- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
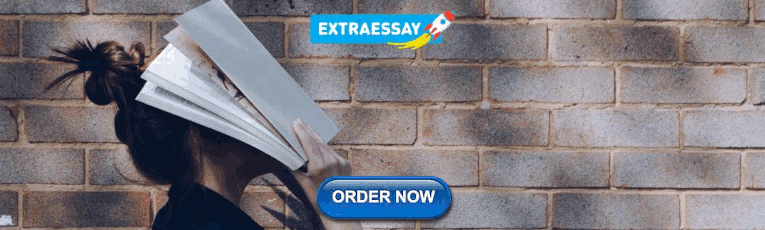
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Staging Ground badges
Earn badges by improving or asking questions in Staging Ground.
Idiomatic way of assigning a value from an if else condition in Rust
What is the preferred way of creating or assigning one of several objects to variables inside an "if - else" construct in Rust? Because of the scoping, it seems the variable must be created outside the if - else. Neither of the ways I've thought of seem very nice. Using strings as an example, here's one way, but it generates a warning about an unused assignment:
Another alternative is this:
It's shorter and doesn't generate a warning, but means s is being assigned twice, and means that "second".to_string() runs but is wasted if condition is true. If instead of simple string creation these were expensive operations (perhaps with side effects) this method wouldn't be suitable.
Is there a better alternative?

2 Answers 2
In Rust, an if/else block is an expression. That is to say, the block itself has a value, equivalent to the last expression in whatever section was executed. With that in mind, I would structure your code like this:
- 1 Thanks - I think I had seen that mentioned before but had not caught on to the significance of it. – Bob Commented Dec 30, 2018 at 23:19
- 1 @Bob: The fact that if and match are expression really trims down the number of cases in which mutable variables are necessary, embrace it wholeheartedly :) – Matthieu M. Commented Dec 31, 2018 at 17:44
- I have seen the conditions like if let p = fun() { } else {} What is the purpose of making an assignment inside the if condition? – rbansal Commented Mar 23, 2021 at 17:50
- How would this generalize to assigning two or more variables based on the same conditions? Repeating the if block for each assignment sure can't be the answer. – Christoph90 Commented Apr 25, 2021 at 5:46
- 4 @Christoph90: Use a tuple: let (a, b) = if condition { ("first", 1) } else { ("second", 2) }; – Benjamin Lindley Commented Apr 25, 2021 at 5:52
Giving another perspective of Benjanmin's answer might help to deepen someone's understanding of what is happening.
Same thing done by the compiler just written in another way:

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged rust or ask your own question .
- The Overflow Blog
- Brain Drain: David vs Goliath
- How API security is evolving for the GenAI era
- Featured on Meta
- Preventing unauthorized automated access to the network
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
- Feedback Requested: How do you use the tagged questions page?
- Proposed designs to update the homepage for logged-in users
Hot Network Questions
- Are apples 25% air?
- Could compressed air be used in a 'whipper'
- \biguplus downward facing version
- How does speed telemetry displayed in SpaceX live videos work?
- Why can't I align an image by the top border of tabular?
- The shortcut hint
- Science fiction novel where technology is strictly controlled (by the church?) and the female protagonist is seeking a device that is a powerful laser
- prefer (don't force) https but allow http on Linux (html, or wordpress)
- What's the Name of this Debating/Rhetorical Strategy?
- How might a creature be so adapted to the temperature of its home planet that it can't survive on any other without protection?
- Who was revolutionary?
- Learning music theory on guitar and piano at the same time
- Why do some of the Galilean moons receive so much less radiation than others?
- Does GNU Tar have a single option to get absolutely everything about a tree
- Card design with long and short text options
- Whatever decision he made, I would support it
- Can I recharge Airpods Pro 1 with Airpods Pro 2 Case?
- Need to replace special character "/" with another string
- Can I become a software programmer with an Information Sciences degree?
- How to translate interjections/sounds like "whoa" and "gulp" into German?
- Using MIT Python PyPI package with GPLv2-or-later Python package dependency in non-GPLv2-or-later-compliant project
- Can you (and has anyone) answer correctly and still get 100 points?
- How did Johannes Kepler figure out the radius of each planet’s orbit?
