- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
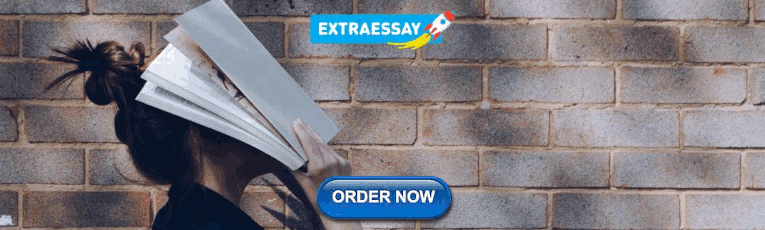
Destructuring assignment
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables.
Description
The object and array literal expressions provide an easy way to create ad hoc packages of data.
The destructuring assignment uses similar syntax but uses it on the left-hand side of the assignment instead. It defines which values to unpack from the sourced variable.
Similarly, you can destructure objects on the left-hand side of the assignment.
This capability is similar to features present in languages such as Perl and Python.
For features specific to array or object destructuring, refer to the individual examples below.
Binding and assignment
For both object and array destructuring, there are two kinds of destructuring patterns: binding pattern and assignment pattern , with slightly different syntaxes.
In binding patterns, the pattern starts with a declaration keyword ( var , let , or const ). Then, each individual property must either be bound to a variable or further destructured.
All variables share the same declaration, so if you want some variables to be re-assignable but others to be read-only, you may have to destructure twice — once with let , once with const .
In many other syntaxes where the language binds a variable for you, you can use a binding destructuring pattern. These include:
- The looping variable of for...in for...of , and for await...of loops;
- Function parameters;
- The catch binding variable.
In assignment patterns, the pattern does not start with a keyword. Each destructured property is assigned to a target of assignment — which may either be declared beforehand with var or let , or is a property of another object — in general, anything that can appear on the left-hand side of an assignment expression.
Note: The parentheses ( ... ) around the assignment statement are required when using object literal destructuring assignment without a declaration.
{ a, b } = { a: 1, b: 2 } is not valid stand-alone syntax, as the { a, b } on the left-hand side is considered a block and not an object literal according to the rules of expression statements . However, ({ a, b } = { a: 1, b: 2 }) is valid, as is const { a, b } = { a: 1, b: 2 } .
If your coding style does not include trailing semicolons, the ( ... ) expression needs to be preceded by a semicolon, or it may be used to execute a function on the previous line.
Note that the equivalent binding pattern of the code above is not valid syntax:
You can only use assignment patterns as the left-hand side of the assignment operator. You cannot use them with compound assignment operators such as += or *= .
Default value
Each destructured property can have a default value . The default value is used when the property is not present, or has value undefined . It is not used if the property has value null .
The default value can be any expression. It will only be evaluated when necessary.
Rest property
You can end a destructuring pattern with a rest property ...rest . This pattern will store all remaining properties of the object or array into a new object or array.
The rest property must be the last in the pattern, and must not have a trailing comma.
Array destructuring
Basic variable assignment, destructuring with more elements than the source.
In an array destructuring from an array of length N specified on the right-hand side of the assignment, if the number of variables specified on the left-hand side of the assignment is greater than N , only the first N variables are assigned values. The values of the remaining variables will be undefined.
Swapping variables
Two variables values can be swapped in one destructuring expression.
Without destructuring assignment, swapping two values requires a temporary variable (or, in some low-level languages, the XOR-swap trick ).
Parsing an array returned from a function
It's always been possible to return an array from a function. Destructuring can make working with an array return value more concise.
In this example, f() returns the values [1, 2] as its output, which can be parsed in a single line with destructuring.
Ignoring some returned values
You can ignore return values that you're not interested in:
You can also ignore all returned values:
Using a binding pattern as the rest property
The rest property of array destructuring assignment can be another array or object binding pattern. The inner destructuring destructures from the array created after collecting the rest elements, so you cannot access any properties present on the original iterable in this way.
These binding patterns can even be nested, as long as each rest property is the last in the list.
On the other hand, object destructuring can only have an identifier as the rest property.
Unpacking values from a regular expression match
When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to unpack the parts out of this array easily, ignoring the full match if it is not needed.
Using array destructuring on any iterable
Array destructuring calls the iterable protocol of the right-hand side. Therefore, any iterable, not necessarily arrays, can be destructured.
Non-iterables cannot be destructured as arrays.
Iterables are only iterated until all bindings are assigned.
The rest binding is eagerly evaluated and creates a new array, instead of using the old iterable.
Object destructuring
Basic assignment, assigning to new variable names.
A property can be unpacked from an object and assigned to a variable with a different name than the object property.
Here, for example, const { p: foo } = o takes from the object o the property named p and assigns it to a local variable named foo .
Assigning to new variable names and providing default values
A property can be both
- Unpacked from an object and assigned to a variable with a different name.
- Assigned a default value in case the unpacked value is undefined .
Unpacking properties from objects passed as a function parameter
Objects passed into function parameters can also be unpacked into variables, which may then be accessed within the function body. As for object assignment, the destructuring syntax allows for the new variable to have the same name or a different name than the original property, and to assign default values for the case when the original object does not define the property.
Consider this object, which contains information about a user.
Here we show how to unpack a property of the passed object into a variable with the same name. The parameter value { id } indicates that the id property of the object passed to the function should be unpacked into a variable with the same name, which can then be used within the function.
You can define the name of the unpacked variable. Here we unpack the property named displayName , and rename it to dname for use within the function body.
Nested objects can also be unpacked. The example below shows the property fullname.firstName being unpacked into a variable called name .
Setting a function parameter's default value
Default values can be specified using = , and will be used as variable values if a specified property does not exist in the passed object.
Below we show a function where the default size is 'big' , default co-ordinates are x: 0, y: 0 and default radius is 25.
In the function signature for drawChart above, the destructured left-hand side has a default value of an empty object = {} .
You could have also written the function without that default. However, if you leave out that default value, the function will look for at least one argument to be supplied when invoked, whereas in its current form, you can call drawChart() without supplying any parameters. Otherwise, you need to at least supply an empty object literal.
For more information, see Default parameters > Destructured parameter with default value assignment .
Nested object and array destructuring
For of iteration and destructuring, computed object property names and destructuring.
Computed property names, like on object literals , can be used with destructuring.
Invalid JavaScript identifier as a property name
Destructuring can be used with property names that are not valid JavaScript identifiers by providing an alternative identifier that is valid.
Destructuring primitive values
Object destructuring is almost equivalent to property accessing . This means if you try to destruct a primitive value, the value will get wrapped into the corresponding wrapper object and the property is accessed on the wrapper object.
Same as accessing properties, destructuring null or undefined throws a TypeError .
This happens even when the pattern is empty.
Combined array and object destructuring
Array and object destructuring can be combined. Say you want the third element in the array props below, and then you want the name property in the object, you can do the following:
The prototype chain is looked up when the object is deconstructed
