TEAM LICENSES: Save money and learn new skills through a Hacking with Swift+ team license >>
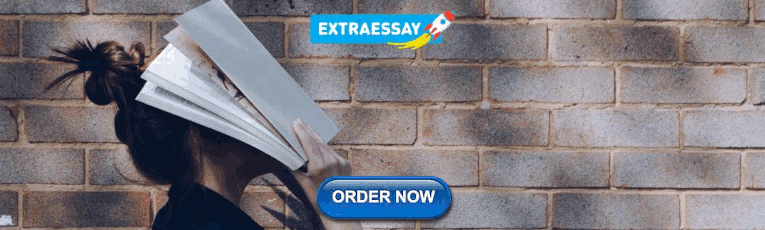
SOLVED: presentationMode.wrappedValue.dismiss() BREAKS when underlying view is updated
Forums > SwiftUI
| I have ViewA which presents ViewB via a .sheet modifier in ViewA. ViewB uses @Environment(.presentationMode) var presentationmode and presentationMode.wrappedValue.dismiss() to dismiss itself. This works most of the time. However, if the underlying ViewA is updated while ViewB is presented (e.g. the device is rotated causing ViewA to update), ViewB's presentationMode.wrappedValue.dismiss() no longer works. I am using the examples provided by Paul, but cannot find a solution to this problem. Am I doing something wrong? Thanks G |
| However, just for anyone experiencing this problem, I found that you should also take care not to update the underlying view (e.g. ViewA) via any mechanism (e.g. timer, etc.) while ViewB is presented. This will cause the presentationMode in ViewB to "detach" from ViewA, thus breaking the dismiss functionality. If anyone else can explain this better, or has a work around, please comment. Thanks G |
SPONSORED Superwall lets you build & test paywalls without shipping updates. Run experiments, offer sales, segment users, update locked features and more at the click of button. Best part? It's FREE for up to 250 conversions / mo and the Superwall team builds out 100% custom paywalls – free of charge.
Sponsor Hacking with Swift and reach the world's largest Swift community!
Archived topic
This topic has been closed due to inactivity, so you can't reply. Please create a new topic if you need to.
All interactions here are governed by our code of conduct .

You are not logged in
Link copied to your pasteboard.

Articles, podcasts and news about Swift development, by John Sundell .
Dismissing a SwiftUI modal or detail view
When building iOS and Mac apps, it’s very common to want to present certain views either modally, or by pushing them onto the current navigation stack. For example, here we’re presenting a MessageDetailView as a modal, using SwiftUI’s built-in sheet modifier in combination with a local @State property that keeps track of whether the detail view is currently being presented:
To learn more about @State and the rest of SwiftUI’s state management system, check out this guide .
But now the question is — how do we dismiss that MessageDetailsView once it’s been presented? One way to do that would be to inject our above isShowingDetails property into our MessageDetailsView as a binding, which the detail view could then set to false to dismiss itself:
While the above pattern certainly works, it requires us to manually implement that binding connection every time that we want to enable our users to dismiss a modal. So, there has to be a more convenient way, right?
The good news is that there is , and that’s to use the presentationMode environment value, which gives us access to an object that can be used to dismiss any view, regardless of how it’s being presented:
With the above in place, we no longer have to manually inject our isShowingDetails property as a binding — SwiftUI will automatically set that property to false whenever our sheet gets dismissed. As an added bonus, the above pattern even works if we were to present our MessageDetailsView by pushing it onto the navigation stack, rather than displaying it as a sheet. In that situation, our view would get “popped” from the navigation stack when the presentation mode’s dismiss method is called. Neat!
However, there’s one thing that’s somewhat awkward about the above implementation, and that’s that we have to access our environment value’s wrappedValue in order to be able to call its dismiss method (because it’s actually a Binding , not just a raw value). So, to address that, Apple is introducing a new version of the above API in iOS 15 (and the rest of their 2021 operating systems) which is simply called dismiss . That new API gives us an action that can be invoked directly — like this:
Now, if you’ve been reading Swift by Sundell for a while, then you might think that the next thing that I will point out is that the above dismiss closure can be directly passed to our Button as its action , but that’s actually not the case. It turns out that dismiss is not a closure, but rather a struct that uses Swift’s relatively new call as function feature.
