
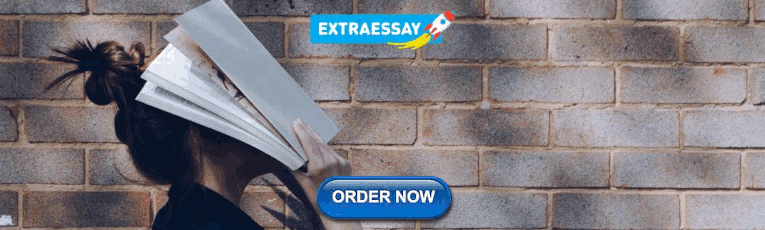
Nick McCullum
Software Developer & Professional Explainer
NumPy Indexing and Assignment
Hey - Nick here! This page is a free excerpt from my $199 course Python for Finance, which is 50% off for the next 50 students.
If you want the full course, click here to sign up.
In this lesson, we will explore indexing and assignment in NumPy arrays.
The Array I'll Be Using In This Lesson
As before, I will be using a specific array through this lesson. This time it will be generated using the np.random.rand method. Here's how I generated the array:
Here is the actual array:
To make this array easier to look at, I will round every element of the array to 2 decimal places using NumPy's round method:
Here's the new array:
How To Return A Specific Element From A NumPy Array
We can select (and return) a specific element from a NumPy array in the same way that we could using a normal Python list: using square brackets.
An example is below:
We can also reference multiple elements of a NumPy array using the colon operator. For example, the index [2:] selects every element from index 2 onwards. The index [:3] selects every element up to and excluding index 3. The index [2:4] returns every element from index 2 to index 4, excluding index 4. The higher endpoint is always excluded.
A few example of indexing using the colon operator are below.
Element Assignment in NumPy Arrays
We can assign new values to an element of a NumPy array using the = operator, just like regular python lists. A few examples are below (note that this is all one code block, which means that the element assignments are carried forward from step to step).
arr[2:5] = 0.5
Returns array([0. , 0. , 0.5, 0.5, 0.5])
As you can see, modifying second_new_array also changed the value of new_array .
Why is this?
By default, NumPy does not create a copy of an array when you reference the original array variable using the = assignment operator. Instead, it simply points the new variable to the old variable, which allows the second variable to make modification to the original variable - even if this is not your intention.
This may seem bizarre, but it does have a logical explanation. The purpose of array referencing is to conserve computing power. When working with large data sets, you would quickly run out of RAM if you created a new array every time you wanted to work with a slice of the array.
Fortunately, there is a workaround to array referencing. You can use the copy method to explicitly copy a NumPy array.
An example of this is below.
As you can see below, making modifications to the copied array does not alter the original.
So far in the lesson, we have only explored how to reference one-dimensional NumPy arrays. We will now explore the indexing of two-dimensional arrays.
Indexing Two-Dimensional NumPy Arrays
To start, let's create a two-dimensional NumPy array named mat :
There are two ways to index a two-dimensional NumPy array:
- mat[row, col]
- mat[row][col]
I personally prefer to index using the mat[row][col] nomenclature because it is easier to visualize in a step-by-step fashion. For example:
You can also generate sub-matrices from a two-dimensional NumPy array using this notation:
Array referencing also applies to two-dimensional arrays in NumPy, so be sure to use the copy method if you want to avoid inadvertently modifying an original array after saving a slice of it into a new variable name.
Conditional Selection Using NumPy Arrays
NumPy arrays support a feature called conditional selection , which allows you to generate a new array of boolean values that state whether each element within the array satisfies a particular if statement.
An example of this is below (I also re-created our original arr variable since its been awhile since we've seen it):
You can also generate a new array of values that satisfy this condition by passing the condition into the square brackets (just like we do for indexing).
An example of this is below:
Conditional selection can become significantly more complex than this. We will explore more examples in this section's associated practice problems.
In this lesson, we explored NumPy array indexing and assignment in thorough detail. We will solidify your knowledge of these concepts further by working through a batch of practice problems in the next section.
NumPy: Get and set values in an array using various indexing
This article explains how to get and set values, such as individual elements or subarrays (e.g., rows or columns), in a NumPy array ( ndarray ) using various indexing.
- Indexing on ndarrays — NumPy v1.26 Manual
Basics of selecting values in an ndarray
Specify with integers, specify with slices, specify with a list of boolean values: boolean indexing, specify with a list of integers: fancy indexing, combine different specification formats, assign new values to selected ranges, views and copies.
See the following articles for information on deleting, concatenating, and adding to ndarray .
- NumPy: Delete rows/columns from an array with np.delete()
- NumPy: Concatenate arrays with np.concatenate, np.stack, etc.
- NumPy: Insert elements, rows, and columns into an array with np.insert()
The NumPy version used in this article is as follows. Note that functionality may vary between versions.
Individual elements or subarrays (such as rows or columns) in an ndarray can be selected by specifying their positions or ranges in each dimension with commas, as in [○, ○, ○, ...] . The trailing : can be omitted, making [○, ○, :, :] equivalent to [○, ○] .
For a 2D array, [i] selects the ith row, and [:, i] selects the ith column (indexes start from 0 ). More details will be provided later.
Positions in each dimension can be specified not only as integers but also in other formats such as lists or slices, allowing for the selection of any subarray.
Positions (indexes) are specified as integers ( int ).
Indexes start from 0 , and negative values can be used to specify positions from the end ( -1 represents the last). Specifying a non-existent position results in an error.
The same applies to multi-dimensional arrays. Positions are specified for each dimension.
You can omit the specification of later dimensions.
In a 2D array, [i] , equivalent to [i, :] , selects the ith row as a 1D array, and [:, i] selects the ith column.
Ranges can be selected with slices ( start:end:step ).
- NumPy: Slicing ndarray
Example with a 1D array:
Example with a 2D array:
The trailing : can be omitted.
Using a slice i:i+1 selects a single row or column, preserving the array's dimensions, unlike selection with an integer ( int ), which reduces the dimensions.
- NumPy: Get the number of dimensions, shape, and size of ndarray
Slices preserve the original array's dimensions, while integers reduce them. This difference can affect outcomes or cause errors in operations like concatenation, even with the same range selected.
Specifying a list or ndarray of Boolean values ( True or False ) matching the dimensions' sizes selects True positions, similar to masking.
An error occurs if the sizes do not match.
Rows or columns can be extracted using a slice : .
Note that specifying a list of Boolean values for multiple dimensions simultaneously does not yield the expected result. Using np.ix_() is necessary.
- numpy.ix_ — NumPy v1.26 Manual
As with slices, selecting a range of width 1 (a single row or column) preserves the original array's number of dimensions.
A comparison on an ndarray yields a Boolean ndarray . Using this for indexing with [] selects True values, producing a flattened 1D array.
- NumPy: Compare two arrays element-wise
Specify multiple conditions using & (AND), | (OR), and ~ (NOT) with parentheses () . Using and , or , not , or omitting parentheses results in an error.
- How to fix "ValueError: The truth value ... is ambiguous" in NumPy, pandas
For methods of extracting rows or columns that meet certain conditions using Boolean indexing, refer to the following article.
- NumPy: Extract or delete elements, rows, and columns that satisfy the conditions
It is also possible to select ranges with a list or ndarray of integers.
Order can be inverted or repeated, and using negative values is allowed. Essentially, it involves creating a new array by selecting specific positions from the original array.
As with Boolean indexing, specifying lists for multiple dimensions simultaneously does not yield the expected result. Using np.ix_() is necessary.
As in the 1D example, the order can be inverted or repeated, and negative values are also permissible.
When selecting with a list of one element, the original array's number of dimensions is preserved, in contrast to specifying with an integer.
Different formats can be used to specify each dimension.
A combination of a list of Boolean values and a list of integers requires the use of np.ix_() .
Note that np.ix_() can only accept 1D lists or arrays.
For example, when specifying multiple lists for arrays of three dimensions or more, np.ix_() is required. However, it cannot be combined with integers or slices in the same selection operation.
Integers can be specified as lists containing a single element. In this case, the resulting array retains the same number of dimensions as the original ndarray .
You can use range() to achieve similar functionality as slices. For example, to simulate slicing, retrieve the size of the target dimension using the shape attribute and pass it to range() as in range(a.shape[n])[::] .
- How to use range() in Python
You can assign new values to selected ranges in an ndarray .
Specifying a scalar value on the right side assigns that value to all elements in the selected range on the left side.
Arrays can also be specified on the right side.
If the shape of the selected range on the left side matches that of the array on the right side, it is directly assigned. Non-contiguous locations pose no problem.
If the shape of the selected range on the left side does not match that of the array on the right side, it is assigned through broadcasting.
An error occurs if the shapes cannot be broadcast.
For more information on broadcasting, refer to the following article.
- NumPy: Broadcasting rules and examples
The specification format used for each dimension when selecting subarrays determines whether a view or a copy of the original array is returned.
For example, using slices returns a view.
Whether two arrays refer to the same memory can be checked using np.shares_memory() .
- NumPy: Views and copies of arrays
In the case of a view, changing the value in the selected subarray also changes the value in the original array, and vice versa.
Boolean indexing or fancy indexing returns a copy.
In this case, changing the value in the selected subarray does not affect the original array, and vice versa.
To create a copy of a subarray selected with a slice and process it separately from the original ndarray , use the copy() method.
When combining different specification formats, using Boolean or fancy indexing returns a copy.
Using integers and slices returns a view.
Related Categories
Related articles.
- NumPy: Functions ignoring NaN (np.nansum, np.nanmean, etc.)
- NumPy: Generate random numbers with np.random
- NumPy: Ellipsis (...) for ndarray
- List of NumPy articles
- NumPy: arange() and linspace() to generate evenly spaced values
- NumPy: Trigonometric functions (sin, cos, tan, arcsin, arccos, arctan)
- NumPy: np.sign(), np.signbit(), np.copysign()
- NumPy: Set the display format for ndarray
- NumPy: Meaning of the axis parameter (0, 1, -1)
- NumPy: Read and write CSV files (np.loadtxt, np.genfromtxt, np.savetxt)
- OpenCV, NumPy: Rotate and flip image
- NumPy: Set whether to print full or truncated ndarray
- NumPy: Calculate cumulative sum and product (np.cumsum, np.cumprod)
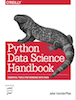
The text is released under the CC-BY-NC-ND license , and code is released under the MIT license . If you find this content useful, please consider supporting the work by buying the book !
The Basics of NumPy Arrays
< Understanding Data Types in Python | Contents | Computation on NumPy Arrays: Universal Functions >
Data manipulation in Python is nearly synonymous with NumPy array manipulation: even newer tools like Pandas ( Chapter 3 ) are built around the NumPy array. This section will present several examples of using NumPy array manipulation to access data and subarrays, and to split, reshape, and join the arrays. While the types of operations shown here may seem a bit dry and pedantic, they comprise the building blocks of many other examples used throughout the book. Get to know them well!
We'll cover a few categories of basic array manipulations here:
- Attributes of arrays : Determining the size, shape, memory consumption, and data types of arrays
- Indexing of arrays : Getting and setting the value of individual array elements
- Slicing of arrays : Getting and setting smaller subarrays within a larger array
- Reshaping of arrays : Changing the shape of a given array
- Joining and splitting of arrays : Combining multiple arrays into one, and splitting one array into many
NumPy Array Attributes ¶
First let's discuss some useful array attributes. We'll start by defining three random arrays, a one-dimensional, two-dimensional, and three-dimensional array. We'll use NumPy's random number generator, which we will seed with a set value in order to ensure that the same random arrays are generated each time this code is run:
Each array has attributes ndim (the number of dimensions), shape (the size of each dimension), and size (the total size of the array):
Another useful attribute is the dtype , the data type of the array (which we discussed previously in Understanding Data Types in Python ):
Other attributes include itemsize , which lists the size (in bytes) of each array element, and nbytes , which lists the total size (in bytes) of the array:
In general, we expect that nbytes is equal to itemsize times size .
Array Indexing: Accessing Single Elements ¶
If you are familiar with Python's standard list indexing, indexing in NumPy will feel quite familiar. In a one-dimensional array, the $i^{th}$ value (counting from zero) can be accessed by specifying the desired index in square brackets, just as with Python lists:
To index from the end of the array, you can use negative indices:
In a multi-dimensional array, items can be accessed using a comma-separated tuple of indices:
Values can also be modified using any of the above index notation:
Keep in mind that, unlike Python lists, NumPy arrays have a fixed type. This means, for example, that if you attempt to insert a floating-point value to an integer array, the value will be silently truncated. Don't be caught unaware by this behavior!
