A Complete React Boilerplate Tutorial — From Zero to Hero

By Leonardo Maldonado
When we’re starting learn React, to make our projects we need to make a boilerplate from scratch or use some provided by the community. Almost all the times it’s the create-react-app that we use to create an app with no build configuration. Or we just make our own simple boilerplate from scratch.
From this, it comes to mind: why not make a boilerplate with all dependencies that I always use and leave it ready? The community also thought that way, so now we have several community-created boilerplates. Some are more complex than others, but they always have the same goal of saving the maximum amount of time.
This article will teach you how you can build your own boilerplate from scratch with the main dependencies used in the React community today. We’re gonna use some of the moderns features most common these days and from there you can customize it any way you want.
The boilerplate created by this article will be available here!
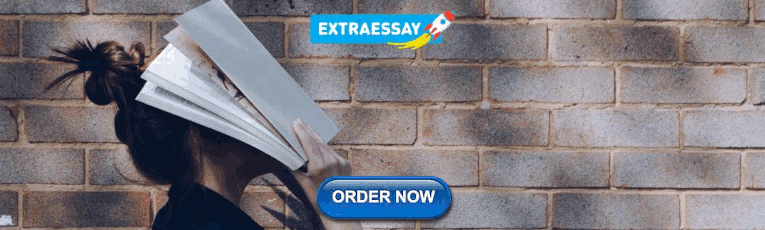
Getting started
First of all, we’re going to create a folder to start our boilerplate. You can name it whatever you want, I’m gonna name mine react-bolt .
Open your terminal, and create it like this:
Now, go to your created folder, and type the following command:
NPM will create a package.json file for you, and all the dependencies that you installed and your commands will be there.
Now, we’re going to create the basic folder structure for our boilerplate. It’s gonna be like this for now:
Webpack is the most famous module-bundler for JavaScript applications nowadays. Basically, it bundles all your code and generates one or more bundles. You can learn more about it here .
In this boilerplate we’re going to use it, so install all these dependencies:
Now in our config folder, we’re going to create another folder called webpack , then inside that webpack folder create 5 files.
Create a file called paths.js . Inside that file is going to be the target directory for all your output files.
Inside it, put all this code:
Now, create another file called rules.js , and put the following code there:
After that, we’re going to create 3 more files:
webpack.common.babel.js
webpack.dev.babel.js
webpack.prod.babel.js
Basically, in our webpack.common.babel.js file, we’ve set up our entry and output configuration and included as well any plugins that are required. In the webpack.dev.babel.js file, we’ve set the mode to development. And in our webpack.prod.babel.js file, we’ve set the mode to production.
After that, in our root folder, we’re going to create the last webpack file called webpack.config.js and put in the following code:
Our webpack config is ready, so now we’re going to work on other parts of the boilerplate with Babel, ESLint, Prettier, etc.
I think that almost everyone who works with React has probably heard about Babel and how this simple transpiler helps our lives. If you don’t know what it is, Babel it’s basically a transpiler that converts your JavaScript code into plain old ES5 JavaScript that can run in any browser.
We’re going to use a bunch of Babel plugins, so in our root folder, install:
After this, we’re gonna create a file in our root folder called .babelrc and inside that file, we’re going to put the following code:
Now our project is compiled by Babel, and we can use the next-generation JavaScript syntax without any problems.
The most used tool for linting projects nowadays is ESLint. It is really helpful to find certain classes of bugs, such as those related to variable scope, assignment to undeclared variables, and so on.
First, install the following dependencies:
Then, in our root folder, create a file called .eslintrc and put the following code there:
Prettier is basically a code formatter. It parses your code and re-prints it with its own rules that take the maximum line length into account, wrapping code when necessary.
You just need to install it:
And in our root folder, create a file called .prettierrc and put the following code there:
React is an open-source JavaScript application library to build user interfaces. It was developed by Facebook and has a huge community behind it. If you are reading this article, I assume that you already know about React, but if you want to learn more about it, you can read up here .
