Become a sponsor
The official
Vue.js Certification
Get certified!
The state is, most of the time, the central part of your store. People often start by defining the state that represents their app. In Pinia the state is defined as a function that returns the initial state. This allows Pinia to work in both Server and Client Side.
If you are using Vue 2, the data you create in state follows the same rules as the data in a Vue instance, i.e. the state object must be plain and you need to call Vue.set() when adding new properties to it. See also: Vue#data .
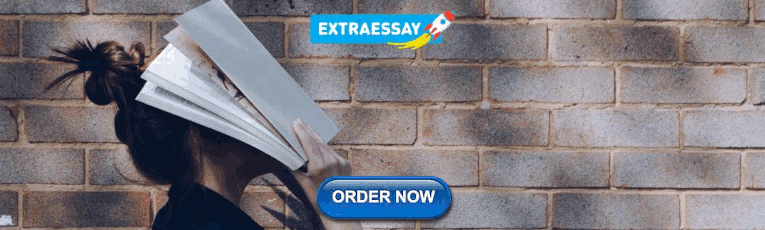
TypeScript â
You don't need to do much in order to make your state compatible with TS: make sure strict , or at the very least, noImplicitThis , is enabled and Pinia will infer the type of your state automatically! However, there are a few cases where you should give it a hand with some casting:
If you prefer, you can define the state with an interface and type the return value of state() :
Accessing the state â
By default, you can directly read and write to the state by accessing it through the store instance:
Note you cannot add a new state property if you don't define it in state() . It must contain the initial state. e.g.: we can't do store.secondCount = 2 if secondCount is not defined in state() .
Resetting the state â
In Option Stores , you can reset the state to its initial value by calling the $reset() method on the store:
Internally, this calls the state() function to create a new state object and replaces the current state with it.
In Setup Stores , you need to create your own $reset() method:
Usage with the Options API â
For the following examples, you can assume the following store was created:
If you are not using the Composition API, and you are using computed , methods , ..., you can use the mapState() helper to map state properties as readonly computed properties:
Modifiable state â
If you want to be able to write to these state properties (e.g. if you have a form), you can use mapWritableState() instead. Note you cannot pass a function like with mapState() :
You don't need mapWritableState() for collections like arrays unless you are replacing the whole array with cartItems = [] , mapState() still allows you to call methods on your collections.
Mutating the state â
Apart from directly mutating the store with store.count++ , you can also call the $patch method. It allows you to apply multiple changes at the same time with a partial state object:
However, some mutations are really hard or costly to apply with this syntax: any collection modification (e.g. pushing, removing, splicing an element from an array) requires you to create a new collection. Because of this, the $patch method also accepts a function to group these kinds of mutations that are difficult to apply with a patch object:
The main difference here is that $patch() allows you to group multiple changes into one single entry in the devtools. Note that both direct changes to state and $patch() appear in the devtools and can be time traveled (not yet in Vue 3).
Replacing the state â
You cannot exactly replace the state of a store as that would break reactivity. You can however patch it :
You can also set the initial state of your whole application by changing the state of the pinia instance. This is used during SSR for hydration .
Subscribing to the state â
You can watch the state and its changes through the $subscribe() method of a store, similar to Vuex's subscribe method . The advantage of using $subscribe() over a regular watch() is that subscriptions will trigger only once after patches (e.g. when using the function version from above).
By default, state subscriptions are bound to the component where they are added (if the store is inside a component's setup() ). Meaning, they will be automatically removed when the component is unmounted. If you also want to keep them after the component is unmounted, pass { detached: true } as the second argument to detach the state subscription from the current component:
You can watch the whole state on the pinia instance with a single watch() :
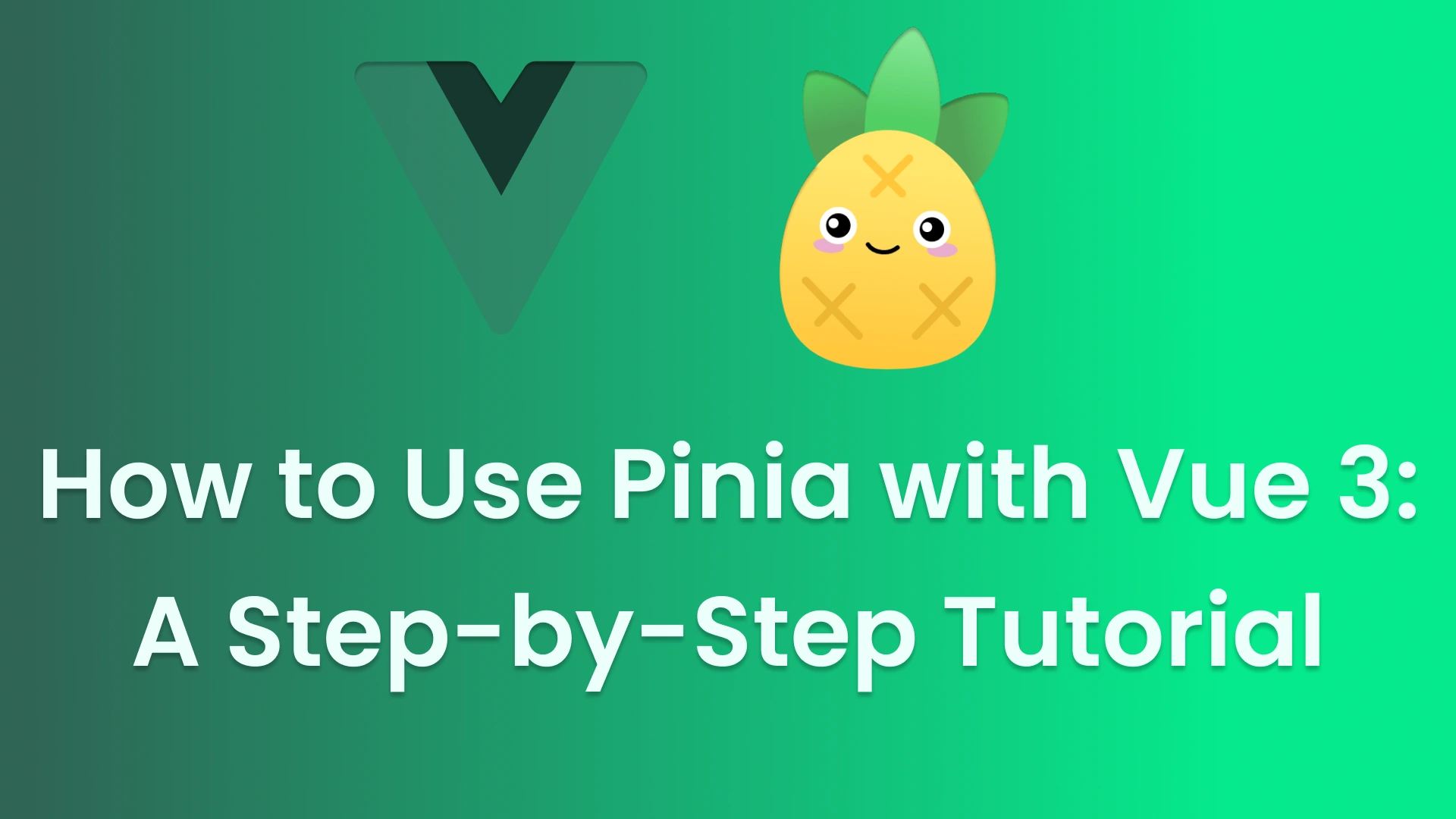
How To Use Pinia With Vue 3: A Step-by-Step Tutorial
Modified at 2023-04-09
Vue 3 is a dynamic JavaScript framework that makes building robust and dynamic user interfaces a breeze. While Vue 3's state management system is powerful, managing the state of a complex application can be a daunting task. That's where Pinia comes in. Pinia is a simple yet powerful state management system that makes managing your application state a lot easier. In this tutorial, we'll take a step-by-step approach to getting you started with Pinia in Vue 3.
Introduction
Pinia provides a simple, reactive store that allows you to manage application state in a centralized location. It's designed to work seamlessly with Vue 3, and offers features like lazy state initialization, time-travel debugging, and TypeScript support out of the box.
Understanding Pinia
Pinia is a lightweight, standalone state management library for Vue 3 applications. It provides a reactive store that allows you to manage application state in a centralized location. The store can be used to store any type of data, including primitives, objects, and arrays.
In Pinia, you define stores using the defineStore function. Stores can have state, actions, and getters.
Installing and Setting up Pinia
To use Pinia in your Vue 3 application, you'll first need to install it:
Once Pinia is installed, you'll need to create a Pinia instance and install it in your Vue app:
This creates a Pinia instance and installs it in your Vue app using the app.use() method.
Creating a Pinia Store
Once Pinia is set up, you can start creating stores to manage your application state. Let's create a simple store to manage a todo list:
In this example, we've defined a store with an id of 'todo' , a todos array in its state, and two actions: addTodo and removeTodo . The addTodo action takes a todo object as its argument and pushes it to the todos array. The removeTodo action takes a todo object as its argument and removes it from the todos array.
Adding State and Actions to Your Pinia Store
Now that we have a basic Pinia store setup and connected to our Vue app, let's start adding some state and actions to it.
To add state to our store, we can simply define a property on the store object:
Here, we've added a todos property to our store's state object, which is initialized as an empty array.
To add an action to our store, we define a method on the store object:
Here, we've defined an addTodo action that takes a text argument and pushes a new todo object onto the todos array with that text and a done property set to false .
With our state and actions defined, we can now use them in our Vue components.
Using Pinia in a Vue Component
Now that we have our Pinia store set up, we can use it in our Vue components. In this section, we'll create a simple component to demonstrate how to use Pinia.
Let's create a TodoList component that displays a list of todos and allows the user to add new todos.
First, we'll import the defineComponent function from Vue:
Next, we'll import our Pinia store:
Then, we'll define our TodoList component:
We start by calling useTodoStore() to get a reference to our Pinia store. We then define our component using the defineComponent() function from Vue . Inside the component setup function, we define our template using the JSX syntax.
In our template, we display a list of todos by mapping over the todos array from our Pinia store. We also display an input field and a button to add new todos. When the user clicks the button, we call the addTodo() method on our Pinia store.
Note that we're using the v-model directive on our input field to bind it to the newTodoText property in our Pinia store. This means that when the user types in the input field, the newTodoText property in our store is updated automatically.
Pitfalls of Destructuring Your Pinia Store
One common pitfall when using Pinia stores in Vue components is to destructure the store object directly. For example:
This can lead to issues because the todos variable is now a standalone reactive object, separate from the actual Pinia store. This means that changes made to todos will not be reflected in the store, and vice versa.
To avoid this pitfall, it's important to always reference the store directly, like this:
This ensures that our reactive data is always connected to the Pinia store.
Exploring Pinia Getters
In addition to state and actions, Pinia also provides getters, which are functions that return computed values based on the current state of the store.
To define a getter in our store, we can add a getters object to our store definition:
Here, we've defined a doneTodosCount getter that returns the number of todos in the todos array that have a done property set to true .
Accessing Other Getters from a Getter
One useful feature of Pinia getters is that they can access other getters from the same store. This allows us to compose more complex computed values from simpler ones.
For example, we can define a undoneTodosCount getter that calculates the number of todos that are not yet done by subtracting the doneTodosCount from the total number of todos:
Here, we've defined an undoneTodosCount getter that subtracts the doneTodosCount getter from the total number of todos in the todos array.
Accessing Getters from Other Pinia Stores
Sometimes you may need to access getters from other stores within your store. You can do this by passing the other store as an argument to your getter. For example:
In this example, the combinedValue getter in useStore2 is accessing the value getter from useStore1 by passing it as an argument to the getter.
Passing Arguments to Getters
You can also pass arguments to getters to make them more dynamic. To do this, simply define the argument in the getter function and pass it when you call the getter:
In this example, the getTodoById getter takes an id argument and returns the corresponding todo from the todos array.
Tips on Pinia
Aside from the basics of creating and using a Pinia store, there are some additional tips that can make your experience with Pinia even better.
Persisting Pinia Data in Local Storage
One of the most important aspects of any web application is to ensure that the user's data is persisted even after they close their browser. Fortunately, Pinia makes this incredibly easy by providing a plugin that allows you to automatically persist your Pinia store data in local storage.
To use this plugin, you can install it via npm:
Once installed, you can use it by importing it and passing it to the createPinia function:
With this plugin, your Pinia store data will be automatically persisted in local storage and rehydrated when the user revisits your web application.
Using Composables with the Pinia Store
Another way to make your experience with Pinia even better is to use composables with your Pinia store. While Pinia stores are great for managing your application state, they can quickly become cluttered with complex logic.
Composables allow you to extract and encapsulate logic that can be shared across components or even across multiple Pinia stores. For example, you might have a composable that handles sorting and filtering logic for a list of data that is used in multiple components.
To use a composable with your Pinia store, you can simply import it and use it within your store's methods or getters:
By using composables with your Pinia store, you can keep your store code clean and maintainable while still having access to powerful logic that can be reused across your application.
In this tutorial, we covered the basics of Pinia and how to use it with Vue 3. We learned how to install and set up Pinia, create a Pinia store, use Pinia in a Vue component, add state and actions to our Pinia store, explore Pinia getters, and some tips on how to make your experience with Pinia even better.
Pinia is a powerful and flexible state management solution for Vue 3, and it offers a lot of benefits over traditional Vuex. With Pinia, you get better type safety, better performance, and a simpler and more intuitive API. So if you're looking for a great state management solution for your Vue 3 application, be sure to give Pinia a try!
- Building a Responsive Navigation Bar with TailwindCSS
- Mastering Gradients: Learn How to Create Linear Gradients with CSS!
Learn How To Use Vuejs3 Pinia Store Management With Simple Example
In this article we will explore and learn how to use the new state management library Pinia alongside Vue3 Composition Api.
In my previous projects in Vuejs i am using Vuex as a statement management. Vuex is still a powerful store manager that provides global state accompanied with previous versions with Vuejs and still be used in Vue3. Of course if you are building a new Vue 3 project you can still use Vuex.
However as per Vuex docs, that mentions that Vuex 3 and 4 will still be maintained. and it’s unlikely to add new functionalities to it.Â
For this reason the official state management library for Vue has changed to Pinia . Pinia has almost the exact same or enhanced API as Vuex 5. We will explore some of Pinia features in this article using a simple example.
What we will do
We will be building a simple Todo list app using Vue3.
In the command line create a new vue app using vue-cli:
You will be prompted to select a preset choose Default (Vue 3) ([Vue 3] babel, eslint) and hit enter.
Installing Pinia
After success installation cd into the project, we will install Pinia:
now run this command to launch the app
This will launch a development server at localhost :8080
Initialize Pinia Instance
The next step is to provide the Pinia plugin in the Vue app. So open src/main.js and modify as follows:
As you see in this code we imported the createPinia() function from pinia to create a new Pinia instance. Then invoking the createPinia() and assign it to pinia variable and finally we provide it to Vue using app.use(pinia) . That’s it.
Store Structure
Pinia store structure is similar to Vuex store but it also extremely simpler in terms of:
- Pinia has a state object.
- Pinia has getters .
- Pinia has actions .
- Pinia doesn’t have Mutations .
- More compatible with Composition Api.
- No need to define namespaced modules for multiple store.
- Nesting stores inside each other is so easy than Vuex.
Defining Store
Store is defined using defineStore() function from pinia:
The defineStore() function accepts two arguments, (unique name, options object). The unique name or the id used to identify the store in the devtools, like namespace name in Vuex.
By convention the return value from defineStore() should be name be prefixed with “use” keyword like useTodosStore , useUser in the same way as composables in Composition Api
