Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python exercises.
You can test your Python skills with W3Schools' Exercises.
We have gathered a variety of Python exercises (with answers) for each Python Chapter.
Try to solve an exercise by filling in the missing parts of a code. If you're stuck, hit the "Show Answer" button to see what you've done wrong.
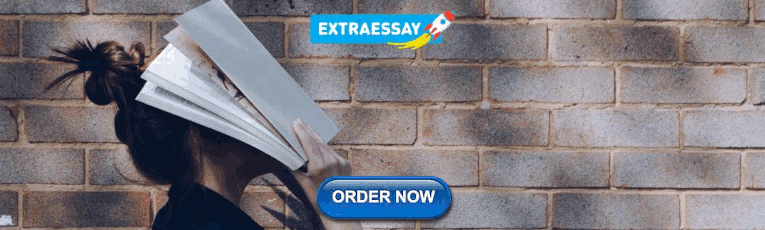
Count Your Score
You will get 1 point for each correct answer. Your score and total score will always be displayed.
Start Python Exercises
Start Python Exercises ❯
If you don't know Python, we suggest that you read our Python Tutorial from scratch.
Kickstart your career
Get certified by completing the course

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
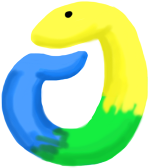
Practice Python
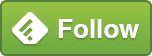
Beginner Python exercises
- Why Practice Python?
- Why Chilis?
- Resources for learners
All Exercises

All Solutions
- 1: Character Input Solutions
- 2: Odd Or Even Solutions
- 3: List Less Than Ten Solutions
- 4: Divisors Solutions
- 5: List Overlap Solutions
- 6: String Lists Solutions
- 7: List Comprehensions Solutions
- 8: Rock Paper Scissors Solutions
- 9: Guessing Game One Solutions
- 10: List Overlap Comprehensions Solutions
- 11: Check Primality Functions Solutions
- 12: List Ends Solutions
- 13: Fibonacci Solutions
- 14: List Remove Duplicates Solutions
- 15: Reverse Word Order Solutions
- 16: Password Generator Solutions
- 17: Decode A Web Page Solutions
- 18: Cows And Bulls Solutions
- 19: Decode A Web Page Two Solutions
- 20: Element Search Solutions
- 21: Write To A File Solutions
- 22: Read From File Solutions
- 23: File Overlap Solutions
- 24: Draw A Game Board Solutions
- 25: Guessing Game Two Solutions
- 26: Check Tic Tac Toe Solutions
- 27: Tic Tac Toe Draw Solutions
- 28: Max Of Three Solutions
- 29: Tic Tac Toe Game Solutions
- 30: Pick Word Solutions
- 31: Guess Letters Solutions
- 32: Hangman Solutions
- 33: Birthday Dictionaries Solutions
- 34: Birthday Json Solutions
- 35: Birthday Months Solutions
- 36: Birthday Plots Solutions
- 37: Functions Refactor Solution
- 38: f Strings Solution
- 39: Character Input Datetime Solution
- 40: Error Checking Solution
Python for Everybody
- Coursera: Python for Everybody Specialization
- edX: Python for Everybody
- FutureLearn: Programming for Everybody (Getting Started with Python)
- FreeCodeCamp
- Free certificates for University of Michigan students and staff
If you log in to this site you have joined a free, global open and online course. You have a grade book, autograded assignments, discussion forums, and can earn badges for your efforts.
We take your privacy seriously on this site, you can review our Privacy Policy for more details.
If you want to use these materials in your own classes you can download or link to the artifacts on this site, export the course material as an IMS Common Cartridge®, or apply for an IMS Learning Tools Interoperability® (LTI®) key and secret to launch the autograders from your LMS.
The code for this site including the autograders, slides, and course content is all available on GitHub . That means you could make your own copy of the course site, publish it and remix it any way you like. Even more exciting, you could translate the entire site (course) into your own language and publish it. I have provided some instructions on how to translate this course in my GitHub repository.
And yes, Dr. Chuck actually has a race car - it is called the SakaiCar . He races in a series called 24 Hours of Lemons .
Python Programming Challenges
Practice your Python skills with these programming challenges. The tasks are meant to be challenging for beginners. If you find them too difficult, try completing our lessons for beginners first.
All challenges have hints and curated example solutions. They also work on your phone, so you can practice Python on the go.
Click a challenge to start.
Python Practice for Beginners: 15 Hands-On Problems

- online practice
Want to put your Python skills to the test? Challenge yourself with these 15 Python practice exercises taken directly from our Python courses!
There’s no denying that solving Python exercises is one of the best ways to practice and improve your Python skills . Hands-on engagement with the language is essential for effective learning. This is exactly what this article will help you with: we've curated a diverse set of Python practice exercises tailored specifically for beginners seeking to test their programming skills.
These Python practice exercises cover a spectrum of fundamental concepts, all of which are covered in our Python Data Structures in Practice and Built-in Algorithms in Python courses. Together, both courses add up to 39 hours of content. They contain over 180 exercises for you to hone your Python skills. In fact, the exercises in this article were taken directly from these courses!
In these Python practice exercises, we will use a variety of data structures, including lists, dictionaries, and sets. We’ll also practice basic programming features like functions, loops, and conditionals. Every exercise is followed by a solution and explanation. The proposed solution is not necessarily the only possible answer, so try to find your own alternative solutions. Let’s get right into it!
Python Practice Problem 1: Average Expenses for Each Semester
John has a list of his monthly expenses from last year:
He wants to know his average expenses for each semester. Using a for loop, calculate John’s average expenses for the first semester (January to June) and the second semester (July to December).
Explanation
We initialize two variables, first_semester_total and second_semester_total , to store the total expenses for each semester. Then, we iterate through the monthly_spending list using enumerate() , which provides both the index and the corresponding value in each iteration. If you have never heard of enumerate() before – or if you are unsure about how for loops in Python work – take a look at our article How to Write a for Loop in Python .
Within the loop, we check if the index is less than 6 (January to June); if so, we add the expense to first_semester_total . If the index is greater than 6, we add the expense to second_semester_total .
After iterating through all the months, we calculate the average expenses for each semester by dividing the total expenses by 6 (the number of months in each semester). Finally, we print out the average expenses for each semester.
Python Practice Problem 2: Who Spent More?
John has a friend, Sam, who also kept a list of his expenses from last year:
They want to find out how many months John spent more money than Sam. Use a for loop to compare their expenses for each month. Keep track of the number of months where John spent more money.
We initialize the variable months_john_spent_more with the value zero. Then we use a for loop with range(len()) to iterate over the indices of the john_monthly_spending list.
Within the loop, we compare John's expenses with Sam's expenses for the corresponding month using the index i . If John's expenses are greater than Sam's for a particular month, we increment the months_john_spent_more variable. Finally, we print out the total number of months where John spent more money than Sam.
Python Practice Problem 3: All of Our Friends
Paul and Tina each have a list of their respective friends:
Combine both lists into a single list that contains all of their friends. Don’t include duplicate entries in the resulting list.
There are a few different ways to solve this problem. One option is to use the + operator to concatenate Paul and Tina's friend lists ( paul_friends and tina_friends ). Afterwards, we convert the combined list to a set using set() , and then convert it back to a list using list() . Since sets cannot have duplicate entries, this process guarantees that the resulting list does not hold any duplicates. Finally, we print the resulting combined list of friends.
If you need a refresher on Python sets, check out our in-depth guide to working with sets in Python or find out the difference between Python sets, lists, and tuples .
Python Practice Problem 4: Find the Common Friends
Now, let’s try a different operation. We will start from the same lists of Paul’s and Tina’s friends:
In this exercise, we’ll use a for loop to get a list of their common friends.
For this problem, we use a for loop to iterate through each friend in Paul's list ( paul_friends ). Inside the loop, we check if the current friend is also present in Tina's list ( tina_friends ). If it is, it is added to the common_friends list. This approach guarantees that we test each one of Paul’s friends against each one of Tina’s friends. Finally, we print the resulting list of friends that are common to both Paul and Tina.
Python Practice Problem 5: Find the Basketball Players
You work at a sports club. The following sets contain the names of players registered to play different sports:
How can you obtain a set that includes the players that are only registered to play basketball (i.e. not registered for football or volleyball)?
This type of scenario is exactly where set operations shine. Don’t worry if you never heard about them: we have an article on Python set operations with examples to help get you up to speed.
First, we use the | (union) operator to combine the sets of football and volleyball players into a single set. In the same line, we use the - (difference) operator to subtract this combined set from the set of basketball players. The result is a set containing only the players registered for basketball and not for football or volleyball.
If you prefer, you can also reach the same answer using set methods instead of the operators:
It’s essentially the same operation, so use whichever you think is more readable.
Python Practice Problem 6: Count the Votes
Let’s try counting the number of occurrences in a list. The list below represent the results of a poll where students were asked for their favorite programming language:
Use a dictionary to tally up the votes in the poll.
In this exercise, we utilize a dictionary ( vote_tally ) to count the occurrences of each programming language in the poll results. We iterate through the poll_results list using a for loop; for each language, we check if it already is in the dictionary. If it is, we increment the count; otherwise, we add the language to the dictionary with a starting count of 1. This approach effectively tallies up the votes for each programming language.
If you want to learn more about other ways to work with dictionaries in Python, check out our article on 13 dictionary examples for beginners .
Python Practice Problem 7: Sum the Scores
Three friends are playing a game, where each player has three rounds to score. At the end, the player whose total score (i.e. the sum of each round) is the highest wins. Consider the scores below (formatted as a list of tuples):
Create a dictionary where each player is represented by the dictionary key and the corresponding total score is the dictionary value.
This solution is similar to the previous one. We use a dictionary ( total_scores ) to store the total scores for each player in the game. We iterate through the list of scores using a for loop, extracting the player's name and score from each tuple. For each player, we check if they already exist as a key in the dictionary. If they do, we add the current score to the existing total; otherwise, we create a new key in the dictionary with the initial score. At the end of the for loop, the total score of each player will be stored in the total_scores dictionary, which we at last print.
Python Practice Problem 8: Calculate the Statistics
Given any list of numbers in Python, such as …
… write a function that returns a tuple containing the list’s maximum value, sum of values, and mean value.
We create a function called calculate_statistics to calculate the required statistics from a list of numbers. This function utilizes a combination of max() , sum() , and len() to obtain these statistics. The results are then returned as a tuple containing the maximum value, the sum of values, and the mean value.