Array Slicing: Accessing Subarrays ¶
Just as we can use square brackets to access individual array elements, we can also use them to access subarrays with the slice notation, marked by the colon ( : ) character. The NumPy slicing syntax follows that of the standard Python list; to access a slice of an array x , use this:
If any of these are unspecified, they default to the values start=0 , stop= size of dimension , step=1 . We'll take a look at accessing sub-arrays in one dimension and in multiple dimensions.
One-dimensional subarrays ¶
A potentially confusing case is when the step value is negative. In this case, the defaults for start and stop are swapped. This becomes a convenient way to reverse an array:
Multi-dimensional subarrays ¶
Multi-dimensional slices work in the same way, with multiple slices separated by commas. For example:
Finally, subarray dimensions can even be reversed together:
Accessing array rows and columns ¶
One commonly needed routine is accessing of single rows or columns of an array. This can be done by combining indexing and slicing, using an empty slice marked by a single colon ( : ):
In the case of row access, the empty slice can be omitted for a more compact syntax:
Subarrays as no-copy views ¶
One important–and extremely useful–thing to know about array slices is that they return views rather than copies of the array data. This is one area in which NumPy array slicing differs from Python list slicing: in lists, slices will be copies. Consider our two-dimensional array from before:
Let's extract a $2 \times 2$ subarray from this:
Now if we modify this subarray, we'll see that the original array is changed! Observe:
This default behavior is actually quite useful: it means that when we work with large datasets, we can access and process pieces of these datasets without the need to copy the underlying data buffer.
Creating copies of arrays ¶
Despite the nice features of array views, it is sometimes useful to instead explicitly copy the data within an array or a subarray. This can be most easily done with the copy() method:
If we now modify this subarray, the original array is not touched:
Reshaping of Arrays ¶
Another useful type of operation is reshaping of arrays. The most flexible way of doing this is with the reshape method. For example, if you want to put the numbers 1 through 9 in a $3 \times 3$ grid, you can do the following:
Note that for this to work, the size of the initial array must match the size of the reshaped array. Where possible, the reshape method will use a no-copy view of the initial array, but with non-contiguous memory buffers this is not always the case.
Another common reshaping pattern is the conversion of a one-dimensional array into a two-dimensional row or column matrix. This can be done with the reshape method, or more easily done by making use of the newaxis keyword within a slice operation:
We will see this type of transformation often throughout the remainder of the book.
Array Concatenation and Splitting ¶
All of the preceding routines worked on single arrays. It's also possible to combine multiple arrays into one, and to conversely split a single array into multiple arrays. We'll take a look at those operations here.
Concatenation of arrays ¶
Concatenation, or joining of two arrays in NumPy, is primarily accomplished using the routines np.concatenate , np.vstack , and np.hstack . np.concatenate takes a tuple or list of arrays as its first argument, as we can see here:
You can also concatenate more than two arrays at once:
It can also be used for two-dimensional arrays:
For working with arrays of mixed dimensions, it can be clearer to use the np.vstack (vertical stack) and np.hstack (horizontal stack) functions:
Similary, np.dstack will stack arrays along the third axis.
Splitting of arrays ¶
The opposite of concatenation is splitting, which is implemented by the functions np.split , np.hsplit , and np.vsplit . For each of these, we can pass a list of indices giving the split points:
Notice that N split-points, leads to N + 1 subarrays. The related functions np.hsplit and np.vsplit are similar:
Similarly, np.dsplit will split arrays along the third axis.
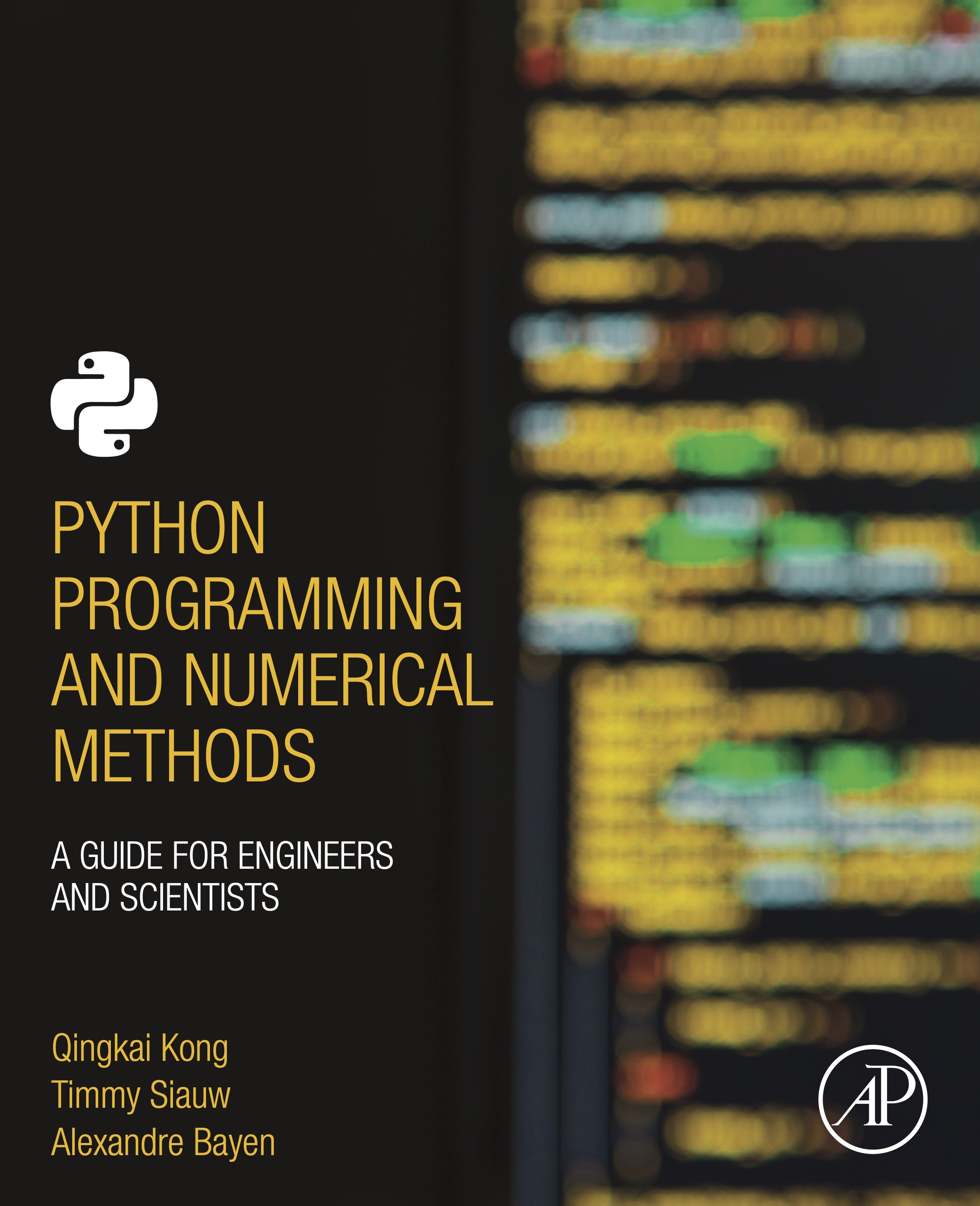
Python Numerical Methods

This notebook contains an excerpt from the Python Programming and Numerical Methods - A Guide for Engineers and Scientists , the content is also available at Berkeley Python Numerical Methods .
The copyright of the book belongs to Elsevier. We also have this interactive book online for a better learning experience. The code is released under the MIT license . If you find this content useful, please consider supporting the work on Elsevier or Amazon !
< 2.6 Data Structure - Dictionaries | Contents | 2.8 Summary and Problems >
Introducing Numpy Arrays ¶
In the 2nd part of this book, we will study the numerical methods by using Python. We will use array/matrix a lot later in the book. Therefore, here we are going to introduce the most common way to handle arrays in Python using the Numpy module . Numpy is probably the most fundamental numerical computing module in Python.
NumPy is important in scientific computing, it is coded both in Python and C (for speed). On its website, a few important features for Numpy is listed:
a powerful N-dimensional array object
sophisticated (broadcasting) functions
tools for integrating C/C++ and Fortran code
useful linear algebra, Fourier transform, and random number capabilities
Here we will only introduce you the Numpy array which is related to the data structure, but we will gradually touch on other aspects of Numpy in the following chapters.
In order to use Numpy module, we need to import it first. A conventional way to import it is to use “np” as a shortened name.
WARNING! Of course, you could call it any name, but conventionally, “np” is accepted by the whole community and it is a good practice to use it for obvious purposes.
To define an array in Python, you could use the np.array function to convert a list.
TRY IT! Create the following arrays:
\(x = \begin{pmatrix} 1 & 4 & 3 \\ \end{pmatrix}\)
\(y = \begin{pmatrix} 1 & 4 & 3 \\ 9 & 2 & 7 \\ \end{pmatrix}\)
NOTE! A 2-D array could use a nested lists to represent, with the inner list represent each row.
Many times we would like to know the size or length of an array. The array shape attribute is called on an array M and returns a 2 × 3 array where the first element is the number of rows in the matrix M and the second element is the number of columns in M. Note that the output of the shape attribute is a tuple. The size attribute is called on an array M and returns the total number of elements in matrix M.
TRY IT! Find the rows, columns and the total size for array y.
NOTE! You may notice the difference that we only use y.shape instead of y.shape() , this is because shape is an attribute rather than a method in this array object. We will introduce more of the object-oriented programming in a later chapter. For now, you need to remember that when we call a method in an object, we need to use the parentheses, while the attribute don’t.
Very often we would like to generate arrays that have a structure or pattern. For instance, we may wish to create the array z = [1 2 3 … 2000]. It would be very cumbersome to type the entire description of z into Python. For generating arrays that are in order and evenly spaced, it is useful to use the arange function in Numpy.
TRY IT! Create an array z from 1 to 2000 with an increment 1.
Using the np.arange , we could create z easily. The first two numbers are the start and end of the sequence, and the last one is the increment. Since it is very common to have an increment of 1, if an increment is not specified, Python will use a default value of 1. Therefore np.arange(1, 2000) will have the same result as np.arange(1, 2000, 1) . Negative or noninteger increments can also be used. If the increment “misses” the last value, it will only extend until the value just before the ending value. For example, x = np.arange(1,8,2) would be [1, 3, 5, 7].
TRY IT! Generate an array with [0.5, 1, 1.5, 2, 2.5].
Sometimes we want to guarantee a start and end point for an array but still have evenly spaced elements. For instance, we may want an array that starts at 1, ends at 8, and has exactly 10 elements. For this purpose you can use the function np.linspace . linspace takes three input values separated by commas. So A = linspace(a,b,n) generates an array of n equally spaced elements starting from a and ending at b.
TRY IT! Use linspace to generate an array starting at 3, ending at 9, and containing 10 elements.
Getting access to the 1D numpy array is similar to what we described for lists or tuples, it has an index to indicate the location. For example:
For 2D arrays, it is slightly different, since we have rows and columns. To get access to the data in a 2D array M, we need to use M[r, c], that the row r and column c are separated by comma. This is referred to as array indexing. The r and c could be single number, a list and so on. If you only think about the row index or the column index, than it is similar to the 1D array. Let’s use the \(y = \begin{pmatrix} 1 & 4 & 3 \\ 9 & 2 & 7 \\ \end{pmatrix}\) as an example.
TRY IT! Get the element at first row and 2nd column of array y .
TRY IT! Get the first row of array y .
TRY IT! Get the last column of array y .
TRY IT! Get the first and third column of array y .
There are some predefined arrays that are really useful. For example, the np.zeros , np.ones , and np.empty are 3 useful functions. Let’s see the examples.
TRY IT! Generate a 3 by 5 array with all the as 0.
TRY IT! Generate a 5 by 3 array with all the element as 1.
NOTE! The shape of the array is defined in a tuple with row as the first item, and column as the second. If you only need a 1D array, then it could be only one number as the input: np.ones(5) .
TRY IT! Generate a 1D empty array with 3 elements.
NOTE! The empty array is not really empty, it is filled with random very small numbers.
You can reassign a value of an array by using array indexing and the assignment operator. You can reassign multiple elements to a single number using array indexing on the left side. You can also reassign multiple elements of an array as long as both the number of elements being assigned and the number of elements assigned is the same. You can create an array using array indexing.
