- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
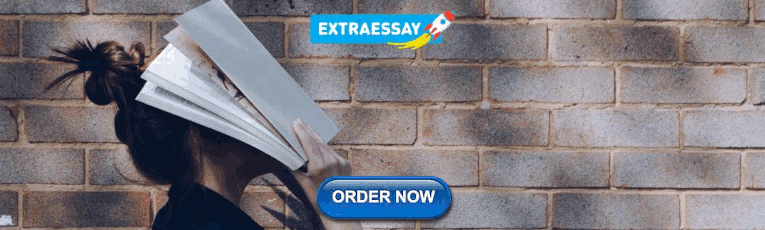
Writing First C++ Program – Hello World Example
C++ is a widely used Object Oriented Programming language and is relatively easy to understand. The “Hello World” program is the first step towards learning any programming language and is also one of the most straightforward programs you will learn.
The Hello World Program in C++ is the basic program that is used to demonstrate how the coding process works. All you have to do is display the message “Hello World” on the console screen.
To write and run C++ programs, you need to set up the local environment on your computer. Refer to the complete article Setting up C++ Development Environment . If you do not want to set up the local environment on your computer, you can also use online IDE to write and run your C++ programs.
C++ Hello World Program
Below is the C++ program to print Hello World.
Working of Hello World Program in C++
Let us now understand every line and the terminologies of the above program.
1. // C++ program to display “Hello World”
This line is a comment line. A comment is used to display additional information about the program. A comment does not contain any programming logic.
When a comment is encountered by a compiler, the compiler simply skips that line of code. Any line beginning with ‘//’ without quotes OR in between /*…*/ in C++ is a comment. Click to know More about Comments.
2. #include
This is a preprocessor directive. The #include directive tells the compiler to include the content of a file in the source code.
For example, #include<iostream> tells the compiler to include the standard iostream file which contains declarations of all the standard input/output library functions. Click to Know More on Preprocessors.
3. using namespace std
This is used to import the entity of the std namespace into the current namespace of the program. The statement using namespace std is generally considered a bad practice. When we import a namespace we are essentially pulling all type definitions into the current scope.
The std namespace is huge. The alternative to this statement is to specify the namespace to which the identifier belongs using the scope operator(::) each time we declare a type. For example, std::cout. Click to know More about using namespace std.
4. int main() { }
A function is a group of statements that are designed to perform a specific task. The main() function is the entry point of every C++ program, no matter where the function is located in the program.
The opening braces ‘{‘ indicates the beginning of the main function and the closing braces ‘}’ indicates the ending of the main function. Click to know More about the main() function.
5. cout<<“Hello World”;
std::cout is an instance of the std::ostream class, that is used to display output on the screen. Everything followed by the character << in double quotes ” ” is displayed on the output device. The semi-colon character at the end of the statement is used to indicate that the statement is ending there. Click to know More on Input/Output.
6. return 0
This statement is used to return a value from a function and indicates the finishing of a function. This statement is basically used in functions to return the results of the operations performed by a function.
7. Indentation
As you can see the cout and the return statement have been indented or moved to the right side. This is done to make the code more readable. We must always use indentations and comments to make the code more readable. Must read the FAQ on the style of writing programs.
Important Points
- Always include the necessary header files for the smooth execution of functions. For example, <iostream> must be included to use std::cin and std::cout .
- The execution of code begins from the main() function.
- It is a good practice to use Indentation and comments in programs for easy understanding.
- cout is used to print statements and cin is used to take inputs.
Similar Reads
- School Programming
- CBSE - Class 11
- school-programming
Please Login to comment...
- How to Watch NFL on NFL+ in 2024: A Complete Guide
- Best Smartwatches in 2024: Top Picks for Every Need
- Top Budgeting Apps in 2024
- 10 Best Parental Control App in 2024
- GeeksforGeeks Practice - Leading Online Coding Platform
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

- Assignment #0: Hello World
Assignment #0: Hello World ¶
Your first assignment is mostly an exercise in following instructions. We'll ask you to modify an existing program so that, when run, the program prints "Hello world!".
Programming jobs often require you to get familiar with, or at least make use of, additional technologies. Here, you'll be using a few web applications, like GitHub and Repl.it, to write your code, run it, save it, and submit your assignment.
So while the coding task may appear straightforward, you'll be introduced to a set of instructions, or workflow , that is important for the rest of your success in this class.
Read this whole page before you start taking any action.
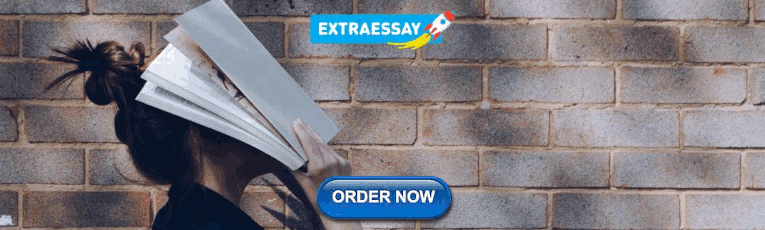
Requirements ¶
Sign up for a GitHub account.
Sign up for a Repl.it account.
Open and accept the GitHub classroom assignment invitation.
Change the code in Repl.it.
Commit the code in Repl.it.
Make sure your assignment passes the test.
If you haven't done so already, create a GitHub account .
If you haven't already done so, create a Repl.it account .
Assignment Invitation ¶
Before starting on any assignment, make sure you are signed into both your Github and Repl.it accounts!
In your Canvas classroom, click on the Assignment 0 link to accept your invitation to start coding your assignment solution.
