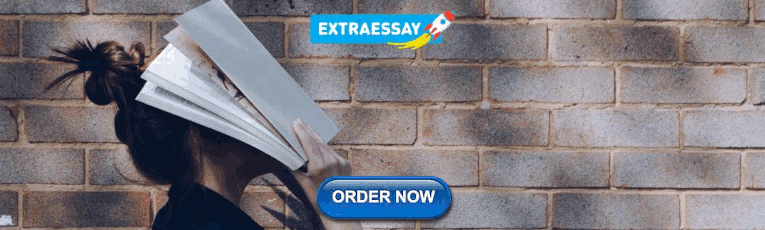
Assignment Expressions: The Walrus Operator
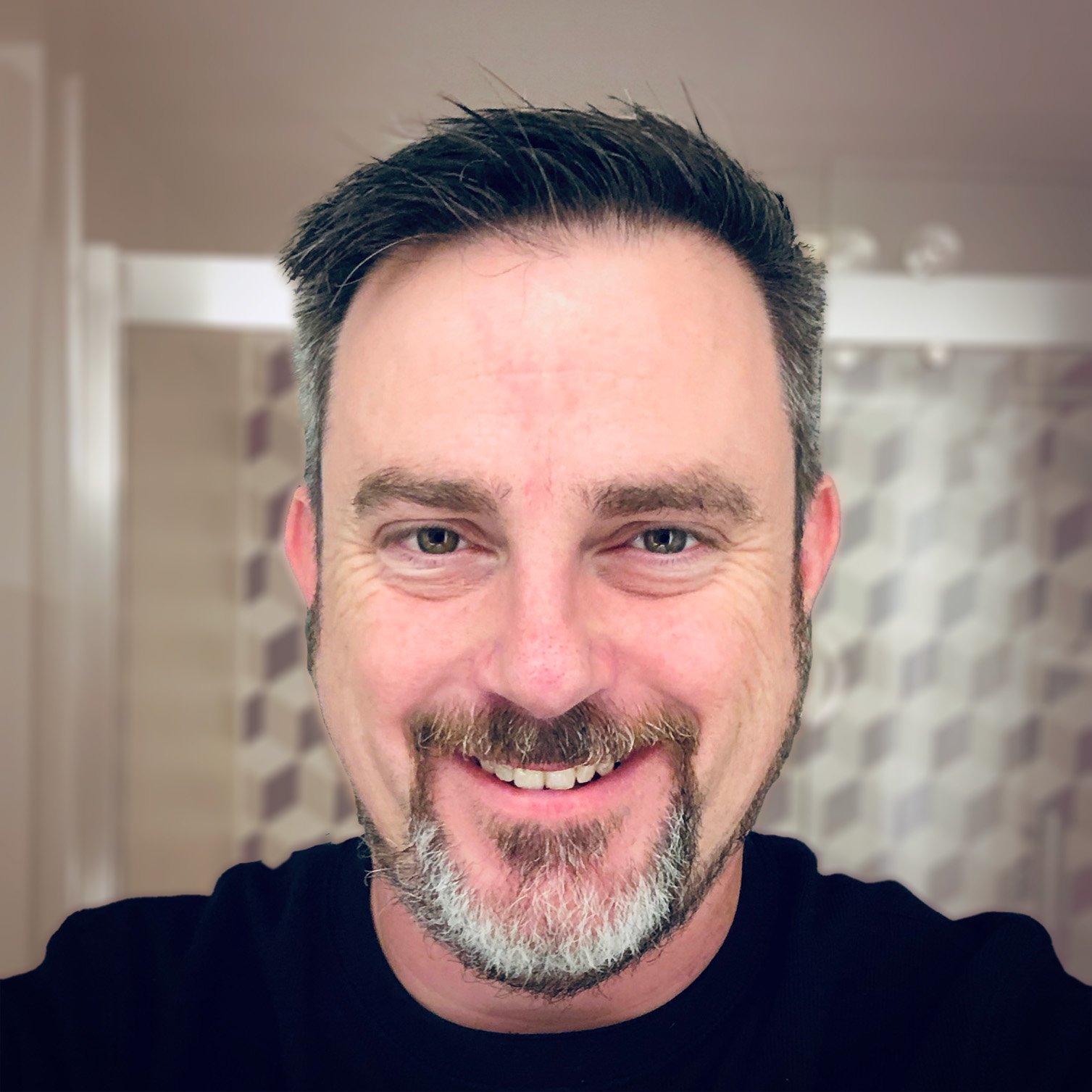
- Discussion (8)
In this lesson, you’ll learn about the biggest change in Python 3.8: the introduction of assignment expressions . Assignment expression are written with a new notation (:=) .This operator is often called the walrus operator as it resembles the eyes and tusks of a walrus on its side.
Assignment expressions allow you to assign and return a value in the same expression. For example, if you want to assign to a variable and print its value, then you typically do something like this:
In Python 3.8, you’re allowed to combine these two statements into one, using the walrus operator:
The assignment expression allows you to assign True to walrus , and immediately print the value. But keep in mind that the walrus operator does not do anything that isn’t possible without it. It only makes certain constructs more convenient, and can sometimes communicate the intent of your code more clearly.
One pattern that shows some of the strengths of the walrus operator is while loops where you need to initialize and update a variable. For example, the following code asks the user for input until they type quit :
This code is less than ideal. You’re repeating the input() statement, and somehow you need to add current to the list before asking the user for it. A better solution is to set up an infinite while loop, and use break to stop the loop:
This code is equivalent to the code above, but avoids the repetition and somehow keeps the lines in a more logical order. If you use an assignment expression, then you can simplify this loop further:
This moves the test back to the while line, where it should be. However, there are now several things happening at that line, so it takes a bit more effort to read it properly. Use your best judgement about when the walrus operator helps make your code more readable.
PEP 572 describes all the details of assignment expressions, including some of the rationale for introducing them into the language, as well as several examples of how the walrus operator can be used. The Python 3.8 documentation also includes some good examples of assignment expressions.
Here are a few resources for more info on using bpython, the REPL (Read–Eval–Print Loop) tool used in most of these videos:
- Discover bpython: A Python REPL With IDE-Like Features
- A better Python REPL: bpython vs python
- bpython Homepage
- bpython Docs
00:00 In this video, you’ll learn about what’s being called the walrus operator. One of the biggest changes in Python 3.8 is the introduction of these assignment expressions. So, what does it do?
00:12 Well, it allows the assignment and the return of a value in the same expression, using a new notation. On the left side, you’d have the name of the object that you’re assigning, and then you have the operator, a colon and an equal sign ( := ), affectionately known as the walrus operator as it resembles the eyes and tusks of a walrus on its side.
00:32 And it’s assigning this expression on the right side, so it’s assigning and returning the value in the same expression. Let me have you practice with this operator with some code.
00:44 Throughout this tutorial, when I use a REPL, I’m going to be using this custom REPL called bpython . I’ll include links on how to install bpython below this video.
00:53 So, how do you use this assignment operator? Let me have you start with a small example. You could have an object named walrus and assign it the value of False , and then you could print it. In Python 3.8, you can combine those two statements and do a single statement using the walrus operator. So inside of print() , you could say walrus , the new object, and use the operator, the assignment expression := , and a space, and then say True . That’s going to do two things. Most notably, in reverse order, it returned the value True . And then it also assigned the value to walrus , and of course the type of 'bool' .
01:38 Keep in mind, the walrus operator doesn’t do anything that isn’t possible without it. It only makes certain constructs a bit more convenient, and can sometimes communicate the intent of your code more clearly.
01:48 Let me show you another example. It’s a pattern that shows some of the strengths of the walrus operator inside of while loops, where you need to initialize and update a variable. For example, create a new file, and name it write_something.py . Here’s write_something.py .
02:09 It starts with inputs , which will be a list. So create a list called inputs .
02:16 Into an object named current , use an input() statement. The input() statement is going to provide a prompt and read a string in from standard input. The prompt will be this, "Write something: " .
02:28 So when the user inputs that, that’ll go into current . So while current != "quit" — if the person has not typed quit yet— you’re going to take inputs and append the current value.
02:44 And then here, you’re asking to "Write something: " again.
02:50 Down here at my terminal, after saving—let’s see, make sure you’re saved. Okay. Now that’s saved.
03:00 So here, I could say, Hello , Welcome , and then finally quit , which then would quit it. So, this code isn’t ideal.
03:08 You’re repeating the input() statement twice, and somehow you need to add current to the list before asking the user for it. So a better solution is going to be to set up maybe an infinite while loop, and then use a break to stop the loop. How would that look?
03:22 You’re going to rearrange this a little bit. Move the while loop up, and say while True:
03:35 and here say if current == "quit": then break . Otherwise, go ahead and append it. So, a little different here, but this is a while loop that’s going to continue as long as it doesn’t get broken out of by someone typing quit . Okay.
03:53 Running it again. And there, you can see it breaking out. Nice. So, that code avoids the repetition and kind of keeps things in a more logical order, but there’s a way to simplify this to use that new assignment expression, the walrus operator. In that case, you’re going to modify this quite a bit.
04:17 Here you’re going to say while , current and then use that assignment operator ( := ) to create current .
04:23 But also, while doing that, check to see that it’s not equal to "quit" . So here, each time that assigns the value to current and it’s returned, so the value can be checked.
04:35 So while , current , assigning the value from the input() , and then if it’s not equal to "quit" , you’re going to append current . Make sure to save.
04:42 Run the code one more time.
04:47 And it works the same. This moves that test all the way back to the while line, where it should be. However, there’s a couple of things now happening all in one line, and that might take a little more effort to read what’s happening and to understand it properly.
05:00 There are a handful of other examples that you could look into to learn a little more about assignment expressions. I’ll include a link to PEP 572, and also a link to the Python docs for version 3.8, both of which include more code examples.
05:14 So you need to use your best judgment as to when this operator’s going to make your code more readable and more useful. In the next video, you’ll learn about the new feature of positional-only arguments.
rajeshboyalla on Dec. 4, 2019
Why do you use list() to initialize a list rather than using [] ?
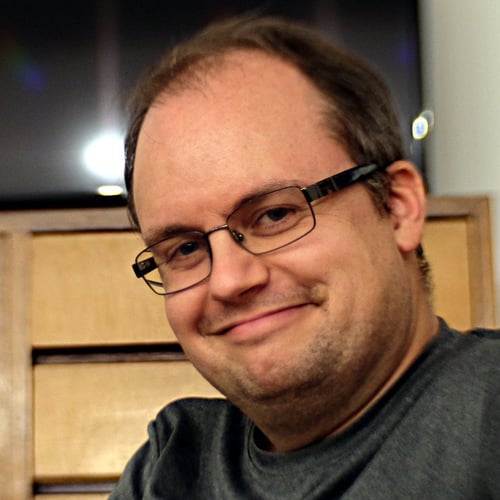
Geir Arne Hjelle RP Team on Dec. 4, 2019
My two cents about list() vs [] (I wrote the original article this video series is based on):
- I find spelling out list() to be more readable and easier to notice and interpret than []
- [] is several times faster than list() , but we’re still talking nanoseconds. On my computer [] takes about 15ns, while list() runs in 60ns. Typically, lists are initiated once, so this does not cause any meaningful slowdown of code.
That said, if I’m initializing a list with existing elements, I usually use [elem1, elem2, ...] , since list(...) has different–and sometimes surprising–semantics.
