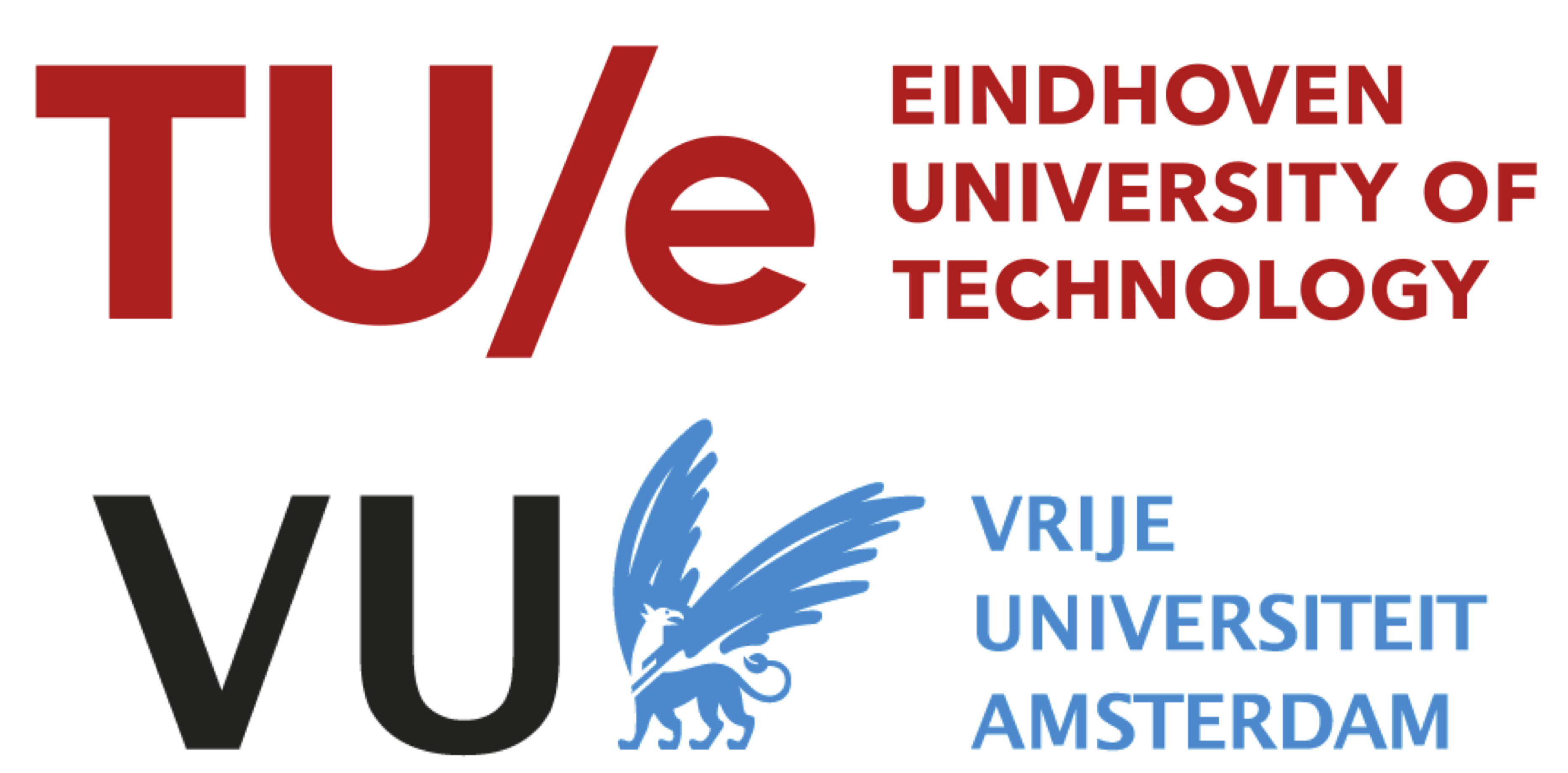
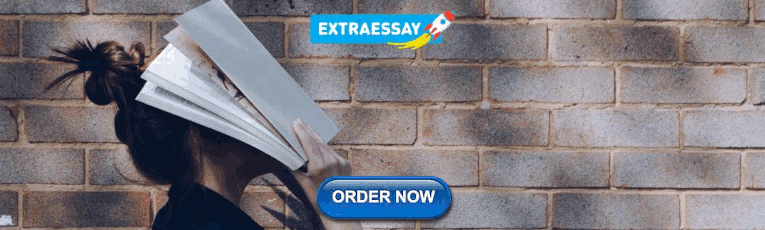
Learning Python by doing
- suggest edit
Computational Thinking
Computational thinking 1 #.
Finding the one definition of Computational Thinking (CT) is a task doomed to failure, mainly because it is hard to have a consensus on what CT means to everyone. To ease the communication and have a shared meaning, we consider CT as an approach to “solve problems using computers as tools” (Beecher, 2017). Notice that CT is not exclusively considered in computer science. On the contrary, CT impacts a wide range of subject areas such as mathematics, engineering, and science in general.
There is a misunderstanding regarding the relationship between CT, computer science, and programming. Some people use them interchangeably, but the three terms are not equivalent. Computer science aims at building and understanding the principles of mathematical computation; while programming –which happens to be a subfield of computer science–focuses on high-quality program writing. Although the three terms are related and might intersect in many points, CT should be seen as a method of problem-solving that is frequently (but not exclusively) used in programming. In essence, problem-solving is a creative process.
Some of the core concepts behind CT are:
Decomposition
Abstraction
Generalization
Hereafter, we will explore what these concepts mean and how they can help you when solving a problem.
Computational thinking
Computational thinking is an approach to problem-solving that involves using a set of practices and principles from computer science to formulate a solution that’s executable by a computer. [ Bee07 ]
Logic is the study of the formal principles of reasoning. These principles determine what can be considered a correct or incorrect argument. An argument is “a change of reasoning that ends up in a conclusion” [ Bee07 ] . Every statement within an argument is called a proposition , and it has a truth value . That is, it is either true or false . Thus, certain expressions like questions (e.g. “are you hungry?”) or commands (e.g. “clean your bedroom!”) cannot be propositions given that they do not have a truth value.
Below we present a very famous argument in the logic field:
Socrates is a man.
All men are mortal.
Therefore, Socrates is mortal.
This argument is composed of three propositions (each one is enumerated). These statements are propositions because all of them have a truth value. However, you can notice that some of these statements are used to arrive at a conclusion. The propositions that form the basis of an argument are known as premises . In our example, propositions 1 and 2 are premises. Lastly, the proposition that is derived from a set of premises is known as a conclusion .
Logic studies the formal principles of reasoning, which determine if an argument is correct or not. An argument is a sequence of premises. Each premise is a statement that has a truth value (i.e. true or false).
Types of Arguments #
Arguments can either be deductive or inductive.
Deductive Arguments #
Deductive arguments are considered a strong form of reasoning because the conclusion is derived from previous premises. However, deductive arguments can fail in two different scenarios:
False premises: One of the premises turns to be false. For example:
I eat healthy food.
Every person eating healthy food is tall.
I am tall. In this example, premise 2 is false. It is not true that every person eating healthy food is tall. Then, this argument fails.
Faulty logic: The conclusion is wrongly derived out from the premises. For example:
All bitterballen are round.
The moon is round.
The moon is a bitterbal. In this case, the conclusion presented in premise 3 is false. It is true that bitterballen are round and that the moon is round, but this does not imply that the moon is a bitterbal! By the way, a “bitterbal” is a typical Dutch snack, to be eaten with a good glass of beer!
Inductive Arguments #
Although deductive arguments are strong, they follow very stern standards that make them difficult to build. Additionally, the real world usually cannot be seen just in black and white–there are many shades in between. This is where inductive arguments play an important role. Inductive arguments do not have an unquestionable truth value. Instead, they deal with probabilities to express the level of confidence in them. Thus, the conclusion of the argument cannot be guaranteed to be true, but you can have a certain confidence in it. An example of an inductive argument comes as follows:
Every time I go out home it is raining.
Tomorrow I will go out from home.
Tomorrow it will be raining.
There is no way you can be certain that tomorrow will rain, but given the evidence, there is a high chance that it will be the case. (The chance might be even higher if we mention that we are living in the Netherlands!)
Deductive and inductive arguments
Arguments can be either deductive or inductive. On the one hand, deductive arguments have premises that have a clear truth value. On the other hand, inductive arguments have premises associated with a level of confidence in them.
Boolean Logic #
Given their binary nature, computers are well suited to deal with deductive reasoning (rather than inductive). To allow them to reason about the correctness of an argument, we use Boolean logic. Boolean logic is a form of logic that deals with deductive arguments–that is, arguments having propositions with a truth value that is either true or false. Boolean logic or Boolean algebra was introduced by George Boole (that is why it is called Boolean) in his book “The Mathematical Analysis of Logic” in 1847. It deals with binary variables (with true or false values) representing propositions and logical operations on them.
Logical Operators #
The main logical operators used in Boolean logic to connect propositions are:
And operator: It is also known as the conjunction operator. It chains premises in such a way that all of them must be true for the conclusion to be true.
Or operator: It is also known as the disjunction operator. It connects premises in such a way that at least one of them must be true for the conclusion to be true.
Not operator: It is also known as the negation operator. It modifies the value of a proposition by flipping its truth value.
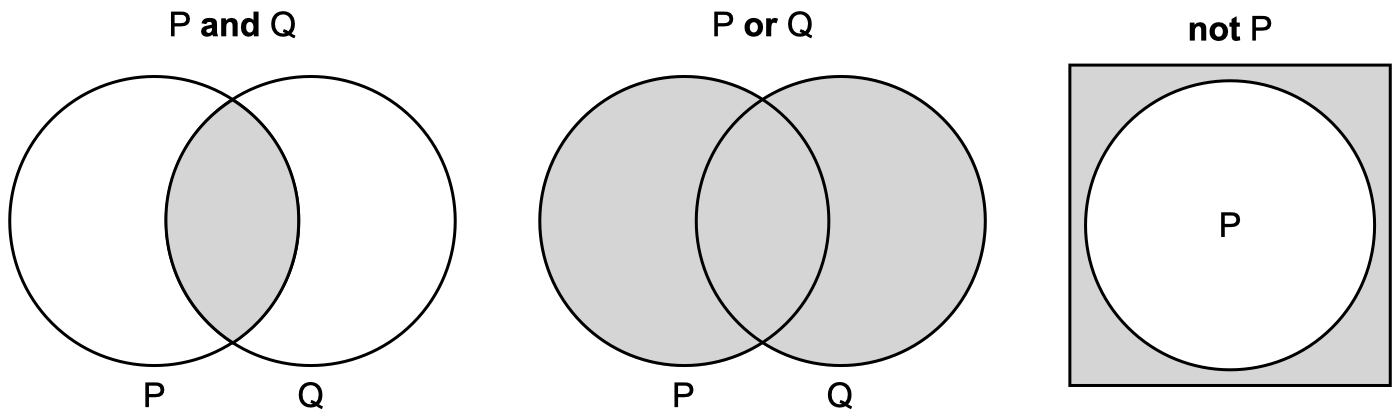
In Boolean logic, propositions are usually represented as letters or variables (see previous figure). For instance, going back to our Socrates argument, we can represent the three propositions as follows:
P: Socrates is a man.
Q: All men are mortal.
R: Therefore, Socrates is mortal.
In order for R to be true, P and Q most be true.
We can now translate this reasoning into Python. To do that use the following table and execute the cell below.
The previous translation to Python code of the deductive argument is correct, however we can do way better! Let us see:
Sometimes conditionals are not really needed; using a Boolean expression as the one shown above is sufficient. Having unneeded language constructs (e.g. conditionals) can negatively impact the readability of your code.
Algorithms #
We can use logic to solve everyday life problems. To do so, we rely on the so-called algorithms. Logic and algorithms are not equivalent. On the contrary, an algorithm uses logic to make decisions for it to solve a well-defined problem. In short, an algorithm is a finite sequence of well-defined instructions that are used to provide a solution to a problem. According to this definition, it seems that algorithms are what we create, refine, and execute every single day of our lives, isn’t it? Think for instance about the algorithm to dress up in the morning or the algorithm (or sequence of steps) that you follow to bake a cake or cook a specific recipe. Those are also algorithms that we have internalized and follow unconsciously.
To design an algorithm, we usually first need to specify the following set of elements:
the problem to be solved or question to be answered. If you do not have a well-defined problem you might end up designing a very efficient but useless algorithm that does not solve the problem at hand. Thus, ensure you understand the problem before thinking about a solution;
the inputs of the algorithm–that is, the data you require to compute the solution;
the output of the algorithm–that is, the expected data that you want to get out from your solution, and;
the algorithm or sequence of steps that you will follow to come up with an answer.
Without having a clear picture of your problem, and the expected inputs and output to solve that problem, it will be unlikely that you will be able to come up with a proper algorithm. Furthermore, beware that for algorithms to be useful, they should terminate at some point (they cannot just run forever without providing a solution!) and output a correct result. An algorithm is correct if it outputs the right solution to every possible input. Proving this might be unfeasible, which is why testing your algorithm (maybe after implementing a program) is crucial.
An algorithm is a finite sequence of clearly defined instructions used to solve a specific problem.
Appeltaart Algorithm #
To be conscious about the process of designing an algorithm, let us create an algorithm to prepare the famous Dutch Appeltaart . The recipe has been taken from the Heel Holland Bakt website .
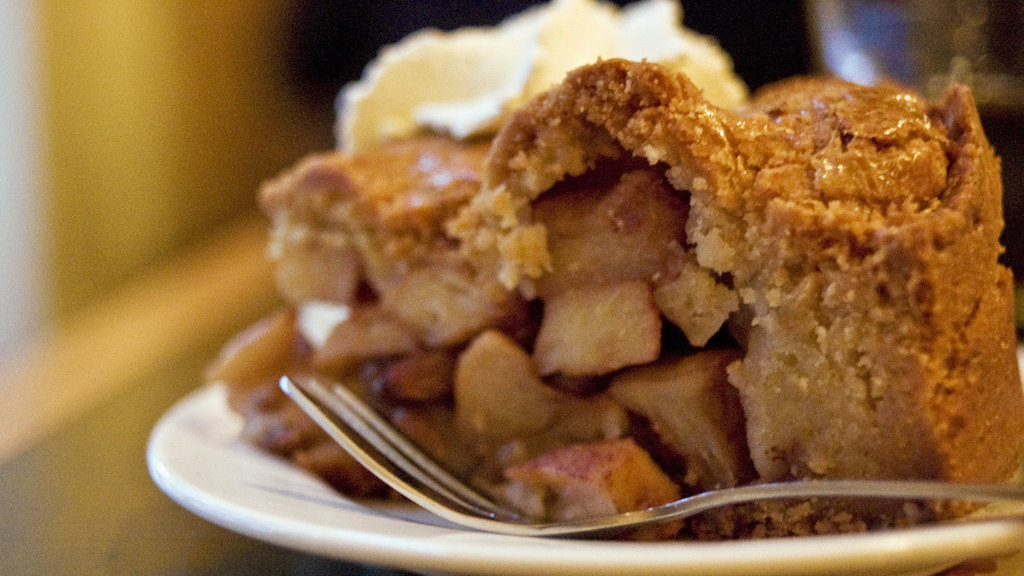
We will start by defining the problem we want to solve. In our case, we want to prepare a classical Dutch Appeltaart .
Inputs (Ingredients) #
The inputs to our algorithm correspond to the ingredients needed to prepare the pie. We list them below:
200 grams of butter
160 grams of brown sugar
0.50 teaspoon of cinnamon
300 grams of white flour
a pinch of salt
breadcrumbs
Apple filling
100 grams of raisins
100 grams of shelled walnuts
5 tablespoons of sugar
3 teaspoons of cinnamon
1 egg for brushing
25 white almonds
Output (Dish) #
The expected output of our algorithm is a delicious and well-prepared Dutch Appeltaart.
Algorithm (Recipe) #
Preparation
Grease a round pan and dust with flour.
Preheat the oven to 180°C.
- Mix the butter with brown sugar and cinnamon.
- Add the flour with a pinch of salt to the butter mixture.
- Add 1 egg to the mixture.
- Knead into a smooth dough and let rest in the fridge for at least 15 minutes.
- Roll out 2/3 of the dough and line the pan with it.
- Spread the breadcrumbs over the bottom.
- Place the pan in the fridge to rest.
- Put the raisins in some rum.
- Peel the apples and cut them into wedges.
- Strain the raisins.
- Mix the apples with raisins, walnuts, sugar, and cinnamon.
- Pour the filling into the pan.
- Cover the top of the pie with the remaining dough.
- Brush the top with a beaten egg and sprinkle with the almonds.
- Bake the apple pie for about 60 minutes.
- Let cool and enjoy!
“The limits of my language mean the limits of my world.” - Ludwig Wittgenstein
In the previous section, we were able to write down the algorithm to prepare a Dutch Appeltaart. Writing down an algorithm is of great importance given that you do not only guarantee that you can execute the algorithm in future opportunities, but you also provide a means to share the algorithm, so other people can benefit from what you designed. But to be able to write down the algorithm, we require an additional tool–that is, a language. In the previous example, we used English to describe our Appeltaart algorithm. However, if you remember correctly, computational thinking relies on computers to solve real-world problems. Thus, the algorithms we want to write down must be written in a language that can be understood by a computer. But beware! The computer is not the only other entity that needs to understand your algorithm: what you write down should be understood by both the machine and other people that might read it afterward (including the you from the future).
Language is a system of communication. It has a syntax and semantics :
The syntax of a language describes the form or structure of the language constructs. The syntax can be defined with a grammar , which is a set of rules that specify how to build a correct sentence in the language.
The semantics describes the meaning of language constructs.
Languages can also be classified as natural or formal :
Natural languages are languages used by people to communicate with each other (e.g. English, Spanish, Dutch, Arabic). They were not designed from the very beginning, on the contrary, they evolved naturally over time.
Formal languages are languages designed by humans for specific domains or purposes. They usually are simplified models of natural language. Examples of formal languages are the mathematical, chemical, or staff (standard music notation) notations. There is also a very important example that interests us the most: programming languages.
Programming Languages #
Programming languages are formal languages with very strict syntax and semantics . These languages are used to write down algorithms. A program is, then, an algorithm implemented using a programming language. To this point, we can make some connections with previous concepts: CT is a method of problem-solving where problems are solved by reusing or designing a new algorithm . This algorithm might later be written down as a program to compute a solution to the original problem.
Python is an example of a programming language. It was conceptualized in 1989 by Guido van Rossum when working at the Centrum Wiskunde & Informatica (CWI), in Amsterdam. Python saw its beginnings in the ABC language. Its name–referring to this monumental sort of snake–actually comes from an old BBC series called Monty Python’s Flying Circus. Guido was reading the scripts of the series and he found out that Python sounded “short, unique, and slightly mysterious”, which is why he decided to adopt the name.
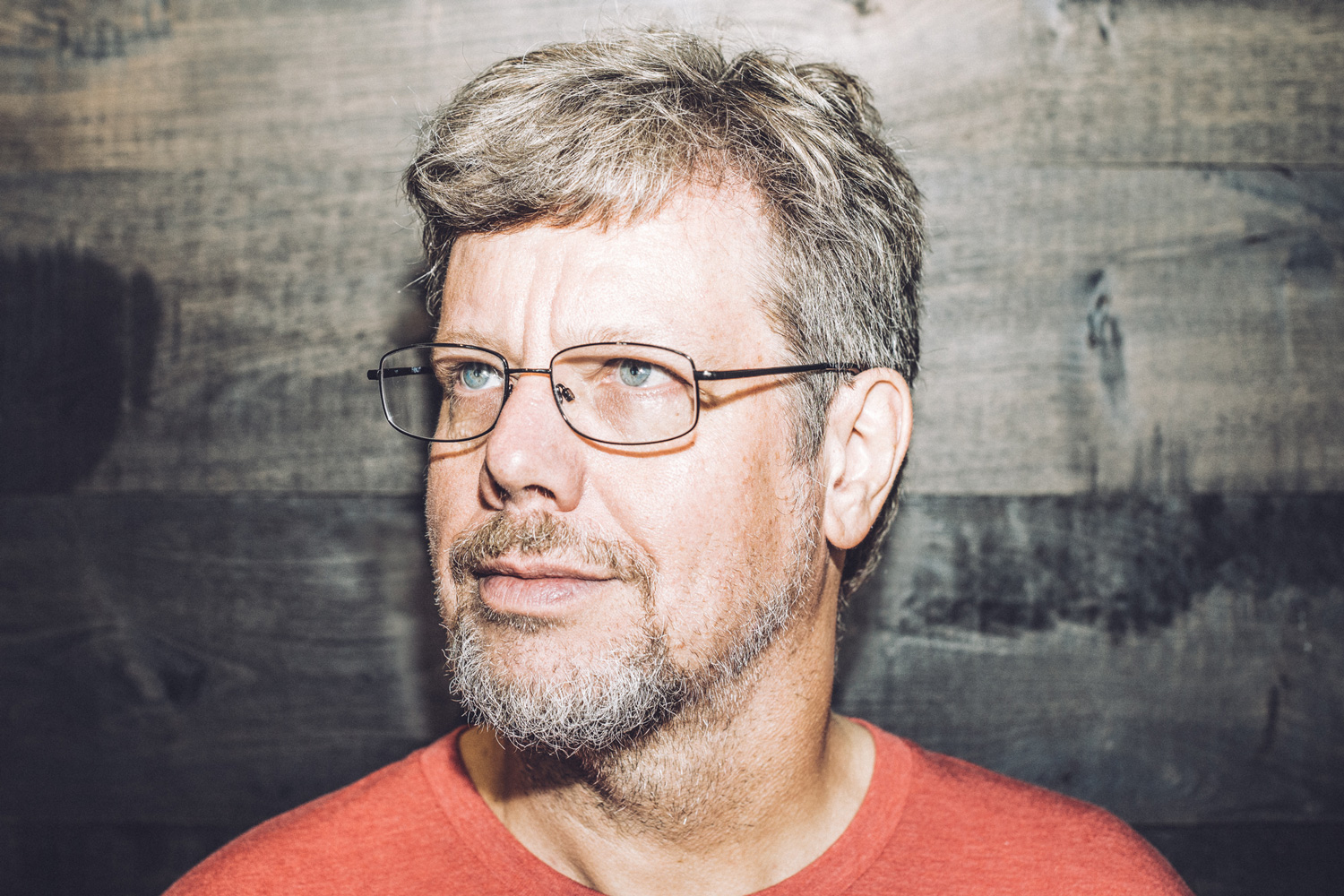
Please clarify that there is a difference between solving a problem, inventing an algorithm, and writing a program.
It maybe the case that to solve a problem you apply a known algorithm, think of sorting words, but it may also be the case that to solve the problem you have to come up with a new algorithm.
The Zen of Python #
In 1999, Tim Peters–one of Python’s main contributors– wrote a collection of 19 principles that influence the design of the Python programming language. This collection is now known as the Zen of Python. Peters mentioned that the 20 \(^{th}\) principle is meant to be filled in by Guido in the years to come. Have a look at the principles. You can consider them as guidelines for the construction of your own programs.
A language is a system of communication, that has syntax and semantics. The syntax describes the form of the language, while the semantics describes its meaning. A language can be either natural (evolves naturally) or formal (designed for a specific domain or purpose). Programming languages are a sort of formal languages and are used to allow communication between humans and computers.
Decomposition #
In 1945 (in the year that the II World War ended), George Pólya, a Hungarian mathematician, wrote a book called “How to Solve It”. The book presents a systematic method of problem-solving that is still relevant today. In particular, he introduced problem-solving techniques known as heuristics . A heuristic is a strategy to solve a specific problem by yielding a good enough solution, but not necessarily an optimal one. There is one important type of heuristic known as decomposition . Decomposition is a divide-and-conquer approach that breaks complex problems into smaller and simpler subproblems, so we can deal with them more efficiently.
In programming, we have diverse constructs that will ease the implementation of decomposition. In particular, classes and functions (or methods ) are a means to divide a complex solution to a complex problem into smaller chunks of code. We will cover these topics later in the course.
Decomposition is a divide-and-conquer approach that breaks complex problems into smaller and simpler subproblems.
Abstraction & Generalization #
Abstraction is the process of hiding irrelevant details in a given context and preserving only the important information. Abstraction is required when generalizing. In fact, generalization is the process of defining general concepts by abstracting common properties from a set of instances. In other words, generalization allows you to formulate a unique concept out of similar but not necessarily identical entities. When generalizing a solution, it becomes simpler because there are fewer concepts to deal with. The solution also becomes more powerful in the sense that it can be applied to more problems of similar nature.
To be able to generalize a solution or algorithm, you need to start developing your ability to recognize patterns. For instance:
A sequence of instructions that are repeating multiple times can be generalized into loops .
Specialized instructions that are employed more than once in different scenarios can be encapsulated in subroutines or functions .
Constraints that determine the flow of execution of your algorithm can be generalized in conditionals .
Abstraction and generalization
Abstraction is the process of preserving relevant information in a given context and hiding irrelevant details. In addition, generalization is the process of defining general concepts by abstracting common properties from a set of instances.
Modelling #
All models are wrong, but some are useful. (Box, 1987)
Humans and computers are not able to completely grasp and understand the real world. Thus, to solve real-world problems, we usually need to create models that help us understand and solve the issue. A model is a representation of an entity. Thus, a model as a representation that, by definition, ignores certain details of the represented entity, is also a type of abstraction . A model in computer science usually consists of:
Entities: core concepts of the represented system.
Relationships: connections among entities within the represented system.
Models usually come in two flavors:
Static models: these models reflect a snapshot of the system at a given moment in time. The state of the system might change in the future but the changes are so small or unfrequent that the model is still useful and sufficient.
Dynamic models: these models explain changes in the state of a system over time. They always involve states (description of the system at a given point in time) and transitions (state changes). Some dynamic models can be executed to predict the state of the system in the future.
When creating a model you should verify that:
The model is useful to solve the problem at hand.
You are strictly following the rules , notation, and conventions to define a model.
The level of accuracy of your model is acceptable to solve the problem (i.e. you keep the strictly needed information).
You have defined the level of precision at which you represent your model (especially when dealing with decimal numbers; how many decimal points are required?).
A model is a representation of an entity. It is a type of abstraction.
Example: Alice’s Adventures in Wonderland #
In this section, we will apply computational thinking to solve a problem from Alice’s Adventures in Wonderland, written by Lewis Caroll. In chapter I, “Down the Rabbit Hole”, Alice found herself falling down into a rabbit hole. She finally reached the ground, coming upon “a heap of sticks and dry leaves”. Alice found herself in a “long, low hall, which was lit up by a row of lamps hanging from the roof”.
“There were doors all around the hall, but they were all locked”. She started “wondering how she was ever to get out again”. She suddenly discovered a tiny golden key on a little three-legged table, all made of solid glass. After looking around, “she came upon a low curtain she had not noticed before, and behind it was a little door about fifteen inches high: she tried the little golden key in the lock, and to her great delight it fitted!” But she was not able to go through it; the doorway was too small.
Later in the chapter, we find out that there was a bottle with a ‘DRINK ME’ label that makes shorter anyone drinking its content. There was also a very small cake with the words ‘EAT ME’ beautifully marked in currant. Anyone eating the cake will grow larger. How can we help Alice go out of the hall through the small doorway behind the curtain?
To solve this problem, assume that:
Alice is 60 inches tall;
Alice can go out of the doorway if she is taller than 10 inches (exclusive) and shorter than 15 inches (inclusive);
one sip of the ‘DRINK ME’ bottle makes anyone get 11 inches shorter;
Alice can only sip from the bottle at a point in time;
one bite of the ‘EAT ME’ cake makes anyone grow 1 inch larger;
Alice can only have a bite of the cake at a point in time, and;
Alice cannot sip from the bottle and a bite of the cake at the same time.
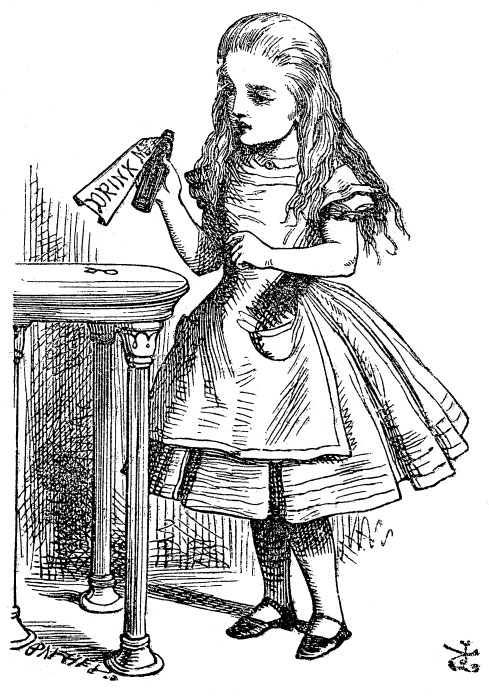
Understanding the Problem #
Problem: Alice wants to go out of the hall. To do so, she needs to go through the doorway behind the curtain, but she is too tall to go through it.
Identifying the Inputs #
The inputs we have at hand are:
Alice’s height (i.e. 60 inches)
Doorway height (i.e. 15 inches)
Height decrease after taking a sip from the ‘DRINK ME’ bottle (i.e. 11 inches)
Height increase after taking a bite from the ‘EAT ME’ cake (i.e. 1 inch)
We abstracted the relevant information to the problem. Details are removed if they are not needed;
thus, the inputs are useful to solve the problem.
The inputs were written in Python. They follow the syntax rules of the programming language.
The identified inputs are enough to accurately solve the problem.
We use integers to represent Alice’s height. There is no need to use floats. Therefore, the level of precision of our model is acceptable to solve the problem.
Identifying the Expected Output #
As a solution to our problem, we want Alice to go out from the hall through the doorway. To do so, her height should be greater than 10 inches and less than or equal to 15 inches.
Output: Alice’s height should be greater than 10 inches and less than or equal to 15 inches.
Designing the Algorithm #
To solve the problem we know that:
Alice must go out from the hall through the doorway if she is larger than 10 inches and shorter or exactly 15 inches.
Alice must drink out from the ‘DRINK ME’ bottle if she is taller than 15 inches–that is, she will shorten 11 inches.
Alice must bite the ‘EAT ME’ cake if she is shorter or exactly 10 inches–that is, she will grow 1 inch.
Steps 2 and 3 shall be repeated until she can to go out from the hall.
We decomposed the problem of getting the right height into two subproblems: making Alice get larger and making Alice get shorter.
We generalized our solution by identifying patterns in our algorithm (e.g. step 4).
Now, we will implement our algorithm in Python.
Let us try to improve our previous algorithm. We can always make it more beautiful , more explicit , simpler , and more readable . Remember that “if the implementation is easy to explain, it may be a good idea” (see the Zen of Python).
Note: In the following code, we will use f-Strings. We will talk more about later in the course, but now you can start grasping what is their use and how we can build them.
Well done! We have applied computational thinking to design a solution to Alice’s problem.
(c) 2021, Lina Ochoa Venegas, Eindhoven University of Technology
This Jupyter Notebook is based on Chapter 1 of [ Bee07 ] and Chapter 1, 8, and 10 of [ Erw17 ] .
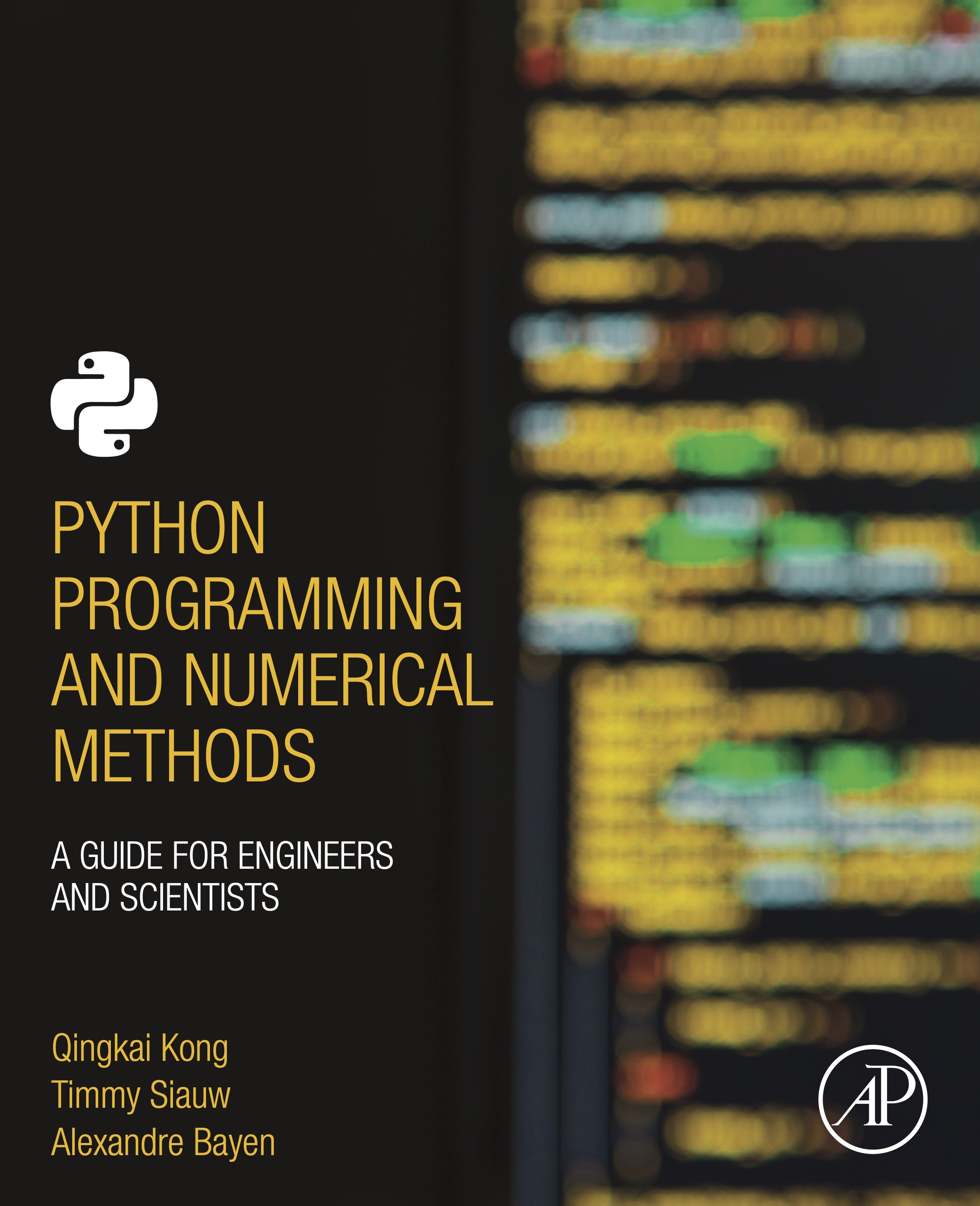
Python Numerical Methods
Python programming and numerical methods: a guide for engineers and scientists ¶.

