- Python Course
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
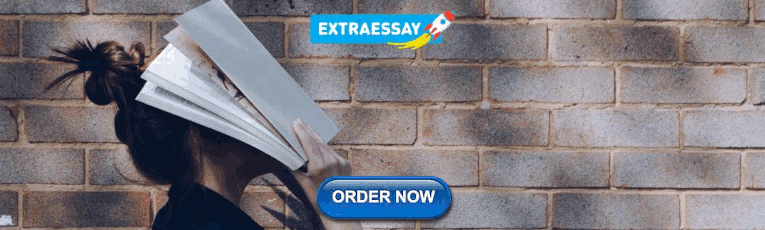
How to Fix IndexError – List Index Out of Range in Python
In Python, the IndexError is a common exception that occurs when trying to access an element that is outside the valid range of indices. Let’s understand this with help of examples and how to fix Index out of Range issue.
I ndexError Examples
Given list consists of 3 elements and hence, last index is 2. We are trying to access element at index 3, which will cause IndexError.
Similarly, we can also get an Indexerror when using negative indices.
Reason for IndexError in Python
1. Accessing Non-Existent Index: When you attempt to access an index of a sequence (such as a list or a string) that is out of range, an Indexerror is raised. Sequences in Python are zero-indexed, which means that the first element’s index is 0, the second element’s index is 1, and so on.
2. Empty List: If you try to access an element from an empty list, an Indexerror will be raised since there are no elements in the list to access.
How to Fix List Index Out of Range
Let’s see some examples that showed how we may solve the error.
- Using Python range()
- Using Python Index()
- Using Try Except Block
Python Fix List Index Out of Range using Range()
The range is used to give a specific range, and the Python range() function returns the sequence of the given number between the given range.
Python Fix List Index Out of Range u sing Index()
Here we are going to create a list and then try to iterate the list using the constant values in for loop .
Reason for the error – The length of the list is 5 and if we are an iterating list on 6 then it will generate the error.
Solving this error without using Python len() or constant Value: To solve this error we will take the index of the last value of the list and then add one then it will become the exact value of length.
Python Fix List Index Out of Range using Try Except Block
If we expect that an index might be out of range, we can use a try-except block to handle the error gracefully.
Similar Reads
- Python How-to-fix
- python-list
Please Login to comment...
- Free Music Recording Software in 2024: Top Picks for Windows, Mac, and Linux
- Best Free Music Maker Software in 2024
- What is Quantum AI? The Future of Computing and Artificial Intelligence Explained
- Noel Tata: Ratan Tata's Brother Named as a new Chairman of Tata Trusts
- GeeksforGeeks Practice - Leading Online Coding Platform
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Staging Ground badges
Earn badges by improving or asking questions in Staging Ground.
How to solve the error 'list assignment index out of range' in python
I try to store the number of weekofyear from the list of data named date_range which stored the data such as
by using the following code
However,the IDE show the error 'list assignment index out of range'. How to solve this problem ?

- Does this answer ur question: stackoverflow.com/questions/64552247/… – Sushil Commented Oct 29, 2020 at 3:15
2 Answers 2
For an explanation of why you get surprising results to your other approach, refer to this answer to your other question
When your list is empty in python you can not assign value to unassigned index of list. so you have 2 options here:
- Use append like : list.append(value)
- make a loop to assign a value to your list before your main for . Like below:

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged python list or ask your own question .
- The Overflow Blog
- What launching rockets taught this CTO about hardware observability
- The team behind Unity 6 explains the new features aimed at helping developers
- Featured on Meta
- Preventing unauthorized automated access to the network
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
- Feedback Requested: How do you use the tagged questions page?
- Proposed designs to update the homepage for logged-in users
Hot Network Questions
- Is it legal (US) to target drunk people and interview them to create (commercial) content a.k.a. street interviews?
- Table only works with blank row?
- Otherworldly – Numberlink / Marching Bands
- How to measure objects in Blender 4.2
- What happens when a car starts moving? The last moment the car is at rest versus the first moment the car moves
- How can I seal the joint between my wall tile and bathroom countertop?
- Is it possible for an overly frugal culture to have high economic growth?
- Sci-Fi Fantasy with a large ant and woman with jewels embedded on the underside of her forearm on the book cover
- Why pick up the arba minim prior to making the bracha?
- Would Acid Fog dissolve the opponent's equipment?
- Debian: apt pin not working for kernel package
- Is the Hilbert Mumford Criterion true over the reals?
- The shortcut hint
- How can I mount a Deore XT brake caliper on a flat-mount fork?
- Card design with long and short text options
- PCB design review of my line following robot
- Rename files with non-Latin characters
- Stick lodging into front wheel - is it preventable?
- Why does Windows 11 display a different ethernet MAC (hardware) address than Linux?
