Javarevisited
Learn Java, Programming, Spring, Hibernate throw tutorials, examples, and interview questions
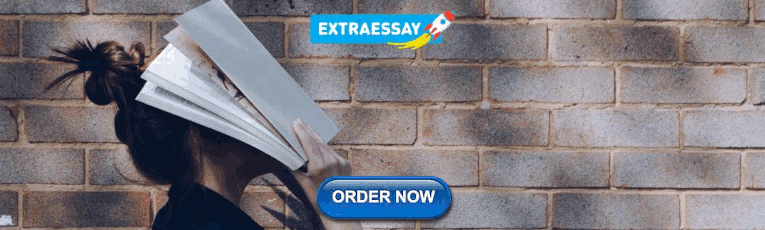
Topics and Categories
- collections
- multithreading
- design patterns
- interview questions
- data structure
- Java Certifications
- jsp-servlet
- online resources
- jvm-internals
Preparing for Java and Spring Boot Interview?
Join my Newsletter, its FREE
Tuesday, March 26, 2024
- Top 50 Java Programs from Coding Interviews

Interview Questions
Describe and compare fail-fast and fail-safe iterators. Give examples.
The main distinction between fail-fast and fail-safe iterators is whether or not the collection can be modified while it is being iterated. Fail-safe iterators allow this; fail-fast iterators do not.
Fail-fast iterators operate directly on the collection itself. During iteration, fail-fast iterators fail as soon as they realize that the collection has been modified (i.e., upon realizing that a member has been added, modified, or removed) and will throw a ConcurrentModificationException . Some examples include ArrayList , HashSet , and HashMap (most JDK1.4 collections are implemented to be fail-fast).
Fail-safe iterates operate on a cloned copy of the collection and therefore do not throw an exception if the collection is modified during iteration. Examples would include iterators returned by ConcurrentHashMap or CopyOnWriteArrayList .
ArrayList , LinkedList , and Vector are all implementations of the List interface. Which of them is most efficient for adding and removing elements from the list? Explain your answer, including any other alternatives you may be aware of.
Of the three, LinkedList is generally going to give you the best performance. Here’s why:
ArrayList and Vector each use an array to store the elements of the list. As a result, when an element is inserted into (or removed from) the middle of the list, the elements that follow must all be shifted accordingly. Vector is synchronized, so if a thread-safe implementation is not needed, it is recommended to use ArrayList rather than Vector.
LinkedList , on the other hand, is implemented using a doubly linked list. As a result, an inserting or removing an element only requires updating the links that immediately precede and follow the element being inserted or removed.
However, it is worth noting that if performance is that critical, it’s better to just use an array and manage it yourself, or use one of the high performance 3rd party packages such as Trove or HPPC .
Why would it be more secure to store sensitive data (such as a password, social security number, etc.) in a character array rather than in a String?
In Java, Strings are immutable and are stored in the String pool. What this means is that, once a String is created, it stays in the pool in memory until being garbage collected. Therefore, even after you’re done processing the string value (e.g., the password), it remains available in memory for an indeterminate period of time thereafter (again, until being garbage collected) which you have no real control over. Therefore, anyone having access to a memory dump can potentially extract the sensitive data and exploit it.
In contrast, if you use a mutable object like a character array, for example, to store the value, you can set it to blank once you are done with it with confidence that it will no longer be retained in memory.
Apply to Join Toptal's Development Network
and enjoy reliable, steady, remote Freelance Java Developer Jobs
What is the ThreadLocal class? How and why would you use it?
A single ThreadLocal instance can store different values for each thread independently. Each thread that accesses the get() or set() method of a ThreadLocal instance is accessing its own, independently initialized copy of the variable. ThreadLocal instances are typically private static fields in classes that wish to associate state with a thread (e.g., a user ID or transaction ID). The example below, from the ThreadLocal Javadoc , generates unique identifiers local to each thread. A thread’s id is assigned the first time it invokes ThreadId.get() and remains unchanged on subsequent calls.
Each thread holds an implicit reference to its copy of a thread-local variable as long as the thread is alive and the ThreadLocal instance is accessible; after a thread goes away, all of its copies of thread-local instances are subject to garbage collection (unless other references to these copies exist).
What is the volatile keyword? How and why would you use it?
In Java, each thread has its own stack, including its own copy of variables it can access. When the thread is created, it copies the value of all accessible variables into its own stack. The volatile keyword basically says to the JVM “Warning, this variable may be modified in another Thread”.
In all versions of Java, the volatile keyword guarantees global ordering on reads and writes to a variable. This implies that every thread accessing a volatile field will read the variable’s current value instead of (potentially) using a cached value.
In Java 5 or later, volatile reads and writes establish a happens-before relationship, much like acquiring and releasing a mutex.
Using volatile may be faster than a lock, but it will not work in some situations. The range of situations in which volatile is effective was expanded in Java 5; in particular, double-checked locking now works correctly.
The volatile keyword is also useful for 64-bit types like long and double since they are written in two operations. Without the volatile keyword you risk stale or invalid values.
One common example for using volatile is for a flag to terminate a thread. If you’ve started a thread, and you want to be able to safely interrupt it from a different thread, you can have the thread periodically check a flag (i.e., to stop it, set the flag to true ). By making the flag volatile, you can ensure that the thread that is checking its value will see that it has been set to true without even having to use a synchronized block. For example:
Compare the sleep() and wait() methods in Java, including when and why you would use one vs. the other.
sleep() is a blocking operation that keeps a hold on the monitor / lock of the shared object for the specified number of milliseconds.
wait() , on the other hand, simply pauses the thread until either (a) the specified number of milliseconds have elapsed or (b) it receives a desired notification from another thread (whichever is first), without keeping a hold on the monitor/lock of the shared object.
sleep() is most commonly used for polling, or to check for certain results, at a regular interval. wait() is generally used in multithreaded applications, in conjunction with notify() / notifyAll() , to achieve synchronization and avoid race conditions.
Tail recursion is functionally equivalent to iteration. Since Java does not yet support tail call optimization, describe how to transform a simple tail recursive function into a loop and why one is typically preferred over the other.
Here is an example of a typical recursive function, computing the arithmetic series 1, 2, 3…N. Notice how the addition is performed after the function call. For each recursive step, we add another frame to the stack.
Tail recursion occurs when the recursive call is in the tail position within its enclosing context - after the function calls itself, it performs no additional work. That is, once the base case is complete, the solution is apparent. For example:
Here you can see that a plays the role of the accumulator - instead of computing the sum on the way down the stack, we compute it on the way up, effectively making the return trip unnecessary, since it stores no additional state and performs no further computation. Once we hit the base case, the work is done - below is that same function, “unrolled”.
Many functional languages natively support tail call optimization, however the JVM does not. In order to implement recursive functions in Java, we need to be aware of this limitation to avoid StackOverflowError s. In Java, iteration is almost universally preferred to recursion.
How can you swap the values of two numeric variables without using any other variables?
You can swap two values a and b without using any other variables as follows:
How can you catch an exception thrown by another thread in Java?
This can be done using Thread.UncaughtExceptionHandler .
Here’s a simple example:
What is the Java Classloader? List and explain the purpose of the three types of class loaders.
The Java Classloader is the part of the Java runtime environment that loads classes on demand (lazy loading) into the JVM (Java Virtual Machine). Classes may be loaded from the local file system, a remote file system, or even the web.
When the JVM is started, three class loaders are used: 1. Bootstrap Classloader: Loads core java API file rt.jar from folder. 2. Extension Classloader: Loads jar files from folder. 3. System/Application Classloader: Loads jar files from path specified in the CLASSPATH environment variable.
Is a finally block executed when an exception is thrown from a try block that does not have a catch block, and if so, when?
A finally block is executed even if an exception is thrown or propagated to the calling code block.
Output can vary, being either:
When designing an abstract class, why should you avoid calling abstract methods inside its constructor?
This is a problem of initialization order. The subclass constructor will not have had a chance to run yet and there is no way to force it to run it before the parent class. Consider the following example class:
This seems like a good start for an abstract Widget: it allows subclasses to fill in width and height , and caches their initial values. However, look when you spec out a typical subclass implementation like so:
Now we’ve introduced a subtle bug: Widget.cachedWidth and Widget.cachedHeight will always be zero for SquareWidget instances! This is because the this.size = size assignment occurs after the Widget constructor runs.
Avoid calling abstract methods in your abstract classes’ constructors, as it restricts how those abstract methods can be implemented.
What variance is imposed on generic type parameters? How much control does Java give you over this?
Java’s generic type parameters are invariant . This means for any distinct types A and B , G<A> is not a subtype or supertype of G<B> . As a real world example, List<String> is not a supertype or subtype of List<Object> . So even though String extends (i.e. is a subtype of) Object , both of the following assignments will fail to compile:
Java does give you some control over this in the form of use-site variance . On individual methods, we can use ? extends Type to create a covariant parameter. Here’s an example:
Even though longs is a List<Long> and not List<Number> , it can be passed to sum .
Similarly, ? super Type lets a method parameter be contravariant . Consider a function with a callback parameter:
forEachNumber allows Callback<Object> to be a subtype of Callback <Number> , which means any callback that handles a supertype of Number will do:
Note, however, that attempting to provide a callback that handles only Long (a subtype of Number ) will rightly fail:
Liberal application of use-site variance can prevent many of the unsafe casts that often appear in Java code and is crucial when designing interfaces used by multiple developers.
What are static initializers and when would you use them?
A static initializer gives you the opportunity to run code during the initial loading of a class and it guarantees that this code will only run once and will finish running before your class can be accessed in any way.
They are useful for performing initialization of complex static objects or to register a type with a static registry, as JDBC drivers do.
Suppose you want to create a static, immutable Map containing some feature flags. Java doesn’t have a good one-liner for initializing maps, so you can use static initializers instead:
Within the same class, you can repeat this pattern of declaring a static field and immediately initializing it, since multiple static initializers are allowed.
If one needs a Set , how do you choose between HashSet vs. TreeSet ?
At first glance, HashSet is superior in almost every way: O(1) add , remove and contains , vs. O(log(N)) for TreeSet .
However, TreeSet is indispensable when you wish to maintain order over the inserted elements or query for a range of elements within the set.
Consider a Set of timestamped Event objects. They could be stored in a HashSet , with equals and hashCode based on that timestamp. This is efficient storage and permits looking up events by a specific timestamp, but how would you get all events that happened on any given day? That would require a O(n) traversal of the HashSet , but it’s only a O(log(n)) operation with TreeSet using the tailSet method:
If Event happens to be a class that we cannot extend or that doesn’t implement Comparable , TreeSet allows us to pass in our own Comparator :
Generally speaking, TreeSet is a good choice when order matters and when reads are balanced against the increased cost of writes.
What are method references, and how are they useful?
Method references were introduced in Java 8 and allow constructors and methods (static or otherwise) to be used as lambdas. They allow one to discard the boilerplate of a lambda when the method reference matches an expected signature.
For example, suppose we have a service that must be stopped by a shutdown hook. Before Java 8, we would have code like this:
With lambdas, this can be cut down considerably:
However, stop matches the signature of Runnable.run ( void return type, no parameters), and so we can introduce a method reference to the stop method of that specific SomeBusyService instance:
This is terse (as opposed to verbose code) and clearly communicates what is going on.
Method references don’t need to be tied to a specific instance, either; one can also use a method reference to an arbitrary object, which is useful in Stream operations. For example, suppose we have a Person class and want just the lowercase names of a collection of people:
A complex lambda can also be pushed into a static or instance method and then used via a method reference instead. This makes the code more reusable and testable than if it were “trapped” in the lambda.
So we can see that method references are mainly used to improve code organization, clarity and terseness.
How are Java enums more powerful than integer constants? How can this capability be used?
Enums are essentially final classes with a fixed number of instances. They can implement interfaces but cannot extend another class.
This flexibility is useful in implementing the strategy pattern, for example, when the number of strategies is fixed. Consider an address book that records multiple methods of contact. We can represent these methods as an enum and attach fields, like the filename of the icon to display in the UI, and any corresponding behaviour, like how to initiate contact via that method:
We can dispense with switch statements entirely by simply using instances of ContactMethod :
This is just the beginning of what can be done with enums. Generally, the safety and flexibility of enums means they should be used in place of integer constants, and switch statements can be eliminated with liberal use of abstract methods.
What does it mean for a collection to be “backed by” another? Give an example of when this property is useful.
If a collection backs another, it means that changes in one are reflected in the other and vice-versa.
For example, suppose we wanted to create a whitelist function that removes invalid keys from a Map . This is made far easier with Map.keySet , which returns a set of keys that is backed by the original map. When we remove keys from the key set, they are also removed from the backing map:
retainAll writes through to the backing map, and allows us to easily implement something that would otherwise require iterating over the entries in the input map, comparing them against allowedKey , etcetera.
Note, it is important to consult the documentation of the backing collection to see which modifications will successfully write through. In the example above, map.keySet().add(value) would fail, because we cannot add a key to the backing map without a value.
What is reflection? Give an example of functionality that can only be implemented using reflection.
Reflection allows programmatic access to information about a Java program’s types. Commonly used information includes: methods and fields available on a class, interfaces implemented by a class, and the runtime-retained annotations on classes, fields and methods.
Examples given are likely to include:
- Annotation-based serialization libraries often map class fields to JSON keys or XML elements (using annotations). These libraries need reflection to inspect those fields and their annotations and also to access the values during serialization.
- Model-View-Controller frameworks call controller methods based on routing rules. These frameworks must use reflection to find a method corresponding to an action name, check that its signature conforms to what the framework expects (e.g. takes a Request object, returns a Response ), and finally, invoke the method.
- Dependency injection frameworks lean heavily on reflection. They use it to instantiate arbitrary beans for injection, check fields for annotations such as @Inject to discover if they require injection of a bean, and also to set those values.
- Object-relational mappers such as Hibernate use reflection to map database columns to fields or getter/setter pairs of a class, and can go as far as to infer table and column names by reading class and getter names, respectively.
A concrete code example could be something simple, like copying an object’s fields into a map:
Such tricks can be useful for debugging, or for utility methods such as a toString method that works on any class.
Aside from implementing generic libraries, direct use of reflection is rare but it is still a handy tool to have. Knowledge of reflection is also useful for when these mechanisms fail.
However, it is often prudent to avoid reflection unless it is strictly necessary, as it can turn straightforward compiler errors into runtime errors.
Nested classes can be static or non-static (also called an inner class). How do you decide which to use? Does it matter?
The key difference between is that inner classes have full access to the fields and methods of the enclosing class. This can be convenient for event handlers, but comes at a cost: every instance of an inner class retains and requires a reference to its enclosing class.
With this cost in mind, there are many situations where we should prefer static nested classes. When instances of the nested class will outlive instances of the enclosing class, the nested class should be static to prevent memory leaks. Consider this implementation of the factory pattern:
At a glance, this design looks good: the WidgetParserFactory hides the implementation details of the parser with the nested class WidgetParserImpl . However, WidgetParserImpl is not static, and so if WidgetParserFactory is discarded immediately after the WidgetParser is created, the factory will leak, along with all the references it holds.
WidgetParserImpl should be made static, and if it needs access to any of WidgetParserFactory ’s internals, they should be passed into WidgetParserImpl ’s constructor instead. This also makes it easier to extract WidgetParserImpl into a separate class should it outgrow its enclosing class.
Inner classes are also harder to construct via reflection due to their “hidden” reference to the enclosing class, and this reference can get sucked in during reflection-based serialization, which is probably not intended.
So we can see that the decision of whether to make a nested class static is important, and that one should aim to make nested classes static in cases where instances will “escape” the enclosing class or if reflection on those nested classes is involved.
What is the difference between String s = "Test" and String s = new String("Test") ? Which is better and why?
In general, String s = "Test" is more efficient to use than String s = new String("Test") .
In the case of String s = "Test" , a String with the value “Test” will be created in the String pool. If another String with the same value is then created (e.g., String s2 = "Test" ), it will reference this same object in the String pool.
However, if you use String s = new String("Test") , in addition to creating a String with the value “Test” in the String pool, that String object will then be passed to the constructor of the String Object (i.e., new String("Test") ) and will create another String object (not in the String pool) with that value. Each such call will therefore create an additional String object (e.g., String s2 = new String("Test") would create an addition String object, rather than just reusing the same String object from the String pool).
There is more to interviewing than tricky technical questions, so these are intended merely as a guide. Not every “A” candidate worth hiring will be able to answer them all, nor does answering them all guarantee an “A” candidate. At the end of the day, hiring remains an art, a science — and a lot of work .
Tired of interviewing candidates? Not sure what to ask to get you a top hire?
Let Toptal find the best people for you.
Our Exclusive Network of Java Developers
Looking to land a job as a Java Developer?
Let Toptal find the right job for you.
Job Opportunities From Our Network
Submit an interview question
Submitted questions and answers are subject to review and editing, and may or may not be selected for posting, at the sole discretion of Toptal, LLC.
Looking for Java Developers?
Looking for Java Developers ? Check out Toptal’s Java developers.
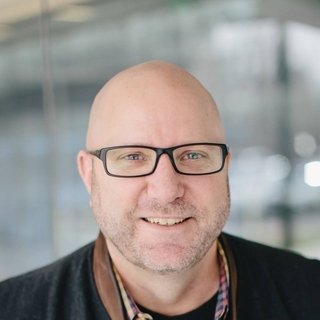
