JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript assignment, javascript assignment operators.
Assignment operators assign values to JavaScript variables.
Operator | Example | Same As |
---|---|---|
= | x = y | x = y |
+= | x += y | x = x + y |
-= | x -= y | x = x - y |
*= | x *= y | x = x * y |
/= | x /= y | x = x / y |
%= | x %= y | x = x % y |
**= | x **= y | x = x ** y |
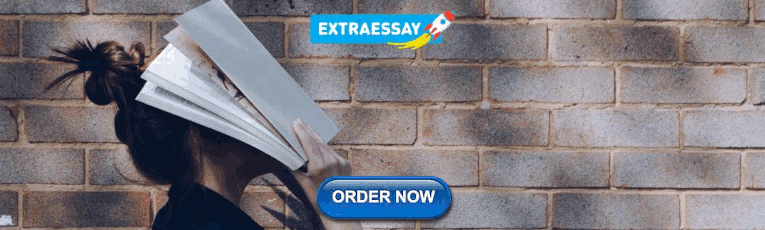
Shift Assignment Operators
Operator | Example | Same As |
---|---|---|
<<= | x <<= y | x = x << y |
>>= | x >>= y | x = x >> y |
>>>= | x >>>= y | x = x >>> y |
Bitwise Assignment Operators
Operator | Example | Same As |
---|---|---|
&= | x &= y | x = x & y |
^= | x ^= y | x = x ^ y |
|= | x |= y | x = x | y |
Logical Assignment Operators
Operator | Example | Same As |
---|---|---|
&&= | x &&= y | x = x && (x = y) |
||= | x ||= y | x = x || (x = y) |
??= | x ??= y | x = x ?? (x = y) |
The = Operator
The Simple Assignment Operator assigns a value to a variable.
Simple Assignment Examples
The += operator.
The Addition Assignment Operator adds a value to a variable.
Addition Assignment Examples
The -= operator.
The Subtraction Assignment Operator subtracts a value from a variable.
Subtraction Assignment Example
The *= operator.
The Multiplication Assignment Operator multiplies a variable.
Multiplication Assignment Example
The **= operator.
The Exponentiation Assignment Operator raises a variable to the power of the operand.
Exponentiation Assignment Example
The /= operator.
The Division Assignment Operator divides a variable.
Division Assignment Example
The %= operator.
The Remainder Assignment Operator assigns a remainder to a variable.
Remainder Assignment Example
Advertisement
The <<= Operator
The Left Shift Assignment Operator left shifts a variable.
Left Shift Assignment Example
The >>= operator.
The Right Shift Assignment Operator right shifts a variable (signed).
Right Shift Assignment Example
The >>>= operator.
The Unsigned Right Shift Assignment Operator right shifts a variable (unsigned).
Unsigned Right Shift Assignment Example
The &= operator.
The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
Bitwise AND Assignment Example
The |= operator.
The Bitwise OR Assignment Operator does a bitwise OR operation on two operands and assigns the result to the variable.
Bitwise OR Assignment Example
The ^= operator.
The Bitwise XOR Assignment Operator does a bitwise XOR operation on two operands and assigns the result to the variable.
Bitwise XOR Assignment Example
The &&= operator.
The Logical AND assignment operator is used between two values.
If the first value is true, the second value is assigned.
Logical AND Assignment Example
The &&= operator is an ES2020 feature .
The ||= Operator
The Logical OR assignment operator is used between two values.
If the first value is false, the second value is assigned.
Logical OR Assignment Example
The ||= operator is an ES2020 feature .
The ??= Operator
The Nullish coalescing assignment operator is used between two values.
If the first value is undefined or null, the second value is assigned.
Nullish Coalescing Assignment Example
The ??= operator is an ES2020 feature .
Test Yourself With Exercises
Use the correct assignment operator that will result in x being 15 (same as x = x + y ).
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Read Tutorial
- Watch Guide Video
- Complete the Exercise
Now that we've talked about operators. Let's talk about something called the compound assignment operator and I'm going make one little change here in case you're wondering if you ever want to have your console take up the entire window you come up to the top right-hand side here you can undock it into a separate window and you can see that it takes up the entire window.
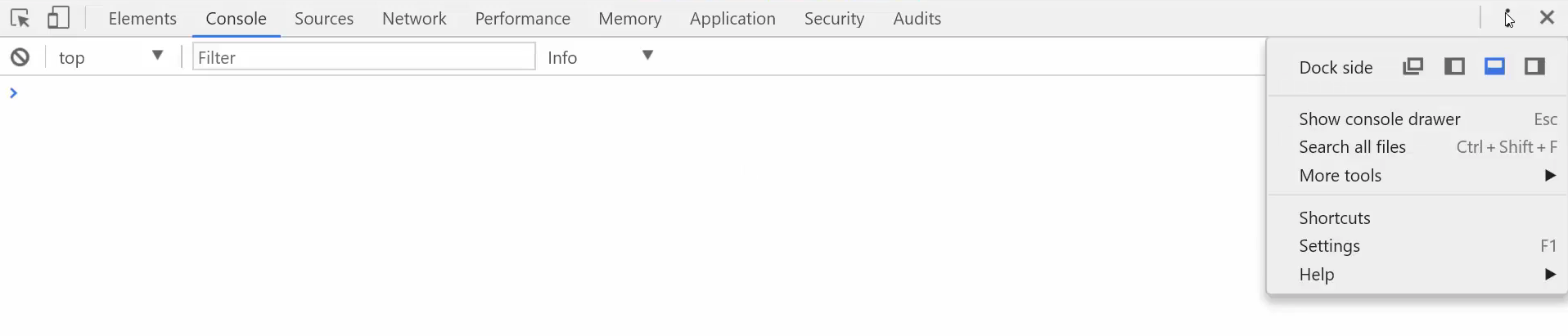
So just a little bit more room now.
Additionally, I have one other thing I'm going to show you in the show notes. I'm going to give you access to this entire set of assignment operators but we'll go through a few examples here. I'm going to use the entire window just to make it a little bit easier to see.
Let's talk about what assignment is. Now we've been using assignment ever since we started writing javascript code. You're probably pretty used to it. Assignment is saying something like var name and then setting up a name
And that is assignment the equals represents assignment.
Now javascript gives us the ability to have the regular assignment but also to have that assignment perform tasks. So for example say that you want to add items up so say that we want to add up a total set of grades to see the total number of scores. I can say var sum and assign it equal to zero.
And now let's create some grades.
I'm going to say var gradeOne = 100.
and then var gradeTwo = 80.
Now with both of these items in place say that we wanted to add these if you wanted to just add both of them together you definitely could do something like sum = (gradeOne + gradeTwo); and that would work.
However, one thing I want to show you is, there are many times where you don't have gradeOne or gradeTwo in a variable. You may have those stored in a database and then you're going to loop through that full set of records. And so you need to be able to add them on the fly. And so that's what a compound assignment operator can do.
Let's use one of the more basic ones which is to have the addition assignment.
Now you can see that sum is equal to 100.
Then if I do
If we had 100 grades we could simply add them just like that.
Essentially what this is equal to is it's a shorthand for saying something like
sum = sum + whatever the next one is say, that we had a gradeThree, it would be the same as doing that. So it's performing assignment, but it also is performing an operation. That's the reason why it's called a compound assignment operator.
Now in addition to having the ability to sum items up, you could also do the same thing with the other operators. In fact literally, every one of the operators that we just went through you can use those in order to do this compound assignment. Say that you wanted to do multiplication you could do sum astrix equals and then gradeTwo and now you can see it equals fourteen thousand four hundred.