The function is called with the provided list and the results are printed individually.
Python Practice Problem 9: Longest and Shortest Words
Given the list of words below ..
… find the longest and the shortest word in the list.
To find the longest and shortest word in the list, we initialize the variables longest_word and shortest_word as the first word in the list. Then we use a for loop to iterate through the word list. Within the loop, we compare the length of each word with the length of the current longest and shortest words. If a word is longer than the current longest word, it becomes the new longest word; on the other hand, if it's shorter than the current shortest word, it becomes the new shortest word. After iterating through the entire list, the variables longest_word and shortest_word will hold the corresponding words.
There’s a catch, though: what happens if two or more words are the shortest? In that case, since the logic used is to overwrite the shortest_word only if the current word is shorter – but not of equal length – then shortest_word is set to whichever shortest word appears first. The same logic applies to longest_word , too. If you want to set these variables to the shortest/longest word that appears last in the list, you only need to change the comparisons to <= (less or equal than) and >= (greater or equal than), respectively.
If you want to learn more about Python strings and what you can do with them, be sure to check out this overview on Python string methods .
Python Practice Problem 10: Filter a List by Frequency
Given a list of numbers …
… create a new list containing only the numbers that occur at least three times in the list.
Here, we use a for loop to iterate through the number_list . In the loop, we use the count() method to check if the current number occurs at least three times in the number_list . If the condition is met, the number is appended to the filtered_list .
After the loop, the filtered_list contains only numbers that appear three or more times in the original list.
Python Practice Problem 11: The Second-Best Score
You’re given a list of students’ scores in no particular order:
Find the second-highest score in the list.
This one is a breeze if we know about the sort() method for Python lists – we use it here to sort the list of exam results in ascending order. This way, the highest scores come last. Then we only need to access the second to last element in the list (using the index -2 ) to get the second-highest score.
Python Practice Problem 12: Check If a List Is Symmetrical
Given the lists of numbers below …
… create a function that returns whether a list is symmetrical. In this case, a symmetrical list is a list that remains the same after it is reversed – i.e. it’s the same backwards and forwards.
Reversing a list can be achieved by using the reverse() method. In this solution, this is done inside the is_symmetrical function.
To avoid modifying the original list, a copy is created using the copy() method before using reverse() . The reversed list is then compared with the original list to determine if it’s symmetrical.
The remaining code is responsible for passing each list to the is_symmetrical function and printing out the result.
Python Practice Problem 13: Sort By Number of Vowels
Given this list of strings …
… sort the list by the number of vowels in each word. Words with fewer vowels should come first.
Whenever we need to sort values in a custom order, the easiest approach is to create a helper function. In this approach, we pass the helper function to Python’s sorted() function using the key parameter. The sorting logic is defined in the helper function.
In the solution above, the custom function count_vowels uses a for loop to iterate through each character in the word, checking if it is a vowel in a case-insensitive manner. The loop increments the count variable for each vowel found and then returns it. We then simply pass the list of fruits to sorted() , along with the key=count_vowels argument.
Python Practice Problem 14: Sorting a Mixed List
Imagine you have a list with mixed data types: strings, integers, and floats:
Typically, you wouldn’t be able to sort this list, since Python cannot compare strings to numbers. However, writing a custom sorting function can help you sort this list.
Create a function that sorts the mixed list above using the following logic:
- If the element is a string, the length of the string is used for sorting.
- If the element is a number, the number itself is used.
As proposed in the exercise, a custom sorting function named custom_sort is defined to handle the sorting logic. The function checks whether each element is a string or a number using the isinstance() function. If the element is a string, it returns the length of the string for sorting; if it's a number (integer or float), it returns the number itself.
The sorted() function is then used to sort the mixed_list using the logic defined in the custom sorting function.
If you’re having a hard time wrapping your head around custom sort functions, check out this article that details how to write a custom sort function in Python .
Python Practice Problem 15: Filter and Reorder
Given another list of strings, such as the one below ..
.. create a function that does two things: filters out any words with three or fewer characters and sorts the resulting list alphabetically.
Here, we define filter_and_sort , a function that does both proposed tasks.
First, it uses a for loop to filter out words with three or fewer characters, creating a filtered_list . Then, it sorts the filtered list alphabetically using the sorted() function, producing the final sorted_list .
The function returns this sorted list, which we print out.
Want Even More Python Practice Problems?
We hope these exercises have given you a bit of a coding workout. If you’re after more Python practice content, head straight for our courses on Python Data Structures in Practice and Built-in Algorithms in Python , where you can work on exciting practice exercises similar to the ones in this article.
Additionally, you can check out our articles on Python loop practice exercises , Python list exercises , and Python dictionary exercises . Much like this article, they are all targeted towards beginners, so you should feel right at home!
You may also like
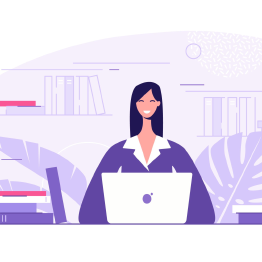
How Do You Write a SELECT Statement in SQL?
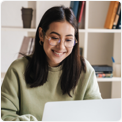
What Is a Foreign Key in SQL?

Enumerate and Explain All the Basic Elements of an SQL Query
Interested in a verified certificate or a professional certificate ?
An introduction to programming using a language called Python. Learn how to read and write code as well as how to test and “debug” it. Designed for students with or without prior programming experience who’d like to learn Python specifically. Learn about functions, arguments, and return values (oh my!); variables and types; conditionals and Boolean expressions; and loops. Learn how to handle exceptions, find and fix bugs, and write unit tests; use third-party libraries; validate and extract data with regular expressions; model real-world entities with classes, objects, methods, and properties; and read and write files. Hands-on opportunities for lots of practice. Exercises inspired by real-world programming problems. No software required except for a web browser, or you can write code on your own PC or Mac.
Whereas CS50x itself focuses on computer science more generally as well as programming with C, Python, SQL, and JavaScript, this course, aka CS50P, is entirely focused on programming with Python. You can take CS50P before CS50x, during CS50x, or after CS50x. But for an introduction to computer science itself, you should still take CS50x!
How to Take this Course
Even if you are not a student at Harvard, you are welcome to “take” this course for free via this OpenCourseWare by working your way through the course’s ten weeks of material. If you’d like to submit the course’s problem sets and final project for feedback, be sure to create an edX account , if you haven’t already. Ask questions along the way via any of the course’s communities !
- If interested in a verified certificate from edX , enroll at cs50.edx.org/python instead.
- If interested in a professional certificate from edX , enroll at cs50.edx.org/programs/python (for Python) or cs50.edx.org/programs/data (for Data Science) instead.
How to Teach this Course
If you are a teacher, you are welcome to adopt or adapt these materials for your own course, per the license .
Holy Python
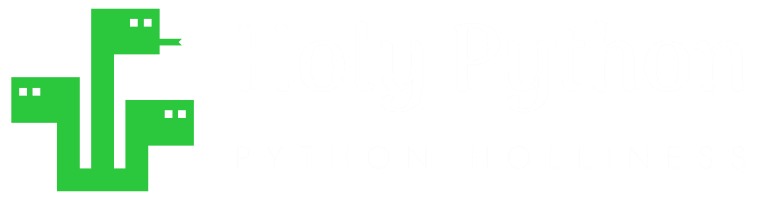
Beginner Python Exercises
Here are some enjoyable Python Exercises for you to solve! We strive to offer a huge selection of Python Exercises so you can internalize the Python Concepts through these exercises.
Among these Python Exercises you can find the most basic Python Concepts about Python Syntax and built-in functions and methods .
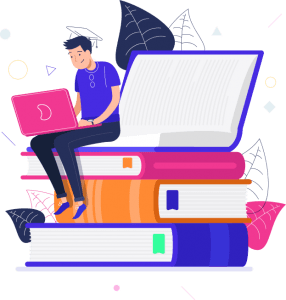
Holy Python is reader-supported. When you buy through links on our site, we may earn an affiliate commission.
Choose the topics you'd like to practice from our extensive exercise list.
Python Beginner Exercises consist of some 125+ exercises that can be solved by beginner coders and newcomers to the Python world.
Majority of these exercises are online and interactive which offers an easier and convenient entry point for beginners.
First batch of the exercises starts with print function as a warm up but quickly, it becomes all about data.
You can start exercising with making variable assignments and move on with the most fundamental data types in Python:
Exercise 1: print() function | (3) : Get your feet wet and have some fun with the universal first step of programming.
Exercise 2: Variables | (2) : Practice assigning data to variables with these Python exercises.
Exercise 3: Data Types | (4) : Integer (int), string (str) and float are the most basic and fundamental building blocks of data in Python.
Exercise 4: Type Conversion | (8) : Practice converting between basic data types of Python when applicable.
Exercise 5: Data Structures | (6) : Next stop is exercises of most commonly used Python Data Structures. Namely;
- Dictionaries and
- Strings are placed under the microscope.
Exercise 6: Lists | (14) : It’s hard to overdo Python list exercises. They are fun and very fundamental so we prepared lots of them. You will also have the opportunity to practice various Python list methods.
Exercise 7: Tuples | (8) : Python Tuples Exercises with basic applications as well as common tuples methods
Exercise 8: Dictionaries | (11) : Practice with Python Dictionaries and some common dictionary methods.
Exercise 9: Strings | (14) : Basic string operations as well as many string methods can be practiced through 10+ Python String Exercises we have prepared for you.
Next batch consist of some builtin Python functions and Python methods. The difference between function and method in Python is that functions are more like standalone blocks of codes that take arguments while methods apply directly on a class of objects.
That’s why we can talk about list methods, string methods, dictionary methods in Python but functions can be used alone as long as appropriate arguments are passed in them.
Exercise 10: len() function | (5) : Python’s len function tells the length of an object. It’s definitely a must know and very useful in endless scenarios. Whether it’s manipulating strings or counting elements in a list, len function is constantly used in computer programming.
Exercise 11: .sort() method | (7) : Practice sort method in Beginner Python Exercises and later on you’ll have the opportunity practice sorted function in Intermediate Python Exercises.
Exercise 12: .pop() method | (3) : A list method pop can be applied to Python list objects. It’s pretty straightforward but actually easy to confuse. These exercises will help you understand pop method better.
Exercise 13: input() function | (6) : Input function is a fun and useful Python function that can be used to acquire input values from the user.