- What is the significance of Balaam's donkey being a she?
- Will this radio receiver work?
- Why does Alien B, who can't see Alien A (and vice versa), crash their ship specifically into Alien A?
- Are there two conflicting definitions of the basechange matrix?
- I have two different statements for the t test and the single tail test
Explore your training options in 10 minutes Get Started
- Graduate Stories
- Partner Spotlights
- Bootcamp Prep
- Bootcamp Admissions
- University Bootcamps
- Coding Tools
- Software Engineering
- Web Development
- Data Science
- Tech Guides
- Tech Resources
- Career Advice
- Online Learning
- Internships
- Apprenticeships
- Tech Salaries
- Associate Degree
- Bachelor's Degree
- Master's Degree
- University Admissions
- Best Schools
- Certifications
- Bootcamp Financing
- Higher Ed Financing
- Scholarships
- Financial Aid
- Best Coding Bootcamps
- Best Online Bootcamps
- Best Web Design Bootcamps
- Best Data Science Bootcamps
- Best Technology Sales Bootcamps
- Best Data Analytics Bootcamps
- Best Cybersecurity Bootcamps
- Best Digital Marketing Bootcamps
- Los Angeles
- San Francisco
- Browse All Locations
- Digital Marketing
- Machine Learning
- See All Subjects
- Bootcamps 101
- Full-Stack Development
- Career Changes
- View all Career Discussions
- Mobile App Development
- Cybersecurity
- Product Management
- UX/UI Design
- What is a Coding Bootcamp?
- Are Coding Bootcamps Worth It?
- How to Choose a Coding Bootcamp
- Best Online Coding Bootcamps and Courses
- Best Free Bootcamps and Coding Training
- Coding Bootcamp vs. Community College
- Coding Bootcamp vs. Self-Learning
- Bootcamps vs. Certifications: Compared
- What Is a Coding Bootcamp Job Guarantee?
- How to Pay for Coding Bootcamp
- Ultimate Guide to Coding Bootcamp Loans
- Best Coding Bootcamp Scholarships and Grants
- Education Stipends for Coding Bootcamps
- Get Your Coding Bootcamp Sponsored by Your Employer
- GI Bill and Coding Bootcamps
- Tech Intevriews
- Our Enterprise Solution
- Connect With Us
- Publication
- Reskill America
- Partner With Us
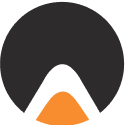
- Resource Center
- Bachelor’s Degree
- Master’s Degree
Python indexerror: list assignment index out of range Solution
An IndexError is nothing to worry about. It’s an error that is raised when you try to access an index that is outside of the size of a list. How do you solve this issue? Where can it be raised?
In this article, we’re going to answer those questions. We will discuss what IndexErrors are and how you can solve the “list assignment index out of range” error. We’ll walk through an example to help you see exactly what causes this error.
Find your bootcamp match
Without further ado, let’s begin!
The Problem: indexerror: list assignment index out of range
When you receive an error message, the first thing you should do is read it. An error message can tell you a lot about the nature of an error.
Our error message is: indexerror: list assignment index out of range.
IndexError tells us that there is a problem with how we are accessing an index . An index is a value inside an iterable object, such as a list or a string.
The message “list assignment index out of range” tells us that we are trying to assign an item to an index that does not exist.
In order to use indexing on a list, you need to initialize the list. If you try to assign an item into a list at an index position that does not exist, this error will be raised.
An Example Scenario
The list assignment error is commonly raised in for and while loops .
We’re going to write a program that adds all the cakes containing the word “Strawberry” into a new array. Let’s start by declaring two variables:
The first variable stores our list of cakes. The second variable is an empty list that will store all of the strawberry cakes. Next, we’re going to write a loop that checks if each value in “cakes” contains the word “Strawberry”.
If a value contains “Strawberry”, it should be added to our new array. Otherwise, nothing will happen. Once our for loop has executed, the “strawberry” array should be printed to the console. Let’s run our code and see what happens:
As we expected, an error has been raised. Now we get to solve it!
The Solution
Our error message tells us the line of code at which our program fails:
The problem with this code is that we are trying to assign a value inside our “strawberry” list to a position that does not exist.
When we create our strawberry array, it has no values. This means that it has no index numbers. The following values do not exist:
We are trying to assign values to these positions in our for loop. Because these positions contain no values, an error is returned.
We can solve this problem in two ways.
Solution with append()
First, we can add an item to the “strawberry” array using append() :
The append() method adds an item to an array and creates an index position for that item. Let’s run our code: [‘Strawberry Tart’, ‘Strawberry Cheesecake’].
Our code works!