This notebook contains an excerpt from the Python Programming and Numerical Methods - A Guide for Engineers and Scientists , the content is also available at Berkeley Python Numerical Methods .
The copyright of the book belongs to Elsevier. We also have this interactive book online for a better learning experience. The code is released under the MIT license . If you find this content useful, please consider supporting the work on Elsevier or Amazon !
Table of Contents ¶
Acknowledgment ¶, part i introduction to python programming ¶, chapter 1. python basics ¶.
1.1 Getting Started with Python
1.2 Python as A Calculator
1.3 Managing Packages
1.4 Introduction to Jupyter Notebook
1.5 Logical Expressions and Operators
1.6 Summary and Problems
CHAPTER 2. Variables and Basic Data Structures ¶
2.1 Variables and Assignment
2.2 Data Structure - Strings
2.3 Data Structure - Lists
2.4 Data Structure - Tuples
2.5 Data Structure - Sets
2.6 Data Structure - Dictionaries
2.7 Introducing Numpy Arrays
2.8 Summary and Problems
CHAPTER 3. Functions ¶
3.1 Function Basics
3.2 Local Variables and Global Variables
3.3 Nested Functions
3.4 Lambda Functions
3.5 Functions as Arguments to Functions
3.6 Summary and Problems
CHAPTER 4. Branching Statements ¶
4.1 If-Else Statements
4.2 Ternary Operators
4.3 Summary and Problems
CHAPTER 5. Iteration ¶
5.1 For Loops
5.2 While Loops
5.3 Comprehensions
5.4 Summary and Problems
CHAPTER 6. Recursion ¶
6.1 Recursive Functions
6.2 Divide and Conquer
6.3 Summary and Problems
CHAPTER 7. Object Oriented Programming (OOP) ¶
7.1 Introduction to OOP
7.2 Class and Object
7.3 Inheritance
7.4 Summary and Problems
CHAPTER 8. Complexity ¶
8.1 Complexity and Big-O Notation
8.2 Complexity Matters
8.3 The Profiler
8.4 Summary and Problems
CHAPTER 9. Representation of Numbers ¶
9.1 Base-N and Binary
9.2 Floating Point Numbers
9.3 Round-off Errors
9.4 Summary and Problems
CHAPTER 10. Errors, Good Programming Practices, and Debugging ¶
10.1 Error Types
10.2 Avoiding Errors
10.3 Try/Except
10.4 Type Checking
10.5 Debugging
10.6 Summary and Problems
CHAPTER 11. Reading and Writing Data ¶
11.1 TXT Files
11.2 CSV Files
11.3 Pickle Files
11.4 JSON Files
11.5 HDF5 Files
11.6 Summary and Problems
CHAPTER 12. Visualization and Plotting ¶
12.1 2D Plotting
12.2 3D Plotting
12.3 Working with Maps
12.4 Animations and Movies
12.5 Summary and Problems
CHAPTER 13. Parallel Your Python ¶
13.1 Parallel Computing Basics
13.2 Multiprocessing
13.3 Use joblib
13.4 Summary and Problems
PART II INTRODUCTION TO NUMERICAL METHODS ¶
Chapter 14. linear algebra and systems of linear equations ¶.
14.1 Basics of Linear Algebra
14.2 Linear Transformations
14.3 Systems of Linear Equations
14.4 Solutions to Systems of Linear Equations
14.5 Solve Systems of Linear Equations in Python
14.6 Matrix Inversion
14.7 Summary and Problems
CHAPTER 15. Eigenvalues and Eigenvectors ¶
15.1 Eigenvalues and Eigenvectors Problem Statement
15.2 The Power Method
15.3 The QR Method
15.4 Eigenvalues and Eigenvectors in Python
15.5 Summary and Problems
CHAPTER 16. Least Squares Regression ¶
16.1 Least Squares Regression Problem Statement
16.2 Least Squares Regression Derivation (Linear Algebra)
16.3 Least Squares Regression Derivation (Multivariable Calculus)
16.4 Least Squares Regression in Python
16.5 Least Square Regression for Nonlinear Functions
16.6 Summary and Problems
CHAPTER 17. Interpolation ¶
17.1 Interpolation Problem Statement
17.2 Linear Interpolation
17.3 Cubic Spline Interpolation
17.4 Lagrange Polynomial Interpolation
17.5 Newton’s Polynomial Interpolation
17.6 Summary and Problems
CHAPTER 18. Series ¶
18.1 Expressing Functions with Taylor Series
18.2 Approximations with Taylor Series
18.3 Discussion on Errors
18.4 Summary and Problems
CHAPTER 19. Root Finding ¶
19.1 Root Finding Problem Statement
19.2 Tolerance
19.3 Bisection Method
19.4 Newton-Raphson Method
19.5 Root Finding in Python
19.6 Summary and Problems
CHAPTER 20. Numerical Differentiation ¶
20.1 Numerical Differentiation Problem Statement
20.2 Finite Difference Approximating Derivatives
20.3 Approximating of Higher Order Derivatives
20.4 Numerical Differentiation with Noise
20.5 Summary and Problems
CHAPTER 21. Numerical Integration ¶
21.1 Numerical Integration Problem Statement
21.2 Riemann’s Integral
21.3 Trapezoid Rule
21.4 Simpson’s Rule
21.5 Computing Integrals in Python
21.6 Summary and Problems
CHAPTER 22. Ordinary Differential Equations (ODEs): Initial-Value Problems ¶
22.1 ODE Initial Value Problem Statement
22.2 Reduction of Order
22.3 The Euler Method
22.4 Numerical Error and Instability
22.5 Predictor-Corrector Methods
22.6 Python ODE Solvers (IVP)
22.7 Advanced Topics
22.8 Summary and Problems
CHAPTER 23. Ordinary Differential Equations: Boundary-Value Problems ¶
23.1 ODE Boundary Value Problem Statement
23.2 The Shooting Method
23.3 Finite Difference Method
23.4 Numerical Error and Instability
23.5 Python ODE Solvers
23.6 Summary and Problems
CHAPTER 24. Fourier Transforms ¶
24.1 The Basics of Waves
24.2 Discrete Fourier Transform (DFT)
24.3 Fast Fourier Transform (FFT)
24.4 FFT in Python
24.5 Summary and Problems
CHAPTER 25. Introduction to Machine Learning ¶
25.1 Concept of Machine Learning
25.2 Classification
25.3 Regression
25.4 Clustering
25.5 Summary and Problems
Appendix A. Getting-Started-with-Python-Windows ¶
Applying Math with Python: Over 70 practical recipes for solving real-world computational math problems
Discover easy-to-follow solutions and techniques to help you to implement applied mathematical concepts such as probability, calculus, and equations using Python's numeric and scientific libraries
Key Features
- Compute complex mathematical problems using programming logic with the help of step-by-step recipes
- Learn how to use Python libraries for computation, mathematical modeling, and statistics
- Discover simple yet effective techniques for solving mathematical equations and apply them in real-world statistics
Book Description
What you will learn.
- Become familiar with basic Python packages, tools, and libraries for solving mathematical problems
- Explore real-world applications of mathematics to reduce a problem in optimization
- Understand the core concepts of applied mathematics and their application in computer science
- Find out how to choose the most suitable package, tool, or technique to solve a problem
- Implement basic mathematical plotting, change plot styles, and add labels to plots using Matplotlib
- Get to grips with probability theory with the Bayesian inference and Markov Chain Monte Carlo (MCMC) methods
Who this book is for
Whether you are a professional programmer or a student looking to solve mathematical problems computationally using Python, this is the book for you. Advanced mathematics proficiency is not a prerequisite, but basic knowledge of mathematics will help you to get the most out of this Python math book. Familiarity with the concepts of data structures in Python is assumed.
IEEE Account
- Change Username/Password
- Update Address
Purchase Details
- Payment Options
- Order History
- View Purchased Documents
Profile Information
- Communications Preferences
- Profession and Education
- Technical Interests
- US & Canada: +1 800 678 4333
- Worldwide: +1 732 981 0060
- Contact & Support
- About IEEE Xplore
- Accessibility
- Terms of Use
- Nondiscrimination Policy
- Privacy & Opting Out of Cookies
A not-for-profit organization, IEEE is the world's largest technical professional organization dedicated to advancing technology for the benefit of humanity. © Copyright 2024 IEEE - All rights reserved. Use of this web site signifies your agreement to the terms and conditions.
- Penn Engineering Online Degrees
- Penn Engineering Online Dual Dual Degree
- Online Graduate Certificates
- Take a Course
- On-Demand Learning
- Computational Thinking for Problem Solving
- Lifelong Learning
Anyone can learn to think like a computer scientist.
In this course, you will learn about the pillars of computational thinking, how computer scientists develop and analyze algorithms, and how solutions can be realized on a computer using the Python programming language. By the end of the course, you will be able to develop an algorithm and express it to the computer by writing a simple Python program.
This course will introduce you to people from diverse professions who use computational thinking to solve problems. You will engage with a unique community of analytical thinkers and be encouraged to consider how you can make a positive social impact through computational thinking.
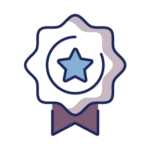
Flexible deadlines
Reset deadlines in accordance to your schedule.
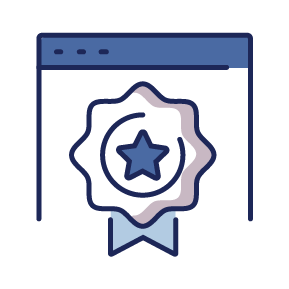
Shareable Certificate
Earn a digital certificate upon completion.
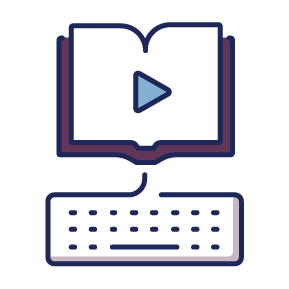
100% online
Start instantly and learn at your own schedule.
Start building your computer science skills
Open course: computational thinking for problem solving.
Get full access to Applied Computational Thinking with Python - Second Edition and 60K+ other titles, with a free 10-day trial of O'Reilly.
There are also live events, courses curated by job role, and more.
Applied Computational Thinking with Python - Second Edition
Read it now on the O’Reilly learning platform with a 10-day free trial.
O’Reilly members get unlimited access to books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.
Book description
Use the computational thinking philosophy to solve complex problems by designing appropriate algorithms to produce optimal results across various domains
Key Features
- Develop logical reasoning and problem-solving skills that will help you tackle complex problems
- Explore core computer science concepts and important computational thinking elements using practical examples
- Find out how to identify the best-suited algorithmic solution for your problem
Book Description
Computational thinking helps you to develop logical processing and algorithmic thinking while solving real-world problems across a wide range of domains. It's an essential skill that you should possess to keep ahead of the curve in this modern era of information technology. Developers can apply their knowledge of computational thinking to solve problems in multiple areas, including economics, mathematics, and artificial intelligence.
This book begins by helping you get to grips with decomposition, pattern recognition, pattern generalization and abstraction, and algorithm design, along with teaching you how to apply these elements practically while designing solutions for challenging problems. You’ll then learn about various techniques involved in problem analysis, logical reasoning, algorithm design, clusters and classification, data analysis, and modeling, and understand how computational thinking elements can be used together with these aspects to design solutions. Toward the end, you will discover how to identify pitfalls in the solution design process and how to choose the right functionalities to create the best possible algorithmic solutions.
By the end of this algorithm book, you will have gained the confidence to successfully apply computational thinking techniques to software development.
What you will learn
- Find out how to use decomposition to solve problems through visual representation
- Employ pattern generalization and abstraction to design solutions
- Build analytical skills to assess algorithmic solutions
- Use computational thinking with Python for statistical analysis
- Understand the input and output needs for designing algorithmic solutions
- Use computational thinking to solve data processing problems
- Identify errors in logical processing to refine your solution design
- Apply computational thinking in domains, such as cryptography, and machine learning
- Who this book is for
This book is for students, developers, and professionals looking to develop problem-solving skills and tactics involved in writing or debugging software programs and applications. Familiarity with Python programming is required.
Table of contents
- Applied Computational Thinking with Python
- Contributors
- About the authors
- About the reviewers
- What this book covers
- To get the most out of this book
- Download the example code files
- Download the color images
- Conventions used
- Get in touch
- Share Your Thoughts
- Download a free PDF copy of this book
- Part 1: An Introduction to Computational Thinking
- Technical requirements
- Learning about computers and the binary system
- Coding theory
- Computational biology
- Data structures
- Information theory
- Automata theory
- Formal language theory
- Symbolic computation
- Computational geometry
- Computational number theory
- Operating systems
- Application software
- Architecture
- Programming languages
- Problem 1 – conditions
- Decomposing problems
- Problem 2 – mathematical algorithms and generalization
- Generalizing patterns
- Designing algorithms
- Problem 3 – children’s soccer party
- Problem 4 – savings and interest
- Algorithms should be clear and unambiguous
- Algorithms should have inputs and outputs that are well defined
- Algorithms should have finiteness
- Algorithms should be feasible
- Algorithms should be language independent
- Problem 1 – an office lunch
- Problem 2 – a catering company
- Algorithm analysis 1 – states and capitals
- Algorithm analysis 2 – terminating or not terminating?
- Applying inductive reasoning
- Applying deductive reasoning
- The and operator
- The or operator
- The not operator
- Syntax errors
- Learning to identify logical errors
- Errors and debugging
- Problem 6A – building an online store
- Converting the flowchart into an algorithm
- Problem 6B – analyzing a simple game problem
- Designing solutions
- Problem 1 – a marketing survey
- Diagramming solutions
- Problem 2 – pizza order
- Problem 3 – delays and Python
- Errors in logic
- Debugging algorithms
- Problem 1 – printing even numbers
- Refining and redefining solutions
- Part 2: Applying Python and Computational Thinking
- Mathematical built-in functions
- Defining and using dictionaries
- Defining and using lists
- Variables in Python
- Working with functions
- Handling files in Python
- Data in Python
- Using iteration in algorithms
- Problem 1 – creating a book library
- Problem 2 – organizing information
- Using inheritance
- Defining input and output
- Problem 1 – building a Caesar cipher
- Problem 2 – finding maximums
- Problem 3 – building a guessing game
- Defining control flow and its tools
- Using nested if statements
- Using for loops and range()
- Using other loops and conditionals
- Revisiting functions
- Decomposing the problem and using Python functionalities
- Generalizing the problem and planning Python algorithms
- Designing and testing the algorithm
- Errors in punctuation
- Errors with indentation
- Global variables
- Local variables
- Errors when using global and local variables
- Part 3: Data Processing, Analysis, and Applications Using Computational Thinking and Python
- Defining experimental data
- Installing libraries
- Using NumPy and pandas
- Using Matplotlib
- Understanding data analysis with Python
- Using the Seaborn library
- Using the SciPy library
- Using the Scikit-Learn library
- Defining ML
- Phase 1 – preparation and problem definition
- Phase 2 – data preprocessing and model development
- Phase 3 – optimization and deployment
- Chocolate cake analogy to ML life cycle
- Types of ML algorithms
- Classifying data
- Using the scikit-learn library
- Defining optimization models
- Using the BIRCH algorithm
- Using the K-means clustering algorithm
- Defining pandas
- Determining when to use pandas
- Data cleaning
- Transforming data
- Reducing data
- Processing, analyzing, and summarizing data using visualizations
- Problem 1 – using Python to analyze historical speeches
- Defining, decomposing, and planning a story
- Problem 3 – using Python to calculate text readability
- Defining the problem (TSP)
- Recognizing the pattern (TSP)
- Generalizing (TSP)
- Designing the algorithm (TSP)
- Defining the problem (cryptography)
- Recognizing the pattern (cryptography)
- Generalizing (cryptography)
- Designing the algorithm (cryptography)
- Problem 6 – using Python in cybersecurity
- Problem 7 – using Python to create a chatbot
- Step 1 – import the required libraries
- Step 2 – define the URL to scrape
- Step 3 – make an HTTP request
- Step 4 – parse the HTML content
- Step 5 – locate the quote containers
- Step 6 – loop through containers and extract data
- Problem 9 – using Python to create a QR code
- Problem 1 – using Python to create tessellations
- Problem 2 – using Python in biological data analysis
- Defining the specific problem to analyze and identify the population
- Defining the problem
- Algorithm and visual representations of data
- The fundamentals of the Multinomial Event Model
- Problem 6 – using Python to analyze genetic data
- Problem 7 – using Python to analyze stocks
- Problem 8 – using Python to create a CNN
- AWS and Python in cloud computing – a brief overview
- Creating a new AWS account
- Understanding IAM in AWS
- Understanding AWS pricing and the Free Tier
- AWS computer services overview
- Setting up Boto3
- Basic Python examples using Boto3
- Further reading
- Why subscribe?
- Packt is searching for authors like you
Product information
- Title: Applied Computational Thinking with Python - Second Edition
- Author(s): Sofía De Jesús, Dayrene Martinez
- Release date: December 2023
- Publisher(s): Packt Publishing
- ISBN: 9781837632305
You might also like
Applying math with python - second edition.
by Sam Morley
Discover easy-to-follow solutions and techniques to help you to implement applied mathematical concepts such as probability, …
Deep Learning with Python, Second Edition
by Francois Chollet
Printed in full color! Unlock the groundbreaking advances of deep learning with this extensively revised new …
Advanced Python Programming - Second Edition
by Quan Nguyen
Write fast, robust, and highly reusable applications using Python's internal optimization, state-of-the-art performance-benchmarking tools, and cutting-edge …
Machine Learning with Python Cookbook, 2nd Edition
by Kyle Gallatin, Chris Albon
This practical guide provides more than 200 self-contained recipes to help you solve machine learning challenges …
Don’t leave empty-handed
Get Mark Richards’s Software Architecture Patterns ebook to better understand how to design components—and how they should interact.
It’s yours, free.
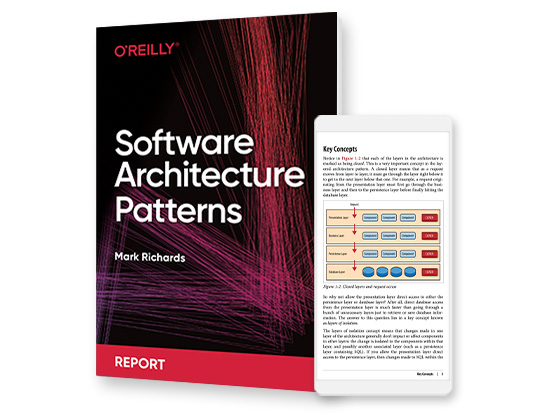
Check it out now on O’Reilly
Dive in for free with a 10-day trial of the O’Reilly learning platform—then explore all the other resources our members count on to build skills and solve problems every day.
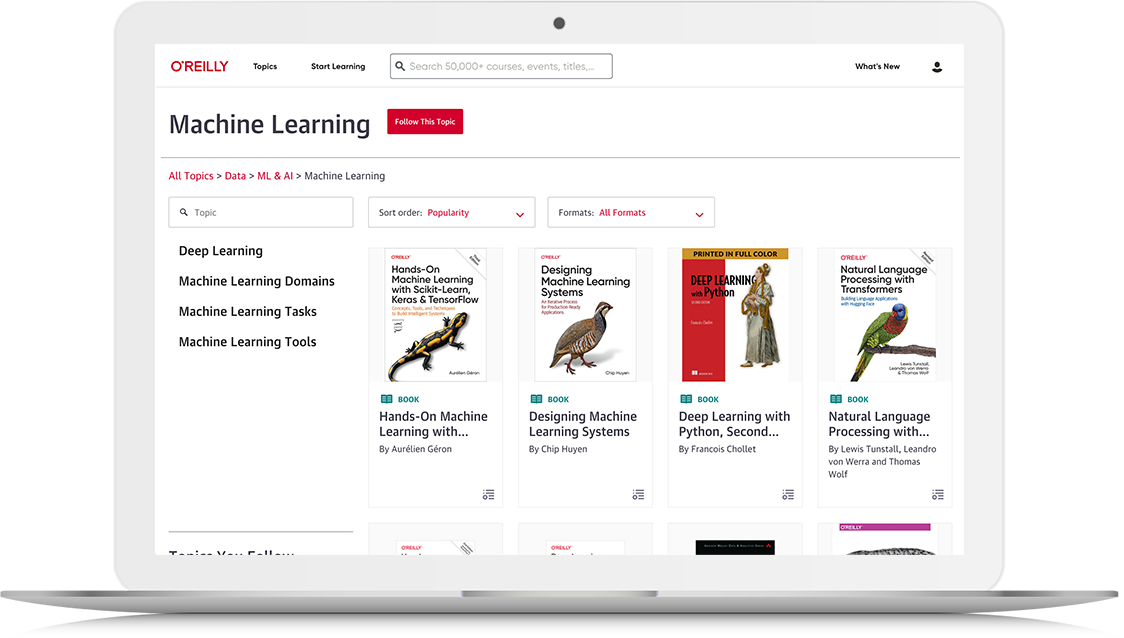
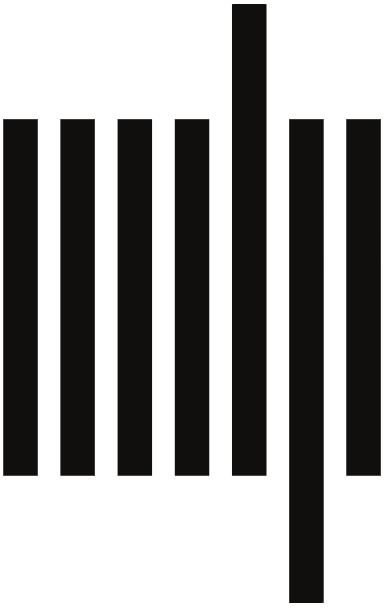
On the site
- Introduction to Computation and Programming Using Python
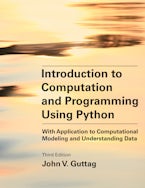
Introduction to Computation and Programming Using Python , third edition
With application to computational modeling and understanding data.
by John V. Guttag
ISBN: 9780262542364
Pub date: January 5, 2021
- Publisher: The MIT Press
664 pp. , 7 x 9 in , 140
ISBN: 9780262363433
Pub date: March 2, 2021
eTextbook rental
- 9780262542364
- Published: January 2021
- 9780262363433
- Published: March 2021
- MIT Press Bookstore
- Penguin Random House
- Barnes and Noble
- Bookshop.org
- Books a Million
Other Retailers:
- Amazon.co.uk
- Waterstones
- Description
The new edition of an introduction to the art of computational problem solving using Python.
This book introduces students with little or no prior programming experience to the art of computational problem solving using Python and various Python libraries, including numpy, matplotlib, random, pandas, and sklearn. It provides students with skills that will enable them to make productive use of computational techniques, including some of the tools and techniques of data science for using computation to model and interpret data as well as substantial material on machine learning.
The book is based on an MIT course and was developed for use not only in a conventional classroom but in a massive open online course (MOOC). It contains material suitable for a two-semester introductory computer science sequence.
This third edition has expanded the initial explanatory material, making it a gentler introduction to programming for the beginner, with more programming examples and many more “finger exercises.” A new chapter shows how to use the Pandas package for analyzing time series data. All the code has been rewritten to make it stylistically consistent with the PEP 8 standards. Although it covers such traditional topics as computational complexity and simple algorithms, the book focuses on a wide range of topics not found in most introductory texts, including information visualization, simulations to model randomness, computational techniques to understand data, and statistical techniques that inform (and misinform) as well as two related but relatively advanced topics: optimization problems and dynamic programming. The book also includes a Python 3 quick reference guide.
All of the code in the book and an errata sheet are available on the book's web page on the MIT Press website.
John V. Guttag is the Dugald C. Jackson Professor of Computer Science and Electrical Engineering at MIT.
Additional Material
Table of Contents
Introduction to Computer Science and Programming edX Course
Author's Website with Code and Errata
Author Video - MIT, edX, and OCW Courses
Introduction to Computer Science and Programming OpenCourseWare
Author Video - Accessibility at Different Levels
Author Video - New Chapters and Sections
Author Video - Use of the Book in Courses
Python Practice for Beginners: 15 Hands-On Problems