Exercise 14: range() function | (5) : You might want to take a real close look at range function because it can be very useful in numerous scenarios.
These range exercises offer an opportunity to get a good practice and become proficient with the usage of Python’s range function.
Exercise 15: Error Handling | (7) : Error Handling is a must know coding skill and Python has some very explanatory Error Codes that will make a coder’s life easier if he/she knows them!
Exercise 16: Defining Functions | (9) : Practicing user defined Python functions will take your programming skills to the next step. Writing functions are super useful and fun. It makes code reusable too.
Exercise 17: Python Slicing Notation | (8) : Python’s slicing notations are very interesting but can be confusing for beginner coders. These exercises will help you master them.
Exercise 18: Python Operators | (6) : Operators are another fundamental concept. We have prepared multiple exercises so they can be confident in using Python Operators.
If you struggle with any of the exercises, you can always refer to the Beginner Python Lessons .
If you’d like to challenge yourself with the next level of Python exercises check out the Intermediate Python Exercises we have prepared for you.
FREE ONLINE PYTHON COURSES
Choose from over 100+ free online Python courses.
Python Lessons
Beginner lessons.
Simple builtin Python functions and fundamental concepts.
Intermediate Lessons
More builtin Python functions and slightly heavier fundamental coding concepts.
Advanced Lessons
Python concepts that let you apply coding in the real world generally implementing multiple methods.
Python Exercises
Beginner exercises.
Basic Python exercises that are simple and straightforward.
Intermediate Exercises
Slightly more complex Python exercises and more Python functions to practice.
Advanced Exercises
Project-like Python exercises to connect the dots and prepare for real world tasks.
Thank you for checking out our Python programming content. All of the Python lessons and exercises we provide are free of charge. Also, majority of the Python exercises have an online and interactive interface which can be helpful in the early stages of learning computer programming.
However, we do recommend you to have a local Python setup on your computer as soon as possible. Anaconda is a free distribution and it comes as a complete package with lots of libraries and useful software (such as Spyder IDE and Jupyter Notebook) You can check out this: simple Anaconda installation tutorial .

Say "Hello, World!" With Python Easy Max Score: 5 Success Rate: 96.24%
Python if-else easy python (basic) max score: 10 success rate: 89.71%, arithmetic operators easy python (basic) max score: 10 success rate: 97.41%, python: division easy python (basic) max score: 10 success rate: 98.68%, loops easy python (basic) max score: 10 success rate: 98.10%, write a function medium python (basic) max score: 10 success rate: 90.31%, print function easy python (basic) max score: 20 success rate: 97.27%, list comprehensions easy python (basic) max score: 10 success rate: 97.69%, find the runner-up score easy python (basic) max score: 10 success rate: 94.16%, nested lists easy python (basic) max score: 10 success rate: 91.69%, cookie support is required to access hackerrank.
Seems like cookies are disabled on this browser, please enable them to open this website
Top 10 Python Programming Homework Help Sites
Every student who wants to achieve good results in programming has to deal with ongoing homework challenges if they want to be truly successful in their academic studies. Classes in programming are not an exception. The thing about programming is that at times you may cope with it without completely understanding the entire process that led to the solution.
Understanding the series of your actions is the toughest aspect of this contemporary and challenging topic of study. And frequently, if not always, the most difficult part of this educational road comes down to resisting the urge to handle everything “blindly” and taking your time to fully understand all the aspects on your own. And in case you don’t have a personal teacher who can explain everything to you, this article offers you 10 websites where professionals can play the role of this teacher by helping you out with homework in programming. And particularly in Python.
1. BOOKWORM HUB
Website: BookwormHub.com
BookwormHub is a group of qualified professionals in many scientific areas. The list of their specializations comprises core fields including math, chemistry, biology, statistics, and engineering homework help . However, its primary focus is on the provision of programming homework assistance including python.
Ordering an entire component of your programming work is a pretty simple and straightforward process that consists of 4 steps:
- Submit your academic request on the website;
- Select a suitable expert that you personally like the most;
- Trace the progress of your order while your expert is working;
- Rate the level of provided assistance.
Besides homework services, BookwormHub also has a blog section that is mainly dedicated to python language and various branches of science that includes a lot of math. On top of that, the website has a 24/7 Customer Support system.
2. DO MY CODING
Website: DoMyCoding.com
One of the greatest places to go for timely, high-quality professional programming assignment assistance is the DoMyCoding website. This service has specialists in 5 key programming fields who can tackle and solve any degree of programming issues. The fields include:
- Java Script
It is never a bad idea to have DoMyCoding in your browser bookmarks since you never know what level of difficulty you may encounter when you obtain a challenging homework project in the future. And it can be especially useful if you have programming coursework that is due at the end of the semester.
3. ASSIGN CODE
Website: AssignCode.com
An established service called AssignCode can handle programming assignments of any level of complexity and was created to meet the guarantees of raising your GPA. The reason they are successful at it is that they have expertise in math, chemistry, physics, biology and engineering in addition to 5 main branches of programming.
You may choose the expert from Assign Code whose accomplishments and milestones most closely match your individual needs by scrolling through the list of their specialists. Therefore, you will have more control over the process of solving your programming issues. Plus, the service’s money-back guarantee is the ideal feature for you as a customer in case you are dissatisfied with any of your orders.
4. CW ASSIGNMENTS
Website: CWAassignments.com
CWAassignments is mostly a narrowly focused programming writing services website that provides homework assistance to IT-oriented students. It has a lot to offer in terms of its professional capabilities. The following categories are among the wide range of offerings they provide to their programming clients:
- Computer science
- R programming
- Data Science
- Computer network
Besides covering everything that relates to IT CWAassignments also offer services on other closely-related or unrelated subjects, such as Math, Calculus, Algebra, Geometry, Chemistry, Engineering, Aviation, Excel, Finance, Accounting, Management, Nursing, Visual basics, and many more.
5. ASSIGNMENT CORE
Website: AssignmentCore.com
Another excellent service that is largely focused on programming assistance but can also handle writing assignments for other fields is AssignmentCore. In particular, it covers Architecture and Design, as well as several subfields of Business and Management, Engineering, Mathematics, and Natural Science.
The list of specifically programmed assistance services includes the following divisions:
- Computer and Web Programming
- Mobile Application Development
- Computer Networking and Cybersecurity
- Data Science and Analysis
The list of slightly-programming-related help services includes:
- Digital Design, UX, and Visual Design
- Database Design and Optimisation
- QA and Software testing
6. LOVELY CODING
Website: LovelyCoding.com
Specialists at LovelyCoding are ready to take on whatever programming issues you throw at them, figure out how to solve them and make the whole thing seem simple, clear, and easy to grasp. The service offers a three-step quality control process and a mechanism for constant client assistance. Three steps make up the request process: submitting the criteria, paying the fee, and receiving the tasks once they have been finished.
Regarding their specialization, the website divided into three sections of programming help.
Software Development Help
- System software
- Application software
- Programming languages
Programming Help
- HTML & CSS
- Machine Learning & R
Project Help
- Software Development
- Web Development
- App Development
- Computer Science
7. FAV TUTOR
Website: FavTutor.com
FavTutor is another strictly narrow-specialized programming help-oriented website that deserves to be mentioned in this article. Its main difference from the previous websites is that it provides help in another format. It is not a task-handling service, but a discipline-explaining service.
Here you have live tutors on Java and Python who will explain the subject personally and in detail. The process comes down to three steps:
- Share your problem
- We assign the best tutor
- Live and 1:1 sessions
You can connect with their teachers at any time of day thanks to the live sessions and their availability around the clock. Besides online tutoring, it also offers you a pack of three complete programming courses which can help you to become more knowledgeable and IT-independent.
- Python for Beginners
- Java Programming
8. LET’S TACLE
Website: LetsTacle.com
LetsTacle is a website that was created specifically to help students with any programming issues that may arise in their college course or process of individual studying. It has positive reviews mainly because of the simplicity of the cute design and a number of highly qualified experts in each programming field. The list of subjects they specialize in includes the following:
- Live Programming Help
- Computer Science Homework Help
- Python Homework Help
- Java Homework Help
- C++ Homework Help
- R Homework Help
- PHP Homework Help
- HTML Homework Help
- JavaScript Homework Help
- SQL Homework Help
- Do My Programming Homework
- Android Assignment Help
Besides the standard pack of homework help services LetsTacle also offers articles and Academy service, which is an option of buying services of a personal Online Python Tutor.
9. HOMEWORK HELP ONLINE
Website: HomeworkHelpOnline.net
HomeworkHelpOnline is a unique programming homework help website in the way that it offers you to order a complete assignment, but it also navigates you on how you can cope with your tasks yourself in their self-help section. HomeworkHelpOnline works in 4 directions:
- Programming
Each direction consists of a large number of branches. The programming includes the following:
- R / Statistics
- SQL Database
- Neural Networks
10. ALL ASSIGNMENT HELP
Website: AllAssignmentHelp.com
The academic assistance website AllassignmentHelp focuses on several math-related and IT-related disciplines. Along with providing assistance with completing tasks that include programming in Python, this site also provides many helpful tools and resources. The resources in the list consist of:
- Free samples
- Question bank
- Academy courses
Additionally, it provides resources like a Reference Generator, Word Counts, Grammar Checker, and Free Plagiarism Report. All of which are extremely helpful and beneficial for academic writing of any kind.
Python Online Compiler
Python Programming
Homework help & tutoring.
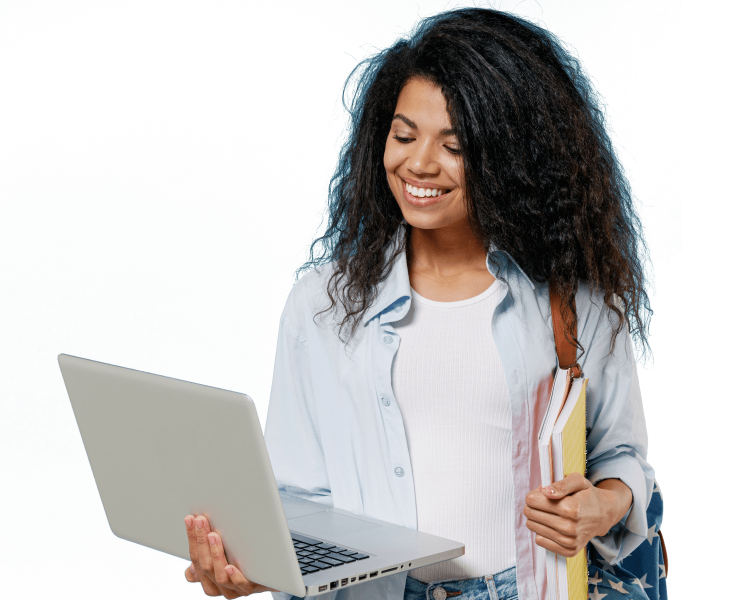
Our name 24HourAnswers means you can submit work 24 hours a day - it doesn't mean we can help you master what you need to know in 24 hours. If you make arrangements in advance, and if you are a very fast learner, then yes, we may be able to help you achieve your goals in 24 hours. Remember, high quality, customized help that's tailored around the needs of each individual student takes time to achieve. You deserve nothing less than the best, so give us the time we need to give you the best.
