How to Get Unstuck When You Hit a Programming Wall

Getting stuck is part of being a programmer, no matter the level. The so-called “easy” problem is actually pretty hard. You’re not exactly sure how to move forward. What you thought would work doesn’t.
The other part of being a programmer is getting yourself unstuck.
I’ve been getting stuck a lot recently, so finding ways to get unstuck has been ever-present on my mind. Here are a few tactics I’ve been using. Maybe they can help you, too.
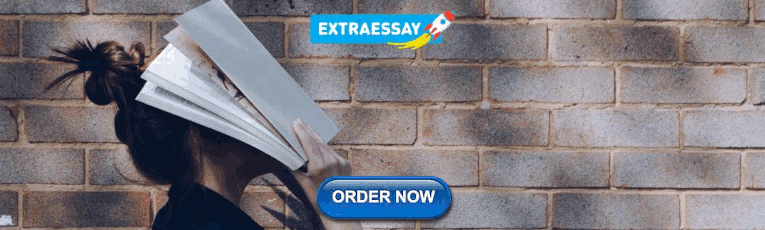
Make the problem concrete
Create a diagram, make a quick sketch, or use actual objects to give yourself a visual. It’ll make the problem a lot easier to think about.
One problem I faced asked me to find the absolute difference between the sums of a square matrix’s diagonals. That’s a mouthful and a lot to keep in my head. So I drew it out: I created a square matrix of numbers and circled the diagonals.

A simple sketch literally made the steps jump out at me: sum one diagonal (which is 15), then the other (which is 17), and then find the absolute difference between them (which is 2).
This approach applies to other problems, too. When I was learning about for loops, I iterated through a pile of almonds. When I’m working on a recursive problem, I’ll make a diagram to see what’s happening on the call stack until I hit my base case.
The commonality is this: make the abstract concrete.
Write out exactly what you’re trying to do
Write down the specific step you’re working on when you feel the all-too-familiar “spinning your wheels” cycle come upon you.
The five or ten seconds it takes to jot down a few words on a piece of paper will help you solidify your thought process and redirect your attention.
Here are some examples:
- Store the course names as keys in the object
- Pass the argument to the callback function
- Reset the “maxValue” variable to 0
Resetting the “maxValue” variable, for example, didn’t solve the problem. But it was an important step in the process. Writing this short phrase got me back on track: it was a reminder of what I’d set out to do. It also ensured I was focused on one thing, not many.
So the next time you find yourself trying the same approach over and over again and getting the same result, stop yourself and ask: “What exactly am I trying to do here?”
Then, write—yes, write—your answer down on a piece of paper.
It’s not enough to think of your response. If I casually “think” to myself, I’ll rush the process and not much (if anything) is gained. I’ve got to write it down.
Simplify your given input
It’s far less intimidating to work with a few things than many. That's why it's helpful to simplify your given input.
One problem gave me a list of three dictionaries. It was only three dictionaries, but that was still two too many.
My job was to sort each dictionary by last name, then by first name (ie, Davey, Sam: [email protected]). However, the problem was easier to think about when I made the list of three dictionaries a list of one.
I solved the problem using a single dictionary. Then, I applied the same logic to the larger problem at hand.
When you simplify your given input, you make the problem much more manageable.
Solve a smaller problem
Spot patterns more easily and understand what you're really asked to do when you solve a smaller version of the problem.
Here’s an example problem from Reuven Lerner’s book, Python Workout :
“Use a list comprehension to reverse the word order of lines in a text file. That is, if the first line is abc def and the second line is ghi jkl, then you should return the list ['def abc', 'jkl ghi'].”
When solving a smaller version of a problem, I find it helpful to remove layers of complexity and use my ideal data structure. In this example, that meant ignoring the text file and list comprehension (layers of complexity) and using a list (my ideal data structure).
Then I solved the problem. I opened up my editor and typed out my ideal data structure.
I reversed the order and got the expected result using a for loop.
Once I got that working, I added the layers of complexity back in one at a time until I solved the problem as the problem statement asked.
Solving a smaller version of the problem helps get you to the heart of what you need to do. It's also another way to make the complex simple.
Take a break
Your brain doesn’t stop thinking just because your fingers stop typing.
Has an idea ever “popped” into your head while you were doing something other than programming? Have you ever returned to a problem after a workout and the solution is staring you in the face? It’s happened to me.
It’s no coincidence that you arrived at your idea or solution when you were doing something else—when you weren’t deliberately working. “Epiphanies may seem to come out of nowhere,” explains Scientific American , “but they are often the product of unconscious mental activity during downtime.”
If you feel like you’re running up against a brick wall, you very well could be. It may be best to take a break. Give your mind some time to digest what you’re working on and return to the problem renewed.
Pair with another programmer
Working with someone else can be a great way to generate ideas and see a problem from another perspective. But I highly recommend you do everything in your power to get yourself unstuck first.
There will always be roadblocks. Learning how to troubleshoot your own problems is a critical skill to learn. It’s easy to say “I don’t get it. Let me ask this senior engineer.” Then, have the senior engineer solve the problem for you. It’s harder to figure it out yourself, but you need to at least try.