TRY IT! Let a = [1, 2, 3, 4, 5, 6]. Reassign the fourth element of A to 7. Reassign the first, second, and thrid elements to 1. Reassign the second, third, and fourth elements to 9, 8, and 7.
TRY IT! Create a zero array b with shape 2 by 2, and set \(b = \begin{pmatrix} 1 & 2 \\ 3 & 4 \\ \end{pmatrix}\) using array indexing.
WARNING! Although you can create an array from scratch using indexing, we do not advise it. It can confuse you and errors will be harder to find in your code later. For example, b[1, 1] = 1 will give the result \(b = \begin{pmatrix} 0 & 0 \\ 0 & 1 \\ \end{pmatrix}\) , which is strange because b[0, 0], b[0, 1], and b[1, 0] were never specified.
Basic arithmetic is defined for arrays. However, there are operations between a scalar (a single number) and an array and operations between two arrays. We will start with operations between a scalar and an array. To illustrate, let c be a scalar, and b be a matrix.
b + c , b − c , b * c and b / c adds a to every element of b, subtracts c from every element of b, multiplies every element of b by c, and divides every element of b by c, respectively.
TRY IT! Let \(b = \begin{pmatrix} 1 & 2 \\ 3 & 4 \\ \end{pmatrix}\) . Add and substract 2 from b. Multiply and divide b by 2. Square every element of b. Let c be a scalar. On your own, verify the reflexivity of scalar addition and multiplication: b + c = c + b and cb = bc.
Describing operations between two matrices is more complicated. Let b and d be two matrices of the same size. b − d takes every element of b and subtracts the corresponding element of d. Similarly, b + d adds every element of d to the corresponding element of b.
TRY IT! Let \(b = \begin{pmatrix} 1 & 2 \\ 3 & 4 \\ \end{pmatrix}\) and \(d = \begin{pmatrix} 3 & 4 \\ 5 & 6 \\ \end{pmatrix}\) . Compute b + d and b - d.
There are two different kinds of matrix multiplication (and division). There is element-by-element matrix multiplication and standard matrix multiplication. For this section, we will only show how element-by-element matrix multiplication and division work. Standard matrix multiplication will be described in later chapter on Linear Algebra. Python takes the * symbol to mean element-by-element multiplication. For matrices b and d of the same size, b * d takes every element of b and multiplies it by the corresponding element of d. The same is true for / and **.
TRY IT! Compute b * d, b / d, and b**d.
The transpose of an array, b, is an array, d, where b[i, j] = d[j, i]. In other words, the transpose switches the rows and the columns of b. You can transpose an array in Python using the array method T .
TRY IT! Compute the transpose of array b.
Numpy has many arithmetic functions, such as sin, cos, etc., can take arrays as input arguments. The output is the function evaluated for every element of the input array. A function that takes an array as input and performs the function on it is said to be vectorized .
TRY IT! Compute np.sqrt for x = [1, 4, 9, 16].
Logical operations are only defined between a scalar and an array and between two arrays of the same size. Between a scalar and an array, the logical operation is conducted between the scalar and each element of the array. Between two arrays, the logical operation is conducted element-by-element.
TRY IT! Check which elements of the array x = [1, 2, 4, 5, 9, 3] are larger than 3. Check which elements in x are larger than the corresponding element in y = [0, 2, 3, 1, 2, 3].
Python can index elements of an array that satisfy a logical expression.
TRY IT! Let x be the same array as in the previous example. Create a variable y that contains all the elements of x that are strictly bigger than 3. Assign all the values of x that are bigger than 3, the value 0.
- Comprehensive Learning Paths
- 150+ Hours of Videos
- Complete Access to Jupyter notebooks, Datasets, References.

101 NumPy Exercises for Data Analysis (Python)
- February 26, 2018
- Selva Prabhakaran
The goal of the numpy exercises is to serve as a reference as well as to get you to apply numpy beyond the basics. The questions are of 4 levels of difficulties with L1 being the easiest to L4 being the hardest.
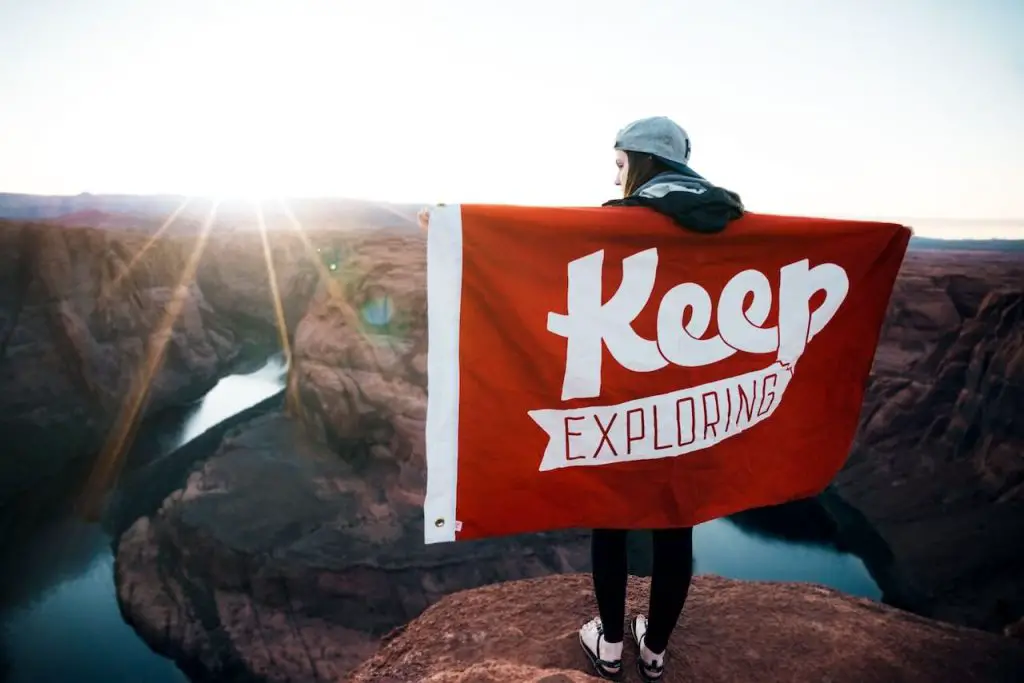
If you want a quick refresher on numpy, the following tutorial is best: Numpy Tutorial Part 1: Introduction Numpy Tutorial Part 2: Advanced numpy tutorials .
Related Post: 101 Practice exercises with pandas .
1. Import numpy as np and see the version
Difficulty Level: L1
Q. Import numpy as np and print the version number.
You must import numpy as np for the rest of the codes in this exercise to work.
To install numpy its recommended to use the installation provided by anaconda.
2. How to create a 1D array?
Q. Create a 1D array of numbers from 0 to 9
Desired output:
3. How to create a boolean array?
Q. Create a 3×3 numpy array of all True’s

4. How to extract items that satisfy a given condition from 1D array?
Q. Extract all odd numbers from arr
5. How to replace items that satisfy a condition with another value in numpy array?
Q. Replace all odd numbers in arr with -1
Desired Output:
6. How to replace items that satisfy a condition without affecting the original array?
Difficulty Level: L2
Q. Replace all odd numbers in arr with -1 without changing arr
7. How to reshape an array?
Q. Convert a 1D array to a 2D array with 2 rows
8. How to stack two arrays vertically?
Q. Stack arrays a and b vertically
9. How to stack two arrays horizontally?
Q. Stack the arrays a and b horizontally.
10. How to generate custom sequences in numpy without hardcoding?
Q. Create the following pattern without hardcoding. Use only numpy functions and the below input array a .
11. How to get the common items between two python numpy arrays?
Q. Get the common items between a and b
12. How to remove from one array those items that exist in another?
Q. From array a remove all items present in array b
13. How to get the positions where elements of two arrays match?
Q. Get the positions where elements of a and b match
14. How to extract all numbers between a given range from a numpy array?
Q. Get all items between 5 and 10 from a .
15. How to make a python function that handles scalars to work on numpy arrays?
Q. Convert the function maxx that works on two scalars, to work on two arrays.
16. How to swap two columns in a 2d numpy array?
Q. Swap columns 1 and 2 in the array arr .
17. How to swap two rows in a 2d numpy array?
Q. Swap rows 1 and 2 in the array arr :
18. How to reverse the rows of a 2D array?
Q. Reverse the rows of a 2D array arr .
19. How to reverse the columns of a 2D array?
Q. Reverse the columns of a 2D array arr .
20. How to create a 2D array containing random floats between 5 and 10?
Q. Create a 2D array of shape 5x3 to contain random decimal numbers between 5 and 10.
21. How to print only 3 decimal places in python numpy array?
Q. Print or show only 3 decimal places of the numpy array rand_arr .
22. How to pretty print a numpy array by suppressing the scientific notation (like 1e10)?
Q. Pretty print rand_arr by suppressing the scientific notation (like 1e10)
23. How to limit the number of items printed in output of numpy array?
Q. Limit the number of items printed in python numpy array a to a maximum of 6 elements.
24. How to print the full numpy array without truncating
Q. Print the full numpy array a without truncating.
25. How to import a dataset with numbers and texts keeping the text intact in python numpy?
Q. Import the iris dataset keeping the text intact.
Since we want to retain the species, a text field, I have set the dtype to object . Had I set dtype=None , a 1d array of tuples would have been returned.
26. How to extract a particular column from 1D array of tuples?
Q. Extract the text column species from the 1D iris imported in previous question.
27. How to convert a 1d array of tuples to a 2d numpy array?
Q. Convert the 1D iris to 2D array iris_2d by omitting the species text field.
28. How to compute the mean, median, standard deviation of a numpy array?
Difficulty: L1
Q. Find the mean, median, standard deviation of iris's sepallength (1st column)
29. How to normalize an array so the values range exactly between 0 and 1?
Difficulty: L2
Q. Create a normalized form of iris 's sepallength whose values range exactly between 0 and 1 so that the minimum has value 0 and maximum has value 1.
30. How to compute the softmax score?
Difficulty Level: L3
Q. Compute the softmax score of sepallength .
31. How to find the percentile scores of a numpy array?
Q. Find the 5th and 95th percentile of iris's sepallength
32. How to insert values at random positions in an array?
Q. Insert np.nan values at 20 random positions in iris_2d dataset
33. How to find the position of missing values in numpy array?
Q. Find the number and position of missing values in iris_2d 's sepallength (1st column)
34. How to filter a numpy array based on two or more conditions?
Q. Filter the rows of iris_2d that has petallength (3rd column) > 1.5 and sepallength (1st column) < 5.0
35. How to drop rows that contain a missing value from a numpy array?
Difficulty Level: L3:
Q. Select the rows of iris_2d that does not have any nan value.
36. How to find the correlation between two columns of a numpy array?
Q. Find the correlation between SepalLength(1st column) and PetalLength(3rd column) in iris_2d
37. How to find if a given array has any null values?
Q. Find out if iris_2d has any missing values.
38. How to replace all missing values with 0 in a numpy array?
Q. Replace all ccurrences of nan with 0 in numpy array
39. How to find the count of unique values in a numpy array?
Q. Find the unique values and the count of unique values in iris's species
40. How to convert a numeric to a categorical (text) array?
Q. Bin the petal length (3rd) column of iris_2d to form a text array, such that if petal length is:
- Less than 3 --> 'small'
- 3-5 --> 'medium'
- '>=5 --> 'large'
41. How to create a new column from existing columns of a numpy array?
Q. Create a new column for volume in iris_2d, where volume is (pi x petallength x sepal_length^2)/3
42. How to do probabilistic sampling in numpy?
Q. Randomly sample iris 's species such that setose is twice the number of versicolor and virginica
Approach 2 is preferred because it creates an index variable that can be used to sample 2d tabular data.
43. How to get the second largest value of an array when grouped by another array?
Q. What is the value of second longest petallength of species setosa
44. How to sort a 2D array by a column
Q. Sort the iris dataset based on sepallength column.
45. How to find the most frequent value in a numpy array?
Q. Find the most frequent value of petal length (3rd column) in iris dataset.
46. How to find the position of the first occurrence of a value greater than a given value?
Q. Find the position of the first occurrence of a value greater than 1.0 in petalwidth 4th column of iris dataset.
47. How to replace all values greater than a given value to a given cutoff?
Q. From the array a , replace all values greater than 30 to 30 and less than 10 to 10.
48. How to get the positions of top n values from a numpy array?
Q. Get the positions of top 5 maximum values in a given array a .
49. How to compute the row wise counts of all possible values in an array?
Difficulty Level: L4
Q. Compute the counts of unique values row-wise.
Output contains 10 columns representing numbers from 1 to 10. The values are the counts of the numbers in the respective rows. For example, Cell(0,2) has the value 2, which means, the number 3 occurs exactly 2 times in the 1st row.