Once you open the assignment invitation, if you are already logged in to your GitHub account, you will see a screen like this:
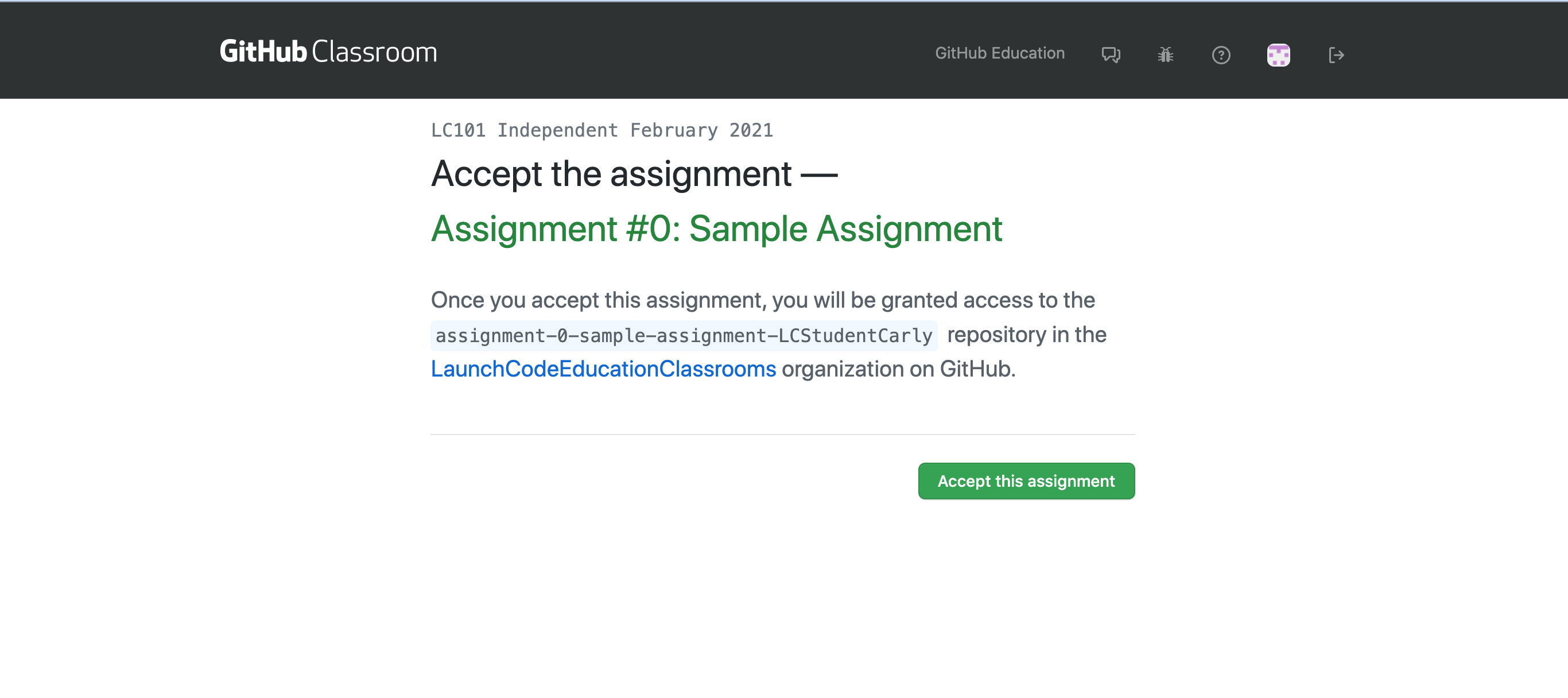
Clicking on the assignment link in Canvas takes you to this page. ¶
Before you hit the green Accept the Assignment button, write down the name of the assignment repository. In this case, that would be: "assignment-0-sample-assignment-LCStudent-Carly". A repository is a Github term for a folder of code. Having the repository name written somewhere will ensure you can access your assignment code if you are interrupted from the rest of the setup process.
Once you have accepted and hit the green button, the next page you will see looks something like this:
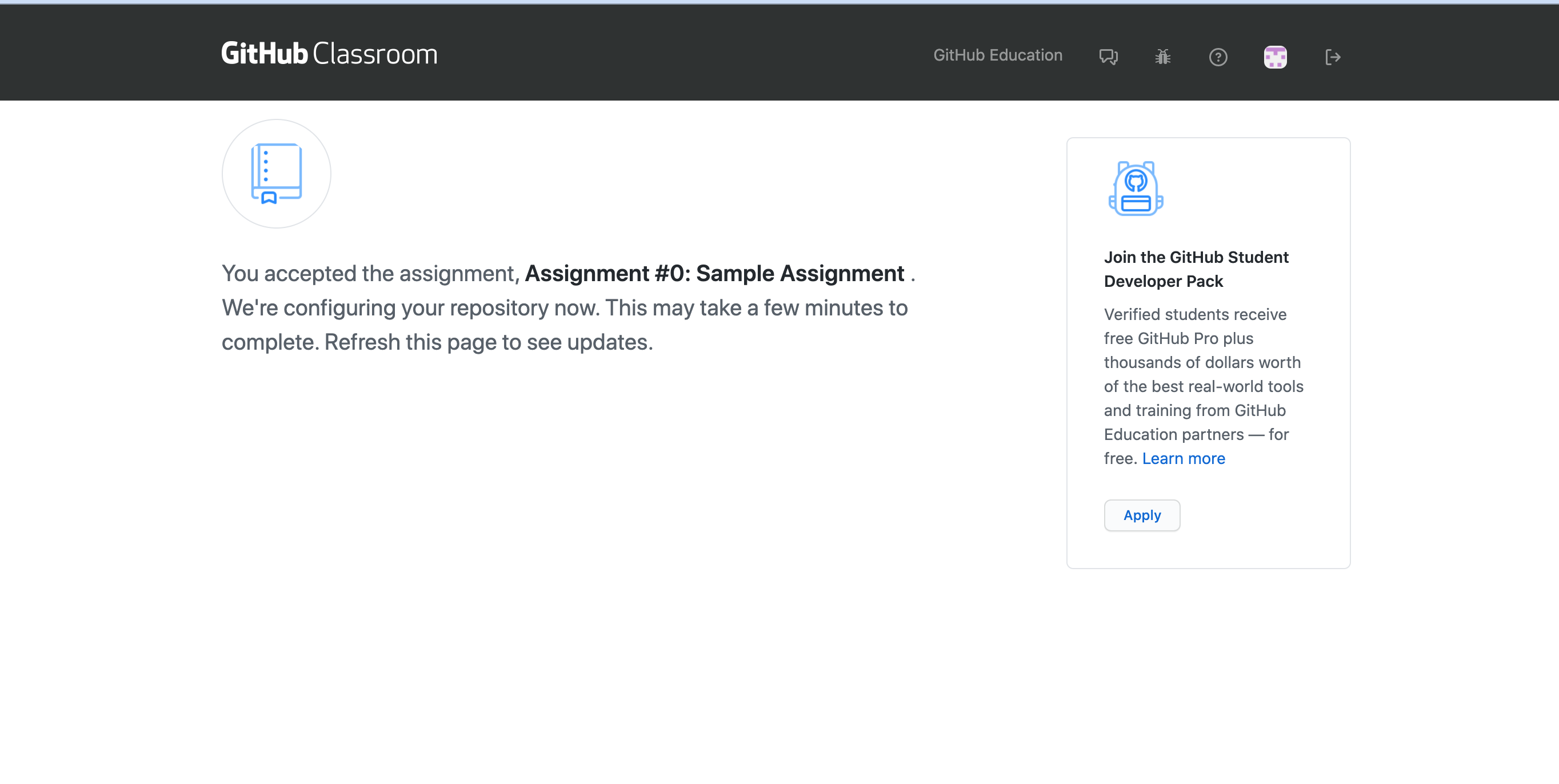