This is that was the exact same as doing sum = whatever the value of sum was times gradeTwo. That gives you the exact same type of process so that is how you can use the compound assignment operators. And if you reference the guide that is included in the show notes. You can see that we have them for each one of these from regular equals all the way through using exponents.
Then for right now don't worry about the bottom items. These are getting into much more advanced kinds of fields like bitwise operators and right and left shift assignments. So everything you need to focus on is actually right at the top for how we're going to be doing this. This is something that you will see in a javascript code. I wanted to include it, so when you see it you're not curious about exactly what's happening.
It's a great shorthand syntax for whenever you want to do assignment but also perform an operation at the same time.
- Documentation for Compound Assignment Operators
- Source code
devCamp does not support ancient browsers. Install a modern version for best experience.
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
JavaScript Assignment Operators
A ssignment operators.
Assignment operators are used to assign values to variables in JavaScript.
Assignment Operators List
There are so many assignment operators as shown in the table with the description.
OPERATOR NAME | SHORTHAND OPERATOR | MEANING |
---|---|---|
a+=b | a=a+b | |
a-=b | a=a-b | |
a*=b | a=a*b | |
a/=b | a=a/b | |
a%=b | a=a%b | |
a**=b | a=a**b | |
a<<=b | a=a<<b | |
a>>=b | a=a>>b | |
a&=b | a=a&b | |
a|=b | a=a | b | |
a^=b | a=a^b | |
| a&&=b | x && (x = y) |
| ||= | x || (x = y) |
| ??= | x ?? (x = y) |
Below we have described each operator with an example code:
Addition assignment operator(+=).
The Addition assignment operator adds the value to the right operand to a variable and assigns the result to the variable. Addition or concatenation is possible. In case of concatenation then we use the string as an operand.
Subtraction Assignment Operator(-=)
The Substraction Assignment Operator subtracts the value of the right operand from a variable and assigns the result to the variable.
Multiplication Assignment Operator(*=)
The Multiplication Assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable.
Division Assignment Operator(/=)
The Division Assignment operator divides a variable by the value of the right operand and assigns the result to the variable.
Remainder Assignment Operator(%=)
The Remainder Assignment Operator divides a variable by the value of the right operand and assigns the remainder to the variable.
Exponentiation Assignment Operator
The Exponentiation Assignment Operator raises the value of a variable to the power of the right operand.
Left Shift Assignment Operator(<<=)
This Left Shift Assignment O perator moves the specified amount of bits to the left and assigns the result to the variable.
Right Shift Assignment O perator(>>=)
The Right Shift Assignment Operator moves the specified amount of bits to the right and assigns the result to the variable.
Bitwise AND Assignment Operator(&=)
The Bitwise AND Assignment Operator uses the binary representation of both operands, does a bitwise AND operation on them, and assigns the result to the variable.
Btwise OR Assignment Operator(|=)
The Btwise OR Assignment Operator uses the binary representation of both operands, does a bitwise OR operation on them, and assigns the result to the variable.
Bitwise XOR Assignment Operator(^=)
The Bitwise XOR Assignment Operator uses the binary representation of both operands, does a bitwise XOR operation on them, and assigns the result to the variable.
Logical AND Assignment Operator(&&=)
The Logical AND Assignment assigns the value of y into x only if x is a truthy value.
Logical OR Assignment Operator( ||= )
The Logical OR Assignment Operator is used to assign the value of y to x if the value of x is falsy.
Nullish coalescing Assignment Operator(??=)
The Nullish coalescing Assignment Operator assigns the value of y to x if the value of x is null.
Supported Browsers: The browsers supported by all JavaScript Assignment operators are listed below:
- Google Chrome
- Microsoft Edge
JavaScript Assignment Operators – FAQs
What are assignment operators in javascript.
Assignment operators in JavaScript are used to assign values to variables. The most common assignment operator is the equals sign (=), but there are several other assignment operators that perform an operation and assign the result to a variable in a single step.
What is the basic assignment operator?
The basic assignment operator is =. It assigns the value on the right to the variable on the left.
What are compound assignment operators?
Compound assignment operators combine a basic arithmetic or bitwise operation with assignment. For example, += combines addition and assignment.
What does the += operator do?
The += operator adds the value on the right to the variable on the left and then assigns the result to the variable.
How does the *= operator work?
The *= operator multiplies the variable by the value on the right and assigns the result to the variable.
Can you use assignment operators with strings?
Yes, you can use the += operator to concatenate strings.
What is the difference between = and ==?
The = operator is the assignment operator, used to assign a value to a variable. The == operator is the equality operator, used to compare two values for equality, performing type conversion if necessary.
What does the **= operator do?
The **= operator performs exponentiation (raising to a power) and assigns the result to the variable.
Similar Reads
- Web Technologies
- javascript-operators
Please Login to comment...
Improve your coding skills with practice.
What kind of Experience do you want to share?
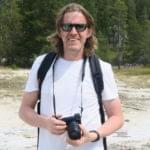
Demystifying JavaScript Operators: What Does That Symbol Mean?
Share this article
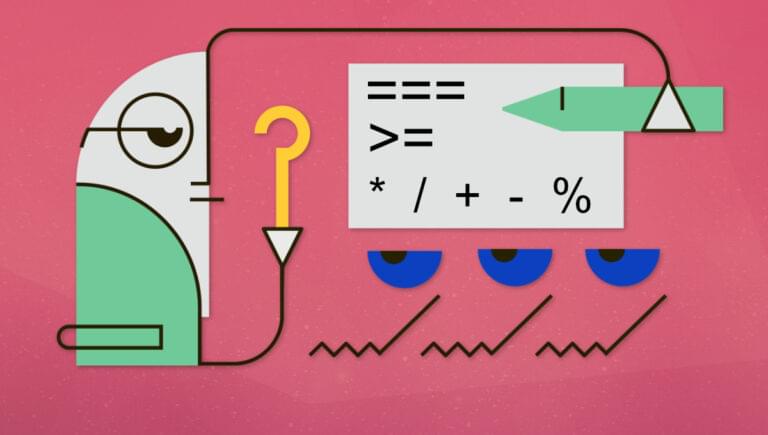
What are JavaScript Operators?
A quick word on terminology, arithmetic operators, assignment operators, comparison operators, logical operators, bitwise operators, other operators, frequently asked questions (faqs) about javascript operators.
In this article, we’re going to examine the operators in JavaScript one by one. We’ll explain their function and demonstrate their usage, helping you to grasp their role in building more complex expressions.
JavaScript, a cornerstone of modern web development, is a robust language full of numerous features and constructs. Among these, operators (special symbols such as + , += , && , or ... ) play an essential role, enabling us to perform different types of calculations and manipulations on variables and values.
Despite their importance, operators can sometimes be a source of confusion for new programmers and seasoned coders alike.
Take a moment to examine this code snippet:
Don’t be alarmed if it seems a bit cryptic. By the time we’re finished, you’ll be able to understand exactly what it does.
Before we dive in, let’s clarify a couple of terms that we’ll be using quite a bit:
An operand is the item that operators work on. If we think of an operator as a kind of action, the operand is what the action is applied to. For example, in the expression 5 + 3, + is the operator (the action of addition), and 5 and 3 are the operands — the numbers being added together. In JavaScript, operands can be of various types, such as numbers, strings, variables, or even more complex expressions.
Coercion is the process of converting a value from one primitive type to another. For example, JavaScript might change a number into a string, or a non-Boolean value into a Boolean. The primitive types in JavaScript are String , Number , BigInt , Boolean , undefined , Symbol or null .