If you’ve sincerely put your best foot forward, then reach out to a programmer with a specific question. This shows respect for the other programmer’s time and it’ll make your pairing session more effective.
Then, ask for a hint—don’t have the programmer solve the problem for you. You’ll undoubtedly encounter a similar situation down the road, so use the pairing session as a learning opportunity. It’ll help you in the long run.
Wrapping up
Getting stuck is frustrating, to be sure. You may try just one of the above tactics and have a “light bulb” moment, or you may need to try a combination and find yourself simply inching along during the process.
But there are two things I’ve learned from embracing the struggle: I’m learning a lot and the breakthrough is coming. Keep at it.
I write about learning to program, and the best ways to go about it ( amymhaddad.com ).
Programmer and writer | howtolearneffectively.com | dailyskillplanner.com
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Code with Jason
The beginner's guide to rails testing.
Get answers to the 8 most common Rails testing questions.
Stuck on a programming problem? These tactics will get you unstuck most of the time
Being stuck is possibly the worst state to be in when programming. And the longer you allow yourself to stay stuck, the harder it is to finally get unstuck. That’s why I find it important to try never to allow myself to get stuck. And in fact, I very rarely do get stuck for any meaningful length of time. Here are my favorite tactics for getting unstuck.
Articulate the problem to yourself
I teach corporate training classes. Often, my students get stuck. When they’re stuck, it’s usually because the problem they’re trying to solve is ill-defined. It’s not possible to solve a problem or achieve a goal when you don’t even know what the problem or goal is.
So when you get stuck, a good first question to ask is: do I even know exactly what the problem is that I’m trying to solve? I recommend going so far as to write it down.
If it’s hard to articulate your goal, there’s a good chance your goal is too large to be tractable. Large goals can’t be worked on directly. For example, if you say your goal is “get to the moon and back”, that goal is too big. What you have to articulate instead are things like figure out how to get into space, figure out how to land a spaceship on the moon, figure out how to build a spacesuit that lets people hang out in space for a while, etc.
Just try something
Far too often I see programmers stare at their code and reason about what would happen if they changed it in such-and-such a way. They run intricate thought experiments so they can, presumably, fix all the code in one go and arrive at the complete solution the very next time they try to run the program. Nine times out of ten, they make the change that they think will solve the problem and then discover they’re wrong.
A better way to move forward is to just try something. The cost of trying is very low compared to the cost of reasoning. Also, trying has the added bonus of supplying empirical data instead of untested hypotheses.
Articulate the problem to someone else
“Rubber duck debugging” is the famous tactic of explaining your problem to a rubber duck on your desk. The phenomenon is that even though the duck is incapable of supplying any advice, the very act of explaining the problem out loud leads you to realize the solution to the problem.
Ironically, most of the “rubber duck” debugging I’ve done in my career has involved explaining my issue to a sentient human being. The result is often the classic scenario where you spend ten minutes explaining the details of a problem to a co-worker, realize the way forward, and then thank your co-worker for his or her help, all without your co-worker uttering a word.
The explanation doesn’t have to be verbal in order for this tactic to work. Typing out the problem can be perfectly effective. This leads me to my next tactic.
Put up a forum question
In my experience many programmers seem to be hesitant to write their own forum questions. I’m not sure why this is, although I have some ideas. Maybe they feel like posting forum questions is for noobs. Maybe they think it will slow them down. Neither of these things are true, though. I’ve been programming for over 20 years and I still post Stack Overflow questions somewhat regularly. And rather than slowing me down, posting a forum question usually speeds me up, not least because the very act of typing out the forum question usually leads me to realize the answer on my own (and usually about 45 seconds after I post the question ).
If you don’t know a good way, try a bad way
Theodore Roosevelt is credited with having said, “In any moment of decision, the best thing you can do is the right thing, the next best thing is the wrong thing, and the worst thing you can do is nothing.” I tend to agree.
Often I’m faced with a programming task that I don’t know how to complete in an elegant way. So, in these situations where I can’t think of a good solution, I do what I can, which is that I just move forward with a bad or stupid solution.
What often happens is that after I put my dumb solution in place, I’ll take a step back, look at what I’ve done, and a better solution will make itself fairly obvious to me. Or at least, a nice solution will be easier for me to think of at this stage than it would have been from the outset.
With programming and in general, people are much better at looking at a bad thing and saying how it could be made good than they are at coming up with a good thing out of thin air.
If you go down a bad path and find that you’ve completely painted yourself into a corner, it’s no big deal. If you’re using version control and using atomic commits , you can just revert back to your last good state. And you can start on a second attempt now that you’re a little older and wiser.
Study the hell out of the problem area
There’s a thought experiment I like to run in my head sometimes.
Let’s say I’m working on a Rails project and I can’t quite figure out how to get a certain form to behave how I want it to behave. I try several things, I check the docs, but I just can’t get it to work how I want.
In these situations I like to ask myself: “If I knew everything there was to know about this topic, would I be stuck?”
And the answer is of course not. The reason why I don’t understand how to get my form to work is exactly that—I don’t understand how to get my form to work. So what I need to do is set to work on gaining that understanding.