Most of the time, you only need to wait about 30 seconds to refresh and see the assignment repository. ¶
When you refresh and your assignment repository has been created, this is what you should see:
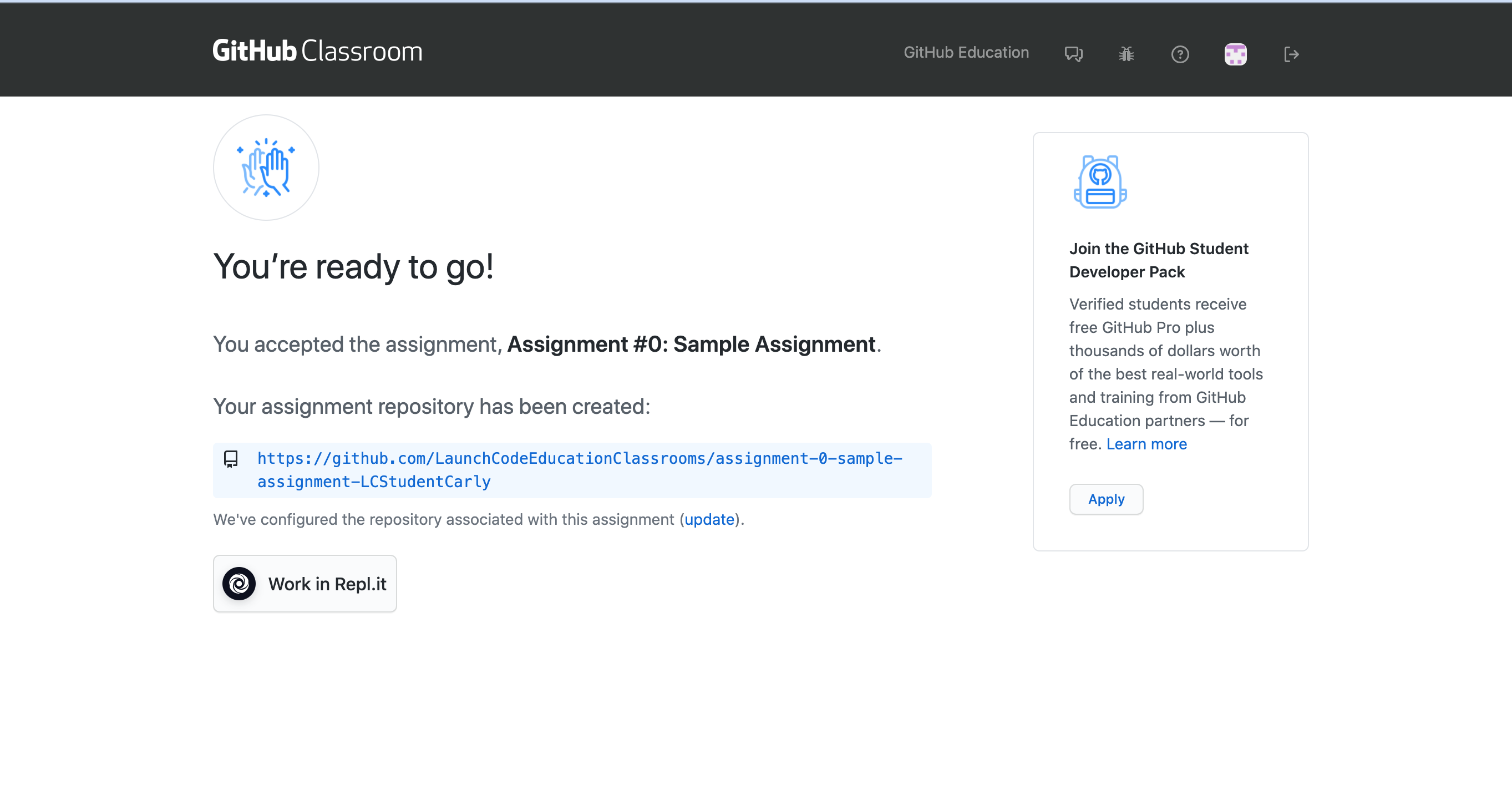
Your assignment code can now be accessed either via Repl.it or GitHub. ¶
We recommend you first open the GitHub assignment repository page before hitting the Work in Repl.it button. When you open your GitHub assignment repository, you will see the same Work in Repl.it button.
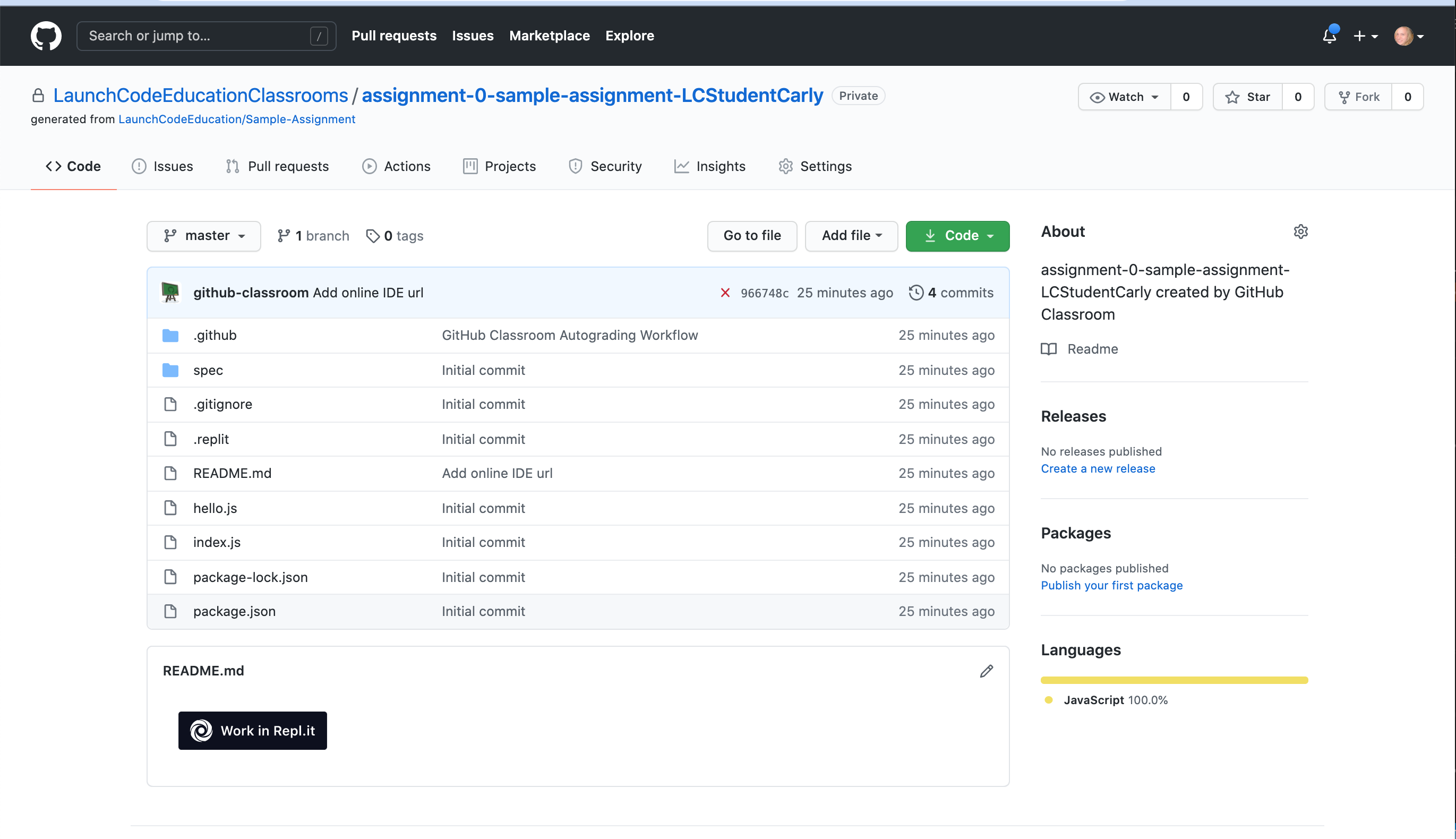
We recommend that you access the assignment Repl.it via the GitHub repository. ¶
The first time you use the Work in Repl.it button, i.e. for this assignment, you will need to authorize your Repl.it account to work with your GitHub account. If you are prompted to do so, follow the steps to link your accounts.