NaN stands for Not a Number . It’s a special value of the Number type that represents an invalid or unrepresentable numeric value.
Truthy values are those that evaluate to true in a Boolean context, while falsy values evaluate to false — with falsy values being false , 0 , -0 , '' , null , undefined , NaN and BigInt(0) . You can read more about truthy and falsy values in Truthy and Falsy Values: When All is Not Equal in JavaScript .
As we explore JavaScript operators, we’ll see these concepts in action and get a better understanding of how they influence the results of our operations.
Arithmetic operators allow us to perform arithmetic operations on values and to transform data. The commonly used arithmetic operators in JavaScript include addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ). Beyond these, we also have the modulus operator ( % ), which returns the remainder of a division operation, and the increment ( ++ ) and decrement ( -- ) operators that modify a value by one.
Addition: +
The addition operator performs two distinct operations: numeric addition and string concatenation. When evaluating expressions using the + operator, JavaScript first coerces both operands to primitive values. Once this is done, it examines the types of both operands.
If one operand is a string, the other operand is also converted to a string, and then the two strings are concatenated. For example:
If both operands are BigInts , BigInt addition is performed. A BigInt is a special numeric type that can deal with numbers larger than the standard Number type can handle.
But if one operand is a BigInt and the other isn’t, JavaScript throws a TypeError :
For all other cases, both operands are converted to numbers, and numeric addition is performed. For example:
Be aware that JavaScript sometimes has a strange idea of what this looks like:
In this case, JavaScript tried to convert the object { a: 1 } to a primitive value, but the best it could do was to convert it to the string [object Object] , which then got concatenated with the number 1.
Subtraction: -
The subtraction operator in JavaScript is straightforward in its usage: it’s used to subtract one number from another. Like the addition operator, it also works with the BigInt type.
If both operands are numbers or can be converted to numbers, JavaScript performs numeric subtraction:
If both operands are BigInts , JavaScript performs BigInt subtraction:
Like the addition operator, subtraction can also produce unexpected results when used with non-numbers. For example, if we try to subtract a something that can’t be converted to a number, JavaScript will return NaN , which stands for “Not a Number”:
Multiplication: *
The multiplication operator works with numbers and BigInts.
Normally, we’ll be multiplying numbers:
If both operands are BigInts , then it performs BigInt multiplication:
As with other operators, JavaScript attempts to convert non-numeric values into numbers. If it can’t do this, it returns NaN :
Division: /
The division operator ( / ) functions with numbers and BigInts , much the same way as + , - and * . It first converts both operands into numbers.
Standard number division:
When dealing with BigInts , the division operator behaves slightly differently. It performs the division and discards any remainder, effectively truncating the result towards zero:
Dividing a number by zero will produce Infinity , unless it’s a BigInt , in which case it throws a RangeError .
If we attempt to divide a number by a value that can’t be converted into a number, JavaScript will usually return NaN .
Modulus (remainder): %
The modulus operator is used to find the remainder after division of one number by another (known as the dividend and the divisor ). This arithmetic operator works with numbers and BigInts .
When we use the % operator, JavaScript first converts the operands to numbers:
This is because three goes into ten three times (making nine), and what’s left over (the remainder) is one.
A common use case for this operator is to check if a number is odd or even:
This uses an arrow function and the triple equals operator that we’ll meet later on.
The modulus operator has some special cases. For example, if one of the operands is NaN , if the dividend is Infinity , or if the divisor is 0 , the operation returns NaN .
On the other hand, if the divisor is Infinity or if the dividend is 0 , the operation returns the dividend.
Increment: ++
The increment operator is used to increase the value of a variable by 1. It can be applied to operands of type Number or BigInt , and its behavior can differ based on whether it’s used in postfix or prefix form.
Postfix increment
If the operator is placed after the operand ( num++ ), the increment operation is performed after the value is returned. In this case, the original value of the variable is used in the current expression, and the variable’s value is incremented afterward:
Prefix increment
If the operator is placed before the operand ( ++num ), the increment operation is performed before the value is returned. In this case, the variable’s value is incremented first, and then the updated value is used in the current expression:
Decrement: --
The decrement operator is used to decrease the value of a variable by 1. Similar to the increment operator, it can be applied to operands of type Number or BigInt . The behavior of the decrement operator can vary based on whether it’s used in postfix or prefix form.
Postfix decrement
When the operator is placed after the operand ( num-- ), the decrement operation is performed after the value is returned. In this case, the original value of the variable is used in the current expression, and the variable’s value is decremented afterward:
Prefix decrement
When the operator is placed before the operand ( --num ), the decrement operation is performed before the value is returned. In this case, the variable’s value is decremented first, and then the updated value is used in the current expression:
The decrement operator, just like the increment operator, can only be used with variables that can be changed:
Miscellaneous arithmetic operators
In addition to the increment and decrement operators, there are other arithmetic operators in JavaScript that we should be aware of.
The unary negation operator ( - ) is used to negate a numeric value, changing its sign to the opposite. For example, -5 would be the negation of the number 5 .
The unary plus operator ( + ) can be used to explicitly convert a value to a number, which can be useful when dealing with string representations of numbers. For example, +'10' converts the string '10' to the number 10 :
The exponentiation operator ( ** ) is used to raise a number to a power. For example, 2 ** 3 represents 2 raised to the power of 3, which results in 8.
It’s also important to note that JavaScript follows operator precedence rules, which determine the order in which operators are evaluated in an expression. For example, multiplication and division have a higher precedence than addition and subtraction, so they are evaluated first:
We can alter the order of evaluation by using the grouping operator () , which is covered in the “ Grouping operator ” section below.
Assignment operators are used to assign values to variables. They also offer a concise and effective way to update the value of a variable based on an expression or other value. In addition to the basic assignment operator ( = ), JavaScript provides compound assignment operators that combine arithmetic or logical operations with assignment.
Assignment: =
This operator is used to assign a value to a variable. It allows us to store a value in a variable so that we can use and reference it later in our code:
The assignment operator assigns the value on the right-hand side of the operator to the variable on the left-hand side.
Additionally, the = operator can be chained to assign the same value to multiple variables in a single line:
Addition assignment: +=
The addition assignment operator is a compound operator that performs an operation and assignment in one step. Specifically, it adds the right operand to the left operand and then assigns the result to the left operand:
This operator isn’t limited to numbers. It can also be used for string concatenation:
When the operands aren’t of the same type, JavaScript applies the same rules of type coercion that we saw previously:
Subtraction assignment: -=
The subtraction assignment operator is another compound operator that performs an operation and assignment in one step. It subtracts the right operand from the left operand and then assigns the result to the left operand:
Like other JavaScript operators, -= performs type coercion when the operands aren’t of the same type. If an operand can be converted to a number, JavaScript will do so:
Otherwise, the result is NaN :
Multiplication assignment: *=
The multiplication assignment operator multiplies the left operand by the right operand and then assigns the result back to the left operand:
When we use operands of different types, JavaScript will try to convert non-numeric string operands to numbers:
If the string operand can’t be converted to a number, the result is NaN .