50. How to convert an array of arrays into a flat 1d array?
Difficulty Level: 2
Q. Convert array_of_arrays into a flat linear 1d array.
51. How to generate one-hot encodings for an array in numpy?
Difficulty Level L4
Q. Compute the one-hot encodings (dummy binary variables for each unique value in the array)
52. How to create row numbers grouped by a categorical variable?
Q. Create row numbers grouped by a categorical variable. Use the following sample from iris species as input.
53. How to create groud ids based on a given categorical variable?
Q. Create group ids based on a given categorical variable. Use the following sample from iris species as input.
54. How to rank items in an array using numpy?
Q. Create the ranks for the given numeric array a .
55. How to rank items in a multidimensional array using numpy?
Q. Create a rank array of the same shape as a given numeric array a .
56. How to find the maximum value in each row of a numpy array 2d?
DifficultyLevel: L2
Q. Compute the maximum for each row in the given array.
57. How to compute the min-by-max for each row for a numpy array 2d?
DifficultyLevel: L3
Q. Compute the min-by-max for each row for given 2d numpy array.
58. How to find the duplicate records in a numpy array?
Q. Find the duplicate entries (2nd occurrence onwards) in the given numpy array and mark them as True . First time occurrences should be False .
59. How to find the grouped mean in numpy?
Difficulty Level L3
Q. Find the mean of a numeric column grouped by a categorical column in a 2D numpy array
Desired Solution:
60. How to convert a PIL image to numpy array?
Q. Import the image from the following URL and convert it to a numpy array.
URL = 'https://upload.wikimedia.org/wikipedia/commons/8/8b/Denali_Mt_McKinley.jpg'
61. How to drop all missing values from a numpy array?
Q. Drop all nan values from a 1D numpy array
np.array([1,2,3,np.nan,5,6,7,np.nan])
62. How to compute the euclidean distance between two arrays?
Q. Compute the euclidean distance between two arrays a and b .
63. How to find all the local maxima (or peaks) in a 1d array?
Q. Find all the peaks in a 1D numpy array a . Peaks are points surrounded by smaller values on both sides.
a = np.array([1, 3, 7, 1, 2, 6, 0, 1])
where, 2 and 5 are the positions of peak values 7 and 6.
64. How to subtract a 1d array from a 2d array, where each item of 1d array subtracts from respective row?
Q. Subtract the 1d array b_1d from the 2d array a_2d , such that each item of b_1d subtracts from respective row of a_2d .
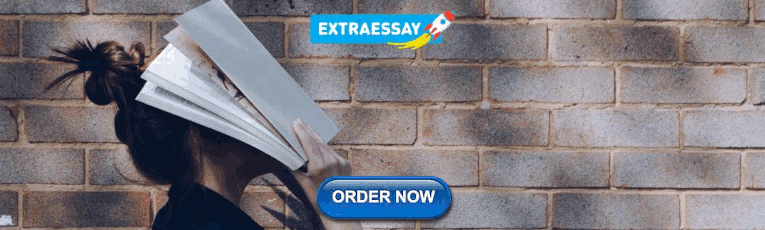
65. How to find the index of n'th repetition of an item in an array
Difficulty Level L2
Q. Find the index of 5th repetition of number 1 in x .
66. How to convert numpy's datetime64 object to datetime's datetime object?
Q. Convert numpy's datetime64 object to datetime's datetime object
67. How to compute the moving average of a numpy array?
Q. Compute the moving average of window size 3, for the given 1D array.
68. How to create a numpy array sequence given only the starting point, length and the step?
Q. Create a numpy array of length 10, starting from 5 and has a step of 3 between consecutive numbers
69. How to fill in missing dates in an irregular series of numpy dates?
Q. Given an array of a non-continuous sequence of dates. Make it a continuous sequence of dates, by filling in the missing dates.
70. How to create strides from a given 1D array?
Q. From the given 1d array arr , generate a 2d matrix using strides, with a window length of 4 and strides of 2, like [[0,1,2,3], [2,3,4,5], [4,5,6,7]..]
More Articles
How to convert python code to cython (and speed up 100x), how to convert python to cython inside jupyter notebooks, install opencv python – a comprehensive guide to installing “opencv-python”, install pip mac – how to install pip in macos: a comprehensive guide, scrapy vs. beautiful soup: which is better for web scraping, add python to path – how to add python to the path environment variable in windows, similar articles, complete introduction to linear regression in r, how to implement common statistical significance tests and find the p value, logistic regression – a complete tutorial with examples in r.
Subscribe to Machine Learning Plus for high value data science content
© Machinelearningplus. All rights reserved.

Machine Learning A-Z™: Hands-On Python & R In Data Science
Free sample videos:.

Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively.
- Introduction
- Introduction to NumPy
- NumPy Array Creation
- NumPy N-d Array Creation
- NumPy Data Types
- NumPy Array Attributes
- NumPy Input Output
- NumPy Array Indexing
- NumPy Array Slicing
- NumPy Array Reshaping
Array Operations
Numpy arithmetic array operations.
- NumPy Array Functions
- NumPy Comparison/Logical Operations
NumPy Math Functions
- NumPy Constants
- NumPy Statistical Functions
NumPy String Functions
Advance NumPy Operations
- NumPy Broadcasting
- NumPy Matrix Operations
- NumPy Set Operations
- NumPy Vectorization
- NumPy Boolean Indexing
- NumPy Fancy Indexing
Additional Topics
- NumPy Random
- NumPy Linear Algebra
- NumPy Histogram
- NumPy Interpolation
- NumPy Files
- NumPy Error Handling
- NumPy Date and Time
- NumPy Data Visualization
NumPy Universal Function
NumPy Tutorials
Numpy Comparison/Logical Operations
- NumPy divide()
- NumPy multiply()
NumPy provides a wide range of operations that can perform on arrays, including arithmetic operations.
NumPy's arithmetic operations are widely used due to their ability to perform simple and efficient calculations on arrays.
In this tutorial, we will explore some commonly used arithmetic operations in NumPy and learn how to use them to manipulate arrays.
- List of Arithmetic Operations
Here's a list of various arithmetic operations along with their associated operators and built-in functions:
To perform each operation, we can either use the associated operator or built-in functions. For example, to perform addition , we can either use the + operator or the add() built-in function.
Next, we will see examples of each of these operations.
- NumPy Array Element-Wise Addition
As mentioned earlier, we can use the both + operator and the built-in function add() to perform element-wise addition between two NumPy arrays. For example,
In the above example, first we created two arrays named: first_array and second_array .
Then, we used the + operator and the add() function to perform element-wise addition respectively.
As we can see, both + and add() give the same result.
- NumPy Array Element-Wise Subtraction
In NumPy, we can either use the - operator or the subtract() function to perform element-wise subtraction between two NumPy arrays. For example,
Here, we have performed subtraction between first_array and second_array using both the - operator and the subtract() function.
- NumPy Array Element-Wise Multiplication
For element-wise multiplication, we can use the * operator or the multiply() function. For example,
Here, we have used * and multiply() to demonstrate two ways of multiplying the two arrays element-wise.
- NumPy Array Element-Wise Division
We can use either the / operator or the divide() function to perform element-wise division between two numpy arrays. For example,
Here, we can see both / and divide() give the same result.
- NumPy Array Element-Wise Exponentiation
Array exponentiation refers to raising each element of an array to a given power.
In NumPy, we can use either the ** operator or the power() function to perform the element-wise exponentiation operation. For example,
In the above example, we have used the ** operator and the power() function to perform exponentiation operation respectively.
Here, ** and power() both raise each element of array1 to the power of 2 .
- NumPy Array Element-Wise Modulus
We can perform a modulus operation in NumPy arrays using the % operator or the mod() function.
This operation calculates the remainder of element-wise division between two arrays.
Let's see an example.
Here, we have performed the element-wise modulus operation using both the % operator and the mod() function.
Table of Contents
Related tutorials.
Programming
- Python »
- 3.13.0b1 Documentation »
- What’s New in Python »
- What’s New In Python 3.13
- Theme Auto Light Dark |
What’s New In Python 3.13 ¶
Thomas Wouters
This article explains the new features in Python 3.13, compared to 3.12.
For full details, see the changelog .
PEP 719 – Python 3.13 Release Schedule
Prerelease users should be aware that this document is currently in draft form. It will be updated substantially as Python 3.13 moves towards release, so it’s worth checking back even after reading earlier versions.
Summary – Release Highlights ¶
Python 3.13 beta is the pre-release of the next version of the Python programming language, with a mix of changes to the language, the implementation and the standard library. The biggest changes to the implementation include a new interactive interpreter, and experimental support for dropping the Global Interpreter Lock ( PEP 703 ) and a Just-In-Time compiler ( PEP 744 ). The library changes contain removal of deprecated APIs and modules, as well as the usual improvements in user-friendliness and correctness.
Interpreter improvements:
A greatly improved interactive interpreter and improved error messages .
Color support in the new interactive interpreter , as well as in tracebacks and doctest output. This can be disabled through the PYTHON_COLORS and NO_COLOR environment variables.
PEP 744 : A basic JIT compiler was added. It is currently disabled by default (though we may turn it on later). Performance improvements are modest – we expect to be improving this over the next few releases.
PEP 667 : The locals() builtin now has defined semantics when mutating the returned mapping. Python debuggers and similar tools may now more reliably update local variables in optimized frames even during concurrent code execution.
New typing features:
PEP 696 : Type parameters ( typing.TypeVar , typing.ParamSpec , and typing.TypeVarTuple ) now support defaults.
PEP 702 : Support for marking deprecations in the type system using the new warnings.deprecated() decorator.
PEP 742 : typing.TypeIs was added, providing more intuitive type narrowing behavior.
PEP 705 : typing.ReadOnly was added, to mark an item of a typing.TypedDict as read-only for type checkers.
Free-threading:
PEP 703 : CPython 3.13 has experimental support for running with the global interpreter lock disabled when built with --disable-gil . See Free-threaded CPython for more details.
Platform support:
PEP 730 : Apple’s iOS is now an officially supported platform. Official Android support ( PEP 738 ) is in the works as well.
Removed modules:
PEP 594 : The remaining 19 “dead batteries” have been removed from the standard library: aifc , audioop , cgi , cgitb , chunk , crypt , imghdr , mailcap , msilib , nis , nntplib , ossaudiodev , pipes , sndhdr , spwd , sunau , telnetlib , uu and xdrlib .
Also removed were the tkinter.tix and lib2to3 modules, and the 2to3 program.
Release schedule changes:
PEP 602 (“Annual Release Cycle for Python”) has been updated:
Python 3.9 - 3.12 have one and a half years of full support, followed by three and a half years of security fixes.
Python 3.13 and later have two years of full support, followed by three years of security fixes.
New Features ¶
A better interactive interpreter ¶.
On Unix-like systems like Linux or macOS, Python now uses a new interactive shell. When the user starts the REPL from an interactive terminal, and both curses and readline are available, the interactive shell now supports the following new features:
Colorized prompts.
Multiline editing with history preservation.
Interactive help browsing using F1 with a separate command history.
History browsing using F2 that skips output as well as the >>> and … prompts.
“Paste mode” with F3 that makes pasting larger blocks of code easier (press F3 again to return to the regular prompt).
The ability to issue REPL-specific commands like help , exit , and quit without the need to use call parentheses after the command name.
If the new interactive shell is not desired, it can be disabled via the PYTHON_BASIC_REPL environment variable.
For more on interactive mode, see Interactive Mode .
(Contributed by Pablo Galindo Salgado, Łukasz Langa, and Lysandros Nikolaou in gh-111201 based on code from the PyPy project.)
Improved Error Messages ¶
The interpreter now colorizes error messages when displaying tracebacks by default. This feature can be controlled via the new PYTHON_COLORS environment variable as well as the canonical NO_COLOR and FORCE_COLOR environment variables. See also Controlling color . (Contributed by Pablo Galindo Salgado in gh-112730 .)
A common mistake is to write a script with the same name as a standard library module. When this results in errors, we now display a more helpful error message:
Similarly, if a script has the same name as a third-party module it attempts to import, and this results in errors, we also display a more helpful error message:
(Contributed by Shantanu Jain in gh-95754 .)