We’re going to install the following dependencies:
And inside our src folder, we’re going to create a simple HTML file index.html and put in the following code:
After that, we’re going to create a simple React project. Inside our src folder, create a index.js file like this:
Inside our src folder we’re going to have the following structure:
Create a file called App.js inside the components folder, and put in the following code:
Redux makes it easy to manage the state of your application. Another way of looking at this is that it helps you manage the data you display and how you respond to user actions. These days a lot of people prefer other options like MobX or just the setState itself, but I’m gonna stick with Redux for this boilerplate.
First, we’re going to install some dependencies:
Then, we’re going to create our Redux store, and put some state there. In our store folder, create an index.js file and put that following code there:
Now, inside our reducers folder create an index.js and put the following code:
Last, we’re gonna to our index.js in our src folder, and wrap the code with the <Provider /> and pas s our store as props to make it available to our application.
It’s going to be like this:
All done. Our Redux store is configured and ready to go.
React Router
React Router is the standard routing library for React. Basically, it keeps your UI in sync with the URL. We’re gonna use it in our boilerplate, so install it:
After that, go to our index.js in our src folder and wrap all the code there with the <BrowserRoute r>.
Our index.js in our src folder it’s going to end up like this:
Styled Components
Styled Components makes CSS easy for everyone, as it helps you organize your React project. Its objective is to write more small and reusable components. We’re gonna use it, and if you want to learn more about it, read up here .
First, install it:
Then, in our App.js file inside our components folder, we’re going to create a simple title using Styled Components. Our title is going to be like this:
And inside our file, we need to import styled components, so our file is going to end up like this:
Jest & React Testing Library
Jest is an open-source JavaScript testing library from Facebook. It makes it easy to test your application, and gives us a lot of information about what is giving the right output and what’s not. React Testing Library is a very light-weight solution for testing React components. Basically, this library is a replacement for Enzyme.
Every application needs some kind of tests. I’m not gonna write tests in this article but I’m gonna show you how you can configure these tools to start testing your applications.
First, we’re gonna install both:
After that, go to our package.json and put the following after all:
Then, go to our config folder, and inside it created another folder called tests and inside that folder, create 2 files.
First, create a file called jest.config.js and put in the following code:
Then, create a file called rtl.setup.js and put in the following code:
All done. Our boilerplate is ready to go and you can use it now.
Now go to our file package.json and put in the following code:
Now, if you run the command npm start and go to localhost:8080 , we should see our application working fine!
If you want to see my final code, the boilerplate created by this article is available here!
I have some ideas for some features that I’d love to include in the boilerplate, so please feel free to contribute!
? F ollow me on Twitter! ⭐ F ollow me on GitHub!
I’m looking for a remote opportunity, so if have any I’d love to hear about it, so please contact me!
freeCodeCamp Programming Tutorials: Python, JavaScript, Git & More
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started

DEV Community

Posted on May 10, 2020 • Updated on May 14, 2020
React Boilerplate - Part 1
Welcome to week two in this article series, "Streamline Workflow." In this week's article we will explore a Boilerplate configuration for React. The idea to to have a turnkey solution, so you can start developing quickly, instead of wasting time reconfiguring the default Create React App (CRA) generated starter, based on your preferred developing environment.
So there are a few approaches we can take:
Stick with Create React App - You can take this approach and reconfigure for your preferences each time. The other variation of this approach is to maintain a modified copy of CRA locally setup the way you like. This could work but remember you will have to maintain and keep the packages updated.
Create a Custom Boilerplate with Webpack - You can roll you own. I have done this in the past. It exposes the Webpack configuration more than the existing CRA does and allows you to fully customize the environment. If you are interested in this approach Robin Wieruch wrote a wonderful tutorial series on how to accomplish this boilerplate. This was my original approach and you can look at and use the code on my Repository if this is a direction you wish to try. In the end I decided, this was to complex for the average beginning end user to maintain.
Create a Custom Boilerplate w/Webpack - This will be the topic of this two part series on a React Boilerplate.