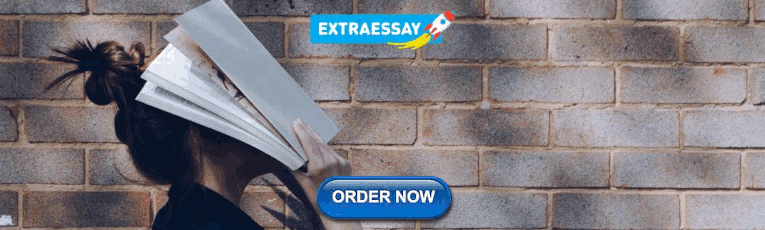
Solution with Initializing an Array
Alternatively, we can initialize our array with some values when we declare it. This will create the index positions at which we can store values inside our “strawberry” array.
To initialize an array, you can use this code:
This will create an array with 10 empty values. Our code now looks like this:
Let’s try to run our code:
Our code successfully returns an array with all the strawberry cakes.
This method is best to use when you know exactly how many values you’re going to store in an array.

"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Our above code is somewhat inefficient because we have initialized “strawberry” with 10 empty values. There are only a total of three cakes in our “cakes” array that could possibly contain “Strawberry”. In most cases, using the append() method is both more elegant and more efficient.
IndexErrors are raised when you try to use an item at an index value that does not exist. The “indexerror: list assignment index out of range” is raised when you try to assign an item to an index position that does not exist.
To solve this error, you can use append() to add an item to a list. You can also initialize a list before you start inserting values to avoid this error.
Now you’re ready to start solving the list assignment error like a professional Python developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication .
What's Next?

Get matched with top bootcamps
Ask a question to our community, take our careers quiz.
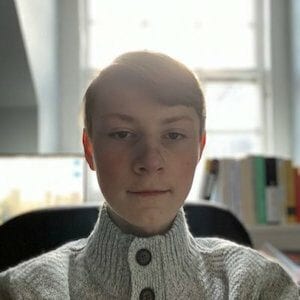
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
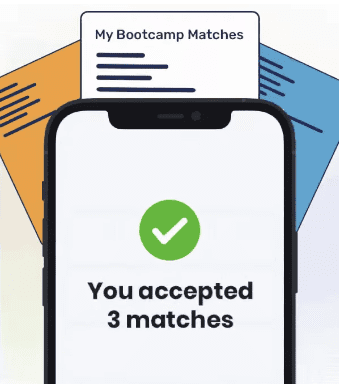
How to Fix the “List index out of range” Error in Python

- learn python
At many points in your Python programming career, you’re going to run into the “List index out of range” error while writing your programs. What does this mean, and how do we fix this error? We’ll answer that question in this article.
The short answer is: this error occurs when you’re trying to access an item outside of your list’s range. The long answer, on the other hand, is much more interesting. To get there, we’ll learn a lot about how lists work, how to index things the bad way and the good way, and finally how to solve the above-mentioned error.
This article is aimed at Python beginners who have little experience in programming. Understanding this error early will save you plenty of time down the road. If you’re looking for some learning material, our Python Basics track includes 3 interactive courses bundled together to get you on your feet.
Indexing Python Lists
Lists are one of the most useful data structures in Python. And they come with a whole bunch of useful methods . Other Python data structures include tuples, arrays, dictionaries, and sets, but we won’t go into their details here. For hands-on experience with these structures, we have a Python Data Structures in Practice course which is suitable for beginners.
A list can be created as follows:
Instead of using square brackets ([]) to define your list, you can also use the list() built-in function.
There are already a few interesting things to note about the above example. First, you can store any data type in a list, such as an integer, string, floating-point number, or even another list. Second, the elements don’t have to be unique: the integer 1 appears twice in the above example.
The elements in a list are indexed starting from 0. Therefore, to access the first element, do the following:
Our list contains 6 elements, which you can get using the len() built-in function. To access the last element of the list, you might naively try to do the following:
This is equivalent to print(x[len(x)]) . Since list indexing starts from 0, the last element has index len(x)–1 . When we try to access the index len(x) , we are outside the range of the list and get the error. A more robust way to get the final element of the list looks like this:
While this works, it’s not the most pythonic way. A better method exploits a nice feature of lists – namely, that they can be indexed from the end of the list by using a negative number as the index. The final element can be printed as follows:
The second last element can be accessed with the index -2, and so on. This means using the index -6 will get back to the first element. Taking it one step further:
Notice this asymmetry. The first error was trying to access the element after the last with the index 6, and the second error was trying to access the element before the first with the index -7. This is due to forward indexing starting at 0 (the start of the list), and backwards indexing starting at -1 (the end of the list). This is shown graphically below:

Looping Through Lists
Whenever you’re working with lists, you’ll need to know about loops. A loop allows you to iterate through all the elements in a list.