If you need assistance with old exams in order to prepare for an upcoming test, we can definitely help. We can't work with you on current exams, quizzes, or tests unless you tell us in writing that you have permission to do so. This is not usually the case, however.
We do not have monthly fees or minimum payments, and there are no hidden costs. Instead, the price is unique for every work order you submit. For tutoring and homework help, the price depends on many factors that include the length of the session, level of work difficulty, level of expertise of the tutor, and amount of time available before the deadline. You will be given a price up front and there is no obligation for you to pay. Homework library items have individual set prices.
We accept credit cards, debit cards, PayPal, Venmo, ApplePay, and GooglePay.
Python is a popular programming language dating back to the early 90s. It is supported by a large number of frameworks, particularly in the web sphere, and designed to maximize code readability. The most recent version is Python 3, which differs from Python 2 due to its improvements that make code easier to write. As a language, Python is largely used as dynamically typed, object oriented and procedural, but it is multi-paradigm and also supports functional programming and strong typing. It is also reflective, which means Python programs are able to modify themselves during execution.
Python Online Tutors
If you're looking for extra Python homework help online, there are many platforms and services that you can turn to for help. Whether you need assistance with Python homework or you want to become a more proficient programmer, there are plenty of ways to improve your coding skill set when it comes to Python programming.
One of the most effective strategies for receiving Python help is working with a professional Python tutor. Tutors can provide individualized guidance to ensure you receive the support you need to become more confident in your programming skills and cultivate the ability to use Python in a variety of applications.
When searching for a Python tutor online, finding a qualified individual with a background in programming is an essential part of improving your education and developing the ability to use these skills in a real-life setting. The right tutor will be able to adapt their instruction for your learning, ensuring that you get the most out of your Python tutoring and gain more confidence in programming inside and outside the classroom.
Python Programming Homework Help With 24HourAnswers
Whenever you're consulting the internet for additional information, it is essential to confirm the source you're using is trustworthy. With our service, you gain access to a diverse range of highly accredited and knowledgeable P ython private tutors online , all of whom are reliable and professionally qualified. Our Python tutors are also available around the clock to answer any questions you have about Python programming.
Some of the ways you can use our online Python homework help include:
- Preparing for an upcoming exam or quiz.
- Asking detailed questions about programming.
- Getting additional help with Python homework.
- Inquiring about the different applications for Python programming.
- Checking your code with a professional programmer.
- Building more confidence in your Python programming abilities.
In addition to the multiple applications of our programming tutoring services, our highly accomplished tutors will go above and beyond to help you with any Python homework or assignment. They can tailor their teaching style to your unique preferences since you'll work with them individually. These one-on-one tutoring sessions ensure that you can get the most out of every lesson, giving you all the tools you need to succeed in the classroom.
Receive Python Homework Help Anytime
Because we're available 24 hours a day, you can turn to us for help with Python homework at any time — even if it's the middle of the night and you need a professional Python programmer to walk you through an assignment or answer a question. As we're an online source of information, you won't have to search for a Python tutor in your local area, eliminating any obstacles that could prevent you from getting the Python homework help you need.
We're a trusted source for college students due to our unwavering dedication to helping you get the most out of your classes and college-level education.Working with us provides a service you won't get anywhere else, allowing you to gain a comprehensive knowledge of Python programming as you work with one of our qualified tutors.
Python Tutorial Help
Python programs are usually written in .py files. The language is split into a number of implementations, including CPython, Jython and IronPython written in C, Java and C# respectively. These different interpretations add extra pieces of functionality and quirks - such as IronPython is able to make use of the .NET Framework, and JPython integrates with Java classes. The core concepts remain the same.
Having set up your environment or installed an IDE, you are ready to start writing Python applications. You can follow the tutorial below to learn some core operations that can be performed in Python. Note that the lines beginning with # are comments, which means they do not get executed in your application. The best way to learn is to type out these programs yourself (rather than copy-paste) as you’ll get a better feel for what it means to write Python.
# First, let's do a basic print operation
print ("Hello, world!")
If you run this application you’ll see the text string in the brackets outputs to the console. This is because ‘print()’ is a method, and we are calling it with an argument (our text).
We can make this a bit more advanced by introducing variables.
# Create a variable and print it
name = "Dave"
print ("Hello " + name)
The + operator when used on text strings concatenates them. As a result, we will see an output based on the constant “Hello “ being joined with the name in the variable. If, however, we add numbers together we will instead perform a mathematical operation:
# Let's do some sums
print (1 + 2)
print (1 - 2)
print (9 / 3)
print (2 * 5)
# Two *s raises the number before to the power of the number after
print ((2 * 5) ** 2)
One thing you’ll notice is that the division operator converts the result to a floating point number (3.0) whereas the other operations remain as integers. If, however, you use a floating point number in the calculation you’ll create a floating point result, as in this example:
# Output will be 10.0, not 10
print (2.0 * 5)
We briefly touched on variables before. It is important to assign values to a variable before you use it, or you will receive an error:
# This is OK
myVariable = 3
print (myVariable)
# This is not
print (aNewVariable)
We can also do conditional operations on our variables. Note that the indentation is used to separate code blocks, and until we de-indent our program, we continue to operate inside the ‘if’ block.
# The interior statements will execute as myVariable (3) is
# greater than or equal to 3
if myVariable >= 3:
print ("Condition passed")
print ("myVariable is more than 2")
# As a result, this won't execute
print ("Condition failed")
print ("myVariable is less than 3")
In this next example we’ll create a list by using square brackets and then iterate over it by using the ‘for’ keyword. This extracts each value in the list, and puts it in the variable ‘number’ before executing the block of code within the 'for' loop. Then the code moves on to the next item in the list:
# Let's count!
for number in [1,2,3,4,5]:
print(number)
The Python code you encounter in the world will consist of these elements - methods, loops, conditions and variables. Understanding these fundamentals is core to being able to move on to more advanced concepts and frameworks, as well as being able to read and write your own Python applications.
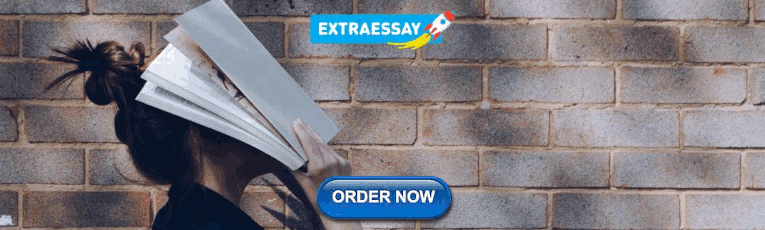
Reach out for Python Tutoring Online
To learn why our Python help is so effective, schedule a session with our online Python tutors today. Unlike other online sources, our tutors can be trusted to fulfill multiple needs and enhance your education. With their professional backgrounds and advanced degrees in coding, they'll make sure you receive the accurate information and coding advice you're looking for.
Whether you're just starting out or you're an advanced Python programmer, we have a tutor that can help you master your Python programming coursework. If you're stuck on a question, submit your homework and one of our Python tutors will help you solve it! If you just want some extra help, contact a Python programming tutor for an online tutoring session. We also have a homework library so that you can get some extra homework practice whenever you need it.
To fulfill our tutoring mission of online education, our college homework help and online tutoring centers are standing by 24/7, ready to assist college students who need homework help with all aspects of Python programming. Our Python tutors can help with all your projects, large or small, and we challenge you to find better online Python programming tutoring anywhere.
College Python Programming Homework Help
Since we have tutors in all Python Programming related topics, we can provide a range of different services. Our online Python Programming tutors will:
- Provide specific insight for homework assignments.
- Review broad conceptual ideas and chapters.
- Simplify complex topics into digestible pieces of information.
- Answer any Python Programming related questions.
- Tailor instruction to fit your style of learning.
With these capabilities, our college Python Programming tutors will give you the tools you need to gain a comprehensive knowledge of Python Programming you can use in future courses.
24HourAnswers Online Python Programming Tutors
Our tutors are just as dedicated to your success in class as you are, so they are available around the clock to assist you with questions, homework, exam preparation and any Python Programming related assignments you need extra help completing.
In addition to gaining access to highly qualified tutors, you'll also strengthen your confidence level in the classroom when you work with us. This newfound confidence will allow you to apply your Python Programming knowledge in future courses and keep your education progressing smoothly.
Because our college Python Programming tutors are fully remote, seeking their help is easy. Rather than spend valuable time trying to find a local Python Programming tutor you can trust, just call on our tutors whenever you need them without any conflicting schedules getting in the way.
Python Lab Assignments
You can not learn a programming language by only reading the language construct. It also requires programming - writing your own code and studying those of others. Solve these assignments, then study the solutions presented here.
CS50's Introduction to Artificial Intelligence with Python
Learn to use machine learning in Python in this introductory course on artificial intelligence.
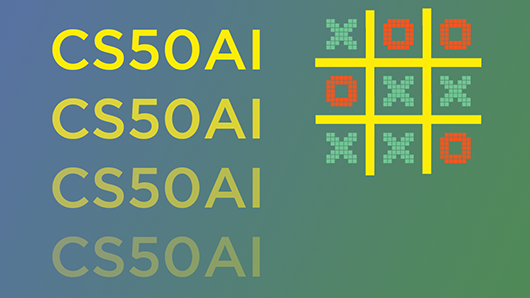
Associated Schools
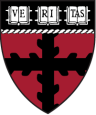
Harvard School of Engineering and Applied Sciences
What you'll learn.