Hello world! ¶
Once you have accepted the assignment, have a look around the various files and get curious about what this code does. Don't be afraid to look - just don't edit any of this code just yet.
After you have done some exploring, use the Repl.it Run button to run the program. To begin with, the program prints just "Hello" . Remember, your task is to to modify the output of this program so that, when run, "Hello world!" is printed.
Take a look at the hello.js file. This is the only file in your starter code that needs changing. You'll see a structure called a function , along with a final module.exports line. We haven't learned about either of these items yet - but we will! If you are so compelled, google these terms to get a brief introduction. If you are not compelled, you don't need to know what they do for the purposes of this class until later lessons.
Set a timer for 5 mins and google search "JavaScript functions" or "JavaScript module.exports". Or look these items up directly on W3Schools or MDN . When the timer is up, write down whatever you have found interesting or most salient from your search.
Although you won't start learning about these terms in this book until later on, getting into the habit of exploring code and google searching topics helps to get you into the developer mindset.
This may not be the only place where this book leaves you with more questions than answers and getting answers from the world wide web is how every developer spends their time.
Back inside of hello.js , you should recognize a string on this line: return "Hello!"; .
return is another keyword related to functions that we have not yet covered. For now, know that return behaves like a surrogate for console.log() . So anything inside of the string that follows it will be printed when your application is run.
It's a good idea to take note of how this file in the starter code looks before you start editing. Changing any code other than this string may cause your program to not run properly, and therefore may not be graded correctly. Making small changes and testing them as you go makes it easier to undo changes you don't want and return to a working state.
With all of this in mind, modify the string on that line so that "Hello world!" is printed.
Commit Your Changes ¶
Now that your program prints "Hello world!" , you'll commit your code. Committing your code is part of a process called version control, which we'll get into in a later lesson. For now, go to the sidebar in your Repl.it window and click on the version control icon.
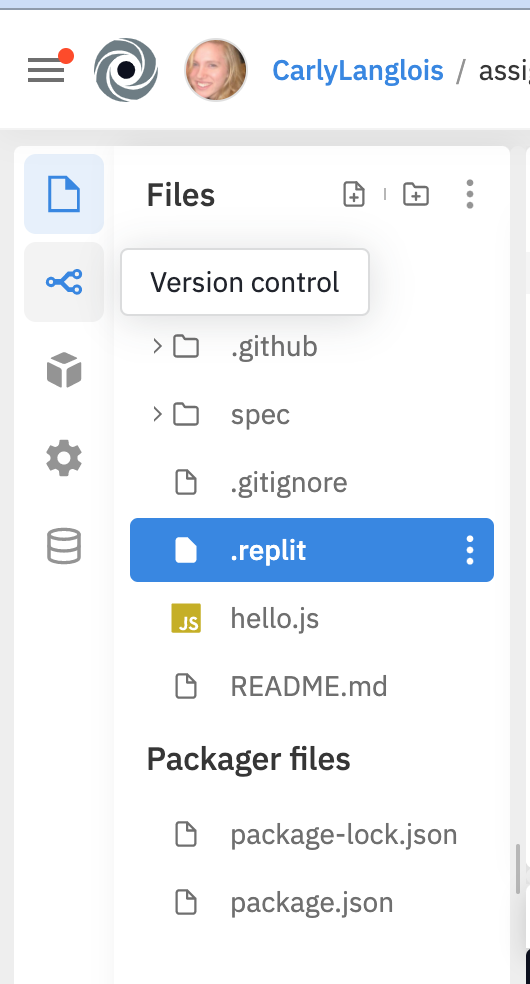
Here, the user selects the version control icon. ¶
When you have opened the version control tab, write a commit message in the text field that prompts "What did you change?". A commit message is a note about what you have changed in your code.
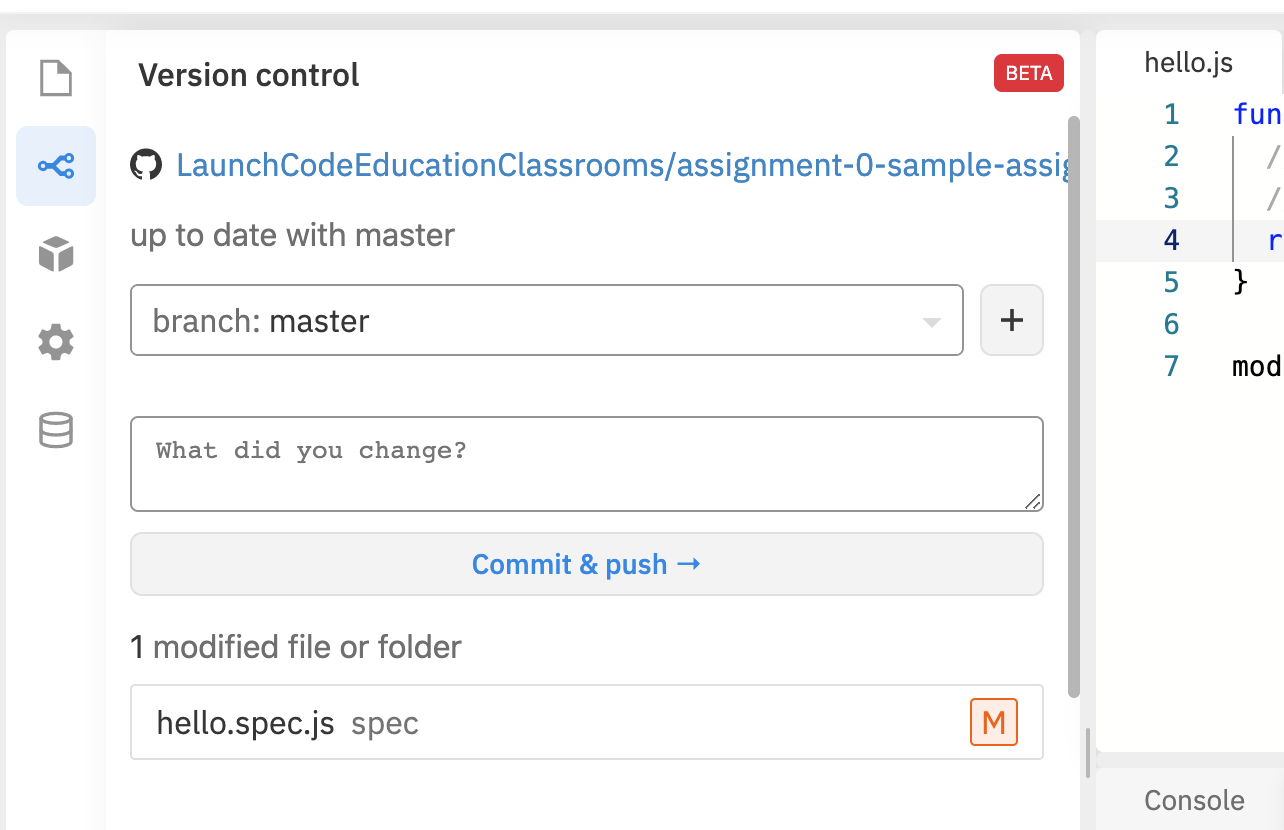
Click on the text box and write a message that conveys what you have changed in the code. ¶
A good commit message in this case would be something like: "Added my personal solution", or "Updated hello.js to print 'hello world!'".
