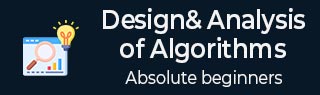
- Design and Analysis of Algorithms
- Basics of Algorithms
- DAA - Introduction to Algorithms
- DAA - Analysis of Algorithms
- DAA - Methodology of Analysis
- DAA - Asymptotic Notations & Apriori Analysis
- DAA - Time Complexity
- DAA - Master's Theorem
- DAA - Space Complexities
- Divide & Conquer
- DAA - Divide & Conquer Algorithm
- DAA - Max-Min Problem
- DAA - Merge Sort Algorithm
- DAA - Binary Search
- DAA - Strassen's Matrix Multiplication
- DAA - Karatsuba Algorithm
- DAA - Towers of Hanoi
- Greedy Algorithms
- DAA - Greedy Algorithms
- DAA - Travelling Salesman Problem
- DAA - Prim's Minimal Spanning Tree
- DAA - Kruskal's Minimal Spanning Tree
- DAA - Dijkstra's Shortest Path Algorithm
- DAA - Map Colouring Algorithm
- DAA - Fractional Knapsack
- DAA - Job Sequencing with Deadline
- DAA - Optimal Merge Pattern
- Dynamic Programming
- DAA - Dynamic Programming
- DAA - Matrix Chain Multiplication
- DAA - Floyd Warshall Algorithm
- DAA - 0-1 Knapsack Problem
- DAA - Longest Common Subsequence Algorithm
- DAA - Travelling Salesman Problem using Dynamic Programming
- Randomized Algorithms
- DAA - Randomized Algorithms
- DAA - Randomized Quick Sort Algorithm
- DAA - Karger's Minimum Cut Algorithm
- DAA - Fisher-Yates Shuffle Algorithm
- Approximation Algorithms
- DAA - Approximation Algorithms
- DAA - Vertex Cover Problem
- DAA - Set Cover Problem
- DAA - Travelling Salesperson Approximation Algorithm
- Sorting Techniques
- DAA - Bubble Sort Algorithm
- DAA - Insertion Sort Algorithm
- DAA - Selection Sort Algorithm
- DAA - Shell Sort Algorithm
- DAA - Heap Sort Algorithm
- DAA - Bucket Sort Algorithm
- DAA - Counting Sort Algorithm
- DAA - Radix Sort Algorithm
- DAA - Quick Sort Algorithm
- Searching Techniques
- DAA - Searching Techniques Introduction
- DAA - Linear Search
- DAA - Interpolation Search
- DAA - Jump Search
- DAA - Exponential Search
- DAA - Fibonacci Search
- DAA - Sublist Search
- DAA - Hash Table
- Graph Theory
- DAA - Shortest Paths
- DAA - Multistage Graph
- DAA - Optimal Cost Binary Search Trees
- Heap Algorithms
- DAA - Binary Heap
- DAA - Insert Method
- DAA - Heapify Method
- DAA - Extract Method
- Complexity Theory
- DAA - Deterministic vs. Nondeterministic Computations
- DAA - Max Cliques
- DAA - Vertex Cover
- DAA - P and NP Class
- DAA - Cook's Theorem
- DAA - NP Hard & NP-Complete Classes
- DAA - Hill Climbing Algorithm
- DAA Useful Resources
- DAA - Quick Guide
- DAA - Useful Resources
- DAA - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary

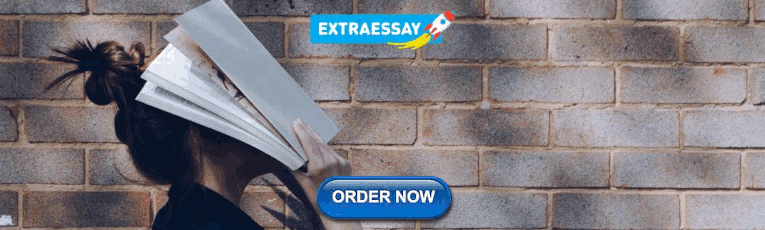
Design and Analysis of Algorithms Tutorial
Daa online compiler or editor, why design and analysis of algorithms, design strategies, analysis of algorithms, applications of daa, who should learn daa, prerequisites to learn daa, daa jobs and opportunities, frequently asked questions about daa.
An Algorithm is a sequence of steps to solve a problem. It acts like a set of instructions on how a program should be executed. Thus, there is no fixed structure of an algorithm. Design and Analysis of Algorithms covers the concepts of designing an algorithm as to solve various problems in computer science and information technology, and also analyse the complexity of these algorithms designed.
The main aim of designing an algorithm is to provide a optimal solution for a problem. Not all problems must have similar type of solutions; an optimal solution for one problem may not be optimal for another. Therefore, we must adopt various strategies to provide feasible solutions for all types of problems.
This tutorial introduces the fundamental concepts of Designing Strategies, Complexity analysis of Algorithms, followed by problems on Graph Theory and Sorting methods. This tutorial also includes the basic concepts on Complexity theory.
In this tutorial, we will provide online compilers and editors to execute programs of all algorithms. The code is written in four different programming languages: C, C++, Java, Python. This will eliminate the need to install a local setup for all these languages.
For instance, let us execute the code for a simple linear search algorithm to work with these online compilers.
One computer problem might have several versions of a solution. In this case, every approach taken to solve the computer problem is correct. However, choosing the best-suited solution will improve the efficiency of the program.
There might be a misconception that smaller algorithms are best-suited solutions in most cases. But, a feasible solution is not based on the length of algorithm, but the one with efficient complexity (time and space complexity).
We study Design and Analysis of Algorithms to analyse the complexity of all the versions of a solution to a computer problem.
There are various types of strategies in order to design algorithms for various problems. Some of them are listed as follows −
- Greedy Approach
- Divide & Conquer Approach
- Dynamic Programming Approach
- Randomization Approach
- Approximation Approach
- Recursive Approach
- Branch and Bound Approach
In this tutorial, we will see various algorithms of each approach to solve various problems.
To analyse the feasibility of an algorithm designed, we calculate the complexity of it. This is represented in three notations, called asymptotic notations. Asymptotic notations are as follows −
- Worst-Case Scenario − Big Oh and Little Oh Notations
- Best-Case Scenario − Big Omega and Little Omega Notations
- Average-Case Scenario − Theta Notation
Each solution is analysed in all scenarios of the problem, and the solution having the best worst-case is said to be optimal. Thus, providing an efficient algorithm.
There are applications of Design and Analysis of Algorithms (DAA) in a wide range of fields. Here are some of the common fields where it is used:
- Computer programming: Used in computer programming to solve problems efficiently. This includes developing algorithms for sorting, searching, and manipulating data structures.
- Big data processing: Also used to develop and analyse algorithms for operations such as data mining, machine learning, and natural language processing, in order to handle large sets of data.
- Networking: Network protocols and algorithms are developed for routing, flow control, congestion control, and network security. DAA is used to design and analyse these algorithms.
- Artificial intelligence: Used to design and analyse algorithms for tasks in Artificial Intelligence such as computer vision, speech recognition, and natural language understanding.
- Scientific computing: DAA in scientific computing is used to develop algorithms for operations like numerical integration, optimization, and simulation.
- Game development: In game development, we use design and analysis of algorithms for pathfinding, collision detection, and physics simulation.
- Cryptography: DAA is also used in the design and analysis of cryptographic algorithms, such as RSA and AES, which are used to secure data transmission and storage.
This tutorial has been designed for students pursuing a degree in any computer science, engineering, and/or information technology related fields. It attempts to help students to grasp the essential concepts involved in algorithm design.
The readers should have basic knowledge of programming and mathematics. The readers should know data structure very well. Moreover, it is preferred if the readers have basic understanding of Formal Language and Automata Theory.
Many top companies are actively recruiting experts in Design and Analysis of Algorithms, and they offer roles such as Software Engineer, Data Scientist, Machine Learning Engineer, and more. These companies need individuals who can solve complex problems, analyse data, and design algorithms to drive their business forward. Here is the list of few such companies −
- JPMorgan Chase
- Goldman Sachs
- Johnson & Johnson
The demand for DAA professionals is continually growing across various sectors. By developing expertise in these areas, you can open up a wide range of career opportunities in some of the world's leading companies.
To get started, there are user-friendly tutorials and resources available to help you master DAA. These materials are designed to prepare you for technical interviews and certification exams, and you can learn at your own pace, anytime and anywhere.
There are many Frequently Asked Questions (FAQs) on Design and Analysis of Algorithms due to the complex nature of this concept. In this section, we will try to answer some of them briefly.
An algorithm is a set of instructions to solve a problem by performing calculations, data processing, or automating reasoning tasks. However, there are always multiple solutions to solving a problem. Design and Analysis of Algorithms provides various ways to design efficient algorithms to solve a problem by analysing their complexities.
Algorithm analysis is an important part of computational complexity theory. The complexity theory provides a theoretical estimation for the required algorithm resources to solve a computational problem. For instance, most algorithms are designed to work with input data of variable length. Analysis of algorithms determines the amount of time and space taken to execute such algorithms.
Here are the summarized list of tips which you can follow to learn Analysis of Algorithms.
- Follow our tutorial step by step from the very beginning.
- Read more articles, watch online courses or buy reference books on Algorithm Analysis to enhance your knowledge.
- Try to design a small algorithm for a simple problem to check your knowledge in these concepts.
As algorithms are not language specific, using any programming language that you are comfortable with is recommended.
Our basic aim while designing an algorithm is to maintain the efficiency of the solution. Algorithms are used in almost all areas of computing. Hence, learning how to design an efficient algorithm is important.
To test the implementation of an algorithm, feed it with diverse types of inputs and observe the outputs generated. If the time taken by the algorithm to be executed and space complexity are efficient even in worst case inputs, your algorithm is feasible.
Time complexity of an algorithm, in general, is simply defined as the time taken by an algorithm to implement each statement in the code. Time complexity can be influenced by various factors like the input size, the methods used and the procedure. An algorithm is said to be the most efficient when the output is produced in the minimal time possible.
Space complexity is a function describing the amount of memory (space) an algorithm takes in terms of the amount of input to the algorithm. So, it is usually computed by combining the auxiliary space and the space used by input values.