Graph search algorithms
Reinforcement learning
Machine learning
Artificial intelligence principles
How to design intelligent systems
How to use AI in Python programs
Course description
AI is transforming how we live, work, and play. By enabling new technologies like self-driving cars and recommendation systems or improving old ones like medical diagnostics and search engines, the demand for expertise in AI and machine learning is growing rapidly. This course will enable you to take the first step toward solving important real-world problems and future-proofing your career.
CS50’s Introduction to Artificial Intelligence with Python explores the concepts and algorithms at the foundation of modern artificial intelligence, diving into the ideas that give rise to technologies like game-playing engines, handwriting recognition, and machine translation. Through hands-on projects, students gain exposure to the theory behind graph search algorithms, classification, optimization, reinforcement learning, and other topics in artificial intelligence and machine learning as they incorporate them into their own Python programs. By course’s end, students emerge with experience in libraries for machine learning as well as knowledge of artificial intelligence principles that enable them to design intelligent systems of their own.
Enroll now to gain expertise in one of the fastest-growing domains of computer science from the creators of one of the most popular computer science courses ever, CS50. You’ll learn the theoretical frameworks that enable these new technologies while gaining practical experience in how to apply these powerful techniques in your work.
Instructors
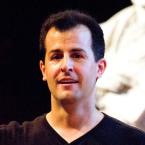
David J. Malan
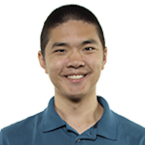
You may also like
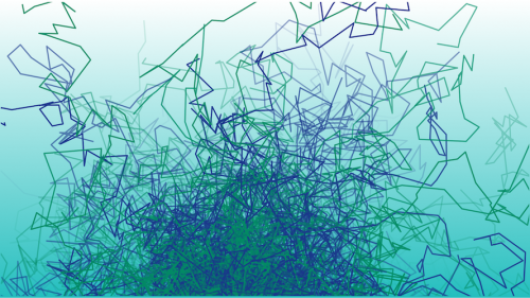
Using Python for Research
Take your introductory knowledge of Python programming to the next level and learn how to use Python 3 for your research.
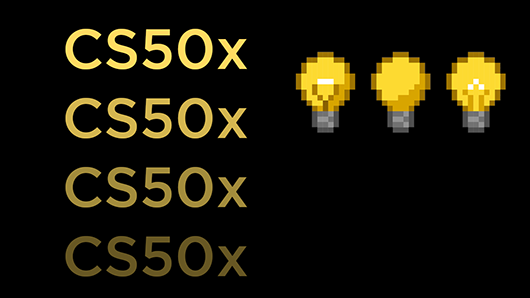
CS50: Introduction to Computer Science
An introduction to the intellectual enterprises of computer science and the art of programming.
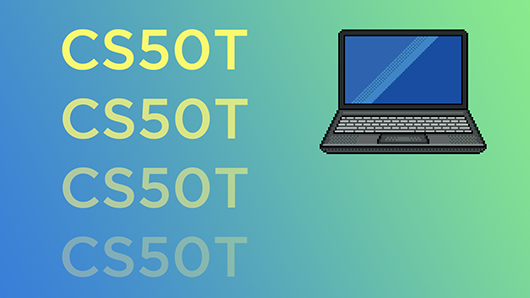
CS50's Understanding Technology
This is CS50’s introduction to technology for students who don’t (yet!) consider themselves computer persons.
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python Operators
Precedence and associativity of operators in python.
- Python Arithmetic Operators
- Difference between / vs. // operator in Python
- Python - Star or Asterisk operator ( * )
- What does the Double Star operator mean in Python?
- Division Operators in Python
- Modulo operator (%) in Python
- Python Logical Operators
- Python OR Operator
- Difference between 'and' and '&' in Python
- not Operator in Python | Boolean Logic
Ternary Operator in Python
- Python Bitwise Operators
Python Assignment Operators
Assignment operators in python.
- Walrus Operator in Python 3.8
- Increment += and Decrement -= Assignment Operators in Python
- Merging and Updating Dictionary Operators in Python 3.9
- New '=' Operator in Python3.8 f-string
Python Relational Operators
- Comparison Operators in Python
- Python NOT EQUAL operator
- Difference between == and is operator in Python
- Chaining comparison operators in Python
- Python Membership and Identity Operators
- Difference between != and is not operator in Python
In Python programming, Operators in general are used to perform operations on values and variables. These are standard symbols used for logical and arithmetic operations. In this article, we will look into different types of Python operators.
- OPERATORS: These are the special symbols. Eg- + , * , /, etc.
- OPERAND: It is the value on which the operator is applied.
Types of Operators in Python
- Arithmetic Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Identity Operators and Membership Operators
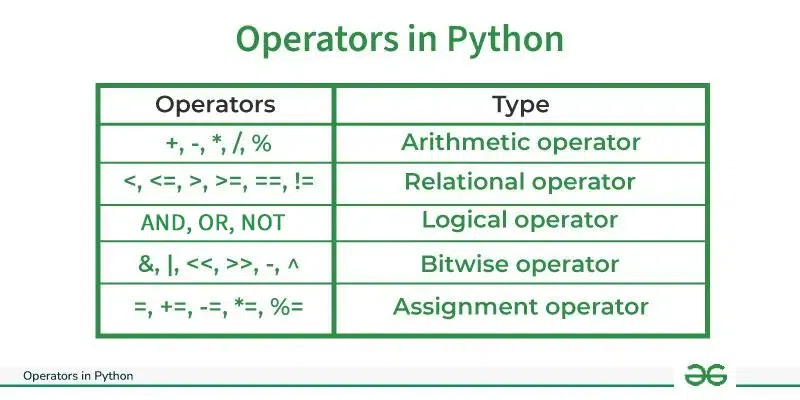
Arithmetic Operators in Python
Python Arithmetic operators are used to perform basic mathematical operations like addition, subtraction, multiplication , and division .
In Python 3.x the result of division is a floating-point while in Python 2.x division of 2 integers was an integer. To obtain an integer result in Python 3.x floored (// integer) is used.
Example of Arithmetic Operators in Python
Division operators.
In Python programming language Division Operators allow you to divide two numbers and return a quotient, i.e., the first number or number at the left is divided by the second number or number at the right and returns the quotient.
There are two types of division operators:
Float division
- Floor division
The quotient returned by this operator is always a float number, no matter if two numbers are integers. For example:
Example: The code performs division operations and prints the results. It demonstrates that both integer and floating-point divisions return accurate results. For example, ’10/2′ results in ‘5.0’ , and ‘-10/2’ results in ‘-5.0’ .
Integer division( Floor division)
The quotient returned by this operator is dependent on the argument being passed. If any of the numbers is float, it returns output in float. It is also known as Floor division because, if any number is negative, then the output will be floored. For example:
Example: The code demonstrates integer (floor) division operations using the // in Python operators . It provides results as follows: ’10//3′ equals ‘3’ , ‘-5//2’ equals ‘-3’ , ‘ 5.0//2′ equals ‘2.0’ , and ‘-5.0//2’ equals ‘-3.0’ . Integer division returns the largest integer less than or equal to the division result.
Precedence of Arithmetic Operators in Python
The precedence of Arithmetic Operators in Python is as follows:
- P – Parentheses
- E – Exponentiation
- M – Multiplication (Multiplication and division have the same precedence)
- D – Division
- A – Addition (Addition and subtraction have the same precedence)
- S – Subtraction
The modulus of Python operators helps us extract the last digit/s of a number. For example:
- x % 10 -> yields the last digit
- x % 100 -> yield last two digits
Arithmetic Operators With Addition, Subtraction, Multiplication, Modulo and Power
Here is an example showing how different Arithmetic Operators in Python work:
Example: The code performs basic arithmetic operations with the values of ‘a’ and ‘b’ . It adds (‘+’) , subtracts (‘-‘) , multiplies (‘*’) , computes the remainder (‘%’) , and raises a to the power of ‘b (**)’ . The results of these operations are printed.
Note: Refer to Differences between / and // for some interesting facts about these two Python operators.
Comparison of Python Operators
In Python Comparison of Relational operators compares the values. It either returns True or False according to the condition.
= is an assignment operator and == comparison operator.
Precedence of Comparison Operators in Python
In Python, the comparison operators have lower precedence than the arithmetic operators. All the operators within comparison operators have the same precedence order.
Example of Comparison Operators in Python
Let’s see an example of Comparison Operators in Python.
Example: The code compares the values of ‘a’ and ‘b’ using various comparison Python operators and prints the results. It checks if ‘a’ is greater than, less than, equal to, not equal to, greater than, or equal to, and less than or equal to ‘b’ .
Logical Operators in Python
Python Logical operators perform Logical AND , Logical OR , and Logical NOT operations. It is used to combine conditional statements.
Precedence of Logical Operators in Python
The precedence of Logical Operators in Python is as follows:
- Logical not
- logical and
Example of Logical Operators in Python
The following code shows how to implement Logical Operators in Python:
Example: The code performs logical operations with Boolean values. It checks if both ‘a’ and ‘b’ are true ( ‘and’ ), if at least one of them is true ( ‘or’ ), and negates the value of ‘a’ using ‘not’ . The results are printed accordingly.
Bitwise Operators in Python
Python Bitwise operators act on bits and perform bit-by-bit operations. These are used to operate on binary numbers.
Precedence of Bitwise Operators in Python
The precedence of Bitwise Operators in Python is as follows:
- Bitwise NOT
- Bitwise Shift
- Bitwise AND
- Bitwise XOR
Here is an example showing how Bitwise Operators in Python work:
Example: The code demonstrates various bitwise operations with the values of ‘a’ and ‘b’ . It performs bitwise AND (&) , OR (|) , NOT (~) , XOR (^) , right shift (>>) , and left shift (<<) operations and prints the results. These operations manipulate the binary representations of the numbers.
Python Assignment operators are used to assign values to the variables.
Let’s see an example of Assignment Operators in Python.
Example: The code starts with ‘a’ and ‘b’ both having the value 10. It then performs a series of operations: addition, subtraction, multiplication, and a left shift operation on ‘b’ . The results of each operation are printed, showing the impact of these operations on the value of ‘b’ .
Identity Operators in Python
In Python, is and is not are the identity operators both are used to check if two values are located on the same part of the memory. Two variables that are equal do not imply that they are identical.
Example Identity Operators in Python
Let’s see an example of Identity Operators in Python.
Example: The code uses identity operators to compare variables in Python. It checks if ‘a’ is not the same object as ‘b’ (which is true because they have different values) and if ‘a’ is the same object as ‘c’ (which is true because ‘c’ was assigned the value of ‘a’ ).