The first type of loop we’ll take a look at is the while loop. You have to be a little more careful with while loops, because a small mistake will make them run forever, requiring you to force the program to quit. Once again, let’s try to naively loop through our list:
In this example we define our index, i , to start from zero. After every iteration of our while loop, we print the list element and then go to the next index with the += assignment operator. (This is a neat little trick, which is like doing i=i+1 .)
By the way, if you forget the final line, you’ll get an infinite loop.
We encountered the index error for the same reason as in the first section – the final element has index len(x)-1 . Just modify the condition of the while statement to reflect this, and it will work without problems.
Most of your looping will be done with a for loop, which we’ll now turn our attention to. A better method to loop through the elements in our list without the risk of running into the index error is to take advantage of the range() built-in function. This takes three arguments, of which only the stop argument is required. Try the following:
The combination of the range() and len() built-in functions takes care of worrying about when to stop indexing our list to avoid the index out of range error entirely. This method, however, is only useful if you care about knowing what the index is.
For example, maybe you want to print out the index and the element. In that case, all you need to do is modify the print() statement to print(i, x[i]) . Try doing this for yourself to see the result. Alternatively, you can use The enumerate() function in Python.
If you just want to get the element, there’s a simpler way that’s much more intuitive and readable. Just loop through the elements of the list directly:
If the user inputs an index outside the range of the list (e.g. 6), they’ll run into the list index error again. We can modify the function to check the input value with an if statement:
Doing this prevents our program from crashing if the index is out of range. You can even use a negative index in the above function.
There are other ways to do error handling in Python that will help you avoid errors like “list index out of range”. For example, you could implement a try-exceptaa block instead of the if-else statement.
To see a try-except block in action, let’s handle a potential index error in the get_value() function we wrote above. Preventing the error looks like this:
As you can probably see, the second method is a little more concise and readable. It’s also less error-prone than explicitly checking the input index with an if-else statement.
Master the “List index out of range” Error in Python
You should now know what the index out of range error in Python means, why it pops up, and how to prevent it in your Python programs.
A useful way to debug this error and understand how your programs are running is simply to print the index and compare it to the length of your list.
This error could also occur when iterating over other data structures, such as arrays, tuples, or even when iterating through a string. Using strings is a little different from using lists; if you want to learn the tools to master this topic, consider taking our Working with Strings in Python course. The skills you learnt here should be applicable to many common use cases.
You may also like
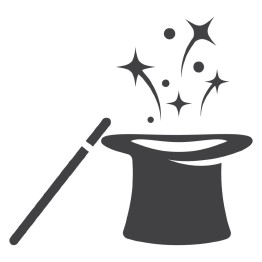
How Do You Write a SELECT Statement in SQL?
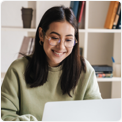
What Is a Foreign Key in SQL?

Enumerate and Explain All the Basic Elements of an SQL Query

- Documentation
- System Status
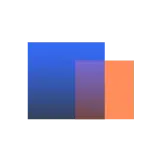
- Rollbar Academy
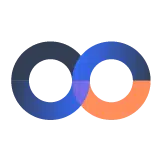
- Software Development
- Engineering Management
- Platform/Ops
- Customer Support
- Software Agency
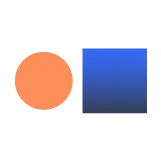
- Low-Risk Release
- Production Code Quality
- DevOps Bridge
- Effective Testing & QA
How to Fix “IndexError: List Assignment Index Out of Range” in Python
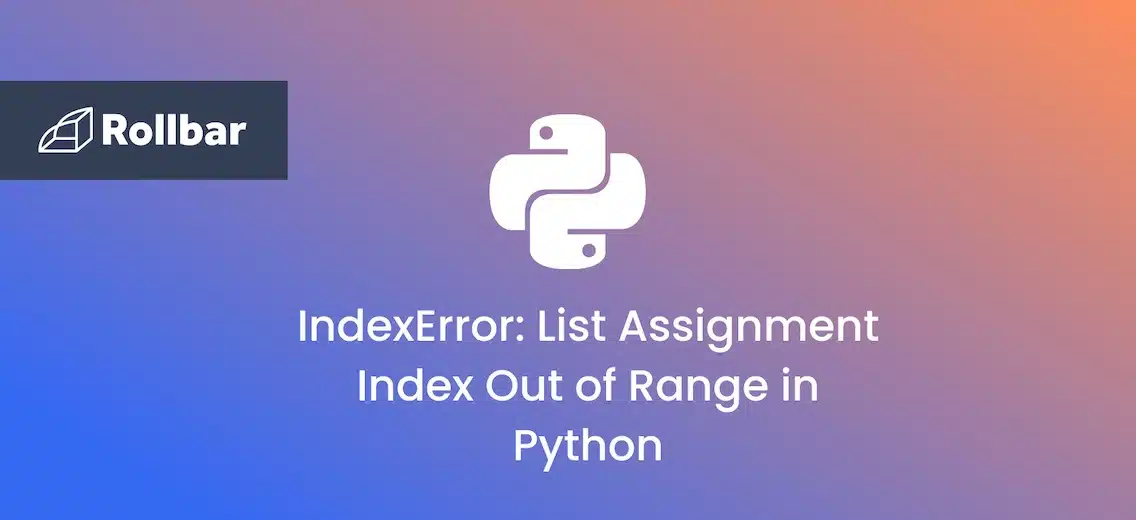
Table of Contents
The IndexError: List Assignment Index Out of Range error occurs when you assign a value to an index that is beyond the valid range of indices in the list. As Python uses zero-based indexing, when you try to access an element at an index less than 0 or greater than or equal to the list’s length, you trigger this error.