Mastering Backtracking: A Comprehensive Guide to Solving Complex Problems in DAA
Learn the general method of backtracking in DAA and how to apply it to solve complex problems. Understand the concept, algorithm, and examples of backtracking.
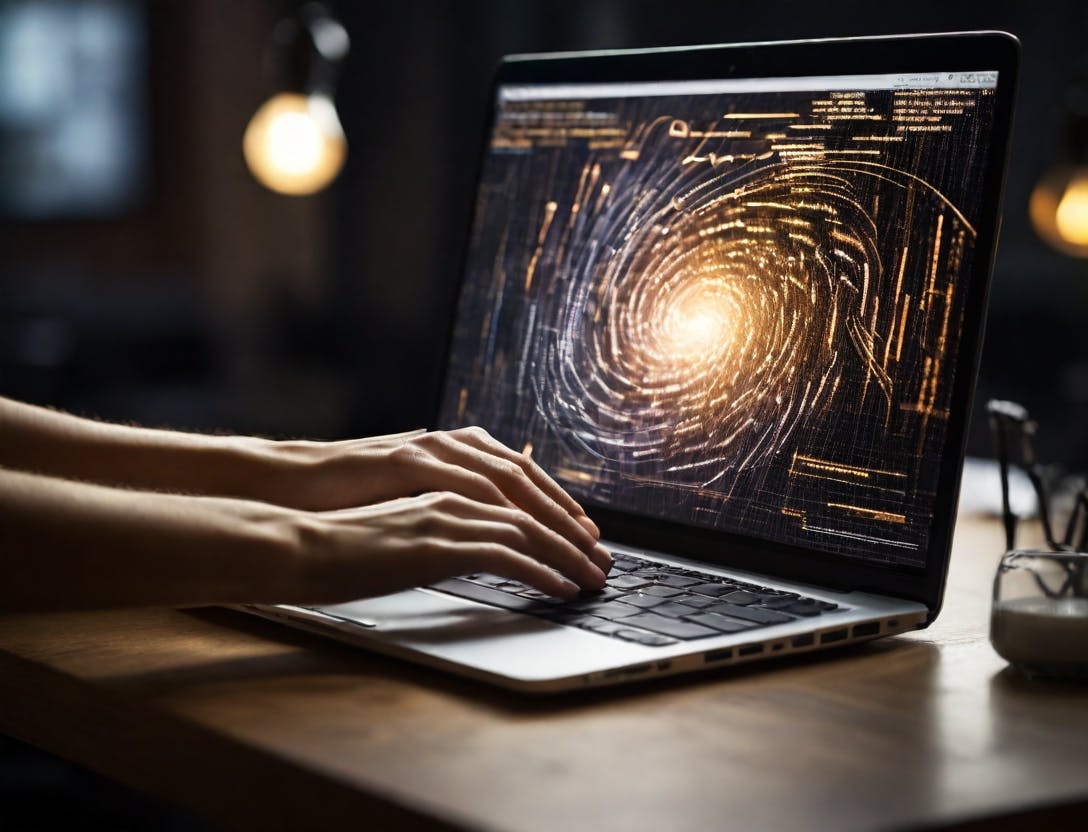
Create an image featuring JavaScript code snippets and interview-related icons or graphics. Use a color scheme of yellows and blues. Include the title '7 Essential JavaScript Interview Questions for Freshers'.
General Method of Backtracking in DAA: A Comprehensive Guide
Backtracking is a fundamental concept in computer science, particularly in the field of Design and Analysis of Algorithms (DAA). It is a powerful technique used to solve complex problems by systematically exploring all possible solutions. In this blog post, we will delve into the general method of backtracking in DAA, its applications, and examples.
What is Backtracking?
Backtracking is a problem-solving strategy that involves recursively exploring all possible solutions to a problem. It is a form of brute force approach that systematically generates all possible solutions and checks if any of them satisfy the problem's constraints. The backtracking algorithm is particularly useful for solving constraint satisfaction problems, where the goal is to find a solution that satisfies a set of constraints.
The General Method of Backtracking
The general method of backtracking involves the following steps:
1. Choose a Variable
Select a variable from the problem's domain. This variable will be used to generate possible solutions.
2. Generate a Possible Solution
Generate a possible solution by assigning a value to the chosen variable.
3. Constraint Checking
Check if the generated solution satisfies the problem's constraints. If it does, then recursively explore the solution space by assigning values to the remaining variables.
4. Backtrack
If the generated solution does not satisfy the constraints, then backtrack by undoing the last assignment and exploring alternative solutions.
Repeat steps 2-4 until a solution is found or all possible solutions have been explored.
Examples of Backtracking Algorithms
1. n-queens problem.
The N-Queens problem is a classic example of a backtracking algorithm. The problem involves placing N queens on an NxN chessboard such that no queen attacks another. The backtracking algorithm works by placing queens one by one on the board, checking for conflicts, and backtracking when a conflict is found.
Sudoku is another popular puzzle that can be solved using backtracking. The algorithm works by filling in the puzzle grid one cell at a time, checking for validity, and backtracking when an invalid solution is found.
3. Traveling Salesman Problem
The Traveling Salesman Problem is a classic problem in computer science that involves finding the shortest possible tour that visits a set of cities and returns to the starting city. The backtracking algorithm works by generating all possible tours and checking for the shortest tour.
Advantages of Backtracking
1. guaranteed solution.
Backtracking guarantees a solution to the problem, provided the problem has a solution.
2. Flexibility
Backtracking can be used to solve a wide range of problems, from simple puzzles to complex optimization problems.
3. Easy to Implement
Backtracking algorithms are relatively easy to implement, especially for small problems.
Disadvantages of Backtracking
1. computational complexity.
Backtracking algorithms can be computationally expensive, especially for large problems.
2. Space Complexity
Backtracking algorithms require a significant amount of memory to store the solution space.
3. Difficulty in Handling Constraints
Backtracking algorithms can struggle to handle complex constraints, leading to inefficient solutions.
Real-World Applications of Backtracking
1. cryptography.
Backtracking is used in cryptography to break certain encryption algorithms.
2. Scheduling
Backtracking is used in scheduling algorithms to find the optimal schedule for a set of tasks.
3. Resource Allocation
Backtracking is used in resource allocation algorithms to allocate resources efficiently.
In conclusion, backtracking is a powerful technique used to solve complex problems in computer science. The general method of backtracking involves choosing a variable, generating a possible solution, constraint checking, backtracking, and repeating the process until a solution is found. Backtracking has numerous advantages, including guaranteed solutions, flexibility, and ease of implementation. However, it also has disadvantages, including high computational complexity, space complexity, and difficulty in handling constraints. Despite these limitations, backtracking has numerous real-world applications in cryptography, scheduling, and resource allocation.