Well, according to Parcel's website, it is a "blazing fast, zero configuration web bundler." This is mostly accurate and we are going to look at the simplicity of this design. Where the Webpack bundler was complex for the beginner, this is super easy, and still robust.
TLTR: If you want to go straight to the code
I am using yarn for this tutorial, but you can use any package manager you are familiar. First let's get the project setup:
- Create a new project directory: mkdir my-react-boilerplate
- Browse to the directory: cd my-react-boilerplate
- Create a package.json: yarn init -y
- Create a src directory mkdir src
- Create index.js in the src directory: touch src/index.js
Your initial package.json should look similar to this:
You need to change the main to src/index.js
Adding Parcel
To start, let's setup React, Babel, and our package bundler:
In the project root, create a configuration file for Babel called .babelrc with the following content:
Add a few scripts to start and build the project:
I use the src directory for most of my content, so, create index.html in the src directory: touch src/index.html . In the index.html file place the following content:
So far your project structure should look like this:
Setup React
Create index.js: touch src/index.js
Add a <div> tag to the body of the index.html with id=app , and add the index.js file as such:
Parcel will use the id in the root div and the script tag to automatically build a template in the bundle in the created dist folder. Let's add to following to the index.js file:
This is a minimalist approach and will technically work. However, if we are building a boilerplate to streamline our workflow, it is honestly not very practical. Let's refactor our setup.
Refactoring React
Create an App.js in the src folder and add the following content:
Refactor the index.js file:
Okay that is it, you are ready to start the development server: yarn start . You can open your browser at http://localhost:1234
Refactor Parcel
So, in my opinion there are a few changes we can make:
- First, I do not like the starting port
- Also, it would be nice if the browser would automatically open after the development server starts.
You can edit the start up script very easily to accommodate these changes: "start": "parcel src/index.html --port 3000 --open" .
This is a very simple setup, with a package bundler with almost zero configuration. However, the boilerplate has a lot of areas we can fill in.
- More tweaks to Parcel startup scripts
- Setup browserlist
- Configure eslint and prettier
- Configure scripts for linting
- Configure husky to lint the source code as a pre-commit hook
- Setup project styling
Stay tuned.
Top comments (0)
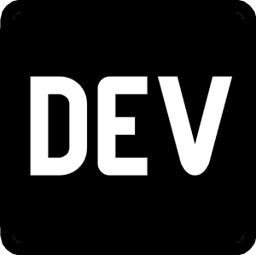
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
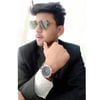
10 Essential VS Code Tips & Tricks for Greater Productivity
Safdar Ali - Aug 25
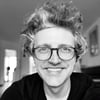
Stay ahead in web development: latest news, tools, and insights #47
Adam - Aug 26
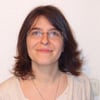
Understanding Kubernetes: part 52 – Kubernetes 1.31 Changelog
Aurélie Vache - Aug 26
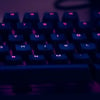
Case Study: The Nine Tails Problem
Paul Ngugi - Aug 9
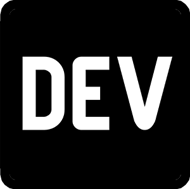
We're a place where coders share, stay up-to-date and grow their careers.