It’s not as complicated as it sounds. Think of it this way: you have a row of ten mailboxes, numbered from 0 to 9. These mailboxes represent the list in Python. Now, if you try to put a letter into mailbox number 10, which doesn't exist, you'll face a problem. Similarly, if you try to put a letter into any negative number mailbox, you'll face the same issue because those mailboxes don't exist either.
The IndexError: List Assignment Index Out of Range error in Python is like trying to put a letter into a mailbox that doesn't exist in our row of mailboxes. Just as you can't access a non-existent mailbox, you can't assign a value to an index in a list that doesn't exist.
Let’s take a look at example code that raises this error and some strategies to prevent it from occurring in the first place.
Example of “IndexError: List Assignment Index Out of Range”
Remember, assigning a value at an index that is negative or out of bounds of the valid range of indices of the list raises the error.
How to resolve “IndexError: List Assignment Index Out of Range”
You can use methods such as append() or insert() to insert a new element into the list.
How to use the append() method
Use the append() method to add elements to extend the list properly and avoid out-of-range assignments.
How to use the insert() method
Use the insert() method to insert elements at a specific position instead of direct assignment to avoid out-of-range assignments.
Now one big advantage of using insert() is even if you specify an index position which is way out of range it won’t give any error and it will just append the element at the end of the list.
Track, Analyze and Manage Errors With Rollbar
Managing errors and exceptions in your code is challenging. It can make deploying production code an unnerving experience. Being able to track, analyze, and manage errors in real-time can help you proceed with more confidence. Rollbar automates error monitoring and triaging, making fixing Python errors easier than ever. Try it today !
Related Resources
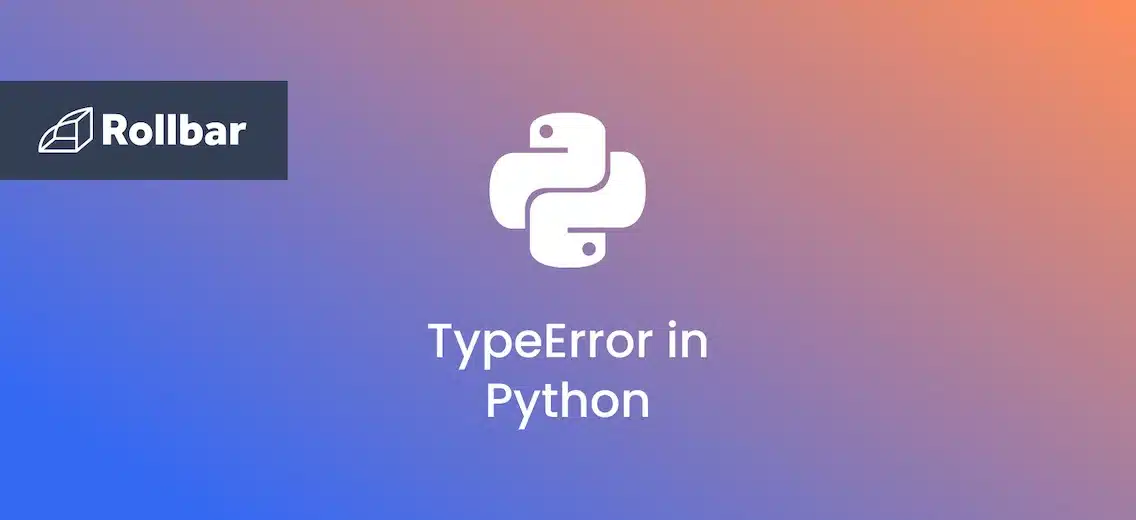
How to Handle TypeError: Cannot Unpack Non-iterable Nonetype Objects in Python
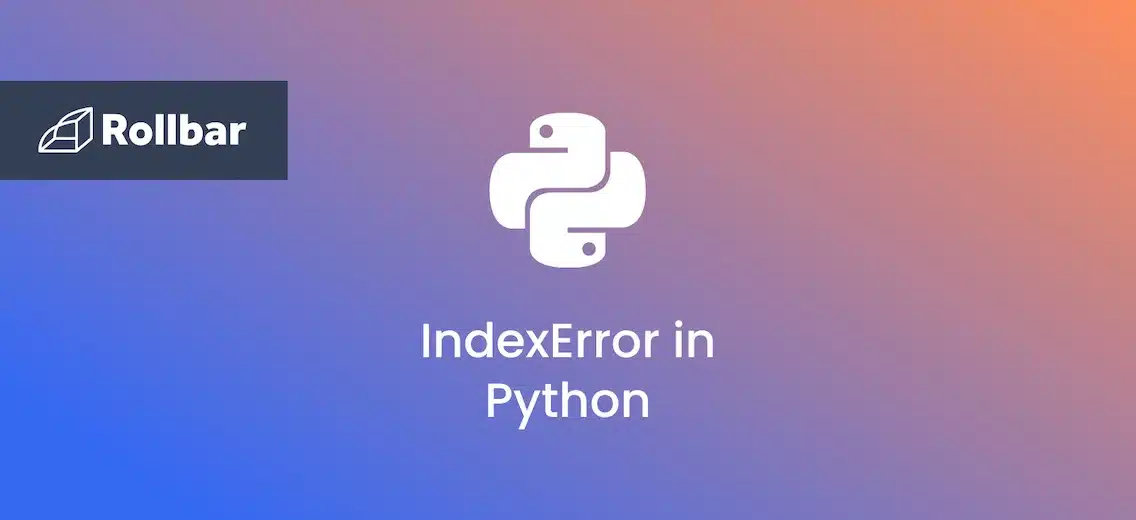
How to Fix IndexError: string index out of range in Python
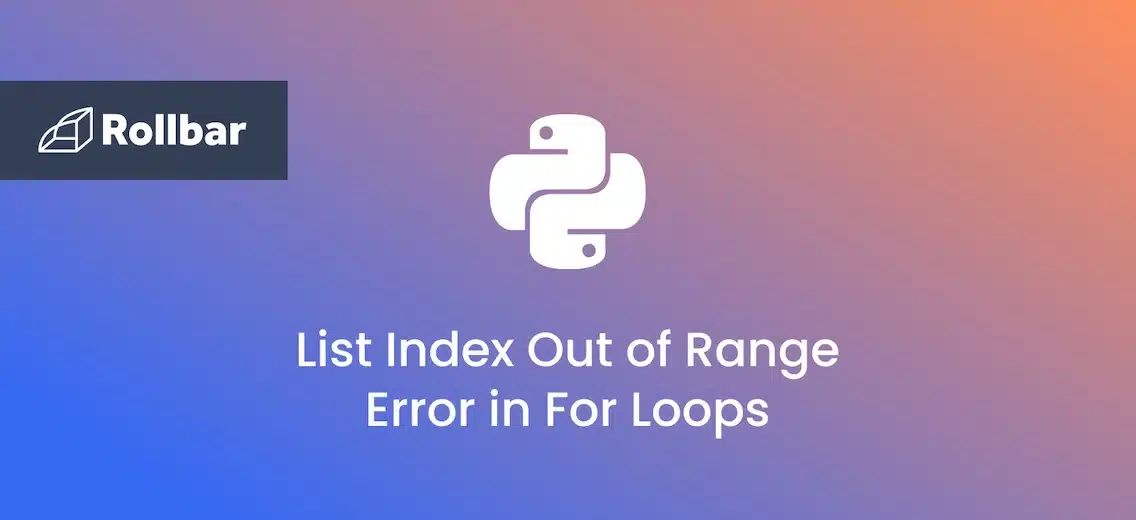
How to Fix Python’s “List Index Out of Range” Error in For Loops
"Rollbar allows us to go from alerting to impact analysis and resolution in a matter of minutes. Without it we would be flying blind."