So, if we wanted to inject the dismiss action directly into our button, then we’d have to pass a reference to its callAsFunction method — like this:
Of course, there’s nothing wrong with wrapping our call to dismiss within a closure (in fact, I prefer doing that in this kind of situation), but I just thought I’d point it out, since it’s interesting to see SwiftUI adopt call as function for the above API and others like it.
So that’s three different ways to dismiss a SwiftUI modal or detail view — two of which that are backward compatible with iOS 14 and earlier, and a modern version that should ideally be used within apps that are targeting iOS 15 (or its sibling operating systems).
I hope that you found this article useful, and if you have any questions, comments, or feedback, then feel free to reach out via either Twitter or email .
Thanks for reading!
- Share this article on Twitter
More on similar topics
90: “the essence of app architecture” with special guest matt gallagher, building an observable type for swiftui views, implicit capturing of self in swift 5.3.
Dismissing SwiftUI Views
SwiftUI has a less clumsy mechanism for dismissing presented views in iOS 15.
Presentation Mode (iOS 13/14)
Before iOS 15, if you wanted to programmatically dismiss a presented view you used the presentationMode environment property. For example, here’s a view where I present a modal sheet from the button action (some details omitted):
Note: To show the view full screen, replace the .sheet modifier with .fullScreenCover :
SwiftUI shows the sheet when we set the state variable isShowingCart to true in the addToCart method:
This presents a modal sheet which you can dismiss by dragging down on the sheet. If you want to dismiss the sheet programmatically you get the presentation mode from the environment:
Then you call the dismiss() method on the wrapped value:
For example, if I have cancel and save buttons on my presented cart view:
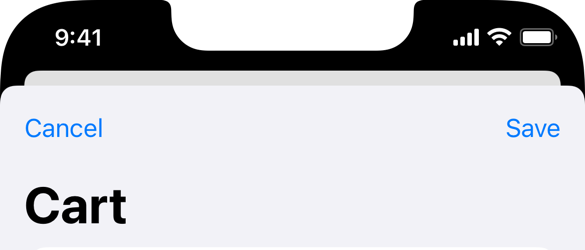
That always seemed a clumsy mechanism to me so I’m happy we have something better in iOS 15.
Dismiss Action (iOS 15)
In iOS 15, Apple deprecated the presentationMode environment property, replacing it with a dismiss action . You get the dismiss action from the environment of the presented view:
You then call the dismiss action directly with no need to mess with wrapped values:
For example, my cart view updated for iOS 15:
Note: Calling dismiss() on a view that’s not presented has no effect .
If you want to check if you’re presenting a view use the isPresented environment property in the view:
This replaces the property of the same name from the now deprecated presentationMode . For example, to do an action when a view is first presented:
Never Miss A Post
Sign up to get my iOS posts and news direct to your inbox and I'll also send you a PDF of my WWDC 2024 Viewing Guide
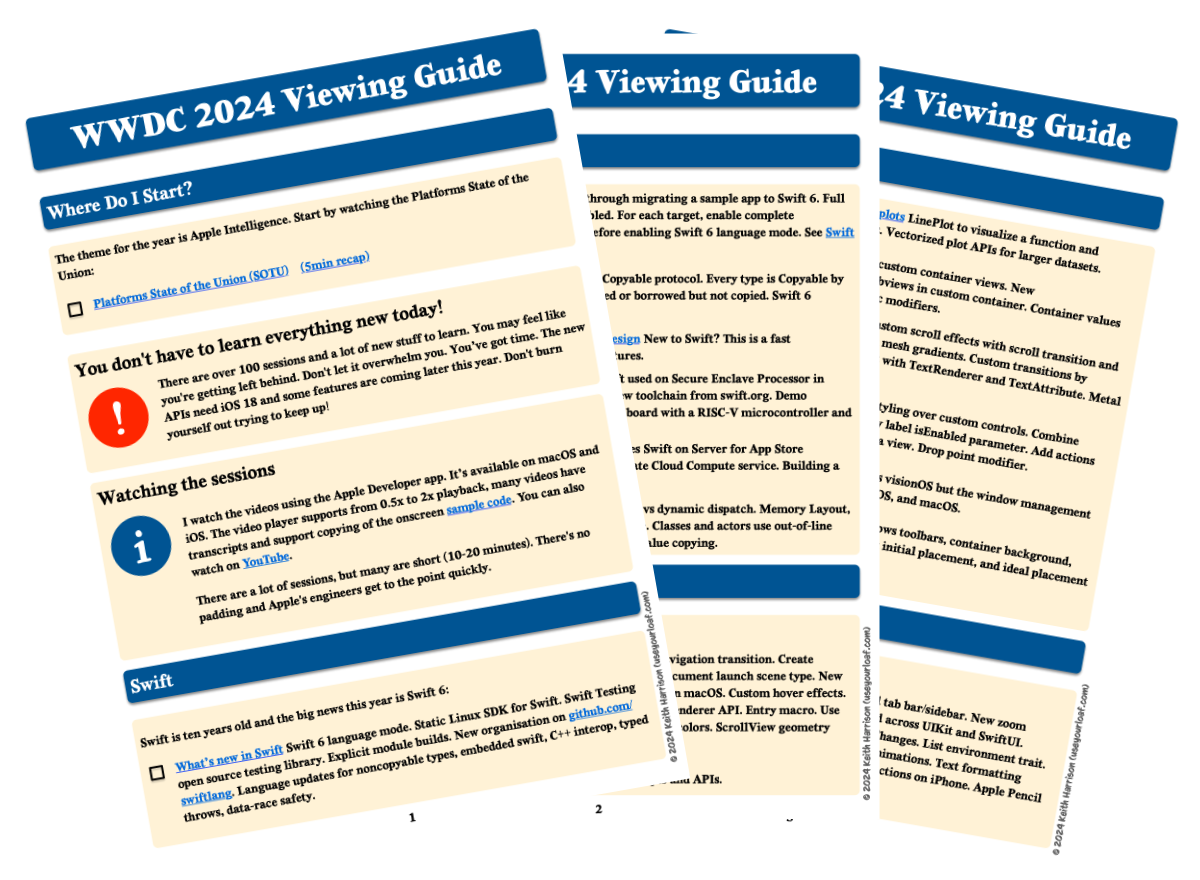
Unsubscribe at any time. See privacy policy .
Composable styling in SwiftUI
This week I want to talk about the styling of views in SwiftUI. SwiftUI provides a pretty composable architecture for building your apps. Every screen in terms of SwiftUI is a function on some data which returns a view. So let’s talk today about composable and highly reusable styling options which we have in SwiftUI.
Whenever I begin a project, I start with defining my brand color for my User Interface. I use brand color as a tint for my buttons, switches, slider, etc. We can easily set the tint color on every view in the app by using accentColor modifier on the root view. Here is a quick example.
SwiftUI uses Environment feature to pass the values implicitly inside any child view. This is how we can give an accent color to every view across the app. To learn more about Environment feature of SwiftUI, check my dedicated post “The power of Environment in SwiftUI” .
Another must-have option which I want to enable on every view in my app is line limit. I want to make every text in my app multi-lined in the case when it is too long. I also need it when a user enables extra large font size for Dynamic Type. It is also straightforward to achieve by adding lineLimit modifier to my root view.
Button styles
I often have a few button types which I reuse across the app. Before SwiftUI, I was using inheritance to apply my styling to UIButtons in UIKit . But SwiftUI relies on composition instead of inheritance, that’s why it provides us ButtonStyle protocol which we can implement to reuse our button styles across the app.
As you can see in the example above, we implement two button styles: OutlinedButton and FilledButton . To apply them to a button in SwiftUI, we have to use button style modifier. Let’s see how we can use them.
I want to note that you can use buttonStyle modifier on any view in SwiftUI and it utilizes Environment feature to share the style with any button inside it.
Text styles
Similar to buttons, I have a few different styling options for my text representation. Unfortunately, SwiftUI doesn’t provide something like TextStyle protocol. But instead, it gives us a much more powerful composition concept, and it is ViewModifier . Let’s take a look at how we can use ViewModifiers to style our Text views.
In the example above, we implement two style modifiers. We also provide an extension for Text component, which allows us easily apply any modifier to a Text . Now we can use our styles by adding textStyle modifier to any Text component.