When deconstructing an object, if a property is not accessed in itself, it will continue to look up along the prototype chain.
Specifications
Specification |
---|
Destructuring assignment
The two most used data structures in JavaScript are Object and Array .
- Objects allow us to create a single entity that stores data items by key.
- Arrays allow us to gather data items into an ordered list.
However, when we pass these to a function, we may not need all of it. The function might only require certain elements or properties.
Destructuring assignment is a special syntax that allows us to “unpack” arrays or objects into a bunch of variables, as sometimes that’s more convenient.
Destructuring also works well with complex functions that have a lot of parameters, default values, and so on. Soon we’ll see that.
Array destructuring
Here’s an example of how an array is destructured into variables:
Now we can work with variables instead of array members.
It looks great when combined with split or other array-returning methods:
As you can see, the syntax is simple. There are several peculiar details though. Let’s see more examples to understand it better.
It’s called “destructuring assignment,” because it “destructurizes” by copying items into variables. However, the array itself is not modified.
It’s just a shorter way to write:
Unwanted elements of the array can also be thrown away via an extra comma:
In the code above, the second element of the array is skipped, the third one is assigned to title , and the rest of the array items are also skipped (as there are no variables for them).
…Actually, we can use it with any iterable, not only arrays:
That works, because internally a destructuring assignment works by iterating over the right value. It’s a kind of syntax sugar for calling for..of over the value to the right of = and assigning the values.
We can use any “assignables” on the left side.
For instance, an object property:
In the previous chapter, we saw the Object.entries(obj) method.
We can use it with destructuring to loop over the keys-and-values of an object:
The similar code for a Map is simpler, as it’s iterable:
There’s a well-known trick for swapping values of two variables using a destructuring assignment:
Here we create a temporary array of two variables and immediately destructure it in swapped order.
We can swap more than two variables this way.
The rest ‘…’
Usually, if the array is longer than the list at the left, the “extra” items are omitted.
For example, here only two items are taken, and the rest is just ignored:
If we’d like also to gather all that follows – we can add one more parameter that gets “the rest” using three dots "..." :
The value of rest is the array of the remaining array elements.
We can use any other variable name in place of rest , just make sure it has three dots before it and goes last in the destructuring assignment.
Default values
If the array is shorter than the list of variables on the left, there will be no errors. Absent values are considered undefined:
If we want a “default” value to replace the missing one, we can provide it using = :
Default values can be more complex expressions or even function calls. They are evaluated only if the value is not provided.
For instance, here we use the prompt function for two defaults:
Please note: the prompt will run only for the missing value ( surname ).
Object destructuring
The destructuring assignment also works with objects.
The basic syntax is:
We should have an existing object on the right side, that we want to split into variables. The left side contains an object-like “pattern” for corresponding properties. In the simplest case, that’s a list of variable names in {...} .
For instance:
Properties options.title , options.width and options.height are assigned to the corresponding variables.
The order does not matter. This works too:
The pattern on the left side may be more complex and specify the mapping between properties and variables.
If we want to assign a property to a variable with another name, for instance, make options.width go into the variable named w , then we can set the variable name using a colon:
The colon shows “what : goes where”. In the example above the property width goes to w , property height goes to h , and title is assigned to the same name.
For potentially missing properties we can set default values using "=" , like this:
Just like with arrays or function parameters, default values can be any expressions or even function calls. They will be evaluated if the value is not provided.
In the code below prompt asks for width , but not for title :
We also can combine both the colon and equality:
If we have a complex object with many properties, we can extract only what we need:
The rest pattern “…”
What if the object has more properties than we have variables? Can we take some and then assign the “rest” somewhere?
We can use the rest pattern, just like we did with arrays. It’s not supported by some older browsers (IE, use Babel to polyfill it), but works in modern ones.
It looks like this:
In the examples above variables were declared right in the assignment: let {…} = {…} . Of course, we could use existing variables too, without let . But there’s a catch.
This won’t work:
The problem is that JavaScript treats {...} in the main code flow (not inside another expression) as a code block. Such code blocks can be used to group statements, like this:
So here JavaScript assumes that we have a code block, that’s why there’s an error. We want destructuring instead.
To show JavaScript that it’s not a code block, we can wrap the expression in parentheses (...) :
Nested destructuring
If an object or an array contains other nested objects and arrays, we can use more complex left-side patterns to extract deeper portions.
In the code below options has another object in the property size and an array in the property items . The pattern on the left side of the assignment has the same structure to extract values from them:
All properties of options object except extra which is absent in the left part, are assigned to corresponding variables:
Finally, we have width , height , item1 , item2 and title from the default value.
Note that there are no variables for size and items , as we take their content instead.
Smart function parameters
There are times when a function has many parameters, most of which are optional. That’s especially true for user interfaces. Imagine a function that creates a menu. It may have a width, a height, a title, an item list and so on.
Here’s a bad way to write such a function:
In real-life, the problem is how to remember the order of arguments. Usually, IDEs try to help us, especially if the code is well-documented, but still… Another problem is how to call a function when most parameters are ok by default.
That’s ugly. And becomes unreadable when we deal with more parameters.
Destructuring comes to the rescue!
We can pass parameters as an object, and the function immediately destructurizes them into variables:
We can also use more complex destructuring with nested objects and colon mappings:
The full syntax is the same as for a destructuring assignment:
Then, for an object of parameters, there will be a variable varName for the property incomingProperty , with defaultValue by default.
Please note that such destructuring assumes that showMenu() does have an argument. If we want all values by default, then we should specify an empty object:
We can fix this by making {} the default value for the whole object of parameters:
In the code above, the whole arguments object is {} by default, so there’s always something to destructurize.
Destructuring assignment allows for instantly mapping an object or array onto many variables.
The full object syntax:
This means that property prop should go into the variable varName and, if no such property exists, then the default value should be used.
Object properties that have no mapping are copied to the rest object.
The full array syntax:
The first item goes to item1 ; the second goes into item2 , and all the rest makes the array rest .
It’s possible to extract data from nested arrays/objects, for that the left side must have the same structure as the right one.
We have an object:
Write the destructuring assignment that reads:
- name property into the variable name .
- years property into the variable age .
- isAdmin property into the variable isAdmin (false, if no such property)
Here’s an example of the values after your assignment:
The maximal salary
There is a salaries object:
Create the function topSalary(salaries) that returns the name of the top-paid person.
- If salaries is empty, it should return null .
- If there are multiple top-paid persons, return any of them.
P.S. Use Object.entries and destructuring to iterate over key/value pairs.
Open a sandbox with tests.
Open the solution with tests in a sandbox.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
Coding Help Tips Resources Tutorials
Google Apps Scripts and Web Development Tutorials and Resources by Laurence Svekis