- online practice
Want to put your Python skills to the test? Challenge yourself with these 15 Python practice exercises taken directly from our Python courses!
There’s no denying that solving Python exercises is one of the best ways to practice and improve your Python skills . Hands-on engagement with the language is essential for effective learning. This is exactly what this article will help you with: we've curated a diverse set of Python practice exercises tailored specifically for beginners seeking to test their programming skills.
These Python practice exercises cover a spectrum of fundamental concepts, all of which are covered in our Python Data Structures in Practice and Built-in Algorithms in Python courses. Together, both courses add up to 39 hours of content. They contain over 180 exercises for you to hone your Python skills. In fact, the exercises in this article were taken directly from these courses!
In these Python practice exercises, we will use a variety of data structures, including lists, dictionaries, and sets. We’ll also practice basic programming features like functions, loops, and conditionals. Every exercise is followed by a solution and explanation. The proposed solution is not necessarily the only possible answer, so try to find your own alternative solutions. Let’s get right into it!
Python Practice Problem 1: Average Expenses for Each Semester
John has a list of his monthly expenses from last year:
He wants to know his average expenses for each semester. Using a for loop, calculate John’s average expenses for the first semester (January to June) and the second semester (July to December).
Explanation
We initialize two variables, first_semester_total and second_semester_total , to store the total expenses for each semester. Then, we iterate through the monthly_spending list using enumerate() , which provides both the index and the corresponding value in each iteration. If you have never heard of enumerate() before – or if you are unsure about how for loops in Python work – take a look at our article How to Write a for Loop in Python .
Within the loop, we check if the index is less than 6 (January to June); if so, we add the expense to first_semester_total . If the index is greater than 6, we add the expense to second_semester_total .
After iterating through all the months, we calculate the average expenses for each semester by dividing the total expenses by 6 (the number of months in each semester). Finally, we print out the average expenses for each semester.
Python Practice Problem 2: Who Spent More?
John has a friend, Sam, who also kept a list of his expenses from last year:
They want to find out how many months John spent more money than Sam. Use a for loop to compare their expenses for each month. Keep track of the number of months where John spent more money.
We initialize the variable months_john_spent_more with the value zero. Then we use a for loop with range(len()) to iterate over the indices of the john_monthly_spending list.
Within the loop, we compare John's expenses with Sam's expenses for the corresponding month using the index i . If John's expenses are greater than Sam's for a particular month, we increment the months_john_spent_more variable. Finally, we print out the total number of months where John spent more money than Sam.
Python Practice Problem 3: All of Our Friends
Paul and Tina each have a list of their respective friends:
Combine both lists into a single list that contains all of their friends. Don’t include duplicate entries in the resulting list.
There are a few different ways to solve this problem. One option is to use the + operator to concatenate Paul and Tina's friend lists ( paul_friends and tina_friends ). Afterwards, we convert the combined list to a set using set() , and then convert it back to a list using list() . Since sets cannot have duplicate entries, this process guarantees that the resulting list does not hold any duplicates. Finally, we print the resulting combined list of friends.
If you need a refresher on Python sets, check out our in-depth guide to working with sets in Python or find out the difference between Python sets, lists, and tuples .
Python Practice Problem 4: Find the Common Friends
Now, let’s try a different operation. We will start from the same lists of Paul’s and Tina’s friends:
In this exercise, we’ll use a for loop to get a list of their common friends.
For this problem, we use a for loop to iterate through each friend in Paul's list ( paul_friends ). Inside the loop, we check if the current friend is also present in Tina's list ( tina_friends ). If it is, it is added to the common_friends list. This approach guarantees that we test each one of Paul’s friends against each one of Tina’s friends. Finally, we print the resulting list of friends that are common to both Paul and Tina.
Python Practice Problem 5: Find the Basketball Players
You work at a sports club. The following sets contain the names of players registered to play different sports:
How can you obtain a set that includes the players that are only registered to play basketball (i.e. not registered for football or volleyball)?
This type of scenario is exactly where set operations shine. Don’t worry if you never heard about them: we have an article on Python set operations with examples to help get you up to speed.
First, we use the | (union) operator to combine the sets of football and volleyball players into a single set. In the same line, we use the - (difference) operator to subtract this combined set from the set of basketball players. The result is a set containing only the players registered for basketball and not for football or volleyball.
If you prefer, you can also reach the same answer using set methods instead of the operators:
It’s essentially the same operation, so use whichever you think is more readable.
Python Practice Problem 6: Count the Votes
Let’s try counting the number of occurrences in a list. The list below represent the results of a poll where students were asked for their favorite programming language:
Use a dictionary to tally up the votes in the poll.
In this exercise, we utilize a dictionary ( vote_tally ) to count the occurrences of each programming language in the poll results. We iterate through the poll_results list using a for loop; for each language, we check if it already is in the dictionary. If it is, we increment the count; otherwise, we add the language to the dictionary with a starting count of 1. This approach effectively tallies up the votes for each programming language.
If you want to learn more about other ways to work with dictionaries in Python, check out our article on 13 dictionary examples for beginners .
Python Practice Problem 7: Sum the Scores
Three friends are playing a game, where each player has three rounds to score. At the end, the player whose total score (i.e. the sum of each round) is the highest wins. Consider the scores below (formatted as a list of tuples):
Create a dictionary where each player is represented by the dictionary key and the corresponding total score is the dictionary value.
This solution is similar to the previous one. We use a dictionary ( total_scores ) to store the total scores for each player in the game. We iterate through the list of scores using a for loop, extracting the player's name and score from each tuple. For each player, we check if they already exist as a key in the dictionary. If they do, we add the current score to the existing total; otherwise, we create a new key in the dictionary with the initial score. At the end of the for loop, the total score of each player will be stored in the total_scores dictionary, which we at last print.
Python Practice Problem 8: Calculate the Statistics
Given any list of numbers in Python, such as …
… write a function that returns a tuple containing the list’s maximum value, sum of values, and mean value.
We create a function called calculate_statistics to calculate the required statistics from a list of numbers. This function utilizes a combination of max() , sum() , and len() to obtain these statistics. The results are then returned as a tuple containing the maximum value, the sum of values, and the mean value.
The function is called with the provided list and the results are printed individually.
Python Practice Problem 9: Longest and Shortest Words
Given the list of words below ..
… find the longest and the shortest word in the list.
To find the longest and shortest word in the list, we initialize the variables longest_word and shortest_word as the first word in the list. Then we use a for loop to iterate through the word list. Within the loop, we compare the length of each word with the length of the current longest and shortest words. If a word is longer than the current longest word, it becomes the new longest word; on the other hand, if it's shorter than the current shortest word, it becomes the new shortest word. After iterating through the entire list, the variables longest_word and shortest_word will hold the corresponding words.
There’s a catch, though: what happens if two or more words are the shortest? In that case, since the logic used is to overwrite the shortest_word only if the current word is shorter – but not of equal length – then shortest_word is set to whichever shortest word appears first. The same logic applies to longest_word , too. If you want to set these variables to the shortest/longest word that appears last in the list, you only need to change the comparisons to <= (less or equal than) and >= (greater or equal than), respectively.
If you want to learn more about Python strings and what you can do with them, be sure to check out this overview on Python string methods .
Python Practice Problem 10: Filter a List by Frequency
Given a list of numbers …
… create a new list containing only the numbers that occur at least three times in the list.
Here, we use a for loop to iterate through the number_list . In the loop, we use the count() method to check if the current number occurs at least three times in the number_list . If the condition is met, the number is appended to the filtered_list .
After the loop, the filtered_list contains only numbers that appear three or more times in the original list.
Python Practice Problem 11: The Second-Best Score
You’re given a list of students’ scores in no particular order:
Find the second-highest score in the list.
This one is a breeze if we know about the sort() method for Python lists – we use it here to sort the list of exam results in ascending order. This way, the highest scores come last. Then we only need to access the second to last element in the list (using the index -2 ) to get the second-highest score.
Python Practice Problem 12: Check If a List Is Symmetrical
Given the lists of numbers below …
… create a function that returns whether a list is symmetrical. In this case, a symmetrical list is a list that remains the same after it is reversed – i.e. it’s the same backwards and forwards.
Reversing a list can be achieved by using the reverse() method. In this solution, this is done inside the is_symmetrical function.
To avoid modifying the original list, a copy is created using the copy() method before using reverse() . The reversed list is then compared with the original list to determine if it’s symmetrical.
The remaining code is responsible for passing each list to the is_symmetrical function and printing out the result.
Python Practice Problem 13: Sort By Number of Vowels
Given this list of strings …
… sort the list by the number of vowels in each word. Words with fewer vowels should come first.
Whenever we need to sort values in a custom order, the easiest approach is to create a helper function. In this approach, we pass the helper function to Python’s sorted() function using the key parameter. The sorting logic is defined in the helper function.
In the solution above, the custom function count_vowels uses a for loop to iterate through each character in the word, checking if it is a vowel in a case-insensitive manner. The loop increments the count variable for each vowel found and then returns it. We then simply pass the list of fruits to sorted() , along with the key=count_vowels argument.
Python Practice Problem 14: Sorting a Mixed List
Imagine you have a list with mixed data types: strings, integers, and floats:
Typically, you wouldn’t be able to sort this list, since Python cannot compare strings to numbers. However, writing a custom sorting function can help you sort this list.
Create a function that sorts the mixed list above using the following logic:
- If the element is a string, the length of the string is used for sorting.
- If the element is a number, the number itself is used.
As proposed in the exercise, a custom sorting function named custom_sort is defined to handle the sorting logic. The function checks whether each element is a string or a number using the isinstance() function. If the element is a string, it returns the length of the string for sorting; if it's a number (integer or float), it returns the number itself.
The sorted() function is then used to sort the mixed_list using the logic defined in the custom sorting function.
If you’re having a hard time wrapping your head around custom sort functions, check out this article that details how to write a custom sort function in Python .
Python Practice Problem 15: Filter and Reorder
Given another list of strings, such as the one below ..
.. create a function that does two things: filters out any words with three or fewer characters and sorts the resulting list alphabetically.
Here, we define filter_and_sort , a function that does both proposed tasks.
First, it uses a for loop to filter out words with three or fewer characters, creating a filtered_list . Then, it sorts the filtered list alphabetically using the sorted() function, producing the final sorted_list .
The function returns this sorted list, which we print out.
Want Even More Python Practice Problems?
We hope these exercises have given you a bit of a coding workout. If you’re after more Python practice content, head straight for our courses on Python Data Structures in Practice and Built-in Algorithms in Python , where you can work on exciting practice exercises similar to the ones in this article.
Additionally, you can check out our articles on Python loop practice exercises , Python list exercises , and Python dictionary exercises . Much like this article, they are all targeted towards beginners, so you should feel right at home!
You may also like
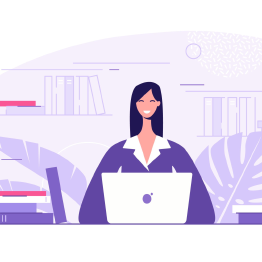
How Do You Write a SELECT Statement in SQL?
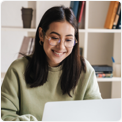
What Is a Foreign Key in SQL?

Enumerate and Explain All the Basic Elements of an SQL Query
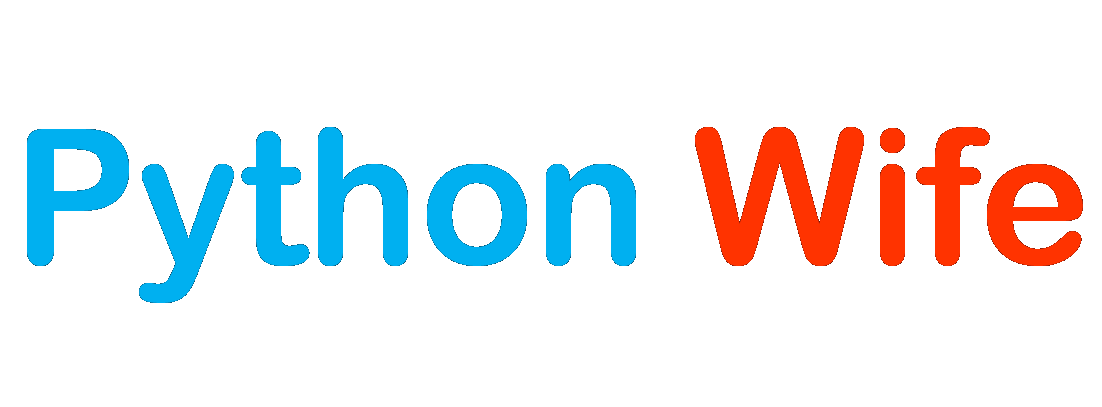
- Computer Vision
- Problem Solving in Python
- Intro to DS and Algo
- Analysis of Algorithm
- Dictionaries
- Linked Lists
- Doubly Linked Lists
- Circular Singly Linked List
- Circular Doubly Linked List
- Tree/Binary Tree
- Binary Search Tree
- Binary Heap
- Sorting Algorithms
- Searching Algorithms
- Single-Source Shortest Path
- Topological Sort
- Dijkstra’s
- Bellman-Ford’s
- All Pair Shortest Path
- Minimum Spanning Tree
- Kruskal & Prim’s
Problem-solving is the process of identifying a problem, creating an algorithm to solve the given problem, and finally implementing the algorithm to develop a computer program .
An algorithm is a process or set of rules to be followed while performing calculations or other problem-solving operations. It is simply a set of steps to accomplish a certain task.
In this article, we will discuss 5 major steps for efficient problem-solving. These steps are:
- Understanding the Problem
- Exploring Examples
- Breaking the Problem Down
- Solving or Simplification
- Looking back and Refactoring
While understanding the problem, we first need to closely examine the language of the question and then proceed further. The following questions can be helpful while understanding the given problem at hand.
- Can the problem be restated in our own words?
- What are the inputs that are needed for the problem?
- What are the outputs that come from the problem?
- Can the outputs be determined from the inputs? In other words, do we have enough information to solve the given problem?
- What should the important pieces of data be labeled?
Example : Write a function that takes two numbers and returns their sum.
- Implement addition
- Integer, Float, etc.
Once we have understood the given problem, we can look up various examples related to it. The examples should cover all situations that can be encountered while the implementation.
- Start with simple examples.
- Progress to more complex examples.
- Explore examples with empty inputs.
- Explore examples with invalid inputs.
Example : Write a function that takes a string as input and returns the count of each character
After exploring examples related to the problem, we need to break down the given problem. Before implementation, we write out the steps that need to be taken to solve the question.
Once we have laid out the steps to solve the problem, we try to find the solution to the question. If the solution cannot be found, try to simplify the problem instead.
The steps to simplify a problem are as follows:
- Find the core difficulty
- Temporarily ignore the difficulty
- Write a simplified solution
- Then incorporate that difficulty
Since we have completed the implementation of the problem, we now look back at the code and refactor it if required. It is an important step to refactor the code so as to improve efficiency.
The following questions can be helpful while looking back at the code and refactoring:
- Can we check the result?
- Can we derive the result differently?
- Can we understand it at a glance?
- Can we use the result or mehtod for some other problem?
- Can you improve the performance of the solution?
- How do other people solve the problem?
Trending Posts You Might Like
- File Upload / Download with Streamlit
- Dijkstra’s Algorithm in Python
- Seaborn with STREAMLIT
- Greedy Algorithms in Python
Author : Bhavya

- Latest Articles
- Top Articles
- Posting/Update Guidelines
- Article Help Forum

- View Unanswered Questions
- View All Questions
- View C# questions
- View C++ questions
- View Javascript questions
- View Visual Basic questions
- View Python questions
- CodeProject.AI Server
- All Message Boards...
- Running a Business
- Sales / Marketing
- Collaboration / Beta Testing
- Work Issues
- Design and Architecture
- Artificial Intelligence
- Internet of Things
- ATL / WTL / STL
- Managed C++/CLI
- Objective-C and Swift
- System Admin
- Hosting and Servers
- Linux Programming
- .NET (Core and Framework)
- Visual Basic
- Web Development
- Site Bugs / Suggestions
- Spam and Abuse Watch
- Competitions
- The Insider Newsletter
- The Daily Build Newsletter
- Newsletter archive
- CodeProject Stuff
- Most Valuable Professionals
- The Lounge
- The CodeProject Blog
- Where I Am: Member Photos
- The Insider News
- The Weird & The Wonderful
- What is 'CodeProject'?
- General FAQ
- Ask a Question
- Bugs and Suggestions