Now create a directory src/store to hold our store files . Create a single file that will define the todos store.
src/store/todos.js
In this code i declared useTodosStore which have these elements:
- state : a function to define the central state, In this case the todos is any array and initialized it with one item and boolean “showForm” and the current updated “id”.
- getters : to define getters for store, it takes the state as first argument. Here we have one getter “ count ” to get todos length.
- actions : Actions define operations on the state like Vuex actions. Here i created one action to create a todo.
Using The Store
To consume and use the store in any component, depends on whether you are using the composition Api or options Api. In our example we are using the composition Api.
Create these components first:
- src/components/Todos.vue
- src/components/ListTodos.vue
- src/components/TodoForm.vue
Next open App.vue and include the <Todos /> component like so:
src/App.vue
The <Todos /> component represent the todos page which include all the other components for listing all todos, todo form.
Now open this component and update it like so:
components/Todos.vue
This page displays all todos. In the top i included the two components <ListTodos /> and <TodoForm /> . The most important part in the setup() function which invokes the useTodosStore() .
Here i want to access the showForm variable from store, using store.$state.showForm to either show the form or hide it. I made it inside the computed() vue helper so that any change in this variable will be triggered. Finally i returned the showForm from setup.
Displaying All Todos
Update ListTodos.vue
This component display a list of todos and button to show the create form. Again in the setup() function invoked useTodosStore() .
The showCreateForm() , and showUpdateForm() show the create and update todo form respectively. Both functions have similar logic. Here we are mutating the state. So i used Pinia function “ $patch “. The $patch function executes several updates to state in one shot.
You can also mutate the state directly by accessing the state and modify like so:
$patch is preferred when modifying multiple items at once. It’s important to wrap the accessed items inside of computed so that updates happen to state reflected instantly.
Finally i returned the data todos, showCreateForm, showUpdateForm .
Creating New Todo
Open TodoForm.vue and update like so:
In the same way i accessed the useTodosStore . I retrieved the current todo id to check if this an update or create form using store.$state.id inside of computed.
I declared a reactive variable “ form ” to hold the form data. I created a method “ handleSubmit ” which triggers when submitting the form.
The logic inside the handleSubmit is to invoke an action from the store to create the todo. In this case i am invoked the create action store.create(form.title, form.description) . Then i mutated the state using store.$patch to hide the form and display the list of todos.
Updating Todo
The update todo part located in the same <TodoForm /> component so let’s make these updates:
TodoForm.vue
Below this line:
Insert this code:
Next modify handleSubmit() function:
Then update the todos store, add new action and getters:
store/todos.js
I added two getters, findById, findIndex and also added new action to update the todo. The getters findById and findByIndex accepts the todoId parameter. To use findById in component:
Removing Todo
To remove todo open ListTodos.vue and make these updates:
Under this function:
Add new function:
Then add to the return statement:
And in the template add the click event for remove like so:
Now let’s add new action in the store:
That’s it. Now we have a fully working todos app using Pinia store and Vue 3.
The full store source
Another Approach To Define Store(Setup Stores)
The store we created in the previous section is called option store which is similar to the Options Api in components which we defined the state, getters, and actions as an object.
However Pinia also allow us to declare a store using another way, instead of providing an options object when calling defineStore() we can pass a setup function like so:
Inside the setup function we can use a similar methods like the setup() function in components composition Api.
Let’s refactor the above store as a setup store
As you see i am using the vue 3 helpers for composition Api like ref(), computed(). So i refactored the state into reactive variables like todos, showForm, id. The getters become computed properties using the computed() helper. The actions become normal methods.
Then all the data i need to be consumed in components in the return statement as an object.
Now what’s left is to refactor the code in every component to use the setup store approach which i will this to you. Refer to Pinia docs in order to learn more and accomplish this.
What's your reaction?
Localstorage and sessionstorage in javascript whether to use, learn about html data-* attributes, you may also like, building a simple application using nuxt 3 vue framework, vuejs pinia using store outside vue components, vuejs 3 setup() function and composables.
You should use storeToRefs NOT a computed property to access the store, see https://stackoverflow.com/a/71677026
Recent Articles
What is a webmanifest file and why it’s important, displaying dialogs and modals with the native html dialog element, spl data structures in php: the splqueue.
Lost your password?
- Backend Development
- Frontend Development
- News & Events
Latest Posts
Laravel inertia react roles permissions crud, laravel inertia react roles permissions crud part2, popular tags.
Get a year access to Vue Mastery with 40% off
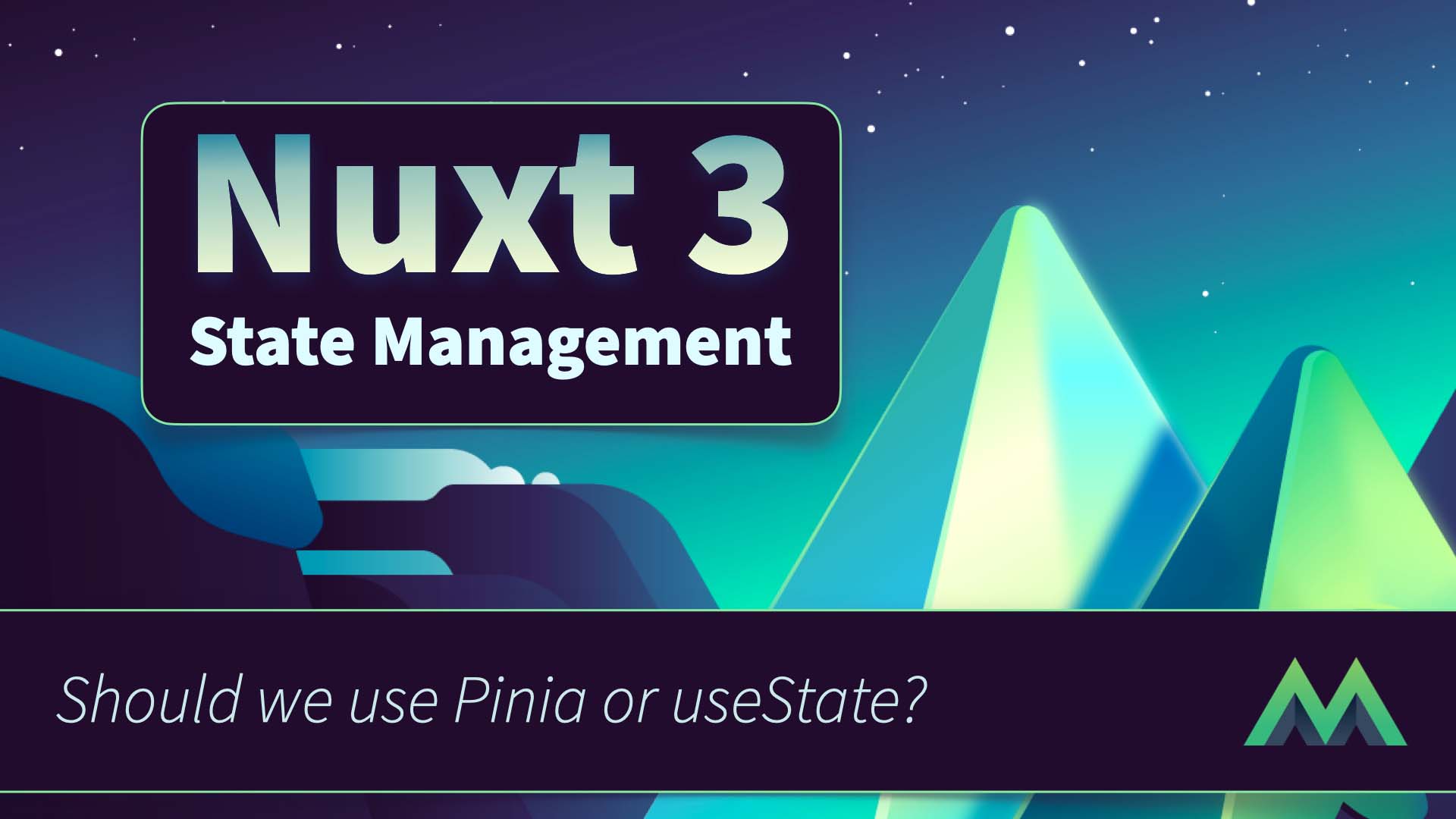
Nuxt 3 State Management: Pinia vs useState
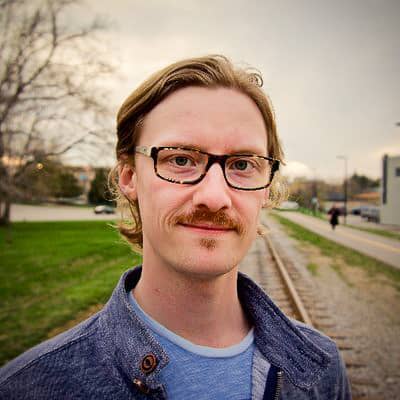
Michael Thiessen
20 June 2022
If youâre a Nuxt developer, or plan to become one with Nuxt 3, you might be wondering what to use for state management.
Do I use Nuxt 3âs own statement management solution: useState ?
Should I use Nuxt 3 with Pinia?
Can I use Pinia and useState together?
Which is better, Pinia vs. useState ?
Hereâs the short answer:
Pinia is what you get if you keep adding more and more features to useState . More complex apps will benefit from the extra features in Pinia, but useState is better for small and simple apps.
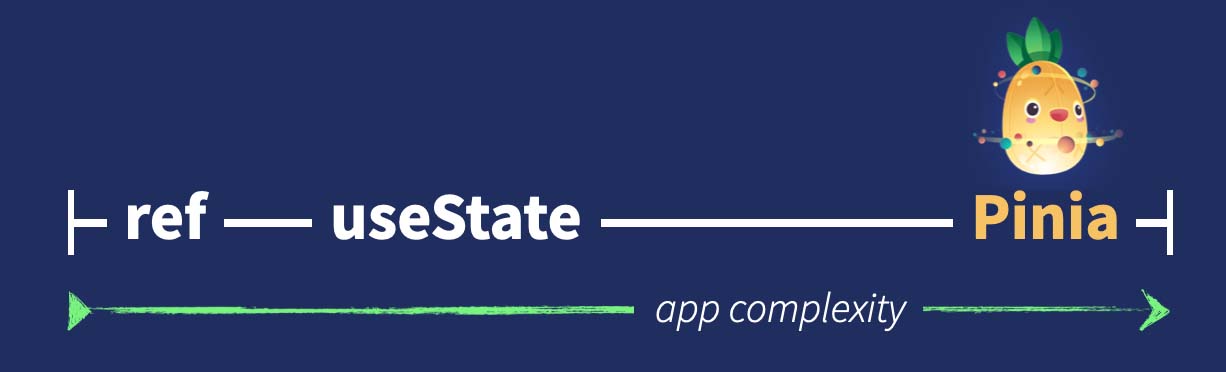
But letâs back up a bit and examine the longer answer. Weâll first cover the problems with ref that spurred the need to create useState . Then we can address what benefits Pinia gives us over Nuxtâs useState .
Whatâs wrong with ref ?
First, we need to address why the Nuxt.js team had to create useState in the first place. Whatâs so wrong with ref anyway?
There are three main reasons, which weâll go over in some detail:
- To combat the problem of Cross-Request State Pollution
- To provide a solution for state hydration
- To make it easier to share state
The concept of cross-request state pollution is less well-known than the other two, so weâll start there.
1. Cross-Request State Pollution
In a typical Vue app, we store state as a singleton.
Whether you use Vuex, Pinia, or just a reactive object, you want to share the same state and the same object across all of your components. Otherwise, whatâs the point?
This works perfectly for client-side apps and SPAs.
But when we move that state management system to the server, we run into issues with cross-request state pollution . It sounds a bit scary, but itâs not that complicated.
When using server-side rendering (SSR), each new request is executed inside of the same application. And because we only have one singleton state object, every request will share the same state . This is bad. It creates the potential for leaked data, security vulnerabilities, and hard-to-pin-down bugs.
The solution to this is pretty simple but difficult to execute correctly: create a new state object for each new request! And instead of needing to figure out how to do this ourselves, we can use useState and get around that issue.
Next, weâll take a look at staying hydrated.
2. State Hydration
When using server-side rendering with Nuxt, our app is first executed on the server to generate the initial HTML. Thereâs a good chance we might want to use a ref or two during that initialization of our components:
Once the app is booted up on the client, weâll have to re-run all of this initialization code. None of these variables are set, so we have to execute the code to figure out what they should be.
But we just did those calculations !
This is where hydration comes in. We take the state weâve already computed on the server and send it along with the appâs HTML, CSS, and other assets. Then, instead of re-calculating everything, we can pick up where we left off!
Unfortunately, though, ref doesnât do this for us. Luckily â you probably guessed it â Nuxtâs useState has hydration built-in. So useState will automatically perform this optimization without us even thinking about it.
With useState , we also get some benefits around sharing our state across the application.
3. Easier state sharing
As your app grows, youâll find that some state needs to be accessed in almost every component.
Things like:
- A userâs unique id or accountId
- A list of features or permissions the current user can access
- Color themes, whether dark mode is turned on or not
Instead of passing props around endlessly, we turn to global state management libraries like Vuex or Pinia⊠or even useState .
Each piece of state is accessed by a unique key but is available anywhere in our app:
This is something that ref canât do!
Why is Pinia better than useState ?
Now that weâve seen why useState was created in the first place, letâs see why you should (usually) use Pinia instead for state management in Nuxt. To understand this, we need to know how Pinia is different from useState , and what features it offers us.
Like I mentioned earlier, you can sum it up this way: Pinia is what you get if you took useState and kept adding more and more practical features.
Pinia offers a better developer experience (DX) than Nuxtâs useState by providing more features that youâll likely need as your application grows in size and complexity. In other words, if you donât use Pinia, thereâs a good chance youâll find yourself re-inventing it and building your own state management library. So why not save yourself the trouble from the start?
There are four main DX improvements that Pinia gives us over useState :
- A very slick DevTools integration
- Stores to organize our state
- Actions and Getters for easier updating and retrieving of state
1. Devtools integration
With Pinia, we get first-class Vue Devtools support, making developing and debugging issues so much easier.
First, we get a timeline of state changes, so we can see how our state updates over time. I canât tell you how many bugs Iâve tracked down this way. One time a toggle wasnât working for me. Every time I clicked it, nothing would happen. But when I looked at the state changes, I could see it was toggled twice every time I clicked it. So then I knew to look for two events being emitted and was able to fix the issue quickly.
Second, we can see the current state of all our stores. We can see all the stores at once, or we can also see the stores alongside any component that is using it.
Third, we get time-travel debugging. This lets us go back in history and replay the state changes in our application. To be honest, Iâve never used this feature much myself, but I also tend to forget that it exists at all!
2. Stores for organization
As applications get larger and more complex, so does the size and complexity of the state. Accessing a flat state with basic keys no longer makes much sense.
With useState we can start to address this by saving whole objects:
Pinia takes this concept and goes further with the idea of stores .
A store in Pinia is a reactive object along with actions and getters (weâll get to those next). But stores in Pinia can also use other stores. This lets us compose our state as we would compose our Vue components:
Here we can use our theme store inside of our user store. This gives us a lot of powerful options for organizing our code. Something that useState doesnât offer unless you build it yourself.
3. Actions and Getters
State is never static, and itâs nice to be able to define specific ways that our state can change through methods.
Pinia Actions
Pinia gives us actions which are a great way to achieve this:
We can call the action like this:
Theyâre also co-located with the state, meaning that these actions are beside the state that they modify. This makes it much easier to understand the code when reading it and refactoring it.
Hunting through multiple files to track down where state is modified takes way too much time and creates the opportunity for many bugs.
Pinia Getters
Pinia also lets us define getters , which are convenient functions for dealing with our state. You can think of them as computed properties for your Pinia stores.
When it comes to state, less is more. We want to save the smallest amount possible and then calculate everything else we need from that tiny piece. This simplifies our state a lot, but re-calculating stuff all the time can become tedious.
This is where our getters come in handy:
If we want to grab the fullName , we can use the getter:
Instead of storing fullName as a separate piece of state, we can calculate it from firstName and lastName . If we stored fullName , weâd always have to update it whenever firstName or lastName are updated, which is no small task. This way, we avoid many bugs because the firstName getter will always be synced and up-to-date with our state.
And like our actions , these getters are always co-located with our state. This makes it easier to update them and understand how they work.
Without actions and getters, weâre left redefining our logic over and over again. Youâd likely write your own system of actions and getters on top of useState . So why not skip ahead and start with that pattern, which comes with Pinia?
When should we use Pinia over useState?
Itâs all about how complex your app is.
We saw that useState has many improvements over ref , specifically when it comes to server-side rendering. But using Pinia with Nuxt 3 has even more improvements over using useState .
However, all of these improvements increase the complexity and size of your application. Itâs essential to choose the right level, where you maximize the toolâs benefits so that the costs are worth it.
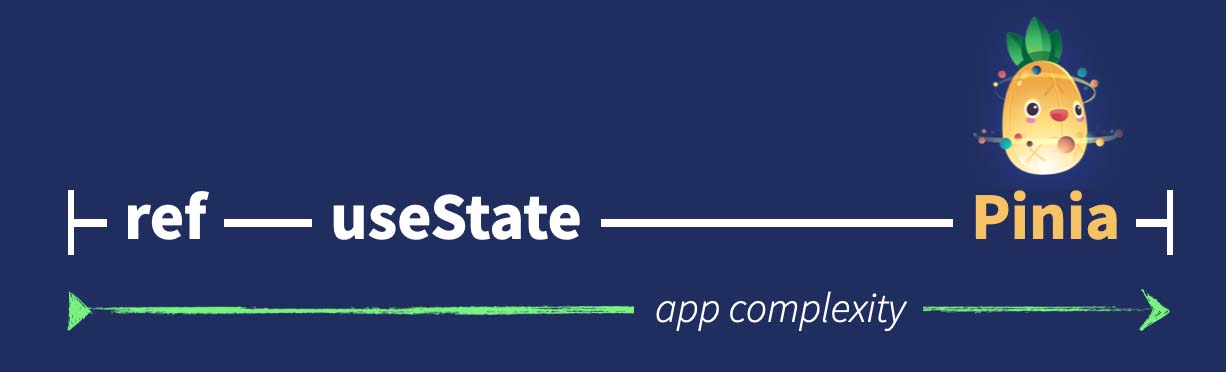
But to make things even simpler, sticking with Pinia for your Nuxt 3 state management isnât a bad idea. If youâd like to learn more about Pinia and Nuxt, I invite you to check out Vue Masteryâs high quality courses , which begin with free tutorials.