Shane Freed
Shane is a Java developer with 25 years of experience building enterprise apps and specializes in writing maintainable, extensible, SOLID code. While 95% of Shane's experience is in the Java realm, his passion is full-stack development with Angular. Shane's served in many different roles, including architect, team lead, lead developer, and individual contributor. While Shane doesn't always have to lead a project, his USMC background makes him perfect for lead roles and mentoring.
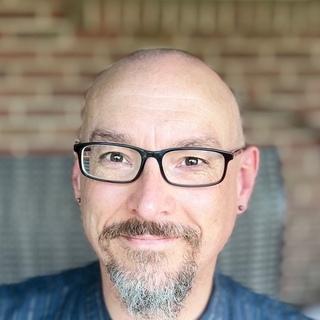
Eric George
Eric is an expert Java software engineer specializing in back-end and microservice development as well as workflow/release engineering. Comfortable with a broad set of libraries and environments, he is also skilled in CI/CD and cloud deployment. Additionally, Eric focuses on test-driven, domain-driven, and object-oriented design.
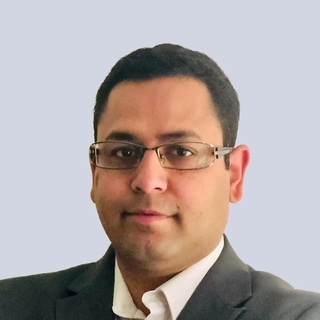
Gaurav Tyagi
Gaurav is a polyglot back-end developer with 20+ years of hands-on experience; he's worked as a freelance developer for the past several years. He primarily works with Java and C and is also comfortable with Go being the primary project language or for infrastructure modules. Thanks to having built products in various industries—like finance, healthcare, media, retail, food, and travel—Gaurav has a range of tech and functional skills, which means he can hit the ground running on a project.
Toptal Connects the Top 3% of Freelance Talent All Over The World.
Join the Toptal community.
Review these 50 questions to crack your Java programming interview
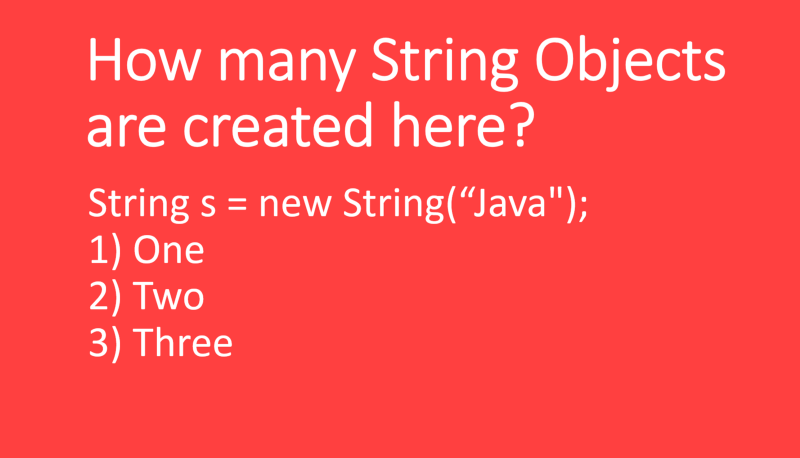
by javinpaul
A list of frequently asked Java questions from programming job interviews.
Hello, everybody! Over the past few years, I have been sharing a lot of Java Interview questions and discussion individually. Many of my readers have requested that I bring them together so that they can have them in the same spot. This post is the result of that.
This article contains more than 50 Java Interview questions covering all important topics like Core Java fundamentals, Java Collection Framework , Java Multithreading and Concurrency , Java IO , JDBC , JVM Internals , Coding Problems , Object-Oriented programming , etc.
The questions are also picked up from various interviews and they are, by no means, very difficult. You might have seen them already in your phone or face-to-face round of interviews.
The questions are also very useful to review important topics like multithreading and collections. I have also shared some useful resources for further learning and improvement like The Complete Java MasterClass to brush up and fill gaps in your Java skills.
So what are we waiting for? Here is the list of some of the most frequently asked Java questions in interviews for both beginner and experienced Java developers.
50+ Java Interview Questions for 2 to 3 years Experienced Programmers
So, without wasting any more of your time, here is my list of some of the frequently asked Core Java Interview Question s for beginner programmers. This list focuses on beginners and less experienced devs, like someone with 2 to 3 years of experience in Java.
1) How does Java achieve platform independence? ( answer ) hint: bytecode and Java Virtual Machine
2) What is ClassLoader in Java? ( answer ) hint: part of JVM that loads bytecodes for classes. You can write your own.
3) Write a Java program to check if a number is Even or Odd? ( answer ) hint: you can use bitwise operator, like bitwise AND, remember, even the number has zero at the end in binary format and an odd number has 1 in the end.
4) Difference between ArrayList and HashSet in Java? ( answer ) hint: all differences between List and Set are applicable here, e.g. ordering, duplicates, random search, etc. See Java Fundamentals: Collections by Richard Warburton to learn more about ArrayList, HashSet and other important Collections in Java.
5) What is double checked locking in Singleton? ( answer ) hint: two-time check whether instances is initialized or not, first without locking and second with locking.
6) How do you create thread-safe Singleton in Java? ( answer ) hint: many ways, like using Enum or by using double-checked locking pattern or using a nested static class.
7) When to use the volatile variable in Java? ( answer ) hint: when you need to instruct the JVM that a variable can be modified by multiple threads and give hint to JVM that does not cache its value.
8) When to use a transient variable in Java? ( answer ) hint: when you want to make a variable non-serializable in a class, which implements the Serializable interface. In other words, you can use it for a variable whose value you don’t want to save. See The Complete Java MasterClass to learn about transient variables in Java.
9) Difference between the transient and volatile variable in Java? ( answer ) hint: totally different, one used in the context of serialization while the other is used in concurrency.
10) Difference between Serializable and Externalizable in Java? ( answer ) hint: Externalizable gives you more control over the Serialization process.
11) Can we override the private method in Java? ( answer ) hint: No, because it’s not visible in the subclass, a primary requirement for overriding a method in Java.
12) Difference between Hashtable and HashMap in Java? ( answer ) hint: several but most important is Hashtable , which is synchronized, while HashMap is not. It's also legacy and slow as compared to HashMap .
13) Difference between List and Set in Java? ( answer ) hint: List is ordered and allows duplicate. Set is unordered and doesn't allow duplicate elements.
14) Difference between ArrayList and Vector in Java ( answer ) hint: Many, but most important is that ArrayList is non-synchronized and fast while Vector is synchronized and slow. It's also legacy class like Hashtable .
15) Difference between Hashtable and ConcurrentHashMap in Java? ( answer ) hint: more scalable. See Java Fundamentals: Collections by Richard Warburton to learn more.
16) How does ConcurrentHashMap achieve scalability? ( answer ) hint: by dividing the map into segments and only locking during the write operation.
17) Which two methods you will override for an Object to be used as Key in HashMap ? ( answer ) hint: equals and hashcode
18) Difference between wait and sleep in Java? ( answer ) hint: The wait() method releases the lock or monitor, while sleep doesn't.
19) Difference between notify and notifyAll in Java? ( answer ) hint: notify notifies one random thread is waiting for that lock while notifyAll inform to all threads waiting for a monitor. If you are certain that only one thread is waiting then use notify , or else notifyAll is better. See Threading Essentials Mini-Course by Java Champion Heinz Kabutz to learn more about threading basics.
20) Why you override hashcode, along with equals() in Java? ( answer ) hint: to be compliant with equals and hashcode contract, which is required if you are planning to store your object into collection classes, e.g. HashMap or ArrayList .
21) What is the load factor of HashMap means? ( answer ) hint: The threshold that triggers the re-sizing of HashMap is generally 0.75, which means HashMap resize itself if it's 75 percent full.
22) Difference between ArrayList and LinkedList in Java? ( answer ) hint: same as an array and linked list, one allows random search while other doesn't. Insertion and deletion easy on the linked list but a search is easy on an array. See Java Fundamentals: Collections , Richard Warburton’s course on Pluralsight, to learn more about essential Collection data structure in Java.
23) Difference between CountDownLatch and CyclicBarrier in Java? ( answer ) hint: You can reuse CyclicBarrier after the barrier is broken but you cannot reuse CountDownLatch after the count reaches to zero.
24) When do you use Runnable vs Thread in Java? ( answer ) hint: always
25) What is the meaning of Enum being type-safe in Java? ( answer ) hint: It means you cannot assign an instance of different Enum type to an Enum variable. e.g. if you have a variable like DayOfWeek day then you cannot assign it value from DayOfMonth enum.
26) How does Autoboxing of Integer work in Java? ( answer ) hint: By using the valueOf() method in Java.
27) Difference between PATH and Classpath in Java? ( answer ) hint: PATH is used by the operating system while Classpath is used by JVM to locate Java binary, e.g. JAR files or Class files. See Java Fundamentals: The Core Platform to learn more about PATH , Classpath , and other Java environment variable.
28) Difference between method overloading and overriding in Java? ( answer ) hint: Overriding happens at subclass while overloading happens in the same class. Also, overriding is a runtime activity while overloading is resolved at compile time.
29) How do you prevent a class from being sub-classed in Java? ( answer ) hint: just make its constructor private
30) How do you restrict your class from being used by your client? ( answer ) hint: make the constructor private or throw an exception from the constructor
31) Difference between StringBuilder and StringBuffer in Java? ( answer ) hint: StringBuilder is not synchronized while StringBuffer is synchronized.
32) Difference between Polymorphism and Inheritance in Java? ( answer ) hint: Inheritance allows code reuse and builds the relationship between class, which is required by Polymorphism, which provides dynamic behavior. See Java Fundamentals: Object-Oriented Design to learn more about OOP features.
33) Can we override static method in Java? ( answer ) hint: No, because overriding resolves at runtime while static method call is resolved at compile time.
34) Can we access the private method in Java? ( answer ) hint: yes, in the same class but not outside the class
35) Difference between interface and abstract class in Java? ( answer ) hint: from Java 8 , the difference is blurred. However, a Java class can still implement multiple interfaces but can only extend one class.
36) Difference between DOM and SAX parser in Java? ( answer ) hint: DOM loads whole XML File in memory while SAX doesn’t. It is an event-based parser and can be used to parse a large file, but DOM is fast and should be preferred for small files.
37) Difference between throw and throws keyword in Java? ( answer ) hint: throws declare what exception a method can throw in case of error but throw keyword actually throws an exception. See Java Fundamentals: Exception Handling to learn more about Exception handling in Java.
38) Difference between fail-safe and fail-fast iterators in Java? ( answer ) hint: fail-safe doesn’t throw ConcurrentModificationException while fail-fast does whenever they detect an outside change on the underlying collection while iterating over it.
39) Difference between Iterator and Enumeration in Java? ( answer ) hint: Iterator also gives you the ability to remove an element while iterating while Enumeration doesn’t allow that.
40) What is IdentityHashMap in Java? ( answer ) hint: A Map , which uses the == equality operator to check equality instead of the equals() method.
41) What is the String pool in Java? ( answer ) hint: A pool of String literals. Remember it's moved to heap from perm gen space in JDK 7.
42) Can a Serializable class contains a non-serializable field in Java? ( answer ) hint: Yes, but you need to make it either static or transient.
43) Difference between this and super in Java? ( answer ) hint: this refers to the current instance while super refers to an instance of the superclass.
44) Difference between Comparator and Comparable in Java? ( answer ) hint: Comparator defines custom ordering while Comparable defines the natural order of objects, e.g. the alphabetic order for String . See The Complete Java MasterClass to learn more about sorting in Java.
45) Difference between java.util.Date and java.sql.Date in Java? ( answer ) hint: former contains both date and time while later contains only date part.
46) Why wait and notify method are declared in Object class in Java? ( answer ) hint: because they require lock which is only available to an object.
47) Why Java doesn’t support multiple inheritances? ( answer ) hint: It doesn’t support because of a bad experience with C++, but with Java 8, it does in some sense — only multiple inheritances of Type are not supported in Java now.
48) Difference between checked and unchecked Exception in Java? ( answer ) hint: In case of checked, you must handle exception using catch block, while in case of unchecked, it’s up to you; compile will not bother you.
49) Difference between Error and Exception in Java? ( answer ) hint: I am tired of typing please check the answer
50) Difference between Race condition and Deadlock in Java? ( answer ) hint: both are errors that occur in a concurrent application, one occurs because of thread scheduling while others occur because of poor coding. See Multithreading and Parallel Computing in Java to learn more about deadlock, Race Conditions, and other multithreading issues.
Closing Notes
Thanks, You made it to the end of the article … Good luck with your programming interview! It’s certainly not going to be easy, but by following this roadmap and guide, you are one step closer to becoming a DevOps engineer .
If you like this article, then please share with your friends and colleagues, and don’t forget to follow javinpaul on Twitter!
Additional Resources
- Java Interview Guide: 200+ Interview Questions and Answers
- Spring Framework Interview Guide — 200+ Questions & Answers
- Preparing For a Job Interview By John Sonmez
- Java Programming Interview Exposed by Markham
- Cracking the Coding Interview — 189 Questions and Answers
- Data Structure and Algorithms Analysis for Job Interviews
- 130+ Java Interview Questions of Last 5 Years
P.S. — If you need some FREE resources to learn Java, you can check out this list of free Java courses to start your preparation. P. S. S. — I have not provided the answer to the interview questions shared in the image “ How many String objects are created in the code?” can you guess and explain?
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- ▼Java Exercises
- Basic Part-I
- Basic Part-II
- Conditional Statement
- Recursive Methods
- Java Enum Types
- Exception Handling
- Java Inheritance
- Java Abstract Classes
- Java Thread
- Java Multithreading
- Java Lambda expression
- Java Generic Method
- Object-Oriented Programming
- Java Interface
- Java Encapsulation
- Java Polymorphism
- File Input-Output
- Regular Expression
- ▼JavaFx Exercises
- JavaFx Exercises Home
- ..More to come..
Java Programming Exercises, Practice, Solution
Java exercises.
Java is the foundation for virtually every type of networked application and is the global standard for developing and delivering embedded and mobile applications, games, Web-based content, and enterprise software. With more than 9 million developers worldwide, Java enables you to efficiently develop, deploy and use exciting applications and services.
The best way we learn anything is by practice and exercise questions. Here you have the opportunity to practice the Java programming language concepts by solving the exercises starting from basic to more complex exercises. A sample solution is provided for each exercise. It is recommended to do these exercises by yourself first before checking the solution.
Hope, these exercises help you to improve your Java programming coding skills. Currently, following sections are available, we are working hard to add more exercises .... Happy Coding!
List of Java Exercises:
- Basic Exercises Part-I [ 150 Exercises with Solution ]
- Basic Exercises Part-II [ 99 Exercises with Solution ]
- Methods [ 23 Exercises with Solution ]
- Data Types Exercises [ 15 Exercises with Solution ]
- Java Enum Types Exercises [ 5 Exercises with Solution ]
- Conditional Statement Exercises [ 32 Exercises with Solution ]
- Java recursive method Exercises [ 15 Exercises with Solution ]
- Math [ 27 Exercises with Solution ]
- Numbers [ 28 Exercises with Solution ]
- Java Inheritance Exercises [ 9 exercises with solution ]
- Java Abstract Classes Exercises [ 12 exercises with solution ]
- Java Interface Exercises [ 11 exercises with solution ]
- Java Encapsulation Exercises [ 7 exercises with solution ]
- Java Polymorphism Exercises [ 12 exercises with solution ]
- Object-Oriented Programming [ 28 Exercises with Solution ]
- Exercises on handling and managing exceptions in Java [ 7 Exercises with Solution ]
- Java Lambda expression Exercises [ 25 exercises with solution ]
- Streams [ 8 Exercises with Solution ]
- Java Thread Exercises [ 7 exercises with solution ]
- Java Miltithreading Exercises [ 10 exercises with solution ]
- Array [ 77 Exercises with Solution ]
- Stack [ 29 Exercises with Solution ]
- Collection [ 126 Exercises with Solution ]
- String [ 107 Exercises with Solution ]
- Input-Output-File-System [ 18 Exercises with Solution ]
- Date Time [ 44 Exercises with Solution ]
- Java Generic Methods [ 7 exercises with solution ]
- Java Unit Test [ 10 Exercises with Solution ]
- Search [ 7 Exercises with Solution ]
- Sorting [ 19 Exercises with Solution ]
- Regular Expression [ 30 Exercises with Solution ]
- JavaFX [ 70 Exercises with Solution ]
Note: If you are not habituated with Java programming you can learn from the following :
- Java Programming Language
More to Come !
Popularity of Programming Language Worldwide, Nov 2023 compared to a year ago:
Rank | Change | Language | Share | Trend |
---|---|---|---|---|
1 | Python | 27.99 % | +0.0 % | |
2 | Java | 15.91 % | -0.8% | |
3 | Javascript | 9.18 % | -0.3% | |
4 | C/C++ | 6.76 % | +0.2% | |
5 | C# | 6.67 % | -0.3 % | |
6 | PHP | 4.86 % | -0.3 % | |
7 | R | 4.45% | +0.4% | |
8 | TypeScript | 2.95 % | +0.1% | |
9 | Swift | 2.7 % | +0.6% | |
10 | Objective-C | 2.32% | +0.2% | |
11 | Rust | `1.98% | +0.3% | |
12 | Go | 1.98% | -0.0% | |
13 | Kotlin | 1.76 % | -0.1% | |
14 | Matlab | 1.6 % | +0.0% | |
15 | Ada | 1.02% | +0.2% | |
16 | Ruby | 1.0 % | -0.1 % | |
17 | Dart | 0.99 % | +0.1 % | |
18 | Powershell | 0.93 % | +0.0 % | |
19 | VBA | 0.93 % | -0.1 % | |
20 | Scala | 0.62 % | -0.1 % | |
21 | Lua | 0.62 % | 0.0 % | |
22 | Abap | 0.58 % | +0.1 % | |
23 | Visual Basic | 0.55 % | -0.1 % | |
24 | Julia | 0.35 % | -0.0 % | |
25 | Groovy | 0.31 % | -0.1 % | |
26 | Perl | 0.31 % | -0.1 % | |
27 | Haskell | 0.27 % | -0.0 % | |
28 | Cobol | 0.25 % | -0.1 % | |
29 | Delphi/Pascal | 0.18 % | +0.2 % |
Source : https://pypl.github.io/PYPL.html
TIOBE Index for November 2023
Nov 2023 | Nov 2022 | Change | Programming Language | Ratings | Change |
---|---|---|---|---|---|
1 | 1 | Python | 14.16% | -3.02% | |
2 | 2 | C | 11.77% | -3.31% | |
3 | 4 | C++ | 10.36% | -0.39% | |
4 | 3 | Java | 8.35% | -3.63% | |
5 | 5 | C# | 7.65% | +3.40% | |
6 | 7 | JavaScript | 3.21% | +0.47% | |
7 | 10 | PHP | 2.30% | +0.61% | |
8 | 6 | Visual Basic | 2.10% | -2.01% | |
9 | 9 | SQL | 1.88% | +0.07% | |
10 | 8 | Assembly language | 1.35% | -0.83% | |
11 | 17 | Scratch | 1.31% | +0.43% | |
12 | 24 | Fortran | 1.30% | +0.74% | |
13 | 11 | Go | 1.19% | +0.05% | |
14 | 15 | MATLAB | 1.15% | +0.14% | |
15 | 28 | Kotlin | 1.15% | +0.68% | |
16 | 14 | Delphi/Object Pascal | 1.14% | +0.07% | |
17 | 18 | Swift | 1.04% | +0.17% | |
18 | 19 | Ruby | 0.99% | +0.14% | |
19 | 12 | R | 0.93% | -0.20% | |
20 | 20 | Rust | 0.91% | +0.16% |
Source : https://www.tiobe.com/tiobe-index/
List of Exercises with Solutions :
- HTML CSS Exercises, Practice, Solution
- JavaScript Exercises, Practice, Solution
- jQuery Exercises, Practice, Solution
- jQuery-UI Exercises, Practice, Solution
- CoffeeScript Exercises, Practice, Solution
- Twitter Bootstrap Exercises, Practice, Solution
- C Programming Exercises, Practice, Solution
- C# Sharp Programming Exercises, Practice, Solution
- PHP Exercises, Practice, Solution
- Python Exercises, Practice, Solution
- R Programming Exercises, Practice, Solution
- Java Exercises, Practice, Solution
- SQL Exercises, Practice, Solution
- MySQL Exercises, Practice, Solution
- PostgreSQL Exercises, Practice, Solution
- SQLite Exercises, Practice, Solution
- MongoDB Exercises, Practice, Solution
[ Want to contribute to Java exercises? Send your code (attached with a .zip file) to us at w3resource[at]yahoo[dot]com. Please avoid copyrighted materials.]
Do not submit any solution of the above exercises at here, if you want to contribute go to the appropriate exercise page.
Follow us on Facebook and Twitter for latest update.
- Weekly Trends and Language Statistics
{{ activeMenu.name }}
- Python Courses
- JavaScript Courses
- Artificial Intelligence Courses
- Data Science Courses
- React Courses
- Ethical Hacking Courses
- View All Courses
Fresh Articles
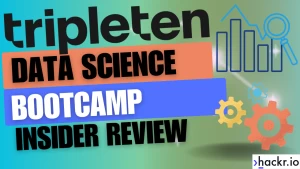
- Python Projects
- JavaScript Projects
- Java Projects
- HTML Projects
- C++ Projects
- PHP Projects
- View All Projects
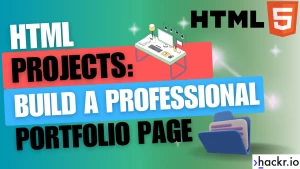
- Python Certifications
- JavaScript Certifications
- Linux Certifications
- Data Science Certifications
- Data Analytics Certifications
- Cybersecurity Certifications
- View All Certifications
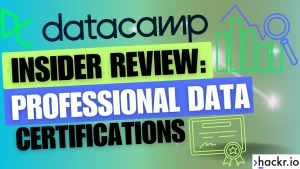
- IDEs & Editors
- Web Development
- Frameworks & Libraries
- View All Programming
- View All Development
- App Development
- Game Development
- Courses, Books, & Certifications
- Data Science
- Data Analytics
- Artificial Intelligence (AI)
- Machine Learning (ML)
- View All Data, Analysis, & AI
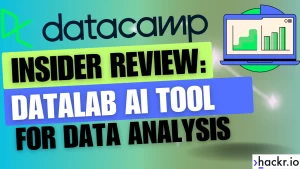
- Networking & Security
- Cloud, DevOps, & Systems
- Recommendations
- Crypto, Web3, & Blockchain
- User-Submitted Tutorials
- View All Blog Content
- JavaScript Online Compiler
- HTML & CSS Online Compiler
- Certifications
- Programming
- Development
- Data, Analysis, & AI
- Online JavaScript Compiler
- Online HTML Compiler
Don't have an account? Sign up
Forgot your password?
Already have an account? Login
Have you read our submission guidelines?
Go back to Sign In