Using Python to Solve Computational Physics Problems

Introduction
Laplace equation is a simple second-order partial differential equation. It is also a simplest example of elliptic partial differential equation. This equation is very important in science, especially in physics, because it describes behaviour of electric and gravitation potential, and also heat conduction. In thermodynamics (heat conduction), we call Laplace equation as steady-state heat equation or heat conduction equation.
In this article, we will solve the Laplace equation using numerical approach rather than analytical/calculus approach. When we say numerical approach, we refer to discretization. Discretization is a process to "transform" the continuous form of differential equation into a discrete form of differential equation; it also means that with discretization, we can transform the calculus problem into matrix algebra problem, which is favored by programming.
Here, we want to solve a simple heat conduction problem using finite difference method. We will use Python Programming Language, Numpy (numerical library for Python), and Matplotlib (library for plotting and visualizing data using Python) as the tools. We'll also see that we can write less code and do more with Python.
In computational physics, we "always" use programming to solve the problem, because computer program can calculate large and complex calculation "quickly". Computational physics can be represented as this diagram.
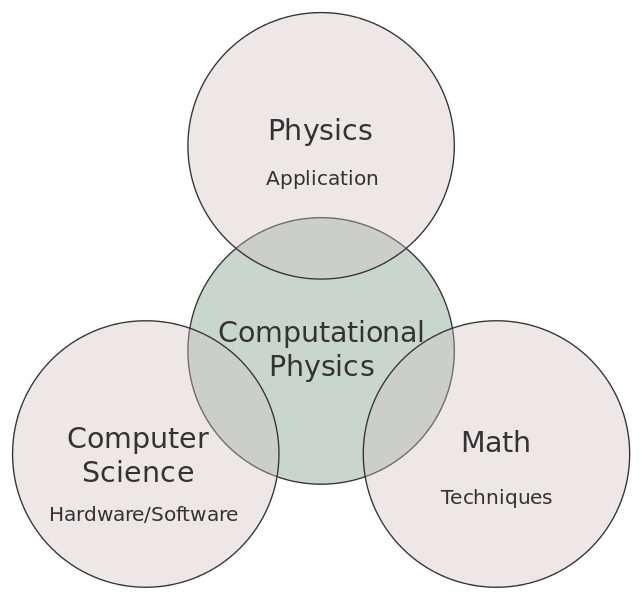
There are so many programming languages that are used today to solve many numerical problems, Matlab for example. But here, we will use Python, the "easy to learn" programming language, and of course, it's free. It also has powerful numerical, scientific, and data visualization library such as Numpy, Scipy, and Matplotlib. Python also provides parallel execution and we can run it in computer clusters.
Back to Laplace equation, we will solve a simple 2-D heat conduction problem using Python in the next section. Here, I assume the readers have basic knowledge of finite difference method, so I do not write the details behind finite difference method, details of discretization error, stability, consistency, convergence, and fastest/optimum iterating algorithm. We will skip many steps of computational formula here.
Instead of solving the problem with the numerical-analytical validation, we only demonstrate how to solve the problem using Python, Numpy, and Matplotlib, and of course, with a little bit of simplistic sense of computational physics, so the source code here makes sense to general readers who don't specialize in computational physics.
Preparation
To produce the result below, I use this environment:
- OS : Linux Ubuntu 14.04 LTS
- Python : Python 2.7
- Numpy : Numpy 1.10.4
- Matplotlib : Matplotlib 1.5.1
If you are running Ubuntu, you can use pip to install Numpy and Matplotib, or you can run this command in your terminal.
and use this command to install Matplotlib:
Note that Python is already installed in Ubuntu 14.04. To try Python, just type Python in your Terminal and press Enter.
You can also use Python, Numpy and Matplotlib in Windows OS, but I prefer to use Ubuntu instead.
Using the Code
This is the Laplace equation in 2-D cartesian coordinates (for heat equation):
Where T is temperature, x is x-dimension, and y is y-dimension. x and y are functions of position in Cartesian coordinates. If you are interested to see the analytical solution of the equation above, you can look it up here .
Here, we only need to solve 2-D form of the Laplace equation. The problem to solve is shown below:
What we will do is find the steady state temperature inside the 2-D plat (which also means the solution of Laplace equation) above with the given boundary conditions (temperature of the edge of the plat). Next, we will discretize the region of the plat, and divide it into meshgrid, and then we discretize the Laplace equation above with finite difference method. This is the discretized region of the plat.
We set Δ x = Δ y = 1 cm , and then make the grid as shown below:
Note that the green nodes are the nodes that we want to know the temperature there (the solution), and the white nodes are the boundary conditions (known temperature). Here is the discrete form of Laplace Equation above.
In our case, the final discrete equation is shown below.
Now, we are ready to solve the equation above. To solve this, we use "guess value" of interior grid (green nodes), here we set it to 30 degree Celsius (or we can set it 35 or other value), because we don't know the value inside the grid (of course, those are the values that we want to know). Then, we will iterate the equation until the difference between value before iteration and the value until iteration is "small enough", we call it convergence. In the process of iterating, the temperature value in the interior grid will adjust itself, it's "selfcorrecting", so when we set a guess value closer to its actual solution, the faster we get the "actual" solution.
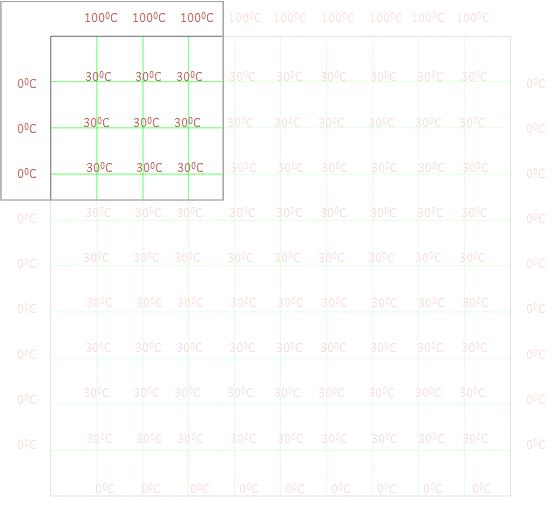
We are ready for the source code. In order to use Numpy library, we need to import Numpy in our source code, and we also need to import Matplolib.Pyplot module to plot our solution. So the first step is to import necessary modules.
and then, we set the initial variables into our Python source code:
What we will do next is to set the "plot window" and meshgrid . Here is the code:
np.meshgrid() creates the mesh grid for us (we use this to plot the solution), the first parameter is for the x-dimension, and the second parameter is for the y-dimension. We use np.arange() to arrange a 1-D array with element value that starts from some value to some value, in our case, it's from 0 to lenX and from 0 to lenY . Then we set the region: we define 2-D array, define the size and fill the array with guess value, then we set the boundary condition, look at the syntax of filling the array element for boundary condition above here.
Then we are ready to apply our final equation into Python code below. We iterate the equation using for loop.
You should be aware of the indentation of the code above, Python does not use bracket but it uses white space or indentation. Well, the main logic is finished. Next, we write code to plot the solution, using Matplotlib.
That's all, This is the complete code .
It's pretty short, huh ? Okay, you can copy-paste and save the source code, name it findif.py . To execute the Python source code, open your Terminal, and go to the directory where you locate the source code, type:
and press Enter . Then the plot window will appear.

You can try to change the boundary condition's value, for example, you change the value of right edge temperature to 30 degree Celcius ( Tright = 30 ), then the result will look like this:

Points of Interest
Python is an "easy to learn" and dynamically typed programming language, and it provides (open source) powerful library for computational physics or other scientific discipline. Since Python is an interpreted language, it's slow as compared to compiled languages like C or C++, but again, it's easy to learn. We can also write less code and do more with Python, so we don't struggle to program, and we can focus on what we want to solve.
In computational physics, with Numpy and also Scipy (numeric and scientific library for Python), we can solve many complex problems because it provides matrix solver (eigenvalue and eigenvector solver), linear algebra operation, as well as signal processing, Fourier transform, statistics, optimization, etc.
In addition to its use in computational physics, Python is also used in machine learning, even Google's TensorFlow uses Python.
- 21 st March, 2016: Initial version
This article, along with any associated source code and files, is licensed under The Code Project Open License (CPOL)
Comments and Discussions
Use Ctrl+Left/Right to switch messages, Ctrl+Up/Down to switch threads, Ctrl+Shift+Left/Right to switch pages.
Introduction to Computer Science Using Python: A Computational Problem-Solving Focus
- Charles Dierbach
- John Wiley & Sons (US)
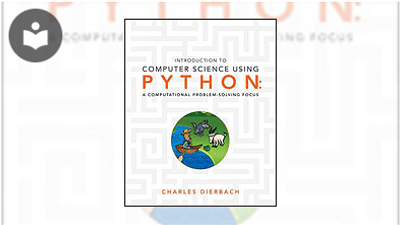
Introduction to Computer Science Using Python: A Computational Problem-Solving Focus introduces readers to programming and computational problem-solving via a back-to-basics, step-by-step, objects-late approach that makes this book easy to teach and learn from. Readers are provided with a thorough conceptual grounding in computational problem solving before introducing them to specific Python syntax, thus giving them the background to become successful programmers in any language. Dierbach also offers readers a thorough grounding in imperative programming before introducing them to object-oriented programming. His step-by-step pedagogical approach makes this an accessible and reader-friendly introduction to programming that eases readers into program-writing through a variety of hands-on exercises.
Charles Dierbach is an Associate Professor of computer science at Towson University, and has regularly taught introductory undergraduate computer science courses for the past thirty-five years. He received his Ph.D. in Computer Science from the University of Delaware. While a lecturer there, he received the Outstanding Teaching Award from the undergraduate chapter of the ACM. At Towson, he served as Director of the Undergraduate Computer Science program for over ten years. In addition to teaching introductory computer science courses, Dr. Dierbach also teaches undergraduate and graduate courses in object-oriented design and programming.
In this Book
- Introduction
- Data and Expressions
- Control Structures
- Objects and Their Use
- Modular Design
- Dictionaries and Sets
- Object-Oriented Programming
- Computing and Its Developments
YOU MIGHT ALSO LIKE
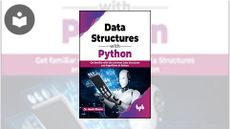
PX1224: Computational Skills for Problem Solving
Computational Physics
Welcome to the home page Computational Physics Courses in the School of Physics and Astronomy at Cardiff University.
This page includes the content for PX1224: Computational Skills for Problem Solving
The course uses the Python programming language and the Jupyter development environment.
Why learn computational skills?
Most real world physics and astronomy problems are difficult to solve exactly using the mathematical techniques that you are learning. The problems which are assigned in classes are specially chosen so that you can solve them with the techniques you have learned. However, most problems cannot be solved in this manner and in general we must either:
- Make some approximations in order to be able to solve the problem. For example, it's quite common to neglect air resistance when solving mechanics problems. There is a real skill in knowing which effects can safely be ignored when seeking approximate solutions.
- Make use of computational methods to solve the problem numerically. This involves taking the problem and implementing the solution on a computer. Again, this will not provide an "exact" solution and care must be taken with assessing the numerical accuracy of the solution.
Computers are also very useful for analyzing experimental data. As a simple example, it is much quicker (and more accurate) to calculate a best fit line, and associated errors, on a computer rather than making the plot by hand. The same is true for more complex experimental data which can only be analyzed on a computer due to both the complexity of the data and the size of the data sets.
Computers can also be used for simulations. Often, it is useful to have an idea of the result you might expect from an experiment before performing it. If you can code up a simulated experiment on a computer, then you can run the experiment many, many times to get a sense of the results that you would expect to obtain.
In the first year course, we will introduce some basic computational techniques, and show how they can be used to solve problems that you’ve seen in other courses and labs. This is expanded upon in the second and third year computing classes.
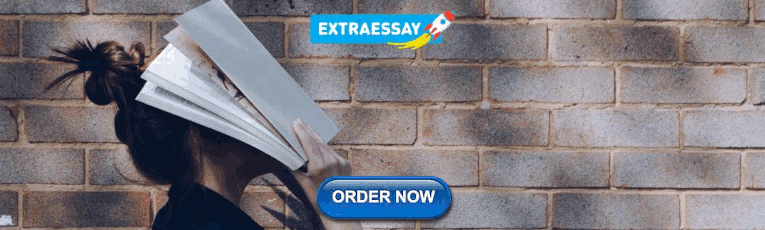
Why Python?
Python is a relatively new language (started in 1990) that has become widely used in recent years. There are several reasons that we have chosen to use the Python programming language in the Cardiff computational physics courses:
- Python is an interactive language. This means that you don't have to compile and then run the code before you see what it does; you can execute each line as you type it. This feature makes it much faster to get going in Python.
- Python is freely available. Python runs on Windows, Mac and Linux (in case you were wondering) and can be freely downloaded and used on any of these systems. This means that you will be able to download and install Python on any PC or laptop that you have access to.
- Gravitational wave astronomy
- Herschel Space Observatory Astronomy
- Nanophysics
- Brain Imaging
- Python is used in the real world. Companies that use Python internally include Google, Yahoo, and Lucasfilm Ltd. For more examples, see Organizations Usign Python . It is also regularly rated as one of the ten most used languages.
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python Exercise with Practice Questions and Solutions
- Python List Exercise
- Python String Exercise
- Python Tuple Exercise
- Python Dictionary Exercise
- Python Set Exercise
Python Matrix Exercises
- Python program to a Sort Matrix by index-value equality count
- Python Program to Reverse Every Kth row in a Matrix
- Python Program to Convert String Matrix Representation to Matrix
- Python - Count the frequency of matrix row length
- Python - Convert Integer Matrix to String Matrix
- Python Program to Convert Tuple Matrix to Tuple List
- Python - Group Elements in Matrix
- Python - Assigning Subsequent Rows to Matrix first row elements
- Adding and Subtracting Matrices in Python
- Python - Convert Matrix to dictionary
- Python - Convert Matrix to Custom Tuple Matrix
- Python - Matrix Row subset
- Python - Group similar elements into Matrix
- Python - Row-wise element Addition in Tuple Matrix
- Create an n x n square matrix, where all the sub-matrix have the sum of opposite corner elements as even
Python Functions Exercises
- Python splitfields() Method
- How to get list of parameters name from a function in Python?
- How to Print Multiple Arguments in Python?
- Python program to find the power of a number using recursion
- Sorting objects of user defined class in Python
- Assign Function to a Variable in Python
- Returning a function from a function - Python
- What are the allowed characters in Python function names?
- Defining a Python function at runtime
- Explicitly define datatype in a Python function
- Functions that accept variable length key value pair as arguments
- How to find the number of arguments in a Python function?
- How to check if a Python variable exists?
- Python - Get Function Signature
- Python program to convert any base to decimal by using int() method
Python Lambda Exercises
- Python - Lambda Function to Check if value is in a List
- Difference between Normal def defined function and Lambda
- Python: Iterating With Python Lambda
- How to use if, else & elif in Python Lambda Functions
- Python - Lambda function to find the smaller value between two elements
- Lambda with if but without else in Python
- Python Lambda with underscore as an argument
- Difference between List comprehension and Lambda in Python
- Nested Lambda Function in Python
- Python lambda
- Python | Sorting string using order defined by another string
- Python | Find fibonacci series upto n using lambda
- Overuse of lambda expressions in Python
- Python program to count Even and Odd numbers in a List
- Intersection of two arrays in Python ( Lambda expression and filter function )
Python Pattern printing Exercises
- Simple Diamond Pattern in Python
- Python - Print Heart Pattern
- Python program to display half diamond pattern of numbers with star border
- Python program to print Pascal's Triangle
- Python program to print the Inverted heart pattern
- Python Program to print hollow half diamond hash pattern
- Program to Print K using Alphabets
- Program to print half Diamond star pattern
- Program to print window pattern
- Python Program to print a number diamond of any given size N in Rangoli Style
- Python program to right rotate n-numbers by 1
- Python Program to print digit pattern
- Print with your own font using Python !!
- Python | Print an Inverted Star Pattern
- Program to print the diamond shape
Python DateTime Exercises
- Python - Iterating through a range of dates
- How to add time onto a DateTime object in Python
- How to add timestamp to excel file in Python
- Convert string to datetime in Python with timezone
- Isoformat to datetime - Python
- Python datetime to integer timestamp
- How to convert a Python datetime.datetime to excel serial date number
- How to create filename containing date or time in Python
- Convert "unknown format" strings to datetime objects in Python
- Extract time from datetime in Python
- Convert Python datetime to epoch
- Python program to convert unix timestamp string to readable date
- Python - Group dates in K ranges
- Python - Divide date range to N equal duration
- Python - Last business day of every month in year
Python OOPS Exercises
- Get index in the list of objects by attribute in Python
- Python program to build flashcard using class in Python
- How to count number of instances of a class in Python?
- Shuffle a deck of card with OOPS in Python
- What is a clean and Pythonic way to have multiple constructors in Python?
- How to Change a Dictionary Into a Class?
- How to create an empty class in Python?
- Student management system in Python
- How to create a list of object in Python class
Python Regex Exercises
- Validate an IP address using Python without using RegEx
- Python program to find the type of IP Address using Regex
- Converting a 10 digit phone number to US format using Regex in Python
- Python program to find Indices of Overlapping Substrings
- Python program to extract Strings between HTML Tags
- Python - Check if String Contain Only Defined Characters using Regex
- How to extract date from Excel file using Pandas?
- Python program to find files having a particular extension using RegEx
- How to check if a string starts with a substring using regex in Python?
- How to Remove repetitive characters from words of the given Pandas DataFrame using Regex?
- Extract punctuation from the specified column of Dataframe using Regex
- Extract IP address from file using Python
- Python program to Count Uppercase, Lowercase, special character and numeric values using Regex
- Categorize Password as Strong or Weak using Regex in Python
- Python - Substituting patterns in text using regex
Python LinkedList Exercises
- Python program to Search an Element in a Circular Linked List
- Implementation of XOR Linked List in Python
- Pretty print Linked List in Python
- Python Library for Linked List
- Python | Stack using Doubly Linked List
- Python | Queue using Doubly Linked List
- Program to reverse a linked list using Stack
- Python program to find middle of a linked list using one traversal
- Python Program to Reverse a linked list
Python Searching Exercises
- Binary Search (bisect) in Python
- Python Program for Linear Search
- Python Program for Anagram Substring Search (Or Search for all permutations)
- Python Program for Binary Search (Recursive and Iterative)
- Python Program for Rabin-Karp Algorithm for Pattern Searching
- Python Program for KMP Algorithm for Pattern Searching
Python Sorting Exercises
- Python Code for time Complexity plot of Heap Sort
- Python Program for Stooge Sort
- Python Program for Recursive Insertion Sort
- Python Program for Cycle Sort
- Bisect Algorithm Functions in Python
- Python Program for BogoSort or Permutation Sort
- Python Program for Odd-Even Sort / Brick Sort
- Python Program for Gnome Sort
- Python Program for Cocktail Sort
- Python Program for Bitonic Sort
- Python Program for Pigeonhole Sort
- Python Program for Comb Sort
- Python Program for Iterative Merge Sort
- Python Program for Binary Insertion Sort
- Python Program for ShellSort
Python DSA Exercises
- Saving a Networkx graph in GEXF format and visualize using Gephi
- Dumping queue into list or array in Python
- Python program to reverse a stack
- Python - Stack and StackSwitcher in GTK+ 3
- Multithreaded Priority Queue in Python
- Python Program to Reverse the Content of a File using Stack
- Priority Queue using Queue and Heapdict module in Python
- Box Blur Algorithm - With Python implementation
- Python program to reverse the content of a file and store it in another file
- Check whether the given string is Palindrome using Stack
- Take input from user and store in .txt file in Python
- Change case of all characters in a .txt file using Python
- Finding Duplicate Files with Python
Python File Handling Exercises
- Python Program to Count Words in Text File
- Python Program to Delete Specific Line from File
- Python Program to Replace Specific Line in File
- Python Program to Print Lines Containing Given String in File
- Python - Loop through files of certain extensions
- Compare two Files line by line in Python
- How to keep old content when Writing to Files in Python?
- How to get size of folder using Python?
- How to read multiple text files from folder in Python?
- Read a CSV into list of lists in Python
- Python - Write dictionary of list to CSV
- Convert nested JSON to CSV in Python
- How to add timestamp to CSV file in Python
Python CSV Exercises
- How to create multiple CSV files from existing CSV file using Pandas ?
- How to read all CSV files in a folder in Pandas?
- How to Sort CSV by multiple columns in Python ?
- Working with large CSV files in Python
- How to convert CSV File to PDF File using Python?
- Visualize data from CSV file in Python
- Python - Read CSV Columns Into List
- Sorting a CSV object by dates in Python
- Python program to extract a single value from JSON response
- Convert class object to JSON in Python
- Convert multiple JSON files to CSV Python
- Convert JSON data Into a Custom Python Object
- Convert CSV to JSON using Python
Python JSON Exercises
- Flattening JSON objects in Python
- Saving Text, JSON, and CSV to a File in Python
- Convert Text file to JSON in Python
- Convert JSON to CSV in Python
- Convert JSON to dictionary in Python
- Python Program to Get the File Name From the File Path
- How to get file creation and modification date or time in Python?
- Menu driven Python program to execute Linux commands
- Menu Driven Python program for opening the required software Application
- Open computer drives like C, D or E using Python
Python OS Module Exercises
- Rename a folder of images using Tkinter
- Kill a Process by name using Python
- Finding the largest file in a directory using Python
- Python - Get list of running processes
- Python - Get file id of windows file
- Python - Get number of characters, words, spaces and lines in a file
- Change current working directory with Python
- How to move Files and Directories in Python
- How to get a new API response in a Tkinter textbox?
- Build GUI Application for Guess Indian State using Tkinter Python
- How to stop copy, paste, and backspace in text widget in tkinter?
- How to temporarily remove a Tkinter widget without using just .place?
- How to open a website in a Tkinter window?
Python Tkinter Exercises
- Create Address Book in Python - Using Tkinter
- Changing the colour of Tkinter Menu Bar
- How to check which Button was clicked in Tkinter ?
- How to add a border color to a button in Tkinter?
- How to Change Tkinter LableFrame Border Color?
- Looping through buttons in Tkinter
- Visualizing Quick Sort using Tkinter in Python
- How to Add padding to a tkinter widget only on one side ?
- Python NumPy - Practice Exercises, Questions, and Solutions
- Pandas Exercises and Programs
- How to get the Daily News using Python
- How to Build Web scraping bot in Python
- Scrape LinkedIn Using Selenium And Beautiful Soup in Python
- Scraping Reddit with Python and BeautifulSoup
- Scraping Indeed Job Data Using Python
Python Web Scraping Exercises
- How to Scrape all PDF files in a Website?
- How to Scrape Multiple Pages of a Website Using Python?
- Quote Guessing Game using Web Scraping in Python
- How to extract youtube data in Python?
- How to Download All Images from a Web Page in Python?
- Test the given page is found or not on the server Using Python
- How to Extract Wikipedia Data in Python?
- How to extract paragraph from a website and save it as a text file?
- Automate Youtube with Python
- Controlling the Web Browser with Python
- How to Build a Simple Auto-Login Bot with Python
- Download Google Image Using Python and Selenium
- How To Automate Google Chrome Using Foxtrot and Python
Python Selenium Exercises
- How to scroll down followers popup in Instagram ?
- How to switch to new window in Selenium for Python?
- Python Selenium - Find element by text
- How to scrape multiple pages using Selenium in Python?
- Python Selenium - Find Button by text
- Web Scraping Tables with Selenium and Python
- Selenium - Search for text on page
- Python Projects - Beginner to Advanced
Python Exercise: Practice makes you perfect in everything. This proverb always proves itself correct. Just like this, if you are a Python learner, then regular practice of Python exercises makes you more confident and sharpens your skills. So, to test your skills, go through these Python exercises with solutions.
Python is a widely used general-purpose high-level language that can be used for many purposes like creating GUI, web Scraping, web development, etc. You might have seen various Python tutorials that explain the concepts in detail but that might not be enough to get hold of this language. The best way to learn is by practising it more and more.
The best thing about this Python practice exercise is that it helps you learn Python using sets of detailed programming questions from basic to advanced. It covers questions on core Python concepts as well as applications of Python in various domains. So if you are at any stage like beginner, intermediate or advanced this Python practice set will help you to boost your programming skills in Python.
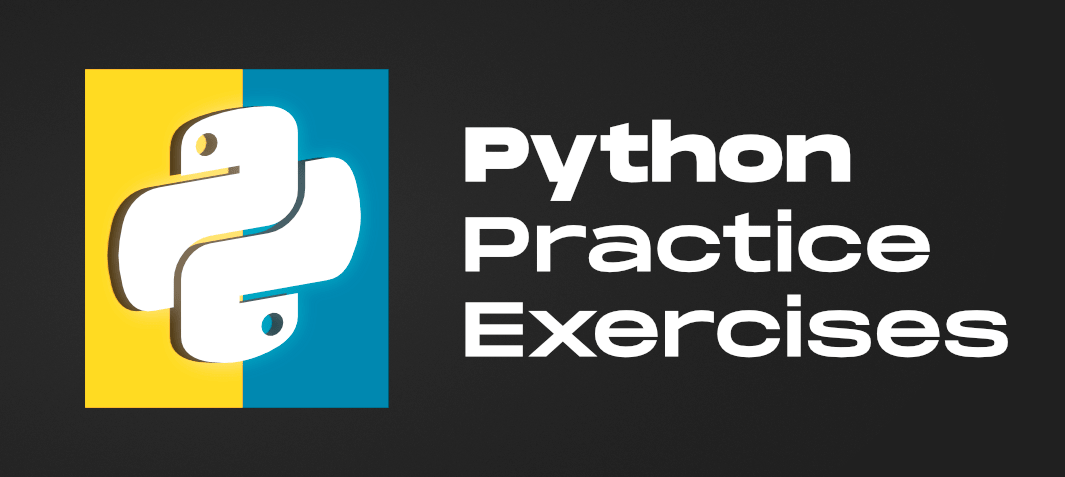
List of Python Programming Exercises
In the below section, we have gathered chapter-wise Python exercises with solutions. So, scroll down to the relevant topics and try to solve the Python program practice set.
Python List Exercises
- Python program to interchange first and last elements in a list
- Python program to swap two elements in a list
- Python | Ways to find length of list
- Maximum of two numbers in Python
- Minimum of two numbers in Python
>> More Programs on List
Python String Exercises
- Python program to check whether the string is Symmetrical or Palindrome
- Reverse words in a given String in Python
- Ways to remove i’th character from string in Python
- Find length of a string in python (4 ways)
- Python program to print even length words in a string
>> More Programs on String
Python Tuple Exercises
- Python program to Find the size of a Tuple
- Python – Maximum and Minimum K elements in Tuple
- Python – Sum of tuple elements
- Python – Row-wise element Addition in Tuple Matrix
- Create a list of tuples from given list having number and its cube in each tuple
>> More Programs on Tuple
Python Dictionary Exercises
- Python | Sort Python Dictionaries by Key or Value
- Handling missing keys in Python dictionaries
- Python dictionary with keys having multiple inputs
- Python program to find the sum of all items in a dictionary
- Python program to find the size of a Dictionary
>> More Programs on Dictionary
Python Set Exercises
- Find the size of a Set in Python
- Iterate over a set in Python
- Python – Maximum and Minimum in a Set
- Python – Remove items from Set
- Python – Check if two lists have atleast one element common
>> More Programs on Sets
- Python – Assigning Subsequent Rows to Matrix first row elements
- Python – Group similar elements into Matrix
>> More Programs on Matrices
>> More Programs on Functions
- Python | Find the Number Occurring Odd Number of Times using Lambda expression and reduce function
>> More Programs on Lambda
- Programs for printing pyramid patterns in Python
>> More Programs on Python Pattern Printing
- Python program to get Current Time
- Get Yesterday’s date using Python
- Python program to print current year, month and day
- Python – Convert day number to date in particular year
- Get Current Time in different Timezone using Python
>> More Programs on DateTime
>> More Programs on Python OOPS
- Python – Check if String Contain Only Defined Characters using Regex
>> More Programs on Python Regex
>> More Programs on Linked Lists
>> More Programs on Python Searching
- Python Program for Bubble Sort
- Python Program for QuickSort
- Python Program for Insertion Sort
- Python Program for Selection Sort
- Python Program for Heap Sort
>> More Programs on Python Sorting
- Program to Calculate the Edge Cover of a Graph
- Python Program for N Queen Problem
>> More Programs on Python DSA
- Read content from one file and write it into another file
- Write a dictionary to a file in Python
- How to check file size in Python?
- Find the most repeated word in a text file
- How to read specific lines from a File in Python?
>> More Programs on Python File Handling
- Update column value of CSV in Python
- How to add a header to a CSV file in Python?
- Get column names from CSV using Python
- Writing data from a Python List to CSV row-wise
>> More Programs on Python CSV
>> More Programs on Python JSON
- Python Script to change name of a file to its timestamp
>> More Programs on OS Module
- Python | Create a GUI Marksheet using Tkinter
- Python | ToDo GUI Application using Tkinter
- Python | GUI Calendar using Tkinter
- File Explorer in Python using Tkinter
- Visiting Card Scanner GUI Application using Python
>> More Programs on Python Tkinter
NumPy Exercises
- How to create an empty and a full NumPy array?
- Create a Numpy array filled with all zeros
- Create a Numpy array filled with all ones
- Replace NumPy array elements that doesn’t satisfy the given condition
- Get the maximum value from given matrix
>> More Programs on NumPy
Pandas Exercises
- Make a Pandas DataFrame with two-dimensional list | Python
- How to iterate over rows in Pandas Dataframe
- Create a pandas column using for loop
- Create a Pandas Series from array
- Pandas | Basic of Time Series Manipulation
>> More Programs on Python Pandas
>> More Programs on Web Scraping
- Download File in Selenium Using Python
- Bulk Posting on Facebook Pages using Selenium
- Google Maps Selenium automation using Python
- Count total number of Links In Webpage Using Selenium In Python
- Extract Data From JustDial using Selenium
>> More Programs on Python Selenium
- Number guessing game in Python
- 2048 Game in Python
- Get Live Weather Desktop Notifications Using Python
- 8-bit game using pygame
- Tic Tac Toe GUI In Python using PyGame
>> More Projects in Python
In closing, we just want to say that the practice or solving Python problems always helps to clear your core concepts and programming logic. Hence, we have designed this Python exercises after deep research so that one can easily enhance their skills and logic abilities.
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?