In an ideal world, a programmer could instantly pinpoint the exact piece of knowledge he or she is missing and then go find that piece of knowledge. And in fact, we’re very fortunate to live in a world where that’s possible. But it’s not possible 100% of the time.
In those cases I set down my code and say okay, universe, I guess this is how it’s gonna be. I’ll go to the relevant educational resource—in the case of this example perhaps the official Rails documentation on forms—and start reading it top to bottom. Again, if I knew everything about Rails forms then I wouldn’t have a problem, so I’ll set out of a journey to learn everything. Luckily, my level of understanding usually becomes sufficient to squash the problem long before I read everything there is to read about it.
Write some tests
One of the reasons I find tests to be a useful development or debugging tool is that tests help save me some mental juggling. When developing or debugging there are two jobs that need to be done: 1) write the code that makes the program behave as desired and 2) verify that the desired behavior is present.
If I write code without tests, I’m at risk of mentally mixing these two jobs and getting muddled. On the other hand, if I write a test for my desired behavior, I’m now free to completely forget about the verification step and focus fully on coding the solution. The fewer balls I have to mentally juggle, the faster I can work.
Take a break
One of my favorite Pink Floyd songs is “ See Emily Play “. In the first chorus they sing “There is no other day / Let’s try it another way.” In a later chorus they sing “There is no other way / Let’s try it another day.” This is great debugging advice.
I often find that if I allow myself to forget about whatever problem I’m working on and go for a walk or something, my subconscious mind will set to work on the problem and surface deliver the solution later. Sometimes this happens 15 minutes later. Sometimes it doesn’t happen until a day or a week later. In any case, it’s often effective.
Work on something else
If all else fails, you can always work on something else. This might feel like a failure, but it’s only a failure if you decide to give up on the original problem permanently. Setting aside one goal in exchange for making progress on a different goal is infinitely better than allowing yourself to stay stuck.
One thought on “ Stuck on a programming problem? These tactics will get you unstuck most of the time ”
Agree 100% — In the shower the next day is the usual Eureka moment.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
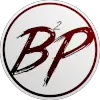
Is It Normal To Be Stuck in Programming Problems?
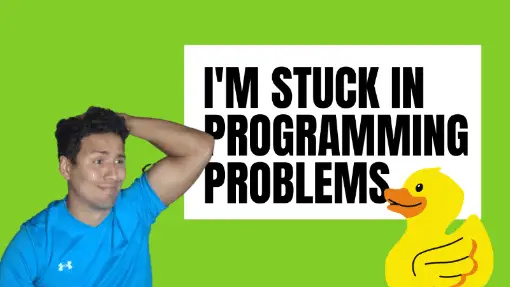
Like many things in life, people get stuck doing certain things. We come across different challenges preventing us from moving forward and taking steps to where we want to head towards to. In a similar way, you get stuck while programming. If you feel embarrassed about asking this question to someone and decided to anonymously find an answer on the web, let me tell you this is an excellent question! and there’s nothing to be ashamed of.
Whether you write code for a living or enjoy developing random projects during your free time, it is normal to get stuck when programming. Whether you are a new programmer or have 20 years of working in the industry, programmers always get stuck with a problem. Programming is not about the act of typing words and writing code, but solving problems.
Some problems will be more challenging than others. Some will seem simple but they are more complex than what we think. Sometimes you believe you can fix the problem in 5 minutes, but you end up spending 10 hours fixing it. Sometimes you don’t even know how long it could take to figure out something and you end up making it work in a couple of hours. Programming could be the best thing it could have ever happened to you when you figure something out or the worst thing that has existed on the planet if you are stuck for hours or days making something work.
Table of Contents
Should I Quit Programming?
Thoughts about quitting programming often come across to new programmers. The main reason is that everything is hard at the beginning. Whether you make your first HTML table, or style the border of a card using CSS, or get data from the database using an API, etc, things seem simple and straightforward are not making you frustrated as your instructor or more senior coworkers can make things work in a matter of seconds while you can make it work in a matter of hours or even days.
It wouldn’t surprise me either if more experienced programmers have thoughts about quitting as well. Regardless of where you are in your programming journey, give it some time before you make any drastic decision.
Contrary to what most people will say, it is ok to quit programming. There is nothing stopping you from quitting programming. There will be people, especially those who are in the industry who will strongly encourage you to not stop programming. However, this decision is personal and people should understand no matter what they think. Sometimes, it is not the best time to program. Sometimes, you don’t have enough time to dedicate to programming. Sometimes people are better at learning other things, and that is normal.
However, before you make the decision of quitting, I will share my piece of advice. I strongly believe anyone can program and make a living of it. Anyone who is willing to go through the struggles and overcome them can learn how to program. That’s why should not quit programming right away. Getting stuck constantly is one of the many obstacles you will find when programming. Training your body to get used to being in uncomfortable situations is hard and sucks for anyone. Nevertheless, once you pass through those obstacles the feeling of satisfaction is priceless and cannot be described with the joy once you make things work out.
If you are reading this article, that means you have internet. Think about the people who wanted to be programmers at other times. There was no such thing as StackOverflow, or YouTube, or Google, or even worst no internet. Learning how to program had to come from a pure desire to make things work. Programmers had to buy and read through extensive technical books in hopes to get an answer to help them get unstuck.