From Vuex to Pinia Vue 3 Composition API
- Intermediate
Transition your state management from Vuex to Pinia with the guidance of a Vue Core Team Member.
Pinia Q&A Vue 3 Composition API
Learn from the expert in this recording of a live Q&A with Creator of Pinia Eduardo San Martin Morote.
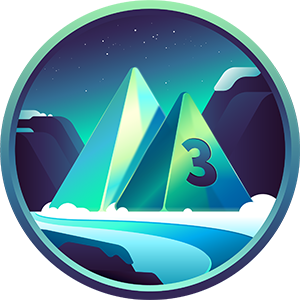
Nuxt 3 Essentials Vue 3 Composition API
Learn this intuitive framework that includes built-in tools and structure to build Vue apps that can scale.
In this article:
Dive deeper into vue today.
Access our entire course library with a special discount.
Download the cheatsheets
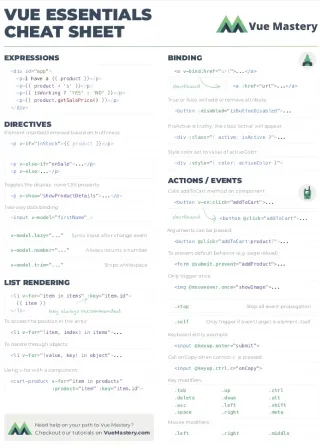

