Hi, That was valuable information. Thank you, and will consider your suggestions in future. | · | | Last Visit: 31-Dec-99 18:00 Last Update: 10-Sep-24 18:16 | | | Use Ctrl+Left/Right to switch messages, Ctrl+Up/Down to switch threads, Ctrl+Shift+Left/Right to switch pages.   Ternary OperatorAt the end of this lesson, you should be able to answer the following: What is the ternary operator? Where can I use the ternary operator? How do I use the ternary operator in an expression? In Lesson 7 , we learned about the if-else statement that lets us represent a branching path in our programs. In this lesson, we will learn another way to depict a conditional expression. The ternary operator ?: is useful to make quick if-else conditions that evaluates to a result. Line 2 is using a ternary operator expression, in conjunction with a variable declaration. We have declared a variable named evolvedForm . Can you guess what type this variable will be? evolvedForm is a string variable. The inferred value comes from the result of the ternary operator expression. Let's break it down: First is isFemale , followed by a ? . The part that comes before the ? is the condition. isFemale is a boolean variable declared in Line 1, with the value of true . Next is a string value, Nidorina , followed by a : . The part that comes before the : is the result of the whole expression when the condition is true . Finally we have the string value Nidorino after the : . The part that comes after the : is the else clause or alternate path - the result of the expression when the condition is false . In short, the syntax of the ternary operator expression is: Run the code and add a Console.WriteLine(evolvedForm); statement to display the result. Can you guess what the value of evolvedForm will be? How about if isFemale is set to false ? Here's what our code will look like as a regular if-else statement. As we can see, using the ternary operator makes for more concise code! Just like a regular if-else statement, the condition part of the ternary operator (the part before the ? ) can have more complex conditional expressions, like age > 20 . We can even chain ternary expressions. Use this sparingly, however - it can affect the readability of our code. Below, the result of the first ternary expression has been indented so it's easier to read the code. Using the first example in this lesson as a guide, change the if-else statement in the piece of code below to use a ternary expression. Last updated 2 years ago C# Language- Getting started with C# Language
- Learn Tutorial
- How to Start Learning C# While Still in College
- Awesome Book
- Awesome Community
- Awesome Course
- Awesome Tutorial
- Awesome YouTube
- .NET Compiler Platform (Roslyn)
- Access Modifiers
- Access network shared folder with username and password
- Accessing Databases
- Action Filters
- Aliases of built-in types
- An overview of c# collections
- Anonymous types
- ASP.NET Identity
- AssemblyInfo.cs Examples
- Async/await, Backgroundworker, Task and Thread Examples
- Async-Await
- Asynchronous Socket
- BackgroundWorker
- Binary Serialization
- BindingList
- Built-in Types
- C# 3.0 Features
- C# 4.0 Features
- C# 5.0 Features
- C# 6.0 Features
- C# 7.0 Features
- C# Authentication handler
- Checked and Unchecked
- CLSCompliantAttribute
- Code Contracts
- Code Contracts and Assertions
- Collection Initializers
- Comments and regions
- Common String Operations
- Conditional Statements
- Constructors and Finalizers
- Creating a Console Application using a Plain-Text Editor and the C# Compiler (csc.exe)
- Creating Own MessageBox in Windows Form Application
- Creational Design Patterns
- Cryptography (System.Security.Cryptography)
- Data Annotation
- DateTime Methods
- Dependency Injection
- Diagnostics
- Dynamic type
- Equality Operator
- Equals and GetHashCode
- Exception Handling
- Expression Trees
- Extension Methods
- File and Stream I/O
- FileSystemWatcher
- Func delegates
- Function with multiple return values
- Functional Programming
- Garbage Collector in .Net
- Generating Random Numbers in C#
- Generic Lambda Query Builder
- Getting Started: Json with C#
- Handling FormatException when converting string to other types
- Hash Functions
- How to use C# Structs to create a Union type (Similar to C Unions)
- IComparable
- IDisposable interface