Hit the commit & push button. Voila - your solution is submitted (pushed) to the GitHub graders.
Check Your Solution ¶
Once you've committed your work, head to Github to see that your solution passes the tests.
The easiest way to do this is to click on the hyperlink at the top of the Repl.it version control tab.
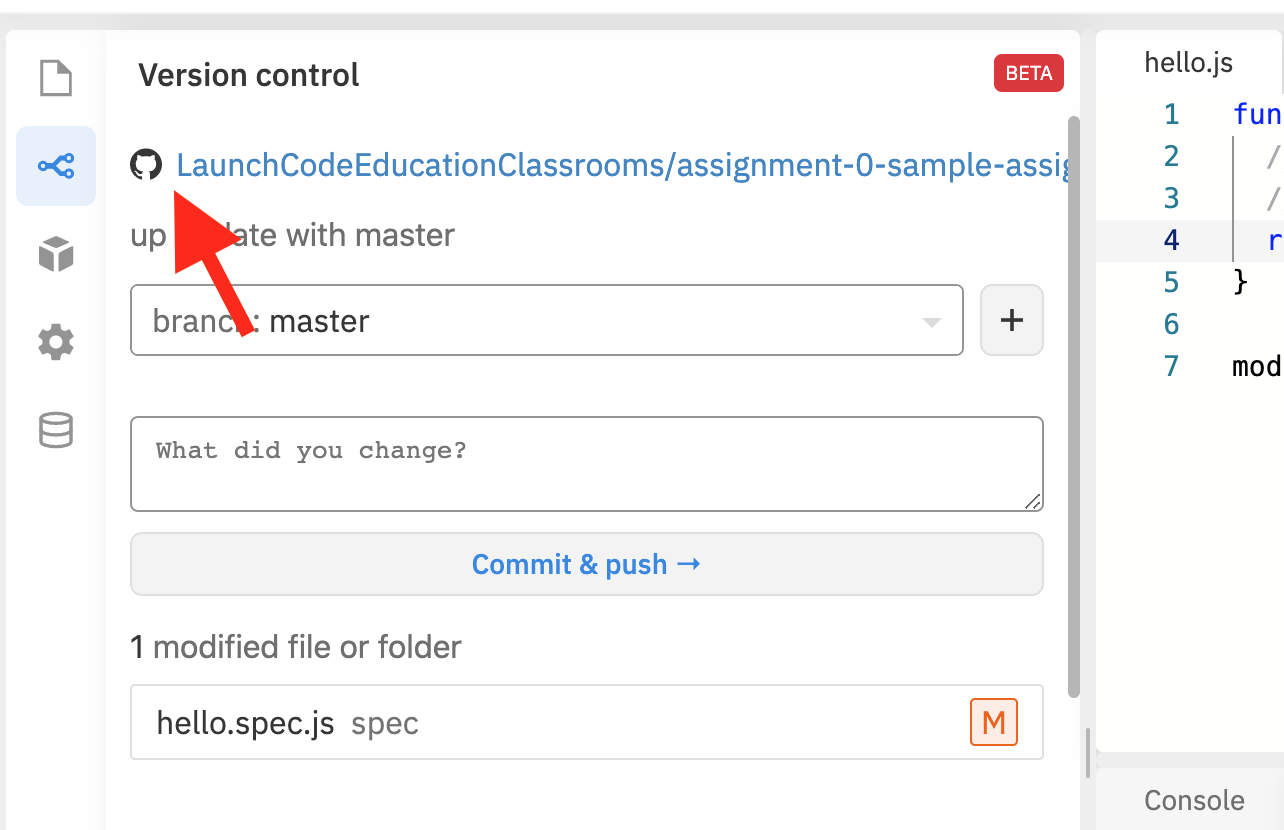
The hyperlink pointed to here takes you to the GitHub location of your assignment. ¶
If your solution passes the grading requirements, you will see a green check mark near your latest commit.
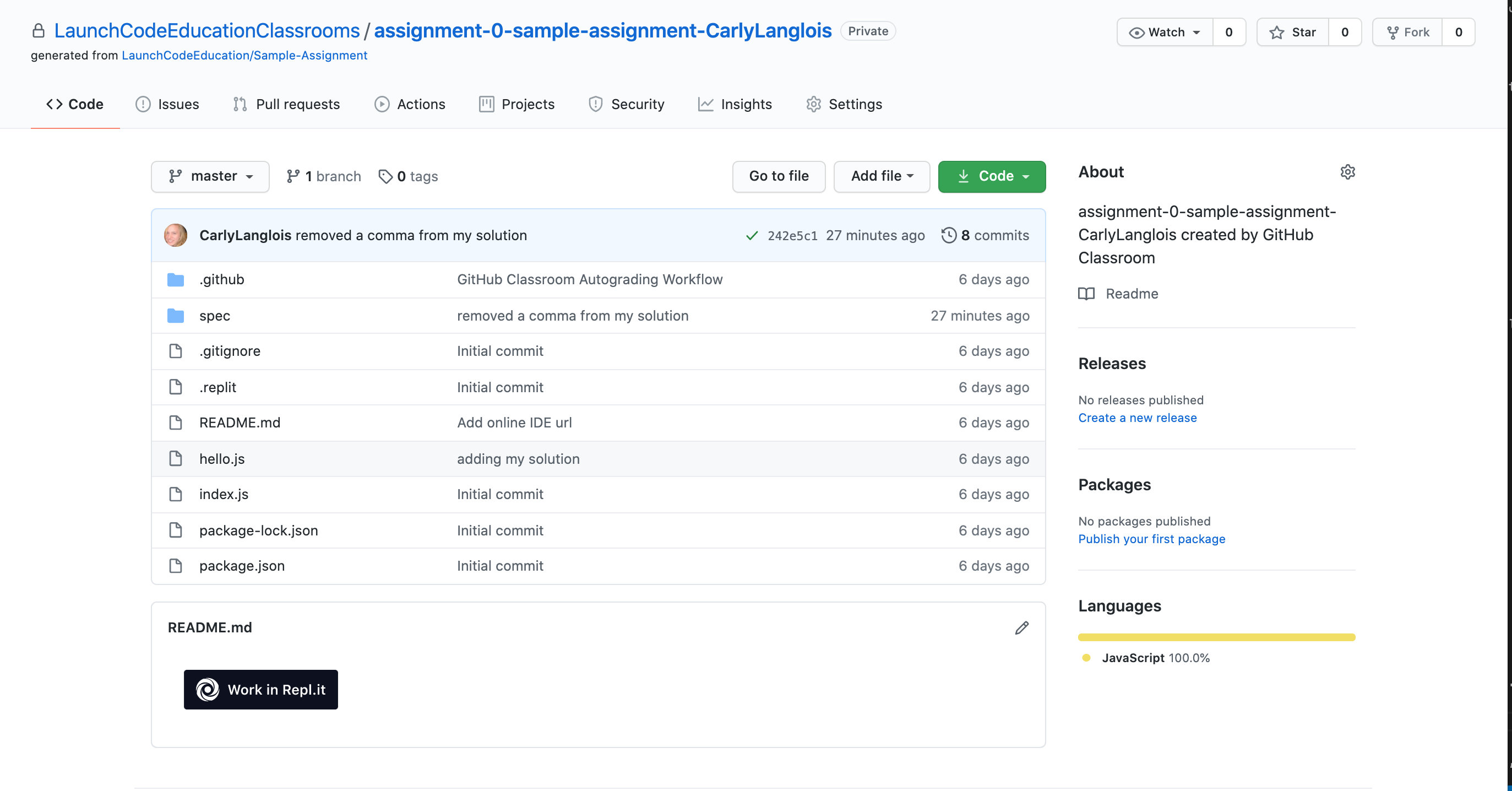
The latest commit message on this assignment is "removed a comma from my solution". The green check to the right of the message indicates that the solution passed. ¶
If your solution does not pass, you will see a red x in its place.