Top 80 Java Interview Questions and Answers [2024]
To land the job, it helps to review common and challenging Java interview questions. After all, the class-based, general-purpose, object-oriented programming language is one of the most widely used programming languages in the world .
We prepared these Java interview questions with answers from experts. Expect to cover the basics with beginner-friendly topics and much more advanced challenges with specific code to help professional Software Developers and Android Applications Developers.
Remember that, with a plethora of great features , the programming language is preferred not only by seasoned experts but also by those new to the programming world. Our interview questions start with basic Java concepts and progress to much more difficult challenges.
Whether you're seeking a new gig or hiring to expand your team, read on to review the best Java interview questions for 2024.
- Top Java Interview Questions and Answers
We also recommend you brush up on your Java skills with this Java Cheat Sheet before starting your Java interview preparation.
We have divided these interview questions into several sections. Check the breakdown below to review basic, advanced, OOPs, exception handling, and programming Java interview questions. We also have specific examples of code. Review the coding Java interview questions section for reference.
Basic Java Interview Questions
1. what is java.
Java is an object-oriented, high-level, general-purpose programming language originally designed by James Gosling and further developed by the Oracle Corporation. It is one of the most popular programming languages in the world .
2. What is the Java Virtual Machine?
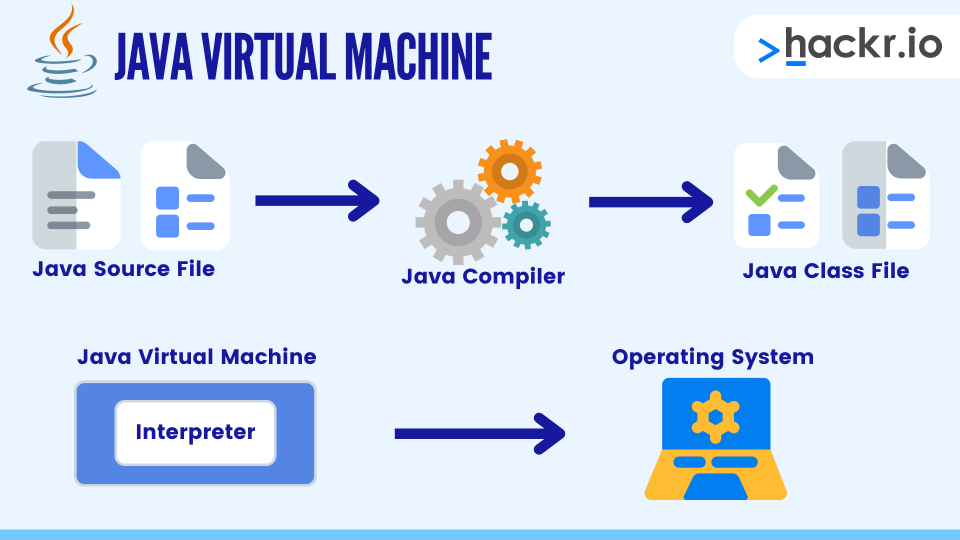
JVM is a program that interprets the intermediate Java byte code and generates the desired output. It is because of bytecode and JVM that programs written in Java are highly portable.
3. What are the features of Java?
The following are the various features of the Java programming language:
- High Performance: Using a JIT (Just-In-Time) compiler allows high performance in Java. The JIT compiler converts the Java bytecode into machine language code, which then gets executed by the JVM.
- Multi-threading: A thread is a flow of execution. The JVM creates a thread which is called the main thread. Java allows the creation of several threads using either extending the thread class or implementing the Runnable interface.
- OOPs Concepts: Java follows various OOPs concepts , namely abstraction, encapsulation, inheritance, object-oriented, and polymorphism
- Platform Independency: Java makes use of the Java Virtual Machine or JVM, which allows a single Java program to operate on multiple platforms without any modifications.
You may want to check out detailed explanations of Java features here .
4. How does Java enable high performance?
In Just-in-Time compilation, the required code is executed at run time. Typically, it involves translating bytecode into machine code and then executing it directly. It allows for high performance. The JIT compiler is enabled by default in Java and gets activated as soon as a method is called.
It then compiles the bytecode of the Java method into native machine code.
After that, the JVM calls the compiled code directly instead of interpreting it.
5. What are the differences between JVM, JRE, and JDK?
|
|
|
|
| Java Virtual Machine | Java Runtime Environment | Java Development Kit |
| It provides a runtime environment to execute Java bytecode. | It is a set of software tools used for developing Java applications. | It is a software development environment used to develop Java applications. |
| It is a runtime instance created when we run a Java class. | It exists physically. | It exists physically. |
| Its implementation is known as JRE | It is the implementation of JVM | It is an implementation of any one of the below given Java Platforms released by Oracle Corporation: Standard Edition Java Platform Enterprise Edition Java Platform Micro Edition Java Platform |
6. What is the JIT compiler?
JIT compiler runs after the program is executed and compiles the code into a faster form, hosting the CPU's native instructing set. JIT can access dynamic runtime information, whereas a standard compiler doesn't and can make better optimizations like inlining functions that are used frequently.
7. What are Java IDEs?
A Java IDE is a software that allows Java developers to easily write as well as debug Java programs. It is basically a collection of various programming tools, accessible via a single interface, and several helpful features, such as code completion and syntax highlighting.
Codenvy, Eclipse, and NetBeans are some of the most popular Java IDEs .
8. Java is a platform-independent language. Why?
Java does not depend on any particular hardware or software because it is compiled by the compiler and then converted into byte code. Byte code is platform-independent and can run on multiple systems. The only requirement is that Java needs a runtime environment, i.e., JRE, which is a set of tools used for developing Java applications.
9. Explain Typecasting.
The concept of assigning a variable of one data type to a variable of another data type. This is not possible for the boolean data type. There are two types: implicit and explicit.
10. What are the different types of typecasting?
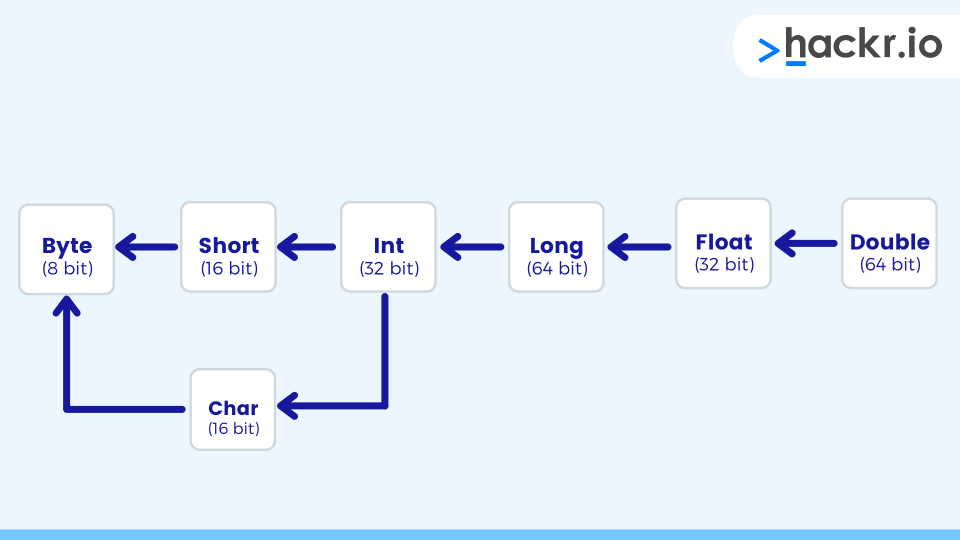
The different types of typecasting are:
- Implicit: Storing values from a smaller data type to the larger data type. It is automatically done by the compiler.
- Explicit: Storing the value of a larger data type into a smaller data type. This results in information loss:
- Truncation: While converting a value from a larger data type to a smaller data type, the extra data will be truncated. This code example explains it :
After execution, the variable i will contain only 3 and not the decimal portion.
- Out of Range: Typecasting does not allow assigning value more than its range; if that happens then the data is lost in such cases. This code example explains it:
long l = 123456789;
byte b = ( byte ) l; // byte is of not the same range as long so there will be loss of data.
11. Explain access modifiers in Java.
Access modifiers are predefined keywords in Java that are used to restrict the access of a class, method, constructor, and data member in another class. Java supports four access modifiers:
|
|
|
|
|
Same class | yes | yes | yes | yes |
Same package subclass | yes | no | yes | yes |
Same package non-subclass | yes | no | yes | yes |
Different package subclass | no | no | yes | yes |
Different package non-subclass | no | no | no | yes |
12. What are the default values for local variables?
The local variables are not initialized to any default value, neither primitives nor object references.
OOPs Java Interview Questions
13. what is object-oriented programming .
OOPs is a programming paradigm centered around objects rather than functions. It is not a tool or a programming language, it is a paradigm that was designed to overcome the flaws of procedural programming .
There are many languages that follow OOPs concepts — some popular ones are Java, Python, and Ruby. Some frameworks also follow OOPs concepts, such as Angular.
14. Explain the OOPs concepts.
The following are the various OOPS Concepts:
- Abstraction: Representing essential features without the need to give out background details. The technique is used for creating a new suitable data type for some specific application.
- Aggregation: All objects have their separate lifecycle, but ownership is present. No child object can belong to some other object except for the parent object.
- Association: The relationship between two objects, where each object has its separate lifecycle. There is no ownership.
- Class: A group of similar entities.
- Composition: Also called the death relationship, it is a specialized form of aggregation. Child objects don't have a lifecycle. As such, they automatically get deleted if the associated parent object is deleted.
- Encapsulation: Refers to the wrapping up of data and code into a single entity. This allows the variables of a class to be only accessible by the parent class and no other classes.
- Inheritance: When an object acquires the properties of some other object, it is called inheritance. It results in the formation of a parent-child relationship amongst classes involved. This offers a robust and natural mechanism of organizing and structuring software.
- Object: Denotes an instance of a class. Any class can have multiple instances. An object contains the data as well as the method that will operate on the data
- Polymorphism: Refers to the ability of a method, object, or variable to assume several forms.
Decision Making Java Interview Questions
15. Differentiate between break and continue.
|
|
Used with both loop and switch statement. | Used with only loop statements. |
It terminates the loop or switch block. | It does not terminate but skips to the next iteration. |
Classes, Objects, and Methods Java Interview Questions
16. what is an object.
An instance of a Java class is known as an object. Two important properties of a Java object are behavior and state . An object is created as soon as the JVM comes across the new keyword.
17. Define classes in Java.
A class is a collection of objects of similar data types. Classes are user-defined data types and behave like built-in types of a programming language.
Syntax of a class:
Example of Class:
18. What are static methods and variables?
A class has two sections: one declares variables, and the other declares methods. These are called instance variables and instance methods, respectively. They are termed so because every time a class is instantiated, a new copy of each of them is created.
Variables and methods can be created that are common to all objects and accessed without using a particular object by declaring them static. Static members are also available to be used by other classes and methods.
19. What do you mean by Constructor?
A constructor is a method that has the same name as that of the class to which it belongs. As soon as a new object is created, a constructor corresponding to the class gets invoked. Although the user can explicitly create a constructor, it is created on its own as soon as a class is created. This is known as the default constructor. Constructors can be overloaded.
If an explicitly-created constructor has a parameter, then it is necessary to create another constructor without a parameter.
20. What are local variables and instance variables?
Variables that are only accessible to the method or code block in which they are declared are known as local variables. Instance variables, on the other hand, are accessible to all methods in a class.
While local variables are declared inside a method or a code block, instance variables are declared inside a class but outside a method. Even when not assigned, instance variables have a value that can be null, 0, 0.0, or false. This isn't the case with local variables that need to be assigned a value, where failing to assign a value will yield an error. Local variables are automatically created when a method is called and destroyed as soon as the method exits. For creating instance variables, the new keyword must be used.
21. What is Method Overriding?
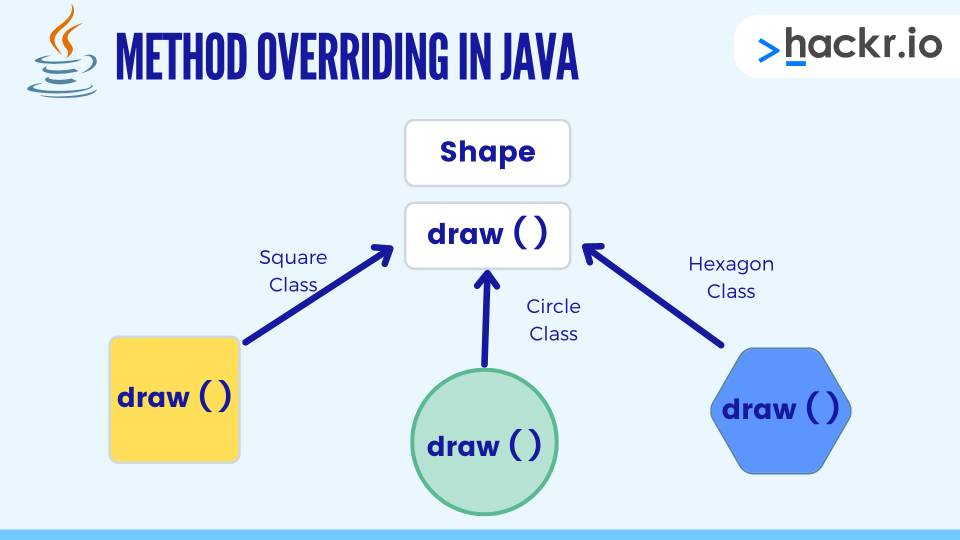
Method overriding in Java allows a subclass to offer a specific implementation of a method that has already been provided by its parent or superclass. Method overriding happens if the subclass method and the Superclass method have:
- The same name
- The same argument
- The same return type
22. What is Overloading?
Overloading is the phenomenon when two or more different methods (method overloading) or operators (operator overloading) have the same representation. For example, the + operator adds two integer values but concatenates two strings. Similarly, an overloaded function called Add can be used for two purposes
- To add two integers
- To concatenate two strings
Unlike method overriding, method overloading requires two overloaded methods to have the same name but different arguments. The overloaded functions may or may not have different return types.
23. What role does the final keyword play in Java? What impact does it have on a variable, method, and class?
The final keyword in Java is a non-access modifier that applies only to a class, method, or variable. It serves a different purpose based on the context where it is used.
- With a class: When a class is declared as final, then it is disabled from being subclassed i.e., no class can extend the final class.
- With a method: Any method accompanying the final keyword is restricted from being overridden by the subclass.
- With a variable: A variable followed by the final keyword is not able to change the value that it holds during the program execution. So, it behaves like a constant.
Arrays, Strings and Vectors Java Interview Questions
24. draw a comparison between array and arraylist..
An array necessitates stating the size during the time of declaration, while an array list doesn't necessarily require size as it changes size dynamically. To put an object into an array, there is the need to specify the index. However, no such requirement is in place for an array list. While an array list is parameterized, an array is not parameterized.
25. What are the differences between String, Stringbuilder, and Stringbuffer?
String variables are stored in a constant string pool. With the change in the string reference, it becomes impossible to delete the old value. For example, if a string has stored a value "Old," then adding the new value "New" will not delete the old value. It will still be there, however, in a dormant state. In a Stringbuffer, values are stored in a stack. With the change in the string reference, the new value replaces the older value. The Stringbuffer is synchronized (and therefore, thread-safe) and offers slower performance than the StringBuilder, which is also a Stringbuffer but is not synchronized. Hence, performance is faster in Stringbuilder than the Stringbuffer.
26. What is the String Pool?
The string pool is a collection of strings stored in the heap memory refers to. Whenever a new object is created, it is checked if it is already present in the string pool. If it is already present, then the same reference is returned to the variable, otherwise a new object is created in the string pool, and the respective reference is returned.
- Advanced Java Interview Questions
Interfaces and Abstract Classes Java Interview Questions
27. what do you know about interfaces.
A Java interface is a template that has only method declarations and not method implementations. It is a workaround for achieving multiple inheritances in Java. Some worth remembering important points regarding Java interfaces are:
- A class that implements the interface must provide an implementation for all methods declared in the interface.
- All methods in an interface are internally public abstract void.
- All variables in an interface are internally public static final.
- Classes do not extend but implement interfaces.
28. How is an Abstract class different from an Interface?
There are several differences between an Abstract class and an Interface in Java, summed up as follows:
- Constituents: An abstract class contains instance variables, whereas an interface can contain only constants.
- Constructor and Instantiation: While an interface has neither a constructor nor it can be instantiated, an abstract class can have a default constructor that is called whenever the concrete subclass is instantiated.
- Implementation of Methods – All classes that implement the interface need to provide an implementation for all the methods contained by it. A class that extends the abstract class, however, doesn't require implementing all the methods contained in it. Only abstract methods need to be implemented in the concrete subclass.
- Type of Methods: Any abstract class has both abstract as well as non-abstract methods. Interface, on the other hand, has only a single abstract method.
29. Please explain Abstract class and Abstract method.
An abstract class in Java is a class that can't be instantiated. Such a class is typically used for providing a base for subclasses to extend as well as implementing the abstract methods and overriding or using the implemented methods defined in the abstract class.
To create an abstract class, it needs to be followed by the abstract keyword. Any abstract class can have both abstract as well as non-abstract methods. A method in Java that only has the declaration and not implementation is known as an abstract method. Also, an abstract method name is followed by the abstract keyword. Any concrete subclass that extends the abstract class must provide an implementation for abstract methods.
30. What is multiple inheritance? Does Java support multiple inheritance? If not, how can it be achieved?
If a subclass or child class has two parent classes, that means it inherits the properties from two base classes; it has multiple inheritances. Java does not have multiple inheritances as in case the parent classes have the same method names. Then at runtime, it becomes ambiguous, and the compiler is unable to decide which method to execute from the child class.
Packages Java Interview Questions
31. what are packages in java state some advantages..
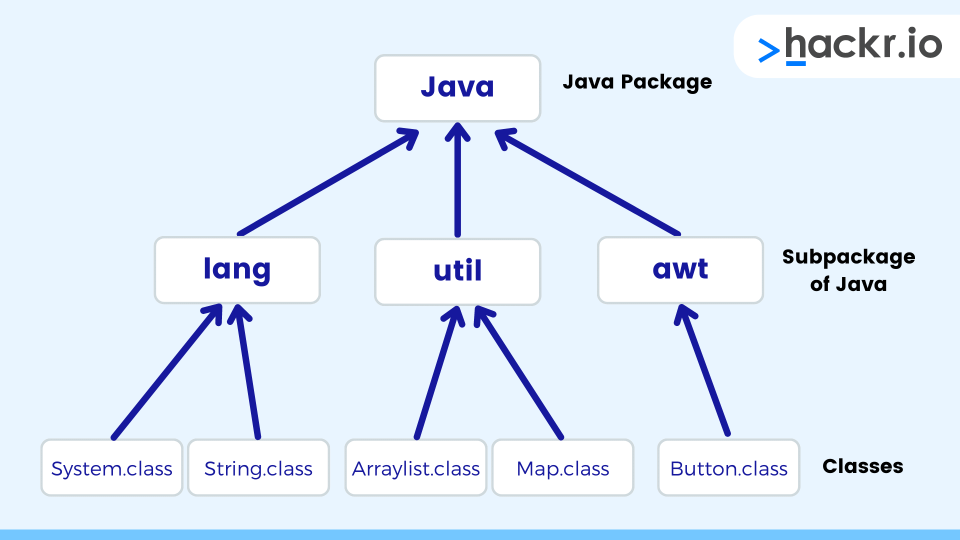
Packages are Java's way of grouping a variety of classes and/or interfaces together. The functionality of the objects decides how they are grouped. Packagers act as "containers" for classes.
Enlisted below are the advantages of Packages:
- Classes of other programs can be reused.
- Two classes with the same can exist in two different packages.
- Packages can hide classes, thus denying access to certain programs and classes meant for internal use only.
- They also separate design from coding.
Multithreading Java Interview Questions
32. how do you make a thread in java give examples..
To make a thread in Java, there are two options:
- Extend the Thread Class : The thread is available in the java.lang.Thread class. To make a thread, you need to extend a thread class and override the run method. For example,
A disadvantage of using the thread class is that it becomes impossible to extend any other classes.
Nonetheless, it is possible to overload the run() method in the class
- Implement Runnable Interface: Another way of making a thread in Java is by implementing a runnable interface. For doing so, there is the need to provide the implementation for the run() method that is defined as follows:
33. Why do we use the yield() method?
The yield() method belongs to the thread class. It transfers the currently running thread to a runnable state and also allows the other threads to execute. In other words, it gives equal priority threads a chance to run. Because yield() is a static method, it does not release any lock.
34. Explain the thread lifecycle in Java.
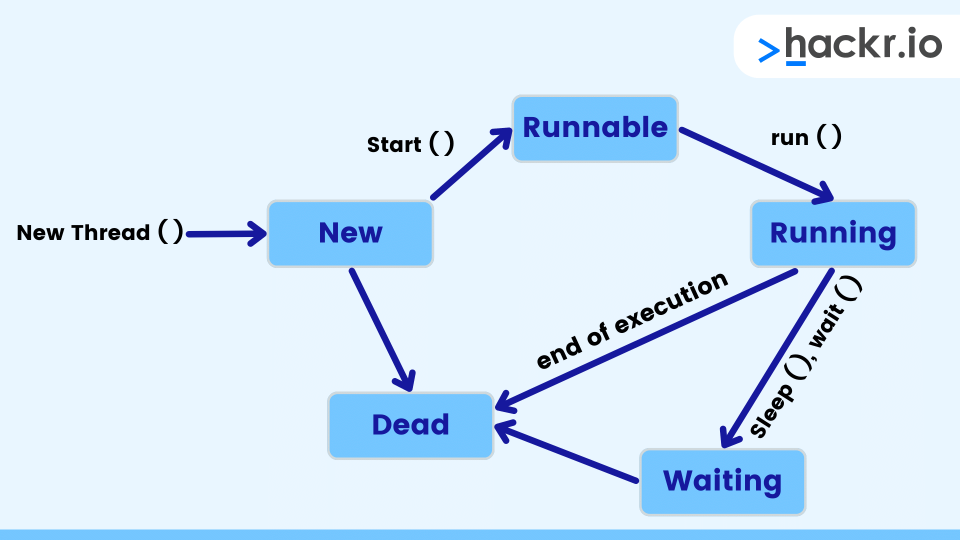
The thread lifecycle has the following states and follows the following order:
- New: In the very first state of the thread lifecycle, the thread instance is created, and the start() method is yet to be invoked. The thread is considered alive now.
- Runnable: After invoking the start() method, but before invoking the run() method, a thread is in the runnable state. A thread can also return to the runnable state from waiting or sleeping.
- Running: The thread enters the running state after the run() method is invoked. This is when the thread begins execution.
- Non-Runnable: Although the thread is alive, it is not able to run. Typically, it returns to the runnable state after some time.
- Terminated: The thread enters the terminated state once the run() method completes its execution. It is not alive now.
35. When is the Runnable interface preferred over thread class and vice-versa?
In Java, it is possible to extend only one class. Hence, the thread class is only extended when no other class needs to be extended. If it is required for a class to extend some other class than the thread class, then we need to use the Runnable interface.
36. Draw a comparison between notify() and notifyAll() methods.
The notify() method is used for sending a signal to wake up a single thread in the waiting pool. Contrarily, the notifyAll() method is used for sending a signal to wake up all threads in a waiting pool.
37. How will you distinguish processes from threads?
There are several fundamental differences between a process and a thread, stated as follows:
- Definition: A process is an executing instance of a program whereas, a thread is a subset of a process.
- Changes: A change made to the parent process doesn't affect child processes. However, a change in the main thread can yield changes in the behavior of other threads of the same process.
- Communication – While processes require inter-process communication for communicating with sibling processes, threads can directly communicate with other threads belonging to the same process.
- Control: Processes are controlled by the operating system and can control only child processes. On the contrary, threads are controlled by the programmer and are capable of exercising control over threads of the same process to which they belong.
- Dependence: Processes are independent entities while threads are dependent entities
- Memory: Threads run in shared memory spaces, but processes run in separate memory spaces.
38. What is the join() method? Give an example.
We use the join() method for joining one thread with the end of the currently running thread. It is a non-static method and has an overloaded version. Consider the example below:
The main thread starts execution in the example mentioned above. As soon as the execution reaches the code t.start(), then the thread t starts its stack for execution. The JVM switches between the main thread and the thread there. Once the execution reaches the t.join(), then the thread t alone is executed and allowed to complete its task. Afterwards, the main thread resumes execution.
39. How do you make a thread stop in Java?
There are three methods in Java to stop the execution of a thread:
- Blocking: This method is used to put the thread in a blocked state. The execution resumes as soon as the condition of the blocking is met. For instance, the ServerSocket.accept() is a blocking method that listens for incoming socket connections and resumes the blocked thread only when a connection is made.
- Sleeping: This method is used for delaying the execution of the thread for some time. A thread upon which the sleep() method is used is said to enter the sleep state. It enters the runnable state as soon as it wakes up i.e., the sleep state is finished. The time for which the thread needs to enter the sleep state is mentioned inside the braces of the sleep() method. It is a static method.
- Waiting: Although it can be called on any Java object, the wait() method can only be called from a synchronized block.
Exception Handling Java Interview Questions
40. what are the various types of exceptions how do you handle them.
Java has provision for two types of exceptions:
- Checked Exceptions: Classes that extend the Throwable class, except Runtime exception and Error, are called checked exceptions. Such exceptions are checked by the compiler during the compile time. These types of exceptions must either have appropriate try/catch blocks or be declared using the throws keyword. ClassNotFoundException is a checked exception.
- Unchecked Exceptions: Such exceptions aren't checked by the compiler during the compile time. As such, the compiler doesn't necessitate handling unchecked exceptions. Arithmetic Exception and ArrayIndexOutOfBounds Exception are unchecked exceptions.
Exceptions in Java are handled in two ways:
Declaring the throws keyword: We can declare the exception using throws keyword at the end of the method. For example:
Using try/catch: Any code segment that is expected to yield an exception is surrounded by the try block. Upon the occurrence of the exception, it is caught by the catch block that follows the try block. For example:
41. Draw the Java Exception Hierarchy.
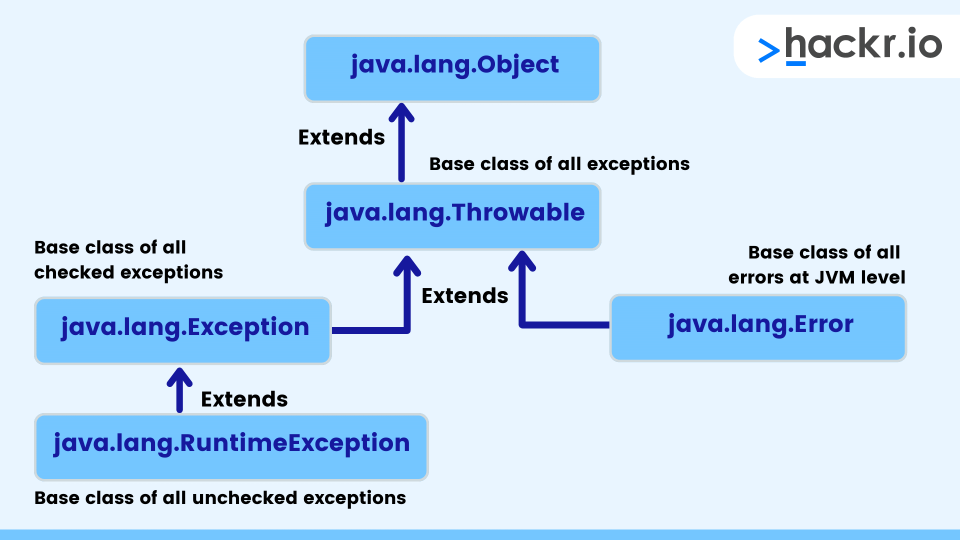
42. Is it possible to write multiple catch blocks under a single try block?
Yes, it is possible to write several catch blocks under a single try block. However, the approach needs to be from specific to general. The following example demonstrates it:
43. How does the throw keyword differ from the throws keyword?
While the throws keyword allows declaring an exception, the throw keyword is used to explicitly throw an exception.
Checked exceptions can't be propagated with throw only, but throws allow doing so without the need for anything else.
The throws keyword is followed by a class, whereas the throw keyword is followed by an instance. The throw keyword is used within the method, but the throws keyword is used with the method signature.
Furthermore, it is not possible to throw multiple exceptions, but it is possible to declare multiple exceptions.
44. Explain various exceptions handling keywords in Java.
There are two crucial exception handling keywords in Java, followed by the third keyword final, which may or may not be used after handling exceptions.
If and when a code segment has chances of having an abnormality or an error, it is placed within a try block. When the exception is raised, it is handled and caught by the catch block.
The try block must have a catch() or a final() or both blocks after it.
When an exception is raised in the try block, it is handled in the catch block.
This block is executed regardless of the exception. It can be placed either after try{} or catch {} block.
45. Explain exception propagation.
The method at the top of the stack throws an exception if it is not caught. It moves to the next method and goes on until caught.
The stack of the above code is:
If an exception occurred in the add() method is not caught, then it moves to the method addition(). It is then moved to the main() method, where the flow of execution stops. It is called Exception Propagation.
File Handling Java Interview Questions
46. is an empty file name with .java extension a valid file name.
Yes, Java permits us to save our java file by .java only. It is compiled by javac and run by the java class name. Here’s an example:
To compile: javac.java
To run: Java Any
Collections Java Interview Questions
47. what are collections what are their constituents.
A group of objects in Java is known as collections. Java.util package contains, along with date and time facilities, internationalization, legacy collection classes, etc., the various classes and interfaces for collecting. Alternatively, collections can be considered as a framework designed for storing the objects and manipulating the design in which the objects are stored. You can use collections to perform the following operations on objects:
- Manipulation
Following are the various constituents of the collections framework:
- Classes: Array List, Linked List, Lists, and Vector
- Interfaces: Collection, List, Map, Queue, Set, Sorted Map, and Sorted Set
- Maps: HashMap, HashTable, LinkedHashMap, and TreeMap
- Queues: Priority Queue
- Sets: Hash Set, Linked Hash Set, and Tree Set
48. How do you differentiate HashMap and HashTable?
HashMap in Java is a map-based collection class, used for storing key & value pairs. It is denoted as HashMap<Key, Value> or HashMap<K, V> HashTable is an array of a list, where each list is called a bucket.
Values contained in a HashTable are unique and depend on the key. Methods are not synchronized in HashMap, while key methods are synchronized in HashTable.