Thinking about quitting programming in those times seemed more justifiable due to all the struggles programmers faced due to the technology stages they were during their times. The desire to move forward and develop great things lead to many programmers making it more accessible for newer generations to get their feet wet in the world of programming. With more and more available resources to learn how to program and even to solve common programmatic problems, there has never been a better time to get in the world of programming than now.
What to Do? 13 Tips Get You Unstuck as a Programmer
As previously mentioned, it is ok to get stuck. What matters is what we do to get out of that situation. As you gain more and more experience you start developing the skills to get unstuck quicker. There are some tips in case you need help trying to move forward. Chances are you have tried some of the following tips. In case you haven’t tried any, give them a try!
1. Recognize You Are Stuck
Recognizing you are stuck might not be that obvious at first. However, realizing you are in a place where there are more unknowns than clarity is more powerful than you think. Failing to recognize it could lead to spending unnecessary time trying to make things work that are not that necessary.
Not because you don’t know how to do something and can’t figure out how to make it work within 5 to 10 minutes that means you are stuck. Confusing the definition of getting stuck and not trying more could hurt your abilities to solve problems in the long term.
2. Relax (At Least Try To Calm Down)
As programmers, generally we encounter ourselves in the following scenario: Start working on a task, writing some code that works, come across with a bug, try to get it solved, if you haven’t solved it yet, try harder to get the problem solved, frustration starts to increase, try anything that works, become fully frustrated, destroy the keyboard. For some, this sounds funny as it is not too far from reality. The problem turns harder to solve when we start getting frustrated.
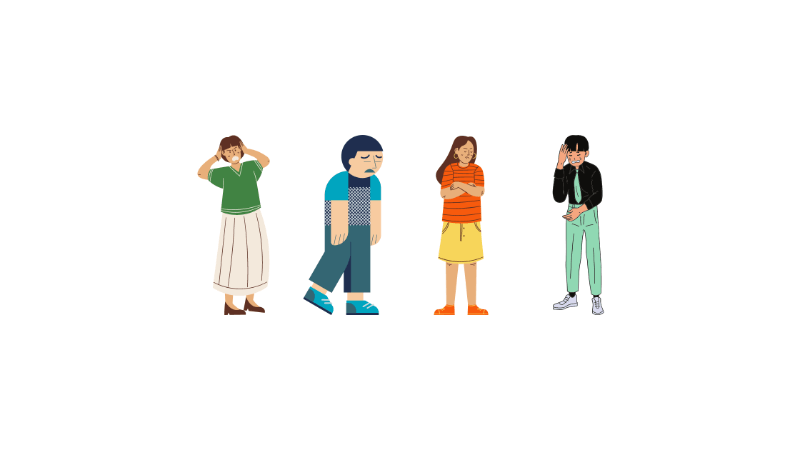
It is crazy to think how the way you feel impacts your ability to solve problems. Senior programmers often recommend coding when you don’t think of anything that is bugging your brain constantly such as paying the bills, worrying about your kids’ health, looking for another job, thinking about the discussion you had with your relative, etc. Your brain is not fully focused on what needs to be focused. Being frustrated leads to the same. By being frustrated, you become more concerned about not making things work rather than finding alternatives to making it work.
One of my bosses once told me: One of the best work happens when we are relaxed. It’s true. It gives freedom to your brain to explore and discover new ways to make things happen. Sometimes, frustration makes us keep the focus on the solution we are trying to implement which got us stuck at first. This leads us to not try different alternatives because we insist on making work the first piece of code written.
3. Read and Try to Understand The Error
This one seems obvious, but it isn’t. It is not a secret we live in a world with access to information about anything. In the programming world, you can find just about any kind of solutions to common problems programmers come across. Unfortunately, that is not always the case.
Going directly to your favorite search engine and try finding a solution for the problem is what leads you to get stuck. Why? because you don’t have true clarity of what the error is. Oftentimes, we get error logs displayed in the terminal and we don’t even read at the whole log. We only see the part of the log that says “[Error]” or “error” and automatically turn our heads to googling.
Sometimes the bug is as simple as a variable name typo causing the application to crash. Take your time to read and understand the error as this will allow you to better choose what next to do, whether it is to try a different solution, ask someone else for help, or googling.
4. Talk it Outloud
Haven’t you had a time when you talked to someone about a problem but you needed to provide more context for that person to understand better what is going on for them to help you, but you ended up finishing the conversation before the other person talked as you realized what you needed to do?