Algorithm Design Techniques in DAA
When it comes to solve problems, especially in computer science, algorithms are something that play a crucial role. An algorithm is a set of rules for solving a certain problem quickly. But the question is how to design an algorithm to solve that problem? So IT professionals are upgrading their knowledge and developing different approaches to construct an effective algorithm to fulfil the expanding demands of industry. Different algorithm design techniques and their applications are discussed in this article.
Brute-Force Search
It is a simple approach of addressing a problem that relies on huge processing power and testing of all possibilities to improve efficiency. Suppose you forgot the combination of a 4-digit padlock and to avoid purchasing the new one, you have to open the lock using brute-force search method. You will have to try all possible 4-digit combinations from 0 to 9 to unlock it. That combination could be anything between 0000 to 9999, hence there are 10,000 combinations. So we can say that in the worst case, you have to try 10, 000 times to find your actual combination.
Brute Force Search for combination of padlock
The time complexity of brute force is O(mn), which can also be written as O(n*m). This means that if we need to search a string of “n” characters in a string of “m” characters, then it would take “n*m” tries.
Divide and Conquer
Divide and conquer algorithm works on top-down approach and is preferred for large problems. As the name says divide and conquer, it follows following steps:
Step 1: Divide the problem into several subproblems.
Step 2: Conquer or solve each sub-problem.
Step 3: Combine each sub-problem to get the required result.
Divide and Conquer solve each subproblem recursively, so each subproblem will be the smaller original problem.
Greedy Algorithm
In Greedy Algorithm, resources are divided in a loop depending on the maximum and immediate availability at any given stage of execution. This method is used to solve optimization problems in which set of input values are given, that are required either to be increased or decreased according to the objective. Greedy Algorithm always chooses the option, which appears to be the best at the moment. That is why it is known as greedy algorithm. It may not always give the optimized solution. There are two stages to solve the problem using greedy algorithm:
- Examining the list of Items.
- Optimization
This means that a greedy algorithm selects the best immediate option and does not rethink its decisions. When it comes to optimizing a solution, this simply implies that the greedy solution will seek out local optimum solutions, which could be multiple, and may skip a global optimal solution. For example, greedy algorithm in animation below aims to locate the path with the largest sum.
With a goal of reaching the largest sum, at each step, the greedy algorithm will choose what appears to be the optimal immediate choice, so it will choose 12 instead of 3 at the second step and will not reach the best solution, which contains 99.
Dynamic Programming
Dynamic Programming (DP) is an algorithmic technique for solving optimization problems by breaking them into simpler sub-problems and storing each sub-solution so that the corresponding sub-problem can be solved only once. Dynamic Programming is a good methodology for optimization problems that seek the maximal or minimal solution with restrictions as it searches through all possible sub-problems and never recomputes the conclusion to any sub-problem.
It’s an algorithmic strategy for breaking down an optimization problem into smaller sub-problems and leveraging the fact that the best solution for the overall problem is defined by the best solution for its sub-problems. For example in case of Fibonacci Series in which each number is the sum of the two preceding numbers. Suppose the first two numbers of the series are 0, 1. If it is asked to find the nth number of the series, we can do that as follows:
Dynamic Programming Example
Here, to solve the overall problem i.e., Fib(n) , we have to break it down into two smaller sub-problems i.e., Fib(n-1) and Fib(n-2). Hence, we can use Dynamic Programming to solve above mentioned problem, which is elaborated in more detail in the following figure:
Fibonacci Series using Dynamic Programming
Branch and Bound Algorithm
For combinatory, discrete, and general mathematical optimization problems, branch and bound algorithms are applied to determine the optimal solution. A branch and bound algorithm searches the set of all possible solutions before recommending the best one. This algorithm enumerates possible candidate solutions in a stepwise manner by exploring all possible set of solutions.
Branch and Bound Algorithm Example
First of all we build a rooted decision tree where the root node represents the entire search space. Each child node is a part of the solution set and is a partial solution. Based on the optimal solution, we set an upper and lower bound for a given problem before constructing the rooted decision tree and we need to make a decision about which node to include in the solution set at each level. It is very important to find upper and lower bound and to find upper bound any local optimization method can be used. It can also be found by picking any point in the search space and convex relaxation. Whereas, duality can be used for finding lower bound.
Randomized Algorithm
Randomized Algorithm refers to an algorithm that uses random numbers to determine what to do next at any point in its logic. In a standard algorithm, it is usually used to reduce either the running time, or time complexity, or the memory used, or space complexity. The algorithm works by creating a random number, r, from a set of numbers and making decisions based on its value. This algorithm could assist in making a decision in a situation of doubt by flipping a coin or drawing a card from a deck.
Randomized Algorithm Flowchart
When utilizing a randomized method, keep the following two considerations in mind:
- It takes source of random numbers and makes random choices during execution along with the input.
- Behavior of an algorithm varies even on fixed inputs.
Backtracking Algorithms
Backtracking means that if the current solution isn’t working, you should go back and attempt another option. It is a method for resolving issues recursively by attempting to construct a solution incrementally, one piece at a time, discarding any solutions that do not satisfy the problem’s constraints at any point in time. This approach is used to resolve problems having multiple solutions. For example if we want to find all the possible ways of arranging 2 boys and 1 girl on 3 benches with a constraint that Girl should not be on the middle bench. So there will be 3! = 6 possibilities to solve this problem. We will try all possible ways recursively to get the required solution. Those possibilities are as follows:
Backtracking Example Possibilities
Following diagram shows the possible solutions for the above mentioned problem:
Solutions of Backtracking
Related Posts
Asymptotic Analysis in DAA
Program Performance Measurement
Big O Notation: Definition & Examples
Singly Linked List in Data Structure
Shell Sort in Data Structure
Selection Sort in Data Structure
Add comment cancel reply.