Jason on April 3, 2020
Sorry for my ignorance, did the the standard assignment = operator work in this way? I don’t understand what has been gained from adding the := operator. If anything I think it will allow people to write more obfuscated code. But minds better than mine have been working on this, so I’ll have to take their word it is an improvement.
As for the discussion on whether [] is more readable than list(). I’d never seen list() before, so to me [] is better. I’ve only just come over from the dark 2.7 side so maybe it’s an old python programmer thing?
Oh I checked the operation on the assignment operator. I was obviously wrong. lol Still I think the existing operator could’ve been tweaked to do the same thing as := … I’m still on the fence about that one.
gedece on April 3, 2020
you are right in that the existing operator could have worked, but it can lead to something unexpected.
if you do something like
if (newvar = somevar): it gives a traceback, because you are supposed to use == for comparations.
So if you use the normal operator for this, then that expression is valid and you’ll be hard pressed to realize the mistake.
It then makes complete sense to use a different operator as that helps to clarify intent in code.
Jason on April 6, 2020
Yes, I’ve accidentaly done that in other languages before and it can be a difficult to “see” bug.
varelaautumn on Sept. 26, 2020
I watched this earlier today and now tonight I just can’t stop myself from throwing these walrus operators everywhere.
(I’m new and learning so these are just personal fooling around programs)
For example I have this function which cycles through a bunch of other very simple parsing functions that check if my input string is valid in the context of the game state. If the string doesn’t pass one of these parsers it returns a string with an error message such as “Input must be less than 5 characters”. And then the parse_input function returns that error string.
I mean it’s not a huge change, but it saves an extra call of the function, and I feel like it makes it much more readable.
I’m not sure if this other case might be considered abuse of the walrus operator, but I decided to use it twice in one line.
This function repeatedly asks for input. If the input does not pass the parser functions, then the error will be returned and printed out in the while loop. Otherwise the input was valid and it gets returned.
I’m able to pass my input into a function and check the result of that function all while retaining my input and the return of the function as their own variables to be used in the next line.
I think the walrus operator helped me put all the relevant details on the three lines. Like if you just read the first words of each line, it basically says “while error, print error, else return input_string.” I don’t see how I could have done that without this cool walrus operator so I’m really appreciative for this video you made! I’ve been converted to a strong believer in the walrus operator.
Geir Arne Hjelle RP Team on Sept. 26, 2020
@varelaautumn Nice examples, thanks for sharing!
I agree that the walrus operator will not revolutionize your code, but it can bring these sorts of small improvements that add up in the long run.
Become a Member to join the conversation.
Python Enhancement Proposals
- Python »
- PEP Index »
PEP 572 – Assignment Expressions
The importance of real code, exceptional cases, scope of the target, relative precedence of :=, change to evaluation order, differences between assignment expressions and assignment statements, specification changes during implementation, _pydecimal.py, datetime.py, sysconfig.py, simplifying list comprehensions, capturing condition values, changing the scope rules for comprehensions, alternative spellings, special-casing conditional statements, special-casing comprehensions, lowering operator precedence, allowing commas to the right, always requiring parentheses, why not just turn existing assignment into an expression, with assignment expressions, why bother with assignment statements, why not use a sublocal scope and prevent namespace pollution, style guide recommendations, acknowledgements, a numeric example, appendix b: rough code translations for comprehensions, appendix c: no changes to scope semantics.
This is a proposal for creating a way to assign to variables within an expression using the notation NAME := expr .
As part of this change, there is also an update to dictionary comprehension evaluation order to ensure key expressions are executed before value expressions (allowing the key to be bound to a name and then re-used as part of calculating the corresponding value).
During discussion of this PEP, the operator became informally known as “the walrus operator”. The construct’s formal name is “Assignment Expressions” (as per the PEP title), but they may also be referred to as “Named Expressions” (e.g. the CPython reference implementation uses that name internally).
Naming the result of an expression is an important part of programming, allowing a descriptive name to be used in place of a longer expression, and permitting reuse. Currently, this feature is available only in statement form, making it unavailable in list comprehensions and other expression contexts.
Additionally, naming sub-parts of a large expression can assist an interactive debugger, providing useful display hooks and partial results. Without a way to capture sub-expressions inline, this would require refactoring of the original code; with assignment expressions, this merely requires the insertion of a few name := markers. Removing the need to refactor reduces the likelihood that the code be inadvertently changed as part of debugging (a common cause of Heisenbugs), and is easier to dictate to another programmer.
During the development of this PEP many people (supporters and critics both) have had a tendency to focus on toy examples on the one hand, and on overly complex examples on the other.
The danger of toy examples is twofold: they are often too abstract to make anyone go “ooh, that’s compelling”, and they are easily refuted with “I would never write it that way anyway”.
The danger of overly complex examples is that they provide a convenient strawman for critics of the proposal to shoot down (“that’s obfuscated”).
Yet there is some use for both extremely simple and extremely complex examples: they are helpful to clarify the intended semantics. Therefore, there will be some of each below.
However, in order to be compelling , examples should be rooted in real code, i.e. code that was written without any thought of this PEP, as part of a useful application, however large or small. Tim Peters has been extremely helpful by going over his own personal code repository and picking examples of code he had written that (in his view) would have been clearer if rewritten with (sparing) use of assignment expressions. His conclusion: the current proposal would have allowed a modest but clear improvement in quite a few bits of code.
Another use of real code is to observe indirectly how much value programmers place on compactness. Guido van Rossum searched through a Dropbox code base and discovered some evidence that programmers value writing fewer lines over shorter lines.
Case in point: Guido found several examples where a programmer repeated a subexpression, slowing down the program, in order to save one line of code, e.g. instead of writing:
they would write:
Another example illustrates that programmers sometimes do more work to save an extra level of indentation:
This code tries to match pattern2 even if pattern1 has a match (in which case the match on pattern2 is never used). The more efficient rewrite would have been:
Syntax and semantics
In most contexts where arbitrary Python expressions can be used, a named expression can appear. This is of the form NAME := expr where expr is any valid Python expression other than an unparenthesized tuple, and NAME is an identifier.
The value of such a named expression is the same as the incorporated expression, with the additional side-effect that the target is assigned that value:
There are a few places where assignment expressions are not allowed, in order to avoid ambiguities or user confusion:
This rule is included to simplify the choice for the user between an assignment statement and an assignment expression – there is no syntactic position where both are valid.
Again, this rule is included to avoid two visually similar ways of saying the same thing.
This rule is included to disallow excessively confusing code, and because parsing keyword arguments is complex enough already.
This rule is included to discourage side effects in a position whose exact semantics are already confusing to many users (cf. the common style recommendation against mutable default values), and also to echo the similar prohibition in calls (the previous bullet).
The reasoning here is similar to the two previous cases; this ungrouped assortment of symbols and operators composed of : and = is hard to read correctly.
This allows lambda to always bind less tightly than := ; having a name binding at the top level inside a lambda function is unlikely to be of value, as there is no way to make use of it. In cases where the name will be used more than once, the expression is likely to need parenthesizing anyway, so this prohibition will rarely affect code.
This shows that what looks like an assignment operator in an f-string is not always an assignment operator. The f-string parser uses : to indicate formatting options. To preserve backwards compatibility, assignment operator usage inside of f-strings must be parenthesized. As noted above, this usage of the assignment operator is not recommended.
An assignment expression does not introduce a new scope. In most cases the scope in which the target will be bound is self-explanatory: it is the current scope. If this scope contains a nonlocal or global declaration for the target, the assignment expression honors that. A lambda (being an explicit, if anonymous, function definition) counts as a scope for this purpose.
There is one special case: an assignment expression occurring in a list, set or dict comprehension or in a generator expression (below collectively referred to as “comprehensions”) binds the target in the containing scope, honoring a nonlocal or global declaration for the target in that scope, if one exists. For the purpose of this rule the containing scope of a nested comprehension is the scope that contains the outermost comprehension. A lambda counts as a containing scope.
The motivation for this special case is twofold. First, it allows us to conveniently capture a “witness” for an any() expression, or a counterexample for all() , for example:
Second, it allows a compact way of updating mutable state from a comprehension, for example:
However, an assignment expression target name cannot be the same as a for -target name appearing in any comprehension containing the assignment expression. The latter names are local to the comprehension in which they appear, so it would be contradictory for a contained use of the same name to refer to the scope containing the outermost comprehension instead.
For example, [i := i+1 for i in range(5)] is invalid: the for i part establishes that i is local to the comprehension, but the i := part insists that i is not local to the comprehension. The same reason makes these examples invalid too:
While it’s technically possible to assign consistent semantics to these cases, it’s difficult to determine whether those semantics actually make sense in the absence of real use cases. Accordingly, the reference implementation [1] will ensure that such cases raise SyntaxError , rather than executing with implementation defined behaviour.
This restriction applies even if the assignment expression is never executed:
For the comprehension body (the part before the first “for” keyword) and the filter expression (the part after “if” and before any nested “for”), this restriction applies solely to target names that are also used as iteration variables in the comprehension. Lambda expressions appearing in these positions introduce a new explicit function scope, and hence may use assignment expressions with no additional restrictions.
Due to design constraints in the reference implementation (the symbol table analyser cannot easily detect when names are re-used between the leftmost comprehension iterable expression and the rest of the comprehension), named expressions are disallowed entirely as part of comprehension iterable expressions (the part after each “in”, and before any subsequent “if” or “for” keyword):
A further exception applies when an assignment expression occurs in a comprehension whose containing scope is a class scope. If the rules above were to result in the target being assigned in that class’s scope, the assignment expression is expressly invalid. This case also raises SyntaxError :
(The reason for the latter exception is the implicit function scope created for comprehensions – there is currently no runtime mechanism for a function to refer to a variable in the containing class scope, and we do not want to add such a mechanism. If this issue ever gets resolved this special case may be removed from the specification of assignment expressions. Note that the problem already exists for using a variable defined in the class scope from a comprehension.)
See Appendix B for some examples of how the rules for targets in comprehensions translate to equivalent code.
The := operator groups more tightly than a comma in all syntactic positions where it is legal, but less tightly than all other operators, including or , and , not , and conditional expressions ( A if C else B ). As follows from section “Exceptional cases” above, it is never allowed at the same level as = . In case a different grouping is desired, parentheses should be used.
The := operator may be used directly in a positional function call argument; however it is invalid directly in a keyword argument.
Some examples to clarify what’s technically valid or invalid:
Most of the “valid” examples above are not recommended, since human readers of Python source code who are quickly glancing at some code may miss the distinction. But simple cases are not objectionable:
This PEP recommends always putting spaces around := , similar to PEP 8 ’s recommendation for = when used for assignment, whereas the latter disallows spaces around = used for keyword arguments.)
In order to have precisely defined semantics, the proposal requires evaluation order to be well-defined. This is technically not a new requirement, as function calls may already have side effects. Python already has a rule that subexpressions are generally evaluated from left to right. However, assignment expressions make these side effects more visible, and we propose a single change to the current evaluation order:
- In a dict comprehension {X: Y for ...} , Y is currently evaluated before X . We propose to change this so that X is evaluated before Y . (In a dict display like {X: Y} this is already the case, and also in dict((X, Y) for ...) which should clearly be equivalent to the dict comprehension.)
Most importantly, since := is an expression, it can be used in contexts where statements are illegal, including lambda functions and comprehensions.