- IEnumerable
- ILGenerator
- Immutability
- Implementing Decorator Design Pattern
- Implementing Flyweight Design Pattern
- Import Google Contacts
- Including Font Resources
- Inheritance
- Initializing Properties
- INotifyPropertyChanged interface
- Interoperability
- IQueryable interface
- Lambda expressions
- Lambda Expressions
- LINQ Queries
- Linq to Objects
- LINQ to XML
- Lock Statement
- Making a variable thread safe
- Microsoft.Exchange.WebServices
- Named and Optional Arguments
- Named Arguments
- nameof Operator
- Naming Conventions
- Nullable types
- Null-Coalescing Operator
- Null-conditional Operators
- NullReferenceException
- O(n) Algorithm for circular rotation of an array
- Object initializers
- Object Oriented Programming In C#
- ObservableCollection
- "Exclusive or" Operator
- ? : Ternary Operator
- ?. (Null Conditional Operator)
- ?? Null-Coalescing Operator
- => Lambda operator
- Assignment operator '='
- Binary operators with assignment
- Bit-Shifting Operators
- Class Member Operators: Aggregate Object Indexing
- Class Member Operators: Function Invocation
- Class Member Operators: Member Access
- Class Member Operators: Null Conditional Indexing
- Class Member Operators: Null Conditional Member Access
- default Operator
- Implicit Cast and Explicit Cast Operators
- Overloadable Operators
- Overloading equality operators
- Postfix and Prefix increment and decrement
- Relational Operators
- Short-circuiting Operators
- Overload Resolution
- Parallel LINQ (PLINQ)
- Partial class and methods
- Performing HTTP requests
- Pointers & Unsafe Code
- Polymorphism
- Preprocessor directives
- Reactive Extensions (Rx)
- Read & Understand Stacktraces
- Reading and writing .zip files
- Regex Parsing
- Runtime Compile
- Singleton Implementation
- Static Classes
- Stopwatches
- String Concatenate
- String Escape Sequences
- String Interpolation
- String Manipulation
- String.Format
- StringBuilder
- Structural Design Patterns
- Synchronization Context in Async-Await
- System.DirectoryServices.Protocols.LdapConnection
- System.Management.Automation
- T4 Code Generation
- Task Parallel Library
- Task Parallel Library (TPL) Dataflow Constructs
- Type Conversion
- Unsafe Code in .NET
- Using Directive
- Using json.net
- Using SQLite in C#
- Using Statement
- Value type vs Reference type
- Verbatim Strings
- Windows Communication Foundation
- XDocument and the System.Xml.Linq namespace
- XML Documentation Comments
- XmlDocument and the System.Xml namespace
- Yield Keyword
C# Language Operators ? : Ternary OperatorFastest entity framework extensions. Returns one of two values depending on the value of a Boolean expression. The ternary operator is right-associative which allows for compound ternary expressions to be used. This is done by adding additional ternary equations in either the true or false position of a parent ternary equation. Care should be taken to ensure readability, but this can be useful shorthand in some circumstances. In this example, a compound ternary operation evaluates a clamp function and returns the current value if it's within the range, the min value if it's below the range, or the max value if it's above the range. Ternary operators can also be nested, such as: When writing compound ternary statements, it's common to use parenthesis or indentation to improve readability. The types of expression_if_true and expression_if_false must be identical or there must be an implicit conversion from one to the other. The type and conversion requirements apply to your own classes too. Got any C# Language Question? - Advertise with us
- Cookie Policy
- Privacy Policy
Get monthly updates about new articles, cheatsheets, and tricks. This browser is no longer supported. Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support. Use conditional expression for assignment (IDE0045) Property | Value | | IDE0045 | | Use conditional expression for assignment | | Style | | Language rules (expression-level preferences) | | C# and Visual Basic | | Visual Studio 2017 | | | This style rule concerns the use of a ternary conditional expression versus an if-else statement for assignments that require conditional logic. Options specify the behavior that you want the rule to enforce. For information about configuring options, see Option format . dotnet_style_prefer_conditional_expression_over_assignment Property | Value | Description | | dotnet_style_prefer_conditional_expression_over_assignment | | | | Prefer assignments with a ternary conditional | | | Prefer assignments with an if-else statement | | | | Suppress a warningIf you want to suppress only a single violation, add preprocessor directives to your source file to disable and then re-enable the rule. To disable the rule for a file, folder, or project, set its severity to none in the configuration file . To disable all of the code-style rules, set the severity for the category Style to none in the configuration file . For more information, see How to suppress code analysis warnings . - Use conditional expression for return (IDE0046)
- Code style language rules
- Code style rules reference
Additional resources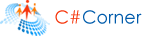 - TECHNOLOGIES
- An Interview Question
 Learn about C# Operators and Their Uses - Dinesh Gabhane
- Feb 26, 2024
- Other Artcile
This article on C# operators from unary and binary operators to overloadable operators, relational operators, implicit and explicit cast operators, short-circuiting operators, the ternary operator, null-conditional operators, "exclusive or" operators, default operators, assignment operators, and sizeof operator. In C#, an operator is a program element that is applied to one or more operands in an expression or statement. Operators that take one operand, such as the increment operator (++) or new, are referred to as unary operators. Operators that take two operands, such as arithmetic operators (+,-,*,/), are referred to as binary operators. One operator, the conditional operator (?:), takes three operands and is the sole ternary operator in C#. Use of C# OperatorsBelow are the operators and their uses 1. Overloadable OperatorsC# allows user-defined types to overload operators by defining static member functions using the operator keyword. The following example illustrates an implementation of the + operator. If we have a Complex class that represents a complex number. And we want to add the option to use the + operator for this class. i.e. We will need to overload the + operator for the class. This is done using a static function and the operator keyword. Operators such as +, -, *, / can all be overloaded. This also includes Operators that don't return the same type (for example, == and != can be overloaded, despite returning booleans) The rule below relating to pairs is also enforced here. Comparison operators have to be overloaded in pairs (e.g. if < is overloaded, > also needs to be overloaded). A full list of overloadable operators (as well as non-overloadable operators and the restrictions placed on some overloadable operators) can be seen at MSDN - Overloadable Operators (C# Programming Guide ). Overloading of the operator was introduced with the pattern-matching mechanism of C# 7.0. For details see Pattern Matching. Given a type Cartesian defined as follows. An overloadable operator could e.g. be defined for Polar coordinates. Which can be used like this. 2. Overloading equality operatorsOverloading just equality operators is not enough. Under different circumstances, all of the following can be called: - object.Equals and object.GetHashCode
- IEquatable<T>.Equals (optional, allows avoiding boxing)
- operator == and operator != (optional, allows using operators)
When overriding Equals, GetHashCode must also be overridden. When implementing Equals, there are many special cases: comparing to objects of a different type, comparing to self, etc. When NOT overridden Equals method and == operator behave differently for classes and structs. For classes just references are compared, and for structs values of properties are compared via reflection which can negatively affect performance. == can not be used for comparing structs unless it is overridden. Generally, equality operations must obey the following rules. - Must not throw exceptions.
- Reflexivity: A always equals A (may not be true for NULL values in some systems).
- Transitivity: if A equals B, and B equals C, then A equals C.
- If A equals B, then A and B have equal hash codes.
- Inheritance tree independence: if B and C are instances of Class2 inherited from Class1:Class1.Equals(A, B) must always return the same value as the call to Class2.Equals(A, B).