When an incorrect keyword argument is passed to a function, the error message now potentially suggests the correct keyword argument. (Contributed by Pablo Galindo Salgado and Shantanu Jain in gh-107944 .)
Classes have a new __static_attributes__ attribute, populated by the compiler, with a tuple of names of attributes of this class which are accessed through self.X from any function in its body. (Contributed by Irit Katriel in gh-115775 .)
Defined mutation semantics for locals() ¶
Historically, the expected result of mutating the return value of locals() has been left to individual Python implementations to define.
Through PEP 667 , Python 3.13 standardises the historical behaviour of CPython for most code execution scopes, but changes optimized scopes (functions, generators, coroutines, comprehensions, and generator expressions) to explicitly return independent snapshots of the currently assigned local variables, including locally referenced nonlocal variables captured in closures.
To ensure debuggers and similar tools can reliably update local variables in scopes affected by this change, FrameType.f_locals now returns a write-through proxy to the frame’s local and locally referenced nonlocal variables in these scopes, rather than returning an inconsistently updated shared dict instance with undefined runtime semantics.
See PEP 667 for more details, including related C API changes and deprecations.
(PEP and implementation contributed by Mark Shannon and Tian Gao in gh-74929 . Documentation updates provided by Guido van Rossum and Alyssa Coghlan.)
Incremental Garbage Collection ¶
The cycle garbage collector is now incremental. This means that maximum pause times are reduced by an order of magnitude or more for larger heaps.
Support For Mobile Platforms ¶
iOS is now a PEP 11 supported platform. arm64-apple-ios (iPhone and iPad devices released after 2013) and arm64-apple-ios-simulator (Xcode iOS simulator running on Apple Silicon hardware) are now tier 3 platforms.
x86_64-apple-ios-simulator (Xcode iOS simulator running on older x86_64 hardware) is not a tier 3 supported platform, but will be supported on a best-effort basis.
See PEP 730 : for more details.
(PEP written and implementation contributed by Russell Keith-Magee in gh-114099 .)
Experimental JIT Compiler ¶
When CPython is configured using the --enable-experimental-jit option, a just-in-time compiler is added which may speed up some Python programs.
The internal architecture is roughly as follows.
We start with specialized Tier 1 bytecode . See What’s new in 3.11 for details.
When the Tier 1 bytecode gets hot enough, it gets translated to a new, purely internal Tier 2 IR , a.k.a. micro-ops (“uops”).
The Tier 2 IR uses the same stack-based VM as Tier 1, but the instruction format is better suited to translation to machine code.
We have several optimization passes for Tier 2 IR, which are applied before it is interpreted or translated to machine code.
There is a Tier 2 interpreter, but it is mostly intended for debugging the earlier stages of the optimization pipeline. The Tier 2 interpreter can be enabled by configuring Python with --enable-experimental-jit=interpreter .
When the JIT is enabled, the optimized Tier 2 IR is translated to machine code, which is then executed.
The machine code translation process uses a technique called copy-and-patch . It has no runtime dependencies, but there is a new build-time dependency on LLVM.
The --enable-experimental-jit flag has the following optional values:
no (default) – Disable the entire Tier 2 and JIT pipeline.
yes (default if the flag is present without optional value) – Enable the JIT. To disable the JIT at runtime, pass the environment variable PYTHON_JIT=0 .
yes-off – Build the JIT but disable it by default. To enable the JIT at runtime, pass the environment variable PYTHON_JIT=1 .
interpreter – Enable the Tier 2 interpreter but disable the JIT. The interpreter can be disabled by running with PYTHON_JIT=0 .
(On Windows, use PCbuild/build.bat --experimental-jit to enable the JIT or --experimental-jit-interpreter to enable the Tier 2 interpreter.)
See PEP 744 for more details.
(JIT by Brandt Bucher, inspired by a paper by Haoran Xu and Fredrik Kjolstad. Tier 2 IR by Mark Shannon and Guido van Rossum. Tier 2 optimizer by Ken Jin.)
Free-threaded CPython ¶
CPython will run with the global interpreter lock (GIL) disabled when configured using the --disable-gil option at build time. This is an experimental feature and therefore isn’t used by default. Users need to either compile their own interpreter, or install one of the experimental builds that are marked as free-threaded . See PEP 703 “Making the Global Interpreter Lock Optional in CPython” for more detail.
Free-threaded execution allows for full utilization of the available processing power by running threads in parallel on available CPU cores. While not all software will benefit from this automatically, programs designed with threading in mind will run faster on multicore hardware.
Work is still ongoing: expect some bugs and a substantial single-threaded performance hit.
The free-threaded build still supports optionally running with the GIL enabled at runtime using the environment variable PYTHON_GIL or the command line option -X gil .
To check if the current interpreter is configured with --disable-gil , use sysconfig.get_config_var("Py_GIL_DISABLED") . To check if the GIL is actually disabled in the running process, the sys._is_gil_enabled() function can be used.
C-API extension modules need to be built specifically for the free-threaded build. Extensions that support running with the GIL disabled should use the Py_mod_gil slot. Extensions using single-phase init should use PyUnstable_Module_SetGIL() to indicate whether they support running with the GIL disabled. Importing C extensions that don’t use these mechanisms will cause the GIL to be enabled, unless the GIL was explicitly disabled with the PYTHON_GIL environment variable or the -X gil=0 option.
pip 24.1b1 or newer is required to install packages with C extensions in the free-threaded build.
Other Language Changes ¶
Allow the count argument of str.replace() to be a keyword. (Contributed by Hugo van Kemenade in gh-106487 .)
Compiler now strip indents from docstrings. This will reduce the size of bytecode cache (e.g. .pyc file). For example, cache file size for sqlalchemy.orm.session in SQLAlchemy 2.0 is reduced by about 5%. This change will affect tools using docstrings, like doctest . (Contributed by Inada Naoki in gh-81283 .)
The compile() built-in can now accept a new flag, ast.PyCF_OPTIMIZED_AST , which is similar to ast.PyCF_ONLY_AST except that the returned AST is optimized according to the value of the optimize argument. (Contributed by Irit Katriel in gh-108113 ).
multiprocessing , concurrent.futures , compileall : Replace os.cpu_count() with os.process_cpu_count() to select the default number of worker threads and processes. Get the CPU affinity if supported. (Contributed by Victor Stinner in gh-109649 .)
os.path.realpath() now resolves MS-DOS style file names even if the file is not accessible. (Contributed by Moonsik Park in gh-82367 .)
Fixed a bug where a global declaration in an except block is rejected when the global is used in the else block. (Contributed by Irit Katriel in gh-111123 .)
Many functions now emit a warning if a boolean value is passed as a file descriptor argument. This can help catch some errors earlier. (Contributed by Serhiy Storchaka in gh-82626 .)
Added a new environment variable PYTHON_FROZEN_MODULES . It determines whether or not frozen modules are ignored by the import machinery, equivalent of the -X frozen_modules command-line option. (Contributed by Yilei Yang in gh-111374 .)
Add support for the perf profiler working without frame pointers through the new environment variable PYTHON_PERF_JIT_SUPPORT and command-line option -X perf_jit (Contributed by Pablo Galindo in gh-118518 .)
The new PYTHON_HISTORY environment variable can be used to change the location of a .python_history file. (Contributed by Levi Sabah, Zackery Spytz and Hugo van Kemenade in gh-73965 .)
Add PythonFinalizationError exception. This exception derived from RuntimeError is raised when an operation is blocked during the Python finalization .
The following functions now raise PythonFinalizationError, instead of RuntimeError :
_thread.start_new_thread() .
subprocess.Popen .
os.fork() .
os.forkpty() .
(Contributed by Victor Stinner in gh-114570 .)
Added name and mode attributes for compressed and archived file-like objects in modules bz2 , lzma , tarfile and zipfile . (Contributed by Serhiy Storchaka in gh-115961 .)
Allow controlling Expat >=2.6.0 reparse deferral ( CVE-2023-52425 ) by adding five new methods:
xml.etree.ElementTree.XMLParser.flush()
xml.etree.ElementTree.XMLPullParser.flush()
xml.parsers.expat.xmlparser.GetReparseDeferralEnabled()
xml.parsers.expat.xmlparser.SetReparseDeferralEnabled()
xml.sax.expatreader.ExpatParser.flush()
(Contributed by Sebastian Pipping in gh-115623 .)
The ssl.create_default_context() API now includes ssl.VERIFY_X509_PARTIAL_CHAIN and ssl.VERIFY_X509_STRICT in its default flags.
ssl.VERIFY_X509_STRICT may reject pre- RFC 5280 or malformed certificates that the underlying OpenSSL implementation otherwise would accept. While disabling this is not recommended, you can do so using:
(Contributed by William Woodruff in gh-112389 .)
The configparser.ConfigParser now accepts unnamed sections before named ones if configured to do so. (Contributed by Pedro Sousa Lacerda in gh-66449 .)
annotation scope within class scopes can now contain lambdas and comprehensions. Comprehensions that are located within class scopes are not inlined into their parent scope. (Contributed by Jelle Zijlstra in gh-109118 and gh-118160 .)
Classes have a new __firstlineno__ attribute, populated by the compiler, with the line number of the first line of the class definition. (Contributed by Serhiy Storchaka in gh-118465 .)
from __future__ import ... statements are now just normal relative imports if dots are present before the module name. (Contributed by Jeremiah Gabriel Pascual in gh-118216 .)
New Modules ¶
Improved modules ¶.
Add parameter deprecated in methods add_argument() and add_parser() which allows to deprecate command-line options, positional arguments and subcommands. (Contributed by Serhiy Storchaka in gh-83648 .)
Add 'w' type code ( Py_UCS4 ) that can be used for Unicode strings. It can be used instead of 'u' type code, which is deprecated. (Contributed by Inada Naoki in gh-80480 .)
Add clear() method in order to implement MutableSequence . (Contributed by Mike Zimin in gh-114894 .)
The constructors of node types in the ast module are now stricter in the arguments they accept, and have more intuitive behaviour when arguments are omitted.
If an optional field on an AST node is not included as an argument when constructing an instance, the field will now be set to None . Similarly, if a list field is omitted, that field will now be set to an empty list, and if a ast.expr_context field is omitted, it defaults to Load() . (Previously, in all cases, the attribute would be missing on the newly constructed AST node instance.)
If other arguments are omitted, a DeprecationWarning is emitted. This will cause an exception in Python 3.15. Similarly, passing a keyword argument that does not map to a field on the AST node is now deprecated, and will raise an exception in Python 3.15.
These changes do not apply to user-defined subclasses of ast.AST , unless the class opts in to the new behavior by setting the attribute ast.AST._field_types .
(Contributed by Jelle Zijlstra in gh-105858 , gh-117486 , and gh-118851 .)
ast.parse() now accepts an optional argument optimize which is passed on to the compile() built-in. This makes it possible to obtain an optimized AST. (Contributed by Irit Katriel in gh-108113 .)
asyncio.loop.create_unix_server() will now automatically remove the Unix socket when the server is closed. (Contributed by Pierre Ossman in gh-111246 .)
asyncio.DatagramTransport.sendto() will now send zero-length datagrams if called with an empty bytes object. The transport flow control also now accounts for the datagram header when calculating the buffer size. (Contributed by Jamie Phan in gh-115199 .)
Add asyncio.Server.close_clients() and asyncio.Server.abort_clients() methods which allow to more forcefully close an asyncio server. (Contributed by Pierre Ossman in gh-113538 .)
asyncio.as_completed() now returns an object that is both an asynchronous iterator and a plain iterator of awaitables. The awaitables yielded by asynchronous iteration include original task or future objects that were passed in, making it easier to associate results with the tasks being completed. (Contributed by Justin Arthur in gh-77714 .)
When asyncio.TaskGroup.create_task() is called on an inactive asyncio.TaskGroup , the given coroutine will be closed (which prevents a RuntimeWarning about the given coroutine being never awaited). (Contributed by Arthur Tacca and Jason Zhang in gh-115957 .)
Improved behavior of asyncio.TaskGroup when an external cancellation collides with an internal cancellation. For example, when two task groups are nested and both experience an exception in a child task simultaneously, it was possible that the outer task group would hang, because its internal cancellation was swallowed by the inner task group.
In the case where a task group is cancelled externally and also must raise an ExceptionGroup , it will now call the parent task’s cancel() method. This ensures that a asyncio.CancelledError will be raised at the next await , so the cancellation is not lost.