ViewModifiers allow us to encapsulate and reuse any logic across the Views . To learn more about ViewModifiers , take a look at my dedicated post “ViewModifiers in SwiftUI” .
Today we learned how to create highly reusable styling components for SwiftUI by using features like ViewModifiers and Environment . I hope you enjoy the post. Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next week!
SwiftUI navigate to another view from a popup
when I click on a mapview pin, a PopupView comes up with some text and a button. I want to be able to navigate to another DetailsView by clicking on the button display in the popup, the button is embedded inside NavigationLink. But clicking on the button nothing happens. How to navigate from button click?
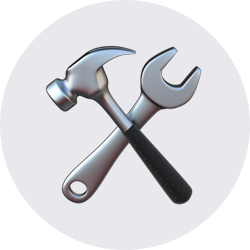
Change @State var pin in PinView to a @Binding var pin
The expression selected = 1 does nothing.
Changed the pin from state to Binding, did not work either
The problem is that the button is dead it is not accepting the tap. I put in debug break in isSelected = true statement. When I tap the button it never hits the breakpoint, it is as if the navigationlink button is inactive. How to make it active.
Dismiss a UIViewRepresentable
Any suggestions on how to dismiss a UIViewRepresentable view? Thank you
Hi @lewis-h , There seems to be some disconnect between your response and the original question.
@flmcl Do you still have issues with this?
Yes sorry I thought I had posted it. I managed with: self.presentation.wrappedValue.dismiss()
This topic was automatically closed after 166 days. New replies are no longer allowed.
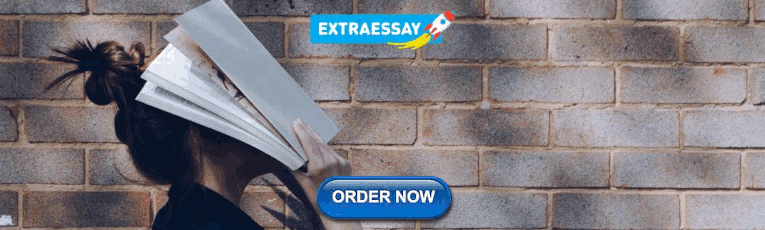
Assignment to @State var in init() doesn't do anything, but the compiler gen'ed one works
The following example summarize what I learned from this long thread. Hopefully this provide quick answer to anyone looking at this in the future. Please read @DevAndArtist 's and @Lantua 's comment in this thread for authoritative explanations.
========================== Original post
This is my simplified example: I need to assign something to @State var value on init. Calling the compiler gen'ed one works, but my hand written init() doesn't:
I need to assign to value in the init() from another Binding parameter. The code shown above is simplified.
Maybe you can call the synthesized init as a work around.

Also, it should be this:
since _value is the actual storage, and is of type State<Int> . That's why your commented code wouldn't even compile.

Now I know what the synth'ed init does to the @State var
Personally, I suggest to not touch a State in your init , even if by recreating the wrapper. If you need an initial value injected, I would go for a Binding instead
I agree with @DeFrenZ . There's still certain amount of magic, like how it can distinguish between multiple State s during init (I suspect it's the init order, but I wouldn't want to rely on that).
After thinking for a while, I believe the best way is to just call the synthesized init, and not even mutate the state during this time.
If you don't know the initial value, you can just leave it to the parents to supply it:
I want to allow cancel for my editing view. So instead of updating the Binding directly which would commit the change immediately, it uses an @State var draft that's initialized from the passed in binding:
Is there better way for this so I an avoid assigning to @State in my init ?
what do you mean?
If you have multiple State in a view, SwiftUI somehow can distinguish between them, which is quite interesting when you think about it. There is definitely some underlying convention there.
Remember that this can be called multiple times, one for each view update. Somehow SwiftUI can still know that State(...) refers to _a .
I'm most certain (from my own experiment, I couldn't find the doc anywhere) that you're not suppose to mutate during View init since that'd be in the body call of the parent view.
In any case, I believe the idiomatic way would be to use View.onAppear(perform:)

I still don't understand what you mean by:
The way I understand @State property wrapper thing is just like C macro, the compiler expand the var to a whole bunch of thing. Much to learn..