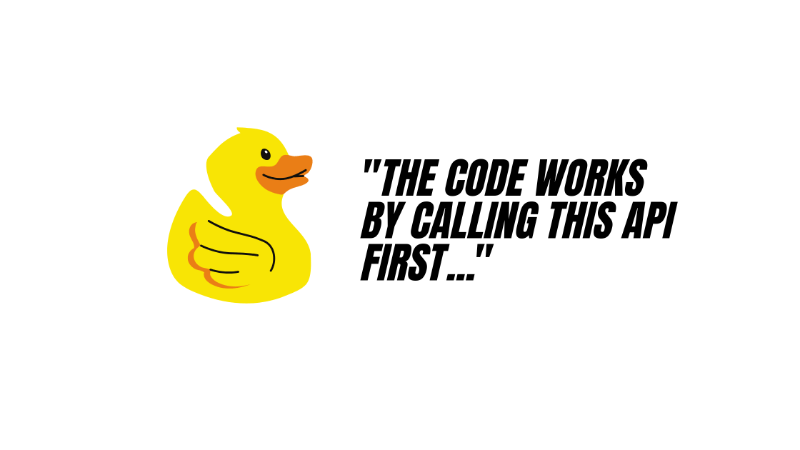
One of the best ways to find solutions is by talking out loud the code, line-by-line, to someone else or to a rubber duck. This is more commonly known as the rubber duck debugging technique. This is useful sometimes and will help you come across a solution without you even trying to think of a solution.
5. Debug Your Code
Logging pieces of information can only get you so far in understanding the root cause of an error. Many programmers like to clutter the code full of logs making it harder to understand. A quick log can oftentimes provide meaningful information. However, that’s not always the case.
Programmers not debugging their code are making it hard on themselves to understand the problem and tu find a solution. There are cases where programmers don’t know how to debug in a specific programming language. Setting up your local environment must be the first thing you do before starting to write code, which includes setting up debugging tools. This could be time-consuming if you haven’t done it before. However, being able to debug your code is a blessing when you have code with thousands of lines and you are trying to spot the problem.
In case you already have your debugging tools set up, I encourage you to take advantage of them. Sometimes we think we understand how the code works. However, once we start debugging we find behaviors we didn’t anticipate when we initially added our piece of code.
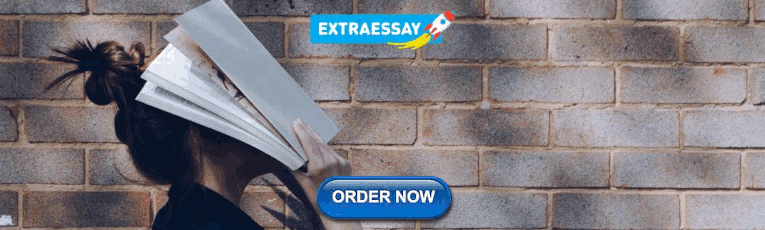
6. Implement an Ugly Solution
Implementing an ugly solution is a hard choice for those who always look for quality code. The reason being is because there is a pre-existing high expectation of delivering high quality all the time, whether it is a highly reusable function or a more performant solution, or following best practices, your brain focuses the attention on writing quality code.
Sometimes an ugly solution can be the best solution. At the end of the day, programmers make things work and not write reusable and high performant code that fails. If something small fails, the whole project could fail, and users quickly lose trust in the software. A better approach is to make things work first, then optimize or improve off of an existing solution. Overengineering is very common when there shouldn’t be. Keep it simple. Keep it ugly. That’ll do the trick sometimes.
7. Comment Out Chunks of Code
This is one of my favorite techniques to understanding what the problem is. Computers run the code that is written. They don’t guess or decide whether or not to execute a piece of code. They just do it. If the code doesn’t exist or is commented out, it won’t run it.
Commenting out chunks of code is a simple and effective approach to getting you unstuck. Sometimes you don’t know what the code does or cannot figure out an unexpected behavior. By commenting out certain pieces of code, you will start getting helpful cues of where the code breaks. Sometimes we think it is failing at the end of a function when in reality it is breaking at the beginning of a function.
Commenting out code won’t get you unstuck, but having a clear understanding of the problem is better than trying all things possible to make something work without knowing what you wrote in the first place.
8. Google it, aka Research, Research, and Research
It is funny how we have adopted the word “google” to mean to search it online. Programmers should be ok googling as many times as they need to . At the end of the day, it is a tool. It is not about the quality of the tools, but how well you know how to use the tools. Like many things in life, it takes time to master how to use the tools and it is no different when googling.
Sometimes you notice other people googling for the same solution you are stuck for hours and they are successful after you had spent hours and hours googling in hopes to get a solution. With more time and experience, you will be able to improve your research skills and discover search patterns that will allow you to find the information you need to move forward rather than remain stuck.
9. Ask Questions to Someone Else
There is nothing wrong with asking for help or having a second pair of eyes to look at our code. Although oftentimes junior programmers ask questions to more experienced programmers, there is nothing wrong if you have 15 years of experience and you ask someone who has a few years of experience on their back.
It is complex to know it all in the world of programming. There will always be something someone knows that you don’t know. It is surprising sometimes to see where some of the best answers come from, and some of them might be from people you never thought they could.
10. Ask Questions in Forums
Many programmers look for other solutions to common problems, read StackOverflow and GitHub forums but never post a question. There might be different reasons why many don’t do it.
Some people are afraid to ask questions publicly and expose themselves to being pointed at as unintelligent. However, sometimes is surprising to see how many people have the same question you have.
Other people don’t do it because of laziness. We become lazy at doing certain simple things without realizing it. For instance, some programmers don’t ask questions in forums because they don’t have an account in that forum, even if they visit the forum over and over. It is quick to create an account. However, the desire for looking for similar questions leads up to not even try posting the question. In other cases, programmers need the answer right away and can’t wait for someone to answer the question in the forum. Posting the question in a forum and working on sometime while you get some answers might most efficient use of your time in some cases.