Division assignment: /=
Like its siblings, the division assignment operator performs an operation on the two operands and then assigns the result back to the left operand:
Otherwise, the rules we discussed for the division operator above apply:
Modulus assignment: %=
The modulus assignment operator performs a modulus operation on the two operands and assigns the result to the left operand:
Otherwise, the rules we discussed for the modulus operator apply:
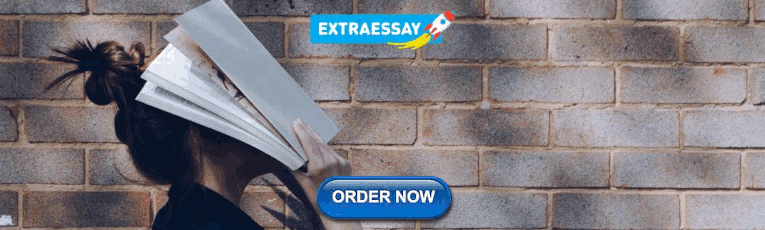
Exponentiation assignment: **=
The exponentiation assignment operator performs exponentiation, where the left operand is the base and the right operand is the exponent, and assigns the result to the left operand:
Here, num is raised to the power of 3, and the result (8) is assigned back to num .
As before, when the second operand is not a number, JavaScript will attempt to convert it with varying degrees of success:
Bitwise assignment operators
While we’ve been focusing on arithmetic operators so far, JavaScript also supports a set of assignment operators that work at the bit level. These are the bitwise assignment operators . If you’re familiar with binary numbers and bit manipulation, these operators will be right up your alley.
These operators include:
- Bitwise AND assignment ( &= )
- Bitwise OR assignment ( |= )
- Bitwise XOR assignment ( ^= )
- Left shift assignment ( <<= )
- Right shift assignment ( >>= )
- Unsigned right shift assignment ( >>>= )
Each of these JavaScript assignment operators performs a specific bitwise operation on the binary representations of the numbers involved and assigns the result back to the left operand.
We’ll explore bitwise operators in more detail in the “ Bitwise Operators ” section below.
Leaving the realm of arithmetic, let’s dive into another significant group of JavaScript operators — the comparison operators. As the name implies, comparison operators are used to compare values and return a Boolean result. Comparison operators are the underpinning of many programming decisions and control structures — from simple condition checks to complex logical operations.
Equality: ==
The equality operator is used to check whether two values are equal to each other. It returns a Boolean result. However, it’s important to note that this comparison operator performs a loose equality check, meaning that if the operands are of different types, JavaScript will try to convert them to a common type before making the comparison:
Things get slightly more complicated dealing with objects and arrays. The == operator checks whether they refer to the same location in memory, not whether their contents are identical:
In this case, JavaScript doesn’t attempt to convert and compare the values within the objects or arrays. Instead, it checks whether they’re the same object (that is, whether they occupy the same memory space).
You can read more about loose equality comparisons here .
Inequality: !=
The inequality operator is used to check whether two values are not equal to each other. Like the == operator, it performs a loose inequality check. This means that, if they’re of different types, it will try to convert the operands to a common type before making the comparison:
Similar to the == operator, when comparing objects and arrays, the != operator checks whether they refer to the same memory location, not whether their content is identical.
Strict equality ( === ) and strict inequality ( !== )
The strict equality and strict inequality comparison operators are similar to their non-strict counterparts ( == and != ), but they don’t perform type coercion. If the operands are of different types, they’re considered different, no matter their values.
Here’s how they work:
For objects and arrays, the strict equality operator behaves the same way as the loose equality operator: it checks whether they refer to the same object, not whether their contents are identical.
NaN is a special case. It’s the only value in JavaScript that isn’t strictly equal to itself:
You can read more about strict equality comparisons here .
Greater than: >
The greater than operator checks if the left operand is greater than the right operand, returning a Boolean result. This comparison is straightforward with numeric values:
When comparing non-numeric values, JavaScript applies a process of conversion to make them comparable. If both values are strings, they’re compared based on their corresponding positions in the Unicode character set:
If one or both of the operands aren’t strings, JavaScript tries to convert them to numeric values for the comparison:
Certain special rules apply for specific values during this conversion. For instance, null is converted to 0 , undefined is converted to NaN , and Boolean values true and false are converted to 1 and 0 respectively. However, if either value is NaN after conversion, the operator will always return false :
Less than: <
The less than operator returns true if the left operand is less than the right operand, and false otherwise. The same rules apply as for the greater than operator; only the order of operands is reversed:
Like the > operator, the < operator uses coercion to convert operands to a common type before making the comparison.
Greater than or equal to ( >= ) and less than or equal to ( <= )
The greater than or equal to ( >= ) and less than or equal to ( <= ) operators function similarly to their < and > counterparts, with the added condition of equality.
For the >= operator, it returns true if the left operand is greater than or equal to the right operand, and false otherwise. Conversely, the <= operator returns true if the left operand is less than or equal to the right operand, and false otherwise. Coercion rules and type conversion, as explained in the sections on < and > directly above, apply here as well:
Logical operators in JavaScript offer a way to work with multiple conditions simultaneously. They’re an integral part of decision-making constructs in programming, such as if statements, and for loops.
Mastering logical operators is key for controlling the flow of our code.
Logical AND: &&
When used with Boolean values, the logical AND operator returns true if all conditions are true and false otherwise.
However, with non-Boolean values, it gets more interesting:
- The operator evaluates conditions from left to right.
- If it encounters a value that can be converted to false (known as a falsy value), it stops and returns that value.
- If all values are truthy, it returns the last truthy value.
For example:
The && operator’s ability to return the value of the operands makes it a versatile tool for conditional execution and setting default values. For example, we can use the && operator to execute a function or a block of code only if a certain condition is met:
In this case, renderWelcomeMessage will only be executed if userIsLoggedIn is true . If userIsLoggedIn is false , the operation will stop at userIsLoggedIn and renderWelcomeMessage won’t be called. This pattern is often used with React to conditionally render components.
Logical OR: ||
When used with Boolean values, the logical OR operator returns true if at least one condition is true and false otherwise.
It can also return non-Boolean values, performing what’s known as short-circuit evaluation . It evaluates conditions from left to right, stopping and returning the value of the first truthy condition encountered. If all conditions are falsy, it returns the last falsy value:
Logical NOT: !
The logical NOT operator is used to reverse the Boolean value of a condition or expression. Unlike the && and || operators, the ! operator always returns a Boolean value.
If the condition is truthy (that is, it can be converted to true ), the operator returns false . If the condition is falsy (that is, it can be converted to false ), the operator returns true :
We can use the ! operator twice to convert a value to its Boolean equivalent:
Nullish coalescing operator: ??
The nullish coalescing operator checks whether the value on its left is null or undefined , and if so, it returns the value on the right. Otherwise, it returns the value on the left.
Though similar to the || operator in some respects, the ?? operator differs in its handling of falsy values.
Whereas the || operator returns the right-hand operand if the left-hand operand is any falsy value (such as null , undefined , false , 0 , NaN , '' ), the ?? operator only does so when the left-hand operand is null or undefined :
Setting default values with logical operators
The || and ?? logical operators are useful for setting default values in programs. Here’s an example of doing this with the || operator:
Here’s an example with the ?? operator:
The main difference between these logical operators (as highlighted above) is how they treat falsy values:
Bitwise operators in JavaScript offer a way to perform operations at the binary level, directly manipulating bits in a number’s binary representation. While these operators can be instrumental in specific tasks like data encoding, decoding, and processing, they’re not frequently used in day-to-day JavaScript programming.
In this article, we’ll provide an overview of these operators so you can recognize and understand them, but we won’t delve deeply into their usage given their relatively niche application.