You're correct, more or less, but here's the thing, when SwiftUI calls body twice:
You call FooView.init twice, which means you have two FooView s, but somehow SwiftUI is able to figure out that FooView.state s from different FooView s refer to the same State , maintaining the illusion of persistence.
Since it's very common to use the compiler synthesized init to initialize view's @State, very likely mutate state in init is allowed.
I think SwiftUI do "init phase" to generate the view body data structure, separate from "diff'ing/run/update" on this data structure.If so, then it's okay to mutate inside init's.
Wish Apple can just tell us a little...
Note that, in the synthesized init, it looks like this:
as per the property wrapper proposal . While the warning refers to the mutation of the wrapped value like this:
I'm a little surprise, as you've noted, that it doesn't set warning on
which would be warned inside body . But the result is very much unexpected still.
If @State mutate in view init is not allowed, I think SwiftUI would surely give out warning like it does inside body. But this is just my guess.
The issue is that this validity is a framework (SwiftUI) issue, not the language, so not sure that can be even checked by the compiler
Not the compiler, SwiftUI does the runtime check: it give out runtime warning if you modify state inside body.
I skipped most of the conversation. If you want to be on the safe side, don't try overriding State 's initial value during init . It will only work once during very first view creation (important: not value initialization) and only if that view in the view tree gets re-created / replaced with a different view of the same type but internally different id.
If you really need that functionally here is what you could copy paste into your codebase: swiftui_helper_property_wrapper.swift · GitHub

Oh and as a side note there is this artificial issue: [SR-12202] Property wrappers initialization is somehow artificially restricted for `State` · Issue #54627 · apple/swift · GitHub
Basically you cannot rely on the property = value syntax in the init if the PW has no mutable setter on its wrappedValue property. I understand why this is how it is right now, but theoretically the compiler should be able to diagnose this correctly and allow default initialization right through the wrapped property as a shorthand syntax (& kinda like late / out of line initialization) from within an init . That's why I consider this as a bug.
Related discussion: Why does this property wrapper code compile?
Get the Reddit app
For SwiftUI discussion, questions and showcasing SwiftUI is a UI development framework by Apple that lets you declare interfaces in an intuitive manner. Please keep content related to SwiftUI only. For Swift programming related content, visit r/Swift. For iOS programming related content, visit r/iOSProgramming
In Xcode 12 Beta 6 dismissing sheet no longer works?
In Xcode 12 Beta 6, programmatically dismissing a sheet no longer seems to work.
My current approach is to use
self.presentation.wrappedValue.dismiss()
But this seems to have stopped working. So I changed it to passing a Binding of the isSheetPresented bool to the view inside of the sheet and toggle that instead. That doesn't seem to be working either.
Is anyone else experiencing this as well or is it something faulty with my code?
EDIT: figured it out, my problem was that .sheet was attached to the button inside ToolbarItem. This should not be the case. .sheet doesn’t have to be attached to the button that triggers it, and can be attached higher up (I put it on the direct child of NavigationView).
By continuing, you agree to our User Agreement and acknowledge that you understand the Privacy Policy .
Enter the 6-digit code from your authenticator app
You’ve set up two-factor authentication for this account.
Enter a 6-digit backup code
Create your username and password.
Reddit is anonymous, so your username is what you’ll go by here. Choose wisely—because once you get a name, you can’t change it.
Reset your password
Enter your email address or username and we’ll send you a link to reset your password
Check your inbox
An email with a link to reset your password was sent to the email address associated with your account
Choose a Reddit account to continue
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
presentationMode.wrappedValue.dismiss() not working properly
I'm using the sheet method to display a simple form and I pass into it a couple of varsiables. The problem is that if I click the button which performs the .dismiss() method after changing the variables passed in it doesn't work. Instead if I directly click the button it works normally. Here's the code:
I also checked if the isPresented variable changes value and it's actually toggled when i click the button but the sheet stays there.
Here's the code where I use the form:
show_modal_edit is a list of Bool, I checked the values and apparently the correct one is passed to the isPresented field of .sheet().
I've setup the following test version of your code and all is working well for me on ios 13.4 and macos catalyst after renaming Data to Datax.