Mastering JavaScript Destructuring Assignment: A Comprehensive Guide
Destructuring assignment is a powerful feature in JavaScript introduced with ES6 (ECMAScript 2015). It allows you to unpack values from arrays or properties from objects into distinct variables in a more concise and readable manner. This guide will help you understand how to effectively use destructuring assignment in your JavaScript code, complete with examples and best practices.
What is Destructuring Assignment?
Destructuring assignment is a syntax that enables you to unpack values from arrays or objects into individual variables. This can greatly enhance the readability and maintainability of your code.
Destructuring Arrays
Array destructuring lets you extract values from an array into separate variables.
Basic Array Destructuring
const fruits = [‘apple’, ‘banana’, ‘cherry’];
const [first, second, third] = fruits;
console.log(first); // apple
console.log(second); // banana
console.log(third); // cherry
Skipping Items
You can skip items in the array by leaving empty slots.
const [first, , third] = fruits;
Using Rest Operator
The rest operator (…) can be used to collect the remaining elements.
const numbers = [1, 2, 3, 4, 5];
const [first, second, …rest] = numbers;
console.log(first); // 1
console.log(second); // 2
console.log(rest); // [3, 4, 5]
Destructuring Objects
Object destructuring allows you to unpack properties from an object into distinct variables.
Basic Object Destructuring
const person = {
name: ‘Alice’,
age: 30,
city: ‘New York’
const { name, age, city } = person;
console.log(name); // Alice
console.log(age); // 30
console.log(city); // New York
Renaming Variables
You can rename variables while destructuring.
const { name: personName, age: personAge, city: personCity } = person;
console.log(personName); // Alice
console.log(personAge); // 30
console.log(personCity); // New York
Default Values
You can set default values for variables in case the property does not exist in the object.
age: 30
const { name, age, city = ‘Unknown’ } = person;
console.log(city); // Unknown
Nested Destructuring
Destructuring can be used to extract values from nested objects and arrays.
Nested Object Destructuring
address: {
street: ‘123 Main St’,
city: ‘New York’
}
const { name, address: { street, city } } = person;
console.log(street); // 123 Main St
Nested Array Destructuring
const numbers = [1, [2, 3], 4, 5];
const [first, [second, third], fourth] = numbers;
console.log(third); // 3
console.log(fourth); // 4
Destructuring Function Parameters
Destructuring is particularly useful for handling function parameters, making the function signatures more readable and flexible.
Destructuring Objects in Function Parameters
function greet({ name, age }) {
return `Hello, my name is ${name} and I am ${age} years old.`;
console.log(greet(person)); // Hello, my name is Alice and I am 30 years old.
Destructuring Arrays in Function Parameters
function sum([a, b]) {
return a + b;
const numbers = [5, 10];
console.log(sum(numbers)); // 15
Real-World Example: API Response Handling
Destructuring is extremely useful when dealing with API responses.
const response = {
status: 200,
data: {
user: {
id: 1,
name: ‘Alice’,
email: ‘[email protected]’
}
const { status, data: { user: { id, name, email } } } = response;
console.log(status); // 200
console.log(id); // 1
console.log(email); // [email protected]
Destructuring assignment is a powerful feature in JavaScript that simplifies the process of extracting values from arrays and objects. By mastering destructuring, you can write cleaner, more concise, and more readable code. Practice using destructuring in various scenarios to become proficient in this technique.
Share this:
Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
- JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
- JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
- JavaScript throw Statement
- JavaScript Modules
JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
JavaScript Constructor Function
- JavaScript console.log()
JavaScript Destructuring Assignment
- JavaScript Destructuring
The destructuring assignment introduced in ES6 makes it easy to assign array values and object properties to distinct variables . For example, Before ES6:
Note : The order of the name does not matter in object destructuring.
For example, you could write the above program as:
Note : When destructuring objects, you should use the same name for the variable as the corresponding object key.
For example,
If you want to assign different variable names for the object key, you can use:
- Array Destructuring
You can also perform array destructuring in a similar way. For example,
- Assign Default Values
You can assign the default values for variables while using destructuring. For example,
In the above program, arrValue has only one element. Hence,
- the x variable will be 10
- the y variable takes the default value 7
In object destructuring, you can pass default values in a similar way. For example,
- Swapping Variables
In this example, two variables are swapped using the destructuring assignment syntax.
You can skip unwanted items in an array without assigning them to local variables. For example,
In the above program, the second element is omitted by using the comma separator , .
Assign Remaining Elements to a Single Variable
You can assign the remaining elements of an array to a variable using the spread syntax ... . For example,
Here, one is assigned to the x variable. And the rest of the array elements are assigned to y variable.
You can also assign the rest of the object properties to a single variable. For example,
Note : The variable with the spread syntax cannot have a trailing comma , . You should use this rest element (variable with spread syntax) as the last variable.
- Nested Destructuring Assignment
You can perform nested destructuring for array elements. For example,
Here, the variable y and z are assigned nested elements two and three .
In order to execute the nested destructuring assignment, you have to enclose the variables in an array structure (by enclosing inside [] ).
You can also perform nested destructuring for object properties. For example,
In order to execute the nested destructuring assignment for objects, you have to enclose the variables in an object structure (by enclosing inside {} ).
Note : Destructuring assignment feature was introduced in ES6 . Some browsers may not support the use of the destructuring assignment. Visit Javascript Destructuring support to learn more.
Table of Contents
- Skipping Items
- Arbitrary Number of Elements
Sorry about that.
Related Tutorials
JavaScript Tutorial