However, HashMap doesn't have thread safety, while HashTable has the same.
For iterating values, HashMap uses an iterator and HashTable uses an enumerator. HashTable doesn't allow anything that is null, while HashMap allows one null key and several null values.
In terms of performance, HashTable is slow. Comparatively, HashMap is faster.
49. What is a Map and what are the types?
A Java Map is an object that maps keys to values. It can't contain duplicate keys, and each key can map to only one value. In order to determine whether two keys are the same or distinct, Map makes use of the equals() method. There are 4 types of Map in Java, described as follows:
- HashMap: It is an unordered and unsorted map and hence, is a good choice when there is no emphasis on the order. A HashMap allows one null key and multiple null values and doesn't maintain any insertion order.
- HashTable: Doesn't allow anything null and has methods that are synchronized. As it allows for thread safety, the performance is slow.
- LinkedHashMap: Slower than a HashMap but maintains insertion order and has a faster iteration.
- TreeMap: A sorted Map providing support for constructing a sort order using a constructor.
50. What is a Priority Queue?
A priority queue, like a regular queue, is an abstract data type, but it has a priority associated with each element contained by it.
The element with the high priority is served before the element with low priority in a priority queue. Elements in a priority queue are ordered either according to the comparator or naturally. The order of the elements in a priority queue represents their relative priority.
51. What is a Set? Explain the types in Java Collections.
In Java, a set is a collection of unique objects. It uses the equals() method to determine whether two objects are the same or not. The various types of set in Java Collections are:
- Hash Set: An unordered and unsorted set that uses the hash code of the object for adding values. Used when the order of the collection isn't important
- Linked Hash Set: This is an ordered version of the hash set that maintains a doubly-linked list of all the elements. Used when iteration order is mandatory. Insertion order is the same as that of how elements are added to the Set.
- Tree Set: One of the two sorted collections in Java, it uses Read-Black tree structure and ensures that the elements are present in the ascending order.
52. What is ordered and sorted when it comes to collections?
- Ordered: Values are stored in a collection in a specific order, but the order is independent of the value. Example: List
- Sorted: The collection has an order which is dependent on the value of an element. Example: SortedSet
Miscellaneous Java Interview Questions
53. what are the various types of garbage collectors in java.
The Java programming language has four types of garbage collectors:
- Serial Garbage Collector: Using only a single thread for garbage collection, the serial garbage collector works by holding all the application threads. It is designed especially for single-threaded environments. Because serial garbage collector freezes all application threads while performing garbage collection, it is most suitable for command-line programs only. For using the serial garbage collector, one needs to turn on the -XX:+UseSerialGC JVM argument.
- Parallel Garbage Collector: Also known as the throughput collector, the parallel garbage collector is the default garbage collector of the JVM. It uses multiple threads for garbage collection, and like a serial garbage collector freezes all application threads during garbage collection.
- CMS Garbage Collector: Short for Concurrent Mark Sweep, CMS garbage collector uses multiple threads for scanning the heap memory for marking instances for eviction, followed by sweeping the marked instances. There are only two scenarios when the CMS garbage collector holds all the application threads:
- When marking the referenced objects in the tenured generation space.
- If there is a change in the heap memory while performing the garbage collection, CMS garbage collector ensures better application throughput over parallel garbage collectors by using more CPU resources. For using the CMS garbage collector, the XX:+USeParNewGC JVM argument needs to be turned on.
- G1 Garbage Collector: Used for large heap memory areas, G1 garbage collector works by separating the heap memory into multiple regions and then executing garbage collection in them in parallel. Unlike the CMS garbage collector that compacts the memory on STW (Stop The World) situations , G1 garbage collector compacts the free heap space right after reclaiming the memory. Also, the G1 garbage collector prioritizes the region with the most garbage. Turning on the –XX:+UseG1GC JVM argument is required for using the G1 garbage collector.
54. What do you understand by synchronization? What is its most significant disadvantage?
If several threads try to access a single block of code, then there is an increased chance of producing inaccurate results. Synchronization is used to prevent this. Using the synchronization keyword makes a thread need a key to access the synchronized code. Simply, synchronization allows only one thread to access a block of code at a time. Each Java object has a lock, and every lock has only one key. A thread can access a synchronized method only if it can get the key to the lock of the object. The following example demonstrates synchronization:
Note : It is recommended to avoid implementing synchronization for all methods. This is because when only one thread can access the synchronized code, the next thread needs to wait. Consequently, it results in slower performance of the program.
55. What is the difference between execute(), executeQuery(), and executeUpdate()?
- execute(): Used for executing an SQL query. It returns TRUE if the result is a ResultSet, like running Select queries, and FALSE if the result is not a ResultSet, such as running an Insert or an Update query.
- executeQuery(): Used for executing Select queries. It returns the ResultSet, which is not null, even if no records are matching the query. The executeQuery() method must be used when executing select queries so that it throws the java.sql.SQLException with the 'executeQuery method cannot be used for update' message when someone tries to execute an Insert or Update statement.
- executeUpdate(): Used for executing Delete/Insert/Update statements or DDL statements that return nothing. The output varies depending on whether the statements are Data Manipulation Language (DML) statements or Data Definition Language (DDL) statements. The output is an integer and equals the total row count for the former case, and 0 for the latter case.
Note : The execute() method needs to be used only in a scenario when there is no certainty about the type of statement. In all other cases, either use executeQuery() or executeUpdate() method.
56. Provide an example of the Hibernate architecture.
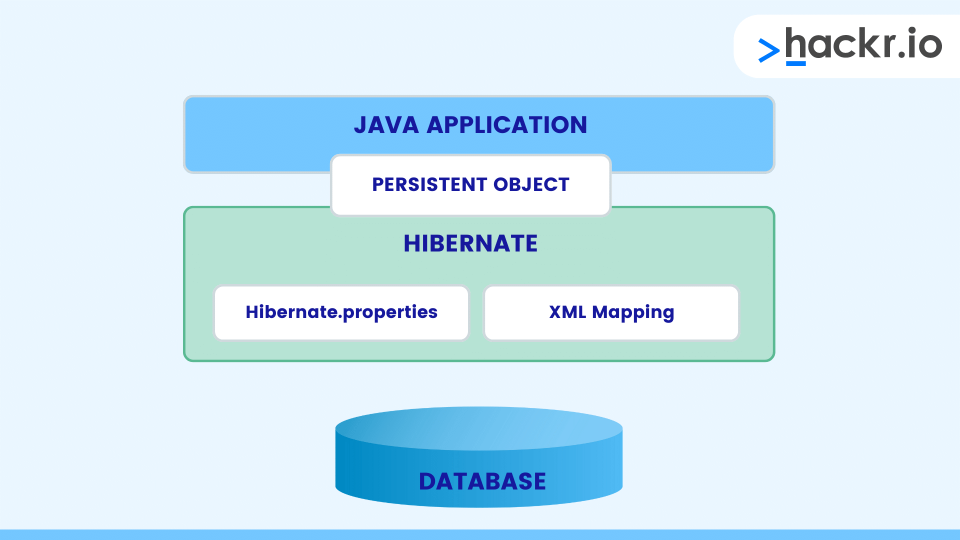
57. Could you demonstrate how to delete a cookie in JSP with a code example?
The following code demonstrates deleting a cookie in JSP:
58. Write suitable code examples to demonstrate the use of final, final, and finalize.
Final: The final keyword is used for restricting a class, method, and variable. A final class can't be inherited, a final method is disabled from overriding, and a final variable becomes a constant i.e., its value can't be changed.
Finally: Any code inside the final block will be executed, irrespective of whether an exception is handled or not.
Finalize: The finalize method performs the clean up just before the object is garbage collected.
59. What purpose does the Volatile variable serve?
The value stored in a volatile variable is not read from the thread's cache memory but from the main memory. Volatile variables are primarily used during synchronization.
60. Please compare serialization and deserialization.
Serialization is the process by which Java objects are converted into the byte stream.
Deserialization is the exact opposite process of serialization where Java objects are retrieved from the byte stream.
A Java object is serialized by writing it to an ObjectOutputStream and deserialized by reading it from an ObjectInputStream.
61. What is OutOfMemoryError?
Typically, the OutOfMemoryError exception is thrown when the JVM is not able to allocate an object due to running out of memory. In such a situation, no memory could be reclaimed by the garbage collector.
There can be several reasons that result in the OutOfMemoryError exception, of which the most notable ones are:
- Holding objects for too long
- Trying to process too much data at the same time
- Using a third-party library that caches strings
- Using an application server that doesn't perform a memory cleanup post the deployment
- When a native allocation can't be satisfied
62. Explain public static void main(String args[ ]) in Java
The execution Java program starts with public static void main(String args[ ]), also called the main() method.
- public: It is an access modifier defining the accessibility of the class or method. Any class can access the main() method defined public in the program.
- static: The keyword indicates the variable, or the method is a class method. The method main() is made static so that it can be accessed without creating the instance of the class. When the method main() is not made static, the compiler throws an error because the main() is called by the JVM before any objects are made, and only static methods can be directly invoked via the class.
- void: It is the return type of the method. Void defines the method that does not return any type of value.
- main: JVM searches this method when starting the execution of any program, with the particular signature only.
- String args[]: The parameter passed to the main method.
Java Programming Masterclass Updated to Java 17
63. What are wrapper classes in Java?
Wrapper classes are responsible for converting the Java primitives into the reference types (objects). A class is dedicated to every primitive data type. They are known as wrapper classes because they wrap the primitive data type into an object of that class. It is present in the Java.lang package. The table below displays the different primitive types and wrapper classes.
|
|
boolean | Boolean |
char | Character |
double | Double |
float | Float |
int | Integer |
long | Long |
64. Explain the concept of boxing, unboxing, autoboxing, and auto unboxing.
- Boxing: The concept of putting a primitive value inside an object is called boxing.
- Unboxing: Getting the primitive value from the object.
- Autoboxing: Assigning a value directly to an integer object.
- Auto unboxing: Getting the primitive value directly into the integer object.
65. Define the Singleton class. How can a class be made Singleton?
A Singleton class allows only one instance of the class to be created. A class can be made singleton with the following steps:
- Creating a static instance of the class with the class.
- By not allowing the user to create an instance with default constructor by defining a private constructor.
- Create a static method to return the object of an instance of A.
66. What if the public static void is replaced by static public void, will the program still run?
Yes, the program would compile and run without any errors as the order of the specifiers doesn't matter.
67. What is the difference between == and equals()?
|
|
It is a method of String class | It is an operator |
Content comparison | Address comparison |
68. Why don't we use pointers in Java?
Pointers are considered to be unsafe, and increase the complexity of the program, adding the concept of pointers can be contradicting. Also, JVM is responsible for implicit memory allocation; thus, to avoid direct access to memory by the user, pointers are discouraged in Java.
69. What is the difference between this() and super()?
|
|
Represents the current instance of the class | Represents the current instance of the parent/base class |
It is used to call the default constructor of the same class | It is used to call the default constructor of the parent/base class. |
Accesses method of the current class | Accesses method of the base class |
Points current class instance | Points to the superclass instance. |
Must be the first line of the block | It must be the first line of the block. |
Java Coding Interview Questions
Apart from having good knowledge about concepts of Java programming, you are also tested for your skills in coding in Java programming language. The following are Java coding interview questions that are relevant for freshers and are quite popular amongst Java programming interviews.
70. Take a look at the two code snippets below. What is the important difference between the two?
Code snippet i. is an example of method overloading while the code snippet ii. demonstrates method overriding.
71. Write a program for string reversal without using an inbuilt function.
72. write a program to delete duplicates from an array., 73. write a program to reverse a number., 74. write a program that implements binary search., 75. write a program to check if a number is prime., 76. write a program to print fibonacci series., 77. write a program to check if the given string is a palindrome., 78. write a program to print the following pattern., 79. write a program to swap two numbers., 80. write a program to check if the given number is an armstrong number..
These core Java Interview Questions and Java Programming Interview Questions are a great way to prepare you for the interview. Review the answers, including the coding examples, and put your best foot forward.
Please note that we also provided a PDF for your preparation so that you can download and learn and prepare on the go:
Download Java Interview Questions PDF
Prefer on-demand videos? Check out this course for further reading and preparation for a Java-based interview: Java Interview Guides: 200+ Interview Question and Answer
Otherwise, we recommend this book to help you succeed in your future Java interviews: Elements of Programming Interviews in Java: The insider guide second edition
- Frequently Asked Questions
1. What are the basic Java questions asked in an interview?
There are several basic Java interview questions that can appear in an interview. Look at the ones we’ve listed above to get a sense of them.
2. How should I prepare for a Java interview?
You should prepare for a Java interview by learning both the theory and practicing coding. There are several related questions above.
3. What are the advanced Java interview questions?
Advanced Java interview questions can be of many kinds, including both theory and coding-based questions. Check out the list of Java programming questions above to see what they are like.
People are also reading:
- Best Java Courses
- Top 10 Java Certifications
- Best Java Books
- Best Java Projects
- Programming Interview Questions
- Core Java Cheatsheet - Introduction to Programming in Java
- Difference between Java vs Javascript
- Top 10 Java Frameworks
- Best Way to Learn Java
- Constructor in Java
- Prime Number Program in Java
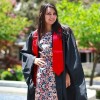
Simran works at Hackr as a technical writer. The graduate in MS Computer Science from the well known CS hub, aka Silicon Valley, is also an editor of the website. She enjoys writing about any tech topic, including programming, algorithms, cloud, data science, and AI. Traveling, sketching, and gardening are the hobbies that interest her.
Subscribe to our Newsletter for Articles, News, & Jobs.
Disclosure: Hackr.io is supported by its audience. When you purchase through links on our site, we may earn an affiliate commission.
In this article
- Top 48 Networking Interview Questions and Answers in 2024 Computer Networks Career Development Interview Questions
- Top 20 REST API Interview Questions & Answers [2024] Web Development Career Development Interview Questions
- How to Extract a Java Substring [with Code Examples] Java
Please login to leave comments
Always be in the loop.
Get news once a week, and don't worry — no spam.
{{ errors }}
{{ message }}
- Help center
- We ❤️ Feedback
- Advertise / Partner
- Write for us
- Privacy Policy
- Cookie Policy
- Change Privacy Settings
- Disclosure Policy
- Terms and Conditions
- Refund Policy
Disclosure: This page may contain affliate links, meaning when you click the links and make a purchase, we receive a commission.
Top 20 Java coding interview questions for 2024
Become a Software Engineer in Months, Not Years
From your first line of code, to your first day on the job — Educative has you covered. Join 2M+ developers learning in-demand programming skills.
Coding interviews are aimed at gauging how well-prepared a candidate is in terms of language proficiency, foundational knowledge, problem-solving skills, and soft skills. For a position that explicitly mentions a language, such as a job listing for a Java developer, it makes even more sense to spend some time and energy polishing Java skills by exploring the common interview questions asked in such interviews. Doing this not only allows us to benefit directly from the experience of others but more importantly, it’s an opportunity to learn things that we are not aware are gaps in our knowledge.
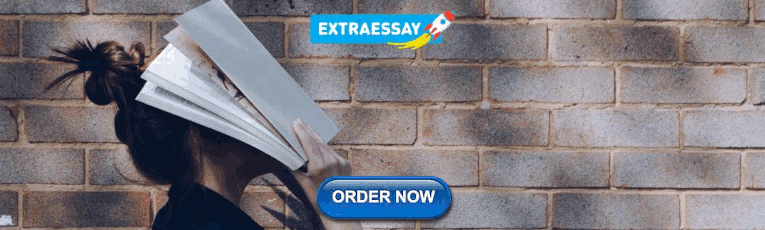
Java interview questions
In this blog, we cover 20 Java language-specific interview questions on a variety of topics. We’re also including answers to these questions to help you prepare for them. For more interview questions, check out the links at the end of this blog.
11. What’s a Java lambda expression?
Lambda expressions , introduced in Java 8, are objects that implement a functional interface. The syntax of a lambda specifies the definition of the sole abstract method of a functional interface.
This syntax consists of three parts:
- The first is a list of arguments enclosed in parentheses. The parentheses can be omitted if only a single argument is present.
- The list of arguments is followed by the arrow operator -> .
- The arrow operator -> is followed by a block of code included within braces {} . The braces can be omitted in case the block consists of just a single expression corresponding to the value being returned.
For example, the following lambda takes two numeric arguments and returns the sum of these.
Note: The types of variables in the lambda can be specified explicitly but can also be inferred from the surrounding context.
The following code shows two different ways to write the same lambda expression. The lambda expressions on line 5 and lines 7–10 take no argument. They simply print a message and return nothing. Note that the right-hand sides give an implementation of the run method of the Runnable interface. The objects created on line 5 and lines 7–10 therefore implement the Runnable interface and can be used to create threads on line 12 and line 14 .
12. How is the forEach loop useful?
The forEach loop can be used to iterate over a collection. Its argument must be an instance that’s an implementation of the Consumer functional interface. A lambda argument passed as an argument to the loop is applied to each element of the collection. For instance, in the following code on line 11 , the lambda expression is applied to each element, i , in a list. The input i is consumed, and nothing is returned.
A forEach loop can also be passed a method reference which specifies the function that can be applied to each element of the iterable on which it is invoked. For instance, the following line of code prints each element in the list:
13. What type of variables must be final or effectively final when used in a lambda?
There are three types of variables in Java.
- Instance variables: These belong to individual class instances.
- Static variables: These belong to the class.
- Local variables: These are declared in methods or blocks of code.
All three types of variables, when in scope, can be used in a lambda expression. However, the local variables can be used only if they are either explicitly declared final or they undergo no change after their first assignment. So, for all practical purposes, they are treated by the compiler as effectively final. The lambda expression on lines 10–19 below use variables of all three kinds, but if we comment out line 22 the local variable does not remain effectively final, resulting in a compiler error.
14. How can we clone an object in Java?
Cloning an object means making a copy of that object. The Object class defines a method clone() that is inherited by all other classes since the Object class is at the top of the inheritance hierarchy for Java. However, clone() defined for the Object class only makes shallow copies and must be overridden if deep copies are required.
A class whose instances are required to be cloned must implement the Cloneable interface, otherwise, calling clone() on such instances will throw the CloneNotSupported exception.
In the following code, we show two classes Whole and Part that implement the Cloneable interface. In the Part class, we just call the clone() function of the superclass (see line 12 ). On the other hand, the class Whole contains a data member of type Part . If we were to use the clone() method of the superclass, it would make a copy of the address stored in the reference variable part instead of making its deep copy. So we explicitly make a deep copy of part on line 34 .
15. What’s an inner class, and why is it used?
A nested Java class that’s not static is called an inner class . An instance of an outer class must be created first in order to create an instance of an inner class, as shown on lines 34–35 in the widget below.
Inner classes are useful for organizing code with the aim of ensuring encapsulation.
In the code above, we use the public access modifier with the inner class. But just like for other data members, we could have also used the private or protected access modifiers with the inner class.
16. What’s an anonymous class?
An anonymous class is a nested class that has no name and is defined and instantiated all at once. For this reason, an anonymous class can be instantiated only once. Note that an anonymous class is used to either extend another class or implement an interface. To create an anonymous class instance, the new operator is applied to the name of its superclass or the name of the interface being implemented, as shown on lines 11–23 in the widget below.
17. What’s the difference between an iterable and an iterator?
An iterable is an object that implements the Iterable interface. All Java collections implement this interface. This enables us to iterate over these collections using an iterator returned by the iterator() method, as well as by using the forEach() method.
An iterator , on the other hand, is an object that implements the Iterator interface. It supports the hasNext() method which can be used for checking if there is a non-null element at the next position in the collection. If the element exists, it can be retrieved using the next() method. It also supports the remove() method, which can be used for safely removing an element from the iterable during the iteration. The element removed is the same as the one returned by the previous call to next() . The following code shows how these methods can be used on lines 15–18 .
Note that when an iterator is in use, direct additions or removals from a collection can result in a ConcurrentModificationException exception being thrown. The iterators that implement this behavior are called fail-fast iterators .
18. What’s the difference between a HashMap and a TreeMap ?
Both are Java collections used for storing key-value pairs. Internally, the HashMap class is implemented like a hash table, but the TreeMap class is implemented as a red-black tree, which is a balanced binary search tree. So retrieval, addition, and checking for containment of an element is O ( 1 ) O(1) O ( 1 ) for HashMap vs. O ( log n ) O(\log n) O ( lo g n ) for TreeMap . Searching is asymptotically the same at O ( log n ) O(\log n) O ( lo g n ) for both.
On the other hand, a tree map has a distinct advantage over a hash map in that the keys stored in it are ordered, and if an application has a need for ordering data, then it’s a better fit. The order in which the keys are stored is said to be the natural order and is specified by the implementation of compareTo in the Comparable interface. By default, the numeric keys in a tree map are stored under the usual ordering on the set of real numbers. Keys that are strings are ordered alphabetically.
The following example shows a few method calls that retrieve ordered data from a tree map.
19. What purpose does the synchronized keyword serve?
The synchronized keyword is applied to code blocks or methods to ensure that each such code block can be executed by only one thread at a time. Each code block is synchronized over an object shown as follows:
The synchronized keyword can also be applied directly to a method name. In such a case, the method is synchronized on the this object. For instance, in the following code, the increment() method defined on lines 6–16 is synchronized on the this object.
A thread that enters the code of one of the synchronized methods has the lock over the this object, and all other threads are blocked from entering and executing any method or code block synchronized on the same object.
Once the thread holding the lock exits the code block, the lock over the object is released.
If we remove the synchronized keyword from line 6 , we note that in one execution (see below), the first thread prints 0 Ping , then the second thread prints 0 Pong , followed by the first thread printing 2 Ping . The fact that 0 was printed twice and 1 was never printed indicates that the threads were switched while increment() was still being executed.
Learn in-demand tech skills in half the time
Mock Interview
Skill Paths
Assessments
Learn to Code
Tech Interview Prep
Generative AI
Data Science
Machine Learning
GitHub Students Scholarship
Early Access Courses
For Individuals
Try for Free
Gift a Subscription
Become an Author
Become an Affiliate
Earn Referral Credits
Cheatsheets
Frequently Asked Questions
Privacy Policy
Cookie Policy
Terms of Service
Business Terms of Service
Data Processing Agreement
Copyright © 2024 Educative, Inc. All rights reserved.
Learn Java and Programming through articles, code examples, and tutorials for developers of all levels.
- online courses
- certification
- free resources
Top 53 Java Programs for Coding and Programming Interviews
50+ java coding problems from programming job interviews.
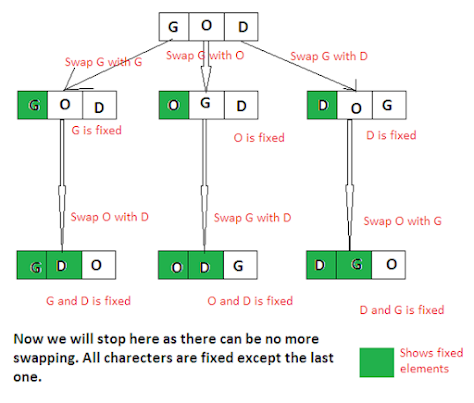
- 10 Courses to Prepare for Programming Interviews
- 10 Books to Prepare Technical Programming/Coding Job Interviews
- 20+ String Algorithms Interview Questions
- 25 Software Design Interview Questions for Programmers
- 20+ array-based Problems for interviews
- 40 Binary Tree Interview Questions for Java Programmers
- 10 Dynamic Programming Questions from Coding Interviews
- 25 Recursion Programs from Coding Interviews
- Review these Java Interview Questions for Programmers
- 7 Best Courses to learn Data Structure and Algorithms
- 10 OOP Design Interview Questions with solution
- Top 30 Object-Oriented Programming Questions
- Top 5 Courses to learn Dynamic Programming for Interviews
- 10 Algorithm Books Every Programmer Should Read
- Top 5 Data Structure and Algorithm Books for Java Developers
- 100+ Data Structure and Algorithm Questions with Solution
- 75+ Coding Interview Questions for 2 to 5 years experience
- 10 Programming and Coding Job interview courses for programmers
good questions, thanks
Feel free to comment, ask questions if you have any doubt.
Java 8 Interview Questions(+ Answers)
Last updated: January 11, 2024
- >= Java 8