10 React Boilerplates You Should Not Miss in 2022
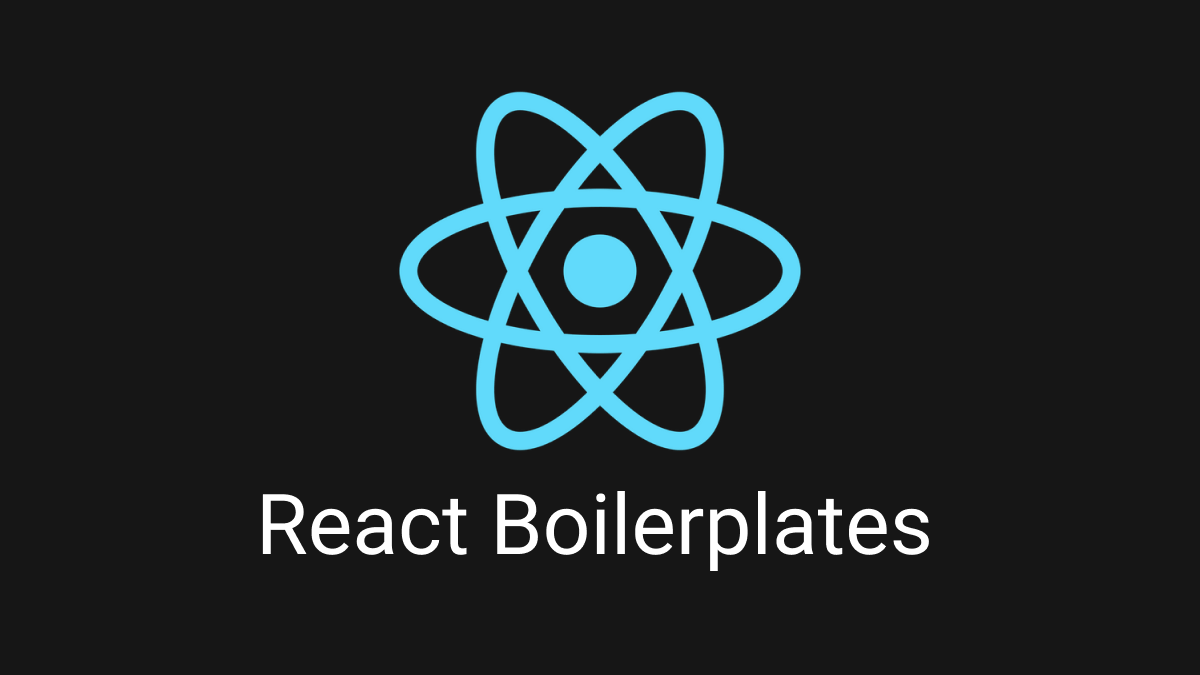
Are you looking for React boilerplates? You’ll find all the great ones here. In this blog, we will learn and find out all the major React boilerplates that you should not miss in 2022. But first, for those who are unfamiliar with boilerplates, let us clarify a few points before diving into the best ones.
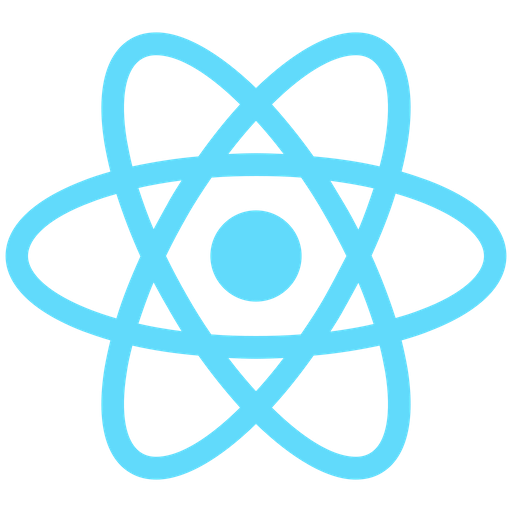
What is a boilerplate?
A boilerplate has nothing to do with a boiler or plate. Rather, it is a chunk of code that we can reuse a lot of times to repeat the process in particular sections. According to Wikipedia, “boilerplate is a section of code that is used interchangeably to provide this or that function”. Since developers go through a lot of code writing, boilerplate code saves their time and gives comfort.
To reap the benefits of a boilerplate, clone it in Git for your project’s needs. After that, use a hot reloading function to refresh code changes.
Difference between boilerplates & Starter kits
These are not well-defined terms. These terms are frequently used interchangeably. However, in our opinion, the Starter Kit is a larger package that is used only once per project, similar to the code generated by create-react-app. Whereas boilerplate is used for smaller tasks such as the files we create for a single redux action or component.