Top 5 mistakes to avoid when using Pinia
This article provides some common mistakes when using Pinia and how to fix them.
Eduardo San Martin Morote
November 28, 2023
Pinia, the official state management solution for Vue 3, just reached 4 years old! đ This means weâve had plenty of time to see it in action, to see it succeed but also fail. Let me share with you some of the most common mistakes I have seen in projects using Pinia and how to fix them.
Here's the TL;DR:
Calling useStore() in the wrong places
Yolo casting empty objects, using reactive() for objects that can be replaced.
- Using Deep reactivity with large collections
Storing URL state in the store
Let's dive into each of these mistakes and how to fix them!
Does this message ring a bell?
In Pinia, all stores are defined with defineStore() , which doesn't return a store instance like we had in Vuex:
This store object could be used right away and was accessible in components through $store . In Pinia, defineStore() returns a function that we need to call to get the store instance:
This function is in fact a composable and that gives away where it should be called: within the setup() of components. Wait, that's it? No, not at all! You can technically also call it within other composables as long as they are called within the setup() of components. But you can also call them within other stores and within some special functions :
Here is an example calling it within a store:
Pretty useful, isn't it!
But what are these special functions ? Usually, they come from other libraries that are connected to the Vue app through a nice advanced API [runWithContext()](https://vuejs.org/api/application.html#app-runwithcontext) . I am certain you won't need to use this method in your app but it allows libraries like Vue Router and Pinia to use composables that rely on [inject / provide ]( https://vuejs.org/guide/components/provide-inject.html#provide-inject ). This includes using the router within stores and using the stores within navigation guards!
But wait, I've used the store in other places before and everything worked just fine đ€. This limitation is only important when dealing with SSR (Server-Side Rendering). In Single Page applications, there is no risk of cross-state pollution (fancy way of saying your application is not secure). Therefore, you can just call the useStore() function anytime after installing the pinia plugin :
That being said, I recommend you to stick the rules mentioned above rather than relying on the fact that it works in SPA. It will make your code more predictable and easier to maintain.
I have seen this mistake a lot in the past year. It's a very easy one to make and it's not only related to Pinia, but also TypeScript. Very often you have objects that start undefined but they get populated before you get to use them. One example is the user object in an authentication store:
The error here is totally normal and actually a good thing: it forces out to verify that the user is authenticated before calling updateAvatar() . But it's also annoying because we know that the user will be defined before we call updateAvatar() . So, depending on your experience with TypeScript, you might be tempted to do this:
And poof! The error is gone! But what did we just do? We just told TypeScript that the user is always defined, even when it's not. I like to call this the YOLO cast. It's, in my opinion, worse than using as any or @ts-ignore because with those, we are accepting that we couldn't type something properly. So we just decide that we still need to get that feature shipped and we'll come back to it later (but we never do). Casting an empty object to a type is, however, straight-out lying to ourselves. The object is right there, empty, it's clearly not a user. We are making it impossible for TypeScript to detect certain runtime errors like nested objects read:
The proper way is to pass the User type to ref() :
This will make user.value be of type User | undefined and TypeScript will force us to check if the user is defined before accessing its properties. Another possible version is using ref<User | null>(null) with the explicit initial value.
reactive() is really neat because it allows us not to write .value everywhere. But it's also limited to objects and you can't replace the object itself. Let me show you what I mean:
If we try to reassign a value to items , we will get a Syntax Error, which is great! This error won't go unnoticed. The real problem comes when we use this outside of the store:
This is supposed to clear the todo list, except it doesn't. And worst of all is that we don't get any error about it đ±. At first, it will look like it worked but what we end up doing is disconnecting todo.items from the actual source of truth, located at store.$state.items . This will break Devtools, SSR Hydration, and Plugins. Overall, we end up with bugs that are really hard to trace.
My recommendation is to stick to ref() for arrays and objects. You can still use reactive() with collections like Set and Map because they are less likely to be replaced thanks to their method clear() . You can also stick to ref() in general, of course!
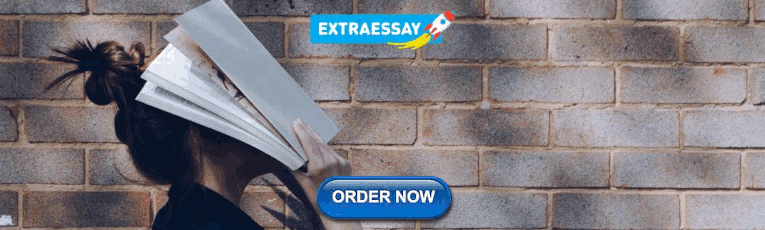
Using Deep reactivity with complex data
In Vue, Deep reactivity is the default. This is convenient. Things just work. However, it comes at the cost of performance when dealing with complex data like large collections that never change. One common example is fetching large set of data like products that we display on the page. They are usually fetched as a whole:
While the overhead is, in most scenarios negligible. It can become a problem when the data is large and the user is on a slower device. Opting out of Vue's deep reactivity change is a very simple change, it comes down to using one of the shallow equivalents or the [markRaw()](https://vuejs.org/api/reactivity-advanced.html#markraw) helper.
With shallowRef() only .value = ... will trigger reactivity. Very limited, but enough in some cases.
If you want to learn more about this topic, I recommend you to read this page in Vue.js Documentation about using shallow reactivity to reduce reactivity overhead.
I'm pretty much giving away the solution in the title so let's explore the problem first. Let's say we have a page that displays a list of products. We want to allow the user to filter the products by category. The user selects a filter on the page and we use that filter on our store:
While this solution works while the user is on the page, the selected category is completely lost if they reload the page or share the link with a friend. And it's such a shame, because the fix is so simple and it improves the user experience so much!
Within stores, we can get the current route with useRoute() , and the router instance with useRouter() . This allows us to create a computed property that returns the category from the URL but also, that can be set to push to the URL :
And that's it! Now the user can share the link with their friends and they will see the same products. They can also reload the page and the category will still be selected. This is a very simple example but it can be applied to any other state that you want to keep in the URL. You can even use VueUse's [useRouteQuery()](https://vueuse.org/router/useRouteQuery/#useroutequery) or roll your own composable to handle other types of values like numbers, booleans, and arrays.
Avoiding these common mistakes ensures a smoother and more maintainable application development process with Pinia. I hope you find these tips useful in your own projects. If you have any questions or want to share your own experience, please, reach out to me on Twitter .
The Mastering Pinia Course is Here!
Get a free lesson delivered to your inbox, just click on the button below.