This points to the function in editProduct()
as the possible source of your problem. Specifically, using Data as the name for your type. It seems to clash with the system struct Data type. Try renaming your ObservableObject class to something else (Datax in my test).
Hope this helps track down your issue.
- Thank you for the answer but unfortunately that didn't work for me. I doubt that the cause could be the editPorduct method since in a different file I call a similar method but the dismiss works. Maybe it has to do with the fact that my "EditProductForm" is inside a ForEach. I added the code where i call it in my question. – Guillaume Franzoni Darnois Commented Apr 27, 2020 at 10:19
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged swiftui or ask your own question .
- The Overflow Blog
- Navigating cities of code with Norris Numbers
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Feedback requested: How do you use tag hover descriptions for curating and do...
Hot Network Questions
- What's wrong with my app authentication scheme?
- Garage door not closing when sunlight is bright
- Simple JSON parser in lisp
- How did Jason Bourne know the garbage man isn't CIA?
- Existence of semi-group with local but not global identity
- Giant War-Marbles of Doom: What's the Biggest possible Spherical Vehicle we could Build?
- Can I use "Member, IEEE" as my affiliation for publishing papers?
- Stargate "instructional" videos
- Word to classify what powers a god is associated with?
- Difference between "backpedal" and "change your tune"
- Discrete cops and robbers
- Restarted my computer and the display resolution changed
- Where exactly was this picture taken?
- Sulphur smell in Hot water only
- MOSFETs keep shorting way below rated current
- Very old fantasy adventure movie where the princess is captured by evil, for evil, and turned evil
- Can I use the Chi-square statistic to evaluate theoretical PDFs against an empirical dataset of 60,000 values?
- Why do these finite group Dedekind matrices seem to have integer spectrum when specialized to the order of group elements?
- How to allow just one user to use SSH?
- Venus’ LIP period starts today, can we save the Venusians?
- Does a Way of the Astral Self Monk HAVE to do force damage with Arms of the Astral Self from 10' away, or can it be bludgeoning?
- 90/180 day rule with student resident permit, how strict it is applied
- An interesting example of Horizontal and Vertical Lines in TIKZ?
- Who became an oligarch after the collapse of the USSR
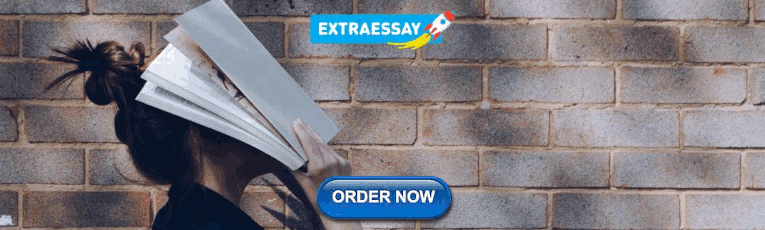
IMAGES
COMMENTS
You can use presentationMode environment variable in your modal view and calling self.presentaionMode.wrappedValue.dismiss() ... (action: { self.presentation.wrappedValue.dismiss() }) { Text("Dismiss") } Share. Follow edited Sep 6, 2019 at 9:10. answered Sep 6, 2019 at 8:54. Tomm P Tomm P. 826 1 1 gold badge 10 10 silver badges 19 19 bronze ...
Yes, just write the self.presentation.wrappedValue.dismiss() where you want the app to dismiss the view. - Prashant Gaikwad. Commented Sep 6, 2022 at 10:22. Add a comment | 23 There is now a way to programmatically pop in a NavigationView, if you would like. This is in beta 5. Notice that you don't need the back button.
The first option is to tell the view to dismiss itself using its presentation mode environment key. Any view can dismiss itself, regardless of how it was presented, using @Environment(\.dismiss), and calling that property as a function will cause the view to be dismissed. For example, we could create a detail view that is able to dismiss itself ...
ViewB uses @Environment(.presentationMode) var presentationmode and presentationMode.wrappedValue.dismiss() to dismiss itself. This works most of the time. However, if the underlying ViewA is updated while ViewB is presented (e.g. the device is rotated causing ViewA to update), ViewB's presentationMode.wrappedValue.dismiss() no longer works.
