- Español – América Latina
- Português – Brasil
- Tiếng Việt
- TensorFlow Core
The TensorFlow tutorials are written as Jupyter notebooks and run directly in Google Colab—a hosted notebook environment that requires no setup. At the top of each tutorial, you'll see a Run in Google Colab button. Click the button to open the notebook and run the code yourself.
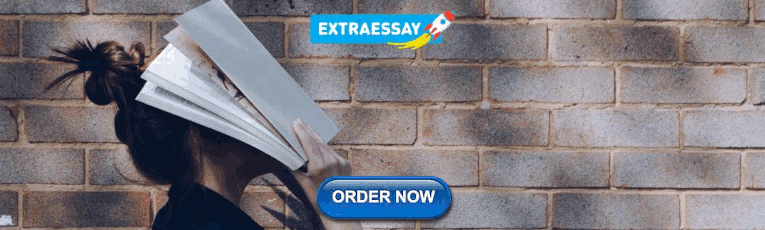
For beginners
Beginner quickstart, keras basics, for experts, advanced quickstart, customization, distributed training, video tutorials, tensorflow ml zero to hero, basic computer vision with ml, libraries and extensions, tensorboard.
- Get started with TensorBoard
- Logging training metrics in Keras
TensorFlow Hub
- Object detection
- Arbitrary style transfer
Model Optimization
- Magnitude-based weight pruning with Keras
- Post-training quantization
TensorFlow Federated
- Federated learning for image classification
- Federated learning for text generation
Neural Structured Learning
- Natural graph regularization for document classification
- Synthetic graph regularization for sentiment classification
TensorFlow Graphics
- Object pose alignment
- Mesh segmentation
- Image operations in TensorFlow Addons
- Normalization layers in TensorFlow Addons.
- TFX developer tutorial
- Serve a model with TensorFlow Serving
- Using TensorFlow Datasets
Probability
- TensorFlow distributions introduction
- Probabilistic regression
- Classifying CIFAR-10 with XLA
- Use XLA with tf.function
Decision Forests
- Train a decision forest model
- Use text and NN features with decision forests
TensorFlow Agents
- Train a deep-Q network with TF Agents
- Reinforcement learning environments
TensorFlow Ranking
- TF-Ranking Keras user guide
- TF Ranking for sparse features
- Generating Piano music with Transformer
TensorFlow updates
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License , and code samples are licensed under the Apache 2.0 License . For details, see the Google Developers Site Policies . Java is a registered trademark of Oracle and/or its affiliates.
Last updated 2023-09-19 UTC.
TensorFlow.js Livestream: Deep Learning in the browser
- Introduction to Deep Learning with TensorFlow
Welcome to part two of Deep Learning with Neural Networks and TensorFlow, and part 44 of the Machine Learning tutorial series. In this tutorial, we are going to be covering some basics on what TensorFlow is, and how to begin using it.
Libraries like TensorFlow and Theano are not simply deep learning libraries, they are libraries *for* deep learning. They are actually just number-crunching libraries, much like Numpy is. The difference is, however, a package like TensorFlow allows us to perform specific machine learning number-crunching operations like derivatives on huge matricies with large efficiency. We can also easily distribute this processing across our CPU cores, GPU cores, or even multiple devices like multiple GPUs. But that's not all! We can even distribute computations across a distributed network of computers with TensorFlow. So, while TensorFlow is mainly being used with machine learning right now, it actually stands to have uses in other fields, since really it is just a massive array manipulation library.
What is a tensor? Up to this point in the machine learning series, we've been working mainly with vectors (numpy arrays), and a tensor can be a vector. Most simply, a tensor is an array-like object, and, as you've seen, an array can hold your matrix, your vector, and really even a scalar.
At this point, we just simply need to translate our machine learning problems into functions on tensors, which is possible with just about every single ML algorithm. Consider the neural network. What does a neural network break down into?
We have data ( X ), weights ( w ), and thresholds ( t ). Are all of these tensors? X will be the dataset (an array), so that's a tensor. The weights are also an array of weight values, so they're tensors too. Thresholds? Same as weights. Thus, our neural network is indeed a function of X , w , and t , or f(Xwt) , so we are all set and can certainly use TensorFlow, but how?