Build better Webapps!

How to Watch Pinia State Inside Vue 3 Components
- January 25, 2023
In this tutorial, we will explore multiple ways to watch Pinia store (state, and getters) inside a Vue component. Let’s get started!
Watch a Single State Property Change
I will demonstrate with a user auth state example. Let’s imagine we have a user store that stores a single-state property for simplicity, we can call it isLoggedIn . First, define the store:
Then, use it in the component:
Now, if we click on the login button isLoggedIn will become true. Correspondingly, if we click on logout, isLoggedIn will become false.
Since we want to use the isLoggedIn property inside the template and have it reactive, we need to convert it into a ref. We use storeToRefs it for that.
To watch the property we can simply use the watch function from vue:
This works, however, there could be another use case for the store where we don’t want to use storeToRefs. For example, were only interested in watching and not rendering. Then we can use userStore.isLoggedIn directly.
However, if you try replacing isLoggedIn it won’t work. We need to wrap the value within an anonymous function:
In general, this applies to any reactive source that is not a ref! more on that in the official docs .
Comparatively, when using something like VueUse whenever , we should follow the same pattern, since the composable uses watch in the background:
Watch Multiple State Property Changes
Earlier, we demonstrated watching a single property value change. However, we can also watch the whole store. In other words, when any property changes we get notified inside our component!
Using the $subscribe method, we can pass an anonymous function that will be executed every time a change happens:
If we trigger a change, we will see the following in the console:

As you can see, we get in the mutation object and events object which we can use to figure out which property changed, In addition, we get the fully updated state as a second parameter.
Furthermore, if we change multiple properties within our action, we will still get a single update notification.
Add another property to the state and mutate it in the login action:
and in the console you shall see:

Watch Getters Value Changes
We can watch getters in a similar fashion to watching the state. We use an anonymous function when using the value directly, and we watch the value directly when it is a ref.
If we add in the store a getter:
then we can watch it in this manner:
Thank you for reading! If you want to learn more about Pinia and Vue make sure to check the other tutorials .
Amenallah Hsoumi
Senior Software Engineer, Indie Hacker, building cool stuff on the web!
Related Posts