3. Relational Operators - EqualsCheck whether the supplied operands (arguments) are equal. Unlike Java, the equality comparison operator works natively with strings. The equality comparison operator will work with operands of differing types if an implicit cast exists from one to the other. If no suitable implicit cast exists, you may call an explicit cast or use a method to convert to a compatible type. Unlike Visual Basic.NET, the equality comparison operator is not the same as the equality assignment operator. Not to be confused with the assignment operator (=). For value types, the operator returns true if both operands are equal in value. For reference types, the operator returns true if both operands are equal in reference (not value). An exception is that string objects will be compared with value equality. Check whether the supplied operands are not equal. This operator effectively returns the opposite result to that of the equals (==) operator Greater Than Check whether the first operand is greater than the second operand. Check whether the first operand is less than the second operand. Greater Than Equal To Check whether the first operand is greater than equal to the second operand. Less Than Equal To Check whether the first operand is less than equal to the second operand. 4. Implicit Cast and Explicit Cast OperatorsC# allows user-defined types to control assignment and casting through the use of explicit and implicit keywords. The signature of the method takes the form. The method cannot take any more arguments, nor can it be an instance method. It can, however, access any private members of the type it is defined within. An example of both an implicit and explicit cast. Allowing the following cast syntax. The cast operators can work both ways, going from your type and going to your type. Finally, the as keyword, which can be involved in casting within a type hierarchy, is not valid in this situation. Even after defining either an explicit or implicit cast, you cannot do. It will generate a compilation error. 5. Short-circuiting OperatorsBy definition, the short-circuiting boolean operators will only evaluate the second operand if the first operand can not determine the overall result of the expression. It means that if you are using the && operator as firstCondition && secondCondition it will evaluate secondCondition only when firstCondition is true and of course, the overall result will be true only if both of firstOperand and secondOperand are evaluated to true. This is useful in many scenarios, for example, imagine that you want to check that your list has more than three elements but you also have to check if the list has been initialized to not run into NullReferenceException. You can achieve this as below: mList.Count > 3 will not be checked until myList != null is met. Logical AND && is the short-circuiting counterpart of the standard boolean AND (&) operator. || is the short-circuiting counterpart of the standard boolean OR (|) operator. Example usage 6. Ternary OperatorReturns one of two values depending on the value of a Boolean expression. The ternary operator is right-associative which allows for compound ternary expressions to be used. This is done by adding additional ternary equations in either the true or false position of a parent ternary equation. Care should be taken to ensure readability, but this can be useful shorthand in some circumstances. In this example, a compound ternary operation evaluates a clamp function and returns the current value if it's within the range, the min value if it's below the range, or the max value if it's above the range. Ternary operators can also be nested, such as. When writing compound ternary statements, it's common to use parenthesis or indentation to improve readability. The types of expression_if_true and expression_if_false must be identical or there must be an implicit conversion from one to the other. The type and conversion requirements apply to your own classes too. 7. ?. (Null Conditional Operator)Introduced in C# 6.0, the Null Conditional Operator ?. will immediately return null if the expression on its lefthand side evaluates to null, instead of throwing a NullReferenceException. If its left-hand side evaluates to a nonnull value, it is treated just like a normal. operator. Note that because it might return null, its return type is always a nullable type. That means that a struct or primitive type, is wrapped into a Nullable<T>. This comes in handy when firing events. Normally you would have to wrap the event call in an if statement checking for null and raise the event afterwards, which introduces the possibility of a race condition. Using the Null conditional operator this can be fixed in the following way: 8. "Exclusive or" OperatorThe operator for an "exclusive or" (for short XOR) is: ^ This operator returns true when one, but only one, of the supplied bools is true. 9. default OperatorValue Type (where T: struct) The built-in primitive data types, such as char, int, and float, as well as user-defined types declared with struct, or enum. Their default value is new T(). Reference Type (where T: class) Any class, interface, array, or delegate type. Their default value is null : 10. Assignment operator '='The assignment operator = sets the left-hand operand's value to the value of the right-hand operand, and returns that value. 