- Computers & Technology
- Computer Science

Enjoy fast, free delivery, exclusive deals, and award-winning movies & TV shows with Prime Try Prime and start saving today with fast, free delivery
Amazon Prime includes:
Fast, FREE Delivery is available to Prime members. To join, select "Try Amazon Prime and start saving today with Fast, FREE Delivery" below the Add to Cart button.
- Cardmembers earn 5% Back at Amazon.com with a Prime Credit Card.
- Unlimited Free Two-Day Delivery
- Streaming of thousands of movies and TV shows with limited ads on Prime Video.
- A Kindle book to borrow for free each month - with no due dates
- Listen to over 2 million songs and hundreds of playlists
- Unlimited photo storage with anywhere access
Important: Your credit card will NOT be charged when you start your free trial or if you cancel during the trial period. If you're happy with Amazon Prime, do nothing. At the end of the free trial, your membership will automatically upgrade to a monthly membership.
Return this item for free
Free returns are available for the shipping address you chose. You can return the item for any reason in new and unused condition: no shipping charges
- Go to your orders and start the return
- Select the return method

Download the free Kindle app and start reading Kindle books instantly on your smartphone, tablet, or computer - no Kindle device required .
Read instantly on your browser with Kindle for Web.
Using your mobile phone camera - scan the code below and download the Kindle app.

Image Unavailable

- To view this video download Flash Player
Computational Physics: Problem Solving with Python 4th Edition
Purchase options and add-ons.
- An exceptionally broad range of topics, from simple matrix manipulations to intricate computations in nonlinear dynamics
- A whole suite of supplementary material: Python programs, Jupyter notebooks and videos
Computational Physics is ideal for students in physics, engineering, materials science, and any subjects drawing on applied physics.
- ISBN-10 3527414258
- ISBN-13 978-3527414253
- Edition 4th
- Publisher Wiley-VCH
- Publication date March 25, 2024
- Language English
- Dimensions 6.69 x 1.2 x 9.61 inches
- Print length 592 pages
- See all details

Customers who viewed this item also viewed

Editorial Reviews
From the back cover, about the author.
Cristian C. Bordeianu, PhD, taught Physics and Computer Science at the Military College “Stefan cel Mare,” Campulung Moldovenesc, Romania.
Product details
- Publisher : Wiley-VCH; 4th edition (March 25, 2024)
- Language : English
- Paperback : 592 pages
- ISBN-10 : 3527414258
- ISBN-13 : 978-3527414253
- Item Weight : 2.44 pounds
- Dimensions : 6.69 x 1.2 x 9.61 inches
- #124 in Fractal Mathematics
- #482 in Mathematical Physics (Books)
- #1,666 in Artificial Intelligence & Semantics
Customer reviews
Customer Reviews, including Product Star Ratings help customers to learn more about the product and decide whether it is the right product for them.
To calculate the overall star rating and percentage breakdown by star, we don’t use a simple average. Instead, our system considers things like how recent a review is and if the reviewer bought the item on Amazon. It also analyzed reviews to verify trustworthiness.
No customer reviews
- Amazon Newsletter
- About Amazon
- Accessibility
- Sustainability
- Press Center
- Investor Relations
- Amazon Devices
- Amazon Science
- Sell on Amazon
- Sell apps on Amazon
- Supply to Amazon
- Protect & Build Your Brand
- Become an Affiliate
- Become a Delivery Driver
- Start a Package Delivery Business
- Advertise Your Products
- Self-Publish with Us
- Become an Amazon Hub Partner
- › See More Ways to Make Money
- Amazon Visa
- Amazon Store Card
- Amazon Secured Card
- Amazon Business Card
- Shop with Points
- Credit Card Marketplace
- Reload Your Balance
- Amazon Currency Converter
- Your Account
- Your Orders
- Shipping Rates & Policies
- Amazon Prime
- Returns & Replacements
- Manage Your Content and Devices
- Recalls and Product Safety Alerts
- Conditions of Use
- Privacy Notice
- Consumer Health Data Privacy Disclosure
- Your Ads Privacy Choices
Academia.edu no longer supports Internet Explorer.
To browse Academia.edu and the wider internet faster and more securely, please take a few seconds to upgrade your browser .
Enter the email address you signed up with and we'll email you a reset link.
- We're Hiring!
- Help Center
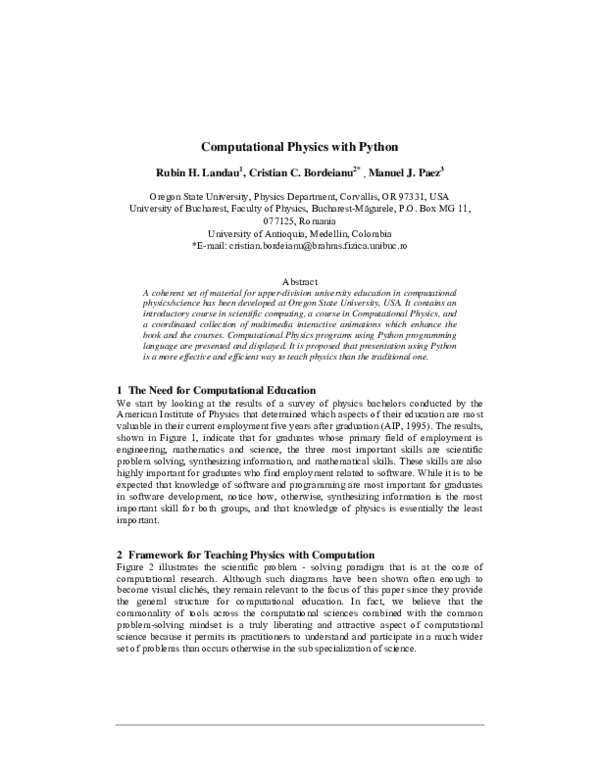
Computational Physics with Python