TensorFlow works by first defining and describing our model in abstract, and then, when we are ready, we make it a reality in the session. The description of the model is what is known as your "Computation Graph" in TensorFlow terms. Let's play with a simple example. First, let's construct the graph:
So we have some values. Now, we can do things with those values, such as multiplication:
Notice that the output is just an abstract tensor still. No actual calculations have been run, only operations created. Each operation, or "op," in our computation graph is a "node" in the graph.
To actually see the result, we need to run the session. Generally, you build the graph first, then you "launch" the graph:
We can also assign the output from the session to a variable:
When you are finished with a session, you need to close it in order to free up the resources that were used:
After closing, you can still reference that output variable, but you cannot do something like:
...which would just return an error. Another option you have is to utilize Python's with statement:
If you are not familiar with what this does, basically, it will use the session for the block of code following the statement, and then automatically close the session when done, the same way it works if you open a file with the with statement.
You can also use TensorFlow on multiple devices, and even multiple distributed machines. An example for running some computations on a specific GPU would be something like:
Code from: TensorFlow docs . The tf.matmul is a matrix multiplication function.
The above code would run the calcuation on the 2nd system GPU. If you installed the CPU version with me, then this isn't currently an option, but you should still be aware of the possibility down the line. The GPU version of TensorFlow requires CUDA to be properly set up (along with needing a CUDA-enabled GPU). I have a few CUDA enabled GPUs, and would like to eventually cover their use as well, but that's for another day!
Now that we have the basics of TensorFlow down, I invite you down the rabbit hole of creating a Deep Neural Network in the next tutorial. If you need to install TensorFlow, the installation process is very simple if you are on Mac or Linux. On Windows, not so much. The next tutorial is optional, and it is just us installing TensorFlow on a Windows machine.
The next tutorial: Deep Learning with TensorFlow - Creating the Neural Network Model
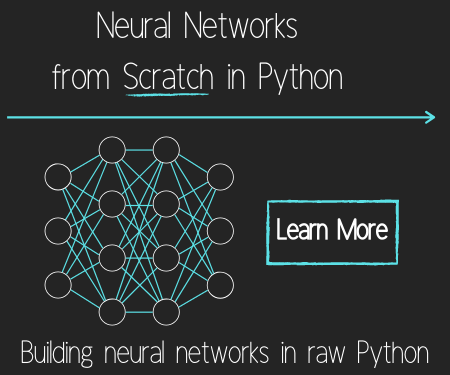
Deep-Learning-Specialization-Coursera
This repo contains the updated version of all the assignments/labs (done by me) of deep learning specialization on coursera by andrew ng. it includes building various deep learning models from scratch and implementing them for object detection, facial recognition, autonomous driving, neural machine translation, trigger word detection, etc., deep learning specialization coursera [updated version 2021].
Announcement
[!IMPORTANT] Check our latest paper (accepted in ICDAR’23) on Urdu OCR
This repo contains all of the solved assignments of Coursera’s most famous Deep Learning Specialization of 5 courses offered by deeplearning.ai
Instructor: Prof. Andrew Ng
This Specialization was updated in April 2021 to include developments in deep learning and programming frameworks. One of the most major changes was shifting from Tensorflow 1 to Tensorflow 2. Also, new materials were added. However, Most of the old online repositories still don’t have old codes. This repo contains updated versions of the assignments. Happy Learning :)
Programming Assignments
Course 1: Neural Networks and Deep Learning
- W2A1 - Logistic Regression with a Neural Network mindset
- W2A2 - Python Basics with Numpy
- W3A1 - Planar data classification with one hidden layer
- W3A1 - Building your Deep Neural Network: Step by Step¶
- W3A2 - Deep Neural Network for Image Classification: Application
Course 2: Improving Deep Neural Networks: Hyperparameter tuning, Regularization and Optimization
- W1A1 - Initialization
- W1A2 - Regularization
- W1A3 - Gradient Checking
- W2A1 - Optimization Methods
- W3A1 - Introduction to TensorFlow
Course 3: Structuring Machine Learning Projects
- There were no programming assignments in this course. It was completely thoeretical.