Rect boilerplate vs React boilerplate template
1. Open-Source development tool. | 1. Free / Open-Source / Paid development tool. |
2. Reduces the developing time. | 2. Reduces the developing time. |
3. Reusable parts of code. | 3. Case Frame / Layout |
4. Piece of writing, actual text, and images. | 4. Parts/features like a CSS style sheet, color palette, etc. |
Best React boilerplate?
Because developers’ preferences differ, there is no such thing as the best react boilerplate. You may have them based on their popularity but not any other way. Each boilerplate is useful for resolving various tasks. The topic is very similar to asking anyone which JS library is best for front-end development. React developers would vote for React, and Vue developers would defend Vue. Simple as that.
Evaluati on Points
- Clean code structure
- Code splitting
- Comments presence
- Well documentation
- Responsive navigation system
- Server and client code for setup
- Scalability
- Easy testing tools
- Support & maintaining options
The most important benefits of using boilerplates are time savings and a short learning curve. Simply incorporating a code template rather than writing everything from scratch saves time. Furthermore, thanks to a specific frame scaffolding code, the designer may be able to see the code more clearly in the future.
However, every good thing has a drawback, so be aware that the downside of ready-made coding tools may be additional code maintenance and the need to navigate someone else’s code. Also, boilerplate commands may dump configuration information in the form of code. You’ll have to manually resolve bug lists, clean up a lot of code, and update the dependencies from the original repository.
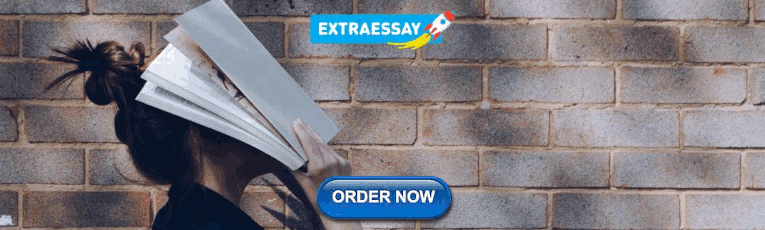
When to use React b oilerplate?
The use of React boilerplate depends on the size of your project. It is best to use boilerplates for light and responsive web apps. For long-term and time-consuming sites, it’s better to put effort into deep learning, DevOps toolchain, etc.
To be honest, there are a couple of hundred React boilerplates available on GitHub. So, for choosing a particular one or a few, you need to have a clear goal. For example, you will build an app using React, TypeScript, Redux, etc.
We’ve carefully handpicked 15 React boilerplates for different purposes. Please keep reading to unveil these great React boilerplates!
10 React Boilerplates
The list is in no particular order.
1. Gatsby Static
Gatsby is a fantastic boilerplate for building static websites or apps in React.js that are optimized for speed. It’s the ideal open-source framework for creating, say, a company’s e-commerce store. It has a starter kit gallery and provides the fastest outputs pre-rendered HTML and CSS to ensure the quickest load time. Learn about Gatsby with the official guide and a gallery of starter kits .
2. React Redux Universal
React Redux Universal is a boilerplate that includes react-router, redux, saga, webpack 3, jest with coverage, and enzyme. Its functionality allows you to perform actions on both the server and client sides. Redux eliminates boilerplate code and enables hot–reloading in the first place. Redux is suitable for multiple UI layers and has a robust ecosystem of addons to choose from.
3. Electron React b oilerplate
To begin with, the Electron boilerplate has multiple entry points and a well-deserved reputation among coders, with 18.7k stars on GitHub. The three pillars of this boilerplate are hot reloading, incremental typing, and high code performance. Second, Electron allows you to switch between apps without having to refresh the page. It is compatible with electron-builder , TypeScript , eslint , webpack , and Babel .
4. React Slingshot
One more efficient tool to equip your React app is react-slingshot. It is a starter kit / boilerplate with Babel, hot reloading, testing, lining, and a working example app built-in. Its advantages are the only npm start command to get started through any modern browser. It works with a bunch of common technologies like SASS, ESLint, React, Redux, React Router , and others. Make the fast and highly automated production build – by typing npm run build. Available for macOS, Linux, and Windows as well. Currently, it has 9.8k GitHub stars.