RELATED TOPICS
- We're Hiring!
- Help Center
- Find new research papers in:
- Health Sciences
- Earth Sciences
- Cognitive Science
- Mathematics
- Computer Science
- Academia ©2024
Thank you for visiting nature.com. You are using a browser version with limited support for CSS. To obtain the best experience, we recommend you use a more up to date browser (or turn off compatibility mode in Internet Explorer). In the meantime, to ensure continued support, we are displaying the site without styles and JavaScript.
- View all journals
- Explore content
- About the journal
- Publish with us
- Sign up for alerts
- Review Article
- Published: 16 May 2024
A guide to artificial intelligence for cancer researchers
- Raquel Perez-Lopez ORCID: orcid.org/0000-0002-9176-0130 1 ,
- Narmin Ghaffari Laleh ORCID: orcid.org/0000-0003-0889-3352 2 ,
- Faisal Mahmood ORCID: orcid.org/0000-0001-7587-1562 3 , 4 , 5 , 6 , 7 , 8 &
- Jakob Nikolas Kather ORCID: orcid.org/0000-0002-3730-5348 2 , 9 , 10
Nature Reviews Cancer ( 2024 ) Cite this article
1363 Accesses
120 Altmetric
Metrics details
- Cancer imaging
- Mathematics and computing
- Tumour biomarkers
Artificial intelligence (AI) has been commoditized. It has evolved from a specialty resource to a readily accessible tool for cancer researchers. AI-based tools can boost research productivity in daily workflows, but can also extract hidden information from existing data, thereby enabling new scientific discoveries. Building a basic literacy in these tools is useful for every cancer researcher. Researchers with a traditional biological science focus can use AI-based tools through off-the-shelf software, whereas those who are more computationally inclined can develop their own AI-based software pipelines. In this article, we provide a practical guide for non-computational cancer researchers to understand how AI-based tools can benefit them. We convey general principles of AI for applications in image analysis, natural language processing and drug discovery. In addition, we give examples of how non-computational researchers can get started on the journey to productively use AI in their own work.
This is a preview of subscription content, access via your institution
Access options
Access Nature and 54 other Nature Portfolio journals
Get Nature+, our best-value online-access subscription
24,99 € / 30 days
cancel any time
Subscribe to this journal
Receive 12 print issues and online access
195,33 € per year
only 16,28 € per issue
Buy this article
- Purchase on Springer Link
- Instant access to full article PDF
Prices may be subject to local taxes which are calculated during checkout
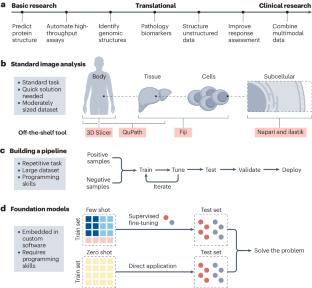
Similar content being viewed by others
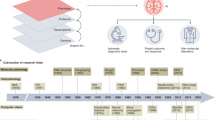
Artificial intelligence in histopathology: enhancing cancer research and clinical oncology
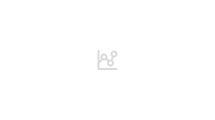
Guiding principles for the responsible development of artificial intelligence tools for healthcare
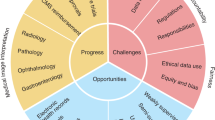
AI in health and medicine
Jiang, T., Gradus, J. L. & Rosellini, A. J. Supervised machine learning: a brief primer. Behav. Ther. 51 , 675–687 (2020).
Article PubMed PubMed Central Google Scholar
Alloghani, M., Al-Jumeily, D., Mustafina, J., Hussain, A. & Aljaaf, A. J. in Supervised and Unsupervised Learning for Data Science (eds Berry, M. W. et al.) 3–21 (Springer International, 2020).
Yala, A. et al. Optimizing risk-based breast cancer screening policies with reinforcement learning. Nat. Med. 28 , 136–143 (2022).
Article CAS PubMed Google Scholar
Kaufmann, E. et al. Champion-level drone racing using deep reinforcement learning. Nature 620 , 982–987 (2023).
Article CAS PubMed PubMed Central Google Scholar
Nasteski, V. An overview of the supervised machine learning methods. Horizons 4 , 51–62 (2017).
Article Google Scholar
Dike, H. U., Zhou, Y., Deveerasetty, K. K. & Wu, Q. Unsupervised learning based on artificial neural network: a review. In 2 018 IEEE International Conference on Cyborg and Bionic Systems (CBS) 322–327 (2018).
Shurrab, S. & Duwairi, R. Self-supervised learning methods and applications in medical imaging analysis: a survey. PeerJ Comput. Sci. 8 , e1045 (2022).
Wang, X. et al. Transformer-based unsupervised contrastive learning for histopathological image classification. Med. Image Anal. 81 , 102559 (2022).
Article PubMed Google Scholar
Wang, X. et al. RetCCL: clustering-guided contrastive learning for whole-slide image retrieval. Med. Image Anal. 83 , 102645 (2023).
Vinyals, O. et al. Grandmaster level in StarCraft II using multi-agent reinforcement learning. Nature 575 , 350–354 (2019).
Zhao, Y., Kosorok, M. R. & Zeng, D. Reinforcement learning design for cancer clinical trials. Stat. Med. 28 , 3294–3315 (2009).
Sapsford, R. & Jupp, V. Data Collection and Analysis (SAGE, 2006).
Yamashita, R., Nishio, M., Do, R. K. G. & Togashi, K. Convolutional neural networks: an overview and application in radiology. Insights Imaging 9 , 611–629 (2018).
Chowdhary, K. R. in Fundamentals of Artificial Intelligence (ed. Chowdhary, K. R.) 603–649 (Springer India, 2020).
Hochreiter, S. & Schmidhuber, J. Long short-term memory. Neural Comput. 9 , 1735–1780 (1997).
Vaswani, A. et al. Attention is all you need. Preprint at https://doi.org/10.48550/arXiv.1706.03762 (2017).
Shmatko, A., Ghaffari Laleh, N., Gerstung, M. & Kather, J. N. Artificial intelligence in histopathology: enhancing cancer research and clinical oncology. Nat. Cancer 3 , 1026–1038 (2022).
Wagner, S. J. et al. Transformer-based biomarker prediction from colorectal cancer histology: a large-scale multicentric study. Cancer Cell 41 , 1650–1661.e4 (2023).
Khan, A. et al. A survey of the vision transformers and their CNN-transformer based variants. Artif. Intell. Rev. 56 , 2917–2970 (2023).
Hamm, C. A. et al. Deep learning for liver tumor diagnosis part I: development of a convolutional neural network classifier for multi-phasic MRI. Eur. Radiol. 29 , 3338–3347 (2019).
Ren, J., Eriksen, J. G., Nijkamp, J. & Korreman, S. S. Comparing different CT, PET and MRI multi-modality image combinations for deep learning-based head and neck tumor segmentation. Acta Oncol. 60 , 1399–1406 (2021).
Unger, M. & Kather, J. N. A systematic analysis of deep learning in genomics and histopathology for precision oncology. BMC Med. Genomics 17 , 48 (2024).
Gawehn, E., Hiss, J. A. & Schneider, G. Deep learning in drug discovery. Mol. Inform. 35 , 3–14 (2016).
Bayramoglu, N., Kannala, J. & Heikkilä, J. Deep learning for magnification independent breast cancer histopathology image classification. In 2016 23rd International Conference on Pattern Recognition (ICPR) 2440–2445 (IEEE, 2016).
Galon, J. et al. Type, density, and location of immune cells within human colorectal tumors predict clinical outcome. Science 313 , 1960–1964 (2006).
Schmidt, U., Weigert, M., Broaddus, C. & Myers, G. Cell detection with star-convex polygons. In Medical Image Computing and Computer Assisted Intervention — MICCAI 2018. Lecture Notes in Computer Science Vol. 11071 (eds Frangi, A. et al.) https://doi.org/10.1007/978-3-030-00934-2_30 (Springer, 2018).
Edlund, C. et al. LIVECell—a large-scale dataset for label-free live cell segmentation. Nat. Methods 18 , 1038–1045 (2021).
Bankhead, P. et al. QuPath: open source software for digital pathology image analysis. Sci. Rep. 7 , 16878 (2017).
Schneider, C. A., Rasband, W. S. & Eliceiri, K. W. NIH image to imageJ: 25 years of image analysis. Nat. Methods 9 , 671–675 (2012).
Rueden, C. T. et al. ImageJ2: ImageJ for the next generation of scientific image data. BMC Bioinformatics 18 , 529 (2017).
Schindelin, J. et al. Fiji: an open-source platform for biological-image analysis. Nat. Methods 9 , 676–682 (2012).
Linkert, M. et al. Metadata matters: access to image data in the real world. J. Cell Biol. 189 , 777–782 (2010).
Gómez-de-Mariscal, E. et al. DeepImageJ: a user-friendly environment to run deep learning models in ImageJ. Nat. Methods 18 , 1192–1195 (2021).
Betge, J. et al. The drug-induced phenotypic landscape of colorectal cancer organoids. Nat. Commun. 13 , 3135 (2022).
Park, T. et al. Development of a deep learning based image processing tool for enhanced organoid analysis. Sci. Rep. 13 , 19841 (2023).
Belthangady, C. & Royer, L. A. Applications, promises, and pitfalls of deep learning for fluorescence image reconstruction. Nat. Methods 16 , 1215–1225 (2019).
Echle, A. et al. Deep learning in cancer pathology: a new generation of clinical biomarkers. Br. J. Cancer 124 , 686–696 (2021).
Cifci, D., Foersch, S. & Kather, J. N. Artificial intelligence to identify genetic alterations in conventional histopathology. J. Pathol. 257 , 430–444 (2022).
Greenson, J. K. et al. Pathologic predictors of microsatellite instability in colorectal cancer. Am. J. Surg. Pathol. 33 , 126–133 (2009).
Kather, J. N. et al. Deep learning can predict microsatellite instability directly from histology in gastrointestinal cancer. Nat. Med. 25 , 1054–1056 (2019).
Echle, A. et al. Clinical-grade detection of microsatellite instability in colorectal tumors by deep learning. Gastroenterology 159 , 1406–1416.e11 (2020).
Kather, J. N. et al. Pan-cancer image-based detection of clinically actionable genetic alterations. Nat. Cancer 1 , 789–799 (2020).
Fu, Y. et al. Pan-cancer computational histopathology reveals mutations, tumor composition and prognosis. Nat. Cancer 1 , 800–810 (2020).
Coudray, N. et al. Classification and mutation prediction from non-small cell lung cancer histopathology images using deep learning. Nat. Med. 24 , 1559–1567 (2018).
Schmauch, B. et al. A deep learning model to predict RNA-seq expression of tumours from whole slide images. Nat. Commun. 11 , 3877 (2020).
Binder, A. et al. Morphological and molecular breast cancer profiling through explainable machine learning. Nat. Mach. Intell. 3 , 355–366 (2021).
Loeffler, C. M. L. et al. Predicting mutational status of driver and suppressor genes directly from histopathology with deep learning: a systematic study across 23 solid tumor types. Front. Genet. 12 , 806386 (2022).
Chen, R. J. et al. Pan-cancer integrative histology-genomic analysis via multimodal deep learning. Cancer Cell 40 , 865–878.e6 (2022).
Bilal, M. et al. Development and validation of a weakly supervised deep learning framework to predict the status of molecular pathways and key mutations in colorectal cancer from routine histology images: a retrospective study. Lancet Digit. Health 3 , e763–e772 (2021).
Yamashita, R. et al. Deep learning model for the prediction of microsatellite instability in colorectal cancer: a diagnostic study. Lancet Oncol. 22 , 132–141 (2021).
Echle, A. et al. Artificial intelligence for detection of microsatellite instability in colorectal cancer—a multicentric analysis of a pre-screening tool for clinical application. ESMO Open 7 , 100400 (2022).
Schirris, Y., Gavves, E., Nederlof, I., Horlings, H. M. & Teuwen, J. DeepSMILE: contrastive self-supervised pre-training benefits MSI and HRD classification directly from H&E whole-slide images in colorectal and breast cancer. Med. Image Anal. 79 , 102464 (2022).
Jain, M. S. & Massoud, T. F. Predicting tumour mutational burden from histopathological images using multiscale deep learning. Nat. Mach. Intell . 2 , 356–362 (2020).
Xu, H. et al. Spatial heterogeneity and organization of tumor mutation burden with immune infiltrates within tumors based on whole slide images correlated with patient survival in bladder cancer. J. Pathol. Inform. 13 , 100105 (2022).
Chen, S. et al. Deep learning-based approach to reveal tumor mutational burden status from whole slide images across multiple cancer types. Preprint at https://doi.org/10.48550/arXiv.2204.03257 (2023).
Shamai, G. et al. Artificial intelligence algorithms to assess hormonal status from tissue microarrays in patients with breast cancer. JAMA Netw. Open 2 , e197700 (2019).
Beck, A. H. et al. Systematic analysis of breast cancer morphology uncovers stromal features associated with survival. Sci. Transl. Med. 3 , 108ra113 (2011).
Arslan, S. et al. A systematic pan-cancer study on deep learning-based prediction of multi-omic biomarkers from routine pathology images. Commun. Med. 4 , 48 (2024).
Campanella, G. et al. Clinical-grade computational pathology using weakly supervised deep learning on whole slide images. Nat. Med. 25 , 1301–1309 (2019).
Lu, M. Y. et al. AI-based pathology predicts origins for cancers of unknown primary. Nature 594 , 106–110 (2021).
Kleppe, A. et al. A clinical decision support system optimising adjuvant chemotherapy for colorectal cancers by integrating deep learning and pathological staging markers: a development and validation study. Lancet Oncol. 23 , 1221–1232 (2022).
Jiang, X. et al. End-to-end prognostication in colorectal cancer by deep learning: a retrospective, multicentre study. Lancet Digit. Health 6 , e33–e43 (2024).
Zeng, Q. et al. Artificial intelligence-based pathology as a biomarker of sensitivity to atezolizumab–bevacizumab in patients with hepatocellular carcinoma: a multicentre retrospective study. Lancet Oncol. 24 , 1411–1422 (2023).
Ghaffari Laleh, N., Ligero, M., Perez-Lopez, R. & Kather, J. N. Facts and hopes on the use of artificial intelligence for predictive immunotherapy biomarkers in cancer. Clin. Cancer Res. 29 , 316–323 (2022).
Pedersen, A. et al. FastPathology: an open-source platform for deep learning-based research and decision support in digital pathology. IEEE Access 9 , 58216–58229 (2021).
Pocock, J. et al. TIAToolbox as an end-to-end library for advanced tissue image analytics. Commun. Med. 2 , 120 (2022).
Lu, M. Y. et al. Data-efficient and weakly supervised computational pathology on whole-slide images. Nat. Biomed. Eng. 5 , 555–570 (2021).
El Nahhas, O. S. M. et al. From whole-slide image to biomarker prediction: a protocol for end-to-end deep learning in computational pathology. Preprint at https://doi.org/10.48550/arXiv.2312.10944 (2023).
Paszke, A. et al. PyTorch: an imperative style, high-performance deep learning library. Preprint at https://doi.org/10.48550/arXiv.1912.01703 (2019).
Jorge Cardoso, M. et al. MONAI: an open-source framework for deep learning in healthcare. Preprint at https://doi.org/10.48550/arXiv.2211.02701 (2022).
Goode, A., Gilbert, B., Harkes, J., Jukic, D. & Satyanarayanan, M. OpenSlide: a vendor-neutral software foundation for digital pathology. J. Pathol. Inform. 4 , 27 (2013).
Martinez, K. & Cupitt, J. VIPS—a highly tuned image processing software architecture. In IEEE Int.Conf. Image Processing 2005 ; https://doi.org/10.1109/icip.2005.1530120 (2005).
Dolezal, J. M. et al. Deep learning generates synthetic cancer histology for explainability and education. NPJ Precis. Oncol. 7 , 49 (2023).
Plass, M. et al. Explainability and causability in digital pathology. Hip Int. 9 , 251–260 (2023).
Google Scholar
Reis-Filho, J. S. & Kather, J. N. Overcoming the challenges to implementation of artificial intelligence in pathology. J. Natl Cancer Inst. 115 , 608–612 (2023).
Aggarwal, R. et al. Diagnostic accuracy of deep learning in medical imaging: a systematic review and meta-analysis. NPJ Digit. Med. 4 , 65 (2021).
Rajput, D., Wang, W.-J. & Chen, C.-C. Evaluation of a decided sample size in machine learning applications. BMC Bioinformatics 24 , 48 (2023).
Ligero, M. et al. Minimizing acquisition-related radiomics variability by image resampling and batch effect correction to allow for large-scale data analysis. Eur. Radiol. 31 , 1460–1470 (2021).
Zwanenburg, A. et al. The image biomarker standardization initiative: standardized quantitative radiomics for high-throughput image-based phenotyping. Radiology 295 , 328–338 (2020).
van Griethuysen, J. J. M. et al. Computational radiomics system to decode the radiographic phenotype. Cancer Res. 77 , e104–e107 (2017).
Fedorov, A. et al. 3D Slicer as an image computing platform for the quantitative imaging network. Magn. Reson. Imaging 30 , 1323–1341 (2012).
Yushkevich, P. A. et al. User-guided 3D active contour segmentation of anatomical structures: significantly improved efficiency and reliability. Neuroimage 31 , 1116–1128 (2006).
Khader, F. et al. Multimodal deep learning for integrating chest radiographs and clinical parameters: a case for transformers. Radiology 309 , e230806 (2023).
Yu, A. C., Mohajer, B. & Eng, J. External validation of deep learning algorithms for radiologic diagnosis: a systematic review. Radiol. Artif. Intell. 4 , e210064 (2022).
US FDA. Artificial intelligence and machine learning (AI/ML)-enabled medical devices; https://www.fda.gov/medical-devices/software-medical-device-samd/artificial-intelligence-and-machine-learning-aiml-enabled-medical-devices (2023).
Bruker Corporation. Artificial intelligence in NMR; https://www.bruker.com/en/landingpages/bbio/artificial-intelligence-in-nmr.html (2024).
Wasserthal, J. TotalSegmentator: tool for robust segmentation of 104 important anatomical structures in CT images. GitHub https://doi.org/10.5281/zenodo.6802613 (2023).
Garcia-Ruiz, A. et al. An accessible deep learning tool for voxel-wise classification of brain malignancies from perfusion MRI. Cell Rep. Med. 5 , 101464 (2024).
Lång, K. et al. Artificial intelligence-supported screen reading versus standard double reading in the Mammography Screening with Artificial Intelligence trial (MASAI): a clinical safety analysis of a randomised, controlled, non-inferiority, single-blinded, screening accuracy study. Lancet Oncol. 24 , 936–944 (2023).
Bera, K., Braman, N., Gupta, A., Velcheti, V. & Madabhushi, A. Predicting cancer outcomes with radiomics and artificial intelligence in radiology. Nat. Rev. Clin. Oncol. 19 , 132–146 (2022).
Núñez, L. M. et al. Unraveling response to temozolomide in preclinical GL261 glioblastoma with MRI/MRSI using radiomics and signal source extraction. Sci. Rep. 10 , 19699 (2020).
Müller, J. et al. Radiomics-based tumor phenotype determination based on medical imaging and tumor microenvironment in a preclinical setting. Radiother. Oncol. 169 , 96–104 (2022).
Amirrashedi, M. et al. Leveraging deep neural networks to improve numerical and perceptual image quality in low-dose preclinical PET imaging. Comput. Med. Imaging Graph. 94 , 102010 (2021).
Zinn, P. O. et al. A coclinical radiogenomic validation study: conserved magnetic resonance radiomic appearance of periostin-expressing glioblastoma in patients and xenograft models. Clin. Cancer Res. 24 , 6288–6299 (2018).
Lin, Y.-C. et al. Diffusion radiomics analysis of intratumoral heterogeneity in a murine prostate cancer model following radiotherapy: pixelwise correlation with histology. J. Magn. Reson. Imaging 46 , 483–489 (2017).
Moor, M. et al. Foundation models for generalist medical artificial intelligence. Nature 616 , 259–265 (2023).
Chen, R. J. et al. Towards a general-purpose foundation model for computational pathology. Nat. Med. 30 , 850–862 (2024).
Unger, M. & Kather, J. N. Deep learning in cancer genomics and histopathology. Genome Med. 16 , 44 (2024).
Zhou, Y. et al. A foundation model for generalizable disease detection from retinal images. Nature 622 , 156–163 (2023).
Filiot, A. et al. Scaling self-supervised learning for histopathology with masked image modeling. Preprint at bioRxiv https://doi.org/10.1101/2023.07.21.23292757 (2023).
Campanella, G. et al. Computational pathology at health system scale—self-supervised foundation models from three billion images. Preprint at https://doi.org/10.48550/arXiv.2310.07033 (2023).
Vorontsov, E. et al. Virchow: a million-slide digital pathology foundation model. Preprint at https://doi.org/10.48550/arXiv.2309.07778 (2023).
Clusmann, J. et al. The future landscape of large language models in medicine. Commun. Med. 3 , 141 (2023).
Bubeck, S. et al. Sparks of artificial general intelligence: early experiments with GPT-4. Preprint at https://doi.org/10.48550/arXiv.2303.12712 (2023).
Truhn, D., Reis-Filho, J. S. & Kather, J. N. Large language models should be used as scientific reasoning engines, not knowledge databases. Nat. Med. 29 , 2983–2984 (2023).
Adams, L. C. et al. Leveraging GPT-4 for post hoc transformation of free-text radiology reports into structured reporting: a multilingual feasibility study. Radiology 307 , e230725 (2023).
Truhn, D. et al. Extracting structured information from unstructured histopathology reports using generative pre-trained transformer 4 (GPT-4). J. Pathol. 262 , 310–319 (2023).
Wiest, I. C. et al. From text to tables: a local privacy preserving large language model for structured information retrieval from medical documents. Preprint at bioRxiv https://doi.org/10.1101/2023.12.07.23299648 (2023).
Singhal, K. et al. Large language models encode clinical knowledge. Nature 620 , 172–180 (2023).
Truhn, D. et al. A pilot study on the efficacy of GPT-4 in providing orthopedic treatment recommendations from MRI reports. Sci. Rep. 13 , 20159 (2023).
Wang, H. et al. Scientific discovery in the age of artificial intelligence. Nature 620 , 47–60 (2023).
Derraz, B. et al. New regulatory thinking is needed for AI-based personalised drug and cell therapies in precision oncology. NPJ Precis. Oncol . https://doi.org/10.1038/s41698-024-00517-w (2024).
Extance, A. ChatGPT has entered the classroom: how LLMs could transform education. Nature 623 , 474–477 (2023).
Thirunavukarasu, A. J. et al. Large language models in medicine. Nat. Med. 29 , 1930–1940 (2023).
Webster, P. Six ways large language models are changing healthcare. Nat. Med. 29 , 2969–2971 (2023).
Krishnan, R., Rajpurkar, P. & Topol, E. J. Self-supervised learning in medicine and healthcare. Nat. Biomed. Eng. 6 , 1346–1352 (2022).
Meskó, B. Prompt engineering as an important emerging skill for medical professionals: tutorial. J. Med. Internet Res. 25 , e50638 (2023).
Sushil, M. et al. CORAL: expert-curated oncology reports to advance language model inference. NEJM AI 1 , 4 (2024).
Brown, T. B. et al. Language models are few-shot learners. Preprint at https://doi.org/10.48550/arXiv.2005.01416 (2020).
Ferber, D. & Kather, J. N. Large language models in uro-oncology. Eur. Urol. Oncol. 7 , 157–159 (2023).
Jiang, L. Y. et al. Health system-scale language models are all-purpose prediction engines. Nature 619 , 357–362 (2023).
Nori, H. et al. Can generalist foundation models outcompete special-purpose tuning? Case study in medicine. Preprint at https://doi.org/10.48550/arXiv.2311.16452 (2023).
Balaguer, A. et al. RAG vs fine-tuning: pipelines, tradeoffs, and a case study on agriculture. Preprint at https://doi.org/10.48550/arXiv.2401.08406 (2024).
Gemini Team et al. Gemini: a family of highly capable multimodal models. Preprint at https://doi.org/10.48550/arXiv.2312.11805 (2023).
Tisman, G. & Seetharam, R. OpenAI’s ChatGPT-4, BARD and YOU.Com (AI) and the cancer patient, for now, caveat emptor, but stay tuned. Digit. Med. Healthc. Technol. https://doi.org/10.5772/dmht.19 (2023).
Touvron, H. et al. LLaMA: open and efficient foundation language models. Preprint at https://doi.org/10.48550/arXiv.2302.13971 (2023).
Lipkova, J. et al. Artificial intelligence for multimodal data integration in oncology. Cancer Cell 40 , 1095–1110 (2022).
Niehues, J. M. et al. Generalizable biomarker prediction from cancer pathology slides with self-supervised deep learning: a retrospective multi-centric study. Cell Rep. Med. 4 , 100980 (2023).
Foersch, S. et al. Multistain deep learning for prediction of prognosis and therapy response in colorectal cancer. Nat. Med. 29 , 430–439 (2023).
Boehm, K. M. et al. Multimodal data integration using machine learning improves risk stratification of high-grade serous ovarian cancer. Nat. Cancer 3 , 723–733 (2022).
Vanguri, R. et al. Multimodal integration of radiology, pathology and genomics for prediction of response to PD-(L)1 blockade in patients with non-small cell lung cancer. Nat. Cancer 3 , 1151–1164 (2022).
Shifai, N., van Doorn, R., Malvehy, J. & Sangers, T. E. Can ChatGPT vision diagnose melanoma? An exploratory diagnostic accuracy study. J. Am. Acad. Dermatol . 90 , 1057–1059 (2024).
Liu, H., Li, C., Wu, Q. & Lee, Y. J. Visual instruction tuning. Preprint at https://doi.org/10.48550/arXiv.2304.08485 (2023).
Li, C. et al. LLaVA-med: training a large language-and-vision assistant for biomedicine in one day. Preprint at https://doi.org/10.48550/arXiv.2306.00890 (2023).
Lu, M. Y. et al. A foundational multimodal vision language AI assistant for human pathology. Preprint at https://doi.org/10.48550/arXiv.2312.07814 (2023).
Adalsteinsson, V. A. et al. Scalable whole-exome sequencing of cell-free DNA reveals high concordance with metastatic tumors. Nat. Commun. 8 , 1324 (2017).
Zhang, Z. et al. Uniform genomic data analysis in the NCI Genomic Data Commons. Nat. Commun. 12 , 1226 (2021).
Vega, D. M. et al. Aligning tumor mutational burden (TMB) quantification across diagnostic platforms: phase II of the Friends of Cancer Research TMB Harmonization Project. Ann. Oncol. 32 , 1626–1636 (2021).
Anaya, J., Sidhom, J.-W., Mahmood, F. & Baras, A. S. Multiple-instance learning of somatic mutations for the classification of tumour type and the prediction of microsatellite status. Nat. Biomed. Eng. 8 , 57–67 (2023).
Chen, B. et al. Predicting HLA class II antigen presentation through integrated deep learning. Nat. Biotechnol. 37 , 1332–1343 (2019).
Jumper, J. et al. Highly accurate protein structure prediction with AlphaFold. Nature 596 , 583–589 (2021).
Callaway, E. What’s next for AlphaFold and the AI protein-folding revolution. Nature 604 , 234–238 (2022).
Cheng, J. et al. Accurate proteome-wide missense variant effect prediction with AlphaMissense. Science 381 , eadg7492 (2023).
Barrio-Hernandez, I. et al. Clustering predicted structures at the scale of the known protein universe. Nature 622 , 637–645 (2023).
Yang, X., Wang, Y., Byrne, R., Schneider, G. & Yang, S. Concepts of artificial intelligence for computer-assisted drug discovery. Chem. Rev. 119 , 10520–10594 (2019).
Mullowney, M. W. et al. Artificial intelligence for natural product drug discovery. Nat. Rev. Drug Discov. 22 , 895–916 (2023).
Jayatunga, M. K. P., Xie, W., Ruder, L., Schulze, U. & Meier, C. AI in small-molecule drug discovery: a coming wave? Nat. Rev. Drug Discov. 21 , 175–176 (2022).
Vert, J.-P. How will generative AI disrupt data science in drug discovery? Nat. Biotechnol. 41 , 750–751 (2023).
Wong, F. et al. Discovery of a structural class of antibiotics with explainable deep learning. Nature 626 , 177–185 (2023).
Swanson, K. et al. Generative AI for designing and validating easily synthesizable and structurally novel antibiotics. Nat. Mach. Intell. 6 , 338–353 (2024).
Janizek, J. D. et al. Uncovering expression signatures of synergistic drug responses via ensembles of explainable machine-learning models. Nat. Biomed. Eng. 7 , 811–829 (2023).
Savage, N. Drug discovery companies are customizing ChatGPT: here’s how. Nat. Biotechnol. 41 , 585–586 (2023).
Boiko, D. A., MacKnight, R., Kline, B. & Gomes, G. Autonomous chemical research with large language models. Nature 624 , 570–578 (2023).
Arnold, C. AlphaFold touted as next big thing for drug discovery—but is it? Nature 622 , 15–17 (2023).
Mock, M., Edavettal, S., Langmead, C. & Russell, A. AI can help to speed up drug discovery—but only if we give it the right data. Nature 621 , 467–470 (2023).
AI’s potential to accelerate drug discovery needs a reality check. Nature 622 , 217 (2023).
Upswing in AI drug-discovery deals. Nat. Biotechnol . 41 , 1361 (2023).
Hutson, M. AI for drug discovery is booming, but who owns the patents? Nat. Biotechnol. 41 , 1494–1496 (2023).
Wong, C. H., Siah, K. W. & Lo, A. W. Estimation of clinical trial success rates and related parameters. Biostatistics 20 , 273–286 (2019).
Subbiah, V. The next generation of evidence-based medicine. Nat. Med. 29 , 49–58 (2023).
Yuan, C. et al. Criteria2Query: a natural language interface to clinical databases for cohort definition. J. Am. Med. Inform. Assoc. 26 , 294–305 (2019).
Lu, L., Dercle, L., Zhao, B. & Schwartz, L. H. Deep learning for the prediction of early on-treatment response in metastatic colorectal cancer from serial medical imaging. Nat. Commun. 12 , 6654 (2021).
Trebeschi, S. et al. Prognostic value of deep learning-mediated treatment monitoring in lung cancer patients receiving immunotherapy. Front. Oncol. 11 , 609054 (2021).
Castelo-Branco, L. et al. ESMO guidance for reporting oncology real-world evidence (GROW). Ann. Oncol. 34 , 1097–1112 (2023).
Morin, O. et al. An artificial intelligence framework integrating longitudinal electronic health records with real-world data enables continuous pan-cancer prognostication. Nat. Cancer 2 , 709–722 (2021).
Yang, X. et al. A large language model for electronic health records. NPJ Digit. Med. 5 , 194 (2022).
Huang, X., Rymbekova, A., Dolgova, O., Lao, O. & Kuhlwilm, M. Harnessing deep learning for population genetic inference. Nat. Rev. Genet. 25 , 61–78 (2024).
Pawlicki, Lee, D.-S., Hull & Srihari. Neural network models and their application to handwritten digit recognition. In IEEE 1988 Int. Conf. Neural Networks (eds Pawlicki, T. F. et al.) 63–70 (1988).
Chui, M. et al. The economic potential of generative AI: the next productivity frontier. McKinsey https://www.mckinsey.com/capabilities/mckinsey-digital/our-insights/the-economic-potential-of-generative-ai-the-next-productivity-frontier (2023).
Dell’Acqua, F. et al. Navigating the jagged technological frontier: field experimental evidence of the effects of AI on knowledge worker productivity and quality. Harvard Business School https://www.hbs.edu/ris/Publication%20Files/24-013_d9b45b68-9e74-42d6-a1c6-c72fb70c7282.pdf (2023).
Boehm, K. M., Khosravi, P., Vanguri, R., Gao, J. & Shah, S. P. Harnessing multimodal data integration to advance precision oncology. Nat. Rev. Cancer 22 , 114–126 (2022).
Gilbert, S., Harvey, H., Melvin, T., Vollebregt, E. & Wicks, P. Large language model AI chatbots require approval as medical devices. Nat. Med. 29 , 2396–2398 (2023).
Mobadersany, P. et al. Predicting cancer outcomes from histology and genomics using convolutional networks. Proc. Natl Acad. Sci. USA 115 , E2970–E2979 (2018).
Chang, Y. et al. A survey on evaluation of large language models. ACM Trans. Intell. Syst. Technol. 15 , 1–45 (2024).
Lin, T., Wang, Y., Liu, X. & Qiu, X. A survey of transformers. AI Open 3 , 111–132 (2022).
Download references
Acknowledgements
R.P.-L. is supported by LaCaixa Foundation, a CRIS Foundation Talent Award (TALENT19-05), the FERO Foundation, the Instituto de Salud Carlos III-Investigacion en Salud (PI18/01395 and PI21/01019), the Prostate Cancer Foundation (18YOUN19) and the Asociación Española Contra el Cancer (AECC) (PRYCO211023SERR). J.N.K. is supported by the German Cancer Aid (DECADE, 70115166), the German Federal Ministry of Education and Research (PEARL, 01KD2104C; CAMINO, 01EO2101; SWAG, 01KD2215A; TRANSFORM LIVER, 031L0312A; and TANGERINE, 01KT2302 through ERA-NET Transcan), the German Academic Exchange Service (SECAI, 57616814), the German Federal Joint Committee (TransplantKI, 01VSF21048), the European Union’s Horizon Europe and innovation programme (ODELIA, 101057091; and GENIAL, 101096312), the European Research Council (ERC; NADIR, 101114631) and the National Institute for Health and Care Research (NIHR; NIHR203331) Leeds Biomedical Research Centre. The views expressed are those of the authors and not necessarily those of the NHS, the NIHR or the Department of Health and Social Care. This work was funded by the European Union. Views and opinions expressed are, however, those of the authors only and do not necessarily reflect those of the European Union. Neither the European Union nor the granting authority can be held responsible for them.
Author information
Authors and affiliations.
Radiomics Group, Vall d’Hebron Institute of Oncology, Vall d’Hebron Barcelona Hospital Campus, Barcelona, Spain
Raquel Perez-Lopez
Else Kroener Fresenius Center for Digital Health, Technical University Dresden, Dresden, Germany
Narmin Ghaffari Laleh & Jakob Nikolas Kather
Department of Pathology, Brigham and Women’s Hospital, Harvard Medical School, Boston, MA, USA
Faisal Mahmood
Department of Pathology, Massachusetts General Hospital, Harvard Medical School, Boston, MA, USA
Cancer Program, Broad Institute of Harvard and MIT, Cambridge, MA, USA
Cancer Data Science Program, Dana-Farber Cancer Institute, Boston, MA, USA
Department of Biomedical Informatics, Harvard Medical School, Boston, MA, USA
Harvard Data Science Initiative, Harvard University, Cambridge, MA, USA
Department of Medicine I, University Hospital Dresden, Dresden, Germany
Jakob Nikolas Kather
Medical Oncology, National Center for Tumour Diseases (NCT), University Hospital Heidelberg, Heidelberg, Germany
You can also search for this author in PubMed Google Scholar
Contributions
All authors contributed substantially to discussion of the content and reviewed and/or edited the manuscript before the submission. R.P.-L., N.G.L. and J.N.K. researched data for the article and wrote the article.
Corresponding author
Correspondence to Jakob Nikolas Kather .
Ethics declarations
Competing interests.
J.N.K. declares consulting services for Owkin, DoMore Diagnostics, Panakeia, Scailyte, Mindpeak and MultiplexDx; holds shares in StratifAI GmbH; has received a research grant from GSK; and has received honoraria from AstraZeneca, Bayer, Eisai, Janssen, MSD, BMS, Roche, Pfizer and Fresenius. R.P.-L. declares research funding by AstraZeneca and Roche, and participates in the steering committee of a clinical trial sponsored by Roche, not related to this work. All other authors declare no competing interests.
Peer review
Peer review information.
Nature Reviews Cancer thanks the anonymous reviewer(s) for their contribution to the peer review of this work.
Additional information
Publisher’s note Springer Nature remains neutral with regard to jurisdictional claims in published maps and institutional affiliations.
Related links
Hugging Face: https://huggingface.co/
You.com: https://you.com
Supplementary information
Supplementary information.
(API). A set of tools and protocols for building software and applications, enabling software to communicate with AI models.
(ANNs). Computational models loosely inspired by the structure and function of the human brain, consisting of interconnected layers of nodes, called neurons, that process input data and learn to recognize patterns and make decisions.
The use of algorithms, machine learning and image analysis techniques to extract information from digital pathology images.
A field of AI that focuses on enabling computers to analyse and interpret visual data, such as images and videos.
(CNNs). A type of deep neural network that is especially effective for analysing visual imagery and used in image analysis.
Deep learning is a subfield of machine learning that uses artificial neural networks with multiple layers, called deep neural networks, to learn and extract highly complex features and patterns from raw input data.
Visual representations captured and stored in a digital format, consisting of a grid of pixels, with each pixel representing a colour intensity value.
The practice of converting glass slides into digital slides that can be viewed, managed and analysed on a computer.
Techniques in AI that provide insights and explanations on how the AI model arrived at its conclusions, thus making the decision-making process of the AI more transparent.
AI systems that can generate new content (text, images or music) that is similar to the content on which it was trained, often creating novel and coherent outputs.
Extremely high-resolution digital images consisting of 1 billion pixels, obtained by scanning tissue slides with a slide scanner.
(GPUs). Specialized hardware used to rapidly process large blocks of data simultaneously, used in computer gaming and AI.
(LLMs). Advanced AI models trained on vast amounts of text data, capable of analysing, generating and manipulating human language, often at the human level 174 .
A type of neural network particularly good at processing sequences of data (such as time series or language), with a capability to remember information for a certain time.
A subset of AI focusing on the development of algorithms and models that enable computers to learn and improve their performance on a specific task without being explicitly instructed how to achieve this.
(NLP). A branch of AI that helps computers to analyse, interpret and respond to human language in a useful way.
Crafting inputs or questions in a way that guides AI models, particularly LLMs, to provide the most effective and accurate responses.
Types of a neural network model that excel at processing sequences of data, such as sentences in text, by focusing on different parts of the sequence to make predictions 175 .
The three-dimensional equivalent of a pixel in images, representing a value on a regular grid in three-dimensional space, commonly used in medical imaging such as MRI and CT scans.
Rights and permissions
Springer Nature or its licensor (e.g. a society or other partner) holds exclusive rights to this article under a publishing agreement with the author(s) or other rightsholder(s); author self-archiving of the accepted manuscript version of this article is solely governed by the terms of such publishing agreement and applicable law.
Reprints and permissions
About this article
Cite this article.
Perez-Lopez, R., Ghaffari Laleh, N., Mahmood, F. et al. A guide to artificial intelligence for cancer researchers. Nat Rev Cancer (2024). https://doi.org/10.1038/s41568-024-00694-7
Download citation
Accepted : 09 April 2024
Published : 16 May 2024
DOI : https://doi.org/10.1038/s41568-024-00694-7
Share this article
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
Quick links
- Explore articles by subject
- Guide to authors
- Editorial policies
Sign up for the Nature Briefing: Cancer newsletter — what matters in cancer research, free to your inbox weekly.