How to Use Object Destructuring in JavaScript
Object destructuring is a useful JavaScript feature to extract properties from objects and bind them to variables.
Even better, object destructuring can extract multiple properties in a single statement, can access properties from nested objects, and can set a default value if the property doesn't exist.
This post will help you understand how to use object destructuring in JavaScript.
Table of Contents
1. the need for object destructuring, 2. extracting a property, 3. extracting multiple properties, 4. default values, 6. extracting properties from nested objects, 7. extracting a dynamic name property, 8. rest object after destructuring, 9.1 bind properties to variables, 9.2 function parameter destructuring, 10. summary.
Imagine you'd like to extract some properties of an object. In a pre-ES2015 environment, you'd need to write the following code:
Open the demo.
The property hero.name value is assigned to the variable name . Same way hero.realName value is assigned to realName .
By writing var name = hero.name , you have to mention the name binding 2 times, and the same for realName . This way of accessing properties and assigning them to variables requires boilerplate code.
That's where the object destructuring syntax is useful: you can read a property and assign its value to a variable without duplicating the property name. What is more, you can read multiple properties from the same object in just one statement!
Let's refactor the above script and apply the object destructuring to access the properties name and realName :
const { name, realName } = hero is an object destructuring assignment. This statement defines the variables name and realName , then assigns to them the values of properties hero.name and hero.realName correspondingly.
Compare two approaches to accessing the object properties:
it's visible that object destructuring is handier because neither the property names nor the object variable is duplicated.
The syntax of object destructuring is pretty simple:
Where identifier is the name of the property to access and expression should evaluate to an object. After the destructuring, the variable identifier contains the property value.
Here's the equivalent code using a property accessor :
Let's try object destructuring in practice:
The statement const { name } = hero defines the variable name and initializes it with the value of hero.name property.
To destructure the object into multiple properties, enumerate as many properties as you like adding commas , in-between:
Where identifier1 , ..., identifierN are names of properties to access, and expression should evaluate to an object. After the destructuring, the variables identifier1 , ..., identifierN contain corresponding properties values.
Here's the equivalent code:
Let's take a look again at the example from the first section, where 2 properties are extracted:
const { name, realName } = hero creates 2 variables name and realName assigned with values of corresponding properties hero.name and hero.realName .
If the destructured object doesn't have the property specified in the destructuring assignment, then the variable is assigned with undefined . Let's see how it happens:
After destructuring the variable enemies is undefined because the property enemies doesn't exist in the object hero .
Fortunately, you can set a default value if the property doesn't exist in the destructured object:
Where identifier is the name of the property to access and expression should evaluate to an object. After destructuring, the variable identifier contains the property value or is assigned with defaultValue if the property identifier doesn't exist.
Let's change the previous code sample, and use the default value feature:
Now, instead of being undefined , the variable enemies defaults to ['Joker'] .
To create variables of different names than the properties you can use the aliasing feature of object destructuring.
identifier is the name of the property to access, aliasIdentifier is the variable name, and expression should evaluate to an object. After destructuring, the variable aliasIdentifier contains the property value.
The equivalent code:
Here's an example of an object destructuring alias feature:
Looking at const { realName: secretName } = hero , the destructuring defines a new variable secretName (alias variable) and assigns to it the value of hero.realName .
In the previous examples, the objects were plain: the properties have primitive data types (e.g. strings).
But objects can be nested in other objects. In other words, some properties can contain objects.
In such a case, you still can use the object destructuring and access properties from deep. Here's the basic syntax:
nestedObjectProp is the name of the property that holds a nested object. identifier is the property name to access from the nested object. expression should evaluate to the destructured object.
After destructuring, the variable identifier contains the property value of the nested object.
The above syntax is equivalent to:
The level of nesting to extract properties from is unlimited. If you want to extract properties from deep, just add more nested curly braces:
For example, the object hero contains a nested object { city: 'Gotham'} .
The object destructuring const { address: { city } } = hero accesses the property city from the nested object and creates a variable city having the property value.
You can extract into variables properties with a dynamic name (the property name is known at runtime):
propName expression should evaluate to a property name (usually a string), and the identifier should indicate the variable name created after destructuring. expression should evaluate to the object you'd like to destructure.
An equivalent code without object destructuring:
Let's look at an example where prop holds the property name:
const { [prop]: name } = hero is an object destructuring that assigns to variable name the value hero[prop] , where prop is a variable holding the property name.
The rest syntax is useful to collect the remaining properties after destructuring:
Where identifier is the name of the property to access and expression should evaluate to an object. After destructuring, the variable identifier contains the property value. rest variable is a plain object with the remaining properties.
For example, let's extract the property name , but collect the rest of the properties into a variable rest :
The destructuring const { name, ...realHero } = hero extracts the property name . Also, the remaining properties ( realName and company ) are collected into rest .
9. Common use cases
As seen in many examples before, the object destructuring binds property values to variables.
The object destructuring can assign values to variables declared using const , let , and var . Or even assign to an already existing variable.
For example, here's how to destructure using let statement:
How to destructure using var statement:
And how to destructure to an already declared variable:
I find it satisfying to combine for..of cycle with object destructuring to extract the property right away:
Object destructuring can be placed anywhere where an assignment happens.
For example, you could destruct an object right inside the parameter of a function:
function({ name }) destructures the function parameter and creates a variable name holding the value of name property.
Object destructuring is a powerful feature to extract properties from an object and bind these values to variables.
I like object destructuring due to the concise syntax and the ability to extract multiple variables in one statement.
Have questions regarding object destructuring? Ask in a comment below!
Like the post? Please share!
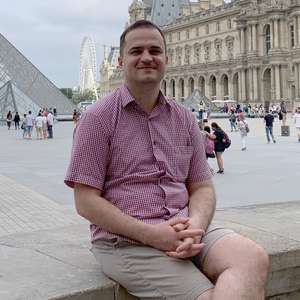
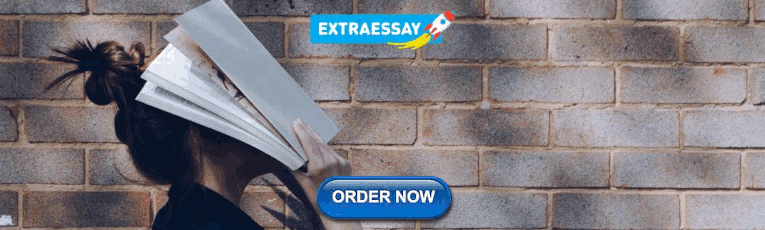
About Dmitri Pavlutin
Popular posts.
Destructuring in JavaScript – How to Destructure Arrays and Objects