Now that the new version of REST With Spring - “REST With Spring Boot” is finally out, the current price will be available until the 22nd of June , after which it will permanently increase by 50$
>> GET ACCESS NOW
Azure Spring Apps is a fully managed service from Microsoft (built in collaboration with VMware), focused on building and deploying Spring Boot applications on Azure Cloud without worrying about Kubernetes.
The Enterprise plan comes with some interesting features, such as commercial Spring runtime support, a 99.95% SLA and some deep discounts (up to 47%) when you are ready for production.
>> Learn more and deploy your first Spring Boot app to Azure.
And, you can participate in a very quick (1 minute) paid user research from the Java on Azure product team.
Slow MySQL query performance is all too common. Of course it is. A good way to go is, naturally, a dedicated profiler that actually understands the ins and outs of MySQL.
The Jet Profiler was built for MySQL only , so it can do things like real-time query performance, focus on most used tables or most frequent queries, quickly identify performance issues and basically help you optimize your queries.
Critically, it has very minimal impact on your server's performance, with most of the profiling work done separately - so it needs no server changes, agents or separate services.
Basically, you install the desktop application, connect to your MySQL server , hit the record button, and you'll have results within minutes:
>> Try out the Profiler
A quick guide to materially improve your tests with Junit 5:
Do JSON right with Jackson
Download the E-book
Get the most out of the Apache HTTP Client
Get Started with Apache Maven:
Get started with Spring and Spring Boot, through the reference Learn Spring course:
>> LEARN SPRING
Building a REST API with Spring?
The AI Assistant to boost Boost your productivity writing unit tests - Machinet AI .
AI is all the rage these days, but for very good reason. The highly practical coding companion, you'll get the power of AI-assisted coding and automated unit test generation . Machinet's Unit Test AI Agent utilizes your own project context to create meaningful unit tests that intelligently aligns with the behavior of the code. And, the AI Chat crafts code and fixes errors with ease, like a helpful sidekick.
Simplify Your Coding Journey with Machinet AI :
>> Install Machinet AI in your IntelliJ
Get non-trivial analysis (and trivial, too!) suggested right inside your IDE or Git platform so you can code smart, create more value, and stay confident when you push.
Get CodiumAI for free and become part of a community of over 280,000 developers who are already experiencing improved and quicker coding.
Write code that works the way you meant it to:
>> CodiumAI. Meaningful Code Tests for Busy Devs
Looking for the ideal Linux distro for running modern Spring apps in the cloud?
Meet Alpaquita Linux : lightweight, secure, and powerful enough to handle heavy workloads.
This distro is specifically designed for running Java apps . It builds upon Alpine and features significant enhancements to excel in high-density container environments while meeting enterprise-grade security standards.
Specifically, the container image size is ~30% smaller than standard options, and it consumes up to 30% less RAM:
>> Try Alpaquita Containers now.
Yes, Spring Security can be complex, from the more advanced functionality within the Core to the deep OAuth support in the framework.
I built the security material as two full courses - Core and OAuth , to get practical with these more complex scenarios. We explore when and how to use each feature and code through it on the backing project .
You can explore the course here:
>> Learn Spring Security
DbSchema is a super-flexible database designer, which can take you from designing the DB with your team all the way to safely deploying the schema .
The way it does all of that is by using a design model , a database-independent image of the schema, which can be shared in a team using GIT and compared or deployed on to any database.
And, of course, it can be heavily visual, allowing you to interact with the database using diagrams, visually compose queries, explore the data, generate random data, import data or build HTML5 database reports.
>> Take a look at DBSchema
Creating PDFs is actually surprisingly hard. When we first tried, none of the existing PDF libraries met our needs. So we made DocRaptor for ourselves and later launched it as one of the first HTML-to-PDF APIs.
We think DocRaptor is the fastest and most scalable way to make PDFs , especially high-quality or complex PDFs. And as developers ourselves, we love good documentation, no-account trial keys, and an easy setup process.
>> Try DocRaptor's HTML-to-PDF Java Client (No Signup Required)
1. Introduction
In this tutorial, we’re going to explore some of the JDK8-related questions that might pop up during an interview.
Java 8 is a platform release packed with new language features and library classes. Most of these new features are geared towards achieving cleaner and more compact code, while some add new functionality that has never before been supported in Java.
Further reading:
Memory management in java interview questions (+answers), java collections interview questions, 2. java 8 general knowledge, q1. what new features were added in java 8.
Java 8 ships with several new features, but the most significant are the following:
- Lambda Expressions − a new language feature allowing us to treat actions as objects
- Method References − enable us to define Lambda Expressions by referring to methods directly using their names
- Optional − special wrapper class used for expressing optionality
- Functional Interface – an interface with maximum one abstract method; implementation can be provided using a Lambda Expression
- Default methods − give us the ability to add full implementations in interfaces besides abstract methods
- Nashorn, JavaScript Engine − Java-based engine for executing and evaluating JavaScript code
- Stream API − a special iterator class that allows us to process collections of objects in a functional manner
- Date API − an improved, immutable JodaTime-inspired Date API
Along with these new features, lots of feature enhancements are done under the hood at both the compiler and JVM level.
3. Method References
Q1. what is a method reference.
A method reference is a Java 8 construct that can be used for referencing a method without invoking it. It’s used for treating methods as Lambda Expressions. They only work as syntactic sugar to reduce the verbosity of some lambdas. This way the following code:
Can become:
A method reference can be identified by a double colon separating a class or object name, and the name of the method. It has different variations, such as constructor reference:
Static method reference:
Bound instance method reference:
Unbound instance method reference:
We can read a detailed description of method references with full examples by following this link and this one .
Q2. What Is the Meaning of String::Valueof Expression?
It’s a static method reference to the valueOf method of the String class.
4. Optional
Q1. what is optional how can it be used.
Optional is a new class in Java 8 that encapsulates an optional value, i.e. a value that is either there or not. It’s a wrapper around an object, and we can think of it as a container of zero or one element.
Optional has a special Optional.empty() value instead of wrapped null . Thus it can be used instead of a nullable value to get rid of NullPointerException in many cases.
We can read a dedicated article about Optional here .
The main purpose of Optional , as designed by its creators, is to be a return type of methods that previously would return null . Such methods would require us to write boilerplate code to check the return value, and we could sometimes forget to do a defensive check. In Java 8, an Optional return type explicitly requires us to handle null or non-null wrapped values differently.
For instance, the Stream.min() method calculates the minimum value in a stream of values. But what if the stream is empty? If it wasn’t for Optional , the method would return null or throw an exception.
However, it returns an Optional value, which may be Optional.empty() (the second case). This allows us to easily handle such cases:
It’s worth noting that Optional is not a general purpose class like Option in Scala. It’s not recommended that we use it as a field value in entity classes, which is clearly indicated by it not implementing the Serializable interface.
5. Functional Interfaces
Q1. describe some of the functional interfaces in the standard library.
There are a lot of functional interfaces in the java.util.function package. The more common ones include, but are not limited to:
- Function – it takes one argument and returns a result
- Consumer – it takes one argument and returns no result (represents a side effect)
- Supplier – it takes no arguments and returns a result
- Predicate – it takes one argument and returns a boolean
- BiFunction – it takes two arguments and returns a result
- BinaryOperator – it is similar to a BiFunction , taking two arguments and returning a result. The two arguments and the result are all of the same types.
- UnaryOperator – it is similar to a Function , taking a single argument and returning a result of the same type
For more on functional interfaces, see the article “Functional Interfaces in Java 8.”
Q2. What Is a Functional Interface? What Are the Rules of Defining a Functional Interface?
A functional interface is an interface with one single abstract method ( default methods do not count), no more, no less.
Where an instance of such an interface is required, a Lambda Expression can be used instead. More formally put: Functional interfaces provide target types for lambda expressions and method references.
The arguments and return type of such an expression directly match those of the single abstract method.
For instance, the Runnable interface is a functional interface, so instead of:
We could simply do:
Functional interfaces are usually annotated with the @FunctionalInterface annotation, which is informative and doesn’t affect the semantics.
6. Default Method
Q1. what is a default method and when do we use it.
A default method is a method with an implementation, which can be found in an interface.
We can use a default method to add a new functionality to an interface, while maintaining backward compatibility with classes that are already implementing the interface:
Usually when we add a new abstract method to an interface, all implementing classes will break until they implement the new abstract method. In Java 8, this problem was solved by using the default method.
For example, the Collection interface does not have a forEach method declaration. Thus adding such a method would simply break the whole collections API.
Java 8 introduced the default method so that the Collection interface can have a default implementation of the forEach method without requiring the classes implementing this interface to implement the same.
Q2. Will the Following Code Compile?
Yes, the code will compile because it follows the functional interface specification of defining only a single abstract method. The second method, count , is a default method that does not increase the abstract method count.
7. Lambda Expressions
Q1. what is a lambda expression and what is it used for.
In very simple terms, a lambda expression is a function that we can reference and pass around as an object.
Moreover, lambda expressions introduce functional style processing in Java, and facilitate the writing of compact and easy-to-read code.
As a result, lambda expressions are a natural replacement for anonymous classes such as method arguments. One of their main uses is to define inline implementations of functional interfaces.
Q2. Explain the Syntax and Characteristics of a Lambda Expression
A lambda expression consists of two parts, the parameter part and the expressions part separated by a forward arrow:
Any lambda expression has the following characteristics:
- Optional type declaration – when declaring the parameters on the left-hand side of the lambda, we don’t need to declare their types as the compiler can infer them from their values. So int param -> … and param ->… are all valid
- Optional parentheses – when only a single parameter is declared, we don’t need to place it in parentheses. This means param -> … and (param) -> … are all valid, but when more than one parameter is declared, parentheses are required
- Optional curly braces – when the expressions part only has a single statement, there is no need for curly braces. This means that param – > statement and param – > {statement;} are all valid, but curly braces are required when there is more than one statement
- Optional return statement – when the expression returns a value and it is wrapped inside curly braces, then we don’t need a return statement. That means (a, b) – > {return a+b;} and (a, b) – > {a+b;} are both valid
To read more about Lambda expressions, follow this link and this one .
8. Nashorn Javascript
Q1. what is nashorn in java8.
Nashorn is the new Javascript processing engine for the Java platform that shipped with Java 8. Until JDK 7, the Java platform used Mozilla Rhino for the same purpose, as a Javascript processing engine.
Nashorn provides better compliance with the ECMA normalized JavaScript specification and better runtime performance than its predecessor.
Q2. What Is JJS?
In Java 8, jjs is the new executable or command line tool we use to execute Javascript code at the console.
Q1. What Is a Stream? How Does It Differ From a Collection?
In simple terms, a stream is an iterator whose role is to accept a set of actions to apply on each of the elements it contains.
The stream represents a sequence of objects from a source such as a collection, which supports aggregate operations. They were designed to make collection processing simple and concise. Contrary to the collections, the logic of iteration is implemented inside the stream, so we can use methods like map and flatMap for performing a declarative processing.
Additionally, the Stream API is fluent and allows pipelining:
Another important distinction from collections is that streams are inherently lazily loaded and processed.
Q2. What Is the Difference Between Intermediate and Terminal Operations?
We combine stream operations into pipelines to process streams. All operations are either intermediate or terminal.
Intermediate operations are those operations that return Stream itself, allowing for further operations on a stream.
These operations are always lazy, i.e. they do not process the stream at the call site. An intermediate operation can only process data when there is a terminal operation. Some of the intermediate operations are filter , map and flatMap .
In contrast, terminal operations terminate the pipeline and initiate stream processing. The stream is passed through all intermediate operations during terminal operation call. Terminal operations include forEach , reduce, Collect and sum .
To drive this point home, let’s look at an example with side effects:
The output will be as follows:
As we can see, the intermediate operations are only triggered when a terminal operation exists.
Q3. What Is the Difference Between Map and flatMap Stream Operation?
There is a difference in signature between map and flatMap . Generally speaking, a map operation wraps its return value inside its ordinal type, while flatMap does not.
For example, in Optional , a map operation would return Optional<String> type, while flatMap would return String type.
So after mapping, we need to unwrap (read “flatten”) the object to retrieve the value, whereas after flat mapping, there is no such need as the object is already flattened. We apply the same concept to mapping and flat mapping in Stream .
Both map and flatMap are intermediate stream operations that receive a function and apply this function to all the elements of a stream.
The difference is that for the map , this function returns a value, but for flatMap , this function returns a stream. The flatMap operation “flattens” the streams into one.
Here’s an example where we take a map of users’ names and lists of phones and “flatten” it down to a list of phones of all the users using flatMap :
Q4. What Is Stream Pipelining in Java 8?
Stream pipelining is the concept of chaining operations together. We do this by splitting the operations that can happen on a stream into two categories: intermediate operations and terminal operations.
Each intermediate operation returns an instance of Stream itself when it runs. Therefore, we can set up an arbitrary number of intermediate operations to process data, forming a processing pipeline.
There must then be a terminal operation which returns a final value and terminates the pipeline.
10. Java 8 Date and Time API
Q1. tell us about the new date and time api in java 8.
A long-standing problem for Java developers has been the inadequate support for the date and time manipulations required by ordinary developers.
The existing classes such as java.util.Date and SimpleDateFormatter aren’t thread-safe, leading to potential concurrency issues for users.
Poor API design is also a reality in the old Java Data API. Here’s just a quick example: years in java.util.Date start at 1900, months start at 1, and days start at 0, which is not very intuitive.
These issues and several others have led to the popularity of third-party date and time libraries, such as Joda-Time.
In order to address these problems and provide better support in JDK, a new date and time API, which is free of these problems, has been designed for Java SE 8 under the package java.time .
11. Conclusion
In this article, we explored several important technical interview questions with a bias on Java 8. This is by no means an exhaustive list, but it contains questions that we think are most likely to appear in each new feature of Java 8.
Even if we’re just starting out, ignorance of Java 8 isn’t a good way to go into an interview, especially when Java appears strongly on a resume. It is therefore important that we take some time to understand the answers to these questions and possibly do more research.
Good luck in the interview.
Slow MySQL query performance is all too common. Of course it is.
The Jet Profiler was built entirely for MySQL , so it's fine-tuned for it and does advanced everything with relaly minimal impact and no server changes.
Explore the secure, reliable, and high-performance Test Execution Cloud built for scale. Right in your IDE:
Basically, write code that works the way you meant it to.
AI is all the rage these days, but for very good reason. The highly practical coding companion, you'll get the power of AI-assisted coding and automated unit test generation . Machinet's Unit Test AI Agent utilizes your own project context to create meaningful unit tests that intelligently aligns with the behavior of the code.
Get started with Spring Boot and with core Spring, through the Learn Spring course:
>> CHECK OUT THE COURSE
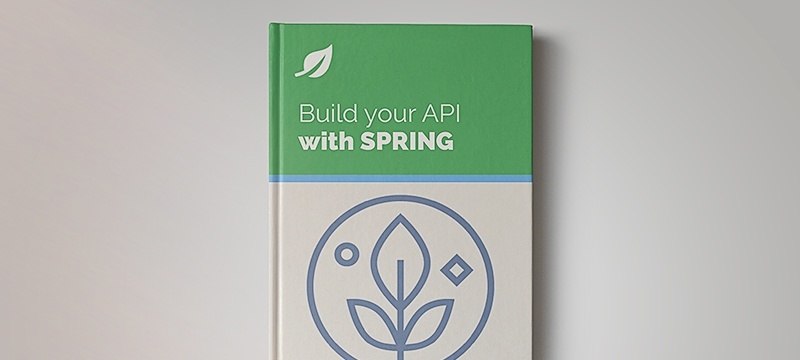
Follow the Java Category
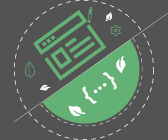
Java Coding Practice
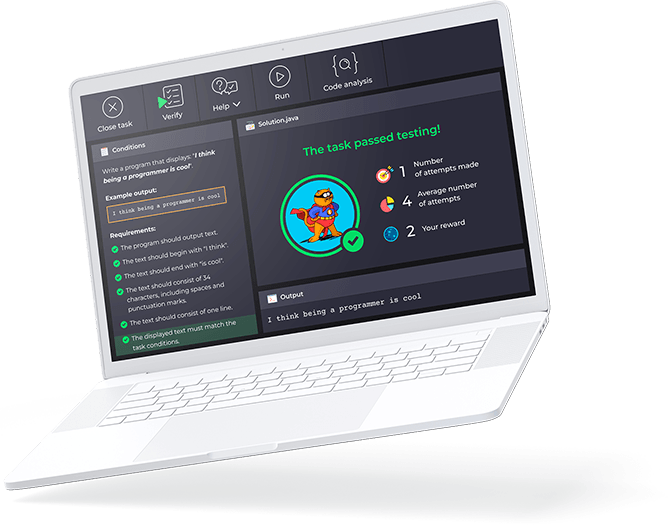
What kind of Java practice exercises are there?
How to solve these java coding challenges, why codegym is the best platform for your java code practice.
- Tons of versatile Java coding tasks for learners with any background: from Java Syntax and Core Java topics to Multithreading and Java Collections
- The support from the CodeGym team and the global community of learners (“Help” section, programming forum, and chat)
- The modern tool for coding practice: with an automatic check of your solutions, hints on resolving the tasks, and advice on how to improve your coding style
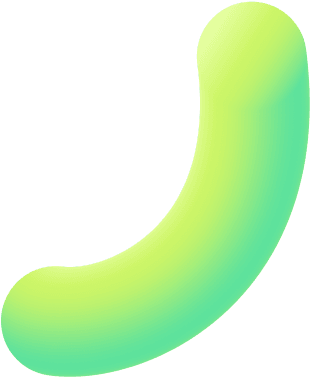
Click on any topic to practice Java online right away
Practice java code online with codegym.
In Java programming, commands are essential instructions that tell the computer what to do. These commands are written in a specific way so the computer can understand and execute them. Every program in Java is a set of commands. At the beginning of your Java programming practice , it’s good to know a few basic principles:
- In Java, each command ends with a semicolon;
- A command can't exist on its own: it’s a part of a method, and method is part of a class;
- Method (procedure, function) is a sequence of commands. Methods define the behavior of an object.
Here is an example of the command:
The command System.out.println("Hello, World!"); tells the computer to display the text inside the quotation marks.
If you want to display a number and not text, then you do not need to put quotation marks. You can simply write the number. Or an arithmetic operation. For example:
Command to display the number 1.
A command in which two numbers are summed and their sum (10) is displayed.
As we discussed in the basic rules, a command cannot exist on its own in Java. It must be within a method, and a method must be within a class. Here is the simplest program that prints the string "Hello, World!".
We have a class called HelloWorld , a method called main() , and the command System.out.println("Hello, World!") . You may not understand everything in the code yet, but that's okay! You'll learn more about it later. The good news is that you can already write your first program with the knowledge you've gained.
Attention! You can add comments in your code. Comments in Java are lines of code that are ignored by the compiler, but you can mark with them your code to make it clear for you and other programmers.
Single-line comments start with two forward slashes (//) and end at the end of the line. In example above we have a comment //here we print the text out
You can read the theory on this topic here , here , and here . But try practicing first!
Explore the Java coding exercises for practicing with commands below. First, read the conditions, scroll down to the Solution box, and type your solution. Then, click Verify (above the Conditions box) to check the correctness of your program.
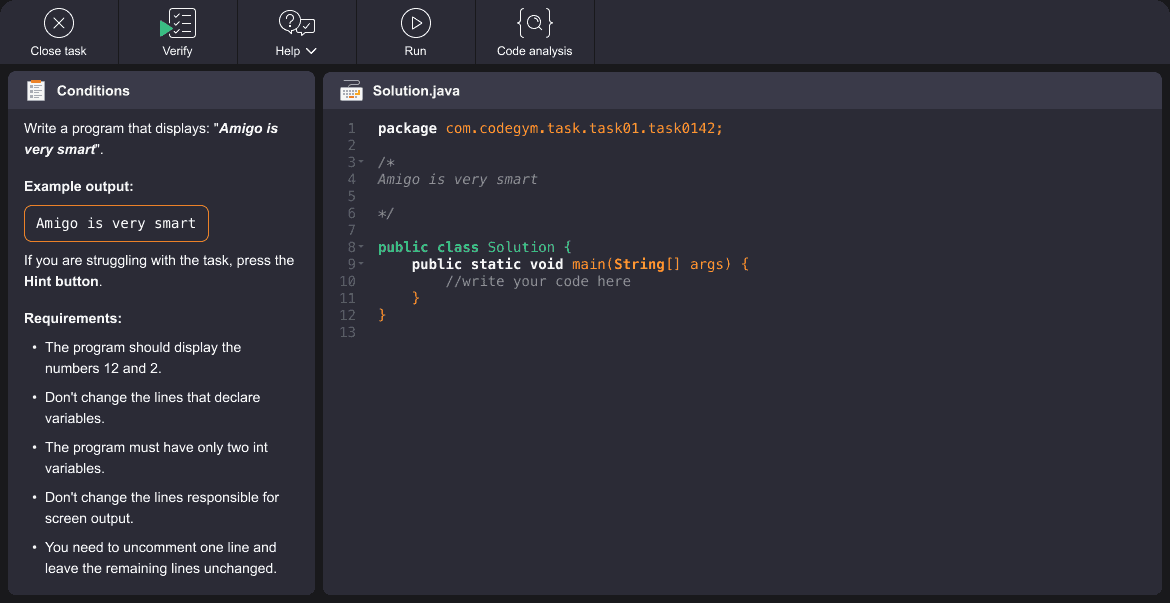
The two main types in Java are String and int. We store strings/text in String, and integers (whole numbers) in int. We have already used strings and integers in previous examples without explicit declaration, by specifying them directly in the System.out.println() operator.
In the first case “I am a string” is a String in the second case 5 is an integer of type int. However, most often, in order to manipulate data, variables must be declared before being used in the program. To do this, you need to specify the type of the variable and its name. You can also set a variable to a specific value, or you can do this later. Example:
Here we declared a variable called a but didn't give it any value, declared a variable b and gave it the value 5 , declared a string called s and gave it the value Hello, World!
Attention! In Java, the = sign is not an equals sign, but an assignment operator. That is, the variable (you can imagine it as an empty box) is assigned the value that is on the right (you can imagine that this value was put in the empty box).
We created an integer variable named a with the first command and assigned it the value 5 with the second command.
Before moving on to practice, let's look at an example program where we will declare variables and assign values to them:
In the program, we first declared an int variable named a but did not immediately assign it a value. Then we declared an int variable named b and "put" the value 5 in it. Then we declared a string named s and assigned it the value "Hello, World!" After that, we assigned the value 2 to the variable a that we declared earlier, and then we printed the variable a, the sum of the variables a and b, and the variable s to the screen
This program will display the following:
We already know how to print to the console, but how do we read from it? For this, we use the Scanner class. To use Scanner, we first need to create an instance of the class. We can do this with the following code:
Once we have created an instance of Scanner, we can use the next() method to read input from the console or nextInt() if we should read an integer.
The following code reads a number from the console and prints it to the console:
Here we first import a library scanner, then ask a user to enter a number. Later we created a scanner to read the user's input and print the input out.
This code will print the following output in case of user’s input is 5:
More information about the topic you could read here , here , and here .
See the exercises on Types and keyboard input to practice Java coding:
Conditions and If statements in Java allow your program to make decisions. For example, you can use them to check if a user has entered a valid password, or to determine whether a number is even or odd. For this purpose, there’s an 'if/else statement' in Java.
The syntax for an if statement is as follows:
Here could be one or more conditions in if and zero or one condition in else.
Here's a simple example:
In this example, we check if the variable "age" is greater than or equal to 18. If it is, we print "You are an adult." If not, we print "You are a minor."
Here are some Java practice exercises to understand Conditions and If statements:
In Java, a "boolean" is a data type that can have one of two values: true or false. Here's a simple example:
The output of this program is here:
In addition to representing true or false values, booleans in Java can be combined using logical operators. Here, we introduce the logical AND (&&) and logical OR (||) operators.
- && (AND) returns true if both operands are true. In our example, isBothFunAndEasy is true because Java is fun (isJavaFun is true) and coding is not easy (isCodingEasy is false).
- || (OR) returns true if at least one operand is true. In our example, isEitherFunOrEasy is true because Java is fun (isJavaFun is true), even though coding is not easy (isCodingEasy is false).
- The NOT operator (!) is unary, meaning it operates on a single boolean value. It negates the value, so !isCodingEasy is true because it reverses the false value of isCodingEasy.
So the output of this program is:
More information about the topic you could read here , and here .
Here are some Java exercises to practice booleans:
With loops, you can execute any command or a block of commands multiple times. The construction of the while loop is:
Loops are essential in programming to execute a block of code repeatedly. Java provides two commonly used loops: while and for.
1. while Loop: The while loop continues executing a block of code as long as a specified condition is true. Firstly, the condition is checked. While it’s true, the body of the loop (commands) is executed. If the condition is always true, the loop will repeat infinitely, and if the condition is false, the commands in a loop will never be executed.
In this example, the code inside the while loop will run repeatedly as long as count is less than or equal to 5.
2. for Loop: The for loop is used for iterating a specific number of times.
In this for loop, we initialize i to 1, specify the condition i <= 5, and increment i by 1 in each iteration. It will print "Count: 1" to "Count: 5."
Here are some Java coding challenges to practice the loops:
An array in Java is a data structure that allows you to store multiple values of the same type under a single variable name. It acts as a container for elements that can be accessed using an index.
What you should know about arrays in Java:
- Indexing: Elements in an array are indexed, starting from 0. You can access elements by specifying their index in square brackets after the array name, like myArray[0] to access the first element.
- Initialization: To use an array, you must declare and initialize it. You specify the array's type and its length. For example, to create an integer array that can hold five values: int[] myArray = new int[5];
- Populating: After initialization, you can populate the array by assigning values to its elements. All elements should be of the same data type. For instance, myArray[0] = 10; myArray[1] = 20;.
- Default Values: Arrays are initialized with default values. For objects, this is null, and for primitive types (int, double, boolean, etc.), it's typically 0, 0.0, or false.
In this example, we create an integer array, assign values to its elements, and access an element using indexing.
In Java, methods are like mini-programs within your main program. They are used to perform specific tasks, making your code more organized and manageable. Methods take a set of instructions and encapsulate them under a single name for easy reuse. Here's how you declare a method:
- public is an access modifier that defines who can use the method. In this case, public means the method can be accessed from anywhere in your program.Read more about modifiers here .
- static means the method belongs to the class itself, rather than an instance of the class. It's used for the main method, allowing it to run without creating an object.
- void indicates that the method doesn't return any value. If it did, you would replace void with the data type of the returned value.
In this example, we have a main method (the entry point of the program) and a customMethod that we've defined. The main method calls customMethod, which prints a message. This illustrates how methods help organize and reuse code in Java, making it more efficient and readable.
In this example, we have a main method that calls the add method with two numbers (5 and 3). The add method calculates the sum and returns it. The result is then printed in the main method.
All composite types in Java consist of simpler ones, up until we end up with primitive types. An example of a primitive type is int, while String is a composite type that stores its data as a table of characters (primitive type char). Here are some examples of primitive types in Java:
- int: Used for storing whole numbers (integers). Example: int age = 25;
- double: Used for storing numbers with a decimal point. Example: double price = 19.99;
- char: Used for storing single characters. Example: char grade = 'A';
- boolean: Used for storing true or false values. Example: boolean isJavaFun = true;
- String: Used for storing text (a sequence of characters). Example: String greeting = "Hello, World!";
Simple types are grouped into composite types, that are called classes. Example:
We declared a composite type Person and stored the data in a String (name) and int variable for an age of a person. Since composite types include many primitive types, they take up more memory than variables of the primitive types.
See the exercises for a coding practice in Java data types:
String is the most popular class in Java programs. Its objects are stored in a memory in a special way. The structure of this class is rather simple: there’s a character array (char array) inside, that stores all the characters of the string.
String class also has many helper classes to simplify working with strings in Java, and a lot of methods. Here’s what you can do while working with strings: compare them, search for substrings, and create new substrings.
Example of comparing strings using the equals() method.
Also you can check if a string contains a substring using the contains() method.
You can create a new substring from an existing string using the substring() method.
More information about the topic you could read here , here , here , here , and here .
Here are some Java programming exercises to practice the strings:
In Java, objects are instances of classes that you can create to represent and work with real-world entities or concepts. Here's how you can create objects:
First, you need to define a class that describes the properties and behaviors of your object. You can then create an object of that class using the new keyword like this:
It invokes the constructor of a class.If the constructor takes arguments, you can pass them within the parentheses. For example, to create an object of class Person with the name "Jane" and age 25, you would write:
Suppose you want to create a simple Person class with a name property and a sayHello method. Here's how you do it:
In this example, we defined a Person class with a name property and a sayHello method. We then created two Person objects (person1 and person2) and used them to represent individuals with different names.
Here are some coding challenges in Java object creation:
Static classes and methods in Java are used to create members that belong to the class itself, rather than to instances of the class. They can be accessed without creating an object of the class.
Static methods and classes are useful when you want to define utility methods or encapsulate related classes within a larger class without requiring an instance of the outer class. They are often used in various Java libraries and frameworks for organizing and providing utility functions.
You declare them with the static modifier.
Static Methods
A static method is a method that belongs to the class rather than any specific instance. You can call a static method using the class name, without creating an object of that class.
In this example, the add method is static. You can directly call it using Calculator.add(5, 3)
Static Classes
In Java, you can also have static nested classes, which are classes defined within another class and marked as static. These static nested classes can be accessed using the outer class's name.
In this example, Student is a static nested class within the School class. You can access it using School.Student.
More information about the topic you could read here , here , here , and here .
See below the exercises on Static classes and methods in our Java coding practice for beginners:

Recent Posts

5 Reasons Why Anyone Can Learn to Code
by Editorial Team
Coding has become an essential skill in today’s digital age, opening doors to countless opportunities across various industries. Many people, however, still hold the belief that coding is a complex skill reserved for tech geniuses. This couldn’t be further from the...

Land Your Dream Developer Job in Months with SkillReactor
by Abdul Wasay
Are you a coder looking to break into the tech industry or upskill to land your dream developer job? Then look no further than SkillReactor! This article will explore how our unique EdTech platform can empower you to achieve your coding goals faster than traditional...

Thinking of moving to a new job? Here’s how to resign appropriately
by Amanda Kavanagh
By Amanda Kavanagh While many of us may harbour fantasies about quitting a job with panache — the 1998 film Half Baked comes to mind — our long-term career view usually prevents drastic action. No matter how long you’ve worked somewhere, chances are you want to...

Best Software Engineer Certifications in 2024
In today's dynamic tech landscape, staying ahead of the curve is crucial for software engineers. Earning industry-recognized software engineer certifications demonstrates your commitment to professional development and validates your proficiency in specific technical...

by Abdul Wasay | Mar 25, 2024
Java Coding Interview Questions You Need to Know
Did you know that Java is one of the world’s most widely used programming languages? It powers countless applications and systems, from enterprise software to Android apps. As a result, Java coding interview questions have become a crucial part of the hiring process for Java developers . If you’re aspiring to land a job as a Java developer, you must have a strong grasp of these questions.
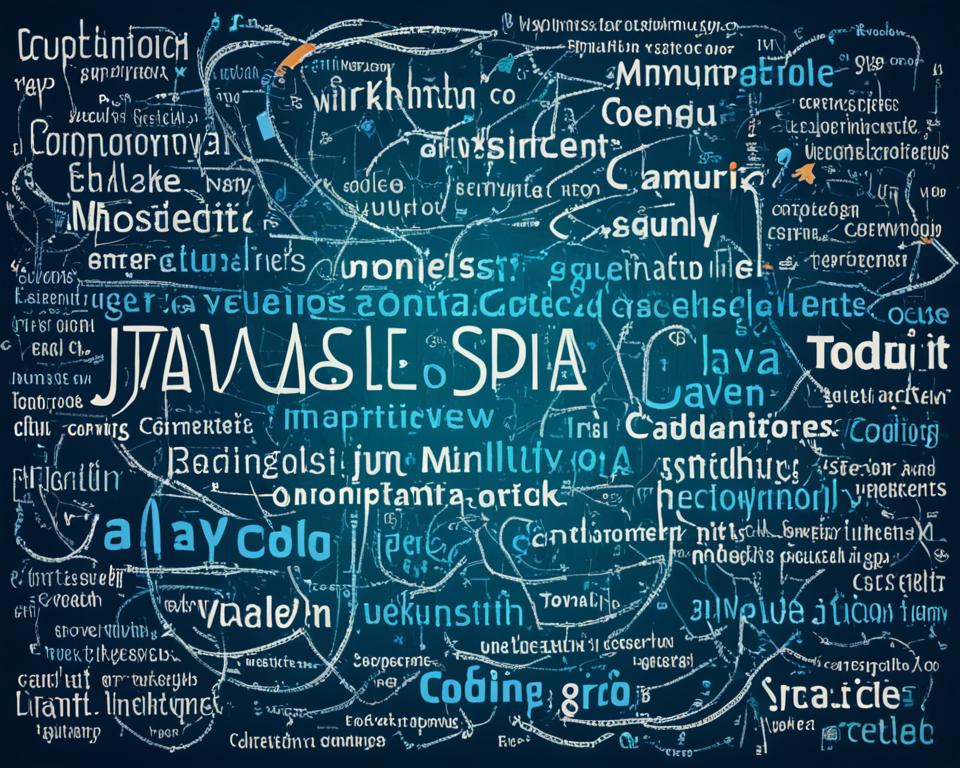
Key Takeaways:
- Mastering Java coding interview questions is crucial for Java developers aiming to excel in interviews.
- A strong understanding of Java coding interview questions increases your chances of success.
- Java is widely used in various industries, making it a valuable skill for developers.
- Preparing for common Java coding interview questions helps build your technical skills.
- Stay updated with the latest Java features and practices to stand out in interviews.
Common Java Coding Interview Questions
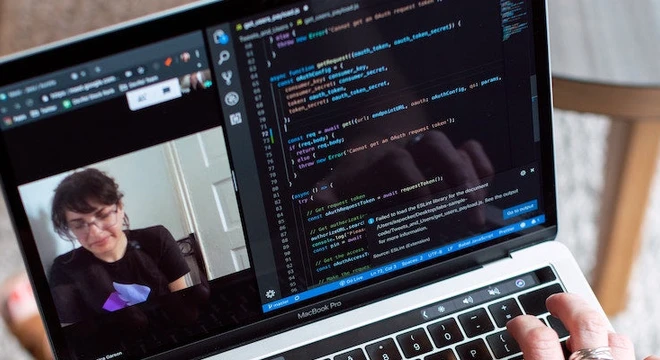
This section will explore some of the most commonly asked Java coding interview questions. These questions cover fundamental concepts that every Java developer should be familiar with. Understanding and preparing for these questions can greatly increase your chances of success in your Java coding interviews.
Write a program that prints the numbers from 1 to 100. But for multiples of three, print “Fizz” instead of the number, and for the multiples of five, print “Buzz”. For numbers that are multiples of both three and five, print “FizzBuzz”.
Palindrome Check
Write a function to determine if a given string is a palindrome. A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward.
Fibonacci Series
Create a program that generates the Fibonacci series up to a given number of terms. The Fibonacci series is a sequence of numbers where each number is the sum of the two preceding ones.
Reverse a String
Write a function that reverses a given string. For example, if the input is “Hello”, the output should be “olleH”.
Find the Missing Number
Given an array of integers from 1 to n, one number is missing. Write a function to find the missing number in the array.
These are just a few examples of common Java coding interview questions. Practicing and familiarizing yourself with various questions to build your confidence and problem-solving skills is important.
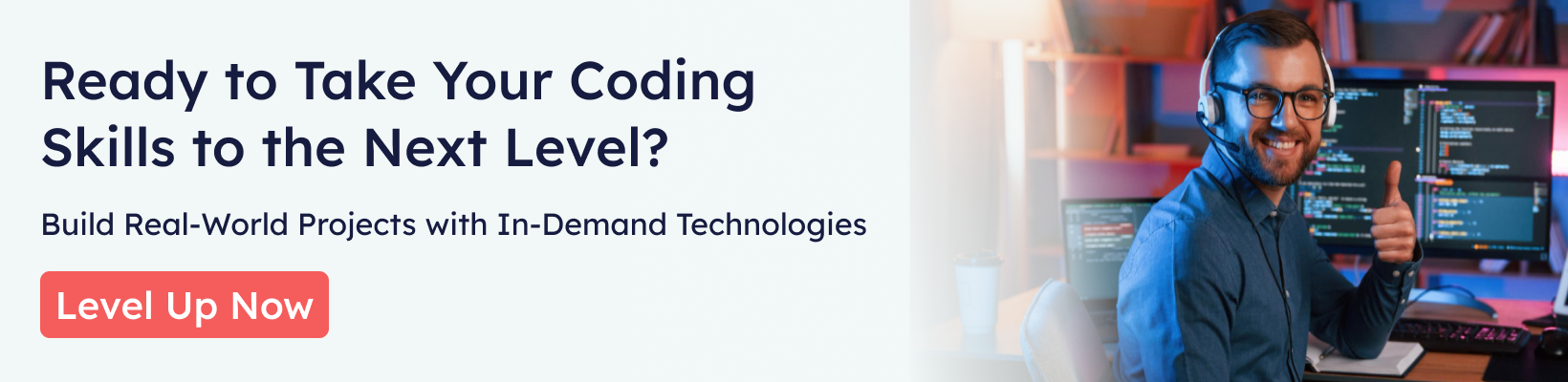
Question | Description | Sample Input | Sample Output |
---|---|---|---|
FizzBuzz | Print numbers from 1 to 100, replacing multiples of three with “Fizz”, multiples of five with “Buzz”, and multiples of both three and five with “FizzBuzz”. | N/A | 1, 2, Fizz, 4, Buzz, Fizz, 7, 8, Fizz, Buzz, 11, Fizz, 13, 14, FizzBuzz, … |
Palindrome Check | Determine if a given string is a palindrome. | “racecar” | true |
Fibonacci Series | Generate the Fibonacci series up to a given number of terms. | 5 | 0, 1, 1, 2, 3 |
Reverse a String | Reverse a given string. | “world” | “dlrow” |
Find the Missing Number | Find the missing number in an array of integers from 1 to n. | [1, 2, 4, 5] | 3 |
Core Java Interview Questions
When interviewing candidates for Java developer positions, assessing their understanding of core Java principles is crucial. Core Java interview questions focus on the foundational concepts of the Java programming language, ensuring that candidates possess a strong grasp of the fundamentals. This section explores essential core Java interview questions that evaluate a candidate’s knowledge and expertise in key areas.
1. What is the difference between JDK, JRE, and JVM?
The Java Development Kit (JDK) is a software development environment that provides tools, libraries, and documentation for developing Java applications. The Java Runtime Environment (JRE) is an implementation of the Java Virtual Machine (JVM) that allows you to run Java applications. The Java Virtual Machine (JVM) is an abstract machine that interprets Java bytecode, enabling platform independence by translating the bytecode into machine-specific instructions.
2. Explain the key features of object-oriented programming in Java.
Encapsulation: Bundling data and methods within a class to hide implementation details. Inheritance: Allowing classes to inherit attributes and behaviors from other classes. Polymorphism: Using a single interface to represent multiple forms. Abstraction: Providing a simplified view of complex systems by defining classes based on common characteristics.
It is essential to assess a candidate’s understanding of these core Java concepts and ability to apply them in real-world scenarios. By evaluating their responses to these interview questions, you can gauge the depth of their knowledge and experience with Java programming.
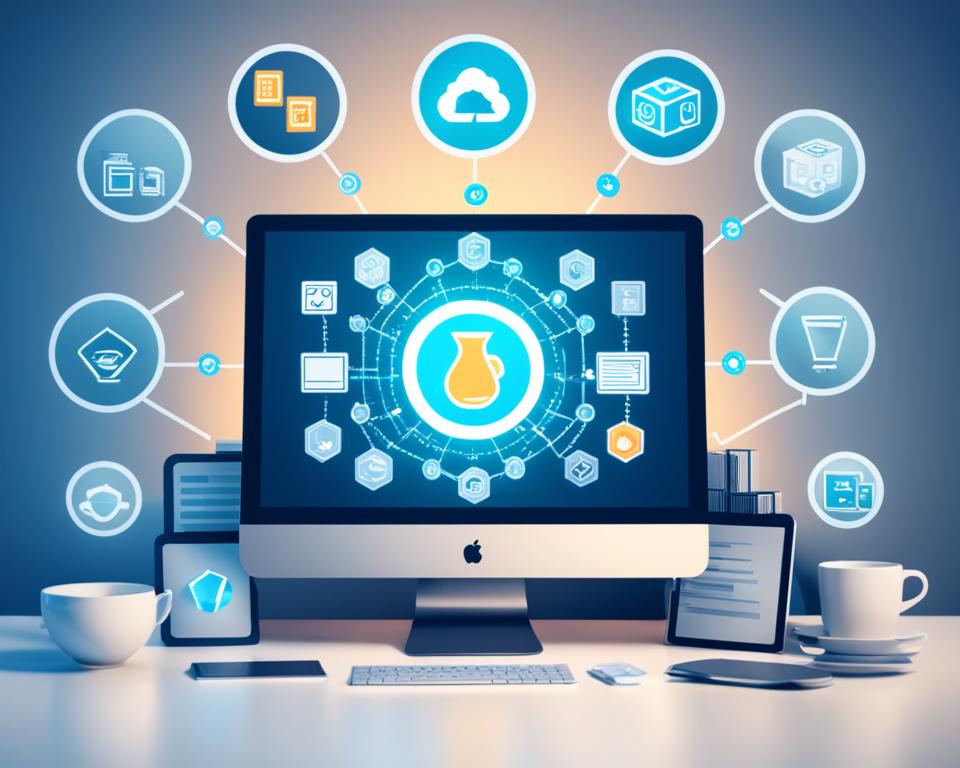
The next section will explore advanced Java coding interview questions that challenge candidates with complex programming problems and advanced Java concepts.
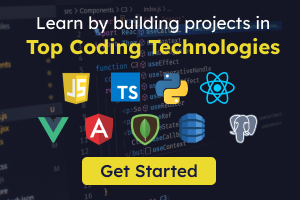
Advanced Java Coding Interview Questions
Aspiring Java developers who wish to stand out in interviews must be familiar with the common Java coding interview questions and prepared for more advanced ones. This section presents a collection of challenging Java coding questions that require a deep understanding of advanced Java concepts and principles.
1. Explain the concept of multithreading in Java.
Multithreading is the ability of a Java program to execute multiple threads concurrently, allowing for parallel task execution. It enables efficient utilization of system resources and improves overall program performance. However, managing multiple threads requires synchronization and coordination to avoid issues such as race conditions and deadlocks.
2. What are the differences between method overriding and method overloading?
Method overriding occurs when a subclass defines a method with the same name and signature as an inherited method from its superclass. The overriding method provides a different implementation, replacing the behavior of the superclass method for objects of the subclass. Method overloading, on the other hand, involves defining multiple methods with the same name but different parameters within a class. Overloaded methods enable flexibility by allowing different ways to invoke the same method based on the number and types of arguments passed.
3. Explain the concept of generics in Java.
Generics in Java allow classes and methods to be parameterized by type, providing compile-time type safety. Using generics enables the creation of reusable code independent of specific types, enhancing code readability and maintainability. Generics, such as ArrayList and HashMap, are commonly used with collections to enforce type constraints and enable the compiler to detect type mismatches at compile time.
Tip: When dealing with generics, it is important to specify the appropriate type parameters to ensure type safety and prevent runtime errors.
4. Describe the concept of exception handling in Java.
Exception handling in Java allows programmers to handle and recover from exceptional conditions or errors that may occur during program execution. It involves the use of try-catch blocks to catch and handle exceptions. The catch block contains code to handle specific types of exceptions, providing an alternative course of action when an exception occurs. Additionally, the final block ensures that certain code is executed regardless of whether an exception is thrown.
5. What are the advantages and disadvantages of using an abstract class in Java?
An abstract class in Java serves as a blueprint from which other classes can inherit. It provides a common interface and defines methods and variables that subclasses can implement or override. The advantages of using an abstract class include promoting code reusability, enforcing a consistent structure across related classes, and allowing for future extension through subclassing. However, one disadvantage is that Java does not allow multiple inheritance, so a class can only inherit from one abstract class.
Advantages | Disadvantages |
---|---|
Enables code reusability | Java does not allow multiple inheritance |
Defines common methods and variables for subclasses | |
Provides a consistent structure across related classes | |
Allows for future extension through subclassing |
By familiarizing themselves with these advanced Java coding interview questions and understanding the underlying concepts, aspiring Java developers can enhance their problem-solving skills and demonstrate their proficiency in advanced Java programming.
Java Programming Interview Questions
When preparing for a Java programming interview, it’s important to have a solid understanding of the key concepts and techniques that are commonly tested. This section focuses on specific areas of Java programming that you should be prepared to discuss and provides examples of interview questions related to these topics.
Object-Oriented Programming
Object-oriented programming (OOP) is a fundamental aspect of Java programming. Interviewers often ask questions to assess your understanding of OOP principles and how they are implemented in Java. Here are some common interview questions related to OOP:
- Explain the concept of inheritance and how it is used in Java.
- What are abstract classes and interfaces, and what is their difference?
- How does polymorphism work in Java? Provide an example.
Exception Handling
Exception handling is an essential part of Java programming. During an interview, you may be asked how to handle exceptions effectively. Here are a few examples of interview questions related to exception handling:
- Explain the difference between checked and unchecked exceptions in Java.
- What is the purpose of the try-catch-finally block? Provide an example.
- How can you create your custom exceptions in Java?
Multithreading
Understanding multithreading is crucial for developing efficient and responsive Java applications. Interviewers may ask questions about multithreading to assess your knowledge in this area. Here are some common interview questions about multithreading:
- Explain the difference between a thread and a process.
- How can you create a new thread in Java? Provide an example.
- What are the advantages and disadvantages of using multithreading in Java?
By familiarizing yourself with these Java programming interview questions, you can feel more confident and prepared for your next interview. Remember to practice answering these questions and be ready to explain your thought process and demonstrate your problem-solving skills.
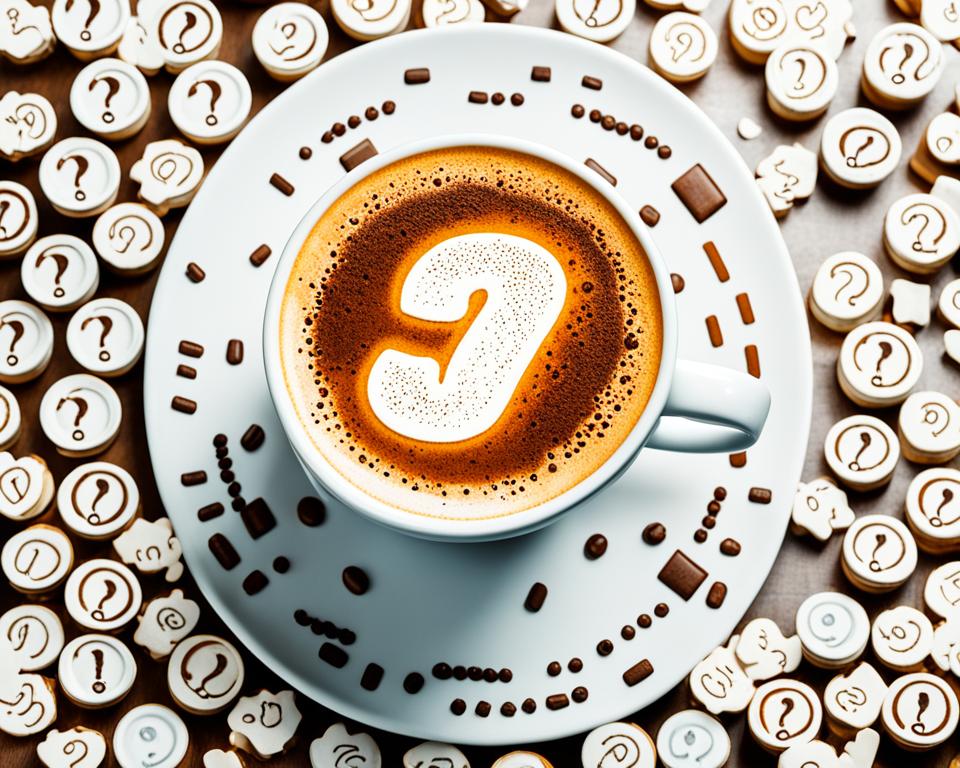
“Having a strong understanding of object-oriented programming, exception handling, and multithreading in Java is crucial for success in coding interviews.”
Tricky Java Coding Interview Questions
In a Java coding interview, candidates often encounter tricky questions that test their problem-solving skills and critical thinking ability. These questions go beyond the basics and require a deep understanding of Java concepts. To help you prepare for these challenging questions, we have highlighted some examples below and insights on how to approach them.
Question 1: Palindrome Check
Determine whether a given string is a palindrome or not. A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward.
Example: Input: “racecar” Output: true Input: “hello” Output: false
To solve this question, you can use two pointers, one starting from the beginning of the string and the other from the end. Compare the characters at each position until the pointers meet. If all the characters match, the string is a palindrome.
Question 2: Find the Missing Number
Given an array of integers from 1 to n with one missing number, find the missing number.
Example: Input: [1, 2, 4, 5, 6] Output: 3
To solve this question, you can calculate the expected sum of all the numbers from 1 to n using the formula (n * (n + 1)) / 2. Then, subtract the sum of the given array from the expected sum to find the missing number.
Question 3: Reverse Linked List
Reverse a singly linked list.
Example: Input: 1 -> 2 -> 3 -> 4 -> 5 Output: 5 -> 4 -> 3 -> 2 -> 1
You can use three-pointers to reverse the links between the nodes to solve this question. Traverse through the linked list and update the next pointers accordingly until you reach the end of the list.
These are just a few examples of tricky Java coding interview questions. By practicing and understanding the underlying concepts, you can improve your problem-solving skills and approach these questions with confidence.
Best Java Coding Interview Questions
Are you preparing for a Java coding interview? This section has curated a list of the best questions for you. These questions cover a wide range of concepts and difficulty levels, ensuring you comprehensively understand Java coding requirements.
Check out the following questions to improve your interview preparation:
- Question 1: Explain the difference between StringBuffer and StringBuilder. Answer: StringBuffer and StringBuilder are both used to manipulate strings in Java. However, there is a significant difference between them. StringBuffer is synchronized and thread-safe, while StringBuilder is not synchronized but faster. It is important to understand when to use each one based on your requirements.
- Question 2: What is the difference between a deep and shallow copy? Answer: In Java, a deep copy creates a completely independent copy of an object, including all its nested objects. On the other hand, a shallow copy creates a new object that references the same nested objects as the original object. Understanding the differences between these two copy types is crucial for avoiding unexpected behavior in your code.