How to use and Test Vue Template Refs with Vitest
- February 14, 2024

How to Detect Outside-Click with Vue 3
- September 9, 2023

How to Type Pinia Store with Typescript
- January 16, 2023
Trending now


DEV Community

Posted on Mar 24, 2023 • Updated on Mar 27, 2023
Pinia - Crash Course for Beginners
Introduction.
Hello everyone, in this crash course we will be talking about Pinia - the state management library for Vue. We will also be building a simple shopping cart project to showcase how Pinia stores work.
You can follow along here:
The code for the project can be found on my GitHub: https://github.com/alexander-gekov/pinia-cart-tutorial
- What is Pinia? (store, state, getters, actions)
- Creating a Shopping Cart Project with Pinia
- Setting up a Vue.js app with Vite
- Installing and Registering Pinia
- Creating a Pinia Store
- Using store in component and Pinia DevTools
Creating the Cart Store
Adding functionality for cart.
- Useful resources
What is Pinia?
Pinia is a state management library for Vue.js.
It is the successor of Vuex the original state management system for Vue. However, nowadays Pinia is the recommended way to manage state as said by the Vue.js Core Team.
The problem that state management solves is the problem of keeping shared state across your Vue components. Without it a lot of the state would have to be passed around using endless amount of props and emits.
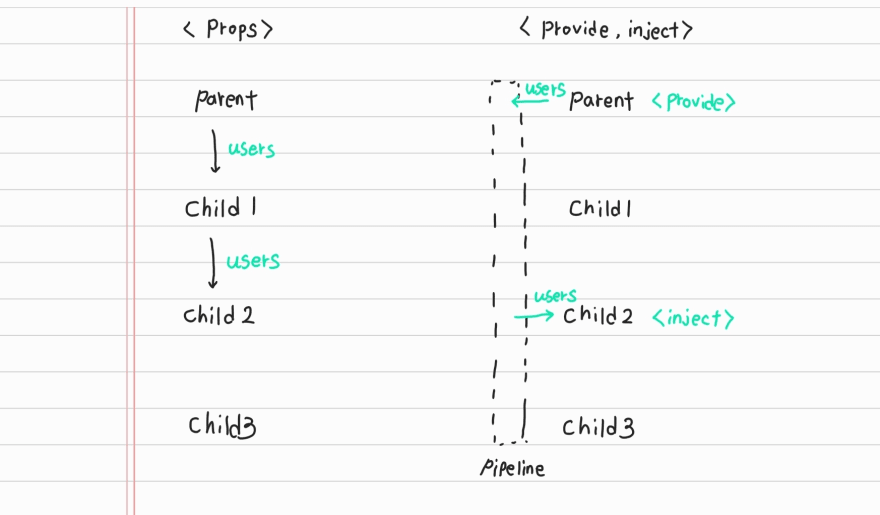
Letâs say we have a user who is authenticated, we would like to share that state across our components. We can also later store it in a cookie or local storage so that it persists even after page loads.
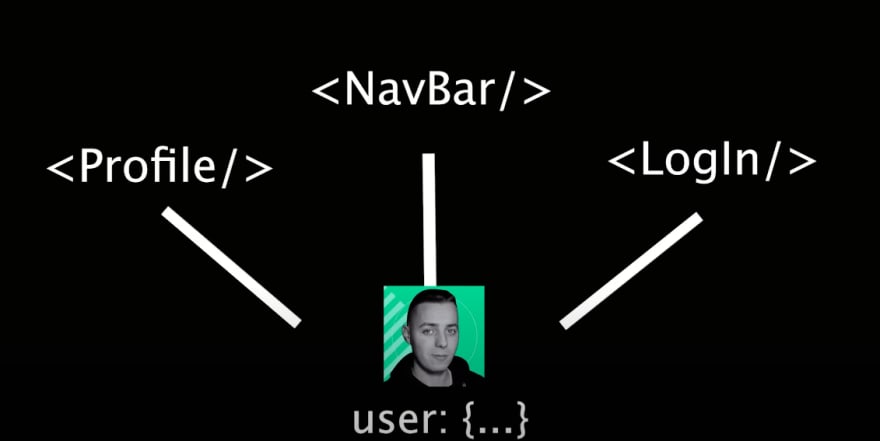
Pinia uses âstoresâ in order to manage state. A Store is compromised of:
- state (The data that we want to share)
- getters (A way for us to get the data from the state, read only)
- actions (Methods that we can use to modify/mutate the data in the state)
- mutations - One of the differences between Pinia and Vuex is that Pinia does not have explicit mutations defined in the store. They were removed from the store definition due to being too verbose. Read till the end to find out how to monitor mutations in your app.
Another differences between Pinia and Vuex is that Pinia is modular. What that means is that Pinia encourages users to have different stores, each corresponding to a different logic in our app. This is a great approach because it follows the separation of concern principle. On the other hand Vuex had developers manage a single bulky store for the whole application. You can see where I am going with thisâŠ
Pinia also has great Typescript support and autocompletion. This helps with the overall development experience, a metric that is becoming ever more important in modern frontend tools.
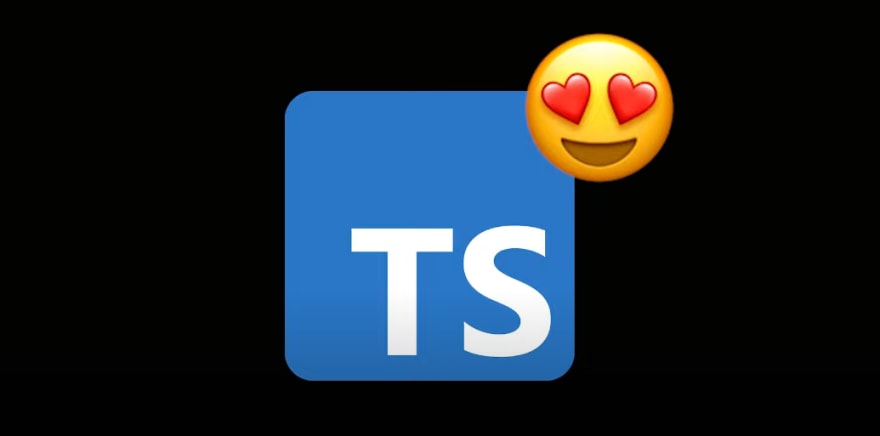
Another reason to try out Pinia, last one I promise đ, is that it is incredibly lightweight with a total bundle size of just 1KB.
Shopping Cart App
What we are going to build today to showcase how Pinia works is a simple ecommerce-like website. We will have some products that the user can add to their cart. The cart in the top right corner will need to keep track of the items that the user has added. It will also have to keep track of the quantity of each product as well as calculate the total count and total price.
The design was made with tailwindcss, however, this article will not be focusing on the styling part. If you want you can go to the GitHub repository and clone it to start with a simple UI template I prepared with hardcoded values.
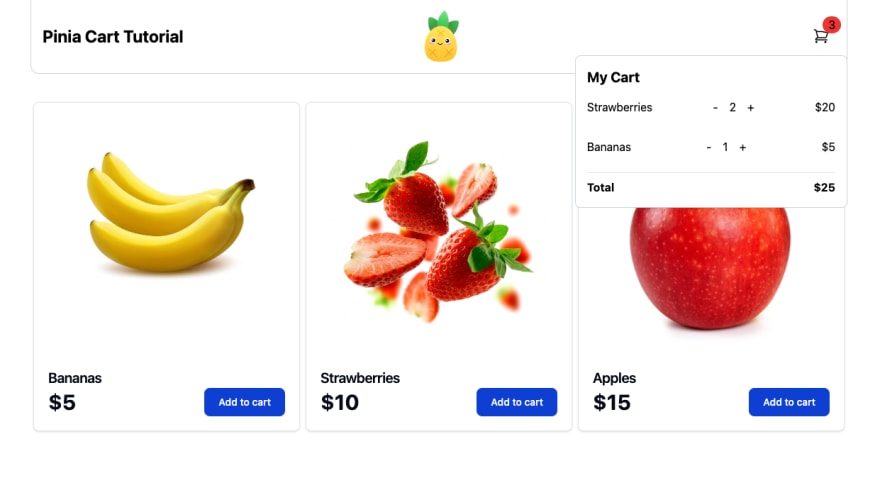
Setting up a Vue.js app with Vite (using vue-ts template)
Letâs start by creating our Vue app. You can use the Vue CLI or Vite for this. I will use Vite as well as the âvue-tsâ template provided by vite.
Once created, cd into the just created project folder and open it in VS Code. In VS Code I will open the terminal and run npm install .
Now, we can remove the boilerplate code - so remove the Hello World and any unnecessary styling. As I mentioned I wonât go over creating the components and styles, but feel free to look at the GitHub link.
Installing and registering Pinia
Letâs install Pinia by running:
Once done, we need to go to our main.ts file and register it like this:
Now, Pinia should be successfully installed.
Creating the Product Pinia Store
Currently we have an array in our App.vue that we are passing to our components through props. While this is by any means, a valid and totally ok way to pass the state. Imagine if the app was more complex and contained a lot more nested components. We should create a store to keep that state of products.
In our src folder, letâs create a folder called stores . In there create a file ProductStore.ts :
- We start by importing the defineStore method from pinia . We then use it to export a const called useProductStore . It is a common convention to prefix stores and composables with the âuseâ word.
- defineStore accepts two arguments, the first being the name of the store, and the second depending on how we write our store (Options API vs Composition API) will either be the object containing the state, getters and actions or a callback that will return an object with the state, getters, actions.
- In our store, we are using the Composition API so: ref and reactive become the state, computed variables become getters and methods become actions.
Here is a simple image showcasing Options API store vs Composition API Store:
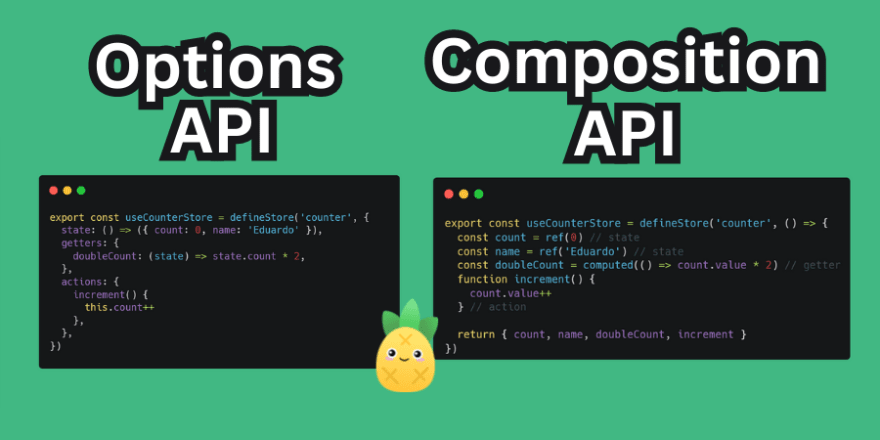
Using the Pinia store in a component
Back in App.vue we can import it like this:
As you can see we import it and then just initialize it. In order to verify that itâs working, we can open our app in Chrome and open Vue Devtools. There should be a new tab for Pinia, where you will be able to see your stores and other relevant data.
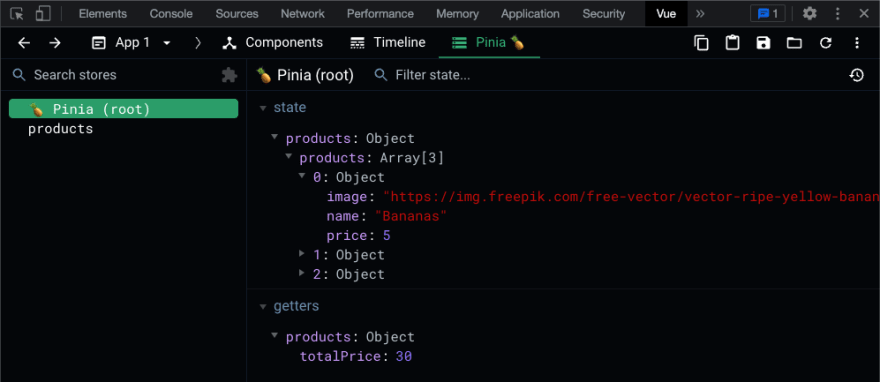
Whatâs more we if we go to the Timeline tab and then to Pinia, we can monitor different actions and mutations happening throughout our app:

Going back to the stores folder, letâs create CartStore.ts :
In this store we keep an array which will hold the products. We have getters for the total count of all products as well as grouping them by their name. This is used to later get the quantity of each product. We also have some actions for adding and removing a product as well as a reset method that will reset the state of the store.
Our App.vue should look like this:
- NavBar - the NavBar contains the shopping cart icon. The NavBar emits an event toggleCart and that is used to toggle the state of showCart which itself toggles the Cart dropdown.
- Cart - which is the Cart Dropdown, where the added products will be displayed. More on that in a moment.
- Product Card - these are our products, we use the productStore to loop over all the products and display them. The Card also emits an event called addToCart. We then use the cartStore to call the addItem action and add the product to the state.
This is how NavBar.vue shoud look like:
- We emit an event âtoggleCartâ
- We have a bubble notifying us how many products are in the cart
- We import the useCartStore , however when we want only one or two variables, we can destructure our store using the storeToRefs helper. It will unsure that reactivity is preserved when destructuring our store.
Lastly, this is how Cart.vue looks like:
- We import the useCartStore and we use it to get access to items , groupedItems , removeItem and addItem .
- We loop over our groupedItems and display the name, then on removing or adding we use the item at index 0, so the item that is first in the array.
- We also calculate the total price per product by using the .reduce method to sum up the individual costs.
- Lastly we also calculate the total price for all products, again using the .reduce method

We are now done with our application. You can go on and try adding items to cart. Changing the quantity to 100, or changing it 0 and it disappearing from the cart.
I hope you liked this crash course about Pinia and managed to use it in our example app. If you have any questions donât hesitate to reach out.
Useful Resources
You can always refer to the official documentation, it is really clear and helpful.
- GitHub Shopping Cart Template Starter
- VueSchoolâs courses
đ  If you want to learn more about Vue and the Vue ecosystem make sure to follow me on my socials. I create Vue content every week and am slowly starting to gain traction so Iâd really appreciate your help!
Top comments (2)
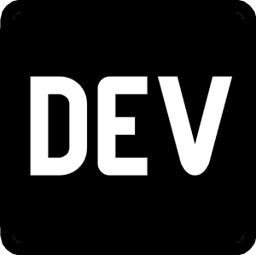
Templates let you quickly answer FAQs or store snippets for re-use.

- Location United States
- Education Self taught
- Work Freelance Software Engineer
- Joined Jul 24, 2017
Great article. I am jumping back into Vue recently. So this crash course in Pinia is most helpful. Looking forward to learning more from your articles and videos.

