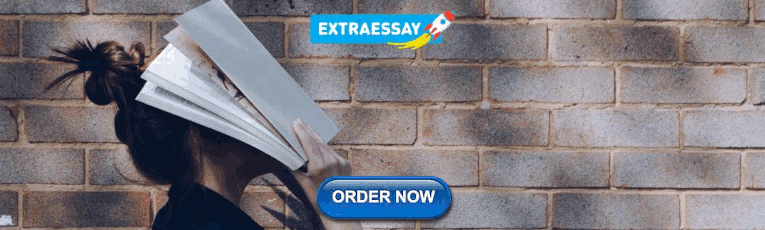
COMMENTS
I implement the operator= functionality assigning/constructing color in the Base class operator if you want to call the Base operator= from the Derived class use: Base::operator=(rhs) in the Derived class operator=() implementation. The signature you've proposed for Derived operator= isn't valid C++ as far as I know.
The assignment operator,"=", is the operator used for Assignment. It copies the right value into the left value. Assignment Operators are predefined to operate only on built-in Data types. Assignment operator overloading is binary operator overloading. Overloading assignment operator in C++ copies all values of one object to another object.
The implicit copy assignment operator. Unlike other operators, the compiler will provide an implicit public copy assignment operator for your class if you do not provide a user-defined one. This assignment operator does memberwise assignment (which is essentially the same as the memberwise initialization that default copy constructors do).
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
C++ Operator Overloading Guidelines. One of the nice features of C++ is that you can give special meanings to operators, when they are used with user-defined classes. This is called operator overloading. You can implement C++ operator overloads by providing special member-functions on your classes that follow a particular naming convention.
If self-assignment can be handled without any extra code, don't add any extra code. But do add a comment so others will know that your assignment operator gracefully handles self-assignment: Example 1a: Fred& Fred::operator= (const Fred& f) {. // This gracefully handles self assignment. *p_ = *f.p_; return *this;
Some operators must be overloaded as member functions of the class. Let's discover the intriguing world of operator overloading in C++. We explore how certain operators, like the assignment, subscript, and function call operators, must be overloaded as member functions, making them integral to class objects. We'll also discuss the flexibility of the function call operator and its varied uses.
In the C++ programming language, the assignment operator, =, is the operator used for assignment.Like most other operators in C++, it can be overloaded.. The copy assignment operator, often just called the "assignment operator", is a special case of assignment operator where the source (right-hand side) and destination (left-hand side) are of the same class type.
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way. Syntax. variable += value; This above expression is equivalent to the expression: variable = variable + value; Example.
The copy assignment operator of the derived class that is implicitly declared by the compiler hides assignment operators of the base class. Use using declaration in the derived class the following way. using A::operator =; B():A(){}; virtual ~B(){}; virtual void doneit(){myWrite();} Another approach is to redeclare the virtual assignment ...
The solution to this is to define an overloaded assignment operator i.e., copy-assignment operator. The next code snippet implements the version of the Person class that can copy assign the two objects of the same class correctly. Notice, though, the if statement in the copy-assignment function guarantees that the operator works correctly even ...
In C++, like other functions, assignment operator function is inherited in derived class. For example, in the following program, base class assignment operator function can be accessed using the derived class object. Output: base class assignment operator called. Summer-time is here and so is the time to skill-up!
Triviality of eligible copy assignment operators determines whether the class is a trivially copyable type. [] NoteIf both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either a prvalue such as a nameless temporary or an xvalue such as the result of std::move), and selects the copy assignment if the argument is an ...
Virtual assignment. It is possible to make the assignment operator virtual. However, unlike the destructor case where virtualization is always a good idea, virtualizing the assignment operator really opens up a bag full of worms and gets into some advanced topics outside of the scope of this tutorial.
Every class declares an operator=.If you don't do it explicitly, the operator is declared implicitly. That (possibly implicit) declaration hides the base member. To unhide it, you need to use a using declaration:. template <typename T> struct Child : Base<T> { using Base<T>::operator=; };
Classes and Objects are part of object-oriented methods and typically provide features such as properties and methods. One of the great features of an object orientated language like C++ is a copy assignment operator that is used with operator= to create a new object from an existing one. In this post, we explain what a copy assignment operator is and its types in usage with some C++ examples.
because this requires the class Child2to be completely defined at the point of the operators definition and the same for the operator defined in Child 2. I tried defining the operator as . Child1 operator=(const Parent & rhs); And that seemed to work in most of the cases, but failed in case of the following:
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Using-declaration introduces a member of a base class into the derived class definition, such as to expose a protected member of base as public member of derived. In this case, nested-name-specifier must name a base class of the one being defined. If the name is the name of an overloaded member function of the base class, all base class member ...
Inheritance is a feature or a process in which, new classes are created from the existing classes. The new class created is called "derived class" or "child class" and the existing class is known as the "base class" or "parent class". The derived class now is said to be inherited from the base class. When we say derived class ...
You just need to call the base class move assignment operator: vertex_shader& operator=(vertex_shader&& rhs) { shader::operator=(std::move(rhs)); return *this; } Share. Improve this answer ... Using move semantics to push a base class instance into a child class instance. 0.