Ok so each person can be assigned to one job, and the idea is to assign each job to one of the person so that all the jobs are done in the quickest way.
Here is my code so far:
I know i might be out of track. I didn't use lower bound in the beginning, i'm actually a little confused how this algorithm exactly works. So even step by step walktrough through the algorithm would be helpful. | ,
Posting Permissions post new threads post replies post attachments edit your posts is are code is code is
Learn some of the best reasons why you should seriously consider bringing your Android mobile development expertise to bear on the Windows 8 platform. Do you have an Android application? How hard would it really be to port to Windows 8? If you've already built for Android, learn what do you really need to know to port your application to Windows Phone 8. Our portal for articles, videos, and news on HTML5, CSS3, and JavaScript See the Windows 8.x apps we've spotlighted or submit your own app to the gallery!
#### end of commenting out RSS feed. --> | 
Advertiser Disclosure: Some of the products that appear on this site are from companies from which TechnologyAdvice receives compensation. This compensation may impact how and where products appear on this site including, for example, the order in which they appear. TechnologyAdvice does not include all companies or all types of products available in the marketplace.
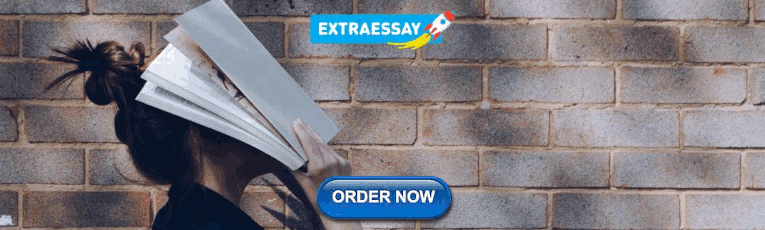
IMAGES
COMMENTS
Solution 1: Brute Force. We generate n! possible job assignments and for each such assignment, we compute its total cost and return the less expensive assignment. Since the solution is a permutation of the n jobs, its complexity is O (n!). Solution 2: Hungarian Algorithm. The optimal assignment can be found using the Hungarian algorithm.
Explanation: The optimal assignment is to assign job 2 to the 1st worker, job 3 to the 2nd worker and job 1 to the 3rd worker. Hence, the optimal cost is 4000 + 3500 + 2000 = 9500. Different approaches to solve this problem are discussed in this article. Approach: The idea is to use the Hungarian Algorithm to solve this problem. The algorithm ...
In a standard version of a job assignment problem, there can be jobs and workers. To keep it simple, we're taking jobs and workers in our example: Job 1 Job 2 Job 3; A: 9: 3: 4: B: 7: 8: 4: C: 10: 5: 2: We can assign any of the available jobs to any worker with the condition that if a job is assigned to a worker, the other workers can't ...
This guide is essential for operations researchers, algorithm designers, and computer science students who are keen to understand and implement optimization algorithms in real-world scenarios. In this tutorial, you'll learn: The fundamentals of the job assignment problem and its significance in operations research and resource management. An ...
Job Assignment problem is one of the popular problem to be solved using branch-and-bound technique. Branch-and-bound technique is applied as to optimize the ...
Converting this problem to a formal mathematical definition we can form the following equations: - cost matrix, where cij - cost of worker i to perform job j. - resulting binary matrix, where xij = 1 if and only if ith worker is assigned to jth job. - one worker to one job assignment. - one job to one worker assignment. - total cost ...
Let there be N workers and N jobs. Any worker can be assigned to perform any job, incurring some cost that may vary depending on the work-job assignment. It is required to perform all jobs by assigning exactly one worker to each job and exactly one job to each agent in such a way that the total cost of the assignment is minimized. - Audorion/Job-Assignment-Problem-Branch-And-Bound
Solution 1: Brute Force. We generate n! possible job assignments and for each such assignment, we compute its total cost and return the less expensive assignment. Since the solution is a permutation of the n jobs, its complexity is O (n!). Solution 2: Hungarian Algorithm. The optimal assignment can be found using the Hungarian algorithm.