Conversely, assignment expressions don’t support the advanced features found in assignment statements:
- Multiple targets are not directly supported: x = y = z = 0 # Equivalent: (z := (y := (x := 0)))
- Single assignment targets other than a single NAME are not supported: # No equivalent a [ i ] = x self . rest = []
- Priority around commas is different: x = 1 , 2 # Sets x to (1, 2) ( x := 1 , 2 ) # Sets x to 1
- Iterable packing and unpacking (both regular or extended forms) are not supported: # Equivalent needs extra parentheses loc = x , y # Use (loc := (x, y)) info = name , phone , * rest # Use (info := (name, phone, *rest)) # No equivalent px , py , pz = position name , phone , email , * other_info = contact
- Inline type annotations are not supported: # Closest equivalent is "p: Optional[int]" as a separate declaration p : Optional [ int ] = None
- Augmented assignment is not supported: total += tax # Equivalent: (total := total + tax)
The following changes have been made based on implementation experience and additional review after the PEP was first accepted and before Python 3.8 was released:
- for consistency with other similar exceptions, and to avoid locking in an exception name that is not necessarily going to improve clarity for end users, the originally proposed TargetScopeError subclass of SyntaxError was dropped in favour of just raising SyntaxError directly. [3]
- due to a limitation in CPython’s symbol table analysis process, the reference implementation raises SyntaxError for all uses of named expressions inside comprehension iterable expressions, rather than only raising them when the named expression target conflicts with one of the iteration variables in the comprehension. This could be revisited given sufficiently compelling examples, but the extra complexity needed to implement the more selective restriction doesn’t seem worthwhile for purely hypothetical use cases.
Examples from the Python standard library
env_base is only used on these lines, putting its assignment on the if moves it as the “header” of the block.
- Current: env_base = os . environ . get ( "PYTHONUSERBASE" , None ) if env_base : return env_base
- Improved: if env_base := os . environ . get ( "PYTHONUSERBASE" , None ): return env_base
Avoid nested if and remove one indentation level.
- Current: if self . _is_special : ans = self . _check_nans ( context = context ) if ans : return ans
- Improved: if self . _is_special and ( ans := self . _check_nans ( context = context )): return ans
Code looks more regular and avoid multiple nested if. (See Appendix A for the origin of this example.)
- Current: reductor = dispatch_table . get ( cls ) if reductor : rv = reductor ( x ) else : reductor = getattr ( x , "__reduce_ex__" , None ) if reductor : rv = reductor ( 4 ) else : reductor = getattr ( x , "__reduce__" , None ) if reductor : rv = reductor () else : raise Error ( "un(deep)copyable object of type %s " % cls )
- Improved: if reductor := dispatch_table . get ( cls ): rv = reductor ( x ) elif reductor := getattr ( x , "__reduce_ex__" , None ): rv = reductor ( 4 ) elif reductor := getattr ( x , "__reduce__" , None ): rv = reductor () else : raise Error ( "un(deep)copyable object of type %s " % cls )
tz is only used for s += tz , moving its assignment inside the if helps to show its scope.
- Current: s = _format_time ( self . _hour , self . _minute , self . _second , self . _microsecond , timespec ) tz = self . _tzstr () if tz : s += tz return s
- Improved: s = _format_time ( self . _hour , self . _minute , self . _second , self . _microsecond , timespec ) if tz := self . _tzstr (): s += tz return s
Calling fp.readline() in the while condition and calling .match() on the if lines make the code more compact without making it harder to understand.
- Current: while True : line = fp . readline () if not line : break m = define_rx . match ( line ) if m : n , v = m . group ( 1 , 2 ) try : v = int ( v ) except ValueError : pass vars [ n ] = v else : m = undef_rx . match ( line ) if m : vars [ m . group ( 1 )] = 0
- Improved: while line := fp . readline (): if m := define_rx . match ( line ): n , v = m . group ( 1 , 2 ) try : v = int ( v ) except ValueError : pass vars [ n ] = v elif m := undef_rx . match ( line ): vars [ m . group ( 1 )] = 0
A list comprehension can map and filter efficiently by capturing the condition:
Similarly, a subexpression can be reused within the main expression, by giving it a name on first use:
Note that in both cases the variable y is bound in the containing scope (i.e. at the same level as results or stuff ).
Assignment expressions can be used to good effect in the header of an if or while statement:
Particularly with the while loop, this can remove the need to have an infinite loop, an assignment, and a condition. It also creates a smooth parallel between a loop which simply uses a function call as its condition, and one which uses that as its condition but also uses the actual value.
An example from the low-level UNIX world:
Rejected alternative proposals
Proposals broadly similar to this one have come up frequently on python-ideas. Below are a number of alternative syntaxes, some of them specific to comprehensions, which have been rejected in favour of the one given above.
A previous version of this PEP proposed subtle changes to the scope rules for comprehensions, to make them more usable in class scope and to unify the scope of the “outermost iterable” and the rest of the comprehension. However, this part of the proposal would have caused backwards incompatibilities, and has been withdrawn so the PEP can focus on assignment expressions.
Broadly the same semantics as the current proposal, but spelled differently.
Since EXPR as NAME already has meaning in import , except and with statements (with different semantics), this would create unnecessary confusion or require special-casing (e.g. to forbid assignment within the headers of these statements).
(Note that with EXPR as VAR does not simply assign the value of EXPR to VAR – it calls EXPR.__enter__() and assigns the result of that to VAR .)
Additional reasons to prefer := over this spelling include:
- In if f(x) as y the assignment target doesn’t jump out at you – it just reads like if f x blah blah and it is too similar visually to if f(x) and y .
- import foo as bar
- except Exc as var
- with ctxmgr() as var
To the contrary, the assignment expression does not belong to the if or while that starts the line, and we intentionally allow assignment expressions in other contexts as well.
- NAME = EXPR
- if NAME := EXPR
reinforces the visual recognition of assignment expressions.
This syntax is inspired by languages such as R and Haskell, and some programmable calculators. (Note that a left-facing arrow y <- f(x) is not possible in Python, as it would be interpreted as less-than and unary minus.) This syntax has a slight advantage over ‘as’ in that it does not conflict with with , except and import , but otherwise is equivalent. But it is entirely unrelated to Python’s other use of -> (function return type annotations), and compared to := (which dates back to Algol-58) it has a much weaker tradition.
This has the advantage that leaked usage can be readily detected, removing some forms of syntactic ambiguity. However, this would be the only place in Python where a variable’s scope is encoded into its name, making refactoring harder.
Execution order is inverted (the indented body is performed first, followed by the “header”). This requires a new keyword, unless an existing keyword is repurposed (most likely with: ). See PEP 3150 for prior discussion on this subject (with the proposed keyword being given: ).
This syntax has fewer conflicts than as does (conflicting only with the raise Exc from Exc notation), but is otherwise comparable to it. Instead of paralleling with expr as target: (which can be useful but can also be confusing), this has no parallels, but is evocative.
One of the most popular use-cases is if and while statements. Instead of a more general solution, this proposal enhances the syntax of these two statements to add a means of capturing the compared value:
This works beautifully if and ONLY if the desired condition is based on the truthiness of the captured value. It is thus effective for specific use-cases (regex matches, socket reads that return '' when done), and completely useless in more complicated cases (e.g. where the condition is f(x) < 0 and you want to capture the value of f(x) ). It also has no benefit to list comprehensions.
Advantages: No syntactic ambiguities. Disadvantages: Answers only a fraction of possible use-cases, even in if / while statements.
Another common use-case is comprehensions (list/set/dict, and genexps). As above, proposals have been made for comprehension-specific solutions.
This brings the subexpression to a location in between the ‘for’ loop and the expression. It introduces an additional language keyword, which creates conflicts. Of the three, where reads the most cleanly, but also has the greatest potential for conflict (e.g. SQLAlchemy and numpy have where methods, as does tkinter.dnd.Icon in the standard library).
As above, but reusing the with keyword. Doesn’t read too badly, and needs no additional language keyword. Is restricted to comprehensions, though, and cannot as easily be transformed into “longhand” for-loop syntax. Has the C problem that an equals sign in an expression can now create a name binding, rather than performing a comparison. Would raise the question of why “with NAME = EXPR:” cannot be used as a statement on its own.
As per option 2, but using as rather than an equals sign. Aligns syntactically with other uses of as for name binding, but a simple transformation to for-loop longhand would create drastically different semantics; the meaning of with inside a comprehension would be completely different from the meaning as a stand-alone statement, while retaining identical syntax.
Regardless of the spelling chosen, this introduces a stark difference between comprehensions and the equivalent unrolled long-hand form of the loop. It is no longer possible to unwrap the loop into statement form without reworking any name bindings. The only keyword that can be repurposed to this task is with , thus giving it sneakily different semantics in a comprehension than in a statement; alternatively, a new keyword is needed, with all the costs therein.
There are two logical precedences for the := operator. Either it should bind as loosely as possible, as does statement-assignment; or it should bind more tightly than comparison operators. Placing its precedence between the comparison and arithmetic operators (to be precise: just lower than bitwise OR) allows most uses inside while and if conditions to be spelled without parentheses, as it is most likely that you wish to capture the value of something, then perform a comparison on it:
Once find() returns -1, the loop terminates. If := binds as loosely as = does, this would capture the result of the comparison (generally either True or False ), which is less useful.
While this behaviour would be convenient in many situations, it is also harder to explain than “the := operator behaves just like the assignment statement”, and as such, the precedence for := has been made as close as possible to that of = (with the exception that it binds tighter than comma).
Some critics have claimed that the assignment expressions should allow unparenthesized tuples on the right, so that these two would be equivalent:
(With the current version of the proposal, the latter would be equivalent to ((point := x), y) .)
However, adopting this stance would logically lead to the conclusion that when used in a function call, assignment expressions also bind less tight than comma, so we’d have the following confusing equivalence:
The less confusing option is to make := bind more tightly than comma.
It’s been proposed to just always require parentheses around an assignment expression. This would resolve many ambiguities, and indeed parentheses will frequently be needed to extract the desired subexpression. But in the following cases the extra parentheses feel redundant:
Frequently Raised Objections
C and its derivatives define the = operator as an expression, rather than a statement as is Python’s way. This allows assignments in more contexts, including contexts where comparisons are more common. The syntactic similarity between if (x == y) and if (x = y) belies their drastically different semantics. Thus this proposal uses := to clarify the distinction.
The two forms have different flexibilities. The := operator can be used inside a larger expression; the = statement can be augmented to += and its friends, can be chained, and can assign to attributes and subscripts.
Previous revisions of this proposal involved sublocal scope (restricted to a single statement), preventing name leakage and namespace pollution. While a definite advantage in a number of situations, this increases complexity in many others, and the costs are not justified by the benefits. In the interests of language simplicity, the name bindings created here are exactly equivalent to any other name bindings, including that usage at class or module scope will create externally-visible names. This is no different from for loops or other constructs, and can be solved the same way: del the name once it is no longer needed, or prefix it with an underscore.
(The author wishes to thank Guido van Rossum and Christoph Groth for their suggestions to move the proposal in this direction. [2] )
As expression assignments can sometimes be used equivalently to statement assignments, the question of which should be preferred will arise. For the benefit of style guides such as PEP 8 , two recommendations are suggested.
- If either assignment statements or assignment expressions can be used, prefer statements; they are a clear declaration of intent.
- If using assignment expressions would lead to ambiguity about execution order, restructure it to use statements instead.