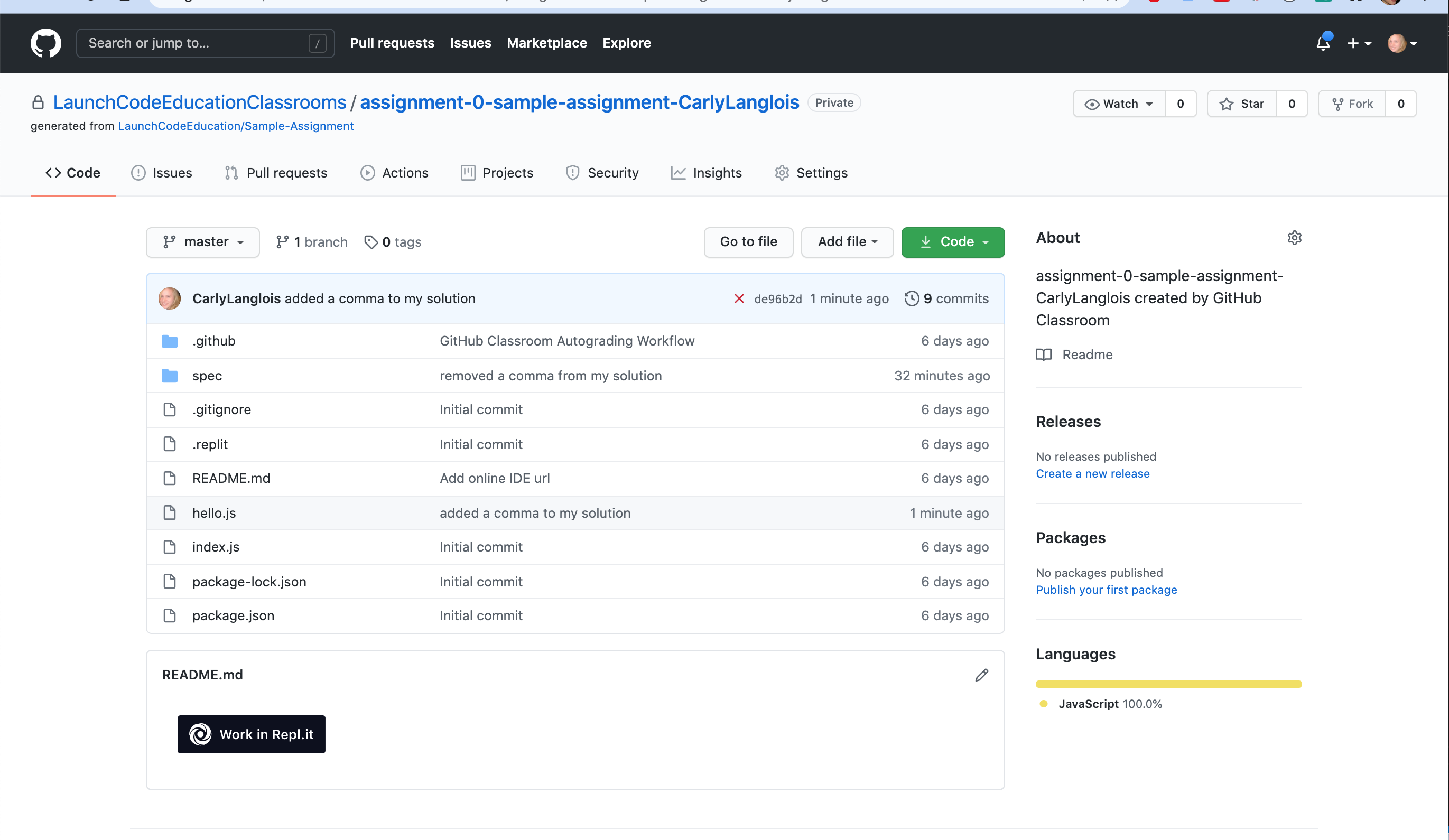
The latest commit, "added a comma to my solution" does not pass the grading requirements. ¶
A red x can always be corrected by repeating the previous steps. These are:
Open Repl.it (you can use the Work in Repl.it button from the GitHub page)
Change the string,
Run the program to visually ensure that "Hello world!" is printed,
Commit and push your changes.
You may make any number of commits to your solution. You won't lose points for pushing commit and push many times. In fact, each assignment is worth only 1 point. In most cases, you won't need to commit and push more than once, however. You can verify that your code runs we expect by running it and seeing the proper "Hello world!" message printed.
In some cases, you may see a yellow dot grading status instead of the green check or red x . This is fine and just means that GitHub is currently building your solution. It will often resolve to either a check or x after a few moments.
When you see a green check, your code passes and you are all finished with the assignment.
If your program is outputting "Hello world!", but you are still not seeing a green check mark, make sure you did not edit any file other than hello.js . An accidental space or extra character can cause problems with Github Classroom's grading. To double check that you have not done so, you can click on the 7-digit code next to the check mark or x. This will bring up which files have been changed and any changes made. If any other files other than hello.js were changed, make sure to undo the changes in Repl.it and commit to Github.

UNDER CONSTRUCTION
~/Desktop/hello> javac HelloWorld.java ~/Desktop/hello> java HelloWorld Hello, World
~/Desktop/hello> javac HelloGoodbye.java ~/Desktop/hello> java HelloGoodbye Kevin Bob Hello Kevin and Bob. Goodbye Bob and Kevin. ~/Desktop/hello> java HelloGoodbye Alejandra Bahati Hello Alejandra and Bahati. Goodbye Bahati and Alejandra.