11. Check the Documentation
Not everyone does this at first. However, the documentation is made to provide a single source of truth to how a programming language, library, framework, or architecture works. Spending some time reading through the documentation gives you a better understanding of how things work as well as provides you with ideas on how to tackle the same problem using different approaches.
12. Take Breaks or Walk Away
When you have tried several things and researched a lot of information but you can’t still make things work, you become tight and stressed. Taking a break is your best bet at this point. Going for a walk or stepping away from the computer are simple, yet effective ways to declutter your mind and relax for a little bit.
You could opt to walk away but still think about the problem you can’t solve. The main difference here is you are not staring at a screen reading your code or online information that distracts you from thinking clear after a while. Being able to put pieces of information in a zero to low distraction environment helps many with coming up with solutions. Just make sure to take a pen and paper so you write it whenever you come across them.
13. Let it Go
There have been cases where software has a particular error that never gets solved as it doesn’t impact the user’s experience or doesn’t compromise the business logic. In other cases, it is an error nobody in the team has been able to solve before due to its complexity.
Regardless of what the reasoning behind is, it is ok to not move forward with attempting to make something work. Initially, you might not feel good about this as per your desire of making things work as a programmer. However, sometimes not insisting to make work things that were not meant to work is the best decision.
Shower Aha! Moments
The best moments are whenever you are doing something different than trying to fix a programming problem and unexpectedly you come across a solution. Aha! Those are the moments you enjoy the best as a programmer and also find it hard to explain to someone why you wanted to work on complex stuff at first. Therefore, if you are stuck, don’t let it get you down. Find that as an opportunity to challenge yourself and demonstrate you can make anything work!
Was this article helpful?
Share your thoughts by replying on Twitter of Become A Better Programmer or to personal my Twitter account .
Are you a #programmer who gets stuck all the time? Is this normal? Programming is frustrating when you cannot figure things out and you are stuck. That's why I decided to talk about this in my latest article! https://t.co/9UZk5clEyr #programminglife #advice — Become A Better Programmer (@bbprogrammer) September 8, 2021
Getting Stuck on a Programming Problem
Trevor Page
Get the Best Java Roadmap
Download the roadmap.
We've all been there before. We're compiling or running some code and it's just not working. You could be seeing things like Java exceptions in your logs or maybe your IDE is telling you there's something wrong with your code and it's being very cryptic.
How on earth are we supposed to go on from this point? If you're fairly new to programming, you're likely going to be dead in the water until you get some help.
So where can you go to get that help? That's what I'm going to talk about today. I've had tons of questions come in from beginner Java programmers who get stuck in a certain spot and have no idea where to turn for help. Here are the places I would turn to for help:
How to Program with Java.com
If you are following along with this site when learning the Java language, I would love it if you would ask your questions in the comments section on any of these posts. Asking your questions here helps in the following ways:
- I just sincerely enjoy helping people out.
- All the other readers of this site will benefit from seeing the answer to your question, as they likely have the SAME question too!
- Gives me ideas on other topics that I can cover if I notice the same questions coming in.
Stackoverflow.com
Whenever I have been REALLY stuck on a complex programming problem, I have turned to this site with great success. You just ask your question, and there are literally TONS of programmers at your disposal who will try to help you get to the bottom of your problem. Many times I've had developers solve my problems by making me realize that the overall design of my solution needs to be tweaked. To me, this is the best solution to any problem, because if I had just tried to “hack” in a solution to a poor design, I would have WAY more problems in the future. Better to solve the root of the problem instead of the symptoms right?
So, overall, this website is extremely helpful as it can “crowdsource” the proper solution.
This seems like a no-brainer, and for the most part it is. But here's a few tricks that I use whenever I'm trying to solve a problem. I typically solve run-time errors in this fashion:
- Isolate the exception in your logs/console
- In your logs/console, find out if you can see the words “Caused By:”
- Copy/paste the line of words after “Caused By:” into Google and search
This tactic is good for finding related articles on people who have had the same problems as you, and seeing how they went about solving them.
Note that if you don't any search results, it's very likely that you have some information in the line that you copy/pasted that's specific to YOUR program. See if you can isolate any words that are specific to your program and remove just those words, then do the search again.
What if you don't see “Caused By:”
Well, no worries, the trick I do here is I can the exception until I can see a Class that I recognize. Let's take a look at the following example stack trace:
This is a problem I recently had with one of the websites I created, and here's how I went about solving it.
- If you look at the stacktrace error as a whole, it seems very intimidating, so I like to simplify it by just looking at the blue underlined text .
- I scan them one by one from top to bottom until I find a Class name that I recognize
Note : The first blue underlined text says org.hibernate.HibernateException so this means that the problem has to do with the Hibernate framework… good to know
- SpringSessionContext.java – Means nothing to me, so I keep looking
- SessionFactoryImpl.java – Nope
- UsersDao.java – Aha! I recognize that class, as I created it to save the information for the Users that belong to my program.