Bitwise AND: &
The bitwise AND operator performs a bitwise AND operation on the binary representations of integers. It returns a new number whose bits are set to 1 if the bits in the same position in both operands are 1. Otherwise, it sets them to 0:
Bitwise OR: |
The bitwise OR operator works similarly to the & operator, but it sets a bit to 1 if at least one of the bits in the same position in the operands is 1:
Bitwise XOR: ^
The bitwise XOR operator is a little different. It sets a bit to 1 only if the bits in the same position in the operands are different (one is 1 and the other is 0):
Bitwise NOT: ~
The bitwise NOT operator ( ~ ) inverts the bits of its operand. It switches 1s to 0s and 0s to 1s in the binary representation of a number:
Note: two’s complement is a method for representing negative integers in binary notation.
Bitwise shift operators: <<, >>, >>>
Bitwise shift operators are used to shift the bits of a binary number to the left or right. In JavaScript, there are three types: left shift ( << ), right shift ( >> ), and zero-fill right shift ( >>> ).
The left shift bitwise operator ( << ) moves bits to the left and fills in with zeros on the right. The right shift operator ( >> ) shifts bits to the right, discarding bits shifted off. The zero-fill right shift operator ( >>> ) also shifts bits to the right but fills in zeros on the left.
These operators are less common in everyday JavaScript coding, but they have uses in more specialized areas like low-level programming, binary data manipulation, and some types of mathematical calculations.
Apart from the commonly used arithmetic, comparison, logical, and bitwise operators, JavaScript offers a variety of unique operators for specific purposes. These include operators for handling conditional logic, managing object properties, controlling the order of operations, and more.
Conditional (ternary) operator: ? :
The conditional ternary operator ( ? : ) is a concise way to make decisions in our JavaScript code. It gets its name from being the only operator that takes three operands. The syntax for this conditional operator is as follows:
The operator works by first evaluating the condition. If the condition is true , it executes the expressionIfTrue , and if it’s false , it executes the expressionIfFalse :
In the code above, the ternary operator checks if age is greater than or equal to 18. Since age is 15, the condition evaluates to false , and 'Minor' is assigned to the status variable.
This operator can be a handy way to write concise if–else statements, but it can also make code more difficult to read if overused or used in complex conditions.
Spread operator: ...
The spread operator ( ... ) allows elements of an iterable (such as an array or a string) to be expanded in places where zero or more arguments or elements are expected. It can be used in function calls, array literals, and object literals:
The spread operator can be a handy tool for creating copies of arrays or objects, concatenating arrays, or passing the elements of an array as arguments to a function.
You can read more about the spread operator in Quick Tip: How to Use the Spread Operator in JavaScript .
Comma operator: ,
The comma operator ( , ) allows multiple expressions to be evaluated in a sequence and returns the result of the last expression. The expressions are evaluated from left to right.
It’s particularly useful when we need to include multiple expressions in a location that only allows for one, such as in the initialization or update sections of a for loop:
In the code above, the comma operator is used to declare and update two variables ( i and j ) in the for loop.
Optional chaining operator: ?.
Optional chaining is a relatively recent addition to JavaScript (as of ES2020) that simplifies the process of accessing deeply nested properties of objects. It helps us to write cleaner and safer code by providing a way to attempt to retrieve a property value without having to explicitly check if each reference in its chain exists:
In the above example, user?.address?.city accesses the city property of user.address if user and user.address both exist. Otherwise, it returns undefined . This approach avoids a common pitfall in JavaScript, where trying to access a property of undefined or null leads to a TypeError :
Before optional chaining, JavaScript developers had to use lengthy conditional logic to avoid such errors:
Pipeline operator: |>
The pipeline operator ( |> ) is intended to improve the readability of code that would otherwise be written with nested function calls. Essentially, it allows us to take the result of one expression and feed it into the next. This is particularly useful when we’re applying a series of transformations to a value:
With the pipeline operator, we’re able rewrite this code like so:
Be aware that, at the time of writing, the pipeline operator is at stage 2 of the ECMAScript proposal process , meaning it’s a draft and is undergoing further iteration and refinement.
Grouping operator: ()
The grouping operator ( () ) in JavaScript is used to change the precedence of evaluation in expressions. This operator doesn’t perform any operations on its value, but it controls the order in which calculations are carried out within an expression.
For example, multiplication and division have higher precedence than addition and subtraction. This means that, in an expression such as 2 + 3 * 4 , the multiplication is done first, resulting in 2 + 12 , and then the addition is performed, giving a result of 14 .
If we want to change the order of operations, we can use the grouping operator. For example, if we want the addition to be done before the multiplication in the previous example, we could write (2 + 3) * 4 . In this case, the addition is performed first, resulting in 5 * 4 , and then the multiplication is performed, giving a result of 20.
The grouping operator allows us to ensure that operations are performed in the order we intend, which can be critical in more complex mathematical or logical expressions.
We’ve spent this article delving into the broad and sometimes complex world of JavaScript operators. These operators enable us to manipulate data, control program flow, and carry out complex computations.
Understanding these operators is not a mere academic exercise; it’s a practical skill that can enhance our ability to write and understand JavaScript.
Remember the confusing code snippet we started with? Let’s revisit that and see if it makes more sense now:
In plain English, this code is finding the maximum of three numbers, x , y , and z .
It does this by using a combination of JavaScript’s ternary operator ( ? : ) and comparison operators ( > ).
Here’s how it works:
- The expression (x > y ? x : y) checks whether x is greater than y .
- If x is greater than y , it returns x ; otherwise, it returns y . In other words, it’s getting the maximum of x and y .
- This result (the maximum of x and y ) is then compared to z with the > operator.
- If the maximum of x and y is greater than z , then second ternary (x > y ? x : y) is evaluated.
- In this ternary, x and y are compared once again and the greater of the two is returned.
- Otherwise, if z is greater than the maximum of x and y , then z is the maximum of the three numbers, and it’s returned.
If you found this explanation confusing, that’s because it is. Nesting ternary operators like this isn’t very readable and the calculation could be better expressed using Math.max :
Nonetheless, understanding how JavaScript operators work is like learning the grammar of a new language. It can be challenging at first, but once we’ve grasped the basics, we’ll be constructing complex sentences (or in our case, code) with ease.
Before I go, if you found this guide helpful and are looking to dive deeper into JavaScript, why not check out Learn to Code with JavaScript over on SitePoint Premium. This book is an ideal starting point for beginners, teaching not just JavaScript — the world’s most popular programming language — but also essential coding techniques that can be applied to other programming languages.
It’s a fun and easy-to-follow guide that will turn you from a novice to a confident coder in no time.
Happy coding!
What are the different types of JavaScript operators and how are they used?
JavaScript operators are symbols that are used to perform operations on operands (values or variables). There are several types of operators in JavaScript, including arithmetic, assignment, comparison, logical, bitwise, string, ternary, and special operators. Each type of operator has a specific function. For example, arithmetic operators are used to perform mathematical operations, while assignment operators are used to assign values to variables. Comparison operators are used to compare two values, and logical operators are used to determine the logic between variables or values.
How does the assignment operator work in JavaScript?
The assignment operator in JavaScript is used to assign values to variables. It is represented by the “=” symbol. For example, if you have a variable named “x” and you want to assign the value “10” to it, you would write “x = 10”. This means that “x” now holds the value “10”. There are also compound assignment operators that perform an operation and an assignment in one step. For example, “x += 5” is the same as “x = x + 5”.
What is the difference between “==” and “===” in JavaScript?
In JavaScript, “==” and “===” are comparison operators, but they work slightly differently. The “==” operator compares the values of two operands for equality, after converting both operands to a common type. On the other hand, the “===” operator, also known as the strict equality operator, compares both the value and the type of the operands. This means that “===” will return false if the operands are of different types, even if their values are the same.