- Location Czech republic, near by capital city
- Joined Jan 25, 2021
Nice article, but a few oddities:
1) Why is there a method in useStore for the total price of all products if it is not used anywhere?
2) Why on the other hand is there no computed method in useCart for the total price in the cart and it is calculated in the template via reduce? (ugly) and also the method on price per product * number of units?
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse

Solving a Booking System Issue on a Photography Website: My Journey in the HNG Internship.
Solomon Uche - Jun 29
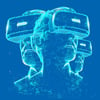
How a âSkeleton Keyâ Could Crack Open Your AIâs Secrets
Amulya Kumar - Jun 29

Introduction to the Periodic Table of DevOps Tools
Aaditya Kediyal - Jun 25

Introducing Animata
Hari Lamichhane - Jun 28
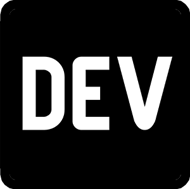
We're a place where coders share, stay up-to-date and grow their careers.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Cannot update states using this in Pinia actions #1267
{{editor}}'s edit
Col-bc may 2, 2022.
I'm very new to Pinia and had only a little experience with Vuex. I'm trying to update the from inside an function but keep getting this error: This is my @/store/index.js fils The only way I've been able to successfully update is if I use the function from within a component or view. Thank you in advance! |
Beta Was this translation helpful? Give feedback.
I believe I found the problem. If use the following syntax for defining actions, they execute without error:
Replies: 2 comments
Col-bc may 2, 2022 author.
I believe I found the problem. If use the following syntax for defining actions, they execute without error: |
lex-gh Nov 25, 2022
Arrow functions don't have their own bindings to |
- Numbered list
- Unordered list
- Attach files
Select a reply
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- PortuguĂȘs (do Brasil)
TypeError: invalid assignment to const "x"
The JavaScript exception "invalid assignment to const" occurs when it was attempted to alter a constant value. JavaScript const declarations can't be re-assigned or redeclared.
What went wrong?
A constant is a value that cannot be altered by the program during normal execution. It cannot change through re-assignment, and it can't be redeclared. In JavaScript, constants are declared using the const keyword.
Invalid redeclaration
Assigning a value to the same constant name in the same block-scope will throw.
Fixing the error
There are multiple options to fix this error. Check what was intended to be achieved with the constant in question.
If you meant to declare another constant, pick another name and re-name. This constant name is already taken in this scope.
const, let or var?
Do not use const if you weren't meaning to declare a constant. Maybe you meant to declare a block-scoped variable with let or global variable with var .
Check if you are in the correct scope. Should this constant appear in this scope or was it meant to appear in a function, for example?
const and immutability
The const declaration creates a read-only reference to a value. It does not mean the value it holds is immutable, just that the variable identifier cannot be reassigned. For instance, in case the content is an object, this means the object itself can still be altered. This means that you can't mutate the value stored in a variable:
But you can mutate the properties in a variable:
TypeError: Assignment to Constant Variable in JavaScript
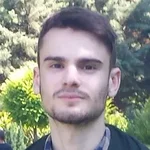
Last updated: Mar 2, 2024 Reading time · 3 min
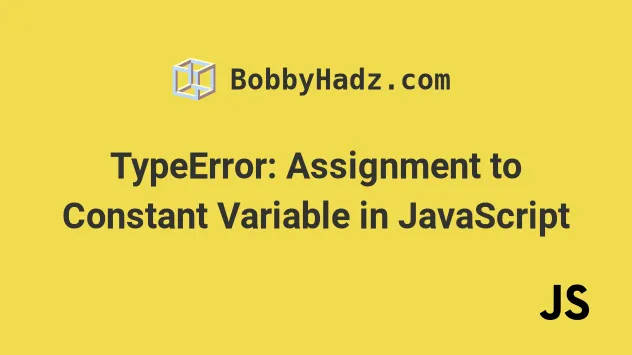
# TypeError: Assignment to Constant Variable in JavaScript
The "Assignment to constant variable" error occurs when trying to reassign or redeclare a variable declared using the const keyword.
When a variable is declared using const , it cannot be reassigned or redeclared.

Here is an example of how the error occurs.
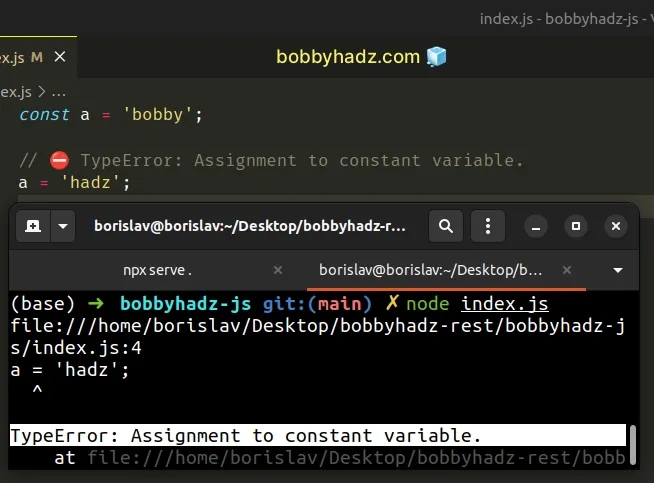
# Declare the variable using let instead of const
To solve the "TypeError: Assignment to constant variable" error, declare the variable using the let keyword instead of using const .
Variables declared using the let keyword can be reassigned.
We used the let keyword to declare the variable in the example.
Variables declared using let can be reassigned, as opposed to variables declared using const .
You can also use the var keyword in a similar way. However, using var in newer projects is discouraged.
# Pick a different name for the variable
Alternatively, you can declare a new variable using the const keyword and use a different name.
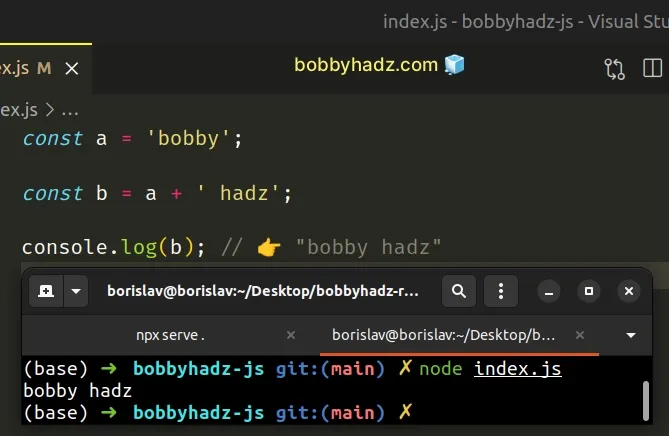
We declared a variable with a different name to resolve the issue.
The two variables no longer clash, so the "assignment to constant" variable error is no longer raised.
# Declaring a const variable with the same name in a different scope
You can also declare a const variable with the same name in a different scope, e.g. in a function or an if block.
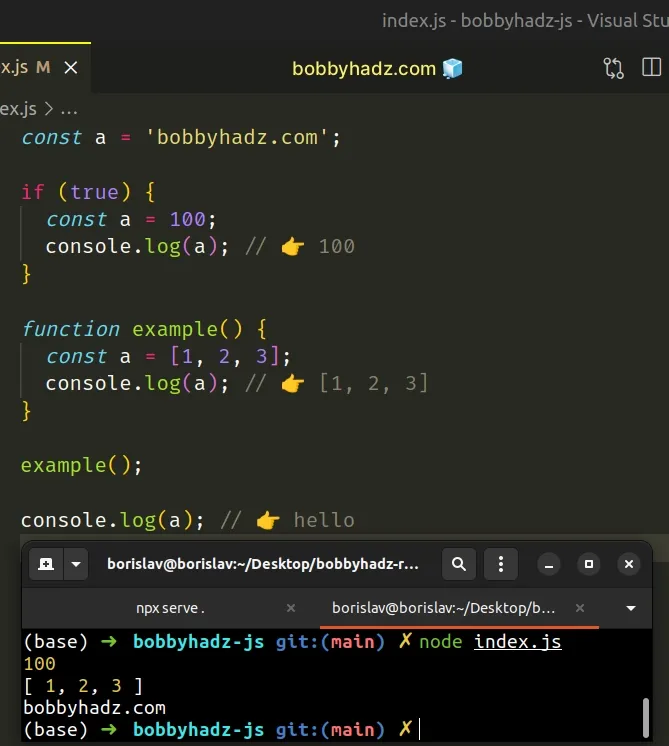
The if statement and the function have different scopes, so we can declare a variable with the same name in all 3 scopes.
However, this prevents us from accessing the variable from the outer scope.
# The const keyword doesn't make objects immutable
Note that the const keyword prevents us from reassigning or redeclaring a variable, but it doesn't make objects or arrays immutable.
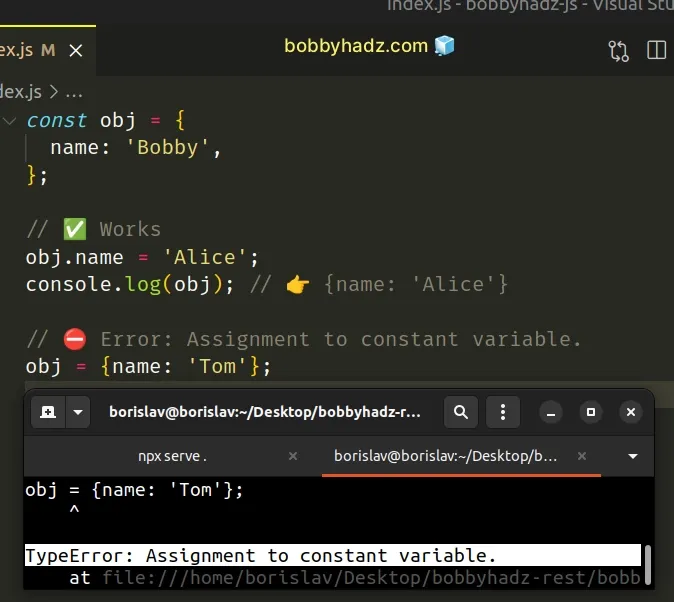
We declared an obj variable using the const keyword. The variable stores an object.
Notice that we are able to directly change the value of the name property even though the variable was declared using const .
The behavior is the same when working with arrays.
Even though we declared the arr variable using the const keyword, we are able to directly change the values of the array elements.
The const keyword prevents us from reassigning the variable, but it doesn't make objects and arrays immutable.
# Additional Resources
You can learn more about the related topics by checking out the following tutorials:
- SyntaxError: Unterminated string constant in JavaScript
- TypeError (intermediate value)(...) is not a function in JS
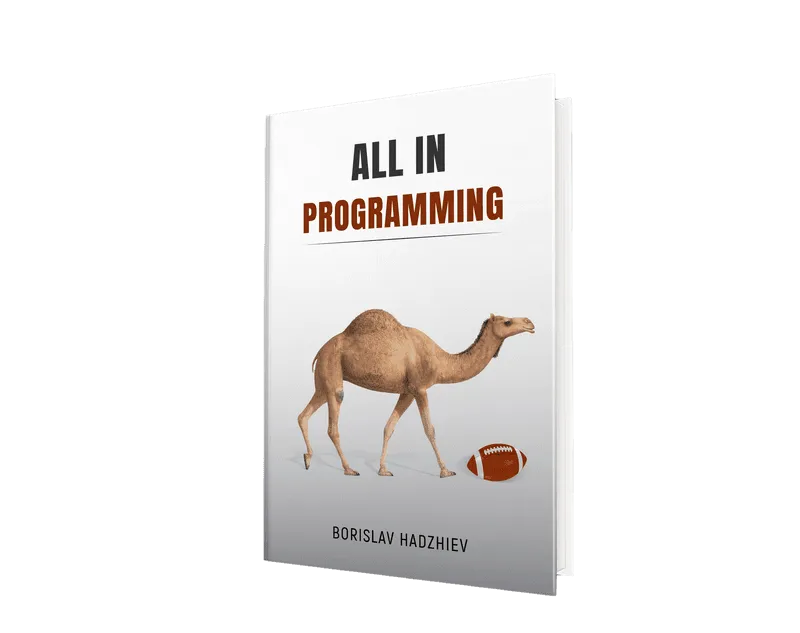
Borislav Hadzhiev
Web Developer
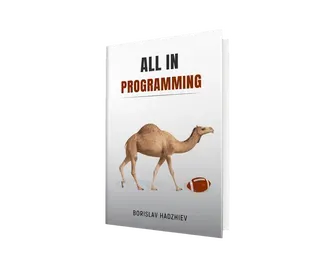
Copyright © 2024 Borislav Hadzhiev
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectivesâą on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Assignment to constant variable
I try to read the user input and send it as a email. But when I run this code it gives me this error: Assignment to constant variable.