Membership Operators in Python
In Python, in and not in are the membership operators that are used to test whether a value or variable is in a sequence.
Examples of Membership Operators in Python
The following code shows how to implement Membership Operators in Python:
Example: The code checks for the presence of values ‘x’ and ‘y’ in the list. It prints whether or not each value is present in the list. ‘x’ is not in the list, and ‘y’ is present, as indicated by the printed messages. The code uses the ‘in’ and ‘not in’ Python operators to perform these checks.
in Python, Ternary operators also known as conditional expressions are operators that evaluate something based on a condition being true or false. It was added to Python in version 2.5.
It simply allows testing a condition in a single line replacing the multiline if-else making the code compact.
Syntax : [on_true] if [expression] else [on_false]
Examples of Ternary Operator in Python
The code assigns values to variables ‘a’ and ‘b’ (10 and 20, respectively). It then uses a conditional assignment to determine the smaller of the two values and assigns it to the variable ‘min’ . Finally, it prints the value of ‘min’ , which is 10 in this case.
In Python, Operator precedence and associativity determine the priorities of the operator.
Operator Precedence in Python
This is used in an expression with more than one operator with different precedence to determine which operation to perform first.
Let’s see an example of how Operator Precedence in Python works:
Example: The code first calculates and prints the value of the expression 10 + 20 * 30 , which is 610. Then, it checks a condition based on the values of the ‘name’ and ‘age’ variables. Since the name is “ Alex” and the condition is satisfied using the or operator, it prints “Hello! Welcome.”
Operator Associativity in Python
If an expression contains two or more operators with the same precedence then Operator Associativity is used to determine. It can either be Left to Right or from Right to Left.
The following code shows how Operator Associativity in Python works:
Example: The code showcases various mathematical operations. It calculates and prints the results of division and multiplication, addition and subtraction, subtraction within parentheses, and exponentiation. The code illustrates different mathematical calculations and their outcomes.
To try your knowledge of Python Operators, you can take out the quiz on Operators in Python .
Python Operator Exercise Questions
Below are two Exercise Questions on Python Operators. We have covered arithmetic operators and comparison operators in these exercise questions. For more exercises on Python Operators visit the page mentioned below.
Q1. Code to implement basic arithmetic operations on integers
Q2. Code to implement Comparison operations on integers
Explore more Exercises: Practice Exercise on Operators in Python
Please Login to comment...
Similar reads.
- python-basics
- Python-Operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
TechConnect: *Online: Fundamentals of Programming with Python Part 1
This event will take place online via Zoom. Must register with a valid email address.
You may join the class session using this link: https://nypl.zoom.us/j/84780081928?pwd=NjFhckQycE9LSkRmOXYwS0diTVhnQT09
Learn the fundamentals of computer programming with the popular language Python. This class will cover concepts fundamental to all programming languages and provide an introduction to coding more generally. This class requires a gmail account to use Google Colab, a browser based coding environment.
You will receive an email 2 hours prior to the start of the class with the link to join the virtual class.
*Please note that some people using older or less common email addresses have experienced issues with receiving emails from TechConnect. Using a Gmail account will prevent this in most instances.
- Class Format: Hands on
- Skill prerequisites: Basic computer skills
- Audience: Adults
Version 10.0 | Substance 3D Painter
- Substance 3D home
- Getting Started overview
- Activation and licenses
- System requirements
- Project creation
- Export overview
- Export window overview
- Export settings
- Output templates
- List of exports
- Export presets overview
- Predefined Presets
- USD PBR Metal Roughness Preset
- Default Presets
- Creating export presets
- Performance
- Assets overview
- Customizing the layout
- Filter by path
- Saved searches
- Advanced search queries
- Adding a new library
- Sub-library tab
- Substance 3D Assets
- Color picker
- Display settings overview
- Environment settings
- Camera settings
- Viewport settings
- Layer stack overview
- Creating layers
- Managing layers
- Masking and effects
- Blending modes
- Layer instancing
- Geometry mask
- Main menu overview
- Window menu
- Viewport menu
- Plugins menu
- Project configuration
- Settings overview
- General preferences
- Libraries configuration
- Shader settings overview
- Updating a shader
- Texture Set overview
- Texture Set list
- Texture Set settings
- Texture Set reassignment
- Viewport overview
- Camera management
- Update checker
- Painting overview
- Paint brush
- Polygon fill
- Smudge tool
- Straight line
- Lazy mouse
- Symmetry overview
- Mirror Symmetry
- Fill projections overview
- Fill (match per UV Tile)
- UV projection
- Tri-planar projection
- Planar projection
- Spherical projection
- Cylindrical projection
- Warp projection
- UV set to UV set projection
- Presets overview
- Creating and saving presets
- Creating particles overview
- Installing the particle editor
- Overview of the particle editor
- Creating a new particle script
- Photoshop brush presets overview
- Exporting Brush Presets from Photoshop
- Importing Photoshop Brush Presets
- Photoshop Brush Parameters Compatibility
- Dynamic strokes overview
- Enabling Dynamic Stroke feature
- Dynamic Stroke Performances
- Creating custom Dynamic Strokes
- Channel painting overview
- Ambient Occlusion
- Vector graphic (.svg & .ai)
- Text resource
- Effects overview
- Compare Mask
- Anchor Point
- Baking overview
- How to bake mesh maps
- Baking visualization settings
- Creating custom effects overview
- Generic filter
- Channel specific filter
- Mesh based input
- Adding resources via drag and drop
- Adding resources via the import window
- Adding content on the hard drive
- Receiving assets from other Substance 3D applications
- Automatic UV Unwrapping
- Physical size
- Smart Materials and Masks
- Subsurface Scattering overview
- Enabling Subsurface in a Project
- Subsurface Parameters
- Subsurface Material Type
- Dynamic Material Layering
- UV Reprojection
- UV Tiles overview
- Image Sequence
- Color management overview
- Color management with Adobe ACE - ICC
- Color management with OpenColorIO
- Post Processing overview
- Color correction
- Depth of Field
- Lens Distortion
- Tone Mapping
- Color Profile
- Iray Renderer overview
- Iray Settings
- Viewer and MDL Settings
- Plugins overview
- Resources Updater
- Sparse Virtual Textures
- Custom Shaders
- SpaceMouse® by 3Dconnexion
- Universal Scene Description (USD)
- Performances guidelines overview
- Conflicts and background applications
- Mesh and UV setup
- GPU Drivers
- NVIDIA Drivers Settings
- GPU VRAM amount and bandwidth
- GPU VRAM and other applications
- Texture Sets amount
- Layer management
- Channels management
- Substance filters and materials
- Viewport display
- Configuring Pens and Tablets
- Exporting the log file
- Exporting a DXDiag
- Crash when working with overclocked GPU
- Forcing the external GPU on Mac OS
- GPU drivers compatibility
- GPU drivers crash with long computations (TDR crash)
- GPU has outdated drivers
- GPU is not recognized
- GPU is not recognized and is mentionned as GDI Generic
- Issues with Nvidia GPUs on recent Mac OS versions
- Multi/Bi-GPU
- Running on integrated GPU
- Painter doesn't start on the right GPU
- Application failed to start because of Qt
- Crash or freeze during startup
- Software conflicts
- Artifacts and glitches on Mac OS with Custom GPUs
- Blocky artifacts appear on textures in the viewport
- Mesh appears pink in the viewport
- Mesh flash to white when moving camera
- Some HDPI scaling values are not working
- Crash during export
- Crash when opening or saving a file
- Crash while baking
- Crash with low virtual memory
- Windows Blue Screens
- Corrupted texture error message
- Shelf resources are gone after 7.2 update
- Error there is no disk in the drive
- Error with missing api-ms-crt dll
- Impossible to drag and drop files into the shelf
- Impossible to use the ALT keyboard shortcut on Linux
- Assets (or shelf) previews are empty
- My exported opacity map is totally black
- Texture dilation or Padding
- Normal map looks incorrect when loaded in layer or tool properties
- Paint Tool bleeds on other UV islands
- A project has been processed as a text file and is now corrupted
- Loading files from a network
- Preserve brush strokes setting stays disabled
- Projects are really big
- Thumbnails in the shelf look incorrect
- Error when importing a Font
- Mesh faces disappear when looking at them from behind
- Viewports and textures are blurry or lack sharpness
- Substance Source plugin doesn't load
- Maintenance is expired dialog on startup
- Preferences and application data location
- Automated installation
- Retrieving the installation path
- Command lines
- Environment variables
- Firewall Configuration
- Querying Current Software Version
- Remote Desktop
- Shelf and Assets location
- Adding resource paths by editing preferences manually
- Editing resource paths manually
- Editing the Shelf Preferences with Python
- Adding saved searches manually
- Preferences and content migration
- Excluding resources in a resource path
- Creating a Javascript plugin
- Remote control with scripting
- Shader API overview
- Changelog - Shader API
- Lib Alpha - Shader API
- Lib Bayer - Shader API
- Lib Defines - Shader API
- Lib Emissive - Shader API
- Lib Env - Shader API
- Lib Normal - Shader API
- Lib PBR - Shader API
- Lib PBR Aniso - Shader API
- Lib Pom - Shader API
- Lib Random - Shader API
- Lib Sampler - Shader API
- Lib Sparse - Shader API
- Lib SSS - Shader API
- Lib Utils - Shader API
- Lib Vectors - Shader API
- All Custom Params - Shader API
- All Engine Params - Shader API
- All Rendering States Params - Shader API
- Layering Bind Materials - Shader API
- Layering Declare Stacks - Shader API
- PBR Material Layering - Shader API
- PBR Metal Rough - Shader API
- Pixelated - Shader API
- Surface Shader - Shader API
- Toon - Shader API
- Release notes overview
- All Changes
Version 10.0
- Version 9.1
- Version 9.0
- Version 8.3
- Version 8.2
- Version 8.1
- Version 7.4
- Version 7.3
- Version 7.2
- Version 2021.1 (7.1.0)
- Version 2020.2 (6.2.0)
- Version 2020.1 (6.1.0)
- Version 2019.3
- Version 2019.2
- Version 2019.1
- Version 2018.3
- Version 2018.2
- Version 2018.1
- Version 2017.4
- Version 2017.3
- Version 2017.2
- Version 2017.1
- Version 2.6
- Version 2.5
- Version 2.4
- Version 2.3
- Version 2.2
Substance 3D Painter 10.0 brings support of Illustrator (.ai) files, integrates Substance 3D Assets, imports Fonts via the Text resources, adds layer stack functionalities in the Python API, and several quality of life improvements.