11. sizeofReturns an int holding the size of a type* in bytes. In an unsafe context, sizeof can be used to return the size of other primitive types and structs. 12. ?? Null-Coalescing OperatorThe Null-Coalescing operator ?? will return the left-hand side when not null. If it is null, it will return the right-hand side. The ?? operator can be chained which allows the removal of if checks. 13. Bit-Shifting OperatorsThe shift operators allow programmers to adjust an integer by shifting all of its bits to the left or the right. The following diagram shows the effect of shifting a value to the left by one digit. Right-Shift 14. Lambda operatorThe => operator has the same precedence as the assignment operator = and is right-associative. It is used to declare lambda expressions and also it is widely used with LINQ Queries. When used in LINQ extensions or queries the type of the objects can usually be skipped as it is inferred by the compiler. The general form of lambda operator is the following. The parameters of the lambda expression are specified before => operator, and the actual expression/statement/block to be executed is to the right of the operator. This operator can be used to easily define delegates, without writing an explicit method. 15. Class Member OperatorsNull Conditional Member Access 16. Class Member OperatorsNull Conditional Indexing 17. Postfix and Prefix increment and decrementPostfix increment X++ will add 1 to x. Postfix decrement X-- will subtract one. ++x is called prefix increment it increments the value of x and then returns x while x++ returns the value of x and then increments. 18. typeofGets System.Type object for a type. To get the run-time type, use the GetType method to obtain the System.Type of the current instance. Operator typeof takes a type name as a parameter, which is specified at compile time. 19. Binary operators with assignmentC# has several operators that can be combined with an = sign to evaluate the result of the operator and then assign the result to the original variable. 20. nameof OperatorReturns a string that represents the unqualified name of a variable, type, or member. The nameof operator was introduced in C# 6.0. It is evaluated at compile-time and the returned string value is inserted inline by the compiler, so it can be used in most cases where the constant string can be used (e.g., the case labels in a switch statement, attributes, etc...). It can be useful in cases like raising & logging exceptions, attributes, MVC Action links, etc... 21. Class Member Operators: Member Access22. class member operators: function invocation, 23. class member operators: aggregate object indexing. 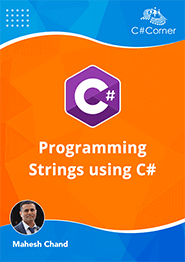 Programming Strings using C#  - Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack OverflowFind centralized, trusted content and collaborate around the technologies you use most. Q&A for work Connect and share knowledge within a single location that is structured and easy to search. Get early access and see previews of new features. Using ternary operator: "only assignment, call, increment..."I have action dictionary defined as: I put there actions like: I'm using this code to run it: It works just fine, but I wanted to use '?' notation to make it shorter: This code shows me error: Error 1 Only assignment, call, increment, decrement, and new object expressions can be used as a statement actions[pair.Key](pair.Value, pair.Key) isn't it a call? Am I missing something? Is it possible to use '?' notation with action dictionaries? I was trying to find something about that but it's hard to find anything about '?' operator and this error because '?' is ignored by google.  - 6 Your not assigning to anything, also is it really any shorter? – Sayse Commented Aug 20, 2013 at 6:37
- 1 @Sayse yes I'm not, but there is that I need to do "assignment, call, increment, decrement ..." and I'm doing call. I prefer this notation, but main point is my curiousity. I really do want to know, what is wrong with my call :) – Kamil Budziewski Commented Aug 20, 2013 at 6:38
- 1 Statement (? : ) is not a call - it is a ternary operator and cannot be used alone - it is the same as to put something like true && false; at new line. – Andrii Kalytiiuk Commented Aug 20, 2013 at 7:05
5 Answers 5Try this instead: This will fix your issue.  - 11 I think this code should not replace the original one. Just because it's one line of code it isn't better. – oddparity Commented Aug 20, 2013 at 7:04
- 4 and why it isn't better my friend? – Kundan Singh Chouhan Commented Aug 20, 2013 at 7:07