The authors wish to thank Alyssa Coghlan and Steven D’Aprano for their considerable contributions to this proposal, and members of the core-mentorship mailing list for assistance with implementation.
Appendix A: Tim Peters’s findings
Here’s a brief essay Tim Peters wrote on the topic.
I dislike “busy” lines of code, and also dislike putting conceptually unrelated logic on a single line. So, for example, instead of:
instead. So I suspected I’d find few places I’d want to use assignment expressions. I didn’t even consider them for lines already stretching halfway across the screen. In other cases, “unrelated” ruled:
is a vast improvement over the briefer:
The original two statements are doing entirely different conceptual things, and slamming them together is conceptually insane.
In other cases, combining related logic made it harder to understand, such as rewriting:
as the briefer:
The while test there is too subtle, crucially relying on strict left-to-right evaluation in a non-short-circuiting or method-chaining context. My brain isn’t wired that way.
But cases like that were rare. Name binding is very frequent, and “sparse is better than dense” does not mean “almost empty is better than sparse”. For example, I have many functions that return None or 0 to communicate “I have nothing useful to return in this case, but since that’s expected often I’m not going to annoy you with an exception”. This is essentially the same as regular expression search functions returning None when there is no match. So there was lots of code of the form:
I find that clearer, and certainly a bit less typing and pattern-matching reading, as:
It’s also nice to trade away a small amount of horizontal whitespace to get another _line_ of surrounding code on screen. I didn’t give much weight to this at first, but it was so very frequent it added up, and I soon enough became annoyed that I couldn’t actually run the briefer code. That surprised me!
There are other cases where assignment expressions really shine. Rather than pick another from my code, Kirill Balunov gave a lovely example from the standard library’s copy() function in copy.py :
The ever-increasing indentation is semantically misleading: the logic is conceptually flat, “the first test that succeeds wins”:
Using easy assignment expressions allows the visual structure of the code to emphasize the conceptual flatness of the logic; ever-increasing indentation obscured it.
A smaller example from my code delighted me, both allowing to put inherently related logic in a single line, and allowing to remove an annoying “artificial” indentation level:
That if is about as long as I want my lines to get, but remains easy to follow.
So, in all, in most lines binding a name, I wouldn’t use assignment expressions, but because that construct is so very frequent, that leaves many places I would. In most of the latter, I found a small win that adds up due to how often it occurs, and in the rest I found a moderate to major win. I’d certainly use it more often than ternary if , but significantly less often than augmented assignment.
I have another example that quite impressed me at the time.
Where all variables are positive integers, and a is at least as large as the n’th root of x, this algorithm returns the floor of the n’th root of x (and roughly doubling the number of accurate bits per iteration):
It’s not obvious why that works, but is no more obvious in the “loop and a half” form. It’s hard to prove correctness without building on the right insight (the “arithmetic mean - geometric mean inequality”), and knowing some non-trivial things about how nested floor functions behave. That is, the challenges are in the math, not really in the coding.
If you do know all that, then the assignment-expression form is easily read as “while the current guess is too large, get a smaller guess”, where the “too large?” test and the new guess share an expensive sub-expression.
To my eyes, the original form is harder to understand:
This appendix attempts to clarify (though not specify) the rules when a target occurs in a comprehension or in a generator expression. For a number of illustrative examples we show the original code, containing a comprehension, and the translation, where the comprehension has been replaced by an equivalent generator function plus some scaffolding.
Since [x for ...] is equivalent to list(x for ...) these examples all use list comprehensions without loss of generality. And since these examples are meant to clarify edge cases of the rules, they aren’t trying to look like real code.
Note: comprehensions are already implemented via synthesizing nested generator functions like those in this appendix. The new part is adding appropriate declarations to establish the intended scope of assignment expression targets (the same scope they resolve to as if the assignment were performed in the block containing the outermost comprehension). For type inference purposes, these illustrative expansions do not imply that assignment expression targets are always Optional (but they do indicate the target binding scope).
Let’s start with a reminder of what code is generated for a generator expression without assignment expression.
- Original code (EXPR usually references VAR): def f (): a = [ EXPR for VAR in ITERABLE ]
- Translation (let’s not worry about name conflicts): def f (): def genexpr ( iterator ): for VAR in iterator : yield EXPR a = list ( genexpr ( iter ( ITERABLE )))
Let’s add a simple assignment expression.
- Original code: def f (): a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def f (): if False : TARGET = None # Dead code to ensure TARGET is a local variable def genexpr ( iterator ): nonlocal TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Let’s add a global TARGET declaration in f() .
- Original code: def f (): global TARGET a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def f (): global TARGET def genexpr ( iterator ): global TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Or instead let’s add a nonlocal TARGET declaration in f() .
- Original code: def g (): TARGET = ... def f (): nonlocal TARGET a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def g (): TARGET = ... def f (): nonlocal TARGET def genexpr ( iterator ): nonlocal TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Finally, let’s nest two comprehensions.
- Original code: def f (): a = [[ TARGET := i for i in range ( 3 )] for j in range ( 2 )] # I.e., a = [[0, 1, 2], [0, 1, 2]] print ( TARGET ) # prints 2
- Translation: def f (): if False : TARGET = None def outer_genexpr ( outer_iterator ): nonlocal TARGET def inner_generator ( inner_iterator ): nonlocal TARGET for i in inner_iterator : TARGET = i yield i for j in outer_iterator : yield list ( inner_generator ( range ( 3 ))) a = list ( outer_genexpr ( range ( 2 ))) print ( TARGET )
Because it has been a point of confusion, note that nothing about Python’s scoping semantics is changed. Function-local scopes continue to be resolved at compile time, and to have indefinite temporal extent at run time (“full closures”). Example:
This document has been placed in the public domain.
Source: https://github.com/python/peps/blob/main/peps/pep-0572.rst
Last modified: 2023-10-11 12:05:51 GMT
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Assignment operators (C# reference)
- 11 contributors
The assignment operator = assigns the value of its right-hand operand to a variable, a property , or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or implicitly convertible to it.
The assignment operator = is right-associative, that is, an expression of the form
is evaluated as
The following example demonstrates the usage of the assignment operator with a local variable, a property, and an indexer element as its left-hand operand:
The left-hand operand of an assignment receives the value of the right-hand operand. When the operands are of value types , assignment copies the contents of the right-hand operand. When the operands are of reference types , assignment copies the reference to the object.
This is called value assignment : the value is assigned.
ref assignment
Ref assignment = ref makes its left-hand operand an alias to the right-hand operand, as the following example demonstrates:
In the preceding example, the local reference variable arrayElement is initialized as an alias to the first array element. Then, it's ref reassigned to refer to the last array element. As it's an alias, when you update its value with an ordinary assignment operator = , the corresponding array element is also updated.
The left-hand operand of ref assignment can be a local reference variable , a ref field , and a ref , out , or in method parameter. Both operands must be of the same type.
Compound assignment
For a binary operator op , a compound assignment expression of the form
is equivalent to
except that x is only evaluated once.
Compound assignment is supported by arithmetic , Boolean logical , and bitwise logical and shift operators.
Null-coalescing assignment
You can use the null-coalescing assignment operator ??= to assign the value of its right-hand operand to its left-hand operand only if the left-hand operand evaluates to null . For more information, see the ?? and ??= operators article.
Operator overloadability
A user-defined type can't overload the assignment operator. However, a user-defined type can define an implicit conversion to another type. That way, the value of a user-defined type can be assigned to a variable, a property, or an indexer element of another type. For more information, see User-defined conversion operators .
A user-defined type can't explicitly overload a compound assignment operator. However, if a user-defined type overloads a binary operator op , the op= operator, if it exists, is also implicitly overloaded.
C# language specification
For more information, see the Assignment operators section of the C# language specification .
- C# operators and expressions
- ref keyword
- Use compound assignment (style rules IDE0054 and IDE0074)
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
Next: Execution Control Expressions , Previous: Arithmetic , Up: Top [ Contents ][ Index ]
7 Assignment Expressions
As a general concept in programming, an assignment is a construct that stores a new value into a place where values can be stored—for instance, in a variable. Such places are called lvalues (see Lvalues ) because they are locations that hold a value.
An assignment in C is an expression because it has a value; we call it an assignment expression . A simple assignment looks like
We say it assigns the value of the expression value-to-store to the location lvalue , or that it stores value-to-store there. You can think of the “l” in “lvalue” as standing for “left,” since that’s what you put on the left side of the assignment operator.
However, that’s not the only way to use an lvalue, and not all lvalues can be assigned to. To use the lvalue in the left side of an assignment, it has to be modifiable . In C, that means it was not declared with the type qualifier const (see const ).
The value of the assignment expression is that of lvalue after the new value is stored in it. This means you can use an assignment inside other expressions. Assignment operators are right-associative so that
is equivalent to
This is the only useful way for them to associate; the other way,
would be invalid since an assignment expression such as x = y is not valid as an lvalue.
Warning: Write parentheses around an assignment if you nest it inside another expression, unless that is a conditional expression, or comma-separated series, or another assignment.
• | The basics of storing a value. | |
• | Expressions into which a value can be stored. | |
• | Shorthand for changing an lvalue’s contents. | |
• | Shorthand for incrementing and decrementing an lvalue’s contents. | |
• | Accessing then incrementing or decrementing. | |
• | How to avoid ambiguity. | |
• | Write assignments as separate statements. |
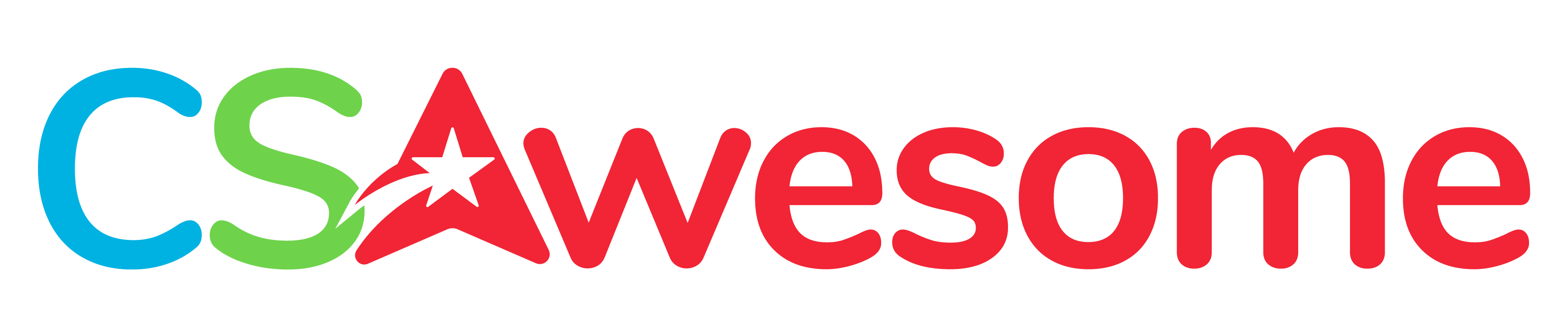
- Table of Contents
- Course Home
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- 1.1 Getting Started
- 1.1.1 Preface
- 1.1.2 About the AP CSA Exam
- 1.1.3 Transitioning from AP CSP to AP CSA
- 1.1.4 Java Development Environments
- 1.1.5 Growth Mindset and Pair Programming
- 1.1.6 Pretest for the AP CSA Exam
- 1.1.7 Survey
- 1.2 Why Programming? Why Java?