Working with JavaScript arrays and objects can be more fun if you destructure them. This helps when you're fetching stored data.
In this article, you will learn how you can take destructuring to the next level in JavaScript arrays and objects.
Table of contents:
- What is an array?
- What is an object?
- What it means to destructure in JavaScript
Destructuring in Arrays
Destructuring in objects, what is an array in javascript.
In JavaScript, an array is a single variable that stores multiple elements. It is a collection of data. We can declare an array in two different ways, which are:
In method 1, you can initialize while declaring your array. In method 2, you declare your array with the number of elements to be stored before initializing.
What is an Object in JavaScript?
In JavaScript, an object is a collection of properties, and a property is an association between a name (or key ) and a value.
Writing an object in JavaScript looks somehow similar to an array, but instead we use curly braces or moustaches to create them. Let's look at the code below showing a car object with its properties:
Notice that what makes up an object is a key followed by its value.
Now that you know the basics of what JavaScript arrays and objects look like, let's talk more about destructuring.
What is Destructuring in JavaScript?
Imagine you want to pick out some shoes from your collection, and you want your favorite blue ones. The very first thing you have to do is to search through your collection and unpack whatever you have there.
Now destructuring is just like that approach you took when looking for your shoes. Destructuring is the act of unpacking elements in an array or object.
Destructuring not only allow us to unpack elements, it also gives you the power to manipulate and switch elements you unpacked depending on the type of operation you want to perform.
Let's see how destructuring works in arrays and objects now.
To destructure an array in JavaScript, we use the square brackets [] to store the variable name which will be assigned to the name of the array storing the element.
The ellipses right after the var2 declared above simply means that we can create more variables depending on how many items we want to remove from the array.
How to Assign Variables With Destructuring
Now, let's say we have an array of 6 colors but we want to get just the first 2 colors in the array. We can use destructuring to get what we want.
Let's take a look at it now:

When we run the above code, we should have red and yellow logged to the console. Awesome!
How to Swap Variables with Destructuring
Now that you know how to assign variables with destructuring, let's look at how you can use destructuring to quickly swap variable values.
Say we have an array of two elements, "food" and "fruits" , and we use destructuring to assign those values to the variables positionOne and positionTwo :
If we later want to swap the values of positionOne and positionTwo without destructuring, we would need to use another variable to temporarily hold the value of one of the current variables, then perform the swap.
For example:
But with destructuring, we could swap the values of positionOne and positionTwo really easily, without having to use a temporary variable:
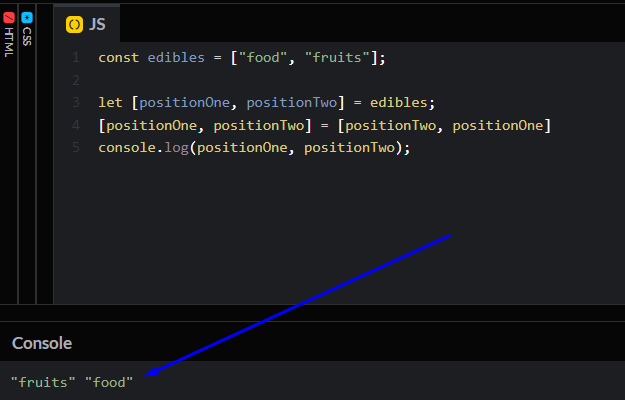
Note that this method of swapping variables doesn't mutate the original array. If you log edibles to the console, you'll see that its value is still ["food", "fruits"] .
How to Mutate Arrays with Destructuring
Mutating means changing the form or value of an element. A value is said to be mutable if it can be changed. With the help of destructing in arrays, we can mutate arrays themselves.
Say we have the same edibles array, and that we want to mutate the array by swapping the order of "food" and "fruits" .
We can do that with destructuring, similar to the way we swapped the values of two variables before:
When destructuring objects, we use curly braces with the exact name of what we have in the object. Unlike in arrays where we can use any variable name to unpack the element, objects allow just the use of the name of the stored data.
Interestingly, we can manipulate and assign a variable name to the data we want to get from the object. Let's see all of that now in code.

Logging what we have to the console shows the value of frontend and backend. Let's now see how to assign a variable name to the object we want to unpack.

As you can see, we have courseOne and courseTwo as the names of the data we want to unpack.
Assigning a variable name will always help us keep our code clean, especially when it comes to working with external data when we want to unpack and reuse it across our code.
Wrapping Up
You've now learned how to work with destructing in arrays and objects. You've also learned how to switch the positions of elements in arrays.
So what next? Try practicing and take your destructuring abilities to next level.
Hi😁! I am Alvin, a software engineer. I love building awesome stuffs, exploring new technologies as well as providing support through mentorship and tutorials to developers
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Home » JavaScript Tutorial » JavaScript Object Destructuring
JavaScript Object Destructuring
Summary : in this tutorial, you’ll learn about JavaScript object destructuring which assigns properties of an object to individual variables.
If you want to learn how to destructure an array , you can check out the array destructuring tutorial .
Introduction to the JavaScript object destructuring assignment
Suppose you have a person object with two properties: firstName and lastName .
Before ES6, when you want to assign properties of the person object to variables, you typically do it like this:
ES6 introduces the object destructuring syntax that provides an alternative way to assign properties of an object to variables:
In this example, the firstName and lastName properties are assigned to the fName and lName variables respectively.
In this syntax:
The identifier before the colon ( : ) is the property of the object and the identifier after the colon is the variable.
Notice that the property name is always on the left whether it’s an object literal or object destructuring syntax.
If the variables have the same names as the properties of the object, you can make the code more concise as follows:
In this example, we declared two variables firstName and lastName , and assigned the properties of the person object to the variables in the same statement.
It’s possible to separate the declaration and assignment. However, you must surround the variables in parentheses:
If you don’t use the parentheses, the JavaScript engine will interpret the left-hand side as a block and throw a syntax error.
When you assign a property that does not exist to a variable using the object destructuring, the variable is set to undefined . For example:
In this example, the middleName property doesn’t exist in the person object, therefore, the middleName variable is undefined .
Setting default values
You can assign a default value to the variable when the property of an object doesn’t exist. For example:
In this example, we assign an empty string to the middleName variable when the person object doesn’t have the middleName property.
Also, we assign the currentAge property to the age variable with the default value of 18.
However, when the person object does have the middleName property, the assignment works as usual:
Destructuring a null object
A function may return an object or null in some situations. For example:
And you use the object destructuring assignment:
The code will throw a TypeError :
To avoid this, you can use the OR operator ( || ) to fallback the null object to an empty object:
Now, no error will occur. And the firstName and lastName will be undefined .
Nested object destructuring
Assuming that you have an employee object which has a name object as the property:
The following statement destructures the properties of the nested name object into individual variables:
It’s possible to do multiple assignment of a property to multiple variables:
Destructuring function arguments
Suppose you have a function that displays the person object:
It’s possible to destructure the object argument passed into the function like this:
It looks less verbose especially when you use many properties of the argument object. This technique is often used in React.
- Object destructuring assigns the properties of an object to variables with the same names by default.

DEV Community

Posted on Dec 30, 2019
Destructuring JavaScript objects with default value
Originally posted at https://varundey.me/blog/destructuring-and-default-values/
Destructuring introduced in JavaScript ES6 is a nifty trick to scoop out properties directly from an object as variables.
Destructure and assign default values - the naive way
But what if you have to do some validation checks on your destructured properties before doing any operation
Destructure and assign default values - the JavaScript way
Though it works perfectly fine but it is boring and redundant. What if we could make use of default values (just like default arguments in functions) right at the time of destructuring objects so that our unpacked property is never undefined .
Easy right? You just need to assign the values as and when you unpack it.
Destructure, rename and assign default values
Neat! But what if we want to rename a parameter and set a default value to it? Pay attention.
Confusing? I bet it is. Here are the steps to it.
- First we destructure properties of the object
- Next, we assign variables to these properties
- After that, assign the default value as how we did in the previous example
And there you have it. Adding default values right at the time of unpacking objects.
Please note that destructuring with default value only works when there is no property to unpack in the object i.e. the property is undefined . This means that JavaScript treats null , false , 0 or other falsy values as a valid property and will not assign default value to them.
I hope this was informative and will help you when you need to do something similar. Let me know what you think about this post in the comments below. ✌️
Top comments (3)
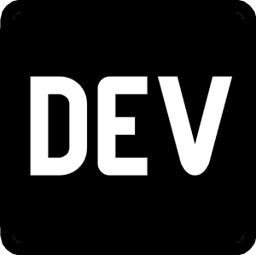
Templates let you quickly answer FAQs or store snippets for re-use.

- Location Ceará
- Education Graduate in Information System
- Joined Nov 20, 2019
Great article!

- Joined Sep 11, 2018
Great article Varun, you really explained this concept well!