- Here is a link to the course
Course 4: Convolutional Neural Networks
- W1A1 - Convolutional Model: step by step
- W1A2 - Convolutional Model: application
- W2A1 - Residual Networks
- W2A2 - Transfer Learning with MobileNet
- W3A1 - Autonomous Driving - Car Detection
- W3A2 - Image Segmentation - U-net
- W4A1 - Face Recognition
- W4A2 - Neural Style transfer
Course 5: Sequence Models
- W1A1 - Building a Recurrent Neural Network - Step by Step
- W1A2 - Character level language model - Dinosaurus land
- W1A3 - Improvise A Jazz Solo with an LSTM Network
- W2A1 - Operations on word vectors
- W2A2 - Emojify
- W3A1 - Neural Machine Translation With Attention
- W3A2 - Trigger Word Detection
- W4A1 - Transformer Network
- W4A2 - Named Entity Recognition - Transformer Application
- W4A3 - Extractive Question Answering - Transformer Application
I’ve uploaded these solutions here, only for being used as a help by those who get stuck somewhere. It may help them to save some time. I strongly recommend everyone to not directly copy any part of the code (from here or anywhere else) while doing the assignments of this specialization. The assignments are fairly easy and one learns a great deal of things upon doing these. Thanks to the deeplearning.ai team for giving this treasure to us.
Connect with me
Name: Abdur Rahman
Institution: Indian Institute of Technology Delhi
Find me on:
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
My notes / works on deep learning from Coursera
y33-j3T/Coursera-Deep-Learning
Folders and files.
Name | Name | |||
---|---|---|---|---|
101 Commits | ||||
Repository files navigation
My notes / works from Coursera courses.
Introduction
Contributing.
This repository contains my solutions for labs and programming assignments on Coursera courses. Certain resources required by the codes may be lacking due to limitations on downloading course materials from Coursera and uploading them to GitHub. The lacking resources are mostly datasets, pre-trained models or certain weight matrices.
TensorFlow: Advanced Techniques (Specialization)
1. custom models, layers, and loss functions with tensorflow, week 1 - functional apis.
- Lab: Functional API Practice
- Lab: Multi-output
- Lab: Siamese network
- Programming Assignment: Multiple Output Models using Keras Functional API
Week 2 - Custom Loss Functions
- Lab: Huber Loss lab
- Lab: Huber Loss object
- Lab: Contrastive loss in the siamese network (same as week 1's siamese network)
- Programming Assignment: Creating a custom loss function
Week 3 - Custom Layers
- Lab: Lambda layer
- Lab: Custom dense layer
- Lab: Activation in a custom layer
- Programming Assignment: Implement a Quadratic Layer
Week 4 - Custom Models
- Lab: Build a basic model
- Lab: Build a ResNet model
- Programming Assignment: Create a VGG network
Week 5 - Bonus Content - Callbacks
- Lab: Built-in Callbacks
- Lab: Custom Callbacks
2. Custom and Distributed Training with Tensorflow
Week 1 - differentiation and gradients.
- Lab: Basic Tensors
- Lab: Gradient Tape Basics
- Programming Assignment: Basic Tensor Operations
Week 2 - Custom Training
- Lab: Training Basics
- Lab: Fashion MNIST using Custom Training Loop
- Programming Assignment: Breast Cancer Prediction
Week 3 - Graph Mode
- Lab: AutoGraph Basics
- Lab: AutoGraph
- Programming Assignment: Horse or Human?
Week 4 - Distributed Training
- Lab: Mirrored Strategy
- Lab: Multi GPU Mirrored Strategy
- Lab: TPU Strategy
- Lab: One Device Strategy
- Programming Assignment: Distributed Strategy
3. Advanced Computer Vision with TensorFlow
Week 1 - introduction to computer vision.
- Lab: Transfer Learning
- Lab: Transfer Learning with ResNet 50
- Lab: Image Classification and Object Localization
- Programming Assignment: Bird Boxes
Week 2 - Object Detection
- Lab: Implement Simple Object Detection
- Lab: Predicting Bounding Boxes for Object Detection
- Programming Assignment: Zombie Detector
Week 3 - Image Segmentation
- Lab: Implement a Fully Convolutional Neural Network
- Lab: Implement a UNet
- Lab: Instance Segmentation Demo
- Programming Assignment: Image Segmentation of Handwritten Digits
Week 4 - Visualization and Interpretability
- Lab: Class Activation Maps with Fashion MNIST
- Lab: Class Activation Maps "Cats vs Dogs"
- Lab: Saliency Maps
- Lab: GradCAM
- Programming Assignment: Cats vs Dogs Saliency Maps
DeepLearning.AI TensorFlow Developer (Specialization)
1. introduction to tensorflow for artificial intelligence, machine learning, and deep learning, week 1 - a new programming paradigm.