In that situation, our view would get "popped" from the navigation stack when the presentation mode's dismiss method is called. Neat! Neat! However, there's one thing that's somewhat awkward about the above implementation, and that's that we have to access our environment value's wrappedValue in order to be able to call its ...
iOS 15. SwiftUI has a less clumsy mechanism for dismissing presented views in iOS 15. Presentation Mode (iOS 13/14) Before iOS 15, if you wanted to programmatically dismiss a presented view you used the presentationModeenvironment property. For example, here's a view where I present a modal sheet from the button action (some details omitted):
A binding to the current presentation mode of the view associated with this environment. iOS 13.0-18.0 Deprecated iPadOS 13.0-18.0 Deprecated Mac Catalyst 13.0+ macOS 10.15-15. Deprecated tvOS 13.0-18.0 Deprecated visionOS 1.0-2.0 Deprecated watchOS 6.0-11.0 Deprecated. var presentationMode: Binding<PresentationMode> { get }
In the above code, the key is self.presentationMode.wrappedValue.dismiss(). Code Explanation: This environment object will help you dismiss the view; Here, you can see that we have a "Cancel" button in a HStack with a spacer so it is aligned on the left. Here is where the actual magic happens.
the result of this is that the presentation mode dismiss will only works for the first view B the cancel button, it will no close the view if you click the button inside the view ... { shouldSave.toogle() self.presentationMode.wrappedValue.dismiss() } }.onDisappear { guard shouldSave else { return } author.name = authorName if isValidAuthor ...
Interesting, that seems like a pretty good solution! There's one other possibility, but it uses iOS 14 so won't be viable for awhile. But, just for posterity, you could store a value in your state that determines when you want to dismiss (such as isPresented), and then use the new onChange(of:perform:) API to listen for when it flips to false, and when it does call presentation.dismiss().
PresentationMode. An indication whether a view is currently presented by another view. iOS 13.0-18.0 Deprecated iPadOS 13.0-18.0 Deprecated Mac Catalyst 13.0+ macOS 10.15-15. Deprecated tvOS 13.0-18.0 Deprecated visionOS 1.0-2.0 Deprecated watchOS 6.0-11.0 Deprecated. struct PresentationMode.
Expand user menu Open settings menu. Log In / Sign Up
First, only ` dismiss` should be enough. You should check the code that presents the warning view. Otherwise, one could assume the both statements are toggling the presentation of the view(one closes and the other opens it again). Try using only dissmis and checking the code that presents the view.
Every screen in terms of SwiftUI is a function on some data which returns a view. So let's talk today about composable and highly reusable styling options which we have in SwiftUI. Enhancing the Xcode Simulators. Compare designs, show rulers, add a grid, quick actions for recent builds. Create recordings with touches & audio, trim and export ...
SwiftUI navigate to another view from a popup. when I click on a mapview pin, a PopupView comes up with some text and a button. I want to be able to navigate to another DetailsView by clicking on the button display in the popup, the button is embedded inside NavigationLink. But clicking on the button nothing happens.
Any suggestions on how to dismiss a UIViewRepresentable view? Thank you. jayantvarma June 12, 2020, 12:12am 3. Hi @lewis-h, There seems to be some disconnect between your response and the original question. ... self.presentation.wrappedValue.dismiss() Thank you. system Closed November 19, 2020, 5:35am 6. This topic was automatically closed ...
The following example summarize what I learned from this long thread. Hopefully this provide quick answer to anyone looking at this in the future. Please read @DevAndArtist's and @Lantua's comment in this thread for authoritative explanations. // How to do View @State var initialization and what problem can arise from doing so... import SwiftUI struct Child: View { // How to hand code a init ...
This has been deprecated on iOS 15, and a new environment variable, dismiss, is introduced: @Environment(\.dismiss) var dismiss. which we can call directly using dismiss() to achieve the same. I know we can do the following to call the appropriate dismiss function and support all versions: if #available(iOS 15, *) {. self.dismiss()
In Xcode 12 Beta 6, programmatically dismissing a sheet no longer seems to work. My current approach is to use…
2. I'm using the sheet method to display a simple form and I pass into it a couple of varsiables. The problem is that if I click the button which performs the .dismiss () method after changing the variables passed in it doesn't work. Instead if I directly click the button it works normally. Here's the code: struct EditProductForm: View {.