How do logical operators work in JavaScript?
Logical operators in JavaScript are used to determine the logic between variables or values. There are three logical operators: AND (&&), OR (||), and NOT (!). The AND operator returns true if both operands are true, the OR operator returns true if at least one of the operands is true, and the NOT operator returns the opposite of the operand.
What is the purpose of the ternary operator in JavaScript?
The ternary operator in JavaScript is a shorthand way of writing an if-else statement. It is called the ternary operator because it takes three operands: a condition, a value to return if the condition is true, and a value to return if the condition is false. The syntax is “condition ? value_if_true : value_if_false”. For example, “x = (y > 5) ? 10 : 20” means that if “y” is greater than 5, “x” will be assigned the value 10; otherwise, “x” will be assigned the value 20.
How are bitwise operators used in JavaScript?
Bitwise operators in JavaScript operate on 32-bit binary representations of the number values. They perform bit-by-bit operations on these binary representations. There are several bitwise operators, including AND (&), OR (|), XOR (^), NOT (~), left shift (<<), right shift (>>), and zero-fill right shift (>>>). These operators can be used for tasks such as manipulating individual bits in a number, which can be useful in certain types of programming, such as graphics or cryptography.
What are string operators in JavaScript?
In JavaScript, the plus (+) operator can be used as a string operator. When used with strings, the + operator concatenates (joins together) the strings. For example, “Hello” + ” World” would result in “Hello World”. There is also a compound assignment string operator (+=) that can be used to add a string to an existing string. For example, “text += ‘ World'” would add ” World” to the end of the existing string stored in the “text” variable.
What are special operators in JavaScript?
Special operators in JavaScript include the conditional (ternary) operator, the comma operator, the delete operator, the typeof operator, the void operator, and the in operator. These operators have specific uses. For example, the typeof operator returns a string indicating the type of a variable or value, and the delete operator deletes a property from an object.
How does operator precedence work in JavaScript?
Operator precedence in JavaScript determines the order in which operations are performed when an expression involves multiple operators. Operators with higher precedence are performed before those with lower precedence. For example, in the expression “2 + 3 * 4”, the multiplication is performed first because it has higher precedence than addition, so the result is 14, not 20.
Can you explain how increment and decrement operators work in JavaScript?
Increment (++) and decrement (–) operators in JavaScript are used to increase or decrease a variable’s value by one, respectively. They can be used in a prefix form (++x or –x) or a postfix form (x++ or x–). In the prefix form, the operation is performed before the value is returned, while in the postfix form, the operation is performed after the value is returned. For example, if x is 5, ++x will return 6, but x++ will return 5 (although x will be 6 the next time it is accessed).
Network admin, freelance web developer and editor at SitePoint .
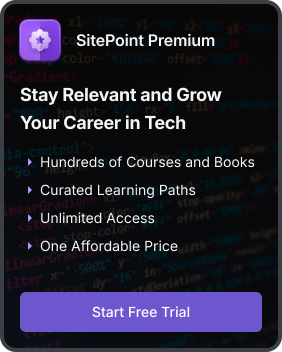
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Remember language
Assignment (=)
Baseline widely available.
This feature is well established and works across many devices and browser versions. It’s been available across browsers since July 2015 .
- See full compatibility
- Report feedback
The assignment ( = ) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
A valid assignment target, including an identifier or a property accessor . It can also be a destructuring assignment pattern .
An expression specifying the value to be assigned to x .
Return value
The value of y .
Thrown in strict mode if assigning to an identifier that is not declared in the scope.
Thrown in strict mode if assigning to a property that is not modifiable .
Description
The assignment operator is completely different from the equals ( = ) sign used as syntactic separators in other locations, which include:
- Initializers of var , let , and const declarations
- Default values of destructuring
- Default parameters
- Initializers of class fields
All these places accept an assignment expression on the right-hand side of the = , so if you have multiple equals signs chained together:
This is equivalent to:
Which means y must be a pre-existing variable, and x is a newly declared const variable. y is assigned the value 5 , and x is initialized with the value of the y = 5 expression, which is also 5 . If y is not a pre-existing variable, a global variable y is implicitly created in non-strict mode , or a ReferenceError is thrown in strict mode. To declare two variables within the same declaration, use:
Simple assignment and chaining
Value of assignment expressions.
The assignment expression itself evaluates to the value of the right-hand side, so you can log the value and assign to a variable at the same time.
Unqualified identifier assignment
The global object sits at the top of the scope chain. When attempting to resolve a name to a value, the scope chain is searched. This means that properties on the global object are conveniently visible from every scope, without having to qualify the names with globalThis. or window. or global. .
Because the global object has a String property ( Object.hasOwn(globalThis, "String") ), you can use the following code:
So the global object will ultimately be searched for unqualified identifiers. You don't have to type globalThis.String ; you can just type the unqualified String . To make this feature more conceptually consistent, assignment to unqualified identifiers will assume you want to create a property with that name on the global object (with globalThis. omitted), if there is no variable of the same name declared in the scope chain.
In strict mode , assignment to an unqualified identifier in strict mode will result in a ReferenceError , to avoid the accidental creation of properties on the global object.
Note that the implication of the above is that, contrary to popular misinformation, JavaScript does not have implicit or undeclared variables. It just conflates the global object with the global scope and allows omitting the global object qualifier during property creation.
Assignment with destructuring
The left-hand side of can also be an assignment pattern. This allows assigning to multiple variables at once.
For more information, see Destructuring assignment .
Specifications
Specification |
---|
Browser compatibility
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators in the JS guide
- Destructuring assignment
- Academy Class

What are compound operators in JavaScript?
In the dynamic landscape of web development, JavaScript stands as a cornerstone, driving interactivity and innovation across the digital realm. As developers strive for more efficient and expressive code, there emerges a powerful tool in their arsenal: compound operators. If you’ve ever found yourself writing repetitive lines of code or seeking ways to enhance your JavaScript proficiency , this blog is your gateway to understanding and harnessing the capabilities of compound operators.
What is a compound operator?
In computer programming, a compound operator is a shorthand notation that combines an arithmetic or bitwise operation with an assignment statement. Instead of writing separate statements for the operation and assignment, a compound operator allows you to perform the operation and assignment in a single step.
What do compound assignment operators do in JavaScript?
Compound assignment operators in JavaScript serve the purpose of combining an assignment operation with another operation, such as arithmetic or bitwise operations. They allow you to perform a calculation and update a variable’s value in a single, concise statement.
What is the additive operator in JavaScript?
In JavaScript, the additive operator refers to the + symbol used for addition. The additive operator can be used for both numerical addition and string concatenation.
Example of using the additive operator in Javascript

Example of concentration with strings

It’s important to note that when the + operator is used with one or more strings, JavaScript treats it as a concatenation operator. If at least one operand is a string, the other operand(s) will be converted to strings, and the concatenation operation will be performed.
What are the 5 compound assignment operators?
The five compound assignment operators in programming languages, including JavaScript, combine an assignment operation with another operation, such as arithmetic or bitwise operations.
Compound Operators
+= compound addition operator, -= compound subtraction operator, %= compound modulo operator, *= compound multiplication operator, /= compound division operator.
These operators combine an assignment and an arithmetic operator. The operators display similar patterns, but, of course, they use their own respective logic and rhythmic symbols. Scroll down for some examples.
What is an Examples of Compound Operators?
In the below example, we are taking the original value of num, which is 5 and we are adding 10 to it, which is 15.