- Location Berlin, Germany
- Education MSc Internet Applications Development
- Work Frontend Engineer at Outfittery
- Joined May 5, 2019
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse

Implementing Caching Strategies: Techniques for High-Performance Web Apps
nayanraj adhikary - Jun 22
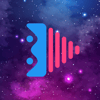
Resolve Content Security Policy (CSP) Issues in Your Discord Activity Using a Node.js Proxy
WavePlay Staff - Jun 17
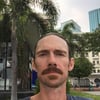
Latest Newsletter: Simulation Theory and Virtual Worlds (Issue #165)
Mark Smith - May 25

_Khojiakbar_ - Jun 17
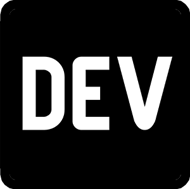
We're a place where coders share, stay up-to-date and grow their careers.
React Tutorial
React hooks, react exercises, react es6 destructuring, destructuring.
To illustrate destructuring, we'll make a sandwich. Do you take everything out of the refrigerator to make your sandwich? No, you only take out the items you would like to use on your sandwich.
Destructuring is exactly the same. We may have an array or object that we are working with, but we only need some of the items contained in these.
Destructuring makes it easy to extract only what is needed.
Destructing Arrays
Here is the old way of assigning array items to a variable:
Here is the new way of assigning array items to a variable:
With destructuring:
When destructuring arrays, the order that variables are declared is important.
If we only want the car and suv we can simply leave out the truck but keep the comma:
Destructuring comes in handy when a function returns an array:
Try it Yourself »
Get Certified!
Destructuring objects.
Here is the old way of using an object inside a function:
Here is the new way of using an object inside a function:
Notice that the object properties do not have to be declared in a specific order.
We can even destructure deeply nested objects by referencing the nested object then using a colon and curly braces to again destructure the items needed from the nested object:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
# Skipping Values In JavaScript Destructuring
You can use blanks to skip over unwanted values 🤓
This way you can avoid useless variable assignments for values you don’t want during destructuring 👍
You can also prefix "_" followed by the variable name you're disregarding. This helps communicates to your team member that it's a useless variable 🤝
# Add Comments to Improve Code Readability
When using the blank space option to skip over values, you can also add comments. This will help communicate to your fellow developers that you are intentionally skipping over the unwanted values.
# Simple Use Case
Here is a simple use case where this could be helpful.
# Community Input
: The underscore will be quite helpful. At least someone reading the codes won't have to trace what the variables look like. It can be stressful though when there are lots of variables in the destructured item (array or object).
: Learning that you can do [ , valueICareAbout] has been very useful. As most linters complain about unused variables.
: In TypeScript the convention is just _
Agreed, but always look at it pragmatically and have code readability in mind. It can get to absurd situation of making your fellow developer (or you in a future) to have to start counting commas to understand the code 😉
: Parsing comma-deliminated data and grabbing only what you need.
: Or, you can even destructure an array like an object where the key is the index and then you rename the key to the variable name you want const {1: name} = ['ignore', 'keep'] . The value for name would be keep due to that being the value at index 1 in the array.
: Or you can even (<-good start for syntactic gymnastics show:D) destructure the length and dynamically use it to get the last item, not knowing that index:
☝️ This example is pretty gangster. It's destructuring the length from the array and assigning it to a new variable l . I can also write this without the assignment.
# Resources
- Share to Twitter Twitter
- Share to Facebook Facebook
- Share to LinkedIn LinkedIn
- Share to Reddit Reddit
- Share to Hacker News Hacker News
- Email Email
Related Tidbits
Better nan check with es6 number.isnan, vue named slot shorthand, padend string method in javascript, creating objects with es6 dynamic keys, fresh tidbits.
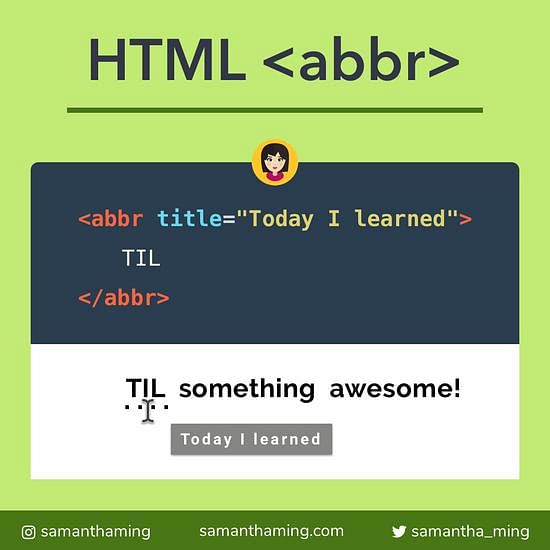
HTML abbr Tag
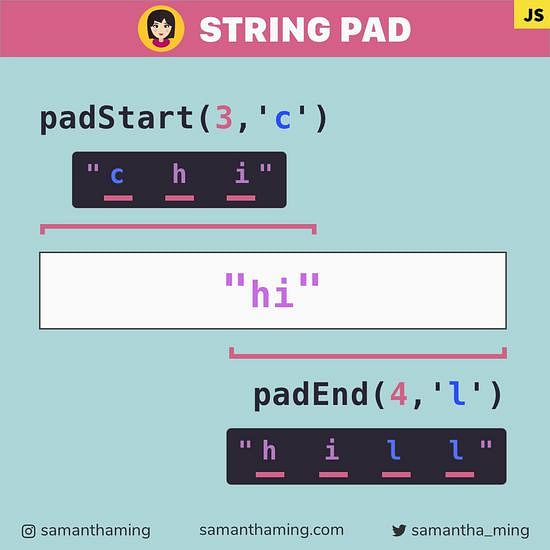
How to Pad a String with padStart and padEnd in JavaScript
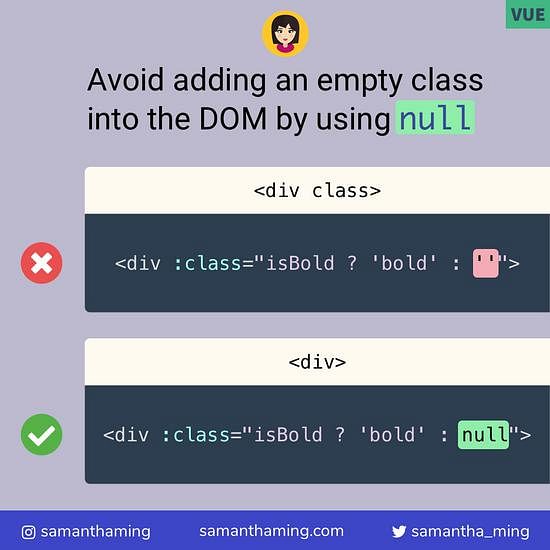
Avoid Empty Class in Vue with Null
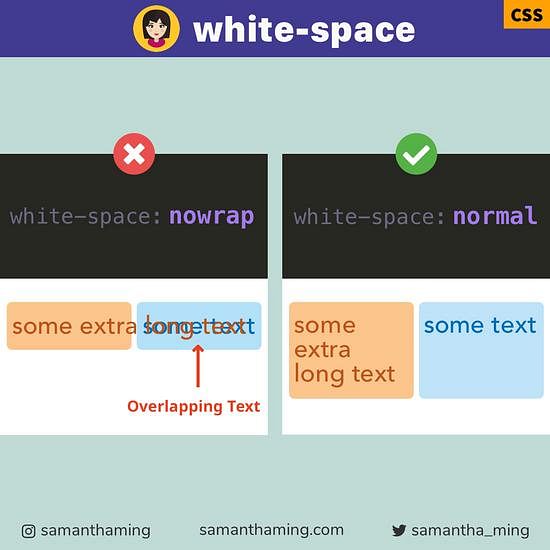
Fix Text Overlap with CSS white-space
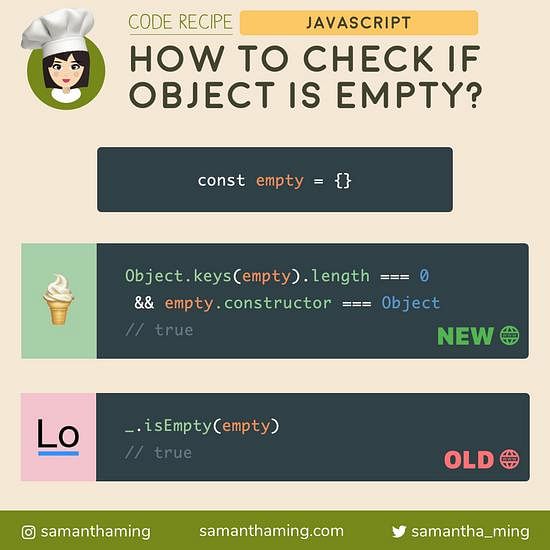
How to Check if Object is Empty in JavaScript
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- Interview Preparation For Software Developers
- Must Coding Questions - Company-wise
- Must Do Coding Questions - Topic-wise
- Company-wise Practice Problems
- Company Preparation
- Competitive Programming
- Software Design-Patterns
- Company-wise Interview Experience
- Experienced - Interview Experiences
- Internship - Interview Experiences
- Problem of the Day
- Topic-wise Practice
- Difficulty Level - School
- Difficulty Level - Basic
- Difficulty Level - Easy
- Difficulty Level - Medium
- Difficulty Level - Hard
- Leaderboard !!
- Explore More...
- Linked List
- Binary Tree
- Binary Search Tree
- Advance Data Structures
- All Data Structures
- Analysis of Algorithms
- Searching Algorithms
- Sorting Algorithms
- Pattern Searching
- Geometric Algorithms
- Mathematical Algorithms
- Randomized Algorithms
- Greedy Algorithms
- Dynamic Programming
- Divide & Conquer
- Backtracking
- Branch & Bound
- All Algorithms
- Tailwind CSS
- Web Browser
- File Formats
- Operating Systems
- Computer Network
- Computer Organization & Architecture
- Compiler Design
- Digital Elec. & Logic Design
- Software Engineering
- Engineering Mathematics
- Complete Data Science Course
- Data Science Tutorial
- Machine Learning Tutorial
- Deep Learning Tutorial
- NLP Tutorial
- Machine Learning Projects
- Data Analysis Tutorial
- Python Tutorial
- Django Tutorial
- Pandas Tutorial
- Kivy Tutorial
- Tkinter Tutorial
- OpenCV Tutorial
- Selenium Tutorial
- GATE CS Notes
- Gate Corner
- Previous Year GATE Papers
- Last Minute Notes (LMNs)
- Important Topic For GATE CS
- GATE Course
- Previous Year Paper: CS exams
- Git Tutorial
- AWS Tutorial
- Docker Tutorial
- Kubernetes Tutorial
- Microsoft Azure Tutorial
- Python Quiz
- JavaScript Quiz
- Data Structures Quiz
- Algorithms Quiz
- Topic-wise MCQs
- CBSE Notes 2023-24
- CBSE Class 8 Notes
- CBSE Class 9 Notes
- CBSE Class 10 Notes
- CBSE Class 11 Notes
- CBSE Class 12 Notes
- School Programming
- English Grammar
- Accountancy
- Business Studies
- Human Resource Management
- Top 100 Puzzles
- Mathematical Riddles
Which of the following is a correct decomposition of 7/8
Which of the following is a correct decomposition of 7/8?
All of the above
This question is part of this quiz :
Please comment below if you find anything wrong in the above post Feeling lost in the world of random DSA topics, wasting time without progress? It's time for a change! Join our DSA course, where we'll guide you on an exciting journey to master DSA efficiently and on schedule. Ready to dive in? Explore our Free Demo Content and join our DSA course, trusted by over 100,000 geeks!
- DSA In JAVA/C++
- DSA in Python
- DSA in JavaScript

- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
destructuring assignment default value [duplicate]
I am learning javascript and I got kind of stuck with ES6 syntax while trying to give a default value to a variable when destructuring. Basically, I am trying to assign a variable giving the value of an object's property to it and if the value is false/null/undefined, I want it to be an empty object. For example,
👆This is what I want and 👇 is ES6 sugar syntax I thought as equivalent to above(it doesn't work tho..)
but somehow, sometimes it seems working in React, but the other times it doesn't.. is it compatibility problem? so confusing!
- ecmascript-6
- variable-assignment
- destructuring
2 Answers 2
You probably confused by the difference between null and undefined, For example:
Taken from: http://wesbos.com/destructuring-default-values/

No, it is not compatibility problem.
Default value will works if there's no value, meaning that it will work if it is undefined . From your example, you're assigning null to prop2 , and null is a defined value.
So it will work if your prop2 is not defined or assigned with undefined
- ahhhh I finally got the actual difference between null and undefined thanks alot! – Jay Jeong Commented Mar 12, 2018 at 17:13
- FWIW, undefined is a value too, but since it's also the value that you get when a property doesn't exist or an argument is not passed it was decided to have the default applied when undefined is the value. – Felix Kling Commented Mar 13, 2018 at 5:04
- Yes, you are right. I might have some problems to explain that in my answer above. Thank you Felix. – Dimitrij Agal Commented Mar 14, 2018 at 4:23
- What if foo is undefined? – worldwildwebdev Commented Mar 21, 2020 at 17:55
- It will return Uncaught ReferenceError: foo is not defined – Dimitrij Agal Commented Mar 23, 2020 at 2:43
Not the answer you're looking for? Browse other questions tagged javascript reactjs ecmascript-6 variable-assignment destructuring or ask your own question .
- Featured on Meta
- Upcoming sign-up experiments related to tags
- Policy: Generative AI (e.g., ChatGPT) is banned
- The [lib] tag is being burninated
- What makes a homepage useful for logged-in users
Hot Network Questions
- Why is my GFCI tripping when i turn the breaker on?
- What could explain that small planes near an airport are perceived as harassing homeowners?
- Viewport Shader Render different from 1 computer to another
- Intersection in toric variety
- Are Dementors found all over the world, or do they only reside in or near Britain?
- \newrefsegment prints title of bib file
- Logical AND (&&) does not short-circuit correctly in #if
- Google Search Console reports "Page with redirect" as "errors", are they?
- Does this double well potential contradict the fact that there is no degeneracy for one-dimensional bound states?
- Are there examples of triple entendres in English?
- D&D 3.5 Shadow Magic / Shadow Conjuration: Can an illusionist create a quasi-real shadow of ANY monster? Or only those on the summon monster lists?
- Is Légal’s reported “psychological trick” considered fair play or unacceptable conduct under FIDE rules?
- Does free multiplicative convolution become free additive convolution under logarithm?
- How is Victor Timely a variant of He Who Remains in the 19th century?
- Cloud masking ECOSTRESS LST data
- How to make D&D easier for kids?
- Why can't LaTeX (seem to?) Support Arbitrary Text Sizes?
- What is a positive coinductive type and why are they so bad?
- Do Christians believe that Jews and Muslims go to hell?
- Why was the animal "Wolf" used in the title "The Wolf of Wall Street (2013)"?
- Do capacitor packages make a difference in MLCCs?
- Is it better to show fake sympathy to maintain a good atmosphere?
- Examples of distribution for which first-order condition is not enough for MLE
- How to engagingly introduce a ton of history that happens in, subjectively, a moment?
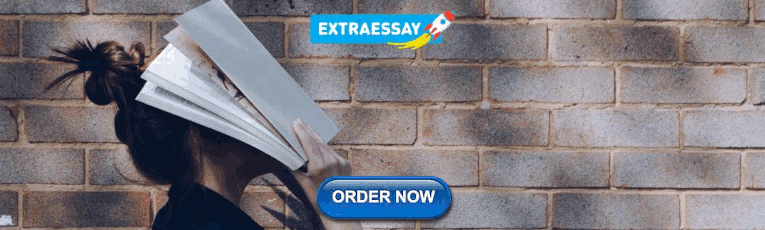
IMAGES
VIDEO
COMMENTS
Unpacking values from a regular expression match. When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to unpack the parts out of this array easily, ignoring the full match if ...
It's called "destructuring assignment," because it "destructurizes" by copying items into variables. However, the array itself is not modified. It's just a shorter way to write: // let [firstName, surname] = arr; let firstName = arr [0]; let surname = arr [1]; Ignore elements using commas.
The destructuring assignment syntax unpack object properties into variables: let {firstName, lastName} = person; It can also unpack arrays and any other iterables: let [firstName, lastName] = person;
3. It is something like what you have can be extracted with the same variable name. The destructuring assignment is a JavaScript expression that makes it possible to unpack values from arrays or properties from objects into distinct variables. Let's get the month values from an array using destructuring assignment.
Destructuring assignment is a powerful feature in JavaScript introduced with ES6 (ECMAScript 2015). It allows you to unpack values from arrays or properties from objects into distinct variables in a more concise and readable manner. This guide will help you understand how to effectively use destructuring assignment in your JavaScript code, complete with examples and…
JavaScript Destructuring. The destructuring assignment introduced in ES6 makes it easy to assign array values and object properties to distinct variables. For example, Before ES6: // assigning object attributes to variables const person = {. name: 'Sara', age: 25, gender: 'female'. }
The destructuring assignment is a cool feature that came along with ES6. Destructuring is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables. That is, we can extract data from arrays and objects and assign them to variables. Why
The destructuring syntax makes it easy to extract properties in lower levels or embedded objects as well. Let's extract the street property of the address embedded object in userDetails. const { firstName, address: { street } } = userDetails; As we saw before, the firstName property, which is a top-level property, is extracted.
Destructuring Assignment is a JavaScript expression that allows to unpack values from arrays, or properties from objects, into distinct variables data can be extracted from arrays, objects, nested objects and assigning to variables. In Destructuring Assignment on the left-hand side defined that which value should be unpacked from the sourced ...
by Kevwe Ochuko. Destructuring in JavaScript is a simplified method of extracting multiple properties from an array by taking the structure and deconstructing it down into its own constituent parts through assignments by using a syntax that looks similar to array literals.. It creates a pattern that describes the kind of value you are expecting and makes the assignment.
Destructing assignment with an array. In this case, a is a new constant which will receive the index 0 of the array [1, 2, 3] (Which has the value 1).The constant declaration's position in the ...
Open the demo. Looking at const { realName: secretName } = hero, the destructuring defines a new variable secretName (alias variable) and assigns to it the value of hero.realName.. 6. Extracting properties from nested objects. In the previous examples, the objects were plain: the properties have primitive data types (e.g. strings).
What is an Object in JavaScript? In JavaScript, an object is a collection of properties, and a property is an association between a name (or key) and a value. Writing an object in JavaScript looks somehow similar to an array, but instead we use curly braces or moustaches to create them.
Introduction to the JavaScript object destructuring assignment. Suppose you have a person object with two properties: firstName and lastName. let person = {. firstName: 'John' , lastName: 'Doe'. }; Code language: JavaScript (javascript) Before ES6, when you want to assign properties of the person object to variables, you typically do it like this:
Here are the steps to it. First we destructure properties of the object. const {a, b, c} = obj; Next, we assign variables to these properties. const {a: A, b: B, c: C} = obj; After that, assign the default value as how we did in the previous example. const {a: A="foo", b: B="bar", c: C="baz"} = obj; And there you have it.
When destructuring arrays, the order that variables are declared is important. If we only want the car and suv we can simply leave out the truck but keep the comma: const vehicles = ['mustang', 'f-150', 'expedition']; const [car,, suv] = vehicles; Destructuring comes in handy when a function returns an array:
Skipping Values In JavaScript Destructuring. You can use blanks to skip over unwanted values 🤓. This way you can avoid useless variable assignments for values you don't want during destructuring 👍. You can also prefix "_" followed by the variable name you're disregarding. This helps communicates to your team member that it's a useless ...
Is it possible to rename a variable when destructuring a nested object in JavaScript? Consider the following code: const obj = {a: 2, b: {c: 3}}; const {a: A, b:{c}} = obj; How can I rename c in above code like I renamed a to A?
Which of the following is a correct decomposition of 7/8? A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
Of course this can become difficult to read the more properties you have. You can also just assign them directly, the good old fashioned way: point.x = otherPoint.x; point.y = otherPoint.y; Or with a loop: for (const prop of ['x','y']) { point[prop] = otherPoint[prop]; } If you want to create a new object from an existing object
8. No, it is not compatibility problem. Default value will works if there's no value, meaning that it will work if it is undefined. From your example, you're assigning null to prop2, and null is a defined value. So it will work if your prop2 is not defined or assigned with undefined. let foo = {.