- What does Rich mean?
- Course List
- Introduction to Rust
- Environment Setup
- First Rust Application
- Variables & Constants
- Control Flow
- if, else if, else, match
- while, for loops
- break & continue
- Intermediate
- Borrowing & Referencing
- Structs (Structures)
- Enums (Enumerations)
- Collections
- Vector Collection
- HashMap Collection
- Advanced Types
- Memory Management
- Smart Pointers
- Multi-Threading
- Concurrency
Rust Conditional Control (if, else if, else, match) Tutorial
In this Rust tutorial we learn how to control the flow of our Rust application with if, else if, else, and match conditional statements.
We also cover how to nest conditional statements inside other conditional statements as well as how to conditionally assign a value to a variable if the if let expression.
- What is conditional control flow
- The if conditional statement
- How to evaluate multiple if conditions
- The conditional else if ladder
- The conditional fallback else statement
- How to nest if, else if and else statements
- The conditional match statement
- Conditional variable assignment (if let)
- The Rust ternary operator
- Summary: Points to remember
Rust allows us to control the flow of our program through specific expressions that evaluate conditions. Based on the result of these conditions, Rust will execute sections of specified code.
As an example, let’s consider a application with a dedicated area for members. Before a user can gain access to the member area, they need to provide the correct login credentials.
If they provide the correct credentials, they are allowed into the exclusive member area. Otherwise, they will be given the option to try again or reset their password if they have forgotten it.
Based on this logic, we can imagine that our code would look something like this.
Our actual code will not look exactly like the example above, but it serves to give you an example of what conditional control is.
We control the flow of our program with the following statements, in combination with relational comparison and logical operators.
We give an if statement a condition to evaluate. If the condition proves true, the compiler will execute the if statement’s code block.
If the condition proves false, the compiler will move on to code below the if statement’s code block.
An if statement is written with the keyword if , followed by the condition(s) we want it to evaluate.
Then, we specify a code block with open and close curly braces. Inside the code block we write one or more expressions to be executed if the evaluation proves true.
Note that an if statement is not terminated with a semicolon. The closing curly brace will tell the compiler where the if statement ends.
In the example above, our variable a has a value less than 10, so the condition proves true. The compiler then prints the message inside the if statement’s code block.
When the compiler has finished executing the if statement, it will move on to any code after it. The same is true when our if statement proves false, the compiler will move on to the next piece of code outside and below the if statement.
In the example above, our if condition proves false. The compiler then moves on to the next section of code after the if statement.
We can evaluate more than one condition in an if statement with the help of the two logical operators && and ||.
Conditional AND is used to test if one condition and another proves true. Both conditions must prove true before the code will execute.
To evaluate multiple conditions with AND, we separate them with the && operator.
In the example above, both of our conditions prove true so the code inside the if code block executes.
The conditional OR is used to test if one condition or another proves true. Only one of the conditions must prove true before the code will execute.
To evaluate multiple conditions with OR, we separate them with the || operator.
In the example above, the first condition proves false, but the second condition proves true so the compiler is allowed to execute the code in the code block.
When we have multiple types of conditions that do not fit within a single if condition, we can write more if statements and combine them into an else if ladder.
The compiler will move to evaluate each if statement in the ladder when it’s done with previous one.
To write a conditional else if statement, we add another if statement below our first, separated by the else keyword.
In the example above, we add a secondary if statement at the end of the first, separated by the else keyword.
Note that an else if statement can only follow an if statement, it cannot stand on its own. The first conditional must always be an if.
We can have as many else if statements in a ladder as we need.
The else statement works as a catch-all fallback for anything that isn’t covered by an if statement or else if ladder.
Because it acts as a catch-all, the else statement doesn’t have a conditional block, only an execution code block.
In the example above, the else statement will execute any result other than number == 10. Because number is not 10, the compiler executes the else execution code block.
We can place if statements inside other if statements, a technique known as nesting. The compiler will perform the evaluations hierarchically, that’s to say, it will start out the outer if statement and move inwards.
To nest an if statement, we simply write it inside the execution code block of another if statement.
In the example above, our outer if statement condition proves true. The compiler then evaluates the inner if statement and executes its code block if its condition proves true.