An added benefit of these changes is that task groups now preserve the cancellation count ( asyncio.Task.cancelling() ).
In order to handle some corner cases, asyncio.Task.uncancel() may now reset the undocumented _must_cancel flag when the cancellation count reaches zero.
(Inspired by an issue reported by Arthur Tacca in gh-116720 .)
Add asyncio.Queue.shutdown() (along with asyncio.QueueShutDown ) for queue termination. (Contributed by Laurie Opperman and Yves Duprat in gh-104228 .)
Accept a tuple of separators in asyncio.StreamReader.readuntil() , stopping when one of them is encountered. (Contributed by Bruce Merry in gh-81322 .)
Add base64.z85encode() and base64.z85decode() functions which allow encoding and decoding Z85 data. See Z85 specification for more information. (Contributed by Matan Perelman in gh-75299 .)
Add copy.replace() function which allows to create a modified copy of an object, which is especially useful for immutable objects. It supports named tuples created with the factory function collections.namedtuple() , dataclass instances, various datetime objects, Signature objects, Parameter objects, code object , and any user classes which define the __replace__() method. (Contributed by Serhiy Storchaka in gh-108751 .)
Add dbm.gnu.gdbm.clear() and dbm.ndbm.ndbm.clear() methods that remove all items from the database. (Contributed by Donghee Na in gh-107122 .)
Add new dbm.sqlite3 backend, and make it the default dbm backend. (Contributed by Raymond Hettinger and Erlend E. Aasland in gh-100414 .)
Change the output of dis module functions to show logical labels for jump targets and exception handlers, rather than offsets. The offsets can be added with the new -O command line option or the show_offsets parameter. (Contributed by Irit Katriel in gh-112137 .)
Color is added to the output by default. This can be controlled via the new PYTHON_COLORS environment variable as well as the canonical NO_COLOR and FORCE_COLOR environment variables. See also Controlling color . (Contributed by Hugo van Kemenade in gh-117225 .)
The doctest.DocTestRunner.run() method now counts the number of skipped tests. Add doctest.DocTestRunner.skips and doctest.TestResults.skipped attributes. (Contributed by Victor Stinner in gh-108794 .)
email.utils.getaddresses() and email.utils.parseaddr() now return ('', '') 2-tuples in more situations where invalid email addresses are encountered instead of potentially inaccurate values. Add optional strict parameter to these two functions: use strict=False to get the old behavior, accept malformed inputs. getattr(email.utils, 'supports_strict_parsing', False) can be used to check if the strict parameter is available. (Contributed by Thomas Dwyer and Victor Stinner for gh-102988 to improve the CVE-2023-27043 fix.)
fractions ¶
Formatting for objects of type fractions.Fraction now supports the standard format specification mini-language rules for fill, alignment, sign handling, minimum width and grouping. (Contributed by Mark Dickinson in gh-111320 .)
The cyclic garbage collector is now incremental, which changes the meanings of the results of gc.get_threshold() and gc.set_threshold() as well as gc.get_count() and gc.get_stats() .
gc.get_threshold() returns a three-item tuple for backwards compatibility. The first value is the threshold for young collections, as before; the second value determines the rate at which the old collection is scanned (the default is 10, and higher values mean that the old collection is scanned more slowly). The third value is meaningless and is always zero.
gc.set_threshold() ignores any items after the second.
gc.get_count() and gc.get_stats() return the same format of results as before. The only difference is that instead of the results referring to the young, aging and old generations, the results refer to the young generation and the aging and collecting spaces of the old generation.
In summary, code that attempted to manipulate the behavior of the cycle GC may not work exactly as intended, but it is very unlikely to be harmful. All other code will work just fine.
Add glob.translate() function that converts a path specification with shell-style wildcards to a regular expression. (Contributed by Barney Gale in gh-72904 .)
importlib ¶
Previously deprecated importlib.resources functions are un-deprecated:
is_resource() open_binary() open_text() path() read_binary() read_text()
All now allow for a directory (or tree) of resources, using multiple positional arguments.
For text-reading functions, the encoding and errors must now be given as keyword arguments.
The contents() remains deprecated in favor of the full-featured Traversable API. However, there is now no plan to remove it.
(Contributed by Petr Viktorin in gh-106532 .)
The io.IOBase finalizer now logs the close() method errors with sys.unraisablehook . Previously, errors were ignored silently by default, and only logged in Python Development Mode or on Python built on debug mode . (Contributed by Victor Stinner in gh-62948 .)
ipaddress ¶
Add the ipaddress.IPv4Address.ipv6_mapped property, which returns the IPv4-mapped IPv6 address. (Contributed by Charles Machalow in gh-109466 .)
Fix is_global and is_private behavior in IPv4Address , IPv6Address , IPv4Network and IPv6Network .
itertools ¶
Added a strict option to itertools.batched() . This raises a ValueError if the final batch is shorter than the specified batch size. (Contributed by Raymond Hettinger in gh-113202 .)
Add the allow_code parameter in module functions. Passing allow_code=False prevents serialization and de-serialization of code objects which are incompatible between Python versions. (Contributed by Serhiy Storchaka in gh-113626 .)
A new function fma() for fused multiply-add operations has been added. This function computes x * y + z with only a single round, and so avoids any intermediate loss of precision. It wraps the fma() function provided by C99, and follows the specification of the IEEE 754 “fusedMultiplyAdd” operation for special cases. (Contributed by Mark Dickinson and Victor Stinner in gh-73468 .)
mimetypes ¶
Add the guess_file_type() function which works with file path. Passing file path instead of URL in guess_type() is soft deprecated . (Contributed by Serhiy Storchaka in gh-66543 .)
The mmap.mmap class now has an seekable() method that can be used when a seekable file-like object is required. The seek() method now returns the new absolute position. (Contributed by Donghee Na and Sylvie Liberman in gh-111835 .)
mmap.mmap now has a trackfd parameter on Unix; if it is False , the file descriptor specified by fileno will not be duplicated. (Contributed by Zackery Spytz and Petr Viktorin in gh-78502 .)
mmap.mmap is now protected from crashing on Windows when the mapped memory is inaccessible due to file system errors or access violations. (Contributed by Jannis Weigend in gh-118209 .)
Move opcode.ENABLE_SPECIALIZATION to _opcode.ENABLE_SPECIALIZATION . This field was added in 3.12, it was never documented and is not intended for external usage. (Contributed by Irit Katriel in gh-105481 .)
Removed opcode.is_pseudo , opcode.MIN_PSEUDO_OPCODE and opcode.MAX_PSEUDO_OPCODE , which were added in 3.12, were never documented or exposed through dis , and were not intended to be used externally.
Add os.process_cpu_count() function to get the number of logical CPUs usable by the calling thread of the current process. (Contributed by Victor Stinner in gh-109649 .)
Add a low level interface for Linux’s timer notification file descriptors via os.timerfd_create() , os.timerfd_settime() , os.timerfd_settime_ns() , os.timerfd_gettime() , and os.timerfd_gettime_ns() , os.TFD_NONBLOCK , os.TFD_CLOEXEC , os.TFD_TIMER_ABSTIME , and os.TFD_TIMER_CANCEL_ON_SET (Contributed by Masaru Tsuchiyama in gh-108277 .)
os.cpu_count() and os.process_cpu_count() can be overridden through the new environment variable PYTHON_CPU_COUNT or the new command-line option -X cpu_count . This option is useful for users who need to limit CPU resources of a container system without having to modify the container (application code). (Contributed by Donghee Na in gh-109595 .)
Add support of os.lchmod() and the follow_symlinks argument in os.chmod() on Windows. Note that the default value of follow_symlinks in os.lchmod() is False on Windows. (Contributed by Serhiy Storchaka in gh-59616 .)
Add support of os.fchmod() and a file descriptor in os.chmod() on Windows. (Contributed by Serhiy Storchaka in gh-113191 .)
os.posix_spawn() now accepts env=None , which makes the newly spawned process use the current process environment. (Contributed by Jakub Kulik in gh-113119 .)
os.posix_spawn() gains an os.POSIX_SPAWN_CLOSEFROM attribute for use in file_actions= on platforms that support posix_spawn_file_actions_addclosefrom_np() . (Contributed by Jakub Kulik in gh-113117 .)
os.mkdir() and os.makedirs() on Windows now support passing a mode value of 0o700 to apply access control to the new directory. This implicitly affects tempfile.mkdtemp() and is a mitigation for CVE-2024-4030 . Other values for mode continue to be ignored. (Contributed by Steve Dower in gh-118486 .)
Add os.path.isreserved() to check if a path is reserved on the current system. This function is only available on Windows. (Contributed by Barney Gale in gh-88569 .)
On Windows, os.path.isabs() no longer considers paths starting with exactly one (back)slash to be absolute. (Contributed by Barney Gale and Jon Foster in gh-44626 .)
Add support of dir_fd and follow_symlinks keyword arguments in shutil.chown() . (Contributed by Berker Peksag and Tahia K in gh-62308 )
Add pathlib.UnsupportedOperation , which is raised instead of NotImplementedError when a path operation isn’t supported. (Contributed by Barney Gale in gh-89812 .)
Add pathlib.Path.from_uri() , a new constructor to create a pathlib.Path object from a ‘file’ URI ( file:// ). (Contributed by Barney Gale in gh-107465 .)
Add pathlib.PurePath.full_match() for matching paths with shell-style wildcards, including the recursive wildcard “ ** ”. (Contributed by Barney Gale in gh-73435 .)
Add pathlib.PurePath.parser class attribute that stores the implementation of os.path used for low-level path parsing and joining: either posixpath or ntpath .
Add recurse_symlinks keyword-only argument to pathlib.Path.glob() and rglob() . (Contributed by Barney Gale in gh-77609 .)
Add follow_symlinks keyword-only argument to is_file() , is_dir() , owner() , group() . (Contributed by Barney Gale in gh-105793 , and Kamil Turek in gh-107962 .)
Return files and directories from pathlib.Path.glob() and rglob() when given a pattern that ends with “ ** ”. In earlier versions, only directories were returned. (Contributed by Barney Gale in gh-70303 .)
Add ability to move between chained exceptions during post mortem debugging in pm() using the new exceptions [exc_number] command for Pdb. (Contributed by Matthias Bussonnier in gh-106676 .)
Expressions/statements whose prefix is a pdb command are now correctly identified and executed. (Contributed by Tian Gao in gh-108464 .)
sys.path[0] will no longer be replaced by the directory of the script being debugged when sys.flags.safe_path is set (via the -P command line option or PYTHONSAFEPATH environment variable). (Contributed by Tian Gao and Christian Walther in gh-111762 .)
zipapp is supported as a debugging target. (Contributed by Tian Gao in gh-118501 .)
breakpoint() and pdb.set_trace() now enter the debugger immediately rather than on the next line of code to be executed. This change prevents the debugger from breaking outside of the context when breakpoint() is positioned at the end of the context. (Contributed by Tian Gao in gh-118579 .)
Add queue.Queue.shutdown() (along with queue.ShutDown ) for queue termination. (Contributed by Laurie Opperman and Yves Duprat in gh-104750 .)
Add a command-line interface . (Contributed by Hugo van Kemenade in gh-54321 .)
Rename re.error to re.PatternError for improved clarity. re.error is kept for backward compatibility.
.pth files are now decoded by UTF-8 first, and then by the locale encoding if the UTF-8 decoding fails. (Contributed by Inada Naoki in gh-117802 .)
A ResourceWarning is now emitted if a sqlite3.Connection object is not closed explicitly. (Contributed by Erlend E. Aasland in gh-105539 .)
Add filter keyword-only parameter to sqlite3.Connection.iterdump() for filtering database objects to dump. (Contributed by Mariusz Felisiak in gh-91602 .)
statistics ¶
Add statistics.kde() for kernel density estimation. This makes it possible to estimate a continuous probability density function from a fixed number of discrete samples. Also added statistics.kde_random() for sampling from the estimated probability density function. (Contributed by Raymond Hettinger in gh-115863 .)
subprocess ¶
The subprocess module now uses the os.posix_spawn() function in more situations. Notably in the default case of close_fds=True on more recent versions of platforms including Linux, FreeBSD, and Solaris where the C library provides posix_spawn_file_actions_addclosefrom_np() . On Linux this should perform similar to our existing Linux vfork() based code. A private control knob subprocess._USE_POSIX_SPAWN can be set to False if you need to force subprocess not to ever use os.posix_spawn() . Please report your reason and platform details in the CPython issue tracker if you set this so that we can improve our API selection logic for everyone. (Contributed by Jakub Kulik in gh-113117 .)