You might want:
I would think the latter option is better, at least stylistically.
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking âPost Your Answerâ, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged node.js nodemailer or ask your own question .
- The Overflow Blog
- How to build open source apps in a highly regulated industry
- Community Products Roadmap Update, July 2024
- Featured on Meta
- We spent a sprint addressing your requests â hereâs how it went
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
- Policy: Generative AI (e.g., ChatGPT) is banned
- The [lib] tag is being burninated
- What makes a homepage useful for logged-in users
Hot Network Questions
- In equation (3) from lecture 7 in Leonard Susskind’s ‘Classical Mechanics’, should the derivatives be partial?
- Can you arrange 25 whole numbers (not necessarily all different) so that the sum of any three successive terms is even but the sum of all 25 is odd?
- Why is a game's minor update (e.g., New World) ~15 GB to download?
- How to clean up interrupted edge loops using geometry nodes and fill holes with quad faces?
- Why does Paul's fight with Feyd-Rautha take so long?
- mirrorlist.centos.org no longer resolve?
- Inversion naming conventions
- Use of Compile[] to optimize the code to run faster
- Sitting on a desk or at a desk? What's the diffrence?
- Ideal diode in parallel with resistor and voltage source
- Will electrolysis hydrolyze esters?
- Contains floating input pins
- Travel to Mexico from India, do I need to pay a fee?
- Why should the Vce be half the value of the supply source?
- Help identifying hollow spring / inverted header sockets
- What does '\($*\)' mean in sed regular expression in a makefile?
- Why would a plane be allowed to fly to LAX but not Maui?
- Tikz Border Problem
- Can you help me to identify the aircraft in a 1920s photograph?
- What’s the highest salary the greedy king can arrange for himself?
- How to maintain dependencies shared among microservices?
- How to make D&D easier for kids?
- How much is USA - Brazil?
- Can you always extend an isometry of a subset of a Hilbert Space to the whole space?
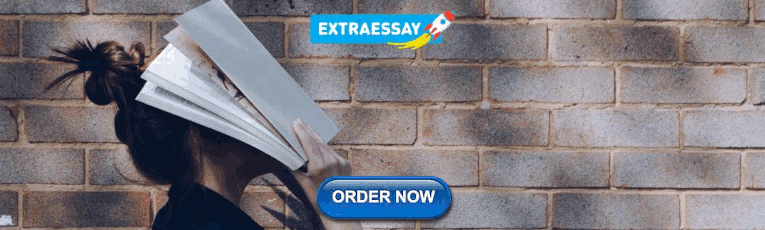
IMAGES
VIDEO
COMMENTS
State is typically just the initialized, typed variables. Getters are computed returnable values without altering the state data. Actions change the state data. You should only change the state data with store actions, not from within the component instance outside of pinia. All the data, the source of truth, stays in pinia the whole time.
The state is, most of the time, the central part of your store. People often start by defining the state that represents their app. In Pinia the state is defined as a function that returns the initial state. This allows Pinia to work in both Server and Client Side. If you are using Vue 2, the data you create in state follows the same rules as ...
// loses reactivity const email = authStore.loginUser creates an email constant with the current value of authStore.loginUser, losing reactivity.To keep reactivity, you could use computed:. import { computed } from 'vue' // keeps reactivity const email = computed({ get() { return authStore.loginUser }, set(val) { authStore.loginUser = val } }) // email is now a computed ref
Stores can have state, actions, and getters. To use Pinia in your Vue 3 application, you'll first need to install it: Once Pinia is installed, you'll need to create a Pinia instance and install it in your Vue app: import { createPinia } from 'pinia'. import App from './App.vue'. const app = createApp(App)
const pinia = createPinia(); const app = createApp(App); app.use(pinia); app.mount('#app') As you see in this code we imported the createPinia () function from pinia to create a new Pinia instance. Then invoking the createPinia () and assign it to pinia variable and finally we provide it to Vue using app.use (pinia). That's it.
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
Pinia offers a better developer experience (DX) than Nuxt's useState by providing more features that you'll likely need as your application grows in size and complexity. In other words, if you don't use Pinia, there's a good chance you'll find yourself re-inventing it and building your own state management library.
Pinia, the official state management solution for Vue 3, just reached 4 years old! đ This means we've had plenty of time to see it in action, to see it succeed but also fail. Let me share with you some of the most common mistakes I have seen in projects using Pinia and how to fix them. Here's the TL;DR: Calling useStore() in the wrong places
Add another property to the state and mutate it in the login action: and in the console you shall see: We can watch getters in a similar fashion to watching the state. We use an anonymous function when using the value directly, and we watch the value directly when it is a ref. If we add in the store a getter:
What is Pinia? Pinia is a state management library for Vue.js. It is the successor of Vuex the original state management system for Vue. However, nowadays Pinia is the recommended way to manage state as said by the Vue.js Core Team. The problem that state management solves is the problem of keeping shared state across your Vue components.
I'm very new to Pinia and had only a little experience with Vuex. I'm trying to update the state from inside an action function but keep getting this error: TypeError: Cannot set properties of undefined (setting 'token') at Proxy.setToken. This is my @/store/index.js fils. import { defineStore } from 'pinia'.
The const declaration creates a read-only reference to a value. It does not mean the value it holds is immutable, just that the variable identifier cannot be reassigned. For instance, in case the content is an object, this means the object itself can still be altered. This means that you can't mutate the value stored in a variable: js.
To solve the "TypeError: Assignment to constant variable" error, declare the variable using the let keyword instead of using const. Variables declared using the let keyword can be reassigned. The code for this article is available on GitHub. We used the let keyword to declare the variable in the example. Variables declared using let can be ...
1. You have to specify <script setup> for Composition API or else you have to expose the properties manually to the template by returning them. <template>. {{ counter }} </template>. <script setup>. import { storeToRefs } from "pinia";
Maybe what you are looking for is Object.assign(resObj, { whatyouwant: value} ). This way you do not reassign resObj reference (which cannot be reassigned since resObj is const), but just change its properties.. Reference at MDN website. Edit: moreover, instead of res.send(respObj) you should write res.send(resObj), it's just a typo
I see two "nested" setTimeout, but this is just a side note. The main issue is that you define packagesList as reactive.This returns an object and not the array you are expecting.
2. Imports are read-only live bindings to the original variable in the exporting module. The "read-only" part means you can't directly modify them. The "live" part means that you can see any modifications made to them by the exporting module. If you have a module that needs to allow other modules to modify the values of its exports (which is ...
What I see is that you assigned the variable apartments as an array and declared it as a constant. Then, you tried to reassign the variable to an object. When you assign the variable as a const array and try to change it to an object, you are actually changing the reference to the variable, which is not allowed using const.. const apartments = []; apartments = { link: getLink, descr ...
Assignment to constant variable. Ask Question Asked 5 years, 6 months ago. Modified 2 months ago. Viewed 95k times 11 I try to read the user input and send it as a email. But when I run this code it gives me this error: Assignment to constant variable. var mail= require ...