Match jobs to machines.! Match personnel to tasks.! Match PU students to writing seminars. Non-obvious applications.! Vehicle routing.! ... Equivalent Assignment Problem c(x, y) 00312 01015 43330 00110 12204 cp(x, y) 3891510 41071614 913111910 813122013 175119 8 13 11 19 13 5 4 3 0 8 9 + 8 - 13 10
1. Solution: The cost function for node x in state space tree for job sequencing problem is defined as, Misplaced &. Where, m = max {i | i ∈ S x } S x is the subset of jobs selected at node x. Upper bound u (x) for node x is defined as, u(x) = suminotinSxPi. Computation of cost and bound for each node using variable tuple size is shown in the ...
Problem 4. Job shop needs to assign 4 jobs to 4 workers. The cost of performing a job is a function of the skills of the workers. Table summarizes the cost of the assignments. Worker1 cannot do job3, and worker 3 cannot do job 4. Determine the optimal assignment using the Hungarian method. Job.
Job Assignment Problem using Branch And Bound. Let there be N workers and N jobs. Any worker can be assigned to perform any job, incurring some cost that may vary depending on the work-job assignment. It is required to perform all jobs by assigning exactly one worker to each job and exactly one job to each agent in such a way that the total ...
The assignment problem is defined as: There are n people who need to be assigned to n jobs, one person per job. The cost that would accrue if the ith person is assigned to the jth job is a known quantity C[i,j] for each pair i, j = 1, 2, ..., n. The problem is to find an assignment with the minimum total cost.
Your goal is to devise an optimal assignment such that the maximum working time of any worker is minimized. Return the minimum possible maximum working time of any assignment. Example 1: Input: jobs = [3,2,3], k = 3 Output: 3 Explanation: By assigning each person one job, the maximum time is 3. Example 2: Input: jobs = [1,2,4,7,8], k = 2 Output ...
The formal definition of the assignment problem (or linear assignment problem) is . Given two sets, A and T, together with a weight function C : A × T → R.Find a bijection f : A → T such that the cost function: (, ())is minimized. Usually the weight function is viewed as a square real-valued matrix C, so that the cost function is written down as: , The problem is "linear" because the cost ...
and each job as a \demand": Each machine as having a supply of 1. Each job has a demand of 1. Each machine, job pair has a cost. This gives us the transportation tableau: J 1 J 2 J 3 J 4 M 1 14 5 8 7 1 M 2 2 12 6 5 1 M 3 7 8 3 9 1 M 4 2 4 6 10 1 Demand 1 1 1 1 4 From this, we could solve it as a transportation problem or as a linear program ...
The decision problem asks whether an assignment assigns at least k jobs, where k is a parameter to the decision problem. Clearly the problem is in NP; one can verify in polynomial time that an assignment satisfies that no machine is overloaded and each job is assigned to one machine. One can do this by checking the jobs assigned to machine i ...
Job Assignment Problem using Branch And Bound. Let there be N workers and N jobs. Any worker can be assigned to perform any job, incurring some cost that may vary depending on the work-job assignment. It is required to perform all jobs by assigning exactly one worker to each job and exactly one job to each agent in such a way that the total ...
Illustration on the Job Assignment Problem Input: n jobs, n employees, and an n x n matrix A where A ij be the cost if person i performs job j. Problem: find a one-to-one matching of the n employees to the n jobs so that the total cost is minimized.
The complexity of this solution of the assignment problem depends on the algorithm by which the search for the maximum flow of the minimum cost is performed. The complexity will be $\mathcal{O}(N^3)$ using Dijkstra or $\mathcal{O}(N^4)$ using Bellman-Ford .
Then X∗ solves the assignment problem specified by C since z(X∗)=0and z(X) ≥ 0 for any other solution X.ByExample5,X∗ is also an optimal solution to the assignment problem specified by C.NotethatX∗ corresponds to the permutation 132. The method used to obtain an optimal solution to the assignment problem specified by C
Data Structure. Java. Python. HTML. Interview Preparation. Menu. Back to Explore Page. You are the head of a firm and you have to assign jobs to people. You have N persons working under you and you have N jobs that are to be done by these persons. Each person has to do exactly one job and each job has to be done by exactly one person.
First of all assignment problem example: 1285.png. Ok so each person can be assigned to one job, and the idea is to assign each job to one of the person so that all the jobs are done in the quickest way. Here is my code so far: Code: #include "Matrix.h". // Program to solve Job Assignment problem. // using Branch and Bound.