Nested if statements are used when we want to evaluate conditions only if other conditions are true.
Note that if you find yourself nesting more than 3 levels deep, you should consider finding a different method. Perhaps a loop.
The match statement will check a single value against a list of values.
If you’re familiar with a languages in the C family, it would be similar to a switch statement, although the syntax is different.
Let’s take a look at the syntax before we explain.
There’s a lot going on here and it may seem confusing at first, so let’s take it step by step.
1. The match statement returns a value, so we can assign the whole statement to a variable to get the result.
2. Now we write the match statement itself. We use the keyword match, followed by the main value we are going to compare any other values to. Finally, we define a code block.
Note that the code block is terminated with a semicolon after the closing curly brace.
3. Inside the code block we will write the values that we want to try and match with the main value.
First, we specify the value we want to match, followed by a => operator. Then, inside another code block, we specify the execution statements if that value matches with the main value.
Each of these values are separated with a comma, and we can have as many as we need.
The final value to match is simply an underscore. The single, standalone, underscore in a match statement represents a catch-all situation if none of the values matched to the main value. Think of it as an else statement.
Now, let’s see a practical example of the match statement.
In the example above, we give a student a grade and store it in a variable. Then we check to see which grade score in the match statement the student’s score matches.
If the compiler finds a match on the left of the => operator, it will execute whatever statement is on the right of the => operator.
In this case, it matched to “B” on the left and so it printed the string “Great job, you got a B!”.
Note that we don’t use the result variable in the example above. This is simply because we don’t need to, the execution statement of the match already prints a message.
If we want to return an actual value, we specify the value instead of an action like println.
In the example above, we replaced the println!() statements with simple string values.
When the compiler finds a match on the left of the => operator, it will return the value on the right of the => into the result variable.
This time, the compiler matched to “A” on the left, so it stored the string “Excellent!” into the result variable.
We then used it in the println!() below the match statement.
We can conditionally assign a value to a variable with an if let expression. To do this, we simply write the if statement where the value should be in the variable initialization.
It’s important to note a few differences here to a normal if statement.
- We must have both an if and else statement.
- The execution expressions must provide a value to be assigned to the variable.
- The individual statements in the code blocks are not terminated with a semicolon.
- The if statement itself is terminated with a semicolon after the else closing curly brace.
In the example above, the if statement will prove true so the value assigned to the number variable is 1.
Let’s see another example.
As we know, a variable can only hold one type of value. If we try to assign different types of values to it through the if statement, the compiler will raise an error.
In the example above, the values we want to assign is of different types.
Eclipse will highlight the potential error for us before we compile. If we go ahead and compile anyway, the following error is raised.
Rust does not have a ternary operator anymore.
In many languages, such as C or Python we can write a shortened version of a simple if/else statement, known as the ternary operator.
Rust, removed the ternary operator back in 2012 . The language doesn’t support a ternary operator anymore.
- We can control the flow of our application with conditional logic through if , else if , else and match statements.
- The if and else if conditional statements must include a condition to evaluate.
- The else statement is a catch-all fallback to if and else if and as such cannot have a condition.
- We can nest if statements inside other if statements. The compiler will evaluate them hierarchically.
- The match statement is similar to a switch statement in many other languages such as C,C++.
- An if let expression places the if statement as the value of a variable declaration.
- An if let expression is terminated with a semicolon after the code block closing brace.
- Values inside the code blocks of an if let expression are not terminated with a semicolon.
Conditionally Assigning Variables in Rust: A Deep Dive into the 'if let' Statement
Abstract: In this article, we'll explore how to conditionally assign variables in Rust using the 'if let' statement. We'll cover the basics of pattern matching, destructuring, and the 'if let' statement, and provide examples to help clarify the concepts.
Conditionally Assigning Variables in Rust: A Deep Dive into the let Statement
In Rust, the let statement is used to declare and initialize variables. However, the let statement has more functionality than just declaring variables. It can also be used to conditionally assign variables based on certain conditions. In this article, we will take a deep dive into the let statement in Rust and explore how to conditionally assign variables.