- 1.3 Variables and Data Types
- 1.4 Expressions and Assignment Statements
- 1.5 Compound Assignment Operators
- 1.6 Casting and Ranges of Values
- 1.7 Unit 1 Summary
- 1.8 Mixed Up Code Practice
- 1.9 Toggle Mixed Up or Write Code Practice
- 1.10 Coding Practice
- 1.11 Multiple Choice Exercises
- 1.3. Variables and Data Types" data-toggle="tooltip">
- 1.5. Compound Assignment Operators' data-toggle="tooltip" >
Time estimate: 90 min.
1.4. Expressions and Assignment Statements ¶
In this lesson, you will learn about assignment statements and expressions that contain math operators and variables.
1.4.1. Assignment Statements ¶
Assignment statements initialize or change the value stored in a variable using the assignment operator = . An assignment statement always has a single variable on the left hand side. The value of the expression (which can contain math operators and other variables) on the right of the = sign is stored in the variable on the left.
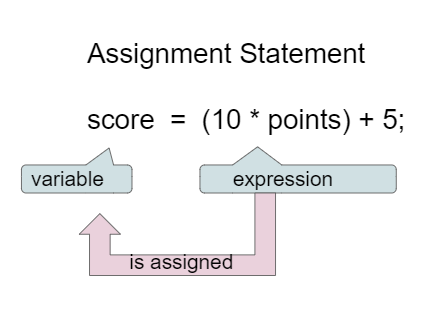
Figure 1: Assignment Statement (variable = expression;) ¶
Instead of saying equals for the = in an assignment statement, say “gets” or “is assigned” to remember that the variable gets or is assigned the value on the right. In the figure above score is assigned the value of the expression 10 times points (which is another variable) plus 5.
The following video by Dr. Colleen Lewis shows how variables can change values in memory using assignment statements.
As we saw in the video, we can set one variable’s value to a copy of the value of another variable like y = x; . This won’t change the value of the variable that you are copying from.
Let’s step through the following code in the Java visualizer to see the values in memory. Click on the Next button at the bottom of the code to see how the values of the variables change. You can run the visualizer on any Active Code in this e-book by just clicking on the Code Lens button at the top of each Active Code.
Activity: CodeLens 1.4.1.2 (asgn_viz1)

1-4-3: What are the values of x, y, and z after the following code executes? You can step through this code by clicking on this Java visualizer link.
- x = 0, y = 1, z = 2
- These are the initial values in the variable, but the values are changed.
- x = 1, y = 2, z = 3
- x changes to y's initial value, y's value is doubled, and z is set to 3
- x = 2, y = 2, z = 3
- Remember that the equal sign doesn't mean that the two sides are equal. It sets the value for the variable on the left to the value from evaluating the right side.
- x = 0, y = 0, z = 3
The following has the correct code to ‘swap’ the values in x and y (so that x ends up with y’s initial value and y ends up with x’s initial value), but the code is mixed up and contains one extra block which is not needed in a correct solution. Drag the needed blocks from the left into the correct order on the right. Check your solution by clicking on the Check button. You will be told if any of the blocks are in the wrong order or if you need to remove one or more blocks. After three incorrect attempts you will be able to use the Help Me button to make the problem easier.
1.4.2. Adding 1 to a Variable ¶
If you use a variable to keep score, you would probably increment it (add one to the current value) whenever score should go up. You can do this by setting the variable to the current value of the variable plus one ( score = score + 1 ) as shown below. The formula would look strange in math class, but it makes sense in coding because it is assigning a new value to the variable on the left that comes from evaluating the arithmetic expression on the right. So, the score variable is set to the previous value of score plus 1.
Try the code below to see how score is incremented by 1. Try substituting 2 instead of 1 to see what happens.
1.4.3. Input with Variables ¶
Variables are a powerful abstraction in programming because the same algorithm can be used with different input values saved in variables. The code below ( Java Scanner Input Repl using the Scanner class or Java Console Input Repl using the Console class) will say hello to anyone who types in their name for different name values. Click on run and then type in your name. Then, try run again and type in a friend’s name. The code works for any name: behold, the power of variables!
Although you will not be tested in the AP CSA exam on using the Java input or the Scanner or Console classes, learning how to do input in Java is very useful and fun. For more information on using the Scanner class, go to https://www.w3schools.com/java/java_user_input.asp , and for the newer Console class, https://howtodoinjava.com/java-examples/console-input-output/ .
1.4.4. Operators ¶
Java uses the standard mathematical operators for addition ( + ), subtraction ( - ), and division ( / ). The multiplication operator is written as * , as it is in most programming languages, since the character sets used until relatively recently didn’t have a character for a real multiplication sign, × , and keyboards still don’t have a key for it. Likewise no ÷ .
You may be used to using ^ for exponentiation, either from a graphing calculator or tools like Desmos. Confusingly ^ is an operator in Java, but it has a completely different meaning than exponentiation and isn’t even exactly an arithmetic operator. You will learn how to use the Math.pow method to do exponents in Unit 2.
Arithmetic expressions can be of type int or double . An arithmetic expression consisting only of int values will evaluate to an int value. An arithmetic expression that uses at least one double value will evaluate to a double value. (You may have noticed that + was also used to combine String and other values into new String s. More on this when we talk about String s more fully in Unit 2.)
Java uses the operator == to test if the value on the left is equal to the value on the right and != to test if two items are not equal. Don’t get one equal sign = confused with two equal signs == . They mean very different things in Java. One equal sign is used to assign a value to a variable. Two equal signs are used to test a variable to see if it is a certain value and that returns true or false as you’ll see below. Also note that using == and != with double values can produce surprising results. Because double values are only an approximation of the real numbers even things that should be mathematically equivalent might not be represented by the exactly same double value and thus will not be == . To see this for yourself, write a line of code below to print the value of the expression 0.3 == 0.1 + 0.2 ; it will be false !

Run the code below to see all the operators in action. Do all of those operators do what you expected? What about 2 / 3? Isn’t it surprising that it prints 0? See the note below.
When Java sees you doing integer division (or any operation with integers) it assumes you want an integer result so it throws away anything after the decimal point in the answer. This is called truncating division . If you need a double answer, you should make at least one of the values in the expression a double like 2.0.
With division, another thing to watch out for is dividing by 0. An attempt to divide an integer by zero will result in an ArithmeticException error message. Try it in one of the active code windows above.
Operators can be used to create compound expressions with more than one operator. You can either use a literal value which is a fixed value like 2, or variables in them. When compound expressions are evaluated, operator precedence rules are used, just like when we do math (remember PEMDAS?), so that * , / , and % are done before + and - . However, anything in parentheses is done first. It doesn’t hurt to put in extra parentheses if you are unsure as to what will be done first or just to make it more clear.
In the example below, try to guess what it will print out and then run it to see if you are right. Remember to consider operator precedence . How do the parentheses change the precedence?
1.4.5. The Remainder Operator ¶
The operator % in Java is the remainder operator. Like the other arithmetic operators is takes two operands. Mathematically it returns the remainder after dividing the first number by the second, using truncating integer division. For instance, 5 % 2 evaluates to 1 since 2 goes into 5 two times with a remainder of 1.
While you may not have heard of remainder as an operator, think back to elementary school math. Remember when you first learned long division, before they taught you about decimals, how when you did a long division that didn’t divide evenly, you gave the answer as the number of even divisions and the remainder. That remainder is what is returned by this operator. In the figures below, the remainders are the same values that would be returned by 2 % 3 and 5 % 2 .
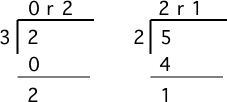
Figure 1: Long division showing the integer result and the remainder ¶
Sometimes people—including Professor Lewis in the next video—will call % the modulo , or mod , operator. That is not actually correct though the difference between remainder and modulo, which uses Euclidean division instead of truncating integer division, only matters when negative operands are involved and the signs of the operands differ. With positive operands, remainder and mod give the same results. Java does have a method Math.floorMod in the Math class if you need to use modulo instead of remainder, but % is all you need in the AP exam.
Here’s the video .
In the example below, try to guess what it will print out and then run it to see if you are right.
The result of x % y when x is smaller than y is always x. The value y can’t go into x at all (goes in 0 times), since x is smaller than y, so the result is just x. So if you see 2 % 3 the result is 2.
1-4-10: What is the result of 158 % 10?
- This would be the result of 158 divided by 10. % gives you the remainder.
- % gives you the remainder after the division.
- When you divide 158 by 10 you get a remainder of 8.
1-4-11: What is the result of 3 % 8?
- 8 goes into 3 no times so the remainder is 3. The remainder of a smaller number divided by a larger number is always the smaller number!
- This would be the remainder if the question was 8 % 3 but here we are asking for the reminder after we divide 3 by 8.
- What is the remainder after you divide 3 by 8?
1.4.6. Programming Challenge : Dog Years ¶
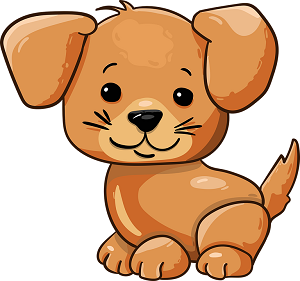
In this programming challenge, you will calculate your age, and your pet’s age from your birthdates, and your pet’s age in dog years. In the code below, type in the current year, the year you were born, the year your dog or cat was born (if you don’t have one, make one up!) in the variables below. Then write formulas in assignment statements to calculate how old you are, how old your dog or cat is, and how old they are in dog years which is 7 times a human year. Finally, print it all out. If you are pair programming, switch drivers (who has control of the keyboard in pair programming) after every line of code.
Calculate your age and your pet’s age from the birthdates, and then your pet’s age in dog years.
Your teacher may suggest that you use a Java IDE like replit.com for this challenge so that you can use input to get these values using the Scanner class . Here is a repl template that you can use to get started if you want to try the challenge with input.
1.4.7. Summary ¶
Arithmetic expressions include expressions of type int and double .
The arithmetic operators consist of + , - , * , / , and % also known as addition, subtraction, multiplication, division, and remainder.
An arithmetic operation that uses two int values will evaluate to an int value. With integer division, any decimal part in the result will be thrown away.
An arithmetic operation that uses at least one double value will evaluate to a double value.
Operators can be used to construct compound expressions.
During evaluation, operands are associated with operators according to operator precedence to determine how they are grouped. ( * , / , % have precedence over + and - , unless parentheses are used to group those.)
An attempt to divide an integer by zero will result in an ArithmeticException .
The assignment operator ( = ) allows a program to initialize or change the value stored in a variable. The value of the expression on the right is stored in the variable on the left.
During execution, expressions are evaluated to produce a single value.
The value of an expression has a type based on the types of the values and operators used in the expression.
1.4.8. AP Practice ¶
The following is a 2019 AP CSA sample question.
1-4-13: Consider the following code segment.
What is printed when the code segment is executed?
- 0.666666666666667
- Don't forget that division and multiplication will be done first due to operator precedence.
- Yes, this is equivalent to (5 + ((a/b)*c) - 1).
- Don't forget that division and multiplication will be done first due to operator precedence, and that an int/int gives an int truncated result where everything to the right of the decimal point is dropped.

1.7 Java | Assignment Statements & Expressions
An assignment statement designates a value for a variable. An assignment statement can be used as an expression in Java.