DAA Tutorial: Design and Analysis of Algorithms
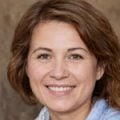
DAA Tutorial Summary
This Design and Analysis of Algorithms Tutorial is designed for beginners with little or no coding experience. It covers algorithm Design and Analysis process concepts.
What is an Algorithm?
An Algorithm is a set of well-defined instructions designed to perform a specific set of tasks. Algorithms are used in Computer science to perform calculations, automatic reasoning, data processing, computations, and problem-solving. Designing an algorithm is important before writing the program code as the algorithm explains the logic even before the code is developed.
DAA Syllabus
Introduction, advanced stuff, why study design and analysis of algorithm.
Design and Analysis of Algorithm help to design the algorithms for solving different types of problems in Computer Science. It also helps to design and analyze the logic on how the program will work before developing the actual code for a program.
Prerequisites for learning DAA Tutorial
For learning this DAA tutorial, you should know the basic programming and mathematics concepts and data structure concepts. The basic knowledge of algorithms will also help you learn and understand the DAA concepts easily and quickly.
What will you learn in this Design and Analysis of Algorithms Tutorial?
In this Design and Analysis of Algorithms tutorial, you will learn the basic concepts about DAA like the introduction to Algorithm, Greedy algorithm, linked list, and arrays in a data structure. You will also learn advanced concepts like Trees in a data structure, search algorithms, sorting algorithms, hash tables, and interview questions related to Algorithms.
DAA (Design and Analysis of Algorithms)
Dcrust syllabus.
CSE 206C Design and Analysis of Algorithms
CATEGORY : ENGINEERING SCIENCE COURSE
Course Objectives:
- To analyze worst-case running times of algorithms based on asymptotic analysis and justify the correctness of algorithms.
- To apply the algorithms and design techniques to solve problems.
- To explain the major graph algorithms and their analyses and to employ graphs to model engineering problems.
- To understand the concepts of tractable and intractable problems and the classes P, NP and NP-complete problems.
Introduction: Characteristics of algorithm. Analysis of algorithm: Asymptotic analysis of complexity bounds – best, average and worst-case behavior; Performance measurements of Algorithm, Time and space trade-offs, Analysis of recursive algorithms through recurrence relations: Substitution method, Recursion tree method and Masters’ theorem.
Click on any topic to read about the topic.
Fundamental Algorithmic Strategies: Brute-Force, Greedy, Dynamic Programming, Branch- and-Bound and Backtracking methodologies for the design of algorithms; Illustrations of these techniques for Problem-Solving, Bin Packing, Knap Sack TSP. Heuristics-characteristics and their application domains.
Graph and Tree Algorithms: Traversal algorithms: Depth First Search (DFS) and Breadth First Search (BFS); Shortest path algorithms, Transitive closure, Minimum Spanning Tree, Topological sorting, Network Flow Algorithm.
Tractable and Intractable Problems: Computability of Algorithms, Computability classes – P, NP, NP-complete and NP-hard. Cook’s theorem, Standard NP-complete problems and Reduction techniques.
Advanced Topics: Approximation algorithms, Randomized algorithms, Class of problems beyond NP – P SPACE
TEXT BOOKS:
- Introduction to Algorithms, 4TH Edition, Thomas H Cormen, MIT Press/McGraw-Hill.Fundamentals of Algorithms – E. Horowitz et al.
REFERENCE BOOKS:
- Algorithm Design, 1ST Edition, Jon Kleinberg and ÉvaTardos, Pearson.
- Algorithm Design: Foundations, Analysis, and Internet Examples, Second Edition, Michael T Goodrich and Roberto Tamassia, Wiley.
- Algorithms — A Creative Approach, 3 RD Edition, UdiManber, Addison-Wesley, Reading, MA.
Course Outcomes: After successful completion of the course students will be able to:
- Analyze worst-case running times of algorithms based on asymptotic analysis and justify the correctness of algorithms.
- Apply the algorithms and design techniques to solve problems;
- Explain the major graph algorithms and their analyses. Employ graphs to model engineering problems,
- Understand the concepts of tractable and intractable problems and the classes P, NP and NP-complete problems.
Related Posts
Aca (advanced computer architecture), ai (artificial intelligence and expert system), aj (advanced java), aj lab (advanced java lab), cd (compiler design), cd lab (compiler design lab), cfcl (cyber forensics and cyber laws), cg (computer graphics), cn (computer networks), coa (computer organisation and architecture, cs (cyber security), daa lab (design and analysis of algorithms lab), dbms (database management systems), dbms lab (database management system lab ), dsa (data structure and algorithms), dsa lab (data structures and algorithms lab), flat (formal languages & automata theory), itw (it workshop) / matlab, mm (multimedia technology), oop (object oriented programming).
- Analysis of Algorithms
- Backtracking
- Dynamic Programming
- Divide and Conquer
- Geometric Algorithms
- Mathematical Algorithms
- Pattern Searching
- Bitwise Algorithms
- Branch & Bound
- Randomized Algorithms
- Learn Data Structures and Algorithms | DSA Tutorial
- Data Structures Tutorial
- Array Data Structure
- String in Data Structure
- Linked List Data Structure
- Stack Data Structure
- Queue Data Structure
- Tree Data Structure
- Heap Data Structure
- Hashing in Data Structure
- Graph Data Structure And Algorithms
- Matrix Data Structure
- Introduction to Set – Data Structure and Algorithm Tutorials
- Introduction to Map – Data Structure and Algorithm Tutorials
- Advanced Data Structures
Algorithm Tutorial
- Searching Algorithms
- Sorting Algorithms
- Recursion Algorithms
- Backtracking Algorithm
- Greedy Algorithms
- Dynamic Programming or DP
- What is Pattern Searching ?
- Divide and Conquer Algorithm
- Branch and Bound Algorithm
Algorithms Tutorial
Competititve programming.
- Competitive Programming - A Complete Guide
Algorithm is a step-by-step procedure for solving a problem or accomplishing a task. In the context of data structures and algorithms, it is a set of well-defined instructions for performing a specific computational task. Algorithms are fundamental to computer science and play a very important role in designing efficient solutions for various problems. Understanding algorithms is essential for anyone interested in mastering data structures and algorithms.
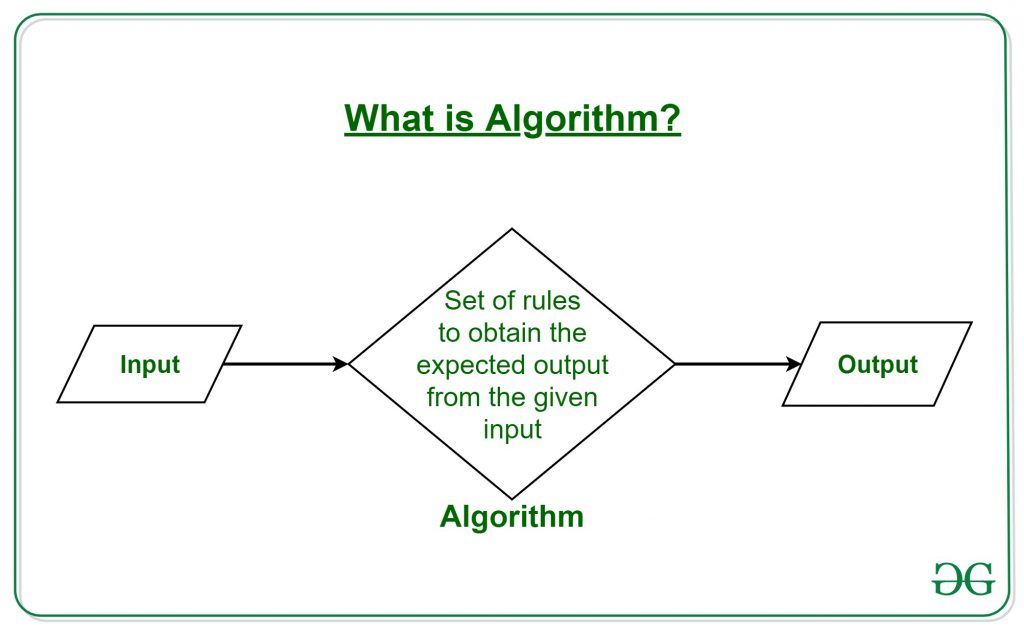
Table of Content
What is an Algorithm?
How do algorithms work.
- What Makes a Good Algorithm?
What is the Need for Algorithms?
Examples of algorithms, how to write an algorithm, learn basics of algorithms, types of algorithms.
An algorithm is a finite sequence of well-defined instructions that can be used to solve a computational problem. It provides a step-by-step procedure that convert an input into a desired output.
Algorithms typically follow a logical structure:
- Input: The algorithm receives input data.
- Processing: The algorithm performs a series of operations on the input data.
- Output: The algorithm produces the desired output.
Characteristics of an Algorithm:
- Clear and Unambiguous: The algorithm should be unambiguous. Each of its steps should be clear in all aspects and must lead to only one meaning.
- Well-defined Inputs: If an algorithm says to take inputs, it should be well-defined inputs. It may or may not take input.
- Well-defined Outputs: The algorithm must clearly define what output will be yielded and it should be well-defined as well. It should produce at least 1 output.
- Finiteness: The algorithm must be finite, i.e. it should terminate after a finite time.
- Feasible: The algorithm must be simple, generic, and practical, such that it can be executed using reasonable constraints and resources.
- Language Independent: Algorithm must be language-independent, i.e. it must be just plain instructions that can be implemented in any language, and yet the output will be the same, as expected.
Algorithms are essential for solving complex computational problems efficiently and effectively. They provide a systematic approach to:
- Solving problems: Algorithms break down problems into smaller, manageable steps.
- Optimizing solutions: Algorithms find the best or near-optimal solutions to problems.
- Automating tasks: Algorithms can automate repetitive or complex tasks, saving time and effort.
Below are some example of algorithms:
- Sorting algorithms: Merge sort, Quick sort, Heap sort
- Searching algorithms: Linear search, Binary search, Hashing
- Graph algorithms: Dijkstra’s algorithm, Prim’s algorithm, Floyd-Warshall algorithm
- String matching algorithms: Knuth-Morris-Pratt algorithm, Boyer-Moore algorithm
To write an algorithm, follow these steps:
- Define the problem: Clearly state the problem to be solved.
- Design the algorithm: Choose an appropriate algorithm design paradigm and develop a step-by-step procedure.
- Implement the algorithm: Translate the algorithm into a programming language.
- Test and debug: Execute the algorithm with various inputs to ensure its correctness and efficiency.
- Analyze the algorithm: Determine its time and space complexity and compare it to alternative algorithms.
- What is Algorithm | Introduction to Algorithms
- Definition, Types, Complexity, Examples of Algorithms
- Algorithms Design Techniques
- Why is analysis of an Algorithm important?
- Asymptotic Analysis
- Worst, Average and Best Cases
- Asymptotic Notations
- Lower and Upper Bound Theory
- Introduction to Amortized Analysis
- What does ‘Space Complexity’ mean?
- Polynomial Time Approximation Scheme
- Accounting Method | Amortized Analysis
- Potential Method in Amortized Analysis
Algorithms can be different types, depending on what they do and how they’re made. Some common types are:
1. Searching and Sorting Algorithms
- Introduction to Searching Algorithms
- Introduction to Sorting Algorithm
- Stable and Unstable Sorting Algorithms
- Lower bound for comparison based sorting algorithms
- Can Run Time Complexity of a comparison-based sorting algorithm be less than N logN?
- Which sorting algorithm makes minimum number of memory writes?
2. Greedy Algorithms
- Introduction to Greedy Algorithms
- Activity Selection Problem
- Huffman Coding
- Job Sequencing Problem
- Quiz on Greedy Algorithms
- Minimum Number of Platforms Required for a Railway/Bus Station
3. Dynamic Programming Algorithms
- Introduction to Dynamic Programming
- Overlapping Subproblems Property
- Optimal Substructure Property
- Longest Increasing Subsequence
- Longest Common Subsequence
- Min Cost Path
- Coin Change
- Matrix Chain Multiplication
- 0-1 Knapsack Problem
- Longest Palindromic Subsequence
- Palindrome Partitioning
4. Pattern Searching Algorithms
- Introduction to Pattern Searching
- Naive Pattern Searching
- KMP Algorithm
- Rabin-Karp Algorithm
- Pattern Searching using a Trie of all Suffixes
- Aho-Corasick Algorithm for Pattern Searching
- Z algorithm (Linear time pattern searching Algorithm)
5. Backtracking Algorithm
- Introduction to Backtracking
- Print all permutations of a given string
- The Knight’s tour problem
- Rat in a Maze
- N Queen Problem
- m Coloring Problem
- Hamiltonian Cycle
6. Divide and Conquer Algorithm
- Introduction to Divide and Conquer
- Write your own pow(x, n) to calculate x*n
- Count Inversions
- Closest Pair of Points
- Strassen’s Matrix Multiplication
7. Geometric Algorithm
- Introduction to Geometric Algorithms
- Closest Pair of Points | O(nlogn) Implementation
- How to check if a given point lies inside or outside a polygon?
- How to check if two given line segments intersect?
- Given n line segments, find if any two segments intersect
- How to check if given four points form a square
- Convex Hull using Jarvis’ Algorithm or Wrapping
8. Mathematical Algorithms
- Introduction to Mathematical Algorithms
- Write an Efficient Method to Check if a Number is Multiple of 3
- Write a program to add two numbers in base 14
- Program for Fibonacci numbers
- Average of a stream of numbers
- Multiply two integers without using multiplication, division and bitwise operators, and no loops
- Babylonian method for square root
- Sieve of Eratosthenes
- Pascal’s Triangle
- Given a number, find the next smallest palindrome
- Program to add two polynomials
- Multiply two polynomials
- Count trailing zeroes in factorial of a number
9. Bitwise Algorithms
- Introduction to Bitwise Algorithms
- Little and Big Endian
- Detect opposite signs
- Turn off the rightmost set bit
- Rotate bits
- Next higher number with same number of set bits
- Swap two nibbles in a byte
10. Graph Algorithms
- Introduction to Graph Algorithms
- Cycles in Graph
- Shortest paths
- Topological Sorting
- Connectivity
11. Randomized Algorithms
- Introduction to Randomized Algorithms
- Linearity of Expectation
- Expected Number of Trials until Success
- Randomized Algorithms | Set 0 (Mathematical Background)
- Randomized Algorithms | Set 1 (Introduction and Analysis)
- Randomized Algorithms | Set 2 (Classification and Applications)
- Randomized Algorithms | Set 3 (1/2 Approximate Median)
- Reservoir Sampling
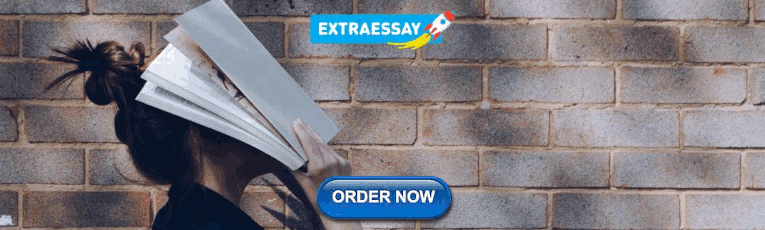
12. Branch and Bound Algorithms
- Branch and Bound | Set 1 (Introduction with 0/1 Knapsack)
- Branch and Bound | Set 2 (Implementation of 0/1 Knapsack)
- Branch and Bound | Set 3 (8 puzzle Problem)
- Branch And Bound | Set 4 (Job Assignment Problem)
- Branch and Bound | Set 5 (N Queen Problem)
- Branch And Bound | Set 6 (Traveling Salesman Problem)
- NP Complete
- Bit Algorithms
Please Login to comment...
Similar reads.

Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Bipolar Disorder
- Therapy Center
- When To See a Therapist
- Types of Therapy
- Best Online Therapy
- Best Couples Therapy
- Best Family Therapy
- Managing Stress
- Sleep and Dreaming
- Understanding Emotions
- Self-Improvement
- Healthy Relationships
- Student Resources
- Personality Types
- Guided Meditations
- Verywell Mind Insights
- 2024 Verywell Mind 25
- Mental Health in the Classroom
- Editorial Process
- Meet Our Review Board
- Crisis Support
Problem-Solving Strategies and Obstacles
Kendra Cherry, MS, is a psychosocial rehabilitation specialist, psychology educator, and author of the "Everything Psychology Book."
:max_bytes(150000):strip_icc():format(webp)/IMG_9791-89504ab694d54b66bbd72cb84ffb860e.jpg)
Sean is a fact-checker and researcher with experience in sociology, field research, and data analytics.
:max_bytes(150000):strip_icc():format(webp)/Sean-Blackburn-1000-a8b2229366944421bc4b2f2ba26a1003.jpg)
JGI / Jamie Grill / Getty Images
- Application
- Improvement
From deciding what to eat for dinner to considering whether it's the right time to buy a house, problem-solving is a large part of our daily lives. Learn some of the problem-solving strategies that exist and how to use them in real life, along with ways to overcome obstacles that are making it harder to resolve the issues you face.
What Is Problem-Solving?
In cognitive psychology , the term 'problem-solving' refers to the mental process that people go through to discover, analyze, and solve problems.
A problem exists when there is a goal that we want to achieve but the process by which we will achieve it is not obvious to us. Put another way, there is something that we want to occur in our life, yet we are not immediately certain how to make it happen.
Maybe you want a better relationship with your spouse or another family member but you're not sure how to improve it. Or you want to start a business but are unsure what steps to take. Problem-solving helps you figure out how to achieve these desires.
The problem-solving process involves:
- Discovery of the problem
- Deciding to tackle the issue
- Seeking to understand the problem more fully
- Researching available options or solutions
- Taking action to resolve the issue
Before problem-solving can occur, it is important to first understand the exact nature of the problem itself. If your understanding of the issue is faulty, your attempts to resolve it will also be incorrect or flawed.
Problem-Solving Mental Processes
Several mental processes are at work during problem-solving. Among them are:
- Perceptually recognizing the problem
- Representing the problem in memory
- Considering relevant information that applies to the problem
- Identifying different aspects of the problem
- Labeling and describing the problem
Problem-Solving Strategies
There are many ways to go about solving a problem. Some of these strategies might be used on their own, or you may decide to employ multiple approaches when working to figure out and fix a problem.
An algorithm is a step-by-step procedure that, by following certain "rules" produces a solution. Algorithms are commonly used in mathematics to solve division or multiplication problems. But they can be used in other fields as well.
In psychology, algorithms can be used to help identify individuals with a greater risk of mental health issues. For instance, research suggests that certain algorithms might help us recognize children with an elevated risk of suicide or self-harm.
One benefit of algorithms is that they guarantee an accurate answer. However, they aren't always the best approach to problem-solving, in part because detecting patterns can be incredibly time-consuming.
There are also concerns when machine learning is involved—also known as artificial intelligence (AI)—such as whether they can accurately predict human behaviors.
Heuristics are shortcut strategies that people can use to solve a problem at hand. These "rule of thumb" approaches allow you to simplify complex problems, reducing the total number of possible solutions to a more manageable set.
If you find yourself sitting in a traffic jam, for example, you may quickly consider other routes, taking one to get moving once again. When shopping for a new car, you might think back to a prior experience when negotiating got you a lower price, then employ the same tactics.
While heuristics may be helpful when facing smaller issues, major decisions shouldn't necessarily be made using a shortcut approach. Heuristics also don't guarantee an effective solution, such as when trying to drive around a traffic jam only to find yourself on an equally crowded route.
Trial and Error
A trial-and-error approach to problem-solving involves trying a number of potential solutions to a particular issue, then ruling out those that do not work. If you're not sure whether to buy a shirt in blue or green, for instance, you may try on each before deciding which one to purchase.
This can be a good strategy to use if you have a limited number of solutions available. But if there are many different choices available, narrowing down the possible options using another problem-solving technique can be helpful before attempting trial and error.
In some cases, the solution to a problem can appear as a sudden insight. You are facing an issue in a relationship or your career when, out of nowhere, the solution appears in your mind and you know exactly what to do.
Insight can occur when the problem in front of you is similar to an issue that you've dealt with in the past. Although, you may not recognize what is occurring since the underlying mental processes that lead to insight often happen outside of conscious awareness .
Research indicates that insight is most likely to occur during times when you are alone—such as when going on a walk by yourself, when you're in the shower, or when lying in bed after waking up.
How to Apply Problem-Solving Strategies in Real Life
If you're facing a problem, you can implement one or more of these strategies to find a potential solution. Here's how to use them in real life:
- Create a flow chart . If you have time, you can take advantage of the algorithm approach to problem-solving by sitting down and making a flow chart of each potential solution, its consequences, and what happens next.
- Recall your past experiences . When a problem needs to be solved fairly quickly, heuristics may be a better approach. Think back to when you faced a similar issue, then use your knowledge and experience to choose the best option possible.
- Start trying potential solutions . If your options are limited, start trying them one by one to see which solution is best for achieving your desired goal. If a particular solution doesn't work, move on to the next.
- Take some time alone . Since insight is often achieved when you're alone, carve out time to be by yourself for a while. The answer to your problem may come to you, seemingly out of the blue, if you spend some time away from others.
Obstacles to Problem-Solving
Problem-solving is not a flawless process as there are a number of obstacles that can interfere with our ability to solve a problem quickly and efficiently. These obstacles include:
- Assumptions: When dealing with a problem, people can make assumptions about the constraints and obstacles that prevent certain solutions. Thus, they may not even try some potential options.
- Functional fixedness : This term refers to the tendency to view problems only in their customary manner. Functional fixedness prevents people from fully seeing all of the different options that might be available to find a solution.
- Irrelevant or misleading information: When trying to solve a problem, it's important to distinguish between information that is relevant to the issue and irrelevant data that can lead to faulty solutions. The more complex the problem, the easier it is to focus on misleading or irrelevant information.
- Mental set: A mental set is a tendency to only use solutions that have worked in the past rather than looking for alternative ideas. A mental set can work as a heuristic, making it a useful problem-solving tool. However, mental sets can also lead to inflexibility, making it more difficult to find effective solutions.
How to Improve Your Problem-Solving Skills
In the end, if your goal is to become a better problem-solver, it's helpful to remember that this is a process. Thus, if you want to improve your problem-solving skills, following these steps can help lead you to your solution:
- Recognize that a problem exists . If you are facing a problem, there are generally signs. For instance, if you have a mental illness , you may experience excessive fear or sadness, mood changes, and changes in sleeping or eating habits. Recognizing these signs can help you realize that an issue exists.
- Decide to solve the problem . Make a conscious decision to solve the issue at hand. Commit to yourself that you will go through the steps necessary to find a solution.
- Seek to fully understand the issue . Analyze the problem you face, looking at it from all sides. If your problem is relationship-related, for instance, ask yourself how the other person may be interpreting the issue. You might also consider how your actions might be contributing to the situation.
- Research potential options . Using the problem-solving strategies mentioned, research potential solutions. Make a list of options, then consider each one individually. What are some pros and cons of taking the available routes? What would you need to do to make them happen?
- Take action . Select the best solution possible and take action. Action is one of the steps required for change . So, go through the motions needed to resolve the issue.
- Try another option, if needed . If the solution you chose didn't work, don't give up. Either go through the problem-solving process again or simply try another option.
You can find a way to solve your problems as long as you keep working toward this goal—even if the best solution is simply to let go because no other good solution exists.
Sarathy V. Real world problem-solving . Front Hum Neurosci . 2018;12:261. doi:10.3389/fnhum.2018.00261
Dunbar K. Problem solving . A Companion to Cognitive Science . 2017. doi:10.1002/9781405164535.ch20
Stewart SL, Celebre A, Hirdes JP, Poss JW. Risk of suicide and self-harm in kids: The development of an algorithm to identify high-risk individuals within the children's mental health system . Child Psychiat Human Develop . 2020;51:913-924. doi:10.1007/s10578-020-00968-9
Rosenbusch H, Soldner F, Evans AM, Zeelenberg M. Supervised machine learning methods in psychology: A practical introduction with annotated R code . Soc Personal Psychol Compass . 2021;15(2):e12579. doi:10.1111/spc3.12579
Mishra S. Decision-making under risk: Integrating perspectives from biology, economics, and psychology . Personal Soc Psychol Rev . 2014;18(3):280-307. doi:10.1177/1088868314530517
Csikszentmihalyi M, Sawyer K. Creative insight: The social dimension of a solitary moment . In: The Systems Model of Creativity . 2015:73-98. doi:10.1007/978-94-017-9085-7_7
Chrysikou EG, Motyka K, Nigro C, Yang SI, Thompson-Schill SL. Functional fixedness in creative thinking tasks depends on stimulus modality . Psychol Aesthet Creat Arts . 2016;10(4):425‐435. doi:10.1037/aca0000050
Huang F, Tang S, Hu Z. Unconditional perseveration of the short-term mental set in chunk decomposition . Front Psychol . 2018;9:2568. doi:10.3389/fpsyg.2018.02568
National Alliance on Mental Illness. Warning signs and symptoms .
Mayer RE. Thinking, problem solving, cognition, 2nd ed .
Schooler JW, Ohlsson S, Brooks K. Thoughts beyond words: When language overshadows insight. J Experiment Psychol: General . 1993;122:166-183. doi:10.1037/0096-3445.2.166
By Kendra Cherry, MSEd Kendra Cherry, MS, is a psychosocial rehabilitation specialist, psychology educator, and author of the "Everything Psychology Book."

How it works
Transform your enterprise with the scalable mindsets, skills, & behavior change that drive performance.
Explore how BetterUp connects to your core business systems.
We pair AI with the latest in human-centered coaching to drive powerful, lasting learning and behavior change.
Build leaders that accelerate team performance and engagement.
Unlock performance potential at scale with AI-powered curated growth journeys.
Build resilience, well-being and agility to drive performance across your entire enterprise.
Transform your business, starting with your sales leaders.
Unlock business impact from the top with executive coaching.
Foster a culture of inclusion and belonging.
Accelerate the performance and potential of your agencies and employees.
See how innovative organizations use BetterUp to build a thriving workforce.
Discover how BetterUp measurably impacts key business outcomes for organizations like yours.
A demo is the first step to transforming your business. Meet with us to develop a plan for attaining your goals.