Release date: 16 May 2024
Major features
New text resource.
This new version introduces the Text resource which is a way to load font files to write text in different contexts (brush, fill projection, Substance image inputs, etc.) to embellish your textures.
Browse your fonts in the Assets window Fonts are now listed in the Assets window under their own filter. They are gathered from different locations on the operating system (and also from the Libraries).
Drag and drop fonts like any other resources Fonts can be used as text resources like any other kind of resource. Drag and drop them to automatically create fill projection. They can also be used in brushes or as input in Substance filters.
Text resource parameters When creating a text resource, you can tweak a few parameters to adjust the look of your text: vertical and horizontal alignment, automatic or manual size, line and character spacing, color, etc.
Wide range of characters and feature supported The Text resource supports right to left writing as well as ligatures . (To be able to write non latin characters a compatible font is required.)
Import custom fonts like regular resource You can import your own fonts files directly into your Library or project like any other resources. Some types of fonts are not supported however, for more information see this documentation page .
For more information about the Text resource , see the dedicated documentation page .
New import of Illustrator files (.Ai)
Following the support for .svg files, this new version also adds the ability to import Illustrator files ( .ai ).
Illustrator (.Ai) file support In this new version .ai files can now be imported and rendered in Painter to be used as resource in brushes, fill projections or as Substance image inputs.
.svg and .ai files share common settings SVG and Illustrator documents share similar settings, notably the resolution, crop area and scope selection parameters. This means that vectorial resource can be managed in a similar way.
Artboard selection Illustrator documents support artboard , when using an .ai file you can also choose between different artboards available via the dedicated setting.
Improved scope selection The scope selection window has been improved with the support of thumbnails, making it easier to browse and select only specific elements. For performance reasons thumbnails are off by default and can be enabled with the Show thumbnails checkbox.
Importing Illustrator ( .ai ) files is currently only supported on Windows and MacOS.
New Substance 3D Assets integration
A new window is available which embeds the Substance 3D Assets website directly inside Painter. This integration makes it easier to browse and download resources directly in your own library.
New Substance 3D Assets window A new dock is available in the interface to browse Substance 3D Assets. If the dock is not visible and closed it can be found again in the dock toolbar on the right of the interface.
Download manager You can see the assets currently being downloaded via the dedicated manager using the bottom left button of the window. Assets that may fail to download can be started again from this list.
Find your downloaded assets easily The button in the bottom right of the window opens a menu with a few actions to help navigate the website but also to shows were assets have been donwloaded.
Upon the first launch a login into your account will be necessary to download assets. This login is then be cached for future uses.
The Substance 3D Assets dock is not available in the Steam version.
New layer stack module in Python API
This release sees the addition of the new layer stack module in our Python API. This API allows to control the layer stack of a project, opening the door to the creation of advanced layer stack plugins and custom tools.
New Layer stack API The new layerstack module allows to control the layer stack of a project in many ways. You can:
- Query and set the selection of layers and effects.
- Create new layers, folders and effects (including filters, anchor points, etc.).
- Instantiate layers.
- Get and set parameters of layers and effects, load resources into them.
- Get and set Substance parameters.
Scoped modifications and pause of the engine Manipulating the layer stack could lead to long computations, this is why we also exposed the possibility to pause and unpause the engine from the API (like in the UI). We also made it possible to group modifications together, for both performance reasons but also to undo a single time multiple operations.
Basic color management With the exposition of the layer stack we needed to introduce the notion of color management in our API. A new colormanagement module has been added to create, tweak colors and choose the color space of bitmaps. (This part of the API isn't complete yet and will be expanded in future versions.)
Query export preset information Export presets are now exposed in our API, allowing to query the list of presets (both predefined and custom). Their content can also be retrieved in a similar format to our existing export textures API.
New possibilities ahead! This new part of the API allows to do a lot of new things, like saving and restoring a selection of layers or changing the random seed of all the resources in a project for example:
For more information on the API, see the documentation included with the application (via Help > Scripting documentation > Python API ) which includes many code snippets to easily get started.
Examples of layer stack plugins can also be found in our online documentation .
Improved normal map painting
In this release we reworked the normal map painting workflow. We notably changed the way we accumulate and blend normal brush stamps. This changes were made to address issues related to painting flow maps.
Fixed accumulation issue Painting over and over an area in the normal channel will no longer saturate or clamp and create holes or artifacts. Switching the normal channel to RGB32F is also no longer needed.
Fixed undo breaking painted strokes Undoing a brush stroke no longer breaks other already painted strokes.
Transparency on zero alpha Brush stamps made with a texture with an alpha at zero will now draw as transparent. The example below shows a brush stamp (left) versus a planar projection (right).
For more information on painting flow map, see the documentation page .
Improved transform manipulators
Several improvements have been made to enhance the usage of the transform manipulators.
Precision mode with CTRL Pressing control while dragging on a manipulator will now enter into a new precision mode which allows more meticulous operations. This change applies to the translate, rotate and scale manipulators. Here is an example before and after pressing CTRL while dragging:
New scale behavior The scale intensity is now based on the current scale value itself and not on the scene size anymore. This makes relative changes easier to do, especially at small values. Combined with the precise mode it makes scaling a lot more pleasant. Another change is scaling down until 0 will no longer go into negative values. This avoid the issue of wanting to scale down a projection and flipping it by accident.
Improved surface manipulator rotation The surface decal manipulator is now a lot more stable when dragging around a surface. It doesn't increase its rotation when just doing back and forth translations. Here is the old behavior compared to the new one:
Camera aligned projection on drag and drop Drag and dropping a resource into the viewport allows to create a warp projection directly on the surface of the mesh. This projection was previously incorrectly rotated, it is now aligned to the camera.
A few other improvements have been added, notably:
Updated Tile Generator The Tile Generator blending mode parameter can now be changed and will modify the result as expected. The resource has also been updated to the latest version available in Substance 3D Designer .
Fixed banding/quality issues on some filters Several filters were stuck on 8 bit precision instead of 16 bit, leading to banding/artifacts when using them (like the histogram scan or directional blur). This is now fixed.
Color space in SBSAR output When the Legacy or OCIO color management workflow is enabled, the SBSAR export will now reference the color space names used in the project on the respective outputs.
Faster resource discovery With the introduction of the Text resource we added a new cache to make crawling of resources on the disk faster on next startup. This is quite notable when resources are installed on a HDD or when a library has gigabytes of resources. This new cache can be disabled with a command line, see the dedicated documentation page for more information.
Many thanks to the website is this arabic ? which was of great help during the development of this version.
Reference to artworks used in the medias above:
- Man wearing black shirt by Lucas Gouvêa
- Pink and green by Pawel Czerwinski
- unDraw illsutrations
- Claude Monet
Release notes
Release date: 2024/05/16 Summary: Major release, edition of the layer stack with Python API, read native Illustrator files, integration of 3D Assets and new text resource
- [Illustrator] Use Illustrator files with art boards in Painter
- [Illustrator][SVG] Add previews in scope selection
- [Substance 3D Assets] Browse, select and download 3D Assets directly in Painter
- [Substance 3D Assets][UI] New panel
- [Substance 3D Assets] Support environment maps and materials
- [Substance 3D Assets] Allow to reload and navigate and open location folder in new Substance 3D Assets panel
- [Substance 3D Assets] Addition of a download manager
- [Text Resource] Allow to use embeddable fonts
- [Text Resource] Allow to render a font/text on a mesh
- [Text Resource] Display fonts from user and other shared paths in Assets panel with a new category
- [Text Resource][Properties] Add support for advanced font properties
- [Text Resource] Allow to search/view fonts in mini-shelves
- [Text Resource] Add error message/dialog when importing an incompatible font
- Miscellaneous
- [Fill projection] Improve Scale manipulator behavior when using small values
- [Manipulators] Add new precise mode when pressing CTRL shortcut
- [Manipulators] Improve surface manipulator stability when translating
- [Export] Add colorspace name in SBSAR outputs
- [Performance] Improve library discovery time of assets on disk
- [Substance] Update to Substance engine version 9.1.2
- [Drag and Drop] Align decal rotation to camera when dropping in viewport
- [Python] Edition of the layer stack
- [Python] Allow to select layer, effect, mask, geo mask in UI
- [Python] Allow to get/set layer blending modes
- [Python] Allow to get/set fill layer projection settings
- [Python] Allow to query Substance material color from a fill layer
- [Python] Allow to query and set uniform colors and resources in layers and effects
- [Python] Allow to create and edit text resources in layer stack
- [Python] Allow to edit active channels on layers and effects
- [Python] Allow to batch actions to have a single undo/redo
- [Python] Allow to load/edit vectorial source parameters
- [Python] Allow to edit layer and effects color properties with color management
- [Python] Allow to query and create instanced layers
- [Python] Allow to add color selection effect
- [Python] Allow to control bitmap image color management
- [Python] Allow to pause/unpause engine
- [Python] Allow to navigate to siblings and parent nodes
- [Python] Allow to create filter/generator effect
- [Python] Allow to add level effect
- [Python] Allow to add smart mask on a layer
- [Python] Allow to create/edit anchor points
- [Python] Allow to get/Set mask on layers
- [Python] Allow to create compare mask effect
- [Python] Allow to query and use presets from Substance resources
- [Python] Allow to list presets and their values via internal_properties function for Substance resources
- [Python] Allow to list predefined export presets
- [Python] Allow to list export presets available in the library
- [Python] Allow to retrieve the content of export presets
- [Crash] Undoing "Remove shader instance" with Ctrl-Z
- [Crash] Create a layer on empty stack if last selection was an effect
- [SVG] Issue with custom cropped area value
- [Auto-Unwrap] Recomputing only the packing without any change to UV orientation results in crash
- [Drag and drop] Lag due to external resources are preloaded multiple times
- [UI] Drag and drop resource thumbnail can hide warning message in layer stack
- [Performance] Masked UV tiles are still computed
- [USD] Wrong highlight for scope selection
- [Resource] Bitmap image gets corrupted after painting in normal channel and saving project
- [USD] Support left-handed vertex mesh ordering
- [Substance] Reset to default always go back to zero for angle widget
- [Engine] Painting with an SVG in a stencil doesn't work
- [Engine] Normal map brush strokes break after an undo
- [Content] Graphic to Material filter has incorrect alpha blending and color space
- [Content] Blending modes on Tile Generator are not working
- [Content] Histogram scan filter produces banding in some cases
- [Content] Baked lighting stylized does not take painted height into account
- [Python] Unexpected error when retrieving instanced layer information after shader change
Known Issues :
- [Color Management] HDR color space conversions with ACE on Linux produce clamped colors
- [Crash][Linux][AMD] Dragging and dropping resources in layer stack on Wayland OS
- [Regression][UI] Right Click Menu is too small on HD screens
- [Crash][Python] USD export triggered by TextureStateEvent
- [Save] Spp project file is lost when "save as" fails
- [MacOS Intel] Crash when importing some presets
- [Illustrator] Cannot import Ai files after server crash without restarting Painter
- [Import] Assets with same name but different extensions are overridden
Get help faster and easier
Quick links
Legal Notices | Online Privacy Policy
Share this page
Language Navigation
- Skip to content
- Skip to search
- Skip to footer
Support & Downloads
- Worldwide - English
- Arabic - عربي
- Brazil - Português
- Canada - Français
- China - 简体中文
- China - 繁體中文 (臺灣)
- Germany - Deutsch
- Italy - Italiano
- Japan - 日本語
- Korea - 한국어
- Latin America - Español
- Netherlands - Nederlands">Netherlands - Nederlands
- Helpful Links
- Licensing Support
- Technology Support
- Support for Cisco Acquisitions
- Support Tools
- Cisco Community

To open or view a case, you need a service contract
Get instant updates on your TAC Case and more

Contact TAC by Phone
800-553-2447 US/Canada
866-606-1866 US/Canada
- Returns Portal
Products by Category
- Unified Communications
- Networking Software (IOS & NX-OS)
- Collaboration Endpoints and Phones
Status Tools
The Cisco Security portal provides actionable intelligence for security threats and vulnerabilities in Cisco products and services and third-party products.