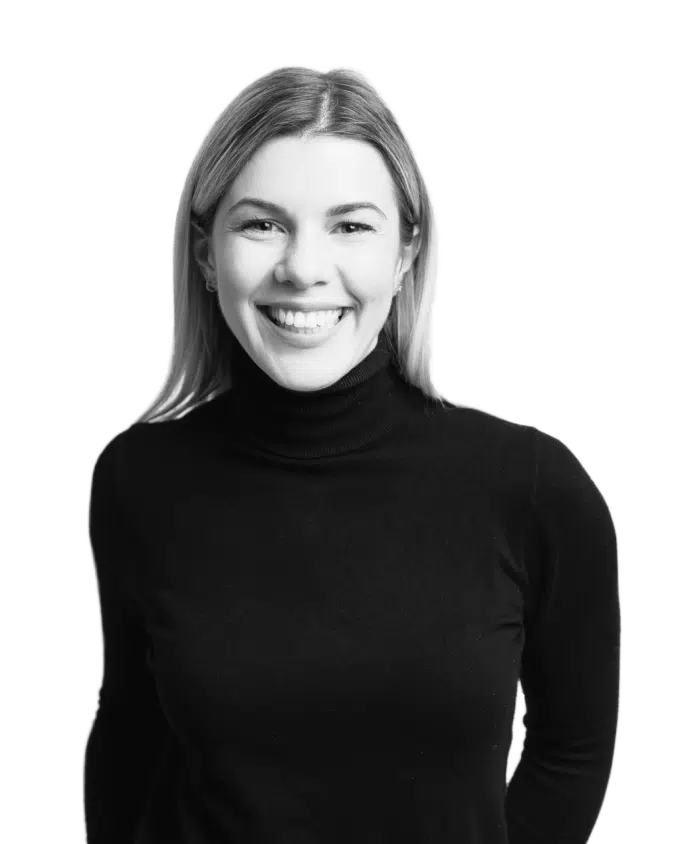
Start continuously improving your code today.
How to Fix Python IndexError: list assignment index out of range
- Python How-To's
- How to Fix Python IndexError: list …
Python IndexError: list assignment index out of range
Fix the indexerror: list assignment index out of range in python, fix indexerror: list assignment index out of range using append() function, fix indexerror: list assignment index out of range using insert() function.
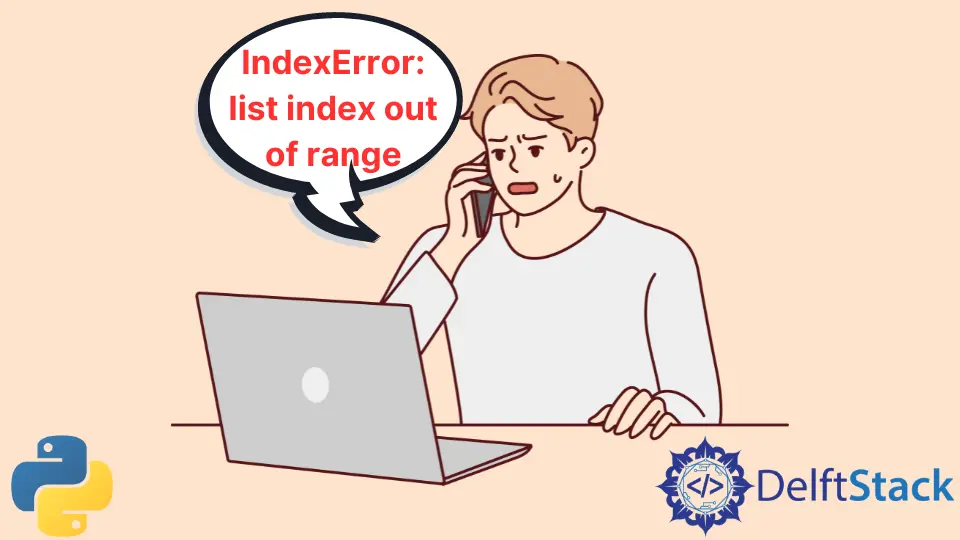
In Python, the IndexError: list assignment index out of range is raised when you try to access an index of a list that doesn’t even exist. An index is the location of values inside an iterable such as a string, list, or array.
In this article, we’ll learn how to fix the Index Error list assignment index out-of-range error in Python.
Let’s see an example of the error to understand and solve it.
Code Example:
The reason behind the IndexError: list assignment index out of range in the above code is that we’re trying to access the value at the index 3 , which is not available in list j .
To fix this error, we need to adjust the indexing of iterables in this case list. Let’s say we have two lists, and you want to replace list a with list b .
You cannot assign values to list b because the length of it is 0 , and you are trying to add values at kth index b[k] = I , so it is raising the Index Error. You can fix it using the append() and insert() .
The append() function adds items (values, strings, objects, etc.) at the end of the list. It is helpful because you don’t have to manage the index headache.
The insert() function can directly insert values to the k'th position in the list. It takes two arguments, insert(index, value) .
In addition to the above two solutions, if you want to treat Python lists like normal arrays in other languages, you can pre-defined your list size with None values.
Once you have defined your list with dummy values None , you can use it accordingly.
There could be a few more manual techniques and logic to handle the IndexError: list assignment index out of range in Python. This article overviews the two common list functions that help us handle the Index Error in Python while replacing two lists.
We have also discussed an alternative solution to pre-defined the list and treat it as an array similar to the arrays of other programming languages.