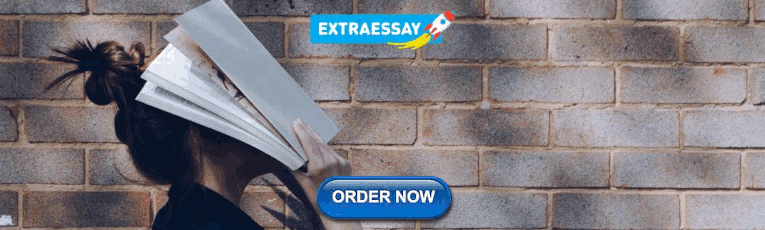
Introduction to the let statement
Conditionally assigning variables with let, using the if let statement, using the match statement, best practices for conditionally assigning variables.
In Rust, the let statement is used to declare and initialize variables. The syntax for the let statement is as follows:
The variable_name is the name of the variable, and value is the value that the variable is initialized with. The let statement is used to declare a single variable, but it can be used in a block to declare multiple variables.
The let statement can be used to conditionally assign variables based on certain conditions. This is done by wrapping the let statement in an if expression. The syntax for conditionally assigning variables with let is as follows:
In this example, the variable_name is conditionally assigned based on the value of the condition . If the condition is true , then variable_name is assigned the value of value . If the condition is false , then variable_name is assigned the value of other_value .
The if let statement is a shorthand way of writing an if expression that only checks for a single pattern. The syntax for the if let statement is as follows:
In this example, the if let statement checks if optional_value contains a value. If it does, then the value is assigned to the value variable, and the code inside the block is executed.
The match statement is a powerful pattern matching tool in Rust. It can be used to conditionally assign variables based on multiple patterns. The syntax for the match statement is as follows:
In this example, the match statement checks the value of value against multiple patterns. If the value matches pattern1 , then the code inside the block is executed. If the value matches pattern2 , then the code inside the block is executed. If the value does not match any of the patterns, then the code inside the _ block is executed.
When conditionally assigning variables in Rust, there are a few best practices to keep in mind:
- Use the if let statement for single pattern matching
- Use the match statement for multiple pattern matching
- Avoid using the let statement inside an if expression, as it can lead to code that is difficult to read and maintain
- Use clear and concise variable names to make the code more readable
Example: Getting data from a URL
Let's look at an example of conditionally assigning variables in Rust. Suppose we want to get data from a URL using the reqwest crate. We want to handle two different sources of data: a JSON blob or an XML file. We can use the let statement to conditionally assign variables based on the type of data we receive.
Here's the code:
In this example, we use the reqwest crate to get the data from the URL. We check if the data is in JSON format by checking if it starts with { . If it does, then we deserialize the data using serde_json . If the data is not in JSON format, then we assume it is in XML format and deserialize it using serde_json .
In Rust, the let statement can be used to conditionally assign variables based on certain conditions. This can be done using an if expression, the if let statement, or the match statement. By following best practices, we can write clean and concise code that is easy to read and maintain.
- Rust Programming Language: Variables and Mutability
- Rust Programming Language: if let
- Rust Programming Language: Match
- reqwest crate documentation
- serde_json crate documentation
Tags: : Rust Programming Variables
Latest news
- Overcoming Slowdowns in WordPress REST API Calls for Your Project
- Understanding Probabilities and Elements with Tosses in Numpy's Binomial Distribution using Python
- Reading Aligned CSV Files with Rainbow CSV Extension
- Setting up gTest project with Conan 2.x
- TypeError: Parameter 'favorite' implicitly has an 'any' type. How to Fix When Uploading to Vercel
- Touch Screen Zooming in WPF OxyPlot.SkiaSharp.Wpf Application
- Uncrustify Configuration Parameters for Adjusting Method Chaining Styling
- Customizing Xamarin Notifications with Xamarin.Firebase.Messaging
- MongoDB Realm JsCollection.watch
- Extracting Data from AWS Redshift and Inserting it into an AS400 DB using Spring Boot Microservice
- Identifying Possible Causes of Flaky PostGIS Errors in PostgreSQL
- Unable to Take Screenshots of Simple Webpages with Selenium Python: A Solution
- Connect Windows Server from Node.js/Express.js Backend to Read Files
- Building Expression ADF using Parameterized Variables: A Step-by-Step Guide
- Rendering 3-4 Diagrams on a React Next.js Page: Diagrams Intermittently Getting Mixed
- Connecting Azure SQL Managed Identity to Function App Service Plan: A Step-by-Step Guide