- Programming Assignment: Exercise 1 (Housing Prices)
Week 2 - Introduction to Computer Vision
- Programming Assignment: Exercise 2 (Handwriting Recognition)
Week 3 - Enchancing Vision with Convolutional Neural Networks
- Programming Assignment: Exercise 3 (Improve MNIST with convolutions)
Week 4 - Using Real-world Images
- Programming Assignment: Exercise 4 (Handling complex images)
2. Convolutional Neural Networks in TensorFlow
Week 1 - exploring a larger dataset.
Programming Assignment: Exercise 1 - Cats vs. Dogs
Week 2 - Augmentation: A Technique to Avoid Overfitting
Programming Assignment: Exercise 2 - Cats vs. Dogs using augmentation
Week 3 - Transfer Learning
Programming Assignment: Exercise 3 - Horses vs. humans using Transfer Learning
Week 4 - Multiclass Classifications
Programming Assignment: Exercise 4 - Multi-class classifier
Unable to download horse-or-human.zip
3. Natural Language Processing in TensorFlow
Week 1 - sentiment in text.
- Ungraded External Tool: Exercise 1 - Explore the BBC news archive
- Ungraded External Tool: Exercise 1 - Explore the BBC news archive (answer)
Week 2 - Word Embeddings
- Ungraded External Tool: Exercise 2 - BBC news archive
- Ungraded External Tool: Exercise 2 - BBC news archive (answer)
Week 3 - Sequence Models
- Ungraded External Tool: Exercise 3 - Exploring overfitting in NLP
- Ungraded External Tool: Exercise 3 - Exploring overfitting in NLP (answer)
Week 4 - Sequence Models and Literature
- Ungraded External Tool: Exercise 4 - Using LSTMs, see if you can write Shakespeare
- Ungraded External Tool: Exercise 4 - Using LSTMs, see if you can write Shakespeare (answer)
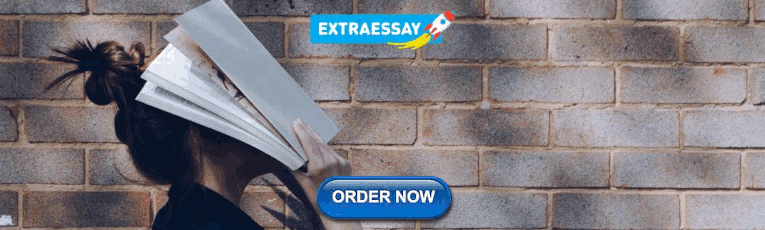
4. Sequences, Time Series and Prediction
Week 1 - sequences and prediction.
- Ungraded External Tool: Exercise 1 - Create and predict synthetic data
- Ungraded External Tool: Exercise 1 - Create and predict synthetic data (answer)
Week 2 - Deep Neural Networks for Time Series
- Ungraded External Tool: Exercise 2 - Predict with a DNN
- Ungraded External Tool: Exercise 2 - Predict with a DNN (answer)
Week 3 - Recurrent Neural Networks for Time Series
- Ungraded External Tool: Exercise 3 - Mean Absolute Error
- Ungraded External Tool: Exercise 3 - Mean Absolute Error (answer)
Week 4 - Real-world Time Series Data
- Ungraded External Tool: Exercise 4 - Sunspots
- Ungraded External Tool: Exercise 4 - Sunspots (answer)
Generative Adversarial Networks (GANs) (Specialization)
1. build basic generative adversarial networks (gans), week 1 - intro to gans.
- Lab: (Optional) Intro to PyTorch
- Programming Assignment: Your First GAN
Week 2 - Deep Convolutional GANs
- Programming Assignment: Deep Convolutional GAN (DCGAN)
Week 3 - Wasserstein GANs with Gradient Penalty
- Lab: (Optional) SN-GAN
- Programming Assignment: WGAN
Week 4 - Conditional GAN & Controllable Generation
- Lab: (Optional) InfoGAN
- Programming Assignment: Conditional GAN
- Programming Assignment: Controllable Generation
2. Build Better Generative Adversarial Networks (GANs)
Week 1 - evaluation of gans.