5. ny times kyt
Every large JavaScript web app requires a common foundation: a configuration to build, run, test, and lint your code. kyt is a toolkit that encapsulates and manages web app configuration. kyt has a wide range of applications in programming and can be used as a dependency in any project. It’s a useful tool for creating dynamic web applications in Node.js.
6. React b oilerplate
With 28.2k stars on GitHub, React boilerplate is a solid and well-thought-out boilerplate in the Javascript UI Libraries. What’s on the inside? Redux, Mocha, Redux-Saga, Jest, React Router, PostCSS, reselect, some code generation scripts, and the super-rich component and font base are all included. They even allow for SEO indexing! That is more than enough to focus on app development and performance.
Razzle boilerplate is a tool that abstracts all of the complex configurations required to build single-page apps and server-side rendering apps into a single dependency. It has the same CSS setup as create-react-app and supports React , Preact , Reason-React , Vue, and Angular. While your app is running, type rs and press enter in the terminal to restart your server. Get started with Razzle right away.
8. Neutrino
Neutrino allows you to create js web apps without the need for an initial configuration file. It is frequently used as a helper in the development of various React and Node.js projects. Neutrino allows you to test, lint, and develop js projects using shared configuration presets and middleware. It is compatible with Jest and Karma for testing, ESLing for fine-tuning linting, various CLI tools, and other tools.
9. React chrome extension boilerplate
With 2k GitHub stars and over 350 forks, this boilerplate is one of the best chrome extension boilerplates for Reactjs projects. You’ll see a live example of this boilerplate from the link below. Webpack and react-transform are used in this boilerplate, as well as Redux. You can perform a hot reload by editing the Popup & Window & Inject page’s related files.
10. Webpack React boilerplate
webpack-react-boilerplate is a React 16 and Webpack 4 boilerplate that uses babel 7. It utilizes the new webpack-dev-server, the react-hot-loader, and CSS-Modules. It is using the most recent versions of React, babel, jest, and enzyme. Click to see real-time server changes and also for more information on the technologies used.
Final Thoughts
Finally, it looks like your search for some good React boilerplates has come to an end. Choosing the right React boilerplate is very important. It is essential to choose the right one especially for scaling, maintaining the app or website in the future, setting up the configurations, and so on.
We wish you the best of luck with your next React-based project. Thank you for joining us!
You Might Also Like These Articles
- Convert TypeScript Project to JavaScript
- Best Open Source React Chart Libraries
- Free Best React Date Picker Components to use
- TypeScript & JavaScript – Which One to Choose
- Best Free Tailwind CSS UI Kits and Components
- Web3 – The Decentralized Web of Future
- Why & How to Get Started with Open-Source Software?
Published in React
- benefits of boilerplates
- Best React Boilerplates
- erence between boilerplates & Starter kits
- razzle boilerplate
- React chrome extension boilerplate
- React Redux Boilerplate
- React slingshot
- Top React Boilerplates
- Webpack React boilerplate
- what is boilerplate
- When to use React boilerplate?
Comments are closed, but trackbacks and pingbacks are open.
Get the Reddit app
A community for discussing anything related to the React UI framework and its ecosystem. Join the Reactiflux Discord (reactiflux.com) for additional React discussion and help.
I made a Free and Open Source Boilerplate written in React and Next.JS, it include everything you need to start a new project in JavaScript: Auth, DB & ORM, Forms, I18n, SEO, CI, Testing (Unit, Integration, E2E and Visual), Monitoring and more
Introducing my go-to boilerplate that has become the backbone of all my React projects over the past 3 years. Creating new projects has always been a tedious process for me, requiring substantial time and effort to set up everything from scratch. That's why I've built my free and open source Next.js Boilerplate: https://github.com/ixartz/Next-js-Boilerplate
I've distilled my experience into a comprehensive boilerplate, streamlining the initial setup for any new project. I understand the time consumption involved in starting afresh, which is precisely why I decided to create a versatile boilerplate featuring all the essential elements for a project.