Add the sys._is_interned() function to test if the string was interned. This function is not guaranteed to exist in all implementations of Python. (Contributed by Serhiy Storchaka in gh-78573 .)
On Windows, the default mode 0o700 used by tempfile.mkdtemp() now limits access to the new directory due to changes to os.mkdir() . This is a mitigation for CVE-2024-4030 . (Contributed by Steve Dower in gh-118486 .)
On Windows, time.monotonic() now uses the QueryPerformanceCounter() clock to have a resolution better than 1 us, instead of the GetTickCount64() clock which has a resolution of 15.6 ms. (Contributed by Victor Stinner in gh-88494 .)
On Windows, time.time() now uses the GetSystemTimePreciseAsFileTime() clock to have a resolution better than 1 μs, instead of the GetSystemTimeAsFileTime() clock which has a resolution of 15.6 ms. (Contributed by Victor Stinner in gh-63207 .)
Add tkinter widget methods: tk_busy_hold() , tk_busy_configure() , tk_busy_cget() , tk_busy_forget() , tk_busy_current() , and tk_busy_status() . (Contributed by Miguel, klappnase and Serhiy Storchaka in gh-72684 .)
The tkinter widget method wm_attributes() now accepts the attribute name without the minus prefix to get window attributes, e.g. w.wm_attributes('alpha') and allows to specify attributes and values to set as keyword arguments, e.g. w.wm_attributes(alpha=0.5) . Add new optional keyword-only parameter return_python_dict : calling w.wm_attributes(return_python_dict=True) returns the attributes as a dict instead of a tuple. (Contributed by Serhiy Storchaka in gh-43457 .)
Add new optional keyword-only parameter return_ints in the Text.count() method. Passing return_ints=True makes it always returning the single count as an integer instead of a 1-tuple or None . (Contributed by Serhiy Storchaka in gh-97928 .)
Add support of the “vsapi” element type in the element_create() method of tkinter.ttk.Style . (Contributed by Serhiy Storchaka in gh-68166 .)
Add the after_info() method for Tkinter widgets. (Contributed by Cheryl Sabella in gh-77020 .)
Add the PhotoImage method copy_replace() to copy a region from one image to other image, possibly with pixel zooming and/or subsampling. Add from_coords parameter to PhotoImage methods copy() , zoom() and subsample() . Add zoom and subsample parameters to PhotoImage method copy() . (Contributed by Serhiy Storchaka in gh-118225 .)
Add the PhotoImage methods read() to read an image from a file and data() to get the image data. Add background and grayscale parameters to PhotoImage method write() . (Contributed by Serhiy Storchaka in gh-118271 .)
traceback ¶
Add show_group parameter to traceback.TracebackException.format_exception_only() to format the nested exceptions of a BaseExceptionGroup instance, recursively. (Contributed by Irit Katriel in gh-105292 .)
Add the field exc_type_str to TracebackException , which holds a string display of the exc_type . Deprecate the field exc_type which holds the type object itself. Add parameter save_exc_type (default True ) to indicate whether exc_type should be saved. (Contributed by Irit Katriel in gh-112332 .)
SimpleNamespace constructor now allows specifying initial values of attributes as a positional argument which must be a mapping or an iterable of key-value pairs. (Contributed by Serhiy Storchaka in gh-108191 .)
Add typing.get_protocol_members() to return the set of members defining a typing.Protocol . Add typing.is_protocol() to check whether a class is a typing.Protocol . (Contributed by Jelle Zijlstra in gh-104873 .)
Add typing.ReadOnly , a special typing construct to mark an item of a typing.TypedDict as read-only for type checkers. See PEP 705 for more details.
Add typing.NoDefault , a sentinel object used to represent the defaults of some parameters in the typing module. (Contributed by Jelle Zijlstra in gh-116126 .)
unicodedata ¶
The Unicode database has been updated to version 15.1.0. (Contributed by James Gerity in gh-109559 .)
Add support for adding source control management (SCM) ignore files to a virtual environment’s directory. By default, Git is supported. This is implemented as opt-in via the API which can be extended to support other SCMs ( venv.EnvBuilder and venv.create() ), and opt-out via the CLI (using --without-scm-ignore-files ). (Contributed by Brett Cannon in gh-108125 .)
The new warnings.deprecated() decorator provides a way to communicate deprecations to static type checkers and to warn on usage of deprecated classes and functions. A runtime deprecation warning may also be emitted when a decorated function or class is used at runtime. See PEP 702 . (Contributed by Jelle Zijlstra in gh-104003 .)
xml.etree.ElementTree ¶
Add the close() method for the iterator returned by iterparse() for explicit cleaning up. (Contributed by Serhiy Storchaka in gh-69893 .)
zipimport ¶
Gains support for ZIP64 format files. Everybody loves huge code right? (Contributed by Tim Hatch in gh-94146 .)
Optimizations ¶
textwrap.indent() is now ~30% faster than before for large input. (Contributed by Inada Naoki in gh-107369 .)
The subprocess module uses os.posix_spawn() in more situations including the default where close_fds=True on many modern platforms. This should provide a noteworthy performance increase launching processes on FreeBSD and Solaris. See the subprocess section above for details. (Contributed by Jakub Kulik in gh-113117 .)
Several standard library modules have had their import times significantly improved. For example, the import time of the typing module has been reduced by around a third by removing dependencies on re and contextlib . Other modules to enjoy import-time speedups include importlib.metadata , threading , enum , functools and email.utils . (Contributed by Alex Waygood, Shantanu Jain, Adam Turner, Daniel Hollas and others in gh-109653 .)
Removed Modules And APIs ¶
Pep 594: dead batteries (and other module removals) ¶.
PEP 594 removed 19 modules from the standard library, deprecated in Python 3.11:
aifc . (Contributed by Victor Stinner in gh-104773 .)
audioop . (Contributed by Victor Stinner in gh-104773 .)
chunk . (Contributed by Victor Stinner in gh-104773 .)
cgi and cgitb .
cgi.FieldStorage can typically be replaced with urllib.parse.parse_qsl() for GET and HEAD requests, and the email.message module or multipart PyPI project for POST and PUT .
cgi.parse() can be replaced by calling urllib.parse.parse_qs() directly on the desired query string, except for multipart/form-data input, which can be handled as described for cgi.parse_multipart() .
cgi.parse_header() can be replaced with the functionality in the email package, which implements the same MIME RFCs. For example, with email.message.EmailMessage :
cgi.parse_multipart() can be replaced with the functionality in the email package (e.g. email.message.EmailMessage and email.message.Message ) which implements the same MIME RFCs, or with the multipart PyPI project.
(Contributed by Victor Stinner in gh-104773 .)
crypt module and its private _crypt extension. The hashlib module is a potential replacement for certain use cases. Otherwise, the following PyPI projects can be used:
bcrypt : Modern password hashing for your software and your servers.
passlib : Comprehensive password hashing framework supporting over 30 schemes.
argon2-cffi : The secure Argon2 password hashing algorithm.
legacycrypt : ctypes wrapper to the POSIX crypt library call and associated functionality.
crypt_r : Fork of the crypt module, wrapper to the crypt_r(3) library call and associated functionality.
imghdr : use the projects filetype , puremagic , or python-magic instead. (Contributed by Victor Stinner in gh-104773 .)
mailcap . The mimetypes module provides an alternative. (Contributed by Victor Stinner in gh-104773 .)
msilib . (Contributed by Zachary Ware in gh-104773 .)
nis . (Contributed by Victor Stinner in gh-104773 .)
nntplib : the nntplib PyPI project can be used instead. (Contributed by Victor Stinner in gh-104773 .)
ossaudiodev : use the pygame project for audio playback. (Contributed by Victor Stinner in gh-104780 .)
pipes : use the subprocess module instead. (Contributed by Victor Stinner in gh-104773 .)
sndhdr : use the projects filetype , puremagic , or python-magic instead. (Contributed by Victor Stinner in gh-104773 .)
spwd : the python-pam project can be used instead. (Contributed by Victor Stinner in gh-104773 .)
sunau . (Contributed by Victor Stinner in gh-104773 .)
telnetlib , use the projects telnetlib3 ` or :pypi:`Exscript instead. (Contributed by Victor Stinner in gh-104773 .)
uu : the base64 module is a modern alternative. (Contributed by Victor Stinner in gh-104773 .)
xdrlib . (Contributed by Victor Stinner in gh-104773 .)
Remove the 2to3 program and the lib2to3 module, deprecated in Python 3.11. (Contributed by Victor Stinner in gh-104780 .)
Remove the tkinter.tix module, deprecated in Python 3.6. The third-party Tix library which the module wrapped is unmaintained. (Contributed by Zachary Ware in gh-75552 .)
configparser ¶
Remove the undocumented configparser.LegacyInterpolation class, deprecated in the docstring since Python 3.2, and with a deprecation warning since Python 3.11. (Contributed by Hugo van Kemenade in gh-104886 .)
Remove deprecated __getitem__() access for importlib.metadata.EntryPoint objects. (Contributed by Jason R. Coombs in gh-113175 .)
Remove locale.resetlocale() function deprecated in Python 3.11: use locale.setlocale(locale.LC_ALL, "") instead. (Contributed by Victor Stinner in gh-104783 .)
logging : Remove undocumented and untested Logger.warn() and LoggerAdapter.warn() methods and logging.warn() function. Deprecated since Python 3.3, they were aliases to the logging.Logger.warning() method, logging.LoggerAdapter.warning() method and logging.warning() function. (Contributed by Victor Stinner in gh-105376 .)
Remove support for using pathlib.Path objects as context managers. This functionality was deprecated and made a no-op in Python 3.9.
Remove undocumented, never working, and deprecated re.template function and re.TEMPLATE flag (and re.T alias). (Contributed by Serhiy Storchaka and Nikita Sobolev in gh-105687 .)
Remove the turtle.RawTurtle.settiltangle() method, deprecated in docs since Python 3.1 and with a deprecation warning since Python 3.11. (Contributed by Hugo van Kemenade in gh-104876 .)
Namespaces typing.io and typing.re , deprecated in Python 3.8, are now removed. The items in those namespaces can be imported directly from typing . (Contributed by Sebastian Rittau in gh-92871 .)
Remove support for the keyword-argument method of creating typing.TypedDict types, deprecated in Python 3.11. (Contributed by Tomas Roun in gh-104786 .)
Remove the following unittest functions, deprecated in Python 3.11:
unittest.findTestCases()
unittest.makeSuite()
unittest.getTestCaseNames()
Use TestLoader methods instead:
unittest.TestLoader.loadTestsFromModule()
unittest.TestLoader.loadTestsFromTestCase()
unittest.TestLoader.getTestCaseNames()
(Contributed by Hugo van Kemenade in gh-104835 .)
Remove the untested and undocumented unittest.TestProgram.usageExit() method, deprecated in Python 3.11. (Contributed by Hugo van Kemenade in gh-104992 .)
Remove cafile , capath and cadefault parameters of the urllib.request.urlopen() function, deprecated in Python 3.6: pass the context parameter instead. Use ssl.SSLContext.load_cert_chain() to load specific certificates, or let ssl.create_default_context() select the system’s trusted CA certificates for you. (Contributed by Victor Stinner in gh-105382 .)
webbrowser ¶
Remove the untested and undocumented webbrowser MacOSX class, deprecated in Python 3.11. Use the MacOSXOSAScript class (introduced in Python 3.2) instead. (Contributed by Hugo van Kemenade in gh-104804 .)
Remove deprecated webbrowser.MacOSXOSAScript._name attribute. Use webbrowser.MacOSXOSAScript.name attribute instead. (Contributed by Nikita Sobolev in gh-105546 .)
New Deprecations ¶
Removed chained classmethod descriptors (introduced in gh-63272 ). This can no longer be used to wrap other descriptors such as property . The core design of this feature was flawed and caused a number of downstream problems. To “pass-through” a classmethod , consider using the __wrapped__ attribute that was added in Python 3.10. (Contributed by Raymond Hettinger in gh-89519 .)
array : array ’s 'u' format code, deprecated in docs since Python 3.3, emits DeprecationWarning since 3.13 and will be removed in Python 3.16. Use the 'w' format code instead. (Contributed by Hugo van Kemenade in gh-80480 .)
ctypes : Deprecate undocumented ctypes.SetPointerType() and ctypes.ARRAY() functions. Replace ctypes.ARRAY(item_type, size) with item_type * size . (Contributed by Victor Stinner in gh-105733 .)
decimal : Deprecate non-standard format specifier “N” for decimal.Decimal . It was not documented and only supported in the C implementation. (Contributed by Serhiy Storchaka in gh-89902 .)
dis : The dis.HAVE_ARGUMENT separator is deprecated. Check membership in hasarg instead. (Contributed by Irit Katriel in gh-109319 .)