- Now I look at the actual error: No Hibernate Session bound to thread, and configuration does not allow creation of non-transactional one here
- So I take that error and copy/paste it into Google armed with the knowledge that it has to do with the Hibernate framework and that it's happening in my UsersDao class, which I use to save user information
- The first result is a question from stackoverflow click here if you want to see it. And what do you know, I scroll down in the stackoverflow question until I see an answer that was given by a user and it has a green checkmark to the left of it. This denotes that the user's problem was solved by this answer. The person answering the question says that you likely need to include the @Transactional annotation on your class.
- I put the @Transactional annotation on my UsersDao class to test and see if this fixes my problem… and what do you know, my problem is SOLVED!!!
Trial and Error
I generally go through a few iterations of the Google approach by using a trial and error approach. If the first proposed solution I find on Google doesn't work, I try a few more. If I've been stuck on the same problem for hours, I do something interesting:
I step away from the problem!
I can't stress this technique enough. I have wasted so many hours of my life by hammering away at a problem with no success, only to take a break or go to bed, and then come back to the problem and solve it in 5 minutes. It's just the way we're wired as humans, as I've done the same thing with learning to play a song on the guitar or piano, you can practice your butt off and never really play the song very well… but after you take a break and come back, you've made surprising progress. Coding is no different, so take a break !
And finally, if I haven't been able to solve the problem over the course of many days, I'll post the question on stackoverflow and always get a solution from someone there… that's just my approach, your mileage may vary, but your secret weapon is that you have ME to help you out should you be frustrated and pulling your hair out!
I hope that helps to shed some light on some troubleshooting techniques, now if you're really serious about learning how to program with Java, I just launched a new website that aims at teaching you Java (as well as other technologies needed to be a real website developer). This tutorial series is very detailed and uses videos as its medium. Unfortunately a subscription to this website is not free, as I had to spend a fair bit of extra money on the hosting of both the website and the videos, and I purchased a neat tablet that allows me to write on the screen using a pen/tablet combination… this gives a real “classroom” feel to the tutorials :)
Take care everyone, and happy learning!
Free Java Roadmap
Discover exactly what you need to learn and where to start in order to become a professional coder.
Coders Campus
Launch your coding career..
© 2021 • Crafty Codr Inc • Terms • Privacy
151 Lyndhurst Drive, Kitchener, Ontario N2B 1B7 Canada
Help Articles
Solve problems with programming assignments, learner help center dec 5, 2022 • knowledge, article details.
If you're having problems with a programming assignment, check for your issue below.
If your problem is with a different kind of assignment, or you don't see your issue here, check our assignment troubleshooting page.
Can't submit programming assignment
If you're having trouble submitting a programming assignment, try the following steps:
- Make sure you saved your files in the correct format. To change the format, re-save your files.
- Decrease the size of the files, if possible.
- Clear your browser's cookies and cache.
- Making sure any ad-blocking software is turned off on your browser or network.
- Ask your peers for help by posting in the course discussion forums. Many programming assignment issues have very specific requirements, so people in the same course will be able to help you more than Coursera's support team.
No grade on programming assignment
If the assignment uses custom grading, it will take up to an hour to get your grades back.
If you've waited longer than an hour and still don't have a grade:
- Check the assignment instructions and make sure you have saved your files in the right format.
- Resubmit the assignment.
Understanding your grade
Because many programming assignment graders use complex logic that the instructor creates, the Coursera support team can’t explain or change programming assignment grades.
To understand your grade on a programming assignment, review the assignment instructions and any feedback you got.
You can also discuss any questions with your peers in the course discussion forums.
Assignment not marked complete
Weeks that include programming assignments may still say in progress even after you've successfully completed the assignment.
Check your Grades tab to confirm that you've successfully completed the assignment.
Token issues
If you're having trouble with a token not syncing or not showing up, try
- Making sure your ad-blocking software is turned off on your browser or network
- Making sure you are logged in with the correct account
- Clearing your cache and cookies
- Using a different browser or new browser window
- Check if your computer’s clock is set accurately
Related Articles
- Number of Views 47.95K
- Number of Views 36.95K
- Number of Views 69.25K
- Number of Views 81.41K
- Number of Views 100.48K
© 2021 Coursera Inc. All rights reserved.
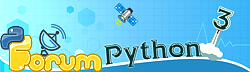
- View Active Threads
- View Today's Posts
- View New Posts
- My Discussions
- Unanswered Posts
- Unread Posts
- Active Threads
- Mark all forums read
- Member List
- Interpreter
I am stuck on functions
- Python Forum
- Python Coding
- 0 Vote(s) - 0 Average
- View a Printable Version
User Panel Messages
Announcements.