- Visualizing ELB Logs in S3 with Grafana
- Effortlessly Change Multiple File Names Based on Spreadsheet Data
- Using jQuery toggle() method inside JSX in a React Project
- Troubleshooting FreeRadius 3.0.27: Global Configuration Requiring 'require_message_authenticator' Not Behaving
- Reset Button Not Clearing Date Selection in Angular Material Date Range Picker
- Troubleshooting Node.js: Missing RabbitMQ Message Not Success Log
- Solving Character Controller Stick to Ground Issues with Raycast and Vertical Velocity
- Limitation in Updating Promocode Attributes: Unable to Delete or Update
- Add Mockito Dependency and Write Test Cases for Android Libraries
- Exploring GnuCOBOL C Interop: Passing Long Values
- Converting Incorrect JSON Format to Correct Format
- Making a Simple Ruler in Leaflet: No Plugin Needed
- Unable to Add Items to Cart or Update Counts on Mango Webpage
- Utilizing Send Message Trigger in Software Development
- Integrating Google API into a Maven Web Scraping Project: A Beginner's Guide
- Understanding Shell Titlebar Click Events in SWT
- Resolving 'Could not find artifact' error in Java Maven project: Dependency path not found
- Error Handling Packages: Separate Longer Delimiters in R
- Blocking User Access: Handling Logins Outside Organization Networks
- Twitter / X
- to navigate
If Expression
If expression link.
There can be multiple conditional constructs using an if statement.
If expression
If…else expression
If…else if…else expression
Nested if expression
Shorthand if expression
Let’s discuss each one of them in detail:-
If expression takes a condition. If the condition within the if expression evaluates to be true, then the block of code is executed.
Syntax The general syntax is:
Illustration link
If…else expression link.
In an if..else construct, if the condition within the if expression evaluates to be false, then the statement within the else block is executed.
The general syntax is:
if…else if…else Expression link
If there are multiple conditions to be checked, then if..else if..else construct is used.
llustration link
The following flow chart explains the concept of an if..else if..else expression:
Nested if Expression link
An if expression inside the body of another if expression is referred to as a nested if expression.
Note: The nested if expression can also be written with a AND expression in an if.
This is true only if the second if statement is the only thing inside the first if.
The following flow chart explains the concept of a nested if statement .
Note: There can be as many levels of nesting as you want.
Shorthand if link
Instead of writing a lengthy if-else construct, we can use a shorthand if.
Note: This is similar to a ternary operator in languages like C and C++.
Note: Expressions can return a value, unlike statements. Recall that the semicolon turns any expression into a statement. It throws away its value and returns a unit () instead.
Note: Uncommenting line 6 in the above code gives an error ❌ since we are trying to convert an expression to a statement and hence not returning a value.
Last updated 25 Jan 2024, 05:11 +0530 . history
navigate_before Precedence and Associativity
If let Expression navigate_next
Your daily dose of the Rust programming Language.
Conditional Assignment
- if let is a shorthand syntax for matching and binding on a single pattern.
- It can be used to conditionally assign a value to a variable.
- It is useful when you only care about one pattern and want to avoid writing a full match expression.
Matching Multiple Conditions
- if let can also be used in combination with else if statements to match multiple conditions.
- This can help to simplify code and avoid nested match expressions.
Struct Matching
- if let can also be used to match on structs and extract their fields.
- This can be useful when you only care about one or two fields in a struct
Enums Matching
- if let can also be used to match on enums and extract their variants.
- This can be useful when you only care about one or two variants of an enum.
Using if let with Result
- The Result type in Rust can be either an Ok variant or an Err variant.
- We can use the if let expression to match the Ok variant and extract the value from it.
- The if let expression can also handle the Err variant with an else block.
if let with while let
- if let can be used in combination with while let to handle pattern matching in a loop.
- This is useful when you want to keep looping while a certain pattern matches.
if let with a wildcard
- If let can also be used with a wildcard (_) to match any value in a particular variable.
- This is particularly useful when we are only interested in knowing if the pattern matches or not.