Unable to download inception_v3_google-1a9a5a14.pth , fid_images_tensor.npz
- Lab: (Optional) Perceptual Path Length
- Programming Assignment: Fréchet Inception Distance
Week 2 - GAN Disadvantages and Bias
- Lab: Alternatives: Variational Autoencoders (VAEs)
- Programming Assignment: Bias
Week 3 - StyleGAN and Advancements
- Lab: (Optional) Components of StyleGAN2
- Lab: (Optional) Components of BigGAN
- Programming Assignment: Components of StyleGAN
3. Apply Generative Adversarial Networks (GANs)
Week 1 - gans for data augmentation and privacy.
- Programming Assignment: Data Augmentation
Week 2 - Image-to-Image Translation with Pix2Pix
Unable to download pix2pix_15000.pth , maps
- Programming Assignment: U-Net
- Programming Assignment: Pix2Pix
Week 3 - Unpaired Translation with CycleGAN
Unable to download horse2zebra , cycleGAN_100000.pth
- Programming Assignment: CycleGAN
Natural Language Processing (Specialization)
1. natural language processing with classification and vector spaces, week 1 - sentiment analysis with logistic regression.
- Lab: Natural Language preprocessing
- Lab: Visualizing word frequencies
- Lab: Visualizing tweets and Logistic Regression models
- Programming Assignment: Assignment: Logistic Regression
Week 2 - Sentiment Analysis with Naive Bayes
- Lab: Visualizing likelihoods and confidence ellipses
- Programming Assignment: Assignment: Naive Bayes
Week 3 - Vector Space Models
- Lab: Linear algebra in Python with Numpy
- Lab: Manipulating word embeddings
- Lab: Another explanation about PCA
- Programming Assignment: Assignment: Word Embeddings
Week 4 - Machine Translation and Document Search
- Lab: Rotation matrices in R2
- Lab: Hash tables
- Programming Assignment: Word Translation
2. Natural Language Processing with Probabilistic Models
Week 1 - autocorrect.
- Lab: Building the vocabulary
- Lab: Candidates from edits
- Programming Assignment: Autocorrect
Week 2 - Part of Speech Tagging and Hidden Markov Models
- Lab: Working with text data: numpy
- Lab: Working with text data: string tags
- Programming Assignment: Part of Speech Tagging
Week 3 - Autocomplete and Language Models
- Lab: Corpus preprocessing for N-grams
- Lab: Building the language model
- Lab: Language model generalization
- Programming Assignment: Autocomplete
Week 4 - Word Embeddings with Neural Networks
- Lab: Data Preparation
- Lab: Intro to CBOW model
- Lab: Training the CBOW model
- Lab: Word Embeddings
- Lab: Word embeddings step by step
- Programming Assignment: Word Embeddings
3. Natural Language Processing with Sequence Models
Week 1 - neural netowrks for sentiment analysis.
- Lab: Introduction to Trax
- Lab: Classes and Subclasses
- Lab: Data Generators
- Programming Assignment: Sentiment with Deep Neural Networks
Week 2 - Recurrent Neural Networks for Language Modelling
- Lab: Hidden State Activation
- Lab: Working with JAX NumPy and Calculating Perplexity
- Lab: Vanilla RNNs, GRUs and the scan function
- Lab: Creating a GRU model using Trax
- Programming Assignment: Deep N-grams
Week 3 - LSTMs and Named Entity Recognition
- Lab: Vanishing Gradients
- Programming Assignment: Named Entity Recognition (NER)
Week 4 - Siamese Networks
- Lab: Creating a Siamese Model using Trax
- Lab: Modified Triplet Loss
- Lab: Evaluate a Siamese Model
- Programming Assignment: Question duplicates
4. Natural Language Processing with Attention Models
Week 1 - neural machine translation.