A Lagrangian approach for solving an axisymmetric thermo-electromagnetic problem. Application to time-varying geometry processes
- Open access
- Published: 08 May 2024
- Volume 50 , article number 45 , ( 2024 )
Cite this article
You have full access to this open access article
- Marta Benítez 1 , 2 ,
- Alfredo Bermúdez 1 , 3 ,
- Pedro Fontán 4 ,
- Iván Martínez 1 , 3 &
- Pilar Salgado 1 , 3
72 Accesses
Explore all metrics
The aim of this work is to introduce a thermo-electromagnetic model for calculating the temperature and the power dissipated in cylindrical pieces whose geometry varies with time and undergoes large deformations; the motion will be a known data. The work will be a first step towards building a complete thermo-electromagnetic-mechanical model suitable for simulating electrically assisted forming processes, which is the main motivation of the work. The electromagnetic model will be obtained from the time-harmonic eddy current problem with an in-plane current; the source will be given in terms of currents or voltages defined at some parts of the boundary. Finite element methods based on a Lagrangian weak formulation will be used for the numerical solution. This approach will avoid the need to compute and remesh the thermo-electromagnetic domain along the time. The numerical tools will be implemented in FEniCS and validated by using a suitable test also solved in Eulerian coordinates.
Article PDF
Download to read the full article text
Similar content being viewed by others
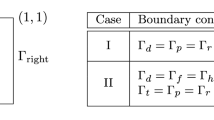
Physics-preserving enriched Galerkin method for a fully-coupled thermo-poroelasticity model
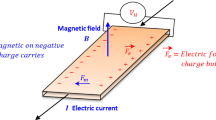
Deformation in a micropolar material under the influence of Hall current and initial stress fields in the context of a double-temperature thermoelastic theory involving phase lag and higher orders
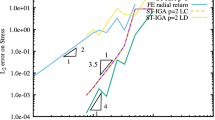
A space-time formulation for time-dependent behaviors at small or finite strains
Avoid common mistakes on your manuscript.
Alonso-Rodríguez, A., Valli, A.: Eddy Current Approximation of Maxwell Equations: Theory. Algorithms and Applications. Springer, Milan (2010)
Alves, J., Acevedo, S., Marie, S., Adams, B., Mocellin, K., Bay, F.: Numerical modeling of electrical upsetting manufacturing processes based on Forge® environment. AIP Conf. Proc. 1896 , 120003 (2017). https://doi.org/10.1063/1.5008141
Bermúdez, A., Rodríguez, R., Salgado, P.: Numerical solution of eddy current problems in bounded domains using realistic boundary conditions. Comput. Methods Appl. Mech. Eng. 194 (2), 411–426 (2005)
Bermúdez, A., Bullón, J., Pena, F., Salgado, P.: A numerical method for transient simulation of metallurgical compound electrodes. Finite Elem. Anal. Des. 39 , 283–299 (2003). https://api.semanticscholar.org/CorpusID:121553921
Bermúdez, A., Gómez, D., Muñiz, M.C., Salgado, P.: Transient numerical simulation of a thermoelectrical problem in cylindrical induction heating furnaces. Adv. Comput. Math. 26 (1–3), 39–62 (2007). https://doi.org/10.1007/s10444-005-7470-9
Bermúdez, A., Gómez, D., Salgado, P.: Mathematical Models and Numerical Simulation in Electromagnetism, UNITEXT , vol. 74. Springer, New York (2014). https://doi.org/10.1007/978-3-319-02949-8
Bermúdez, A., López-Rodríguez, B., Pena, F.J., Rodríguez, R., Salgado, P., Venegas, P.: Numerical solution of an axisymmetric eddy current model with current and voltage excitations. J. Sci. Comput. 91(1), Paper No. 8, 26 (2022). https://doi.org/10.1007/s10915-022-01780-4
Bermúdez, A., López-Rodríguez, B., Rodríguez, R., Salgado, P.: Numerical solution of transient eddy current problems with input current intensities as boundary data. IMA J. Numer. Anal. 32 (3), 1001–1029 (2012). https://doi.org/10.1093/imanum/drr028
Bossavit, A.: Two dual formulations of the 3D eddy currents problem. COMPEL - Int. J. Comput. Math. Electr. Electron. Eng. 4 , 103–116 (1985)
Bossavit, A.: Forces in magnetostatics and their computation. J. Appl. Phys. 67(9), 5812–5814 (1990). https://doi.org/10.1063/1.345972
Bossavit, A.: Differential forms and the computation of fields and forces in Electromagnetism. Eur. J. Mech. B Fluids 10 , 474–488 (1991). https://api.semanticscholar.org/CorpusID:123083223
Bossavit, A.: On “Hybrid” Electric-Magnetic Methods, pp. 237–240. Springer US, Boston, MA (1995). https://doi.org/10.1007/978-1-4615-1961-4_54
Bossavit, A.: Computational Electromagnetism. Variational Formulations, Complementarity, Edge Elements. Variational Formulations, Complementarity, Edge Elements. Academic Press Inc., San Diego, CA, (1998)
Bossavit, A.: Most general “non-local” boundary conditions for the Maxwell equation in a bounded region. COMPEL - Int J Comput Math Electr Electron Eng 19 , (2000)
Bossavit, A., Verite, J.: The “TRIFOU” Code: solving the 3-D eddy-currents problem by using H as state variable. IEEE Trans. Magn. 19 (6), 2465–2470 (1983). https://doi.org/10.1109/TMAG.1983.1062817
Fontán, P.: Mathematical analysis and numerical simulation with pure Lagrangian and semi-Lagrangian methods of problems in continuum mechanics. Ph.D. thesis (2021)
Gurtin, M.E.: An introduction to continuum mechanics, vol. 158. Academic Press, San Diego (2003)
Google Scholar
Lax, M., Nelson, D.F.: Maxwell equations in material form. Phys. Rev. B. 13 (4), 1777–1784 (1976). https://doi.org/10.1103/PhysRevB.13.1777
Logg, A., Mardal, K.A., Wells, G. N.: Automated solution of differential equations by the finite element method. The FEniCS book, Lecture Notes in Computational Science and Engineering , vol. 84. Springer, Heidelberg (2012). https://doi.org/10.1007/978-3-642-23099-8
Monk, P.: Finite element methods for Maxwell’s equations. Numerical Mathematics and Scientific Computation. Oxford University Press, New York (2003). https://doi.org/10.1093/acprof:oso/9780198508885.001.0001
Niyonzima, I., Jiao, Y., Fish, J.: Modeling and simulation of nonlinear electro-thermo-mechanical continua with application to shape memory polymeric medical devices. Comput. Methods Appl. Mech. Eng 350 , 511–534 (2019). https://doi.org/10.1016/j.cma.2019.03.003
Paoli, G., Biro, O., Buchgraber, G: Complex representation in nonlinear time harmonic Eddy current problems. In: Proceeding of the 11th COMPUMAG Conference on the Computation of Electromagnetic Fields. pp. 647–648, COMPUMAG, Rio de Janeiro (1997)
Petzold, T.: Modelling, Analysis and Simulation of Multifrequency Induction Hardening. Ph.D. thesis (2014). https://doi.org/10.14279/depositonce-4118
Quan, Guo-Zheng., Zou, Zhen-Yu., Zhang, Zhi-Hua., Pan, Jia: A study on formation process of secondary upsetting defect in electric upsetting and optimization of processing parameters based on multi-field coupling FEM. Mater. Res. 19 , 856–864 (2016)
Article Google Scholar
Thomas, J., Triantafyllidis, N.: On electromagnetic forming processes in finitely strained solids: theory and examples. J. Mech. Phys. Solids 57 (8), 1391–1416 (2009). https://doi.org/10.1016/j.jmps.2009.04.004
Download references
Acknowledgements
Open Access funding provided thanks to the CRUE-CSIC agreement with Springer Nature.
Open Access funding provided thanks to the CRUE-CSIC agreement with Springer Nature. The research has been developed in collaboration with CIE Galfor through a project granted by the Centre for the Development of Industrial Technology (CDTI) and signed between the company CIE Galfor and Itmati (nowadays, integrated in CITMAga). This work has been partially supported by FEDER, Ministerio de Economía, Industria y Competitividad-AEI research project PID2021-122625OBI00 and by Xunta de Galicia (Spain) research project GRC GI-1563 ED431C 2021/15.
Author information
Authors and affiliations.
Galician Centre for Mathematical Research and Technology (CITMAga), Campus Vida, Santiago de Compostela, E-15782, Spain
Marta Benítez, Alfredo Bermúdez, Iván Martínez & Pilar Salgado
Department of Mathematics, University of A Coruña, Elviña s/n, A Coruña, E-15071, Spain
Marta Benítez
Department of Applied Mathematics, University of Santiago de Compostela, Campus Vida, Santiago de Compostela, E-15782, Spain
Alfredo Bermúdez, Iván Martínez & Pilar Salgado
REPSOL Technology Lab, Autovía de Extremadura s/n, Móstoles, Madrid, 28935, Spain
Pedro Fontán
You can also search for this author in PubMed Google Scholar
Corresponding author
Correspondence to Pilar Salgado .
Ethics declarations
Conflict of interest.
The authors declare that they have no conflict of interest.
Additional information
Publisher's note.
Springer Nature remains neutral with regard to jurisdictional claims in published maps and institutional affiliations.
This article is dedicated to Professor Alain Bossavit on the occasion of his 80th birthday.
Rights and permissions
Open Access This article is licensed under a Creative Commons Attribution 4.0 International License, which permits use, sharing, adaptation, distribution and reproduction in any medium or format, as long as you give appropriate credit to the original author(s) and the source, provide a link to the Creative Commons licence, and indicate if changes were made. The images or other third party material in this article are included in the article's Creative Commons licence, unless indicated otherwise in a credit line to the material. If material is not included in the article's Creative Commons licence and your intended use is not permitted by statutory regulation or exceeds the permitted use, you will need to obtain permission directly from the copyright holder. To view a copy of this licence, visit http://creativecommons.org/licenses/by/4.0/ .
Reprints and permissions
About this article
Benítez, M., Bermúdez, A., Fontán, P. et al. A Lagrangian approach for solving an axisymmetric thermo-electromagnetic problem. Application to time-varying geometry processes. Adv Comput Math 50 , 45 (2024). https://doi.org/10.1007/s10444-024-10121-y
Download citation
Received : 16 October 2023
Accepted : 04 March 2024
Published : 08 May 2024
DOI : https://doi.org/10.1007/s10444-024-10121-y
Share this article
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Lagrangian methods
- Eddy currents
- Thermo-electromagnetic
- Time dependent domain
- Axisymmetric
Mathematics Subject Classification
Advertisement
- Find a journal
- Publish with us
- Track your research
Facility for Rare Isotope Beams
At michigan state university, international research team uses wavefunction matching to solve quantum many-body problems, new approach makes calculations with realistic interactions possible.
FRIB researchers are part of an international research team solving challenging computational problems in quantum physics using a new method called wavefunction matching. The new approach has applications to fields such as nuclear physics, where it is enabling theoretical calculations of atomic nuclei that were previously not possible. The details are published in Nature (“Wavefunction matching for solving quantum many-body problems”) .
Ab initio methods and their computational challenges
An ab initio method describes a complex system by starting from a description of its elementary components and their interactions. For the case of nuclear physics, the elementary components are protons and neutrons. Some key questions that ab initio calculations can help address are the binding energies and properties of atomic nuclei not yet observed and linking nuclear structure to the underlying interactions among protons and neutrons.
Yet, some ab initio methods struggle to produce reliable calculations for systems with complex interactions. One such method is quantum Monte Carlo simulations. In quantum Monte Carlo simulations, quantities are computed using random or stochastic processes. While quantum Monte Carlo simulations can be efficient and powerful, they have a significant weakness: the sign problem. The sign problem develops when positive and negative weight contributions cancel each other out. This cancellation results in inaccurate final predictions. It is often the case that quantum Monte Carlo simulations can be performed for an approximate or simplified interaction, but the corresponding simulations for realistic interactions produce severe sign problems and are therefore not possible.
Using ‘plastic surgery’ to make calculations possible
The new wavefunction-matching approach is designed to solve such computational problems. The research team—from Gaziantep Islam Science and Technology University in Turkey; University of Bonn, Ruhr University Bochum, and Forschungszentrum Jülich in Germany; Institute for Basic Science in South Korea; South China Normal University, Sun Yat-Sen University, and Graduate School of China Academy of Engineering Physics in China; Tbilisi State University in Georgia; CEA Paris-Saclay and Université Paris-Saclay in France; and Mississippi State University and the Facility for Rare Isotope Beams (FRIB) at Michigan State University (MSU)—includes Dean Lee , professor of physics at FRIB and in MSU’s Department of Physics and Astronomy and head of the Theoretical Nuclear Science department at FRIB, and Yuan-Zhuo Ma , postdoctoral research associate at FRIB.
“We are often faced with the situation that we can perform calculations using a simple approximate interaction, but realistic high-fidelity interactions cause severe computational problems,” said Lee. “Wavefunction matching solves this problem by doing plastic surgery. It removes the short-distance part of the high-fidelity interaction, and replaces it with the short-distance part of an easily computable interaction.”
This transformation is done in a way that preserves all of the important properties of the original realistic interaction. Since the new wavefunctions look similar to that of the easily computable interaction, researchers can now perform calculations using the easily computable interaction and apply a standard procedure for handling small corrections called perturbation theory. A team effort
The research team applied this new method to lattice quantum Monte Carlo simulations for light nuclei, medium-mass nuclei, neutron matter, and nuclear matter. Using precise ab initio calculations, the results closely matched real-world data on nuclear properties such as size, structure, and binding energies. Calculations that were once impossible due to the sign problem can now be performed using wavefunction matching.
“It is a fantastic project and an excellent opportunity to work with the brightest nuclear scientist s in FRIB and around the globe,” said Ma. “As a theorist , I'm also very excited about programming and conducting research on the world's most powerful exascale supercomputers, such as Frontier , which allows us to implement wavefunction matching to explore the mysteries of nuclear physics.”
While the research team focused solely on quantum Monte Carlo simulations, wavefunction matching should be useful for many different ab initio approaches, including both classical and quantum computing calculations. The researchers at FRIB worked with collaborators at institutions in China, France, Germany, South Korea, Turkey, and United States.
“The work is the culmination of effort over many years to handle the computational problems associated with realistic high-fidelity nuclear interactions,” said Lee. “It is very satisfying to see that the computational problems are cleanly resolved with this new approach. We are grateful to all of the collaboration members who contributed to this project, in particular, the lead author, Serdar Elhatisari.”
This material is based upon work supported by the U.S. Department of Energy, the U.S. National Science Foundation, the German Research Foundation, the National Natural Science Foundation of China, the Chinese Academy of Sciences President’s International Fellowship Initiative, Volkswagen Stiftung, the European Research Council, the Scientific and Technological Research Council of Turkey, the National Natural Science Foundation of China, the National Security Academic Fund, the Rare Isotope Science Project of the Institute for Basic Science, the National Research Foundation of Korea, the Institute for Basic Science, and the Espace de Structure et de réactions Nucléaires Théorique.
Michigan State University operates the Facility for Rare Isotope Beams (FRIB) as a user facility for the U.S. Department of Energy Office of Science (DOE-SC), supporting the mission of the DOE-SC Office of Nuclear Physics. Hosting what is designed to be the most powerful heavy-ion accelerator, FRIB enables scientists to make discoveries about the properties of rare isotopes in order to better understand the physics of nuclei, nuclear astrophysics, fundamental interactions, and applications for society, including in medicine, homeland security, and industry.
The U.S. Department of Energy Office of Science is the single largest supporter of basic research in the physical sciences in the United States and is working to address some of today’s most pressing challenges. For more information, visit energy.gov/science.
Accessibility Links
- Skip to content
- Skip to search IOPscience
- Skip to Journals list
- Accessibility help
- Accessibility Help
Click here to close this panel.
Purpose-led Publishing is a coalition of three not-for-profit publishers in the field of physical sciences: AIP Publishing, the American Physical Society and IOP Publishing.
Together, as publishers that will always put purpose above profit, we have defined a set of industry standards that underpin high-quality, ethical scholarly communications.
We are proudly declaring that science is our only shareholder.
CUQIpy: II. Computational uncertainty quantification for PDE-based inverse problems in Python
Amal M A Alghamdi 1 , Nicolai A B Riis 1 , Babak M Afkham 1 , Felipe Uribe 4,2 , Silja L Christensen 1 , Per Christian Hansen 1 and Jakob S Jørgensen 5,1,3
Published 4 March 2024 • © 2024 The Author(s). Published by IOP Publishing Ltd Inverse Problems , Volume 40 , Number 4 Special Issue on Big Data Inverse Problems Citation Amal M A Alghamdi et al 2024 Inverse Problems 40 045010 DOI 10.1088/1361-6420/ad22e8
Article metrics
360 Total downloads
Share this article
Author e-mails.
Author affiliations
1 Department of Applied Mathematics and Computer Science, Technical University of Denmark. Richard Petersens Plads, Building 324, 2800 Kongens Lyngby, Denmark
2 School of Engineering Sciences, Lappeenranta-Lahti University of Technology (LUT), Yliopistonkatu 34, 53850 Lappeenranta, Finland
3 Department of Mathematics, The University of Manchester, Oxford Road, Alan Turing Building, Manchester M13 9PL, United Kingdom
Author notes
4 Part of the work by F.U. was done while employed at the Technical University of Denmark.
5 Author to whom any correspondence should be addressed.
Amal M A Alghamdi https://orcid.org/0000-0003-0145-5296
Nicolai A B Riis https://orcid.org/0000-0002-6883-9078
Babak M Afkham https://orcid.org/0000-0003-3203-8874
Felipe Uribe https://orcid.org/0000-0002-1010-8184
Silja L Christensen https://orcid.org/0000-0003-3995-3055
Per Christian Hansen https://orcid.org/0000-0002-7333-7216
Jakob S Jørgensen https://orcid.org/0000-0001-9114-754X
- Received 2 April 2023
- Accepted 26 January 2024
- Published 4 March 2024
Peer review information
Method : Single-anonymous Revisions: 1 Screened for originality? Yes
Buy this article in print
Inverse problems, particularly those governed by Partial Differential Equations (PDEs), are prevalent in various scientific and engineering applications, and uncertainty quantification (UQ) of solutions to these problems is essential for informed decision-making. This second part of a two-paper series builds upon the foundation set by the first part, which introduced CUQIpy, a Python software package for computational UQ in inverse problems using a Bayesian framework. In this paper, we extend CUQIpy's capabilities to solve PDE-based Bayesian inverse problems through a general framework that allows the integration of PDEs in CUQIpy, whether expressed natively or using third-party libraries such as FEniCS. CUQIpy offers concise syntax that closely matches mathematical expressions, streamlining the modeling process and enhancing the user experience. The versatility and applicability of CUQIpy to PDE-based Bayesian inverse problems are demonstrated on examples covering parabolic, elliptic and hyperbolic PDEs. This includes problems involving the heat and Poisson equations and application case studies in electrical impedance tomography and photo-acoustic tomography, showcasing the software's efficiency, consistency, and intuitive interface. This comprehensive approach to UQ in PDE-based inverse problems provides accessibility for non-experts and advanced features for experts.
Export citation and abstract BibTeX RIS
Original content from this work may be used under the terms of the Creative Commons Attribution 4.0 license . Any further distribution of this work must maintain attribution to the author(s) and the title of the work, journal citation and DOI.
1. Introduction
Inverse problems arise in various scientific and engineering applications, where the goal is to infer un-observable features from indirect observations. These problems are often ill-posed, making the inferred solution sensitive to noise in observed data and inaccuracies in forward models [ 19 , 25 ]. Characterizing and evaluating uncertainties due to this sensitivity is crucial when making decisions based on inferred results.
To address these challenges, the field of Uncertainty Quantification (UQ) for inverse problems is in a phase of rapid growth [ 6 , 39 ]. In medical imaging, for instance, UQ analysis allows experts to evaluate the uncertainty in cancer detection, which can directly impact patient treatment decisions [ 40 ]. In flood control and disaster management applications, UQ is needed to assess the risk of floods in specific regions, informing planning and resource allocation [ 30 ].
One particularly important category of inverse problems involves those governed by Partial Differential Equations (PDEs). These problems are encountered in various applications such as medical imaging [ 29 , 41 ], seismic imaging [ 8 , 9 , 38 ], subsurface characterization [ 3 , 4 , 21 ], and non-destructive testing [ 34 ]. PDE-based inverse problems involve inferring parameters in PDE models from observed data, which introduces unique challenges in UQ due to the complex nature of the governing equations.
The Bayesian framework is widely used for UQ in both PDE-based and non-PDE-based inverse problems, as it enables the systematic incorporation of prior information, forward models, and observed data by characterizing the so-called posterior distribution [ 3 , 4 , 9 , 11 , 31 ]. This framework provides a comprehensive and unified approach for addressing the unique challenges of UQ in inverse problems.
1.1. Computational UQ for PDE-based inverse problems with CUQIpy
In this two-part series, we propose a Python software package CUQIpy, for Computational Uncertainty Quantification in Inverse problems. The package aims to make UQ analysis accessible to non-experts while still providing advanced features for UQ experts. The first paper [ 35 ] introduces the core library and components, and presents various test cases.
This second paper focuses on using CUQIpy (version 1.0.0) to solve Bayesian inverse problems where the forward models are governed by PDEs. While numerous software tools exist for modeling and solving PDE systems, such as FEniCS [ 28 ], FiPy [ 18 ], PyClaw [ 26 ], scikit-fem [ 17 ], and Firedrake [ 33 ], only few tools are specifically designed for PDE-based Bayesian inverse problems. The FEniCS-based package hIPPYlib [ 42 , 43 ] is an example of a package that excels in this task.
To make UQ for PDE-based inverse problem more accessible, we propose a general framework for integrating PDE modeling tools into CUQIpy by defining an application programming interface (API) allowing PDE modeling libraries to interact with CUQIpy, regardless of the underlying PDE discretization scheme and implementation. This is possible because a major concept behind the design of CUQIpy is that the core components remain independent from specific forward modeling tools. On the other hand, plugins provide a flexible way to interface with third-party libraries, and in this paper we present CUQIpy-FEniCS as an example of a PDE-based plugin.
We introduce modules and classes in CUQIpy that enable solving PDE-based Bayesian inverse problems, such as sampler , distribution , and the cuqi.pde module. In the latter, the cuqi.pde.PDE class provides an abstract interface for integrating PDE modeling implementations like FEniCS with CUQIpy, simplifying the construction of PDE-based Bayesian inverse problems. The modules cuqi.pde.geometry and cuqipy_fenics.pde.geometry play an essential role, allowing the software to use information about the spaces on which the parameters and data are defined.
We demonstrate the versatility and applicability of CUQIpy through a variety of PDE-based examples, highlighting the integration and capabilities of the software. One example solves a Bayesian inverse problem governed by a one-dimensional (1D) heat equation, which underscores the intuitiveness of CUQIpy's interface and its correspondence to the mathematical problem description. We present an elaborate electric impedance tomography (EIT) case study using the CUQIpy-FEniCS plugin, illustrating integration with third-party PDE modeling libraries. Finally, we examine a photoacoustic tomography (PAT) case, which shows CUQIpy's ability to handle black-box forward models, emphasizing its adaptability to a wide range of applications in PDE-based Bayesian inverse problems. These examples effectively represent different classes of PDEs: parabolic, elliptic, and hyperbolic PDEs, respectively.
We have sought to design a versatile PDE abstraction layer for modeling a variety of PDE-based problems within the general Bayesian inverse problems framework provided by CUQIpy with focus on modularity and an intuitive, user-friendly interface. The goal of this paper is to demonstrate its utility on small to moderate scale problems on which we have found CUQIpy to perform well. Support for specialized PDE problems of high complexity and large-scale computing needs is an important area of development for the CUQIpy framework. We believe that the plugin structure, as exemplified by the CUQIpy-FEniCS plugin presented within, will provide a route to handle large-scale problems. This will combine the efficiency of dedicated third-party libraries (such as fluid dynamics solvers or implementations of adjoint equations, etc) with the convenience of the high-level modeling framework of CUQIpy.
1.2. A motivating example
We give a brief introductory example of the use of CUQIpy to solve a PDE-based inverse problem with the Poisson equation modeled in FEniCS using the CUQIpy-FEniCS plugin. More details of the underlying computational machinery are provided in section 3 .
In CUQIpy we consider the discretized form of this problem,
where A is a nonlinear forward model, which corresponds to solving the discretized PDE to produce the observation y from a log-conductivity given in terms of a parameter x . CUQIpy (and in this case CUQIpy-FEniCS) provides a collection of demonstration test problems including one from which the present forward model can be obtained as:

Download figure:
In CUQIpy we consider x and y vector-valued random variables representing the parameter to be inferred and the data, respectively. To specify a Bayesian inverse problem, we express statistical assumptions on variables and the relations between them. Here, we assume an i.i.d. standard normal distribution on the KL expansion coefficients x and additive i.i.d. Gaussian noise with known standard deviation s noise on the data:
We can specify this in CUQIpy as (with np representing NumPy [ 20 ]):

We note the close similarity between the mathematical expressions and the syntax. Additionally the distributions have been equipped with so-called geometry object G_KL and G_FEM , which capture the interpretation of x as KL coefficients and y as FEM expansion coefficients; this is elaborated in section 3 .
We consider a true log-conductivity as a sample from the prior distribution on x , which we conveniently generate and plot (figure 1 (a)) by

Under the hood, CUQIpy applies Bayes' theorem to construct the posterior distribution, selects a suitable sampler based on the problem structure (in this case the NUTS sampler [ 22 ]), samples the posterior and produces posterior mean and UQ plots (figure 1 ).
1.3. Overview and notation
Having introduced and given a motivating example of UQ with CUQIpy for a PDE-based inverse problem in the present section, we present in section 2 our general framework for integrating PDE-based inverse problems in CUQIpy and illustrate this framework on an inverse problem governed by the 1D heat equation. In section 3 , we describe our CUQIpy-FEniCS plugin that extends CUQIpy to allow UQ on PDE-based inverse problems modeled in FEniCS. We finish with two more elaborate case studies: First, in section 4 we demonstrate how electrical impedance tomography (EIT) with multiple layers of solution parametrization can be modeled with CUQIpy-FEniCS. Second, in section 5 we show how user-provided black-box PDE solvers can be used in CUQIpy in an example of Photo-Acoustic Tomography (PAT). Finally, in section 6 we conclude the paper.
2. Framework for PDE-based Bayesian inverse problems in CUQIpy
In this section, we present our general framework for integrating PDE-based Bayesian inverse problems in CUQIpy. The framework is designed to be as generic as possible allowing—in principle—any PDE-based inverse problem to be handled. This includes PDEs expressed natively in CUQIpy (detailed in the present section), using a third-party PDE library such as FEniCS [ 28 ] (see sections 3 and 4 ) and through a user-provided black-box PDE-solver (see section 5 ). The critical components of this framework are provided by the cuqi.pde module, which contains the PDE class and its subclasses, the supporting Geometry classes, and the PDEModel class, see table 1 .
Table 1. A subset of CUQIpy classes that support integrating PDE-based problems. For a comprehensive list of classes and modules, see the companion paper [ 35 ].
The PDE class provides an abstract interface for representing PDEs, a subclass hereof is LinearPDE representing linear PDE problems. At present two concrete classes have been implemented: SteadyStateLinearPDE and TimeDependentLinearPDE from which a broad selection of steady-state and time-dependent linear PDEs can be handled. The Geometry classes allow us to parametrize in terms of various expansions to enforce desired properties on the solution, such as smoothness or as a step-wise function. The PDEModel class provides the interface to use the PDE as a CUQIpy Model for Bayesian inference. A PDEModel combines a PDE with two Geometry classes for the domain and range geometry to form a forward model of an inverse problem.
We illustrate this framework by an example: a Bayesian inverse problem governed by a 1D heat equation, sections 2.1 – 2.5 . We emphasize that a much wider variety of PDEs can be handled; an example is used only for concreteness of the presentation.
2.1. The 1D heat equation inverse problem
2.2. the discretized heat equation in cuqipy.

We write the k th forward Euler step as follows

To represent this discretized PDE equation in CUQIpy, we need to create a PDE -type object that encapsulates the details of the PDE equation and provides an implementation of the operators S and O , table 1 . Creating a PDE -type class requires a user-provided function that represents the components of the PDE on a standardized form, denoted by PDE_form . For time-dependent problems, the PDE_form function takes as inputs the unknown parameter that we want to infer (in this case g ) and a scalar value for the current time: tau_current

For this 1D time-dependent heat equation, we create a TimeDependentLinearPDE object from the specific PDE_form and the time step vector tau and spatial grid grid :

2.3. The 1D heat forward problem in CUQIpy
We define the discretized forward model of the 1D heat inverse problem

We can now set up the PDE model as

2.4. Parametrization by the Geometry class

We can represent a function in this expansion by our fundamental data structure CUQIarray , which essentially contains a coefficient vector and the geometry, e.g.