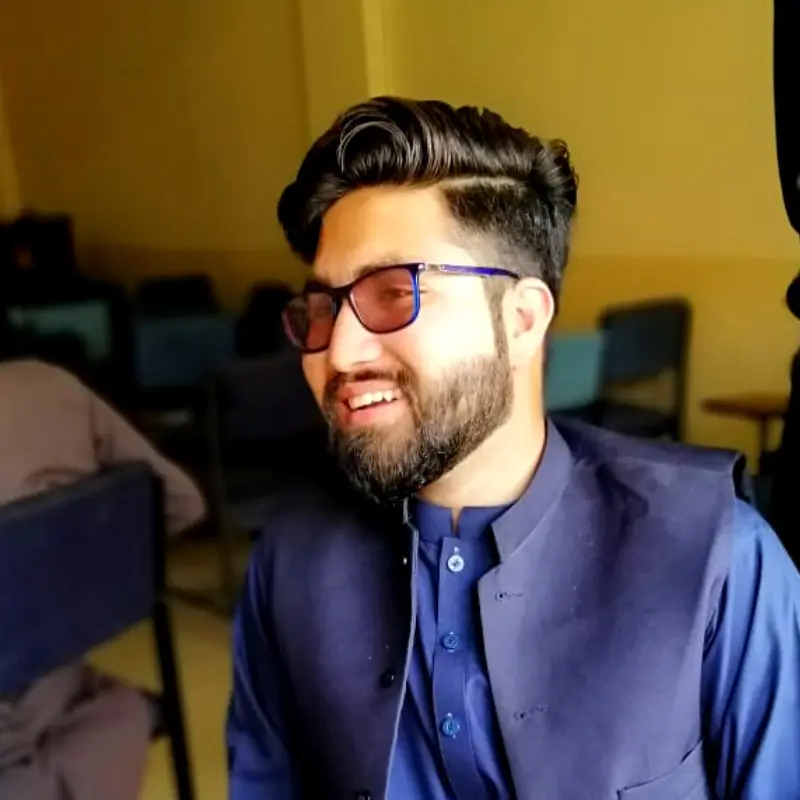
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python
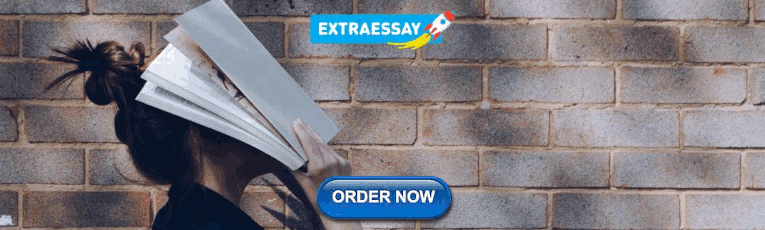
IMAGES
VIDEO
COMMENTS
The reason for the error is that you're trying, as the error message says, to access a portion of the list that is currently out of range. For instance, assume you're creating a list of 10 people, and you try to specify who the 11th person on that list is going to be.
An IndexError is a common error that occurs when you try to access an element in a list, tuple, or other sequence using an index that is out of range. It means that the index you provided is either negative or greater than or equal to the length of the sequence.
Python Indexerror: list assignment index out of range Solution In python, lists are mutable as the elements of a list can be modified. But if you try to modify a value whose index is greater than or equal to the length of the list then you will encounter an Indexerror: list assignment index out of range.
When your list is empty in python you can not assign value to unassigned index of list. so you have 2 options here: Use append like : list.append(value) make a loop to assign a value to your list before your main for. Like below: i = 0 while ( i < index_you_want): list[i] = 0 ... #here your main code
The Python "IndexError: list assignment index out of range" occurs when we try to assign a value at an index that doesn't exist in the list. To solve the error, use the append() method to add an item to the end of the list, e.g. my_list.append('b') .
The “indexerror: list assignment index out of range” is raised when you try to assign an item to an index position that does not exist. To solve this error, you can use append() to add an item to a list. You can also initialize a list before you start inserting values to avoid this error.
You should now know what the index out of range error in Python means, why it pops up, and how to prevent it in your Python programs. A useful way to debug this error and understand how your programs are running is simply to print the index and compare it to the length of your list.
The IndexError: List Assignment Index Out of Range error in Python is like trying to put a letter into a mailbox that doesn't exist in our row of mailboxes. Just as you can't access a non-existent mailbox, you can't assign a value to an index in a list that doesn't exist.
IndexError: list assignment index out of range. The error occurs because the index number [2] doesn’t exist in the animals list. Index assignment in a list only allows you to change existing items. Because the list has two items, the index number ranges from 0 to 1.
In Python, the IndexError: list assignment index out of range is raised when you try to access an index of a list that doesn’t even exist. An index is the location of values inside an iterable such as a string, list, or array. In this article, we’ll learn how to fix the Index Error list assignment index out-of-range error in Python. Python ...