After a variable is declared, you can assign a value to it by using an assignment statement . In Java, the equal sign = is used as the assignment operator . The syntax for assignment statements is as follows:
An expression represents a computation involving values, variables, and operators that, when taking them together, evaluates to a value. For example, consider the following code:
You can use a variable in an expression. A variable can also be used on both sides of the = operator. For example:
In the above assignment statement, the result of x + 1 is assigned to the variable x . Let’s say that x is 1 before the statement is executed, and so becomes 2 after the statement execution.
To assign a value to a variable, you must place the variable name to the left of the assignment operator. Thus the following statement is wrong:
Note that the math equation x = 2 * x + 1 ≠ the Java expression x = 2 * x + 1
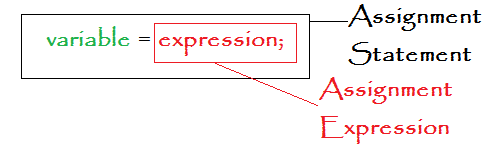
Which is equivalent to:
And this statement
is equivalent to:
Note: The data type of a variable on the left must be compatible with the data type of a value on the right. For example, int x = 1.0 would be illegal, because the data type of x is int (integer) and does not accept the double value 1.0 without Type Casting .
◄◄◄BACK | NEXT►►►
What's Your Opinion? Cancel reply
Enhance your Brain
Subscribe to Receive Free Bio Hacking, Nootropic, and Health Information
HTML for Simple Website Customization My Personal Web Customization Personal Insights
DISCLAIMER | Sitemap | ◘
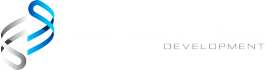
HTML for Simple Website Customization My Personal Web Customization Personal Insights SEO Checklist Publishing Checklist My Tools
Top Posts & Pages

- Skip to main content
- Select language
- Skip to search
- Expressions and operators
- Operator precedence
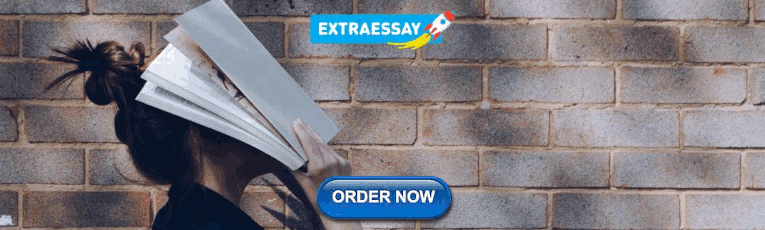
Left-hand-side expressions
« Previous Next »
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more.
A complete and detailed list of operators and expressions is also available in the reference .
JavaScript has the following types of operators. This section describes the operators and contains information about operator precedence.
- Assignment operators
- Comparison operators
- Arithmetic operators
- Bitwise operators
Logical operators
String operators, conditional (ternary) operator.
- Comma operator
Unary operators
- Relational operator
JavaScript has both binary and unary operators, and one special ternary operator, the conditional operator. A binary operator requires two operands, one before the operator and one after the operator:
For example, 3+4 or x*y .
A unary operator requires a single operand, either before or after the operator:
For example, x++ or ++x .
An assignment operator assigns a value to its left operand based on the value of its right operand. The simple assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x .
There are also compound assignment operators that are shorthand for the operations listed in the following table:
Name | Shorthand operator | Meaning |
---|---|---|
Destructuring
For more complex assignments, the destructuring assignment syntax is a JavaScript expression that makes it possible to extract data from arrays or objects using a syntax that mirrors the construction of array and object literals.
A comparison operator compares its operands and returns a logical value based on whether the comparison is true. The operands can be numerical, string, logical, or object values. Strings are compared based on standard lexicographical ordering, using Unicode values. In most cases, if the two operands are not of the same type, JavaScript attempts to convert them to an appropriate type for the comparison. This behavior generally results in comparing the operands numerically. The sole exceptions to type conversion within comparisons involve the === and !== operators, which perform strict equality and inequality comparisons. These operators do not attempt to convert the operands to compatible types before checking equality. The following table describes the comparison operators in terms of this sample code:
Operator | Description | Examples returning true |
---|---|---|
( ) | Returns if the operands are equal. |
|
( ) | Returns if the operands are not equal. | |
( ) | Returns if the operands are equal and of the same type. See also and . | |
( ) | Returns if the operands are of the same type but not equal, or are of different type. | |
( ) | Returns if the left operand is greater than the right operand. | |
( ) | Returns if the left operand is greater than or equal to the right operand. | |
( ) | Returns if the left operand is less than the right operand. | |
( ) | Returns if the left operand is less than or equal to the right operand. |
Note: ( => ) is not an operator, but the notation for Arrow functions .
An arithmetic operator takes numerical values (either literals or variables) as their operands and returns a single numerical value. The standard arithmetic operators are addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ). These operators work as they do in most other programming languages when used with floating point numbers (in particular, note that division by zero produces Infinity ). For example:
In addition to the standard arithmetic operations (+, -, * /), JavaScript provides the arithmetic operators listed in the following table:
Operator | Description | Example |
---|---|---|
( ) | Binary operator. Returns the integer remainder of dividing the two operands. | 12 % 5 returns 2. |
( ) | Unary operator. Adds one to its operand. If used as a prefix operator ( ), returns the value of its operand after adding one; if used as a postfix operator ( ), returns the value of its operand before adding one. | If is 3, then sets to 4 and returns 4, whereas returns 3 and, only then, sets to 4. |
( ) | Unary operator. Subtracts one from its operand. The return value is analogous to that for the increment operator. | If is 3, then sets to 2 and returns 2, whereas returns 3 and, only then, sets to 2. |
( ) | Unary operator. Returns the negation of its operand. | If is 3, then returns -3. |
( ) | Unary operator. Attempts to convert the operand to a number, if it is not already. | returns . returns |
( ) | Calculates the to the power, that is, | returns . returns . |
A bitwise operator treats their operands as a set of 32 bits (zeros and ones), rather than as decimal, hexadecimal, or octal numbers. For example, the decimal number nine has a binary representation of 1001. Bitwise operators perform their operations on such binary representations, but they return standard JavaScript numerical values.
The following table summarizes JavaScript's bitwise operators.
Operator | Usage | Description |
---|---|---|
Returns a one in each bit position for which the corresponding bits of both operands are ones. | ||
Returns a zero in each bit position for which the corresponding bits of both operands are zeros. | ||
Returns a zero in each bit position for which the corresponding bits are the same. [Returns a one in each bit position for which the corresponding bits are different.] | ||
Inverts the bits of its operand. | ||
Shifts in binary representation bits to the left, shifting in zeros from the right. | ||
Shifts in binary representation bits to the right, discarding bits shifted off. | ||
Shifts in binary representation bits to the right, discarding bits shifted off, and shifting in zeros from the left. |
Bitwise logical operators
Conceptually, the bitwise logical operators work as follows:
- The operands are converted to thirty-two-bit integers and expressed by a series of bits (zeros and ones). Numbers with more than 32 bits get their most significant bits discarded. For example, the following integer with more than 32 bits will be converted to a 32 bit integer: Before: 11100110111110100000000000000110000000000001 After: 10100000000000000110000000000001
- Each bit in the first operand is paired with the corresponding bit in the second operand: first bit to first bit, second bit to second bit, and so on.
- The operator is applied to each pair of bits, and the result is constructed bitwise.
For example, the binary representation of nine is 1001, and the binary representation of fifteen is 1111. So, when the bitwise operators are applied to these values, the results are as follows:
Expression | Result | Binary Description |
---|---|---|
Note that all 32 bits are inverted using the Bitwise NOT operator, and that values with the most significant (left-most) bit set to 1 represent negative numbers (two's-complement representation).
Bitwise shift operators
The bitwise shift operators take two operands: the first is a quantity to be shifted, and the second specifies the number of bit positions by which the first operand is to be shifted. The direction of the shift operation is controlled by the operator used.
Shift operators convert their operands to thirty-two-bit integers and return a result of the same type as the left operand.
The shift operators are listed in the following table.
Operator | Description | Example |
---|---|---|
( ) | This operator shifts the first operand the specified number of bits to the left. Excess bits shifted off to the left are discarded. Zero bits are shifted in from the right. | yields 36, because 1001 shifted 2 bits to the left becomes 100100, which is 36. |
( ) | This operator shifts the first operand the specified number of bits to the right. Excess bits shifted off to the right are discarded. Copies of the leftmost bit are shifted in from the left. | yields 2, because 1001 shifted 2 bits to the right becomes 10, which is 2. Likewise, yields -3, because the sign is preserved. |
( ) | This operator shifts the first operand the specified number of bits to the right. Excess bits shifted off to the right are discarded. Zero bits are shifted in from the left. | yields 4, because 10011 shifted 2 bits to the right becomes 100, which is 4. For non-negative numbers, zero-fill right shift and sign-propagating right shift yield the same result. |
Logical operators are typically used with Boolean (logical) values; when they are, they return a Boolean value. However, the && and || operators actually return the value of one of the specified operands, so if these operators are used with non-Boolean values, they may return a non-Boolean value. The logical operators are described in the following table.
Operator | Usage | Description |
---|---|---|
( ) | Returns if it can be converted to ; otherwise, returns . Thus, when used with Boolean values, returns if both operands are true; otherwise, returns . | |
( ) | Returns if it can be converted to ; otherwise, returns . Thus, when used with Boolean values, returns if either operand is true; if both are false, returns . | |
( ) | Returns if its single operand can be converted to ; otherwise, returns . |
Examples of expressions that can be converted to false are those that evaluate to null, 0, NaN, the empty string (""), or undefined.
The following code shows examples of the && (logical AND) operator.
The following code shows examples of the || (logical OR) operator.
The following code shows examples of the ! (logical NOT) operator.
Short-circuit evaluation
As logical expressions are evaluated left to right, they are tested for possible "short-circuit" evaluation using the following rules:
- false && anything is short-circuit evaluated to false.
- true || anything is short-circuit evaluated to true.
The rules of logic guarantee that these evaluations are always correct. Note that the anything part of the above expressions is not evaluated, so any side effects of doing so do not take effect.
In addition to the comparison operators, which can be used on string values, the concatenation operator (+) concatenates two string values together, returning another string that is the union of the two operand strings.
For example,
The shorthand assignment operator += can also be used to concatenate strings.
The conditional operator is the only JavaScript operator that takes three operands. The operator can have one of two values based on a condition. The syntax is:
If condition is true, the operator has the value of val1 . Otherwise it has the value of val2 . You can use the conditional operator anywhere you would use a standard operator.
This statement assigns the value "adult" to the variable status if age is eighteen or more. Otherwise, it assigns the value "minor" to status .
The comma operator ( , ) simply evaluates both of its operands and returns the value of the last operand. This operator is primarily used inside a for loop, to allow multiple variables to be updated each time through the loop.
For example, if a is a 2-dimensional array with 10 elements on a side, the following code uses the comma operator to update two variables at once. The code prints the values of the diagonal elements in the array:
A unary operation is an operation with only one operand.
The delete operator deletes an object, an object's property, or an element at a specified index in an array. The syntax is:
where objectName is the name of an object, property is an existing property, and index is an integer representing the location of an element in an array.
The fourth form is legal only within a with statement, to delete a property from an object.
You can use the delete operator to delete variables declared implicitly but not those declared with the var statement.
If the delete operator succeeds, it sets the property or element to undefined . The delete operator returns true if the operation is possible; it returns false if the operation is not possible.