We give num2 a value of 10 and then we use the operator with the value of 10, and, since we’re subtracting, we end up with the value of 0.

We have a variable titled num3 and we give it a value of 50 and then we use the operator with a value of 9 and the remainder is 5 and that’s our output.

We give num4 a value of 4, use the operator with a value of 9 and this outputs a value of 45.

Our operator is num5 with a value of 50, we then use the operator with a value of 25 which outputs 2.

What is the difference between simple and compound assignment operators?
In JavaScript, assignment operators are used to assign values to variables. There are two main types of assignment operators: simple assignment operators and compound assignment operators.
Simple Assignment Operator (=):
The simple assignment operator (=) is used to assign a value to a variable.
It assigns the value on the right-hand side to the variable on the left-hand side.
let x = 10;
let y = “Hello”;
Compound Assignment Operators:
Compound assignment operators combine the assignment (=) with another operation, such as addition, subtraction, multiplication, etc.
They perform the operation and assign the result to the variable on the left-hand side.
a += 3; // Equivalent to: a = a + 3; (Addition)
let b = 10;
b -= 2; // Equivalent to: b = b – 2; (Subtraction)
c *= 4; // Equivalent to: c = c * 4; (Multiplication)
d /= 2; // Equivalent to: d = d / 2; (Division)
Other compound assignment operators include %= (modulus assignment), **= (exponentiation assignment), <<=, >>=, and >>>= (bitwise shift assignments), among others.
The primary difference lies in the combination of an operation with assignment in compound assignment operators. They provide a more concise way to perform an operation and assign the result to a variable, reducing the need for repetitive variable references.
What is the === operator in JavaScript?
The === operator in JavaScript is the strict equality operator. It is used to compare two values for equality without performing type coercion. This means that both the value and the type of the operands must be the same for the === operator to return true.
How do compound operators improve code efficiency in JavaScript?
Compound operators in JavaScript improve code efficiency in the following ways:
Conciseness: Compound operators combine an arithmetic or bitwise operation with an assignment in a single, concise expression. This reduces the number of lines of code required, making it more readable and maintainable. For example, x += 5 is more concise than x = x + 5.
Reduced Redundancy: By combining operations and assignments, compound operators eliminate the need to repeat variable names, reducing redundancy and the risk of typing errors or bugs.
Improved Readability: Concise code with compound operators is generally easier to read and understand at a glance, as the intended operation and assignment are clearly expressed in a single line.
Potential Performance Gains: While the performance impact may be negligible in most cases, reducing the number of lines of code can potentially lead to slight performance improvements, especially in performance-critical applications or tight loops.
Simplified Expressions: Compound operators simplify complex expressions by combining multiple operations into a single line, making the code more readable and easier to maintain.
For example, instead of writing:

You can use the += operator to simplify the accumulation:

This concise and expressive code is easier to read, maintain, and potentially more efficient, especially in performance-critical scenarios.
How are JavaScript Assignment Operators Utilized in Coding and Web Development?
JavaScript assignment operators play a crucial role in coding and web development by providing a concise and efficient way to assign values to variables. These operators not only facilitate the assignment process but also offer the ability to perform mathematical operations during the assignment, streamlining the coding process and enhancing overall efficiency.
One of the key advantages of JavaScript assignment operators is their versatility. They can be used for simple value assignments, where a variable is assigned a specific value. However, their true power lies in their ability to combine assignments with arithmetic operations, such as addition, subtraction, multiplication, division, and even string concatenation.
By leveraging compound assignment operators like +=, -=, *=, /=, and += (for string concatenation), developers can perform calculations and update variable values in a single, concise statement. This not only reduces the number of lines of code required but also improves code readability and maintainability.
For example, instead of writing separate lines for performing an operation and then assigning the result to a variable, a developer can use a compound assignment operator to achieve the same result in a more efficient manner:

This concise approach not only saves time and effort but also minimizes the risk of introducing errors that can arise from redundant variable references or typos.
Moreover, the use of assignment operators in JavaScript extends beyond simple arithmetic operations. They can also be employed in bitwise operations, string manipulations, and even object property assignments, further enhancing their versatility and applicability in web development scenarios.
By embracing the power of JavaScript assignment operators, developers can write more efficient, readable, and maintainable code, ultimately contributing to the creation of robust and high-performance web applications.
In conclusion, the world of compound operators in JavaScript opens up new avenues for streamlined and efficient coding. From simplifying arithmetic to mastering bitwise operations, these shorthand notations seamlessly integrate with assignment statements, enhancing your programming prowess. If you’re interested in the different types of other operators in javascript then why not take a look at our blog “ What are the different javascript operators? ” If you’re ready to delve deeper into JavaScript and take your coding skills to the next level, then why not explore our comprehensive JavaScript training courses at Academy Class with hands-on learning, suitable for both beginners and seasoned developers?
Have a look at our video about JavaScript Operators below!
Want to learn more about javascript book a course below.
- September 2, 2020
Reviews from our Awesome Students

Our Clients

Have Questions about the Course or Need Advice from our Experts?
Contact us today, book a meeting.
Book a meeting with Sharjil, our expert Learning Paths Advisor.
Give Us A Call (United Kingdom)
- +44 800 043 8889
Give Us A Call (United States)
- +1 332 217 1803
“Brilliant training, I learnt so much and very polite and professional instructor. I also wanted to mention how helpful Sharjil was when setting me up for the course.”
Send Us an Email
General Enquiries: office@academyclass.com
Training Enquires: training@academyclass.com
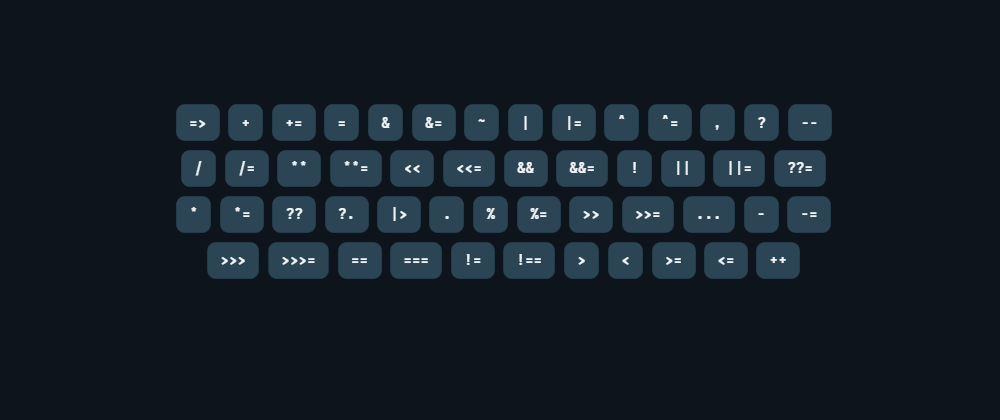
JavaScript expressions and operators handbook
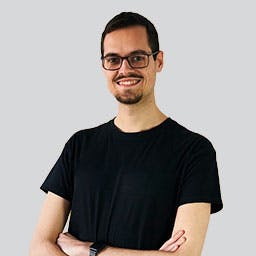
Oct 27, 2022
JavaScript (and therefore, also Node and TypeScript) has a lot of valid operators and expressions that can be overwhelming to learn, specially for newcomers. I will try to sum them all here in a handy and concise way, so this article can be used as a reference for the future, for both experienced and new developers. Be sure that by understanding them, won't be unrealizable task out there for you.
What are expressions and operators?