Check out the Next.js Boilerplate on GitHub: https://github.com/ixartz/Next-js-Boilerplate
This project is more than just a personal toolkit; it's my way of giving back to the community by making it entirely open source. Anyone can effortlessly clone the repository and enjoy the seamless integration of essential features. Furthermore, it's not a rigid structure – feel free to customize and tailor it to your project's specific needs by adding or removing features.
In just 10 minutes, you can empower your project with:
🔐 Authentication
💽 Database & ORM
🧪 Testing: unit, integration, E2E, and Visual
✅ Monitoring
You just need to clone the project and you are ready to go. You don't need to lose your time to set up yourself.
I'm genuinely open to feedback and suggestions, so please feel free to share your thoughts. Let's build something great together!
By continuing, you agree to our User Agreement and acknowledge that you understand the Privacy Policy .
Enter the 6-digit code from your authenticator app
You’ve set up two-factor authentication for this account.
Enter a 6-digit backup code
Create your username and password.
Reddit is anonymous, so your username is what you’ll go by here. Choose wisely—because once you get a name, you can’t change it.
Reset your password
Enter your email address or username and we’ll send you a link to reset your password
Check your inbox
An email with a link to reset your password was sent to the email address associated with your account
Choose a Reddit account to continue
Instantly share code, notes, and snippets.
ELI7VH / react-context-boilerplate.tsx
- Download ZIP
- Star ( 15 ) 15 You must be signed in to star a gist
- Fork ( 3 ) 3 You must be signed in to fork a gist
- Embed Embed this gist in your website.
- Share Copy sharable link for this gist.
- Clone via HTTPS Clone using the web URL.
- Learn more about clone URLs
- Save ELI7VH/8ca0e90e52e902e91c3776ad7b63ad8e to your computer and use it in GitHub Desktop.
import React, { createContext, useContext, useState, useEffect } from "react" | |
type Props = { | |
children: React.ReactNode | |
} | |
type Context = { | |
count: number | |
increment: () => void | |
} | |
// Just find-replace "XContext" with whatever context name you like. (ie. DankContext) | |
const XContext = createContext<Context | null>(null) | |
export const XContextProvider = ({ children }: Props) => { | |
const [count, setCount] = useState(0) | |
useEffect(() => { | |
setCount(42) | |
}, []) | |
const increment = () => { | |
setCount(count + 1) | |
} | |
return ( | |
<XContext.Provider value={{ count, increment }}> | |
{children} | |
</XContext.Provider> | |
) | |
} | |
export const useXContext = () => { | |
const context = useContext(XContext) | |
if (!context) | |
throw new Error("XContext must be called from within the XContextProvider") | |
return context | |
} |
maksnester commented Nov 17, 2021
Exactly what I was looking for, thanks
Sorry, something went wrong.
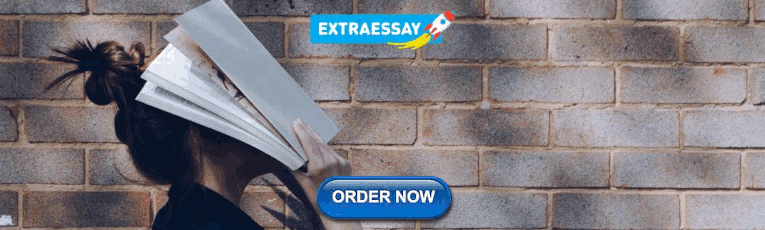
IMAGES
VIDEO
COMMENTS
Builds the app for production to the buildfolder. It correctly bundles React in production mode and optimizes the build for the best performance. The build is minified and the filenames include the hashes. Your app is ready to be deployed! See the section about deploymentfor more information.
2. React with Redux Boilerplate. Description: Integrates Redux for advanced state management, beneficial for large-scale applications where state management becomes complex. Use Case: Complex state management in large-scale applications. Features: Redux, React-Redux, Redux-Thunk. GitHub Repo: React Redux Boilerplate. 3.