- Question 3: How does Java handle memory management? Explain the concept of garbage collection. Answer: Java uses automatic memory management through a process called garbage collection. Garbage collection identifies and frees up memory that the program no longer uses. This automated memory management relieves developers from manual memory allocation and deallocation, making Java a memory-friendly language.
Mastering these Java coding interview questions will enhance your technical skills and boost your confidence during interviews. In the next section, we will examine some more intriguing questions.
Java Interview Questions for Experienced Developers
Experienced Java developers stand out from the crowd with their depth of knowledge and practical experience. When it comes to interviews, these professionals often face different questions that delve deeper into their expertise. This section is designed to address those specific questions and provide valuable insights for experienced developers.
Here, we will explore advanced concepts and scenarios experienced Java developers will likely encounter during interviews. By understanding and preparing for these questions, you can showcase your proficiency in design patterns, performance optimization, architectural principles, and more.
Sample Questions for Experienced Java Developers
- How would you optimize the performance of a Java application?
- Can you explain the differences between checked and unchecked exceptions?
- Discuss the advantages and disadvantages of using multiple inheritance in Java.
- Explain the purpose and usage of the Java Volatile keyword.
- Describe the principles of the Model-View-Controller (MVC) architectural pattern and its implementation in Java.
Experienced Java developers are expected to have a deep understanding of the programming language and its intricacies. Interviewers may ask questions that require practical knowledge, problem-solving skills, and the ability to make architectural decisions.
To further assist you in your preparations, here is a sample table comparing the skills and knowledge required for entry-level Java interviews versus experienced Java interviews:
Skills/Knowledge | Entry-Level Java Interviews | Experienced Java Interviews |
---|---|---|
Core Java | ✔️ | ✔️ |
Object-Oriented Programming | ✔️ | ✔️ |
Design Patterns | ✔️ | |
Concurrency | ✔️ | |
Performance Optimization | ✔️ | |
Architectural Principles | ✔️ |
Remember, the depth of your answers and practical examples of projects you’ve worked on will be crucial in impressing interviewers. Showcase your experience, problem-solving abilities, and the value you can bring as an experienced Java developer.
Top Java Coding Interview Questions
Java coding interview questions are an essential part of the interview process for aspiring Java developers. In this section, we will discuss the top Java coding interview questions that hiring managers frequently ask. By understanding and preparing for these questions, candidates can increase their chances of success in Java coding interviews.
1. What is the difference between ArrayList and LinkedList in Java?
2. Explain the concept of object-oriented programming (OOP) and its main principles.
3. What is the difference between equals() and == in Java?
4. Explain the concept of multithreading in Java and how it works.
5. What is the purpose of the “final” keyword in Java?
6. What are checked and unchecked exceptions in Java?
7. Explain the concept of inheritance in Java and how it is implemented.
8. What is the role of the “static” keyword in Java?
“In Java, static keyword is used to create variables and methods that belong to the class, rather than instances of the class. Static members can be accessed directly using the class name, without creating an object. This can be useful for utility methods or variables that need to be shared across all instances of a class.”
9. What is method overloading in Java?
10. Explain the concept of exception handling in Java and how it helps write robust code.
To summarize, being well-prepared for Java coding interviews can significantly improve your chances of landing a job as a Java developer. By familiarizing yourself with these top Java coding interview questions and practicing your problem-solving skills, you can confidently tackle any coding challenge that comes your way.
Comparison between ArrayList and LinkedList in Java
ArrayList | LinkedList |
---|---|
Implements the List interface. | Implements the List and Deque interfaces. |
Uses a dynamic array to store elements. | Uses a linked list to store elements. |
Provides efficient random access and modification of elements. | Provides efficient insertion and deletion of elements. |
Slower performance for insertion and deletion of elements. | Fast performance for insertion and deletion of elements. |
Requires more memory when compared to LinkedList. | Requires less memory when compared to ArrayList. |
Mastering Java coding interview questions is essential for Java developers looking to excel in their interviews. These questions not only assess a candidate’s technical knowledge but also their problem-solving and critical-thinking skills. By thoroughly studying and practicing these questions, candidates can enhance their understanding of fundamental Java concepts and confidently tackle interview challenges.
Preparing for Java coding interviews involves a combination of theoretical knowledge and practical application. It is important to have a strong understanding of core Java principles and advanced Java concepts. By familiarizing themselves with various Java programming topics, candidates can demonstrate their versatility and ability to adapt to different coding scenarios.
Furthermore, becoming well-versed in Java coding interview questions can significantly boost a candidate’s confidence during the interview process. By knowing how to approach and solve different coding problems, candidates can effectively showcase their technical skills and demonstrate their value to potential employers.
What are Java coding interview questions?
Java coding interview questions assess a candidate’s knowledge and proficiency in Java programming. They cover various aspects of Java, including core concepts, object-oriented programming, data structures, algorithms, and more.
Why are Java coding interview questions important?
Java coding interview questions are important because they help hiring managers evaluate a candidate’s technical skills and problem-solving abilities. Interviewers can assess a candidate’s understanding of Java fundamentals, coding practices, and ability to apply Java concepts to real-world scenarios by asking specific Java coding questions.
What are some common Java coding interview questions?
Common Java coding interview questions include topics such as Java basics (data types, loops, conditionals), object-oriented programming (inheritance, polymorphism), Java collections (ArrayList, HashMap), exception handling, multithreading, and more.
What are core Java interview questions?
Core Java interview questions focus on the fundamental concepts of the Java programming language. They assess a candidate’s knowledge of Java syntax, data types, operators, control flow, classes and objects, inheritance, interfaces, and basic Java libraries.
What are advanced Java coding interview questions?
Advanced Java coding interview questions are designed to evaluate a candidate’s in-depth understanding of complex Java concepts and advanced programming techniques. These questions often cover advanced topics such as multithreading, Java Generics, Java streams, concurrency, design patterns, and performance optimization.
What are Java programming interview questions?
Java programming interview questions focus on assessing a candidate’s ability to apply Java programming concepts to solve specific problems. These questions cover object-oriented programming, exception handling, data structures, algorithms, database connectivity, and Java frameworks.
What are some tricky Java coding interview questions?
Tricky Java coding interview questions are designed to challenge a candidate’s problem-solving abilities and critical thinking skills. They often involve complex scenarios, edge cases, or unexpected behavior of Java language features. Candidates must analyze the problem, consider different approaches, and provide the most appropriate solution.
What are the best Java coding interview questions?
The best Java coding interview questions cover a wide range of Java concepts and difficulty levels. These questions effectively evaluate a candidate’s understanding of core Java principles, ability to write clean and efficient code, and problem-solving skills. They provide a comprehensive assessment of a candidate’s Java programming proficiency.
What are Java interview questions for experienced developers?
Java interview questions for experienced developers are specifically tailored to evaluate their advanced knowledge and practical experience with Java programming. These questions may delve into advanced Java topics, design patterns, database integration, performance tuning, debugging techniques, and frameworks commonly used in enterprise-level applications.
What are the top Java coding interview questions?
The top Java coding interview questions are hiring managers’ most frequently asked questions. These questions cover a variety of Java concepts and are specifically designed to assess a candidate’s problem-solving skills, coding techniques, familiarity with Java libraries/frameworks, and ability to write efficient and maintainable code.
AI Proof Your Career

Secure Your Lifetime 40% Discount for Code Learning. Use code OFF40
Top 100+ SQL Interview Questions and Practice Exercises

- jobs and career
- sql practice
- sql interview questions
Table of Contents
Review Your SQL Knowledge
Practice regularly, familiarize yourself with the testing platform, prepare for different types of questions, additional tips, explore 55+ general sql interview questions, practice, practice, practice, …, sql cheat sheet, data analysis in sql, window functions, common table expressions, advanced sql, good luck with your interview.
Are you gearing up for a SQL interview? This article is packed with over 100 SQL interview questions and practical exercises, organized by topic, to help you prepare thoroughly and approach your interview with confidence.
SQL is essential for many jobs, like data analysis, data science, software engineering, data engineering, testing, and many others. Preparing well for a SQL interview is crucial, no matter what role you're aiming for.
Searching for a new job can be really stressful, whether you're choosing to switch, have been laid off, or are looking for your first job. That's why being well-prepared is essential.
In this article, I've gathered over 100 SQL interview questions and exercises. These questions are spread across various articles published at LearnSQL.com. I have organized the articles by topic. Feel free to explore only the topics related to your specific job. I've also included tips to help you prepare for your interview.
SQL Interview Preparation Tips
Start preparing for your SQL interview well in advance. Once you're invited to an interview (Congratulations!), ask your recruiter what to expect and what is the format of the interview. For the SQL part you can usually expect coding exercises on an automated testing platform, a take-home assignment, or a whiteboard session.
The key to performing well in a SQL interview is practice. You'll likely be nervous, so the more familiar you are with SQL, the more instinctive your responses will become. Practice a variety of SQL problems so that querying becomes second nature to you.
If your interview involves using a specific coding platform, try to get comfortable with it beforehand. Many platforms offer a demo or practice session, so take advantage of this feature to familiarize yourself with the interface. This familiarity can help reduce stress and improve your performance during the actual interview.

- Coding Platform Questions: Whether during the interview or as a take-home task, make sure you understand the typical questions and problems that might appear on these platforms. Practice solving similar problems under timed conditions.
- Whiteboard Interviews: Be ready to write code in pseudocode and discuss your thought process. Focus on explaining the concepts and logic behind your solutions more than the exact syntax, which demonstrates a deeper understanding of the problem-solving process.
- Review Key SQL Concepts: Make sure you're comfortable with all fundamental SQL operations such as joins, subqueries, window functions, and aggregation. Also, review more advanced topics if the job role demands it.
- Mock Interviews: Consider doing mock interviews with friends or mentors to simulate the interview environment. This practice can help you manage time and stress effectively.
- Rest Well: Ensure you're well-rested before the interview day; a clear mind will help you think and perform better.
By incorporating these strategies into your preparation, you can approach your SQL interview with confidence and increase your chances of success.
Begin by refreshing your SQL knowledge, particularly if you haven't used it in a while. In this section we have collected some resources to assist you.
Our "SQL Basics" course is perfect for beginners or anyone needing a brief review. It covers both basic and intermediate SQL topics. In this course, you will actively write SQL code in various exercises, which will help you grow more confident in your SQL skills as you advance.

After you have refreshed the basics, check out these articles filled with SQL interview questions to help you prepare:
- Complete SQL Practice for Interviews — includes 16 SQL interview questions with practical exercises.
- 16 SQL Interview Questions for Business Analysts — SQL interview questions tailored for analysts.
- 8 Common Entry Level SQL Developer Interview Questions — great for beginners.
- Top 15 SQL Interview Questions in 2021 — a compilation of recent and relevant questions.
After refreshing your SQL skills, it’s important to keep practicing. Interviews can be stressful, and even straightforward topics can become challenging under pressure. The more you practice, the more confidently you can handle questions and problem-solving during an interview.
Here are some practice resources we recommend:
- SQL Practice track – This series includes 10 comprehensive SQL practice courses to sharpen your skills, perfect for those looking for hands-on practice. Key courses in this track include:
- SQL Practice Set – Provides a range of exercises across various SQL topics and databases.
- SQL Practice: A Store – Specifically designed for data analysts, this course offers practical SQL tasks using a database from an online store.
- SQL Practice: Blog & Traffic Data – Perfect for marketers and data analysts, this course focuses on analyzing traffic data from a pet store blog.
- SQL Practice Databases – Gathers a variety of datasets for you to practice with. With no guided exercises, you are encouraged to explore the data, formulate your own questions, and find the answers yourself.
You can find many SQL practice materials and premium resources in Your Guide to SQL Practice at LearnSQL.com .
Lastly, we recommend our SQL Basics Cheat Sheet . It is a quick reference guide that covers basic SQL syntax. Keep it handy as you review your SQL knowledge and practice your skills.