~/Desktop/hello> javac-algs4 RandomWord.java ~/Desktop/hello> java-algs4 RandomWord heads tails tails ~/Desktop/hello> java-algs4 RandomWord heads tails heads ~/Desktop/hello> more animals8.txt ant bear cat dog emu fox goat horse ~/Desktop/hello> java-algs4 RandomWord emu ~/Desktop/hello> java-algs4 RandomWord bear
import edu.princeton.cs.algs4.StdIn; import edu.princeton.cs.algs4.StdOut; import edu.princeton.cs.algs4.StdRandom;
Learn C++ practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c++ interactively, c++ examples, c++ "hello, world" program.
Print Number Entered by User
- Add Two Numbers
- Find Quotient and Remainder
- Find Size of int, float, double and char in Your System
- Swap Two Numbers
- Find ASCII Value of a Character
- Multiply two Numbers
C++ Tutorials
- Your First C++ Program
- C++ Comments
- C++ ostream
- C++ Basic Input/Output
- C++ String Class
A "Hello, World!" is a simple program that outputs Hello, World! on the screen. Since it's a very simple program, it's often used to introduce a new programming language to a newbie.
Let's see how C++ "Hello, World!" program works.
If you haven't already set up the environment to run C++ on your computer, visit Install C++ on Your Computer .
C++ "Hello World!" Program
Working of c++ "hello world" program.
- // Your First C++ Program In C++, any line starting with // is a comment . Comments are intended for the person reading the code to better understand the functionality of the program. It is completely ignored by the C++ compiler.
- #include <iostream> The #include is a preprocessor directive used to include files in our program. The above code is including the contents of the iostream file . This allows us to use cout in our program to print output on the screen. For now, just remember that we need to use #include <iostream> to use cout that allows us to print output on the screen.
- int main() {...} A valid C++ program must have the main() function. The curly braces indicate the start and the end of the function. The execution of code beings from this function.
- std::cout << "Hello World!"; std::cout prints the content inside the quotation marks. It must be followed by << followed by the format string. In our example, "Hello World!" is the format string. Note: ; is used to indicate the end of a statement.
- return 0; The return 0; statement is the "Exit status" of the program. In simple terms, the program ends with this statement.
Things to take away
- We use std:cout in order to print output on the screen.
- We must include iostream if we want to use std::cout .
- The execution of code begins from the main() function. This function is mandatory. This is a valid C++ program that does nothing.
In the next tutorial, we will learn about comments in C++.
Sorry about that.
Our premium learning platform, created with over a decade of experience and thousands of feedbacks .
Learn and improve your coding skills like never before.
- Interactive Courses
- Certificates
- 2000+ Challenges
Related Examples
C++ Example
Check Leap Year
Check Whether Number is Even or Odd
Display Fibonacci Series
% java HelloWorld Hello, World
% java HiFour Alice Bob Carol Dave Hi Dave, Carol, Bob, and Alice.
% java HiFour Alejandro Bahati Chandra Deshi Hi Deshi, Chandra, Bahati, and Alejandro.
% java SumThree 2 5 8 2 + 5 + 8 = 15 % java SumThree -2 5 -8 -2 + 5 + -8 = -5
% java Ordered 10 17 49 true % java Ordered 49 17 10 true % java Ordered 10 49 17 false
\( \textrm{distance} = 60 \arccos(\sin x_1 \sin x_2 + \cos x_1 \cos x_2 \cos(y_1 - y_2)) \)
% java GreatCircle 40.35 74.65 48.87 -2.33 // Princeton to Paris 3185.1779271158425 nautical miles % java GreatCircle 48.87 -2.33 40.35 74.65 // Paris to Princeton 3185.1779271158425 nautical miles
\( \begin{align*} white \;&=\; \max \left \{ \, \frac{red}{255}, \, \frac{green}{255}, \, \frac{blue}{255} \, \right \} \\ cyan \;&=\; \Bigl(white - \frac{red}{255} \Bigr) \; \div \; white \\ magenta \;&=\; \Bigl(white-\frac{green}{255}\Bigr) \; \div \; white \\ yellow \;&=\; \Bigl(white-\frac{blue}{255}\Bigr) \; \div \; white \\ black \;&=\; 1 - white \end{align*} \)
red = 75 green = 0 blue = 130 cyan = 0.423076923076923 magenta = 1.0 yellow = 0.0 black = 0.4901960784313726 | red = 255 green = 143 blue = 0 cyan = 0.0 magenta = 0.4392156862745098 yellow = 1.0 black = 0.0 |
Java for Beginners – How to Create Your First "Hello World" Program
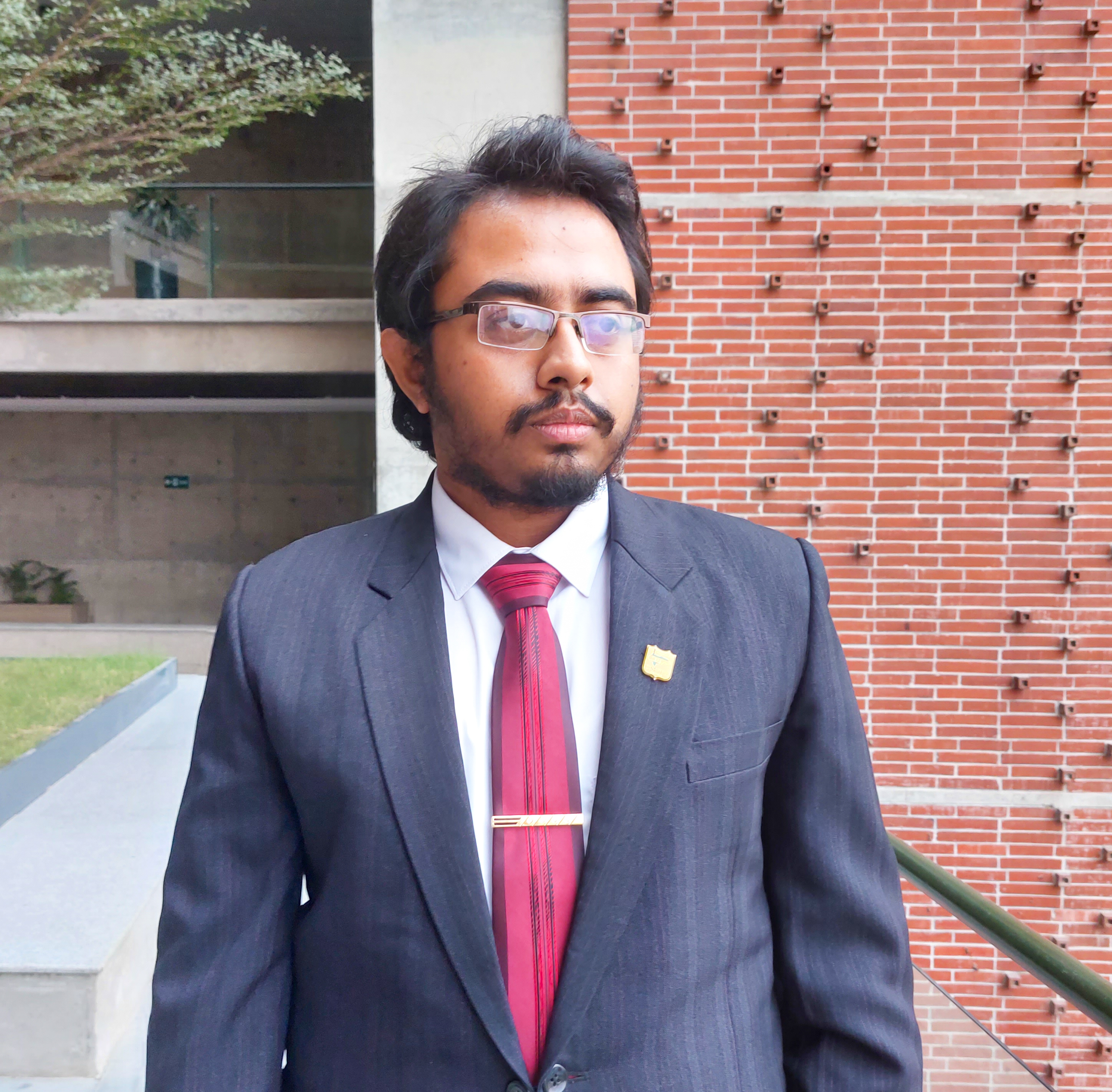
If you are learning a programming language, the first thing you do is print something in the terminal/command prompt.