- What is coaching?
Learn how 1:1 coaching works, who its for, and if it's right for you.
Accelerate your personal and professional growth with the expert guidance of a BetterUp Coach.
Types of Coaching
Navigate career transitions, accelerate your professional growth, and achieve your career goals with expert coaching.
Enhance your communication skills for better personal and professional relationships, with tailored coaching that focuses on your needs.
Find balance, resilience, and well-being in all areas of your life with holistic coaching designed to empower you.
Discover your perfect match : Take our 5-minute assessment and let us pair you with one of our top Coaches tailored just for you.

Research, expert insights, and resources to develop courageous leaders within your organization.
Best practices, research, and tools to fuel individual and business growth.
View on-demand BetterUp events and learn about upcoming live discussions.
The latest insights and ideas for building a high-performing workplace.
- BetterUp Briefing
The online magazine that helps you understand tomorrow's workforce trends, today.
Innovative research featured in peer-reviewed journals, press, and more.
Founded in 2022 to deepen the understanding of the intersection of well-being, purpose, and performance
We're on a mission to help everyone live with clarity, purpose, and passion.
Join us and create impactful change.
Read the buzz about BetterUp.
Meet the leadership that's passionate about empowering your workforce.
For Business
For Individuals
10 Problem-solving strategies to turn challenges on their head

Jump to section
What is an example of problem-solving?
What are the 5 steps to problem-solving, 10 effective problem-solving strategies, what skills do efficient problem solvers have, how to improve your problem-solving skills.
Problems come in all shapes and sizes — from workplace conflict to budget cuts.
Creative problem-solving is one of the most in-demand skills in all roles and industries. It can boost an organization’s human capital and give it a competitive edge.
Problem-solving strategies are ways of approaching problems that can help you look beyond the obvious answers and find the best solution to your problem .
Let’s take a look at a five-step problem-solving process and how to combine it with proven problem-solving strategies. This will give you the tools and skills to solve even your most complex problems.
Good problem-solving is an essential part of the decision-making process . To see what a problem-solving process might look like in real life, let’s take a common problem for SaaS brands — decreasing customer churn rates.
To solve this problem, the company must first identify it. In this case, the problem is that the churn rate is too high.
Next, they need to identify the root causes of the problem. This could be anything from their customer service experience to their email marketing campaigns. If there are several problems, they will need a separate problem-solving process for each one.
Let’s say the problem is with email marketing — they’re not nurturing existing customers. Now that they’ve identified the problem, they can start using problem-solving strategies to look for solutions.
This might look like coming up with special offers, discounts, or bonuses for existing customers. They need to find ways to remind them to use their products and services while providing added value. This will encourage customers to keep paying their monthly subscriptions.
They might also want to add incentives, such as access to a premium service at no extra cost after 12 months of membership. They could publish blog posts that help their customers solve common problems and share them as an email newsletter.
The company should set targets and a time frame in which to achieve them. This will allow leaders to measure progress and identify which actions yield the best results.

Perhaps you’ve got a problem you need to tackle. Or maybe you want to be prepared the next time one arises. Either way, it’s a good idea to get familiar with the five steps of problem-solving.
Use this step-by-step problem-solving method with the strategies in the following section to find possible solutions to your problem.
1. Identify the problem
The first step is to know which problem you need to solve. Then, you need to find the root cause of the problem.
The best course of action is to gather as much data as possible, speak to the people involved, and separate facts from opinions.
Once this is done, formulate a statement that describes the problem. Use rational persuasion to make sure your team agrees .
2. Break the problem down
Identifying the problem allows you to see which steps need to be taken to solve it.
First, break the problem down into achievable blocks. Then, use strategic planning to set a time frame in which to solve the problem and establish a timeline for the completion of each stage.
3. Generate potential solutions
At this stage, the aim isn’t to evaluate possible solutions but to generate as many ideas as possible.
Encourage your team to use creative thinking and be patient — the best solution may not be the first or most obvious one.
Use one or more of the different strategies in the following section to help come up with solutions — the more creative, the better.
4. Evaluate the possible solutions
Once you’ve generated potential solutions, narrow them down to a shortlist. Then, evaluate the options on your shortlist.
There are usually many factors to consider. So when evaluating a solution, ask yourself the following questions:
- Will my team be on board with the proposition?
- Does the solution align with organizational goals ?
- Is the solution likely to achieve the desired outcomes?
- Is the solution realistic and possible with current resources and constraints?
- Will the solution solve the problem without causing additional unintended problems?

5. Implement and monitor the solutions
Once you’ve identified your solution and got buy-in from your team, it’s time to implement it.
But the work doesn’t stop there. You need to monitor your solution to see whether it actually solves your problem.
Request regular feedback from the team members involved and have a monitoring and evaluation plan in place to measure progress.
If the solution doesn’t achieve your desired results, start this step-by-step process again.
There are many different ways to approach problem-solving. Each is suitable for different types of problems.
The most appropriate problem-solving techniques will depend on your specific problem. You may need to experiment with several strategies before you find a workable solution.
Here are 10 effective problem-solving strategies for you to try:
- Use a solution that worked before
- Brainstorming
- Work backward
- Use the Kipling method
- Draw the problem
- Use trial and error
- Sleep on it
- Get advice from your peers
- Use the Pareto principle
- Add successful solutions to your toolkit
Let’s break each of these down.
1. Use a solution that worked before
It might seem obvious, but if you’ve faced similar problems in the past, look back to what worked then. See if any of the solutions could apply to your current situation and, if so, replicate them.
2. Brainstorming
The more people you enlist to help solve the problem, the more potential solutions you can come up with.
Use different brainstorming techniques to workshop potential solutions with your team. They’ll likely bring something you haven’t thought of to the table.
3. Work backward
Working backward is a way to reverse engineer your problem. Imagine your problem has been solved, and make that the starting point.
Then, retrace your steps back to where you are now. This can help you see which course of action may be most effective.
4. Use the Kipling method
This is a method that poses six questions based on Rudyard Kipling’s poem, “ I Keep Six Honest Serving Men .”
- What is the problem?
- Why is the problem important?
- When did the problem arise, and when does it need to be solved?
- How did the problem happen?
- Where is the problem occurring?
- Who does the problem affect?
Answering these questions can help you identify possible solutions.
5. Draw the problem
Sometimes it can be difficult to visualize all the components and moving parts of a problem and its solution. Drawing a diagram can help.
This technique is particularly helpful for solving process-related problems. For example, a product development team might want to decrease the time they take to fix bugs and create new iterations. Drawing the processes involved can help you see where improvements can be made.

6. Use trial-and-error
A trial-and-error approach can be useful when you have several possible solutions and want to test them to see which one works best.
7. Sleep on it
Finding the best solution to a problem is a process. Remember to take breaks and get enough rest . Sometimes, a walk around the block can bring inspiration, but you should sleep on it if possible.
A good night’s sleep helps us find creative solutions to problems. This is because when you sleep, your brain sorts through the day’s events and stores them as memories. This enables you to process your ideas at a subconscious level.
If possible, give yourself a few days to develop and analyze possible solutions. You may find you have greater clarity after sleeping on it. Your mind will also be fresh, so you’ll be able to make better decisions.
8. Get advice from your peers
Getting input from a group of people can help you find solutions you may not have thought of on your own.
For solo entrepreneurs or freelancers, this might look like hiring a coach or mentor or joining a mastermind group.
For leaders , it might be consulting other members of the leadership team or working with a business coach .
It’s important to recognize you might not have all the skills, experience, or knowledge necessary to find a solution alone.
9. Use the Pareto principle
The Pareto principle — also known as the 80/20 rule — can help you identify possible root causes and potential solutions for your problems.
Although it’s not a mathematical law, it’s a principle found throughout many aspects of business and life. For example, 20% of the sales reps in a company might close 80% of the sales.
You may be able to narrow down the causes of your problem by applying the Pareto principle. This can also help you identify the most appropriate solutions.
10. Add successful solutions to your toolkit
Every situation is different, and the same solutions might not always work. But by keeping a record of successful problem-solving strategies, you can build up a solutions toolkit.
These solutions may be applicable to future problems. Even if not, they may save you some of the time and work needed to come up with a new solution.

Improving problem-solving skills is essential for professional development — both yours and your team’s. Here are some of the key skills of effective problem solvers:
- Critical thinking and analytical skills
- Communication skills , including active listening
- Decision-making
- Planning and prioritization
- Emotional intelligence , including empathy and emotional regulation
- Time management
- Data analysis
- Research skills
- Project management
And they see problems as opportunities. Everyone is born with problem-solving skills. But accessing these abilities depends on how we view problems. Effective problem-solvers see problems as opportunities to learn and improve.
Ready to work on your problem-solving abilities? Get started with these seven tips.
1. Build your problem-solving skills
One of the best ways to improve your problem-solving skills is to learn from experts. Consider enrolling in organizational training , shadowing a mentor , or working with a coach .
2. Practice
Practice using your new problem-solving skills by applying them to smaller problems you might encounter in your daily life.
Alternatively, imagine problematic scenarios that might arise at work and use problem-solving strategies to find hypothetical solutions.
3. Don’t try to find a solution right away
Often, the first solution you think of to solve a problem isn’t the most appropriate or effective.
Instead of thinking on the spot, give yourself time and use one or more of the problem-solving strategies above to activate your creative thinking.