Deleting array elements
When you delete an array element, the array length is not affected. For example, if you delete a[3] , a[4] is still a[4] and a[3] is undefined.
When the delete operator removes an array element, that element is no longer in the array. In the following example, trees[3] is removed with delete . However, trees[3] is still addressable and returns undefined .
If you want an array element to exist but have an undefined value, use the undefined keyword instead of the delete operator. In the following example, trees[3] is assigned the value undefined , but the array element still exists:
The typeof operator is used in either of the following ways:
The typeof operator returns a string indicating the type of the unevaluated operand. operand is the string, variable, keyword, or object for which the type is to be returned. The parentheses are optional.
Suppose you define the following variables:
The typeof operator returns the following results for these variables:
For the keywords true and null , the typeof operator returns the following results:
For a number or string, the typeof operator returns the following results:
For property values, the typeof operator returns the type of value the property contains:
For methods and functions, the typeof operator returns results as follows:
For predefined objects, the typeof operator returns results as follows:
The void operator is used in either of the following ways:
The void operator specifies an expression to be evaluated without returning a value. expression is a JavaScript expression to evaluate. The parentheses surrounding the expression are optional, but it is good style to use them.
You can use the void operator to specify an expression as a hypertext link. The expression is evaluated but is not loaded in place of the current document.
The following code creates a hypertext link that does nothing when the user clicks it. When the user clicks the link, void(0) evaluates to undefined , which has no effect in JavaScript.
The following code creates a hypertext link that submits a form when the user clicks it.
Relational operators
A relational operator compares its operands and returns a Boolean value based on whether the comparison is true.
The in operator returns true if the specified property is in the specified object. The syntax is:
where propNameOrNumber is a string or numeric expression representing a property name or array index, and objectName is the name of an object.
The following examples show some uses of the in operator.
The instanceof operator returns true if the specified object is of the specified object type. The syntax is:
where objectName is the name of the object to compare to objectType , and objectType is an object type, such as Date or Array .
Use instanceof when you need to confirm the type of an object at runtime. For example, when catching exceptions, you can branch to different exception-handling code depending on the type of exception thrown.
For example, the following code uses instanceof to determine whether theDay is a Date object. Because theDay is a Date object, the statements in the if statement execute.
The precedence of operators determines the order they are applied when evaluating an expression. You can override operator precedence by using parentheses.
The following table describes the precedence of operators, from highest to lowest.
Operator type | Individual operators |
---|---|
member | |
call / create instance | |
negation/increment | |
multiply/divide | |
addition/subtraction | |
bitwise shift | |
relational | |
equality | |
bitwise-and | |
bitwise-xor | |
bitwise-or | |
logical-and | |
logical-or | |
conditional | |
assignment | |
comma |
A more detailed version of this table, complete with links to additional details about each operator, may be found in JavaScript Reference .
- Expressions
An expression is any valid unit of code that resolves to a value.
Every syntactically valid expression resolves to some value but conceptually, there are two types of expressions: with side effects (for example: those that assign value to a variable) and those that in some sense evaluates and therefore resolves to value.
The expression x = 7 is an example of the first type. This expression uses the = operator to assign the value seven to the variable x . The expression itself evaluates to seven.
The code 3 + 4 is an example of the second expression type. This expression uses the + operator to add three and four together without assigning the result, seven, to a variable. JavaScript has the following expression categories:
- Arithmetic: evaluates to a number, for example 3.14159. (Generally uses arithmetic operators .)
- String: evaluates to a character string, for example, "Fred" or "234". (Generally uses string operators .)
- Logical: evaluates to true or false. (Often involves logical operators .)
- Primary expressions: Basic keywords and general expressions in JavaScript.
- Left-hand-side expressions: Left values are the destination of an assignment.
Primary expressions
Basic keywords and general expressions in JavaScript.
Use the this keyword to refer to the current object. In general, this refers to the calling object in a method. Use this either with the dot or the bracket notation:
Suppose a function called validate validates an object's value property, given the object and the high and low values:
You could call validate in each form element's onChange event handler, using this to pass it the form element, as in the following example:
- Grouping operator
The grouping operator ( ) controls the precedence of evaluation in expressions. For example, you can override multiplication and division first, then addition and subtraction to evaluate addition first.
Comprehensions
Comprehensions are an experimental JavaScript feature, targeted to be included in a future ECMAScript version. There are two versions of comprehensions:
Comprehensions exist in many programming languages and allow you to quickly assemble a new array based on an existing one, for example.
Left values are the destination of an assignment.
You can use the new operator to create an instance of a user-defined object type or of one of the built-in object types. Use new as follows:
The super keyword is used to call functions on an object's parent. It is useful with classes to call the parent constructor, for example.
Spread operator
The spread operator allows an expression to be expanded in places where multiple arguments (for function calls) or multiple elements (for array literals) are expected.
Example: Today if you have an array and want to create a new array with the existing one being part of it, the array literal syntax is no longer sufficient and you have to fall back to imperative code, using a combination of push , splice , concat , etc. With spread syntax this becomes much more succinct:
Similarly, the spread operator works with function calls:
Document Tags and Contributors
- l10n:priority
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Legacy generator function expression
- Logical Operators
- Object initializer
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration`X' before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
- School Guide
- Class 11 Syllabus
- Class 11 Revision Notes
- Maths Notes Class 11
- Physics Notes Class 11
- Chemistry Notes Class 11
- Biology Notes Class 11
- NCERT Solutions Class 11 Maths
- RD Sharma Solutions Class 11
- Math Formulas Class 11
Augmented Assignment Operators in Python
- Assignment Operators in Python
- Assignment Operators In C++
- Assignment Operators in Programming
- Python Arithmetic Operators
- Chaining comparison operators in Python
- Increment and Decrement Operators in Python
- JavaScript Assignment Operators
- Solidity - Assignment Operators
- Difference between == and is operator in Python
- C++ Assignment Operator Overloading
- Python: Operations on Numpy Arrays
- Operator Functions in Python | Set 2
- Operator Functions in Python | Set 1
- Python - Alternate Elements operation on Tuple
- Python Bitwise Operators
- Python NOT EQUAL operator
- Assignment Operators in C
- Java Assignment Operators with Examples
- Compound assignment operators in Java
An assignment operator is an operator that is used to assign some value to a variable. Like normally in Python, we write “ a = 5 “ to assign value 5 to variable ‘a’. Augmented assignment operators have a special role to play in Python programming. It basically combines the functioning of the arithmetic or bitwise operator with the assignment operator. So assume if we need to add 7 to a variable “a” and assign the result back to “a”, then instead of writing normally as “ a = a + 7 “, we can use the augmented assignment operator and write the expression as “ a += 7 “. Here += has combined the functionality of arithmetic addition and assignment.
So, augmented assignment operators provide a short way to perform a binary operation and assigning results back to one of the operands. The way to write an augmented operator is just to write that binary operator and assignment operator together. In Python, we have several different augmented assignment operators like +=, -=, *=, /=, //=, **=, |=, &=, >>=, <<=, %= and ^=. Let’s see their functioning with the help of some exemplar codes:
1. Addition and Assignment (+=): This operator combines the impact of arithmetic addition and assignment. Here,
a = a + b can be written as a += b
2. Subtraction and Assignment (-=): This operator combines the impact of subtraction and assignment.
a = a – b can be written as a -= b
Example:
3. Multiplication and Assignment (*=): This operator combines the functionality of multiplication and assignment.
a = a * b can be written as a *= b
4. Division and Assignment (/=): This operator has the combined functionality of division and assignment.
a = a / b can be written as a /= b
5. Floor Division and Assignment (//=): It performs the functioning of floor division and assignment.
a = a // b can be written as a //= b
6. Modulo and Assignment (%=): This operator combines the impact of the modulo operator and assignment.
a = a % b can be written as a %= b
7. Power and Assignment (**=): This operator is equivalent to the power and assignment operator together.
a = a**b can be written as a **= b
8. Bitwise AND & Assignment (&=): This operator combines the impact of the bitwise AND operator and assignment operator.
a = a & b can be written as a &= b
9. Bitwise OR and Assignment (|=): This operator combines the impact of Bitwise OR and assignment operator.
a = a | b can be written as a |= b
10. Bitwise XOR and Assignment (^=): This augmented assignment operator combines the functionality of the bitwise XOR operator and assignment operator.
a = a ^ b can be written as a ^= b
11. Bitwise Left Shift and Assignment (<<=): It puts together the functioning of the bitwise left shift operator and assignment operator.
a = a << b can be written as a <<= b
12. Bitwise Right Shift and Assignment (>>=): It puts together the functioning of the bitwise right shift operator and assignment operator.
a = a >> b can be written as a >>= b
Please Login to comment...
Similar reads.
- School Learning
- School Programming
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Stack Overflow Public questions & answers
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Talent Build your employer brand
- Advertising Reach developers & technologists worldwide
- Labs The future of collective knowledge sharing
- About the company
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
What does an assignment return?
Why does an expression i = 2 return 2? What is the rule this is based on?
printf("%d\n", i = 2 ); /* prints 2 */
I am in C domain after spending long time in Java/C#. Forgive my ignorance.
5 Answers 5
It evaluates to 2 because that's how the standard defines it. From C11 Standard , section 6.5.16:
An assignment expression has the value of the left operand after the assignment
It's to allow things like this:
(although there's some debate as to whether code like that is a good thing or not.)
Incidentally, this behaviour is replicated in Java (and I would bet that it's the same in C# too).
- 1 I generally am ok with this when a and b are really the same thing as opposed to just happening to be the same value. I use the same reasoning to decide between int presentValue, previousValue; vs. int myAge; int myHeight; . Present and previous values should never have different types while I may choose to use a float for my height instead of an int . Just another layer of intent that can be either captured. – altendky Aug 28, 2015 at 14:09
- 4 Updated link by @SurajJain to C11. What this construct also allows is the (much more useful) if ( ( ptr = func() ) != NULL ) ... – DevSolar Feb 20, 2017 at 9:01
The rule is to return the right-hand operand of = converted to the type of the variable which is assigned to.
- 1 Could you provide a reference? Oli Charlesworth says that in C99 it is the value of the left operand, so this would be a difference with C++? – Benoit Mar 1, 2012 at 10:35
- So, this is in contradiction with your answer (rvalue => lvalue, right side => left side). – Benoit Mar 1, 2012 at 10:39
- 1 There's no real contradiction. "rvalue converted to the variable type" is exactly what the variable (the lvalue) will be after the assignment. Two ways of saying the same. The "lvalue after assignment" rule also applies as-is for += etc. – ugoren Mar 1, 2012 at 11:10
- 2 IIRC there is indeed a difference here - b = 4.5 evaluates to a value 4.5 in C, but in C++ it's an lvalue referring to b . A difficult lvalue to actually use on the LHS of an assignment, since for example (a = b) = 1 is UB anyway due to the lack of a sequence point between the two modifications of a . But I think in C++ you can take the address &(a = 1) and it's the address of a , and in C you can't. Someone correct me if I'm wrong. – Steve Jessop Mar 1, 2012 at 11:18
It consider the expression firstly then print the leftmost variable.
x++ is postfix and ++x is prefix so:

- you know calculations are done from right to left. not really. Calculations can be done in any order, including assignments. Argument values are computed before the function is called, but that's about the only assumption you can make. The important thing to understand is the concept of sequence point. – chqrlie Feb 20, 2017 at 10:03
- You are right man! but I mean the simple ones like x=y+z, I edited the answer, Thank you. – Ahmed Salah Feb 20, 2017 at 10:20
- Assign the value 2 to i
- Evaluate the i variable and display it

In C (almost) all expressions have 2 things 1) a value 2) a side effect
The value of the expression
is 2 ; its side effect is "none";
is 2 ; its side effect is "changing the value in the object named i to 2";
- Fully 50% of possible C expressions are (void) followed by another expression (for certain values of "proportion of an infinite set"). Do they count has having a value, or not? ;-) – Steve Jessop Mar 1, 2012 at 11:21
- No, (void) expressions have no value. Eg: the expression free(pointer) has no value. – pmg Mar 1, 2012 at 11:34
- @pmg: free(pointer) indeed has no value, but it is not worthless, although many programmers consider it not worth the effort. – chqrlie Feb 20, 2017 at 9:59
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c or ask your own question .