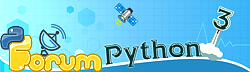
Login to Python Forum
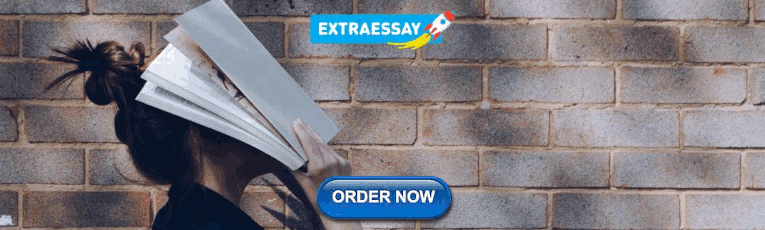
IMAGES
VIDEO
COMMENTS
Pass the argument to the callback function. Reset the "maxValue" variable to 0. Resetting the "maxValue" variable, for example, didn't solve the problem. But it was an important step in the process. Writing this short phrase got me back on track: it was a reminder of what I'd set out to do.
There is one person you can ask: your professor. At worst, they will tell you they can't answer the question. But there's a chance that they can provide some answer that might help: maybe the problem hasn't been worded clearly, or maybe they can suggest that you document any assumption you can make that will "unstuck" you. (You may not have a correct answer, but often there is partial credit ...
1. Try working out small cases by hand. Try to figure out the methodology used to do this. 2. Figure out how you would generalize your methodology to larger cases, as well as how you would overall implement it in code. Once you know the rough outline of how you would implement it in code, you basically have an algorithm.
And in fact, I very rarely do get stuck for any meaningful length of time. Here are my favorite tactics for getting unstuck. Articulate the problem to yourself. I teach corporate training classes. Often, my students get stuck. When they're stuck, it's usually because the problem they're trying to solve is ill-defined.
Assignment to constant variable; Assignment to constant variable JavaScript; Assignment to constant variable JavaScript Sleep Debt Calculator; Tap into programming resources. If you're working through Codecademy course material, we have additional resources to help you get unstuck.
Each type of problem has a different type of solution. In general, I'd say that sometimes you need to redesign your approach to completely avoid the blocker that's causing you trouble. Remember that there are many ways from start to finish, so it'll often help to take a different path. 3. WeaughTeaughPeaugh.
If I ever got stuck on a problem I'd try to take a nap, or just sleep it off depending on the time of day, and I'd invariably figuring it out right away the next time looking at the code. ... The subreddit covers various game development aspects, including programming, design, writing, art, game jams, postmortems, and marketing. It serves as a ...
Now that we know exactly what our code is supposed to do, we have one more step… can you guess what it is?. 5. Replace pseudocode with real code. Here's the fun part. Now that you know for ...
Whether you write code for a living or enjoy developing random projects during your free time, it is normal to get stuck when programming. Whether you are a new programmer or have 20 years of working in the industry, programmers always get stuck with a problem. Programming is not about the act of typing words and writing code, but solving problems.
Stuck on Java Programming Homework Assignment. Ask Question Asked 12 years, 4 months ago. Modified 7 years, 9 months ago. Viewed 1k times 0 I am working on a problem for homework in a Java Programming course, and I am stuck. I will tell you upfront that I am by no means an expert, and don't know much about Java or Programming in general.
This seems like a no-brainer, and for the most part it is. But here's a few tricks that I use whenever I'm trying to solve a problem. I typically solve run-time errors in this fashion: Isolate the exception in your logs/console. In your logs/console, find out if you can see the words "Caused By:". Copy/paste the line of words after ...
Can't submit programming assignment. If you're having trouble submitting a programming assignment, try the following steps: Make sure you saved your files in the correct format. To change the format, re-save your files. Decrease the size of the files, if possible. Clear your browser's cookies and cache. Making sure any ad-blocking software is ...
People mostly get stuck because they instantly try to write programs for their assignments instead of thoroughly analysing them, breaking them down. When you get an assignment, read it multiple times, then take a break and do something else, unrelated to programming. Take a walk, a shower, work out. Give your brain time to process the ...
Hi all, I am working on a lab assignment and I am stuck...probably because it's friday and my brain stopped at 5pm. At any rate, here's the actual problem: Create a Python program that does the following: Each gymnast in a competition receives scores from 5 judges. • Create a loop that loops 5 times • Inside the loop, prompt the user to enter a score (from 0 to 10), and store it in a list ...
I am currently stuck on a programming assignment and could use some help. I have attached what I have already completed and the directions I am stuck on. Thanks in advance. import java.util.Scanner; public class LiamBurnsLab8 {/** * @param args the command line arguments */
Stuck on a programming assignment; don't understand why i'm wrong . I am in a basic programming class so you all will probably think this is a silly question and super basic. But I am struggling with an assignment where, given two sequences of RNA, I have to write a program that checks for base-pair matches between them. The first part of the ...
Hey guys, So I'm taking an intro to programming class and for our third assignment, the professor is asking us to write a program which will take integers... Home. Forums. New posts Search forums. What's new. New posts New media New media comments New profile posts Latest activity. Media.
The item code is 12345 and the customer chooses to pay via two years of hire purchase. The program operates as follows: Write a program, HirePurchase, which prints the invoice for an item on hire purchase as detailed above. The program prompts for the item code, cash price and the number of years on hire purchase. enter image description here
Stuck on programming assignment/homework? This thread is archived New comments cannot be posted and votes cannot be cast Related Topics Programming comments sorted by Best Top New Controversial Q&A More posts from r/learnprogramming subscribers . ermahgerdreddits • I love all these new youtube videos saying "programming isn't enough" ...