Getting started. First of all, we're going to create a folder to start our boilerplate. You can name it whatever you want, I'm gonna name mine react-bolt. Open your terminal, and create it like this: mkdir react-bolt. Now, go to your created folder, and type the following command: npm init -y.
Getting started. First of all, we're going to create a folder to start our boilerplate. You can name it whatever you want, I'm gonna name mine react-bolt. Open your terminal, and create it like this: mkdir react-bolt. Now, go to your created folder, and type the following command: npm init -y.
Install Chrome Redux Dev Tools to see how your application's state changes and travel in time to debug. Type any github username below and see it in action with Redux Dev Tools. Github Username. react-boilerplate. 29447. react-boilerplate-cra-template. 1871.
I am using yarn for this tutorial, but you can use any package manager you are familiar. First let's get the project setup: Create a new project directory: mkdir my-react-boilerplate. Browse to the directory: cd my-react-boilerplate. Create a package.json: yarn init -y. Create a src directory mkdir src.
All done. Our Redux store is configured and ready to go. React Router. React Router is the standard routing library for React. Basically, it keeps your UI in sync with the URL. We're gonna use ...
The Hitchhiker's Guide to react-boilerplate: An introduction for newcomers to this boilerplate. Overview : A short overview of the included tools Commands : Getting the most out of this boilerplate
10 React Boilerplates. The list is in no particular order. 1. Gatsby Static. Gatsby is a fantastic boilerplate for building static websites or apps in React.js that are optimized for speed. It's the ideal open-source framework for creating, say, a company's e-commerce store.
react-boilerplate: A slightly opinionated yet dead simple boilerplate for ReactJS. react-boilerplate. Router Utilizes react-router v5 for client-side routing. Increment; Decrement; Reset; Connected to the Redux store at the / route counter: 0. Increment counter. State Management
Last week, my React Boilerplate project has reached 1000+ stars on GitHub. The project has gained more than around 200 ⭐ in 1-2 days after being promoted by GitHub in the Trending Section. Here is the GitHub repo: React Boilerplate GitHub repo. You can find the React Boilerplate Live demo. Built with latest technology: - React - Next JS
The one true react boilerplate. GitHub Gist: instantly share code, notes, and snippets.
react-boilerplate react-boilerplate Public template 🔥 A highly scalable, offline-first foundation with the best developer experience and a focus on performance and best practices. JavaScript 29.4k 6.1k
I've distilled my experience into a comprehensive boilerplate, streamlining the initial setup for any new project. I understand the time consumption involved in starting afresh, which is precisely why I decided to create a versatile boilerplate featuring all the essential elements for a project.
The Rules. The assignment is to test your skill level to what you claim to be so even if you can't complete the assignment completely, it is still fine to submit a half baked version till the deadline so we can at least analyse the code quality. The deadline is 4 days after the initial commit. Your initial commit is just going to be a simple ...
This command will remove the single build dependency from your project. Instead, it will copy all the configuration files and the transitive dependencies (webpack, Babel, ESLint, etc) right into your project so you have full control over them. All of the commands except eject will still work, but they will point to the copied scripts so you can ...
Contribute to IndSaroj/React-Assignment-1-Boilerplate-new development by creating an account on GitHub.
The boilerplate includes a collection of mixins for common styles, such as flex centering, grid layouts, box shadows, border-radius, z-index layers, and media queries. Form Handling Forms are managed using react-hook-form for simple and performant form management, combined with zod for schema-based validation.
Over this time, the boilerplate has received 1.9k stars on GitHub and has gained significant popularity beyond our company. Now, we've decided to take it a step further and created the Extensive ...
Navigation Menu Toggle navigation. Sign in Product
react-context-boilerplate.tsx This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
This template provides a minimal setup to get React working in Vite with HMR and some ESLint rules. Currently, two official plugins are available: @vitejs/plugin-react uses Babel for Fast Refresh; @vitejs/plugin-react-swc uses SWC for Fast Refresh
Contribute to amsurio/laravel-inertia-react-boilerplate development by creating an account on GitHub.