- Lab: Stack Semantics
- Lab: BLEU Score
- Programming Assignment: NMT with Attention
Week 2 - Text Summarization
- Lab: Attention
- Lab: The Transformer Decoder
- Programming Assignment: Transformer Summarizer
Week 3 - Question Answering
- Lab: SentencePiece and BPE
- Lab: BERT Loss
- Programming Assignment: Question Answering
Week 4 - Chatbot
- Lab: Reformer LSH
- Lab: Revnet
- Programming Assignment: Chatbot
Deep Learning (Specialization)
1. neural networks and deep learning, week 1 - introduction to deep learning.
- No labs / programming assignments
Week 2 - Neural Network Basics
- Practice Programming Assignment: Python Basics with numpy (optional)
- Programming Assignment: Logistic Regression with a Neural Network mindset
Week 3 - Shallow Neural Networks
- Programming Assignment: Planar data classification with a hidden layer
Week 4 - Deep Neural Networks
- Programming Assignment: Building your deep neural network: Step by Step
- Programming Assignment: Deep Neural Network Application
2. Improving Deep Neural Networks Hyperparameter tuning, Regularization and Optimization
Week 1 - practical aspects of deep learning.
- Programming Assignment: Initialization
- Programming Assignment: Regularization
- Programming Assignment: Gradient Checking
Week 2 - Optimization Algorithms
- Programming Assignment: Optimization
Week 3 - Hyperparameter Tuning, Batch Normalization and Programming Frameworks
- Programming Assignment: Tensorflow
3. Structuring Machine Learning Projects
4. convolutional neural networks, week 1 - foundations of convolutional neural networks.
- Programming Assignment: Convolutional Model: step by step
- Programming Assignment: Convolutional model: application
Week 2 - Deep Convolutional Models: Case Studies
- Lab: Keras Tutorial (not graded)
- Programming Assignment: Residual Networks
Week 3 - Object Detection
- Programming Assignment: Car detection with YOLO
Week 4 - Special Applications: Face Recognition & Neural Style Transfer
- Programming Assignment: Art generation with Neural Style Transfer
- Programming Assignment: Face Recognition
5. Sequence Models
Week 1 - recurrent neural networks.
- Programming Assignment: Building a recurrent neural network - step by step
- Programming Assignment: Dinosaur Island - Character-Level Language Modeling
- Programming Assignment: Jazz improvisation with LSTM
Week 2 - Natural Language Processing & Word Embeddings
- Programming Assignment: Operations on word vectors - Debiasing
- Programming Assignment: Emojify
Week 3 - Sequence Models & Attention Mechanism
- Programming Assignment: Neural Machine Translation with Attention
- Programming Assignment: Trigger word detection
- Please refer to CONTRIBUTE.md for details. 😍
Coursera is licensed under the MIT license .
Contributors 2
- Jupyter Notebook 99.9%
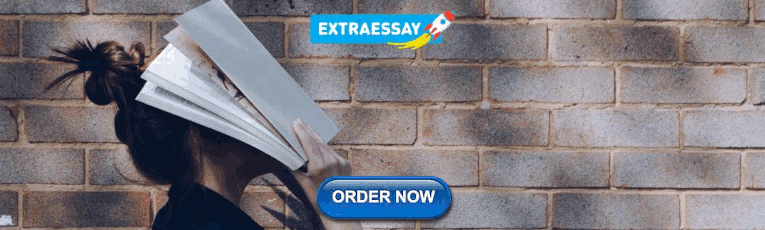
IMAGES
VIDEO
COMMENTS
Introduction to TensorFlow. Welcome to this week's programming assignment! Up until now, you've always used Numpy to build neural networks, but this week you'll explore a deep learning framework that allows you to build neural networks more easily. Machine learning frameworks like TensorFlow, PaddlePaddle, Torch, Caffe, Keras, and many others ...
Notes, programming assignments and quizzes from all courses within the Coursera Deep Learning specialization offered by deeplearning.ai: (i) Neural Networks and Deep Learning; (ii) Improving Deep Neural Networks: Hyperparameter tuning, Regularization and Optimization; (iii) Structuring Machine Learning Projects; (iv) Convolutional Neural Networks; (v) Sequence Models - amanchadha/coursera-deep ...
Week 2-Programming Assignment Python Basics with numpy; ... Week 3-Programming Assignment TensorFlow Introduction; Quizes. Week 1-Practical aspects of Deep Learning; Week 2-Optimization Algorithms; Week 3-Hyperparameter tuning; Grades. Certificate. Course 3: Structuring Machine Learning Projects.