Explore 50+ Specific SQL Topic Interview Questions
After you have refreshed your basic SQL knowledge, you might notice certain topics that are trickier for you or more relevant to your specific job role. In this section we've compiled resources that help you prepare for interview questions on specific SQL topics.
JOINs are a fundamental SQL construction used to combine data from multiple tables. They are also an essential topic at any SQL interview.
In our article The Top 10 SQL JOIN Interview Questions with Answers we've gathered the 10 most common questions about SQL JOINs that you might encounter in interviews. For each question we give you a detailed answer that will highlight what the interviewer is looking for in each question.
If you want to practice SQL JOINs, we recommend our interactive SQL JOINs course . It focuses on exercises specifically about SQL JOINs and contains 93 practice exercises to help you get confidence in your joining skills.
Additionally, we recommend Your Complete Guide to SQL JOINs , a comprehensive article that covers the basic knowledge of SQL JOINs, with additional articles and other resources on our platform.
The GROUP BY clause, paired with aggregate functions, is fundamental in SQL for calculating statistics like counts, averages, and sums from your data. This topic is essential for any SQL interview.
Our article Top 9 SQL GROUP BY Interview Questions provides a collection of the most frequently asked interview questions about GROUP BY . Each question includes a detailed answer, making sure you're prepared to discuss these topics during an interview.
If you are looking for an intermediate-level practice of GROUP BY topics, we recommend our Creating Basic SQL Reports course. It offers 100 exercises that focus on nuances of GROUP BY that can be asked about during an interview. It’s a hands-on course where you write your own SQL queries to help you better understand the issues and commit them to memory.
Furthermore, our article GROUP BY and Aggregate Functions: A Complete Overview gives a thorough explanation of GROUP BY and aggregate functions. This comprehensive guide is an excellent resource to round out your study, ensuring you have a robust understanding of how these functions work and how they can be applied in various scenarios.
We know that many of our users work specifically in the domain of data analysis. For these users, we have prepared an article 25 SQL Interview Questions for Data Analysts , which collects common SQL interview questions that can be asked for a role of data analyst. The article covers intermediate and advanced topics, like CTEs or window functions.
Window functions are an advanced SQL topic. Window functions are particularly useful when writing complex reports in SQL. For this reason, they are essential in data analysis and will come up in any data analysis interview.
Our article Top 10 SQL Window Functions Interview Questions contains the most common interview questions you might encounter regarding window functions. Each question has a detailed answer and links to further resources to help you dive deeper into each topic.
For those looking to refresh their knowledge through practice, we recommend our specialized courses:
- Window Functions – Covers the entire syntax of SQL window functions through interactive, hands-on exercises, making it ideal for those new to window functions or needing a refresher.
- Window Functions Practice Set - Aimed at those already familiar with window functions, this course provides additional practice to help refine your skills and prepare for more complex interview questions.
Additionally, we recommend our Window Functions Cheat Sheet , a handy quick reference guide for window functions. For a more thorough review, SQL Window Functions Guide is a comprehensive article that covers the basics of window functions with links to additional resources.
Common Table Expressions, or CTEs, is another advanced topic crucial for SQL interviews. CTEs help you organize and manage long and complex queries, make writing complex reports easier, and help you query hierarchical structures through recursive queries.
Our article Top 5 SQL CTE Interview Questions compiles essential CTE-related questions you're likely to face in interviews.in an article. Each question in the article is paired with a detailed answer to help you understand what is the most important in each response.
We also recommend our interactive Recursive Queries course that covers the syntax of CTEs through practice. The course is designed to teach the syntax and use of CTEs, including recursive CTEs, through hands-on exercises.
Finally, check out these articles to help you get ready for an advanced SQL interview:
- How to Prepare for an Advanced SQL Interview
- Top 27 Advanced SQL Interview Questions with Answers
- 15 Tricky SQL Interview Questions for Experienced Users
We also suggest our Advanced SQL Practice track, which is an online series of SQL practice courses designed for advanced users.
In this article we have gathered over 100 SQL interview questions and 20 additional resources compiled here to ensure you're thoroughly prepared. To further enhance your preparation, we recommend our All Forever SQL Package . It provides access to all our current and future courses in a single purchase, making it an excellent investment for your ongoing SQL education and interview readiness.
Sign up for free at LearnSQL.com and explore our SQL courses offer . Each month, we offer one of our courses—typically a practical, hands-on course—for free . This gives you a perfect opportunity to try out our resources without any commitment and see how they can help you succeed in your SQL interview. Take advantage of these offers to boost your confidence and sharpen your SQL skills effectively.
You may also like
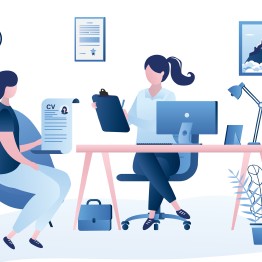
How Do You Write a SELECT Statement in SQL?
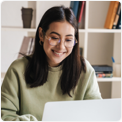
What Is a Foreign Key in SQL?

Enumerate and Explain All the Basic Elements of an SQL Query
Lean Events and Training / Events / Managing to Learn
Managing to Learn
An introduction to a3 leadership and problem-solving..
Available Dates
Online August 5, 2024 - September 16, 2024: 12:00pm - 2:00pm ET
In-Person October 2, 2024 - October 3, 2024: 8:00am - 4:00pm ET
Coach-Led Online Course and Oakland University in Rochester, Michigan
Why you should attend
Learn how to use the A3 Methodology to solve important business problems. An optional one-on-one coaching package is also available for online course only.
In-Person 2-Day Course Oct 2-3: $1,599 Early bird price* $1,999 Regular price *Early bird expires August 23 for October session.
Select from available dates
- August 5, 2024 - September 16, 2024
- October 2, 2024 - October 3, 2024
Today’s unprecedented challenges require superior problem-solving skills not only from you as a leader but everyone you manage.
We’ve taken the unmatched A3 problem-solving process described in Managing to Learn , the award-winning, best-selling workbook by management expert and former CEO John Shook, and put it online with live instruction.
This comprehensive training will teach you how to use the potent A3 methodology, based on the proven scientific method of plan-do-check-act (PDCA), to address an important business problem within your organization.
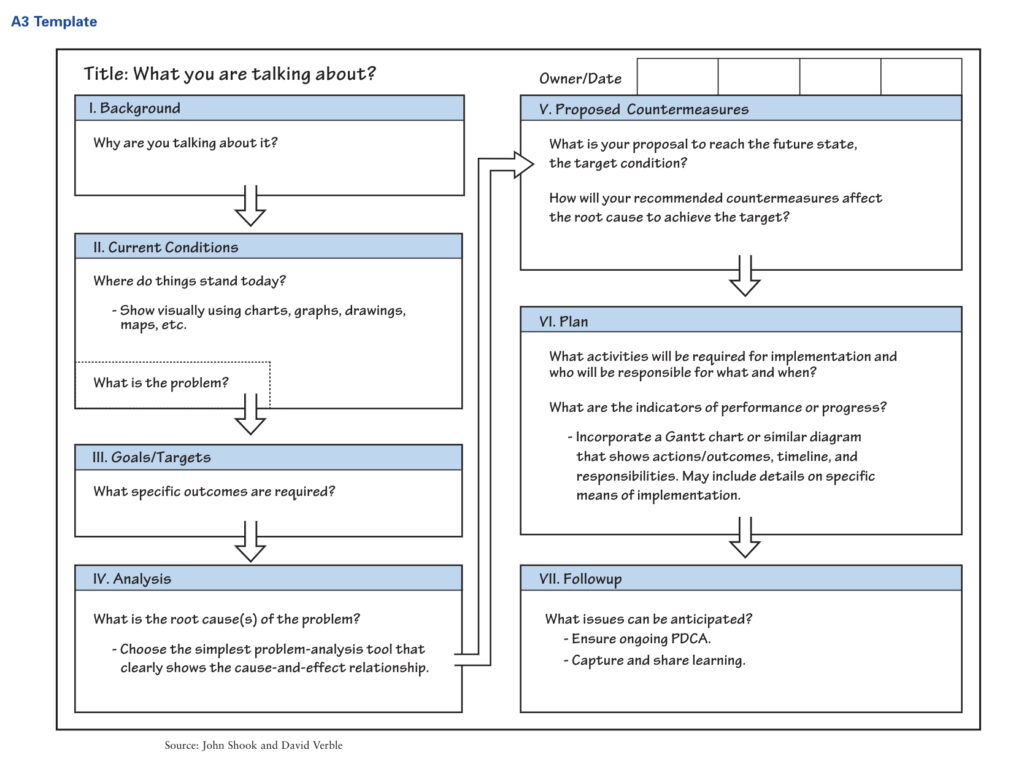
Learning Objectives
What makes the A3 problem-solving approach so powerful is that it is a complete process — a way of thinking, leading, communicating, learning, getting things done, and developing an entire organization of problem solvers.
- Select, define, clarify and investigate a real problem from work.
- Clarify problem situations and define problems as gaps in performance.
- Visualize work processes and focus on the problems in work methods that are affecting performance.
- Investigate and confirm underlying causes and analyze barriers to improvement
- Identify, evaluate, and lead in the selection of countermeasures.
- Lead planning for implementation of countermeasures and follow-up to resolve problems in execution for organizational learning.
What’s Included?
- 14 hours of live, online interaction or 16 hours of hands-on, in-person interaction
- Feedback from facilitators and peers.
- Assignments between live sessions to begin applying the lessons and complete your A3.
- Opportunities to practice discrete skills with live feedback.
Optional One-on-One Coaching Sessions for Online Course
Achieve an even deeper level of learning by taking personal coaching sessions with the class instructor.
These optional, half-hour sessions happen at three critical points as you create an A3 in the workshop:
- completion of the problem situation/current situation section;
- completion of the analysis section and whole left side;
- creation of recommended countermeasures and an implementation plan at the start of the right side.
Personal coaching gives you individual “just-in-time” assistance on your challenges in a private, completely safe online space to share and ask questions.
One-on-one coaching with the instructor will increase your professional skills and value to the company. And all three sessions are only $499.
Buy One-on-One Coaching »
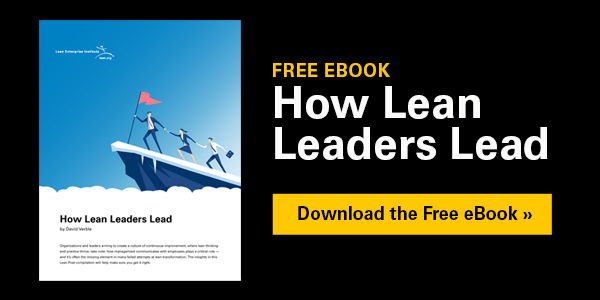
Who Should Attend
- Managers, supervisors or executives who want to develop the structured problem-solving, hypothesis-testing, and fact-based, decision-making skills of teams
- Continuous improvement or change management professionals involved in leading major change improvement initiatives
- HR professionals who wish to develop a curriculum and teach introduce structured problem solving and fact-based decision-making skills within their organization
Group Discounts
Register three or more students from your organization and save 12.5% off every registration. This discount will be automatically applied at checkout when you register your group.
Technology Used
- Communication Channel: All our documents and assignments will be hosted on a browser-based platform. By logging onto our e-learning website, you will be able to view all related materials, announcements, and zoom links.
- Zoom : We will host our live meetings on zoom. You will receive an invitation with the meeting link.
Cancellation Policy
You can cancel your registration for online/live-streaming workshops two weeks before the course’s start date for a full refund. A cancellation occurring within two weeks of the workshop dates will be subject to a $350 fee. Once you have attended a workshop session, you cannot cancel your registration. If you need to cancel, you can do so through your confirmation email from ‘The LEI Events Team’ or email [email protected].
You can cancel your registration for in-person workshops four weeks before the course start date for a full refund. A cancellation occurring within four weeks of the workshop dates will be subject to a $350 fee. Cancelling less than two weeks prior to workshop start is subject to no refund. To cancel, please call LEI at (617) 871-2900 or email [email protected].
Schedule Overview – Remotely
The 7 sessions are hosted over 7 weeks with one to two hours of assignments in between. There are optional coaching sessions available for online courses for an additional $499 (email [email protected] to inquire).
June 10 – July 22, 2024 Course All sessions hosted 3:00 PM – 5:00 PM ET and instructed by Karen Gaudet
- Monday, June 10
- Monday, June. 17
- Monday, June 24
- Monday, July 1
- Monday, July 8
- Monday, July 15
- Monday, July 22
August 5 – September 16, 2024 Course All sessions hosted 12:00 PM – 2:00 PM ET and instructed by Lavon Medlock
- Monday, August 5
- Monday, August 12
- Monday, August 19
- Monday, August 26
- Monday, September 2
- Monday, September 9
- Monday, September 16
Schedule Overview – In-Person
October 2-3, 2024 In-Person Course All sessions hosted 8:00 AM – 4:00 PM ET and instructed by Eric Ethington
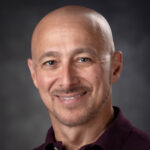
Eric Ethington
Senior Coach and Chief Engineer Product and Process Development Lean Enterprise Institute President, Lean Shift Consulting
Eric has distilled his passion for and knowledge of lean thinking and practice in product and process development, nurtured over 30 years of work experience, into The Power of Process: A Story of Innovative Lean Process Development (2022). Before founding Lean Shift Consulting and becoming a coach and program manager at LEI in 2016, he […]
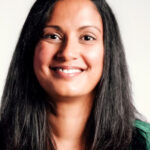
Lavon Medlock
Lavon Medlock has spent over two decades enhancing leaders’ skills in problem-solving and coaching. Skilled in a variety of continuous improvement methods, she has trained leaders in creating effective daily management systems, deployed an integrated facility design approach to new construction projects like a 90,000-square-foot patient tower, and enhanced operations across different sectors. With a […]
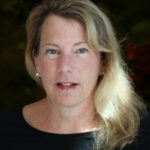
Karen Gaudet
Senior Coach, Lean Enterprise Institute
Karen has over 30 years of experience leading, training, and developing the capability of team members and executives in rapid-growth environments. Most recently, she’s coached clients in various industries as they adopt lean thinking and practices. They include Microsoft (data center construction), Legal Seafood (hospitality); TriMark (distribution); Abiomed (medical research and device manufacturing), and the […]
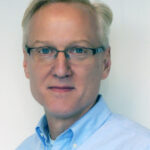
Senior Coach and Chief Engineer, Strategy Lean Enterprise Institute
During his extensive career, Mark has led lean transformations and coached executives in various companies and business sectors. Clients include GE Appliances and Ingersoll Rand (manufacturers); Michigan Medicine and Mt. Sinai (healthcare systems); Turner Construction; Kroger (retail); Legal Seafood (hospitality); and Microsoft (software). As LEI’s chief engineer, strategy, Mark leads the development of new learning […]
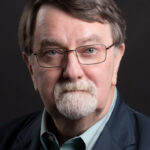
David Verble
Lean Coach, Lean Enterprise Institute Partner, Lean Transformations Group
A performance improvement consultant and leadership coach since 2000, David has been an LEI faculty member for 17 years. Recognized as one of the first Toyota-trained managers to bring A3 thinking from Japan to the United States, he has conducted A3 problem-solving and leadership programs for 30 years. Overall, his work focuses on supporting clients […]
Location: Oakland University 318 Meadow Brook Rd Rochester, MI 48309
Suggested Nearby Hotels:
- Embassy Suites by Hilton Auburn Hills 2300 Featherstone Road, Auburn Hills, MI 48326 This hotel is located approximately 3 miles from Oakland University (~7 minute drive).
- SpringHill Suites Detroit Auburn Hills 4919 Interpark Dr., Orion Township, MI 48359 This hotel is located approximately 4 miles from Oakland University (~10 minute drive).
- Holiday Inn Express & Suites: Auburn Hills 3990 Baldwin Road, Auburn Hills, MI This hotel is located approximately 6 miles from Oakland University (~7 minute drive).
Nearest Airports:
- Detroit Metropolitan Wayne County Airport (DTW) ~46 miles to Oakland University, Rochester, MI
Bring Our Practical, How-to Training to Your Company
Custom Training
Related events.
June 25, 2024 | Coach-Led Online Course
Understanding Lean Transformation
July 18, 2024 | Detroit, Michigan
The Future of People at Work Symposium
September 06, 2024 | Coach-Led Online Course
Hoshin Kanri
Subscribe to get the very best of lean thinking delivered right to your inbox, privacy overview.
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
Welcome to the daily solving of our PROBLEM OF THE DAY with Saurabh Bansal. We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Arrays but also build up problem-solving skills. You have given two sorted arrays arr1[] & arr2[] of distinct elements. The first array has one element extra added in between. Return the index of the extra element.
Note : 0-based indexing is followed.
Input: n = 7, arr1[] = {2,4,6,8,9,10,12}, arr2[] = {2,4,6,8,10,12} Output: 4 Explanation: In the first array, 9 is extra added and it's index is 4.
Give the problem a try before going through the video. All the best!!! Problem Link: https://practice.geeksforgeeks.org/problems/index-of-an-extra-element/1

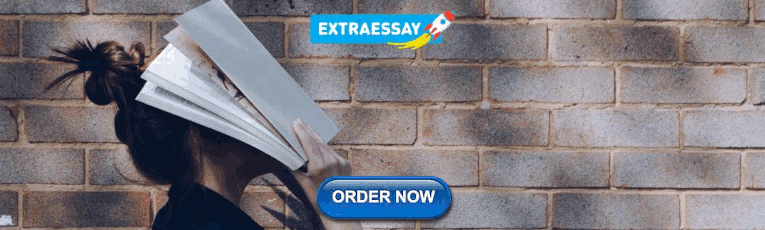
IMAGES
VIDEO
COMMENTS
4. Write a Java program to check if the given number is a prime number. You can write a program to divide the given number n, by a number from 2 to n /2 and check the remainder. If the remainder is 0, then it's not a prime number. The following example code shows one way to check if a given number is a Prime number:
We hope these exercises have helped you understand Java better and you can solve beginner to advanced-level questions on Java programming. Solving these Java programming exercise questions will not only help you master theory concepts but also grasp their practical applications, which is very useful in job interviews. More Java Practice Exercises
Top 50 Java Programs from Coding Interviews Here is a big list of Java programs for Job Interviews. As I said, it includes questions from problem-solving, linked list, array, string, matrix, bitwise operators and other miscellaneous parts of programming. Once you are gone through these questions, you can handle a good number of questions on ...
Algorithm-based questions are a staple of any modern coding interview, as they demonstrate your problem-solving and critical thinking skills. To make sure you don't get caught off guard in your next Java interview, we've put together 15 of the most common algorithm coding questions used by most tech companies and recruiters across the industry. ...
Java Interview Questions and Answers. Java is one of the most popular programming languages in the world, known for its versatility, portability, and wide range of applications. Java is the most used language in top companies such as Uber, Airbnb, Google, Netflix, Instagram, Spotify, Amazon, and many more because of its features and performance.
Java is well-known for its robustness in Object-Oriented Programming (OOP), and it provides a comprehensive foundation essential for developers at every level. This handbook offers a detailed pathway to help you excel in Java interviews. It focuses on delivering insights and techniques relevant to roles in esteemed big tech companies, ensuring ...
Join over 23 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews.
21 Essential Java Interview Questions. *. Toptal sourced essential questions that the best Java developers and engineers can answer. Driven from our community, we encourage experts to submit questions and offer feedback. is an exclusive network of the top freelance software developers, designers, finance experts, product managers, and project ...
This list focuses on beginners and less experienced devs, like someone with 2 to 3 years of experience in Java. 1) How does Java achieve platform independence? ( answer) hint: bytecode and Java Virtual Machine. 2) What is ClassLoader in Java? ( answer) hint: part of JVM that loads bytecodes for classes.
Complete your Java coding practice with our online Java practice course on CodeChef. Solve over 180 coding problems and challenges to get better at Java. ... CodeChef Learn Problem Solving: 287: Multiple Choice Question: NA: Learning SQL: 339: Multiple Choice Question: NA: Parking Charges: 416: 6. ... 30 Questions 1 Hrs 30 Min . View all skill ...
The best way we learn anything is by practice and exercise questions. Here you have the opportunity to practice the Java programming language concepts by solving the exercises starting from basic to more complex exercises. A sample solution is provided for each exercise. It is recommended to do these exercises by yourself first before checking ...
Two important properties of a Java object are behavior and state. An object is created as soon as the JVM comes across the new keyword. 17. Define classes in Java. A class is a collection of objects of similar data types. Classes are user-defined data types and behave like built-in types of a programming language.
Coding interviews are aimed at gauging how well-prepared a candidate is in terms of language proficiency, foundational knowledge, problem-solving skills, and soft skills. For a position that explicitly mentions a language, such as a job listing for a Java developer, it makes even more sense to spend some time and energy polishing Java skills by exploring the common interview questions asked in ...
Java 8 introduced a host of powerful features that have significantly enhanced the Java programming language.Introducing new features such as Lambda Expressions, Stream API, Functional Interfaces, the new Date and Time API, and more.. As a result, Java 8 skills are highly sought after by employers in the tech industry. To help you prepare for your next interview, we have compiled a list of ...
1. For a given array of integers (positive and negative) find the largest sum of a contiguous sequence. 2. Algorithm: Implement a queue using 2 stacks (solution) 3. Algorithm: Integer division without the division operator (/) (solution) 4. How to print All permutations of a Given String in Java ( solution) 5.
Java 165. Answer. The JVM has a heap that is the runtime data area from which memory for all class instances and arrays is allocated. It is created at the JVM start-up. Heap memory for objects is reclaimed by an automatic memory management system which is known as a garbage collector.
As per my experience, most of the questions are related to: the programming language particularities (syntax, core API) problem solving (algorithms, data structures) application design / architecture (design patterns, object oriented programming, best practices…). You'll find below a summary of these question categories and some examples.
1. Introduction. In this tutorial, we're going to explore some of the JDK8-related questions that might pop up during an interview. Java 8 is a platform release packed with new language features and library classes. Most of these new features are geared towards achieving cleaner and more compact code, while some add new functionality that has ...
Boost your coding interview skills and confidence by practicing real interview questions with LeetCode. Our platform offers a range of essential problems for practice, as well as the latest questions being asked by top-tier companies.
How Edabit Works. This is an introduction to how challenges on Edabit work. In the Code tab above you'll see a starter function that looks like this: public static boolean returnTrue () { } All you have to do is type return true; between the curly braces { } and then click the Check button. If you did this correctly, the button will turn re ...
Explore the Java coding exercises for practicing with commands below. First, read the conditions, scroll down to the Solution box, and type your solution. Then, click Verify (above the Conditions box) to check the correctness of your program. Exercise 1 Exercise 2 Exercise 3. Start task.
Btw, if you find this question tough to solve, I suggest you take a look at the Grokking the Coding Interview: Patterns for Coding Questions course on Educative, an interactive portal for coding ...
What are the top Java coding interview questions? The top Java coding interview questions are hiring managers' most frequently asked questions. These questions cover a variety of Java concepts and are specifically designed to assess a candidate's problem-solving skills, coding techniques, familiarity with Java libraries/frameworks, and ...
Practice solving similar problems under timed conditions. Whiteboard Interviews: Be ready to write code in pseudocode and discuss your thought process. Focus on explaining the concepts and logic behind your solutions more than the exact syntax, which demonstrates a deeper understanding of the problem-solving process. Additional Tips
Today's unprecedented challenges require superior problem-solving skills not only from you as a leader but everyone you manage. We've taken the unmatched A3 problem-solving process described in Managing to Learn, the award-winning, best-selling workbook by management expert and former CEO John Shook, and put it online with live instruction.
Find the first repeating element in an array of integers. Solve. Find the first non-repeating element in a given array of integers. Solve. Subarrays with equal 1s and 0s. Solve. Rearrange the array in alternating positive and negative items. Solve. Find if there is any subarray with a sum equal to zero.
Welcome to the daily solving of our PROBLEM OF THE DAY with Jay Dalsaniya. We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Arrays but also build up problem-solving skills. Given a set of n nuts & bolts.There is a one-on-one mapping between nuts and bolts.
Welcome to the daily solving of our PROBLEM OF THE DAY with Saurabh Bansal. We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Arrays but also build up problem-solving skills. You have given two sorted arrays arr1[] & arr2[] of distinct elements. The first array has one element extra added ...