- Featured on Meta
- The 2024 Developer Survey Is Live
- The return of Staging Ground to Stack Overflow
- The [tax] tag is being burninated
- Policy: Generative AI (e.g., ChatGPT) is banned
Hot Network Questions
- Movie I saw in the 80s where a substance oozed off of movie stairs leaving a wet cat behind
- Am I seeing double? What kind of helicopter is this, and how many blades does it actually have?
- What terminal did David connect to his IMSAI 8080?
- Mistake in Proof "Every unique factorization domain is a principal ideal domain"
- Should the increasing energy retained in the atmosphere be considered in relation to global warming?
- How to refer to a library in an interface?
- What is the point of triggering of a national snap election immediately after losing the EU elections?
- How does the Cube of Force interact with teleportation?
- What percentage of light gets scattered by a mirror?
- A trigonometric equation: how hard could it be?
- How do I snap the edges of hex tiles together?
- Does the Gunner feat let you ignore the Reload property?
- Application of Lie group analysis of PDE (beyond calculation of exact solutions)
- Why does mars have a jagged light curve
- Advice on DIY Adjusting Rheem Water Heater Thermostat
- How to Adjust Comparator Output Voltage for Circuit to Work at 3.3V Instead of 5V?
- How often does systemd journal collect/read logs from sources
- Have I ruined my AC by running it with the outside cover on?
- I'm looking for a series where there was a civilization in the Mediterranean basin, which got destroyed by the Atlantic breaking in
- Why "Power & battery" stuck at spinning circle for 4 hours?
- the angular orbital velocity of satellite in circle orbit is constant?
- Tying shoes on Shabbat if you don’t plan to untie them in a day
- Has ever a country by its own volition refused to join United Nations, or those which havent joined it's because they aren't recognized as such by UN?
- Best way to halve 12V battery voltage for 6V device, while still being able to measure the battery level?
Welcome to Microsoft Forms!
- Create and share online surveys, quizzes, polls, and forms.
- Collect feedback, measure satisfaction, test knowledge, and more.
- Easily design your forms with various question types, themes, and branching logic.
- Analyze your results with built-in charts and reports, or export them to Excel for further analysis.
- Integrate Microsoft Forms with other Microsoft 365 apps, such as Teams, SharePoint, and OneDrive, so you can collaborate with others and access your forms from anywhere.
Explore templates
- Template gallery
- Community volunteer registration form
- Employee satisfaction survey
- Competitive analysis study
- Office facility request form
- Vacation and sick leave form
- Post-event feedback survey
- Holiday Party Invitation

An official website of the United States government
Here's how you know
Official websites use .gov A .gov website belongs to an official government organization in the United States.
Secure .gov websites use HTTPS A lock ( Lock Locked padlock ) or https:// means you’ve safely connected to the .gov website. Share sensitive information only on official, secure websites.

Suspended Counterparty Program
FHFA established the Suspended Counterparty Program to help address the risk to Fannie Mae, Freddie Mac, and the Federal Home Loan Banks (“the regulated entities”) presented by individuals and entities with a history of fraud or other financial misconduct. Under this program, FHFA may issue orders suspending an individual or entity from doing business with the regulated entities.
FHFA maintains a list at this page of each person that is currently suspended under the Suspended Counterparty Program.
Suspension Order | |||||
---|---|---|---|---|---|
YiHou Han | San Francisco | California | 03/26/2024 | Indefinite | |
Alex A. Dadourian | Granada Hills | California | 02/08/2024 | Indefinite | |
Tamara Dadyan | Encino | California | 01/10/2024 | Indefinite | |
Richard Ayvazyan | Encino | California | 01/10/2024 | Indefinite | |
Michael C. Jackson | Star | Idaho | 01/10/2024 | Indefinite |
This page was last updated on 03/26/2024
CSCI 111 TEST 3 JESSE

Students also viewed

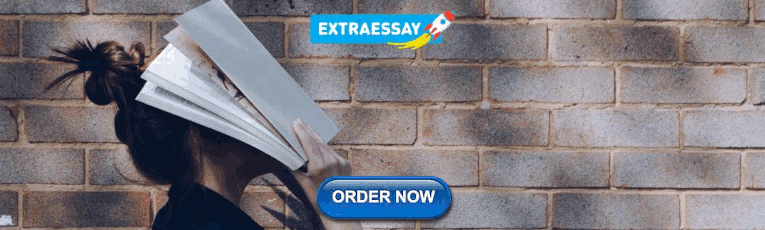
IMAGES
VIDEO
COMMENTS
In this lesson, you'll learn about the biggest change in Python 3.8: the introduction of assignment expressions.Assignment expression are written with a new notation (:=).This operator is often called the walrus operator as it resembles the eyes and tusks of a walrus on its side.. Assignment expressions allow you to assign and return a value in the same expression.
System.out.println ("total cost: " + (unitCost * items) ); } } total cost: 40. Which of the following shows the syntax of an assignment statement? variableName = expression; What are the two steps that take place when an assignment statement is executed? (i) Evaluate the Expression, and (ii) Store the value in the variable.
An assignment expression does not introduce a new scope. In most cases the scope in which the target will be bound is self-explanatory: it is the current scope. ... The following changes have been made based on implementation experience and additional review after the PEP was first accepted and before Python 3.8 was released: for consistency ...
In this article. The assignment operator = assigns the value of its right-hand operand to a variable, a property, or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or ...
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Python 3.8, released in October 2019, adds assignment expressions to Python via the := syntax. The assignment expression syntax is also sometimes called "the walrus operator" because := vaguely resembles a walrus with tusks. Assignment expressions allow variable assignments to occur inside of larger expressions.
In this lesson, you will learn about assignment statements and expressions that contain math operators and variables. 1.4.1. Assignment Statements ¶. Remember that a variable holds a value that can change or vary. Assignment statements initialize or change the value stored in a variable using the assignment operator =.
Assignment (=) The assignment ( =) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
An assignment in C is an expression because it has a value; we call it an assignment expression. A simple assignment looks like. lvalue = value-to-store. We say it assigns the value of the expression value-to-store to the location lvalue, or that it stores value-to-store there. You can think of the "l" in "lvalue" as standing for ...
The value of the expression on the right is stored in the variable on the left. During execution, expressions are evaluated to produce a single value. The value of an expression has a type based on the types of the values and operators used in the expression. 1.4.8. AP Practice¶ The following is a 2019 AP CSA sample question.
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. At a high level, an expression is a valid unit of code that resolves to a value. There are two types of expressions: those that have side effects (such as assigning values) and those that ...
Question: An assignment expression does which of the following?-assigns a Boolean expression to a variable-assigns a value to a variable and evaluates a Boolean expression-gets user input from the console, assigns the value to a variable, and evaluates a Boolean expression-gets user input from the console and assigns the value to a variable
In Java, an assignment statement is an expression that evaluates a value, which is assigned to the variable on the left side of the assignment operator. Whereas an assignment expression is the same, except it does not take into account the variable. That's why the following statements are legal: System.out.println(x = 1); Which is equivalent to:
The parentheses surrounding the expression are optional, but it is good style to use them. You can use the void operator to specify an expression as a hypertext link. The expression is evaluated but is not loaded in place of the current document. The following code creates a hypertext link that does nothing when the user clicks it.
We use Python assignment statements to assign objects to names. The target of an assignment statement is written on the left side of the equal sign (=), and the object on the right can be an arbitrary expression that computes an object. There are some important properties of assignment in Python :- Assignment creates object references instead of co
1. This is an infinite loop. It first assign 10 to c, then compare it with c > 0, then again loop starts, assign 10 to c, compare it with c>0 and so on. Loop never ends. This is equivalent to the following: while(c=10); /* Because c assign a garbage value, but not true for all cases maybe it assign 0 */. while(c);
An assignment expression has the value of the left operand after the assignment. It's to allow things like this: a = b = c; (although there's some debate as to whether code like that is a good thing or not.) Incidentally, this behaviour is replicated in Java (and I would bet that it's the same in C# too). edited Feb 20, 2017 at 8:59.
The expression x = a + c - d is a compound assignment. True. If a variable, num, contains the value 5, the value of the expression num-- is 4. false. The value of the modulus binary expression is a remainder. true. In C, if a single variable is modified more than once in an expression, the result is undefined. true.
Study with Quizlet and memorize flashcards containing terms like The ____ section of a PL/SQL block contains code that creates variables, cursors, and types. a. DECLARE b. EXCEPTION c. END d. BEGIN, ____ are used to change the values of variables. a. Blocks b. Exceptions c. Assignment statements d. Loops, ____ are named memory areas that hold values to allow the retrieval and manipulation of ...
Step 1. Assume both b and c are assigned integer values. Write an if statement that does the following: - Uses an assignment expression (with the walrus operator) to assign the value of b to a. - If the value returned from the assignment expression is greater than c, Assume the variable age has been assigned an integer value, and the variable ...
A unary operation is an operation with only one operand. delete. The delete operator deletes a property from an object.. void. The void operator evaluates an expression and discards its return value.. typeof. The typeof operator determines the type of a given object.. The unary plus operator converts its operand to Number type.
10. Which of the following statements is not true about if statements? -The statements within the braces of a clause have block scope. -An if statement can have an unlimited number of else if clauses. -Every if statement must include an else clause. -You can nest if statements within the if, else if, or else clauses of other if statements.
Microsoft Forms is a web-based application that allows you to: Create and share online surveys, quizzes, polls, and forms. Collect feedback, measure satisfaction, test knowledge, and more.
We do not own the rights to this music*
The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (?), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy. This operator is frequently used as an alternative to an if...else statement.
General Hospital spoilers for next week's episodes of the hit ABC soap tease that the hits keep on coming for the people of Port Charles. May sweeps are long gone, and July sweeps are just ...
Study with Quizlet and memorize flashcards containing terms like Which of the following is not a primary design issue for arithmetic expressions? a. Should array elements be allowed to be operands? b. Does the language allow user-defined operator overloading? c. What is the order of operand evaluation? d. What are the operator associativity rules?, The semantics of an arithmetic expression are ...
FHFA established the Suspended Counterparty Program to help address the risk to Fannie Mae, Freddie Mac, and the Federal Home Loan Banks ("the regulated entities") presented by individuals and entities with a history of fraud or other financial misconduct.
Terms in this set (102) Q7. Which of the loops below produces the same number of loop iterations as the following loop? (count is of type int.) True or False? Every assignment expression has a value and a side effect (object) stored on the left hand side of the expression. True or False? (), ++, and - represent three operators that have the ...