- 6 it's better not to use ternar operator because you do not get any speed increase but the readability of the code is worse(standard coding practices) just in case someone takes over your code he will have it easier to read if else then ternar operators – Goran Štuc Commented Aug 20, 2013 at 8:55
- 2 Avoiding ternary operators makes as much sense as avoiding unary operators - avoiding any operators because of their arity doesn't make much sense to me – Damien_The_Unbeliever Commented Aug 20, 2013 at 14:49
- 2 @GoranŠtuc But it doesn't kill readability here (in my opinion). For me the if/else snippet is verbose while the ternary one is concise . This is a subjective matter, of course. – Thomas Commented Aug 22, 2013 at 12:27
The ?: Conditional operator is defined as: The conditional operator (?:) returns one of two values depending on the value of a Boolean expression Your actions don't return a value, so what is the return value of ?: meant to be?  If you realy have to do it that way, you could try Just to clarify, from : Operator (C# Reference) The condition must evaluate to true or false. If condition is true, first_expression is evaluated and becomes the result. If condition is false, second_expression is evaluated and becomes the result. Only one of the two expressions is evaluated. this is equivelant  There's nothing wrong with your call, per se. The expression actions[pair.Key](pair.Value, pair.Key) is indeed a call. That's not the expression the compiler's complaining about, though. The compiler is referring to the entire conditional-operator expression, which is neither an assignment, call, increment, decrement, nor new object expression, and is therefore not allowed to be a statement by itself. Alternatives include the following: - Assigning the result of the expression to another variable, making the conditional merely a sub-expression of the larger assignment statement
- Factoring the conditional into the index expression, so the overall statement is a single call rather than two separate calls.
Using two independent statements to decide which key to use and then call the function: It still avoids duplicating code, but keeps things more easily readable by not trying to pack everything into one complex statement.  every "? :" statement need to assign to something, meaning that isn't legal because it's not assigning anything. you can do: and that would be legal if actions[pair.Key](pair.Value, pair.Key) and actions[""](pair.Value, pair.Key); return a value  - more precise: every ternary expression has to return something – oddparity Commented Aug 20, 2013 at 7:02
Your AnswerReminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more Sign up or log inPost as a guest. Required, but never shown By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy . Not the answer you're looking for? Browse other questions tagged c# .net or ask your own question .- The Overflow Blog
- The evolution of full stack engineers
- One of the best ways to get value for AI coding tools: generating tests
- Featured on Meta
- Bringing clarity to status tag usage on meta sites
- Join Stack Overflow’s CEO and me for the first Stack IRL Community Event in...
- Feedback requested: How do you use tag hover descriptions for curating and do...
- Staging Ground Reviewer Motivation
- What does a new user need in a homepage experience on Stack Overflow?
Hot Network Questions- Paying a parking fine when I don't trust the recipient
- Inspector tells me that the electrician should have removed green screw from the panel
- package accents seems to be incompatible with Unicode-math
- Remove spaces from the 3rd line onwards in a file on linux
- Is the white man at the other side of the Joliba river a historically identifiable person?
- Can someone confirm this observed bug for `bc`? `scale=0` has no effect!
- Somebody used recommendation by an in-law – should I report it?
- What is the shortest viable hmac for non-critical applications?
- How would the following changes affect this monster's CR?
- Has anyone returned from space in a different vehicle from the one they went up in? And if so who was the first?
- A journal has published an AI-generated article under my name. What to do?
- Is the highlighted part false?
- How resiliant is a private key passphase to brute force attacks?
- Why does ATSAM3X8E have two separate registers for setting and clearing bits?
- Geo Nodes: store attribute "line length" for every point in the line
- Using "provide" with value of a variable
- Engaging students in the beauty of mathematics
- How to fold or expand the wingtips on Boeing 777?
- Can we use "day and night time" instead of "day and night"?
- Long table to fit in two pages
- Why does each leg of my 240V outlet measure 125V to ground, but 217V across the hot wires?
- About Jesus's reading of Isaiah in the synagogue
- Why would the GPL be viral, while EUPL isn't, according to the EUPL authors?
- What do you call the act of rebalance an parse tree so simplistic regex are closest to the root node over variables/identifiers?
 - Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
Ternary Operator in ProgrammingThe Ternary Operator is similar to the if-else statement as it follows the same algorithm as of if-else statement but the ternary operator takes less space and helps to write the if-else statements in the shortest way possible. It is known as the ternary operator because it operates on three operands. Table of Content What is a Ternary Operator?- Syntax of Ternary Operator
- Working of Ternary Operator
- Flowchart of Conditional/Ternary Operator
- Ternary Operator in C
- Ternary Operator in C++
- Ternary Operator in Java
- Ternary Operator in Python
- Ternary Operator in C#
- Ternary Operator in JavaScript
- Applications of Ternary Operator
- Best Practices of Ternary Operator
The ternary operator is a conditional operator that takes three operands: a condition, a value to be returned if the condition is true , and a value to be returned if the condition is false . It evaluates the condition and returns one of the two specified values based on whether the condition is true or false. Syntax of Ternary Operator:The Ternary operator can be in the form It can be visualized into an if-else statement as: Working of Ternary Operator :The working of the Ternary operator is as follows: - Step 1: Expression1 is the condition to be evaluated.
- Step 2A: If the condition( Expression1 ) is True then Expression2 will be executed.
- Step 2B : If the condition( Expression1 ) is false then Expression3 will be executed.
- Step 3: Results will be returned
Flowchart of Conditional/Ternary Operator: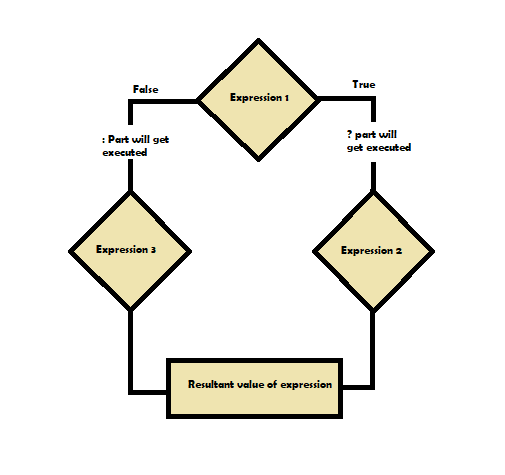 Flowchart of ternary operator Ternary Operator in C:Here are the implementation of Ternary Operator in C language: Ternary Operator in C++:Here are the implementation of Ternary Operator in C++ language: Ternary Operator in Java:Here are the implementation of Ternary Operator in java language: Ternary Operator in Python:Here are the implementation of Ternary Operator in python language: Ternary Operator in C#:Here are the implementation of Ternary Operator in C# language: Ternary Operator in Javascript:Here are the implementation of Ternary Operator in javascript language: Applications of Ternary Operator:- Assigning values based on conditions.
- Returning values from functions based on conditions.
- Printing different messages based on conditions.
- Handling null or default values conditionally.
- Setting properties or attributes conditionally in UI frameworks.
Best Practices of Ternary Operator:- Use ternary operators for simple conditional expressions to improve code readability.
- Avoid nesting ternary operators excessively, as it can reduce code clarity.
- Use parentheses to clarify complex conditions and expressions involving ternary operators.
Conclusion:The conditional operator or ternary operator is generally used when we need a short conditional code such as assigning value to a variable based on the condition. It can be used in bigger conditions but it will make the program very complex and unreadable. Please Login to comment...Similar reads. - Programming
- Best Twitch Extensions for 2024: Top Tools for Viewers and Streamers
- Discord Emojis List 2024: Copy and Paste
- Best Adblockers for Twitch TV: Enjoy Ad-Free Streaming in 2024
- PS4 vs. PS5: Which PlayStation Should You Buy in 2024?