And that first thing is likely printing "Hello World" in the terminal. So that's what I'll show you how to do here if you are learning Java for the first time.
🫵 What You Need to Know First
Before you start writing Java code, there are a few things you should know.
First of all, Java source files have the extension .java . An extension is something that is appended at the end of the file name, and it indicates which type of file it really is.
Different programming languages have different file extensions which help the compilers/interpreters identify which type of programming data the file contains. These extensions also help identify whether that specific compiler/interpreter can support that file format or not.
Second, you have to ensure that you have properly installed the Java compiler (JDK) on your computer. If you do not know anything about that, then simply check out this article (if you are a Windows user).
Also, when we compile the Java source code ( .java file), it generates a .class file. Later we run the .class file. Since Java is a platform-independent language (which means you can run the Java program from any operating system if you have installed the necessary components there), you can simply run this .class file from any operating system you want!
You can use any text editors / IDEs you want. But I prefer Visual Studio Code or IntelliJ IDEA IDE .
And finally, the Java file name and the public class name should be identical.
✍️ How to Create Your First Java File
Now you'll learn how to create a Java file. In this example, I am going to create a file named Main.java .
You can write the following code in that file:
Then simply run the code. If you use the Code Runner extension to run this code using VS Code, it will compile the code first and then create the Main.class file. Later it will run the Main.class file.
As it does this automatically, you almost won't see any time delays. But if you want to become a better programmer and run the code from your terminal, then make sure to check out this article .
😉 Code Explanation
In the code above, we used the public class, and the public class name needs to be identical to the .java filename. If you used a different file name, then the public class name will also need to be different.
For example, if you are using MyJavaFile.java , then the public class would be like this: public class MyJavaFile . Java is a case-sensitive language, so make sure to check that the uppercase-lowercase letters are also identical.
Then we need the main method. The Java compiler always starts compiling from the main method. The main method is public static void main(String[] args) .
For printing something in the terminal, we use the print method. Here the print method is System.out.println("") . You have to provide the thing that you want to print in the terminal in the double quotations.
We use the semicolon ( ; ) to specify the end of a statement. So we use the semicolon after each statement's end.
There you go! I'll discuss more tweaks and advanced cool topics in other articles. 😁
📹 Video Walkthrough
If you're the type of person who enjoys learning from videos, then I have also created a video just for you! Make sure to check it out:
Also, I am putting together a playlist where I am publishing all Java-related content. Make sure to check the playlist from here and get all the code from this GitHub repository .
😀 Conclusion
Thanks for reading this entire article. I hope this helps you get started on your Java programming journey.
You can follow me on:
- GitHub: FahimFBA
- LinkedIn: fahimfba
- Twitter: Fahim_FBA
- YouTube: Code With FahimFBA
- Website: https://fahimbinamin.com/
If you want to support me, then you can also buy me a coffee!
Cover: Photo by AltumCode on Unsplash
Microsoft Research Investigation Contributor to OSS (GitHub: FahimFBA) | Software Engineer | Top Contributor 2022, 2023 @freeCodeCamp | ➡️http://youtube.com/@FahimAmin
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
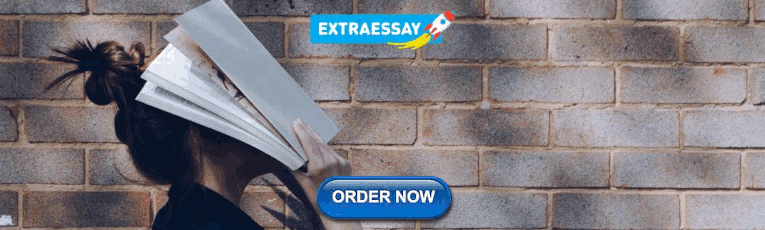
IMAGES
VIDEO
COMMENTS
Hello World program is the most basic program of any programming language. It prints "Hello world" on the screen. In this article, we will display "Hello World" without using WriteLine Method.
Programming Assignment 1: Hello, World. The purpose of this assignment is to introduce you to programming in Java and familiarize you with the mechanics of preparing and submitting assignment solutions. Install our Java programming environment (recommended).
The “Hello World” program is the first step towards learning any programming language and is also one of the most straightforward programs you will learn. The Hello World Program in C++ is the basic program that is used to demonstrate how the coding process works.
A "Hello, World!" is a simple program that outputs Hello, World! on the screen. Since it's a very simple program, it's often used to introduce a new programming language to a newbie. Let's explore how Java "Hello, World!" program works.
Assignment #0: Hello World¶ Your first assignment is mostly an exercise in following instructions. We'll ask you to modify an existing program so that, when run, the program prints "Hello world!".
Install our novice-friendly Java programming environment on your computer by following these step-by-step instructions for Mac OS X, Windows, or Linux. On each assignment, use the Project from the menu at top. As part of these instructions, you will write, compile, and execute the program HelloWorld.java.
A "Hello, World!" is a simple program that outputs Hello, World! on the screen. Since it's a very simple program, it's often used to introduce a new programming language to a newbie. Let's see how C++ "Hello, World!" program works.
Hello, World. The purpose of this assignment is to introduce you to programming in Java and familiarize you with the mechanics of preparing and submitting assignment solutions. You will learn to use DrJava editor for writing, compiling, and executing Java programs, and Dropbox for submitting your work electronically.
A “Hello, world!” program is traditionally used to introduce novice programmers to a programming language. “Hello, world!” is also traditionally used in a sanity test to make sure that a computer language is correctly installed, and that the operator understands how to use it.
If you are learning a programming language, the first thing you do is print something in the terminal/command prompt. And that first thing is likely printing "Hello World" in the terminal. So that's what I'll show you how to do here if you are learning Java for the first time. 🫵 What You Need to Know First.