A CUQIarray allows convenient plotting of the object in context of the geometry:

To employ the step function expansion we pass it as domain geometry:

We can print the model A , print(A) , and get:

2.5. Specifying and solving the PDE-based Bayesian inverse problem
We define the Bayesian inverse problem, assuming additive Gaussian noise, as:

We can draw five samples from the prior distribution and display these by:

Here, prior_samples is a Samples object with the generated samples. It obtains the Geometry -type object from the prior x , here G_step . The prior samples are seen in figure 4 (b). By default, the function values of the samples are plotted, i.e. the step functions.
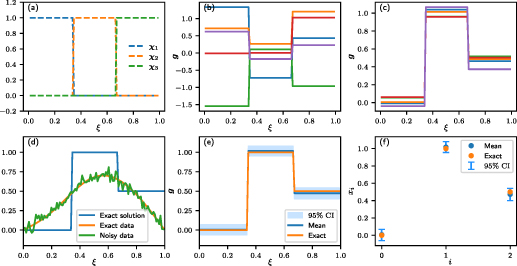
Calling print(joint) , for example, gives:
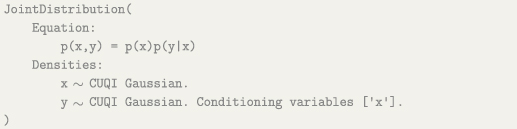
2.6. Posterior samples analysis, and visualization
A basic Samples operation is to plot selected samples (figure 4 (c)):

2.7. Parameterizing the initial condition using KL expansion
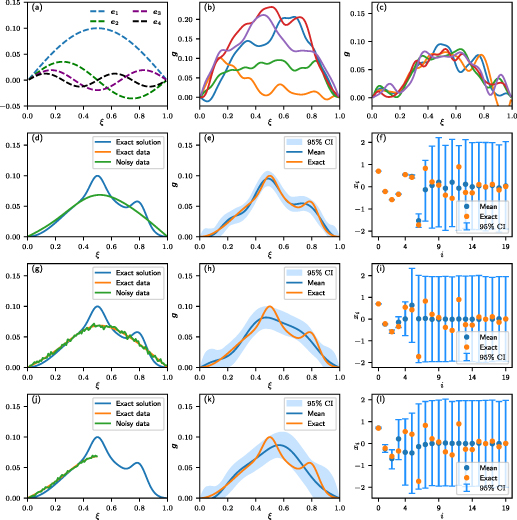
This concludes our overview of solving PDE-based Bayesian inverse problems with CUQIpy. We emphasize that the heat equation example was for demonstration and that the framework can be applied to wide variety of PDE-based inverse problems. In the next section, we show how to handle problems modeled in the FEM platform FEniCS.
3. CUQIpy-FEniCS plugin
FEniCS [ 28 ] is a popular Python package for solving PDEs using the FEM. The extent of its users, both in the academia and the industry, makes a dedicated CUQIpy interface for FEniCS highly desirable. Here we present our interface plugin: CUQIpy-FEniCS, and revisit the 2D Poisson example discussed in section 1.2 to unpack the underlying CUQIpy and CUQIpy-FEniCS components that are used in building the example. In section 4 , we present an elaborate test case of using CUQIpy together with CUQIpy-FEniCS to solve an EIT problem with multiple data sets and in section 5 we showcase using some of the CUQIpy-FEniCS features in solving a PAT problem with a user-provided forward model.
We use the main modules of FEniCS: ufl , the FEniCS unified form language module, and dolfin , the Python interface of the computational high-performance FEniCS C++ backend, DOLFIN . We can import these modules as follows:

The CUQIpy-FEniCS plugin structure can be adopted to create CUQIpy plugins integrating other PDE-based modeling libraries, e.g. the new version FEniCSx [ 36 ].
3.1. PDE -type classes
The CUQIpy-FEniCS plugin defines PDE -type classes, see table 2 , that represent PDE problems implemented using FEniCS. To view the underlying PDE -type class that is used in the 2D Poisson example, we call print(A.pde) , where A is the CUQIpy PDEModel defined in section 1.2 , and obtain:

Table 2. Modules and classes of the CUQIpy-FEniCS plugin.
Specifically, the Poisson PDE is represented by the SteadyStateLinearFEniCSPDE class. Similar to the core CUQIpy PDE -type classes, a CUQIpy-FEniCS PDE -type class contains a PDE form , which is a user-provided Python function that uses FEniCS syntax to express the PDE weak form; more discussion on building weak forms is provided in section 4 in the context of the EIT example. The Python function form inputs are w , the unknown parameter (log-conductivity in the 2D Poisson example), u , the state variable (or trial function), and p , the adjoint variable (or test function); and f is the FEniCS expression of the source term.
The CUQIpy-FEniCS PDE -type classes follow the interface defined by the core CUQIpy abstract PDE class by implementing the methods assemble , solve , and observe .
The assemble method builds the discretized PDE system to be solved, from the provided PDE form. In the Poisson example, section 1.2 , the system that results from discretizing the weak form of the PDE ( 1 ) using the FEM can be written as:
For brevity we do not provide code for building the SteadyStateLinearFEniCSPDE object here, as it is provided by the CUQIpy-FEniCS test problem FEniCSPoisson2D , and stored in A.pde . How to build PDE -like objects is shown in section 2 for the core CUQIpy, and in section 4 for the CUQIpy-FEniCS plugin.
3.2. Geometry -type classes in CUQIpy-FEniCS
Geometry -type classes, as we discussed in section 2 , mainly serve three purposes. First, they interface forward models with samplers and optimizers by providing a dual representation of variables: parameter value and function values representations. Second, they provide visualization capabilities for both representations. Lastly, they allow re-parameterizing the Bayesian inverse problem parameter, e.g. in terms of coefficients of expansion for a chosen basis. CUQIpy-FEniCS Geometry -type classes serve the same goals, see table 2 for a list of these classes.
There are two main data structures in FEniCS, Function and Vector . The former is a class representation of FEM approximation of continuous functions, and the latter is a class representation of the approximation coefficients of expansion. CUQIpy-FEniCS Geometry -type classes, subclassed from cuqi.geometry.Geometry , interpret these data structures and interface them with CUQIpy. Additionally, they provide plotting methods by seamlessly utilizing the FEniCS plotting capabilities. This enables CUQIpy-FEniCS to visualize function value representation of variables, as in figure 1 (a), as well as, parameter value representation of variables, as in figure 1 (f). The plotting implementation details are hidden from the user; and the user is provided with the CUQIpy simple plotting interface as shown for example in section 2 .

mesh is the FEniCS computational mesh representing the physical domain of the problem, and parameter_space is a FEniCS first-order Lagrange polynomial space defined on mesh . We are now ready to create the FEniCSContinuous object as follows

3.3. Integration into CUQIpy through the PDEModel class
The CUQIpy-FEniCS PDE -type and Geometry -type objects provide the building blocks required to create the forward map A , e.g. ( 2 ). The CUQIpy PDEModel combines these FEniCS-dependent objects and interface them to the core CUQIpy library. We run print(A) , where A is the CUQIpy model defined in section 1.2 to see its contents:

We note its domain geometry (in the first line) corresponds to G_KL and its range geometry (second line) is G_FEM . We write A in terms of its components as:
PDEModel provides the forward method that corresponds to applying A . Additionally, it provides a gradient method to compute the gradient of A with respect to the parameter x , if the underlying Geometry and PDE objects have gradient implementation. This enables gradient-based posterior sampling such as by the NUTS sampler which we use in section 1.2 .
The following section gives an elaborate case study of using CUQIpy-FEniCS to solve an EIT problem. We provide details of constructing the software components needed to build and solve the problem using CUQIpy equipped with CUQIpy-FEniCS.
4. CUQIpy-FEniCS example: EIT
EIT is an imaging technique of inferring the conductivity of an object from measurements of the electrical current on the boundary. This is a non-invasive approach in medical imaging for detecting abnormal tissues. Similar techniques are used in many other applications, such as industrial inspection. The underlying mathematical model for EIT is an elliptic PDE. Such PDEs are some of the most popular models for PDE-based inverse problems, e.g. in modeling subsurface flow in a porous medium, and inverse steady-state heat transfer problem with unknown heat conductivity. Hence, the EIT model can be modified for use in a wide range of PDE-based inverse problems.
Inferring discontinuous fields is a common problem in many inverse problems applications. Such fields are used, for example, to model tumors in medical imaging applications, abnormalities in fault detection applications, and inhomogeneity in geophysics applications. A classic approach to incorporate such fields into a Bayesian inverse problem is to use a Markov random field (MRF)-type prior, discussed in detail in [ 35 ]. However, such priors often result in a large set of parameters, yielding inefficient numerical uncertainty quantification methods for fine discretization levels. Recently, a new class of Bayesian priors has emerged where a discontinuous field is constructed using a non-linear transformation of a continuous prior [ 2 , 13 , 24 ]. A continuous prior can be constructed with a relatively few parameters, e.g. using a KL expansion. A deterministic non-linear transformation is then chosen prior to inference to capture the properties of the unknown field being inferred. Therefore, efficient numerical inference methods can be constructed. The level-set prior [ 24 ] is one such prior where discontinuities in the field are defined to be the zeros level-set of a smooth Gaussian random field, e.g. a KL expansion. In this EIT example, we utilize the Bayesian level-set approach to perform uncertainty quantification for the EIT problem.
4.1. Mathematical model of EIT
4.2. finite element discretization and fenics implementation of eit.

We now implement the observation function ( 21 ). Let give_bnd_vals be a Python function that computes function values at the boundaries of Γ. The observation function then takes the form

The FEM discretization of ( 20 ) results in a finite-dimensional system of equations
and the discretized observation model
We now define the EIT forward maps A k to be
4.3. Parameterization of the conductivity σ
In this section we consider the level-set parameterization for the conductivity σ proposed in [ 13 ]. This approach comprises multiple layers of parameterization. In this section we use the geometry module in CUQIpy-FEniCS to implement such layered parameterization.
Let us first define the FEniCS function space in which we expect σ to belong

Here, mesh is the computational mesh used for FEniCS. Recall that parameter_space is a FEniCS function space with linear continuous elements. Similarly, we can define a FEniCS function space solution_space on which the solutions v k defines a function.
Now we define a discrete Gaussian random field r defined on Γ, i.e. realizations of r are FEM expansion coefficients of a random functions defined on Γ. One way to define such a random function is to use a truncated KL-expansion with a Matérn covariance, as discussed in section 3.2 , to approximate r the same way w is approximated in ( 14 ).

We can construct this parameterization in CUQIpy-FEniCS as

Here, heaviside is a Python function that applies the Heaviside map ( 25 ), see the companion code for more implementation details. By passing map = heaviside , FEniCSMappedGeometry applies heviside to G_KL .
We redefine the forward operators to use the parameterizations discussed:
We define the range geometry as a Continuous1D geometry:

4.4. PDEmodel for the EIT problem
Now we have all the components to define a CUQIpy-FEniCS PDE object . We first create a PDE form by combining the left-hand-side and right-hand-side forms, defined in section 4.2 , in a Python tuple as

Since ( 19 ) is a steady state linear PDE, we use the SteadyStateLinearFEniCSPDE class to define this PDE in CUQIpy-FEniCS.

Now we can create a PDEModel that represents the forward operator A 1 and includes information about the parameterization of σ .

4.5. Bayesian formulation and solution
Here, s noise is the standard deviation of the data distribution. We use CUQIpy to implement these distributions.

Examples of prior samples can be found in figure 7 . Note that CUQIpy-FEniCS visualizes these samples as FEniCS functions.
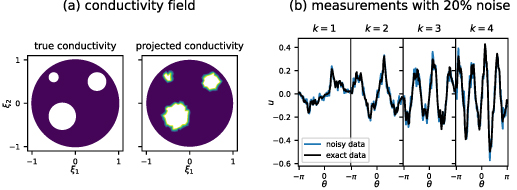
Now we have all the components we need to create a posterior distribution. We first define the joint distribution

In this test case, we use the standard Metropolis-Hastings (MH) algorithm [ 35 , § 2] to sample from the posterior. We pass the posterior as an argument in the initialization and then compute 10 6 samples using this sampler.

In what remains in this section we discuss how to use posterior_samples in CUQIpy-FEniCS to visualize the posterior distribution.
4.6. Posterior samples, post-processing and visualization
We analyze and visualize the posterior using CUQIpy equipped with CUQIpy-FEniCS geometries. We first plot some of the posterior samples using the plot method.

Figure 7. Samples from the prior and posterior distributions of σ . First column: prior samples. Second, third and fourth columns: posterior samples of σ with 5%, 10% and 20% noise level, respectively.
Now we want to estimate and visualize the posterior mean as an estimate for the conductivity field σ . We can achieve this using the command

Figure 8. Estimated conductivity field σ with uncertainty estimates, visualized as Heaviside-mapped KL expansion. (a) Posterior mean (b) point-wise variance.
We use the point-wise variance of the posterior samples as a method for quantifying the uncertainty in the posterior. We can achieve this in CUQIpy-FEniCS by

Finally we visualize the posterior for the expansion coefficients x . We use plot_ci method to visualize the posterior mean and the 95% CIs associated with the parameters. To indicate that we are visualizing the posterior for the coefficient (parameter) x , we pass the argument plot_par = True to the plot_ci method.

Figure 9. Estimation of x , i.e. the first 35 coefficients of the KL expansion in ( 14 ) and their uncertainty. (a) 5% noise (b) 10 % noise (c) 20 % noise.
5. PAT through user-defined PDE models
In many applications of UQ for inverse problems a well-developed forward problem solver is created by the user. Therefore, it is of high interest that CUQIpy and CUQIpy-FEniCS can incorporate such black-box forward solvers.
In this section we discuss how to use a black-box code in CUQIpy and CUQIpy-FEniCS to quantify uncertainties for inverse problems with PDEs. In addition, we discuss how to exploit geometries in CUQIpy-FEniCS, in such cases, to visualize uncertainties, without modifying the original black-box software.
To demonstrate the user-defined features of CUQIpy and CUQIpy-FEniCS, we consider a 1D PAT problem [ 41 ]. In such problems, a short light pulse is illuminated onto an object to create a local initial pressure distribution. This pressure distribution then propagates in the object in the form of ultrasound waves. The PAT problem is then to reconstruct the initial pressure distribution from time-varying ultrasound measurements. For the 1D variant, we consider a 1D pressure profile with r = 2 ultrasound sensors to measure pressure variations.
5.1. Mathematical model of PAT
Let us consider an infinitely long 1D acoustic object with homogeneous acoustic properties (homogeneous wave speed). Assuming that the illumination duration via a light pulse is negligible compared to the speed of wave propagation, we can approximate the propagation of waves in the object by the hyperbolic PDE (linear wave equation)
Here, u is the pressure distribution, g the initial pressure distribution, and τ the time.
5.2. CUQIpy implementation of PAT
We consider a simple Bayesian problem where we assume the components of g have a Gaussian distribution and the pressure measurements are corrupted by additive white Gaussian noise. We can formulate this problem as
We set up the Bayesian Problem with Gaussian prior and data distribution:

Note that CUQIpy, by default, considers Continuous1D geometry for the initial pressure. Therefore, we do not explicitly define it. When incorporating black-box forward solvers within CUQIpy, the user has access to complex priors for g , following examples in the companion paper [ 35 ]. As an example, when the location of jumps in the initial pressure is the quantity of interest in the inverse problem, we can consider a Markov-random-field-type prior. Using such prior distributions is discussed in [ 35 ].

Instead of constructing the posterior and sampling it, we wish to demonstrate how to formulate this same problem using the CUQIpy-FEniCS plugin. Since sampling is done in the same way, we demonstrate it at the end of the following section.
5.3. CUQIpy-FEniCS implementation of PAT
Here, we assume that PAT is a Python function with a FEniCS implementation of the forward operator A . We also assume this function take a FEniCS function g as input and computes the boundary pressure measurement y . Let us first define the FEniCS function space where g defines a function

We set up a geometry for the KL-expansion with CUQIpy-FEniCS (see section 3.2 ) as

We construct a continuous 2D geometry for the observations in which one axis represents the observation times and the other axis represents the sensor locations.

where obs_times and obs_locations are arrays of the observation times and locations. Now we create a CUQIpy model to encapsulate the forward operator PAT with the parametrization, represented by the domain geometry, and the range geometry,

Note that in creating this model we are treating PAT as a black-box function. Now, CUQIpy-FEniCS can utilize the information about domain and range geometries to allow advanced sampling and visualization.
The parameterized Bayesian problem for the PAT now takes the form

In this example we consider the same s_noise , as well as, the same noisy data y_obs as in section 5.2 . Similar to the previous sections we now construct the joint and the posterior distributions.

We can visualize the posterior for g , i.e. the initial pressure distribution, by

This plots the mean function for g and the CIs associated with this estimate in figure 11 . We see that the mean function, in the case with complete data, is a better estimate for the true initial pressure profile compared to the case with partial data. Furthermore, when data corresponding the right boundary is missing, the uncertainty in estimating the right boundary increases.
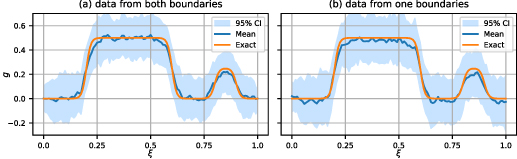
Figure 11. Estimated initial pressure profile g together with the uncertainty estimates. The plots correspond to the (a) full data and (b) partial data.
Finally, we plot the mean and 95% CI for the expansion coefficients x :

In figures 12 (a) and (b) we present the CI plots for the full data (from both boundaries) and the partial data (only from the left boundary), respectively. Note that we only show the first 25 components although we estimate all 100 parameters. We see that the uncertainty of the first coefficient significantly increases for the case with partial data.
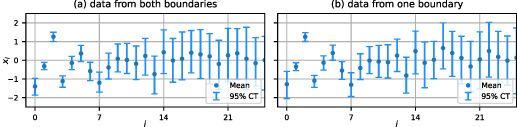
Figure 12. Estimation of the first 25 components of x , i.e. KL expansion coefficients in ( 14 ), and the uncertainty in this estimation. (a) Full data and (b) partial data.
6. Conclusion and future work
In this paper we described our general framework for modeling and solving PDE-based Bayesian inverse problems with the CUQIpy Python software package.We showed how to express PDEs natively in CUQIpy, or using a user-provided black-box PDE solver. We also showed how to formulate statistical assumptions about unknown parameters using CUQIpy and conduct Bayesian inference and uncertainty quantification. We also presented our CUQIpy-FEniCS plugin as an example of how to incorporate modeling by third-party PDE libraries such as the finite-element modeling package FEniCS.
We showed that CUQIpy and CUQIpy-FEniCS provide a consistent and intuitive interface to model and solve PDE-based Bayesian inverse problems, as well as analyze and visualize their solutions. Results were shown for parabolic, elliptic and hyperbolic examples involving the heat and Poisson equations as well as application case studies in EIT and PAT.
Future work includes expanding support for derivatives across distributions, forward models, and geometries, as well as integrating PyTorch automatic differentiation into CUQIpy through the CUQIpy-PyTorch plugin. This will simplify the use of gradient-based samplers such as NUTS, as in the Poisson example in this paper, to help address the computational challenge of MCMC-based sampling of high-dimensional and complicated posterior distributions arising in large-scale inverse problems. The extensible plugin structure can also be used to integrate more PDE-based modeling libraries.
Overall, we believe CUQIpy and its plugins provide a promising platform for solving PDE-based Bayesian inverse problems and have a significant potential for further development and expansion in the future.
Acknowledgments
This work was supported by The Villum Foundation (Grant No. 25893). J S J would like to thank the Isaac Newton Institute for Mathematical Sciences for support and hospitality during the programme 'Rich and Nonlinear Tomography—a multidisciplinary approach' when work on this paper was undertaken. This work was supported by EPSRC Grant Number EP/R014604/1. This work was partially supported by a grant from the Simons Foundation (J S J). F U has been supported by Academy of Finland (Project Number 353095). The authors are grateful to Kim Knudsen for valuable input in regards to PDE-based inversion in medical imaging, to Aksel Kaastrup Rasmussen for a helpful discussion about the EIT problem formulation and to members of the CUQI project for valuable input that helped shape the design of CUQIpy.
Data availability statements
CUQIpy and plugins are available from https://cuqi-dtu.github.io/CUQIpy . The code and data to reproduce the results and figures of the present paper are available from https://github.com/CUQI-DTU/paper-CUQIpy-2-PDE . The data that support the findings of this study are openly available at the following URL/DOI: https://zenodo.org/doi/10.5281/zenodo.10512535 .
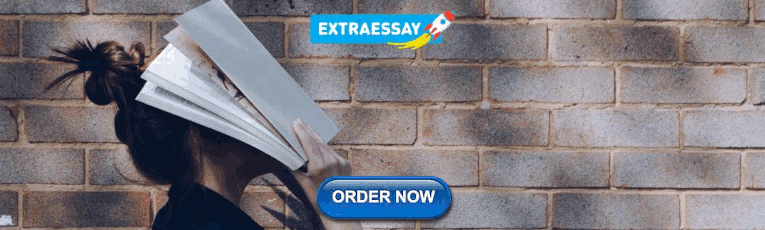
COMMENTS
Computational Thinking. 1. Finding the one definition of Computational Thinking (CT) is a task doomed to failure, mainly because it is hard to have a consensus on what CT means to everyone. To ease the communication and have a shared meaning, we consider CT as an approach to "solve problems using computers as tools" (Beecher, 2017).
14.5 Solve Systems of Linear Equations in Python. 14.6 Matrix Inversion. 14.7 Summary and Problems. CHAPTER 15. Eigenvalues and Eigenvectors ¶ 15.1 Eigenvalues and Eigenvectors Problem Statement. 15.2 The Power Method. 15.3 The QR Method. 15.4 Eigenvalues and Eigenvectors in Python.
How to solve a linear programming problem with Python; ... Linear programming is a set of mathematical and computational tools that allows you to find a particular solution to this system that corresponds to the maximum or minimum of some other linear function. ... The approach for defining and solving the problem is the same as in the previous ...
By the end of this book, you'll have an arsenal of practical coding solutions that can be used and modified to solve a wide range of practical problems in computational mathematics and data science. What you will learn. Become familiar with basic Python packages, tools, and libraries for solving mathematical problems
Computational thinking is a problem-solving process in which the last step is expressing the solution so that it can be executed on a computer. However, before we are able to write a program to implement an algorithm, we must understand what the computer is capable of doing -- in particular, how it executes instructions and how it uses data.
Python Practice Problem 5: Sudoku Solver. Your final Python practice problem is to solve a sudoku puzzle! Finding a fast and memory-efficient solution to this problem can be quite a challenge. The solution you'll examine has been selected for readability rather than speed, but you're free to optimize your solution as much as you want.
In this course, you will learn about the pillars of computational thinking, how computer scientists develop and analyze algorithms, and how solutions can be realized on a computer using the Python programming language. By the end of the course, you will be able to develop an algorithm and express it to the computer by writing a simple Python ...
Apply computational thinking in domains, such as cryptography, and machine learning; Who this book is for. This book is for students, developers, and professionals looking to develop problem-solving skills and tactics involved in writing or debugging software programs and applications. Familiarity with Python programming is required.
MIT's 18-week online course teaches computational thinking and Python programming, covering algorithms, debugging, and data science. Ideal for beginners in computer science. ... use computation to accomplish a variety of goals and provides you with a brief introduction to a variety of topics in computational problem solving . This course is ...
The new edition of an introduction to the art of computational problem solving using Python.This book introduces students with little or no prior programming... Skip to content. Books. Column. View all subjects; New releases ... With Application to Computational Modeling and Understanding Data. by John V. Guttag. Paperback. $75.00. Paperback ...
Introduction to Computer Science Using Python: A Computational Problem-Solving Focus introduces students to programming and computational problem-solving via a back-to-basics, step-by-step, objects-late approach that makes this book easy to teach and learn from. Students are provided with a thorough conceptual grounding in computational problem solving before introducing them to specific ...
An exceptionally broad range of topics, from simple matrix manipulations to intricate computations in nonlinear dynamics. A whole suite of supplementary material: Python programs, Jupyter notebooks and videos. Computational Physics is ideal for students in physics, engineering, materials science, and any subjects drawing on applied physics.
computational problem solving skills. Course Objectives: ⚫ Learn basics of computer programming. ⚫Learn how to solve a given problem. ⚫Learn to use various paradigms of programming. ⚫Learn to test and debug python code. Course Outcomes: At the end of the course, the student will be able to: ⚫ Illustrate problem solving using Python ...
Python Practice Problem 1: Average Expenses for Each Semester. John has a list of his monthly expenses from last year: He wants to know his average expenses for each semester. Using a for loop, calculate John's average expenses for the first semester (January to June) and the second semester (July to December).
Step 4 - Solving or Simplification. Once we have laid out the steps to solve the problem, we try to find the solution to the question. If the solution cannot be found, try to simplify the problem instead. The steps to simplify a problem are as follows: Find the core difficulty.
Instead of solving the problem with the numerical-analytical validation, we only demonstrate how to solve the problem using Python, Numpy, and Matplotlib, and of course, with a little bit of simplistic sense of computational physics, so the source code here makes sense to general readers who don't specialize in computational physics. Preparation
14.3.3 Developing and Solving Optimization Problems with Python. To formulate and solve optimization problems using Python, we follow a similar process as in GAMS. The first step is to gain a clear understanding of the problem at hand. This involves defining the structure of the program, equations, variables, and numerical data.
The same but different. Source: author. Creating a separate but related problem can be a very effective technique in problem solving. It is particularly relevant where you have expertise/resources/skills in a particular area and want to exploit this.
Introduction to Computer Science Using Python: A Computational Problem-Solving Focus introduces readers to programming and computational problem-solving via a back-to-basics, step-by-step, objects-late approach that makes this book easy to teach and learn from. Readers are provided with a thorough conceptual grounding in computational problem solving before introducing them to specific Python ...
This page includes the content for PX1224: Computational Skills for Problem Solving. The course uses the Python programming language and the Jupyter development environment. Why learn computational skills? Most real world physics and astronomy problems are difficult to solve exactly using the mathematical techniques that you are learning.
Dierbach's step-by-step pedagogical approach makes this an accessible and reader-friendly introduction to programming that eases readers into program-writing through a variety of hands-on exercises. Introduction to Computer Science Using Python: A Computational Problem-Solving Focus introduces readers to programming and computational problem-solving via a back-to-basics, step-by-step, objects ...
The use of computation and simulation has become an essential part of the scientific process. Being able to transform a theory into an algorithm requires significant theoretical insight, detailed physical and mathematical understanding, and a working level of competency in programming. This upper-division text provides an unusually broad survey of the topics of modern computational physics ...
The best way to learn is by practising it more and more. The best thing about this Python practice exercise is that it helps you learn Python using sets of detailed programming questions from basic to advanced. It covers questions on core Python concepts as well as applications of Python in various domains.
Computational Physics provides the reader with the essential knowledge to understand computational tools and mathematical methods well enough to be successful. Its philosophy is rooted in "learning by doing", assisted by many sample programs in the popular Python programming language.
The scientific problem-solving paradigm. A problem is set, the tools from multiple disciplines are employed within context, and the continual assessment aides debugging and steering. A key component of many computational programs is having students get actively engaged with projects as if each were an original scientific investigation, and ...
Problem solving (PS), a component of critical thinking (Chaisri et al., 2019; Kuo et al., 2020), is a form of human intelligence that uses a structural phase to find an unknown or developing answer (Jones-Harris & Chamblee, 2017; Polya, 1981); PS organizes thoughts and processes to find a solution.Problem solving is a human skill that is required to deal with the complexity of problems (Durak ...
This Review provides an introductory guide to artificial intelligence (AI)-based tools for non-computational cancer researchers. Here, Perez-Lopez et al. outline the general principles of AI for ...
The aim of this work is to introduce a thermo-electromagnetic model for calculating the temperature and the power dissipated in cylindrical pieces whose geometry varies with time and undergoes large deformations; the motion will be a known data. The work will be a first step towards building a complete thermo-electromagnetic-mechanical model suitable for simulating electrically assisted ...
New approach makes calculations with realistic interactions possibleFRIB researchers are part of an international research team solving challenging computational problems in quantum physics using a new method called wavefunction matching. The new approach has applications to fields such as nuclear physics, where it is enabling theoretical calculations of atomic nuclei that were previously not ...
All CUQIpy -type classes implement three methods: (1) assemble, which performs any assembling that might be needed to prepare the matrices and vectors required to solve the PDE problem, (2) solve, which solves the PDE problem using the assembled components and is equivalent to applying the parameter-to-solution operator S, and (3) observe ...