- Full Stack Developer Roadmap [2024 Updated]
Improve your Coding Skills with Practice What kind of Experience do you want to share?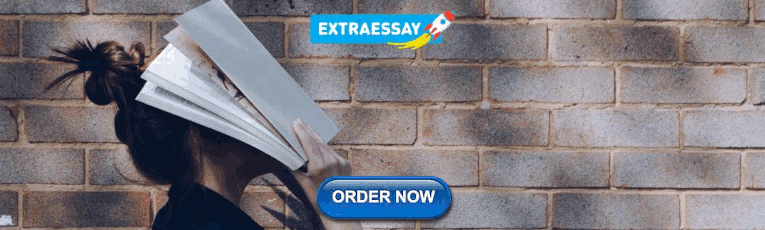 |
COMMENTS
How to use ternary operator in C# - ...
the ternary conditional operator - C# reference
C# ternary (? :) Operator (With Example)
Example: Ternary operator. int x = 10, y = 100; var result = x > y ? "x is greater than y" : "x is less than y"; Console.WriteLine(result); output: x is less than y. Thus, a ternary operator is short form of if else statement. The above example can be re-write using if else condition, as shown below. Example: Ternary operator replaces if statement.
C# Short Hand If...Else (Ternary Operator)
Syntax of C# Ternary Operator. The ternary operator always work with 3 operands: condition_expression has two cases, if condition_expression is true then statement_1 is a return value. However, if condition_expression is false, the operator returns statement_2 . Condition is always a Boolean expression. This operator can replace the if-else ...
Assignment operators (C# reference)
In c#, Ternary Operator (?:) is a decision-making operator, and it is a substitute for the if…else statement in c# programming language. Using Ternary Operator, we can replace multiple lines of if…else statement code into a single line in c# programming language.. The Ternary operator will help you execute the statements based on the defined conditions using the decision-making operator (?:
C# Ternary Operator Offering a Concise and Powerful ...
Really, it's either something that can be used to assign a value, if it's not null, to a variable or an If without an Else packaged nicely in an operator. c# conditional-operator
This tutorial explains the Var, LINQ and Ternary Operator in C# with syntax, usage and programming examples: C# is a strongly typed language i.e. we need to declare a variable before we can use it anywhere in the program. But let's assume a scenario where we don't know what variable type we will be needing in the next step of the program.
Code. C#. void DisplayName( string name) {. //. } Now simply let's assume two names, ' Ahmed ' and ' Jack ', and we are to display the name which has less than 5 characters and pass that name to the above method to be further processed. Using the ternary operator, the approach would be:
The part that comes after the : is the else clause or alternate path - the result of the expression when the condition is false. In short, the syntax of the ternary operator expression is: <condition> ? <result-if-true> : <result-if-false>. Run the code and add a Console.WriteLine(evolvedForm); statement to display the result.
The C# ternary operator tests a condition. It compares 2 values, and it produces a third value that depends on the result of the comparison. Operator notes. The ternary effect can be accomplished with if-statements or other constructs. The ternary operator provides an elegant and equivalent syntax form to the if-statement.
Returns one of two values depending on the value of a Boolean expression. Syntax: Example: Console.WriteLine(name == "Frank" ? "The name is Frank" : "The name is not Frank"); The ternary operator is right-associative which allows for compound ternary expressions to be used. This is done by adding additional ternary equations in either the true ...
The conditional operator, which is a ternary operator (not a unary operator), is not a replacement for an if statement. It is an operator that returns one of two results. While you can chain this to some extent: ... Using C# Ternary Operator. 0. Using the ternary operator in C# for multiple expressions. Hot Network Questions
This style rule concerns the use of a ternary conditional expression versus an if-else statement for assignments that require conditional logic. Options. Options specify the behavior that you want the rule to enforce. For information about configuring options, see Option format. dotnet_style_prefer_conditional_expression_over_assignment
What Is Ternary Operator In C#
One operator, the conditional operator (?:), takes three operands and is the sole ternary operator in C#. Use of C# Operators. Below are the operators and their uses. 1. Overloadable Operators ... C# has several operators that can be combined with an = sign to evaluate the result of the operator and then assign the result to the original variable.
There's nothing wrong with your call, per se. The expression actions[pair.Key](pair.Value, pair.Key) is indeed a call. That's not the expression the compiler's complaining about, though. The compiler is referring to the entire conditional-operator expression, which is neither an assignment, call, increment, decrement, nor new object expression ...
Ternary Operator in Programming