4. Ask for feedback
Receiving feedback is always important for learning and growth. Your perception of your problem-solving skills may be different from that of your colleagues. They can provide insights that help you improve.
5. Learn new approaches and methodologies
There are entire books written about problem-solving methodologies if you want to take a deep dive into the subject.
We recommend starting with “ Fixed — How to Perfect the Fine Art of Problem Solving ” by Amy E. Herman.
6. Experiment
Tried-and-tested problem-solving techniques can be useful. However, they don’t teach you how to innovate and develop your own problem-solving approaches.
Sometimes, an unconventional approach can lead to the development of a brilliant new idea or strategy. So don’t be afraid to suggest your most “out there” ideas.
7. Analyze the success of your competitors
Do you have competitors who have already solved the problem you’re facing? Look at what they did, and work backward to solve your own problem.
For example, Netflix started in the 1990s as a DVD mail-rental company. Its main competitor at the time was Blockbuster.
But when streaming became the norm in the early 2000s, both companies faced a crisis. Netflix innovated, unveiling its streaming service in 2007.
If Blockbuster had followed Netflix’s example, it might have survived. Instead, it declared bankruptcy in 2010.
Use problem-solving strategies to uplevel your business
When facing a problem, it’s worth taking the time to find the right solution.
Otherwise, we risk either running away from our problems or headlong into solutions. When we do this, we might miss out on other, better options.
Use the problem-solving strategies outlined above to find innovative solutions to your business’ most perplexing problems.
If you’re ready to take problem-solving to the next level, request a demo with BetterUp . Our expert coaches specialize in helping teams develop and implement strategies that work.
Boost your productivity
Maximize your time and productivity with strategies from our expert coaches.
Elizabeth Perry, ACC
Elizabeth Perry is a Coach Community Manager at BetterUp. She uses strategic engagement strategies to cultivate a learning community across a global network of Coaches through in-person and virtual experiences, technology-enabled platforms, and strategic coaching industry partnerships. With over 3 years of coaching experience and a certification in transformative leadership and life coaching from Sofia University, Elizabeth leverages transpersonal psychology expertise to help coaches and clients gain awareness of their behavioral and thought patterns, discover their purpose and passions, and elevate their potential. She is a lifelong student of psychology, personal growth, and human potential as well as an ICF-certified ACC transpersonal life and leadership Coach.
8 creative solutions to your most challenging problems
5 problem-solving questions to prepare you for your next interview, what are metacognitive skills examples in everyday life, 31 examples of problem solving performance review phrases, what is lateral thinking 7 techniques to encourage creative ideas, leadership activities that encourage employee engagement, learn what process mapping is and how to create one (+ examples), how much do distractions cost 8 effects of lack of focus, can dreams help you solve problems 6 ways to try, similar articles, the pareto principle: how the 80/20 rule can help you do more with less, thinking outside the box: 8 ways to become a creative problem solver, experimentation brings innovation: create an experimental workplace, effective problem statements have these 5 components, contingency planning: 4 steps to prepare for the unexpected, stay connected with betterup, get our newsletter, event invites, plus product insights and research..
3100 E 5th Street, Suite 350 Austin, TX 78702
- Platform Overview
- Integrations
- Powered by AI
- BetterUp Lead
- BetterUp Manage™
- BetterUp Care™
- Sales Performance
- Diversity & Inclusion
- Case Studies
- Why BetterUp?
- About Coaching
- Find your Coach
- Career Coaching
- Communication Coaching
- Life Coaching
- News and Press
- Leadership Team
- Become a BetterUp Coach
- BetterUp Labs
- Center for Purpose & Performance
- Leadership Training
- Business Coaching
- Contact Support
- Contact Sales
- Privacy Policy
- Acceptable Use Policy
- Trust & Security
- Cookie Preferences

- Interview Q
DAA Tutorial
Asymptotic analysis, analysis of sorting, divide and conquer, lower bound theory, sorting in linear time, binary search trees, red black tree, dynamic programming, greedy algorithm, backtracking, shortest path, all-pairs shortest paths, maximum flow, sorting networks, complexity theory, approximation algo, string matching.
Interview Questions
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

- International
- Today’s Paper
- Premium Stories
- Express Shorts
- Health & Wellness
- Board Exam Results
Anand Mahindra says ‘there’s always a solution’; 6 strategies to become an efficient problem solver
Organisational psychologist and hr consultant at that culture thing, gurleen baruah, says, “consistent efforts and creative problem-solving often lead to successful outcomes..

In a recent post on X, Anand Mahindra, a business leader, highlighted a fundamental truth about problem-solving: it takes patience, persistent effort, and creative thinking to overcome obstacles.
“At first glance, most problems seem unresolvable. There’s always a solution,” he wrote.

Whether you’re facing personal dilemmas, professional hurdles, or societal issues, the principles of consistency and creativity can serve as guiding lights.
At first glance, most problems seem unresolvable. There’s always a solution. All problems take patience, consistent effort—and some alternative thinking to resolve. #MondayMotivaton pic.twitter.com/IPcCfKC70N — anand mahindra (@anandmahindra) April 29, 2024
Organisational psychologist and HR consultant at That Culture Thing, Gurleen Baruah, agrees, “Consistent efforts and creative problem-solving often lead to successful outcomes. Understanding the problem thoroughly before implementing solutions is crucial . By spending time and considering different perspectives, one can gain a holistic understanding of the issue at hand.”
Individuals who cultivate resilience, she adds, are better equipped to navigate challenges, overcome obstacles, and ultimately achieve successful outcomes in their problem-solving endeavors.
Let’s delve into six strategies that harness these principles to tackle problems effectively, as suggested by Baruah:

Start with diagnostics
It’s crucial to first understand the problem from various levels. Imagine going to the doctor, what does the doctor do? They ask you about your symptoms, where it hurts, and to assess, give out tests so that they get the data on what’s really happening. A doctor never prescribes without diagnosing a problem. Similarly, in any problem or challenge, start with diagnosing the issue. Talk to stakeholders, get into the history of it, what led to the problem, and try to get to the root cause of it. Use the “5 whys” method for it.
Hypothesise and brainstorm solutions
The next step would entail designing a hypothesis around the issue. When you identify the root cause of an issue, try to come up with potential solutions that if a set of solutions is implemented, will lead to desired results. Make sure that you think through things and logically try to propose potential solutions . It usually takes brainstorming and divergent thinking. For brainstorming purposes, I recommend the “6 thinking hats” method where participants think through problems and solutions from 6 various standpoints.
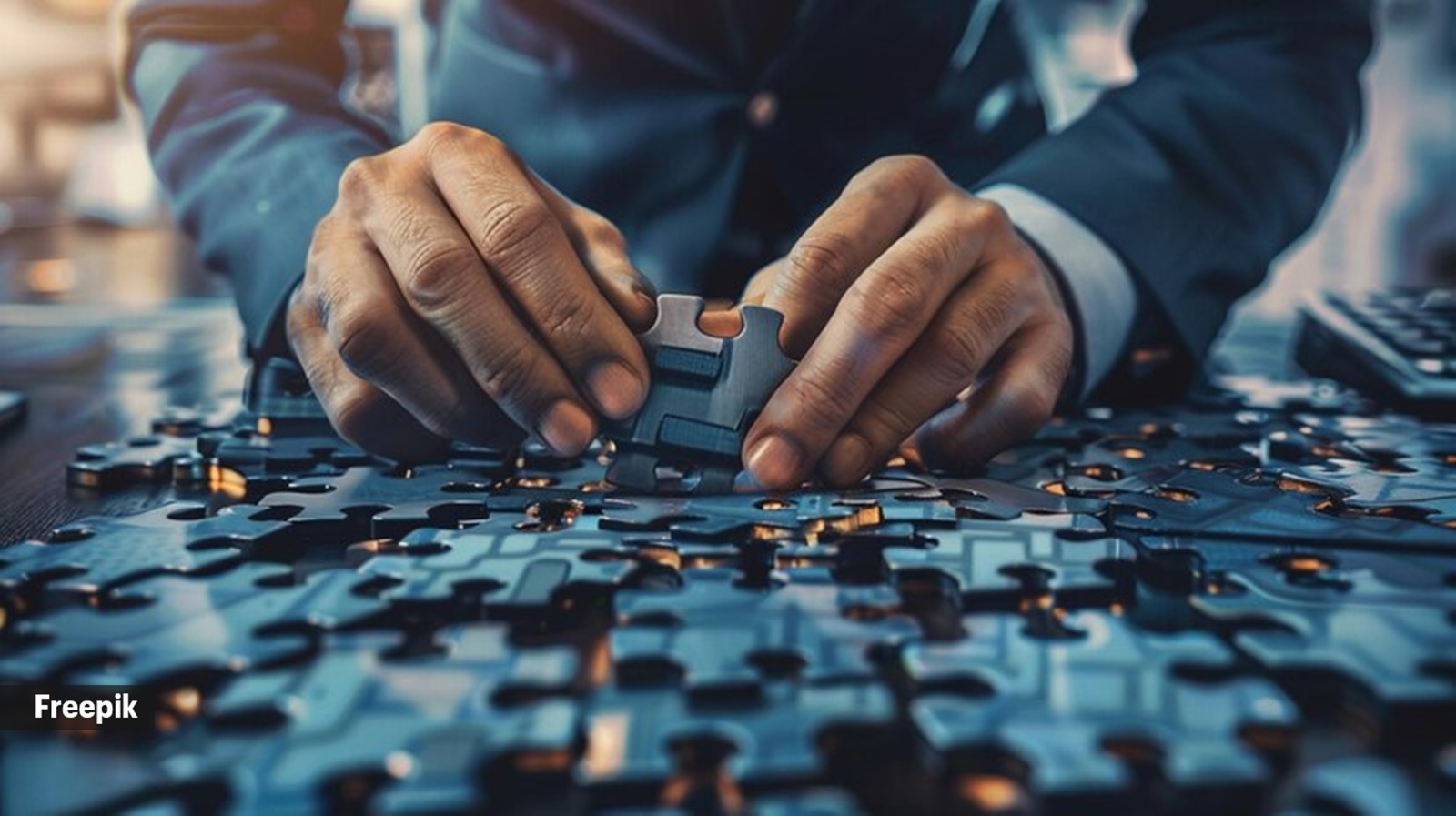
Learn to work within constraints and think outside and inside the box
We’ve all heard of out of the box thinking, right? But does it really work? Imagine a problem and you have solutions but it costs a lot of money but your budget is a fraction of your idea. So no matter how great or out of the box the idea is, it cannot be implemented because there’s less budget . Hence start with boundaries, start with a box (like a boundary) and lay out the limitations and constraints first, and then think of novel ways to solve it.
Stand on the shoulders of giants
Many times you don’t need to think of a solution from scratch. To reach a good solution, take inspiration from history, and research on similar areas, there might be already formed good solutions, like in open source, build on top of that. Use your creativity and imagination on the well-laid-out foundation.
Adopt a growth and learning mindset
In a fast-paced world, skills become redundant overnight. In order to be successful problem solvers, make sure that you adopt a growth mindset . Be open to learning, fail and get up again. Your quickness to learn will be and is a crucial skill in the 21st century.
Perseverance and a persistent attitude
You may witness failures and not apt solutions. It’s ok, get up and try again. Most known problem solvers failed many times before they got successful. Persevere, try again and don’t lose hope. Sometimes we are so close to problem solving when we give up. A little more push and you’re already there. So keep consistently working on it.
- Anand Mahindra
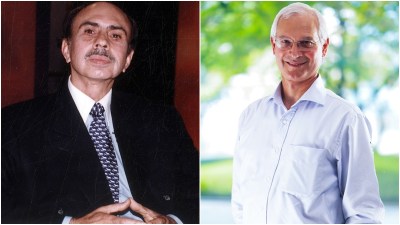
The 127-year-old Godrej Group, spanning from soaps and home appliances to real estate, has reached an agreement to split the conglomerate between two branches of the founding family. Adi and Nadir Godrej will keep Godrej Industries, while Jamshyd and Smita will get Godrej & Boyce and its affiliates, as well as a land bank including prime property in Mumbai.
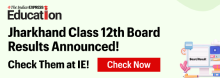
More Lifestyle
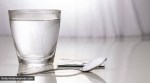
Buzzing Now
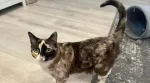
May 01: Latest News
- 01 2 Indian spies expelled from Australia for trying to ‘steal secrets’ in 2020: Australian media
- 02 IPL 2024 points table update: Lucknow pip Chennai for the third spot, Mumbai remain ninth
- 03 Maharashtra poll snippets: Where is Nana Patole?
- 04 Patidar stir: Surat court acquits 3 youths accused of assaulting cops
- 05 US poised to ease restrictions on marijuana in historic shift, but it’ll remain controlled substance
- Elections 2024
- Political Pulse
- Entertainment
- Movie Review
- Newsletters
- Gold Rate Today
- Silver Rate Today
- Petrol Rate Today
- Diesel Rate Today
- Web Stories
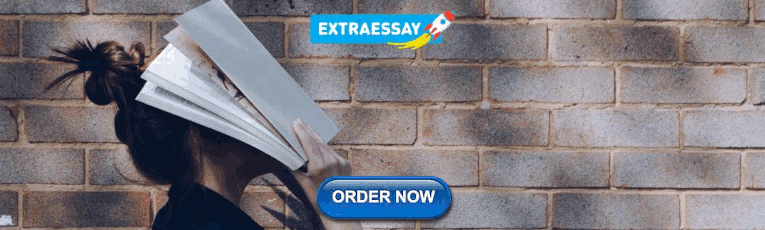
IMAGES
VIDEO
COMMENTS
Design and Analysis of Algorithms. Design and Analysis of Algorithms is a fundamental aspect of computer science that involves creating efficient solutions to computational problems and evaluating their performance. DSA focuses on designing algorithms that effectively address specific challenges and analyzing their efficiency in terms of time ...
The divide-and-conquer strategy DAA 2019 2. Divide and Conquer Algorithms - 3 / 52 The divide-and-conquer strategy solves a problem by: 1. Breaking it into subproblems (smaller instances of the same problem) 2. Recursively solving these subproblems [Base case: If the subproblems are small enough, just solve them by brute force.] 3.
Problem Solving: Different problems require different algorithms, and by having a classification, it can help identify the best algorithm for a particular problem. Performance Comparison: By classifying algorithms, it is possible to compare their performance in terms of time and space complexity, making it easier to choose the best algorithm for a particular use case.
An algorithm is a set of instructions to solve a problem by performing calculations, data processing, or automating reasoning tasks. However, there are always multiple solutions to solving a problem. Design and Analysis of Algorithms provides various ways to design efficient algorithms to solve a problem by analysing their complexities.
Algorithm Design Techniques. 1. Divide and Conquer Approach: It is a top-down approach. The algorithms which follow the divide & conquer techniques involve three steps: Divide the original problem into a set of subproblems. Solve every subproblem individually, recursively. Combine the solution of the subproblems (top level) into a solution of ...
Learn the general method of backtracking in DAA and how to apply it to solve complex problems. Understand the concept, algorithm, and examples of backtracking. ... Backtracking is a problem-solving strategy that involves recursively exploring all possible solutions to a problem. It is a form of brute force approach that systematically generates ...
Divide and conquer algorithm works on top-down approach and is preferred for large problems. As the name says divide and conquer, it follows following steps: Step 1: Divide the problem into several subproblems. Step 2: Conquer or solve each sub-problem. Step 3: Combine each sub-problem to get the required result.
An Algorithm is a set of well-defined instructions designed to perform a specific set of tasks. Algorithms are used in Computer science to perform calculations, automatic reasoning, data processing, computations, and problem-solving. Designing an algorithm is important before writing the program code as the algorithm explains the logic even ...
Analysis of algorithms is the determination of the amount of time and space resources required to execute it. Usually, the efficiency or running time of an algorithm is stated as a function relating the input length to the number of steps, known as time complexity, or volume of memory, known as space complexity.
Backtracking is a problem-solving algorithmic technique that involves finding a solution incrementally by trying different options and undoing them if they lead to a dead end. It is commonly used in situations where you need to explore multiple possibilities to solve a problem, like searching for a path in a maze or solving puzzles like Sudoku .
Divide and Conquer Approach. This strategy is about dividing a problem into more than one subproblems, solving each of them, and then, if necessary, combining their solutions to get a solution to the original problem. We solve many fundamental problems efficiently in computer science by using this strategy. Example problems: Merge Sort , Quick ...
DAA Algorithm with daa tutorial, introduction, Algorithm, Asymptotic Analysis, Control Structure, Recurrence, Master Method, Recursion Tree Method, Sorting Algorithm, Bubble Sort, Selection Sort, Insertion Sort, Binary Search, Merge Sort, Counting Sort, etc. ... The greedy method is a problem-solving strategy in the design and analysis of ...
Divide and Conquer is an algorithmic pattern. In algorithmic methods, the design is to take a dispute on a huge input, break the input into minor pieces, decide the problem on each of the small pieces, and then merge the piecewise solutions into a global solution. This mechanism of solving the problem is called the Divide & Conquer Strategy.
Fundamental Algorithmic Strategies: Brute-Force, Greedy, Dynamic Programming, Branch- and-Bound and Backtracking methodologies for the design of algorithms; Illustrations of these techniques for Problem-Solving, Bin Packing, Knap Sack TSP. Heuristics-characteristics and their application domains.
The first strategy you might try when solving a routine problem is called an algorithm. Algorithms are step-by-step strategies or processes for how to solve a problem or achieve a goal. Another solution that many people use to solve problems is called heuristics. Heuristics are general strategies used to make quick, short-cut
Step 3: Designing efficient solution with pseudocode. This is a stage to use the best experience of DSA problem-solving and apply various problem-solving strategies. One practical truth is: moving from a basic algorithm to the most efficient algorithm is a little difficult in a single step. Each time, we need to optimize the previous algorithm ...
In insight problem-solving, the cognitive processes that help you solve a problem happen outside your conscious awareness. 4. Working backward. Working backward is a problem-solving approach often ...
6. Approximate algorithms are a strategy for overcoming NP-completeness in optimisation problems. The optimal answer is not always ensured by this method. The approximation algorithm aims to reach ...
An algorithm is a step-by-step procedure for solving a problem or accomplishing a task. In the context of data structures and algorithms, it is a set of well-defined instructions for performing a specific computational task. Algorithms are fundamental to computer science and play very important role in designing efficient solutions for various problems.
Problem-solving is a vital skill for coping with various challenges in life. This webpage explains the different strategies and obstacles that can affect how you solve problems, and offers tips on how to improve your problem-solving skills. Learn how to identify, analyze, and overcome problems with Verywell Mind.
10 effective problem-solving strategies. There are many different ways to approach problem-solving. Each is suitable for different types of problems. The most appropriate problem-solving techniques will depend on your specific problem. You may need to experiment with several strategies before you find a workable solution.
The divide-and-conquer strategy DAA 2022 2. Divide and Conquer Algorithms - 3 / 60 The divide-and-conquer strategy solves a problem by: 1. Breaking it into subproblems (smaller instances of the same problem) 2. Recursively solving these subproblems [Base case: If the subproblems are small enough, just solve them by brute force.] 3.
DAA Tutorial. Our DAA Tutorial is designed for beginners and professionals both. Our DAA Tutorial includes all topics of algorithm, asymptotic analysis, algorithm control structure, recurrence, master method, recursion tree method, simple sorting algorithm, bubble sort, selection sort, insertion sort, divide and conquer, binary search, merge sort, counting sort, lower bound theory etc.
14 types of problem-solving strategies. Here are some examples of problem-solving strategies you can practice using to see which works best for you in different situations: 1. Define the problem. Taking the time to define a potential challenge can help you identify certain elements to create a plan to resolve them.
Anand Mahindra says consistent effort & creativity are problem-solving keys. Learn 6 strategies from organizational psychologist Gurleen Baruah to overcome challenges: Diagnose the problem, brainstorm solutions, think creatively with constraints, learn from others, adopt a growth mindset, and persevere.