First what it's first. Let me write down a few paragraphs for the less experienced, that you can ignore if ou already know the difference between those.
As Mozilla writes in their MDN web docs :
At a high level, an expression is a valid unit of code that resolves to a value.
Okay, so now we know what and expression is. But what about operators? To explain that, first you must know that there are two types of expressions: those that have side effects (such as assigning values) and those that purely evaluate. Let's take a look.
Side effects expressions: a = 1 . Here, we are using the = operator to assign the value 1 to the variable a . This is a side effect expression, because it changes the value of the variable a . This expression itself evaluates to the value 1 .
Purely evaluate expressions: a + 1 . This expressions uses the + operator to add the value 1 to the variable a . This is a purely evaluate expression, because it doesn't change the value of the variable a , nor generates any change in our code or program. If a equals 1 from our previous example, this expression is equal to doing 1 + 1 and evaluates itself to the value 2 .
All complex expressions are joined by operators, such as = and + . JavaScript has a lot of operators, that can be classified into multiple categories.
Types of JavaScript operators
There are different types of operators in JavaScript, and I will explain most of them here with examples. However, if you are looking for an specific operator that you don't know it's name or want to learn further details, you can use the marvelous operator lookup tool that Josh Comeau created in his site. Let's go!
Assignment operators
An assignment operator assigns a value to its left operand based on the value of its right operand. The simple assignment operator, as you can imagine, is equal ( = ), but there are also compound assignment operators that are shorthand for some operations. For example:
This not only work for arithmetical operations (including %= and **= ), but also for bitwise operations ( <<= , >>= , >>>= , etc), and boolean operations ( &&= , ||= , ??= ). Don't worry, we will review these type of operations (and their operators) in detail later.
Comparison operators
A comparison operator compares its operands and returns a logical value based on whether the comparison is true.
A note here. If you know about other programming languages, you may notice that JavaScript has a different way of comparing values. In JavaScript, the == and != operators are not the same as === and !== . The first ones are called loose equality and loose inequality , and they are used to compare values that are not of the same type. For example:
In general, when programming with JavaScript or TypeScript, it's considered a good practice to always use the strict equality operators ( === and !== ), unless you especifically want to compare values that are not of the same type.
Arithmetic operators
An arithmetic operator takes numerical values (either literals or variables) as their operands and returns a single numerical value. The standard arithmetic operators are addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ), that are common in most programming languages. However, JavaScript also provided additional arithmetic operators such as the remainder ( % ) and the exponentiation ( ** ) operators, and also the increment ( ++ ) and decrement ( -- ) operators.
Bitwise operators
Bitwise operators treat their operands as a set of 32 bits (zeros and ones in two's complement representation ), perform their operations on such binary representations, and return standard JavaScript numerical values. For example:
There are a subclass of bitwise operators that are used to shift the bits of their first operand the specified number of places to the left or right. For example:
Logical operators
Logical operators are typically used with Boolean (logical) values, like in many other programming languages.
However, the && and || operators actually return the value of one of the specified operands, so if these operators are used with non-Boolean values, they may return a non-Boolean value.
JavaScript unique operators
JavaScript has some unique operators that are not present in other programming languages. These are the ternary operator ( ? ), and the nullish coalescing operator ( ?? ), and the optional chaining operator ( ?. ).
Conditional (ternary) operator
The ternary operator is unique to Javascript in that it requires two separate pseudo-operators, ? and : .
It's used to evaluate a condition, and return a different value depending on whether that condition is truthy (returns the first value) or falsy (returns the second one). It's functionally equivalent to an if/else statement, but because it's an operator, it's usable in an expression. This allows it to be useful in a broader range of situations (eg. within JSX in React).
Nullish coalescing operator
This operator is similar to the Logical OR operator ( || ), except instead of relying on truthy/falsy values, it relies on "nullish" values (there are only 2 nullish values, null and undefined ).
This means it's safer to use when you treat falsy values like 0 as valid.
Similar to Logical OR, it functions as a control-flow operator; it evaluates to the first not-nullish value.
Optional chaining operator
This one is one of my favorites. This operator is similar to the property accessor operator ( . ), it accesses a property on an object.
The difference is that i is safe to chain; if at any point, a nullish value ( null or undefined ) is surfaced, it stops evaluating the expression and returns undefined , avoiding some TypeError .
It will be easier to understand with a example:
Conclusions
As you could see, there are plenty of operators in JavaScript, and they can be used in a variety of ways. Here are not all the possible operators, but the most common ones. If you want to learn more about them, you can check the MDN documentation or the above mentioned operator lookup tool from Josh Comeau.
Happy coding!
I hope my article has helped you, or at least, that you have enjoyed reading it. I do this for fun and I don't need money to keep the blog running. However, if you'd like to show your gratitude, you can pay for my next coffee(s) with a one-time donation of just $1.00. Thank you!
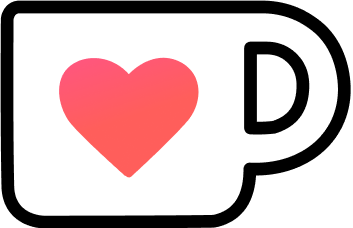
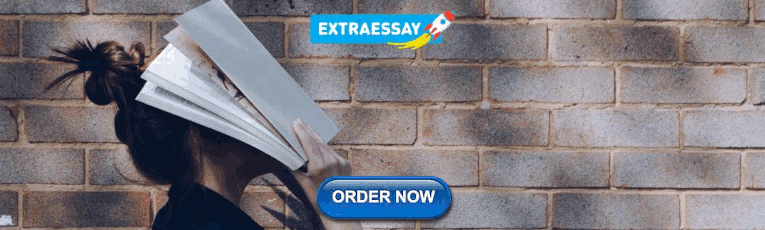
COMMENTS
The simple assignment operator is equal (=), which assigns the value of its right operand to its left operand. That is, x = f() is an assignment expression that assigns the value of f() to x . There are also compound assignment operators that are shorthand for the operations listed in the following table:
Assignment operators assign values to JavaScript variables. Shift Assignment Operators. Bitwise Assignment Operators. Logical Assignment Operators. Note. The Logical assignment operators are ES2020. The = Operator. The Simple Assignment Operator assigns a value to a variable. Simple Assignment Examples. let x = 10; Try it Yourself » let x = 10 + y;
This lesson examines how to work with the Compound Assignment Operator in JavaScript.
What are compound assignment operators? Compound assignment operators combine a basic arithmetic or bitwise operation with assignment. For example, += combines addition and assignment. What does the += operator do? The += operator adds the value on the right to the variable on the left and then assigns the result to the variable.
The addition assignment (+=) operator performs addition (which is either numeric addition or string concatenation) on the two operands and assigns the result to the left operand. x += y is equivalent to x = x + y, except that the expression x is only evaluated once.
In addition to the basic assignment operator (=), JavaScript provides compound assignment operators that combine arithmetic or logical operations with assignment. Assignment: = This...
The assignment (=) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
Compound assignment operators in JavaScript serve the purpose of combining an assignment operation with another operation, such as arithmetic or bitwise operations. They allow you to perform a calculation and update a variable’s value in a single, concise statement.
An assignment operator assigns a value to its left operand based on the value of its right operand. The simple assignment operator, as you can imagine, is equal (=), but there are also compound assignment operators that are shorthand for some operations. For example: let x = 1 // x is now 1 . x += 2 // equals to x + 2. x is now 3 .
In addition to the standard assignment operator, JavaScript has compound assignment operators, which combine an arithmetic operator with =. For example, the addition operator will start with the original value, and add a new value.