- Using a wildcard (_) is the same as using a variable without assigning it to a value.
- The underscore (_) is used to discard values and it is a special variable in Rust.
if let with closures
- You can use if let statements to simplify closures by removing unnecessary match statements.
- If a closure only has a single pattern match, you can use if let to simplify the code and make it more readable.
- The closure will only be executed if the pattern match is successful.
if let with early return
- You can use if let with an early return statement to simplify code and reduce nesting.
- If the pattern match is successful, the return statement will be executed immediately, without any additional code in the block being executed.
- This can be useful for cases where you want to exit a function early if a certain condition is met.
if let with find
- Rust’s standard library provides a find method for searching an iterator for an element that satisfies a predicate.
- We can use if let with find to extract the found value if it exists.
if let with options
- Rust’s Option type is used to represent the presence or absence of a value.
- We can use if let to extract the value from the Option if it contains a value, otherwise do nothing.
- This can be useful when we only care about the case where the Option has a value and want to handle it specially.
You can refer to this Twitter thread for reference
Rust by Example: If/Else
The statement assesses a conditional expression, determining the control flow based on whether the expression evaluates to true or false. | |
If/else statements are similar to other languages. | |
Rust supports six comparison operators: , , , , , . and two boolean operators: (and) and (or). | |
The statement can return a value and can be used in a statement. | |
Using expression, control is determined through pattern matching instead of a conditional expression. |
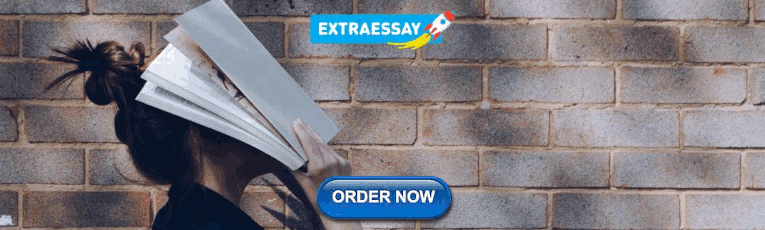
COMMENTS
What is the preferred way of creating or assigning one of several objects to variables inside an "if - else" construct in Rust? Because of the scoping, it seems the variable must be created outside the if - else.
The syntax of an if expression is a condition operand, followed by a consequent block, any number of else if conditions and blocks, and an optional trailing else block. The condition operands must have the boolean type.
Learn the if, else if, else and match statements to conditionally control the flow of our Rust application. We also cover how to nest if statements and how to conditionally assign a value to a variable with the if let expression.
if/else - Rust By Example. Branching with if - else is similar to other languages. Unlike many of them, the boolean condition doesn't need to be surrounded by parentheses, and each condition is followed by a block. if - else conditionals are expressions, and, all branches must return the same type. 1. 2. 3. 4. 5. 6. 7. 8. 9. 10. 11. 12. 13. 14. 15.
A match expression has a scrutinee expression, which is the value to compare to the patterns. The scrutinee expression and the patterns must have the same type. A match behaves differently depending on whether or not the scrutinee expression is a place expression or value expression.
In this article, we'll explore how to conditionally assign variables in Rust using the 'if let' statement. We'll cover the basics of pattern matching, destructuring, and the 'if let' statement, and provide examples to help clarify the concepts.
condition is true. Above Example, x > 5 is a boolean evolution that checks if the value of variable x is greater than 5. as like 7, the value of variable x is greater than 5, and the condition variable is assigned true. Here condition is true is seen as output. ¶ if Expression In Rust.
article. If Expression. There can be multiple conditional constructs using an if statement. If expression. If…else expression. If…else if…else expression. Nested if expression. Shorthand if expression. Let’s discuss each one of them in detail:- If Expression link. If expression takes a condition.
Conditional Assignment. if let is a shorthand syntax for matching and binding on a single pattern. It can be used to conditionally assign a value to a variable. It is useful when you only care about one pattern and want to avoid writing a full match expression.
Rust by Example: If/Else. The if statement assesses a conditional expression, determining the control flow based on whether the expression evaluates to true or false. fn main () {. If/else statements are similar to other languages. if true { println! ("true"); } else if false { println! ("false"); } else { println! ("neither"); }