The TensorFlow platform helps you implement best practices for data automation, model tracking, performance monitoring, and model retraining. Using production-level tools to automate and track model training over the lifetime of a product, service, or business process is critical to success. TFX provides software frameworks and tooling for full ...
TensorFlow 2 quickstart for beginners. This short introduction uses Keras to: Load a prebuilt dataset. Build a neural network machine learning model that classifies images. Train this neural network. Evaluate the accuracy of the model. This tutorial is a Google Colaboratory notebook.
An introduction to TensorFlow! Chip Huyen ([email protected]) CS224N 1/25/2018 1. 2. Agenda Why TensorFlow Graphs and Sessions Linear Regression tf.data word2vec Structuring your model Managing experiments 3. Why TensorFlow? Flexibility + Scalability Popularity 4. import tensorflow as tf 5.
The TensorFlow tutorials are written as Jupyter notebooks and run directly in Google Colab—a hosted notebook environment that requires no setup. At the top of each tutorial, you'll see a Run in Google Colab button. Click the button to open the notebook and run the code yourself.
16. Tensorflow is one of the widely used libraries for implementing Machine learning and other algorithms involving large number of mathematical operations. Tensorflow was developed by Google and it's one of the most popular Machine Learning libraries on GitHub. Google uses Tensorflow for implementing Machine learning in almost all applications.
Introduction to TensorFlow. Before you can build advanced models in TensorFlow 2, you will first need to understand the basics. In this chapter, you'll learn how to define constants and variables, perform tensor addition and multiplication, and compute derivatives. Knowledge of linear algebra will be helpful, but not necessary.
chapter03_introduction-to-keras-and-tf.i - Colab. Skip to main content. This is a companion notebook for the book Deep Learning with Python, Second Edition. For readability, it only contains runnable code blocks and section titles, and omits everything else in the book: text paragraphs, figures, and pseudocode.
Introduction to TensorFlow. Welcome to this week's programming assignment! Up until now, you've always used Numpy to build neural networks, but this week you'll explore a deep learning framework that allows you to build neural networks more easily. Machine learning frameworks like TensorFlow, PaddlePaddle, Torch, Caffe, Keras, and many others ...
The next tutorial is optional, and it is just us installing TensorFlow on a Windows machine. The next tutorial: Deep Learning with TensorFlow - Creating the Neural Network Model. Python Programming tutorials from beginner to advanced on a massive variety of topics. All video and text tutorials are free.
To do this conversion in numpy, you might have to write a few lines of code. In tensorflow, you can use one line of code: tf.one_hot (labels, depth, axis) Exercise: Implement the function below to take one vector of labels and the total number of classes C, and return the one hot encoding. Use tf.one_hot() to do this.
So that it can be used in many use cases, TensorFlow offers the most diverse deployment options. Among other things, it can be used in other programming languages, such as C or Java. With TensorFlow Serving, a powerful system is provided that offers the option to run the models either in the cloud or on on-premise servers.
This repository contains the assignments for the Coursera course Introduction to TensorFlow for Artificial Intelligence, Machine Learning, and Deep Learning. - prabh-me/Introduction-to-TensorFlow-for-Artificial-Intelligence-Machine-Learning-and-Deep-Learning-Coursera
Learn to Code — For Free
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
One of the most major changes was shifting from Tensorflow 1 to Tensorflow 2. Also, new materials were added. However, Most of the old online repositories still don't have old codes. This repo contains updated versions of the assignments. Happy Learning :) Programming Assignments. Course 1: Neural Networks and Deep Learning
Week 1 - Introduction to Deep Learning Quiz Introduction to Deep Learning; Week 2 - Neural Network Basics ... Programming Assignment: TensorFlow Introduction; Course 3 - Structuring Machine Learning Projects. Week 1 - ML Strategy Quiz: Bird Recognition in the City of Peacetopia (Case Study)
Introduction to TensorFlow for Artificial Intelligence, Machine Learning, and Deep Learning ... Programming Assignment: Tensorflow; 3. Structuring Machine Learning Projects. Details. No labs / programming assignments; 4. Convolutional Neural Networks. Details. Week 1 - Foundations of Convolutional Neural Networks.