Get to know any significant issues, other than security vulnerability-related issues, that directly involve Cisco products and typically require an upgrade, workaround, or other customer action.
Check the current status of services and components for Cisco's cloud-based Webex, Security and IoT offerings.
The Cisco Support Assistant (formerly TAC Connect Bot) provides a self-service experience for common case inquiries and basic transactions without waiting in a queue.
Suite of tools to assist you in the day to day operations of your Collaboration infrastructure.
The Cisco CLI Analyzer (formerly ASA CLI Analyzer) is a smart SSH client with internal TAC tools and knowledge integrated. It is designed to help troubleshoot and check the overall health of your Cisco supported software.
My Notifications allows an user to subscribe and receive notifications for Cisco Security Advisories, End of Life Announcements, Field Notices, and Software & Bug updates for specific Cisco products and technologies.
More Support
- Partner Support
- Small Business Product Support
- Business Critical Services
- Customer Experience
- DevNet Developer Support
- Cisco Trust Portal
Cisco Communities
Generate and manage PAK-based and other device licenses, including demo licenses.
Track and manage Smart Software Licenses.
Generate and manage licenses from Enterprise Agreements.
Solve common licensing issues on your own.
Software and Downloads
Find software bugs based on product, release and keyword.
View Cisco suggestions for supported products.
Use the Cisco Software Checker to search for Cisco Security Advisories that apply to specific Cisco IOS, IOS XE, NX-OS and NX-OS in ACI Mode software releases.
Get the latest updates, patches and releases of Cisco Software.
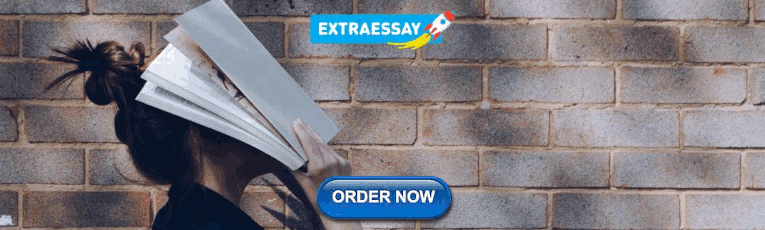
IMAGES
VIDEO
COMMENTS
These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges. All exercises are tested on Python 3. Each exercise has 10-20 Questions. The solution is provided for every question. These Python programming exercises are suitable for all Python developers.
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
Throughout these courses, you'll be given questions and assignments to test your skills. Additionally, some of these courses contain a guided project that allows you to apply everything you've learned. ... Practice with Online Python Tutorials. If online practice exercises, courses, and projects don't appeal to you, here are a few blog-style ...
Return the Sum of Two Numbers. Create a function that takes two numbers as arguments and returns their sum. Examples addition (3, 2) 5 addition (-3, -6) -9 addition (7, 3) 10 Notes Don't forget to return the result. If you get stuck on a challenge, find help in the Resources tab.
Online Compiler, Visual Debugger, and AI Tutor for Python, Java, C, C++, and JavaScript. Python Tutor helps you do programming homework assignments in Python, Java, C, C++, and JavaScript. It contains a unique step-by-step visual debugger and AI tutor to help you understand and debug code.
Beginner Python exercises. Home; Why Practice Python? Why Chilis? Resources for learners; All Exercises. 1: Character Input 2: Odd Or Even 3: List Less Than Ten 4: Divisors 5: List Overlap 6: String Lists 7: List Comprehensions 8: Rock Paper Scissors 9: Guessing Game One 10: List Overlap Comprehensions 11: Check Primality Functions 12: List Ends 13: Fibonacci 14: List Remove Duplicates
Python Exercises. Learn by doing. Exercises will help you to understand the topic deeply. Exercise for each tutorial topic so you can practice and improve your Python skills. Exercises cover Python basics to data structures and other advanced topics. Each Exercise contains ten questions to solve. Practice each Exercise using Code Editor.
Coursera: Python for Everybody Specialization; edX: Python for Everybody; FreeCodeCamp; Free certificates for University of Michigan students and staff; If you log in to this site you have joined a free, global open and online course. You have a grade book, autograded assignments, discussion forums, and can earn badges for your efforts.
There are 7 modules in this course. This course aims to teach everyone the basics of programming computers using Python. We cover the basics of how one constructs a program from a series of simple instructions in Python. The course has no pre-requisites and avoids all but the simplest mathematics. Anyone with moderate computer experience should ...
The tasks are meant to be challenging for beginners. If you find them too difficult, try completing our lessons for beginners first. All challenges have hints and curated example solutions. They also work on your phone, so you can practice Python on the go. Click a challenge to start. Practice your Python skills with online programming challenges.
The best way to learn is by practising it more and more. The best thing about this Python practice exercise is that it helps you learn Python using sets of detailed programming questions from basic to advanced. It covers questions on core Python concepts as well as applications of Python in various domains.
Python Practice Problem 1: Average Expenses for Each Semester. John has a list of his monthly expenses from last year: He wants to know his average expenses for each semester. Using a for loop, calculate John's average expenses for the first semester (January to June) and the second semester (July to December).
Also, try to solve Python list Exercise. Exercise 7: Return the count of a given substring from a string. Write a program to find how many times substring "Emma" appears in the given string. Given: str_x = "Emma is good developer. Emma is a writer" Code language: Python (python) Expected Output: Emma appeared 2 times
An introduction to programming using a language called Python. Learn how to read and write code as well as how to test and "debug" it. Designed for students with or without prior programming experience who'd like to learn Python specifically. Learn about functions, arguments, and return values (oh my!); variables and types; conditionals ...
Exercise 1: print () function | (3) : Get your feet wet and have some fun with the universal first step of programming. Exercise 2: Variables | (2) : Practice assigning data to variables with these Python exercises. Exercise 3: Data Types | (4) : Integer (int), string (str) and float are the most basic and fundamental building blocks of data in ...
Specialization - 5 course series. This Specialization builds on the success of the Python for Everybody course and will introduce fundamental programming concepts including data structures, networked application program interfaces, and databases, using the Python programming language. In the Capstone Project, you'll use the technologies ...
Join over 23 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews.
All Examples. Files. Python Program to Print Hello world! Python Program to Add Two Numbers. Python Program to Find the Square Root. Python Program to Calculate the Area of a Triangle. Python Program to Solve Quadratic Equation. Python Program to Swap Two Variables. Python Program to Generate a Random Number.
One of the greatest places to go for timely, high-quality professional programming assignment assistance is the DoMyCoding website. This service has specialists in 5 key programming fields who can tackle and solve any degree of programming issues. ... which is an option of buying services of a personal Online Python Tutor. 9. HOMEWORK HELP ...
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
Opt out personal data. Agree and proceed. {} Write and run Python code using our online compiler (interpreter). You can use Python Shell like IDLE, and take inputs from the user in our Python compiler.
To fulfill our tutoring mission of online education, our college homework help and online tutoring centers are standing by 24/7, ready to assist college students who need homework help with all aspects of Python Programming. Get Help Now. 24houranswers.com Parker Paradigms, Inc Nashville, TN Ph: (845) 429-5025.
Python Lab Assignments. You can not learn a programming language by only reading the language construct. It also requires programming - writing your own code and studying those of others. Solve these assignments, then study the solutions presented here. python programs with output for class 12 and 11 students.
CS50's Introduction to Artificial Intelligence with Python explores the concepts and algorithms at the foundation of modern artificial intelligence, diving into the ideas that give rise to technologies like game-playing engines, handwriting recognition, and machine translation. Through hands-on projects, students gain exposure to the theory ...
Assignment Operators in Python. Let's see an example of Assignment Operators in Python. Example: The code starts with 'a' and 'b' both having the value 10. It then performs a series of operations: addition, subtraction, multiplication, and a left shift operation on 'b'.
In Python-dedicated online forums, social media groups and chat rooms, beginners can ask questions and learn from more experienced developers. 10 free online Python courses
Learn the fundamentals of computer programming with the popular language Python. This class will cover concepts fundamental to all programming languages and provide an introduction to coding more generally.
Is stackless python the thing thats preventing Eve online from being a real time massive MMO ? Rant or at least the top item in a list of things. Share Add a Comment. Sort by: Best. Open comment sort options ... Also the 1s tick is what allows so many different countries to play eve online all over the world any ping upto 1000ms just doesn't ...
Substance 3D Painter 10.0 brings support of Fonts via Text resources, layer stack functionalities in the Python API, support of Illustrator (.ai) files and several quality of life improvements.
Access Cisco technical support to find all Cisco product documentation, software downloads, case help, tools, resources, and more