Frame objects : Calling frame.clear() on a suspended frame raises RuntimeError (as has always been the case for an executing frame). (Contributed by Irit Katriel in gh-79932 .)
getopt and optparse modules: They are now soft deprecated : the argparse module should be used for new projects. Previously, the optparse module was already deprecated, its removal was not scheduled, and no warnings was emitted: so there is no change in practice. (Contributed by Victor Stinner in gh-106535 .)
gettext : Emit deprecation warning for non-integer numbers in gettext functions and methods that consider plural forms even if the translation was not found. (Contributed by Serhiy Storchaka in gh-88434 .)
glob : The undocumented glob.glob0() and glob.glob1() functions are deprecated. Use glob.glob() and pass a directory to its root_dir argument instead. (Contributed by Barney Gale in gh-117337 .)
http.server : http.server.CGIHTTPRequestHandler now emits a DeprecationWarning as it will be removed in 3.15. Process-based CGI HTTP servers have been out of favor for a very long time. This code was outdated, unmaintained, and rarely used. It has a high potential for both security and functionality bugs. This includes removal of the --cgi flag to the python -m http.server command line in 3.15.
mimetypes : Passing file path instead of URL in guess_type() is soft deprecated . Use guess_file_type() instead. (Contributed by Serhiy Storchaka in gh-66543 .)
re : Passing optional arguments maxsplit , count and flags in module-level functions re.split() , re.sub() and re.subn() as positional arguments is now deprecated. In future Python versions these parameters will be keyword-only . (Contributed by Serhiy Storchaka in gh-56166 .)
pathlib : pathlib.PurePath.is_reserved() is deprecated and scheduled for removal in Python 3.15. Use os.path.isreserved() to detect reserved paths on Windows.
platform : java_ver() is deprecated and will be removed in 3.15. It was largely untested, had a confusing API, and was only useful for Jython support. (Contributed by Nikita Sobolev in gh-116349 .)
pydoc : Deprecate undocumented pydoc.ispackage() function. (Contributed by Zackery Spytz in gh-64020 .)
sqlite3 : Passing more than one positional argument to sqlite3.connect() and the sqlite3.Connection constructor is deprecated. The remaining parameters will become keyword-only in Python 3.15.
Deprecate passing name, number of arguments, and the callable as keyword arguments for the following sqlite3.Connection APIs:
create_function()
create_aggregate()
Deprecate passing the callback callable by keyword for the following sqlite3.Connection APIs:
set_authorizer()
set_progress_handler()
set_trace_callback()
The affected parameters will become positional-only in Python 3.15.
(Contributed by Erlend E. Aasland in gh-107948 and gh-108278 .)
sys : sys._enablelegacywindowsfsencoding() function. Replace it with the PYTHONLEGACYWINDOWSFSENCODING environment variable. (Contributed by Inada Naoki in gh-73427 .)
tarfile : The undocumented and unused tarfile attribute of tarfile.TarFile is deprecated and scheduled for removal in Python 3.16.
traceback : The field exc_type of traceback.TracebackException is deprecated. Use exc_type_str instead.
Creating a typing.NamedTuple class using keyword arguments to denote the fields ( NT = NamedTuple("NT", x=int, y=int) ) is deprecated, and will be disallowed in Python 3.15. Use the class-based syntax or the functional syntax instead. (Contributed by Alex Waygood in gh-105566 .)
When using the functional syntax to create a typing.NamedTuple class or a typing.TypedDict class, failing to pass a value to the ‘fields’ parameter ( NT = NamedTuple("NT") or TD = TypedDict("TD") ) is deprecated. Passing None to the ‘fields’ parameter ( NT = NamedTuple("NT", None) or TD = TypedDict("TD", None) ) is also deprecated. Both will be disallowed in Python 3.15. To create a NamedTuple class with 0 fields, use class NT(NamedTuple): pass or NT = NamedTuple("NT", []) . To create a TypedDict class with 0 fields, use class TD(TypedDict): pass or TD = TypedDict("TD", {}) . (Contributed by Alex Waygood in gh-105566 and gh-105570 .)
typing.no_type_check_decorator() is deprecated, and scheduled for removal in Python 3.15. After eight years in the typing module, it has yet to be supported by any major type checkers. (Contributed by Alex Waygood in gh-106309 .)
typing.AnyStr is deprecated. In Python 3.16, it will be removed from typing.__all__ , and a DeprecationWarning will be emitted when it is imported or accessed. It will be removed entirely in Python 3.18. Use the new type parameter syntax instead. (Contributed by Michael The in gh-107116 .)
User-defined functions : Assignment to a function’s __code__ attribute where the new code object’s type does not match the function’s type, is deprecated. The different types are: plain function, generator, async generator and coroutine. (Contributed by Irit Katriel in gh-81137 .)
wave : Deprecate the getmark() , setmark() and getmarkers() methods of the wave.Wave_read and wave.Wave_write classes. They will be removed in Python 3.15. (Contributed by Victor Stinner in gh-105096 .)
Pending Removal in Python 3.14 ¶
argparse : The type , choices , and metavar parameters of argparse.BooleanOptionalAction are deprecated and will be removed in 3.14. (Contributed by Nikita Sobolev in gh-92248 .)
ast : The following features have been deprecated in documentation since Python 3.8, now cause a DeprecationWarning to be emitted at runtime when they are accessed or used, and will be removed in Python 3.14:
ast.NameConstant
ast.Ellipsis
Use ast.Constant instead. (Contributed by Serhiy Storchaka in gh-90953 .)
collections.abc : Deprecated ByteString . Prefer Sequence or Buffer . For use in typing, prefer a union, like bytes | bytearray , or collections.abc.Buffer . (Contributed by Shantanu Jain in gh-91896 .)
email : Deprecated the isdst parameter in email.utils.localtime() . (Contributed by Alan Williams in gh-72346 .)
importlib : __package__ and __cached__ will cease to be set or taken into consideration by the import system ( gh-97879 ).
importlib.abc deprecated classes:
importlib.abc.ResourceReader
importlib.abc.Traversable
importlib.abc.TraversableResources
Use importlib.resources.abc classes instead:
importlib.resources.abc.Traversable
importlib.resources.abc.TraversableResources
(Contributed by Jason R. Coombs and Hugo van Kemenade in gh-93963 .)
itertools had undocumented, inefficient, historically buggy, and inconsistent support for copy, deepcopy, and pickle operations. This will be removed in 3.14 for a significant reduction in code volume and maintenance burden. (Contributed by Raymond Hettinger in gh-101588 .)
multiprocessing : The default start method will change to a safer one on Linux, BSDs, and other non-macOS POSIX platforms where 'fork' is currently the default ( gh-84559 ). Adding a runtime warning about this was deemed too disruptive as the majority of code is not expected to care. Use the get_context() or set_start_method() APIs to explicitly specify when your code requires 'fork' . See Contexts and start methods .
pathlib : is_relative_to() and relative_to() : passing additional arguments is deprecated.
pkgutil : find_loader() and get_loader() now raise DeprecationWarning ; use importlib.util.find_spec() instead. (Contributed by Nikita Sobolev in gh-97850 .)
master_open() : use pty.openpty() .
slave_open() : use pty.openpty() .
shutil.rmtree() onerror parameter is deprecated in 3.12, and will be removed in 3.14: use the onexc parameter instead.
version and version_info .
execute() and executemany() if named placeholders are used and parameters is a sequence instead of a dict .
date and datetime adapter, date and timestamp converter: see the sqlite3 documentation for suggested replacement recipes.
types.CodeType : Accessing co_lnotab was deprecated in PEP 626 since 3.10 and was planned to be removed in 3.12, but it only got a proper DeprecationWarning in 3.12. May be removed in 3.14. (Contributed by Nikita Sobolev in gh-101866 .)
typing : ByteString , deprecated since Python 3.9, now causes a DeprecationWarning to be emitted when it is used.
urllib : urllib.parse.Quoter is deprecated: it was not intended to be a public API. (Contributed by Gregory P. Smith in gh-88168 .)
xml.etree.ElementTree : Testing the truth value of an Element is deprecated and will raise an exception in Python 3.14.
Pending Removal in Python 3.15 ¶
http.server.CGIHTTPRequestHandler will be removed along with its related --cgi flag to python -m http.server . It was obsolete and rarely used. No direct replacement exists. Anything is better than CGI to interface a web server with a request handler.
locale : locale.getdefaultlocale() was deprecated in Python 3.11 and originally planned for removal in Python 3.13 ( gh-90817 ), but removal has been postponed to Python 3.15. Use locale.setlocale() , locale.getencoding() and locale.getlocale() instead. (Contributed by Hugo van Kemenade in gh-111187 .)
threading : Passing any arguments to threading.RLock() is now deprecated. C version allows any numbers of args and kwargs, but they are just ignored. Python version does not allow any arguments. All arguments will be removed from threading.RLock() in Python 3.15. (Contributed by Nikita Sobolev in gh-102029 .)
typing.NamedTuple :
The undocumented keyword argument syntax for creating NamedTuple classes ( NT = NamedTuple("NT", x=int) ) is deprecated, and will be disallowed in 3.15. Use the class-based syntax or the functional syntax instead.
When using the functional syntax to create a NamedTuple class, failing to pass a value to the fields parameter ( NT = NamedTuple("NT") ) is deprecated. Passing None to the fields parameter ( NT = NamedTuple("NT", None) ) is also deprecated. Both will be disallowed in Python 3.15. To create a NamedTuple class with 0 fields, use class NT(NamedTuple): pass or NT = NamedTuple("NT", []) .
typing.TypedDict : When using the functional syntax to create a TypedDict class, failing to pass a value to the fields parameter ( TD = TypedDict("TD") ) is deprecated. Passing None to the fields parameter ( TD = TypedDict("TD", None) ) is also deprecated. Both will be disallowed in Python 3.15. To create a TypedDict class with 0 fields, use class TD(TypedDict): pass or TD = TypedDict("TD", {}) .
Pending Removal in Python 3.16 ¶
array.array 'u' type ( wchar_t ): use the 'w' type instead ( Py_UCS4 ).
Pending Removal in Future Versions ¶
The following APIs were deprecated in earlier Python versions and will be removed, although there is currently no date scheduled for their removal.
argparse : Nesting argument groups and nesting mutually exclusive groups are deprecated.
~bool , bitwise inversion on bool.
bool(NotImplemented) .
Generators: throw(type, exc, tb) and athrow(type, exc, tb) signature is deprecated: use throw(exc) and athrow(exc) instead, the single argument signature.
Currently Python accepts numeric literals immediately followed by keywords, for example 0in x , 1or x , 0if 1else 2 . It allows confusing and ambiguous expressions like [0x1for x in y] (which can be interpreted as [0x1 for x in y] or [0x1f or x in y] ). A syntax warning is raised if the numeric literal is immediately followed by one of keywords and , else , for , if , in , is and or . In a future release it will be changed to a syntax error. ( gh-87999 )
Support for __index__() and __int__() method returning non-int type: these methods will be required to return an instance of a strict subclass of int .
Support for __float__() method returning a strict subclass of float : these methods will be required to return an instance of float .
Support for __complex__() method returning a strict subclass of complex : these methods will be required to return an instance of complex .
Delegation of int() to __trunc__() method.
calendar : calendar.January and calendar.February constants are deprecated and replaced by calendar.JANUARY and calendar.FEBRUARY . (Contributed by Prince Roshan in gh-103636 .)
codeobject.co_lnotab : use the codeobject.co_lines() method instead.
utcnow() : use datetime.datetime.now(tz=datetime.UTC) .
utcfromtimestamp() : use datetime.datetime.fromtimestamp(timestamp, tz=datetime.UTC) .
gettext : Plural value must be an integer.
importlib :
load_module() method: use exec_module() instead.
cache_from_source() debug_override parameter is deprecated: use the optimization parameter instead.
importlib.metadata :
EntryPoints tuple interface.
Implicit None on return values.
mailbox : Use of StringIO input and text mode is deprecated, use BytesIO and binary mode instead.
os : Calling os.register_at_fork() in multi-threaded process.
pydoc.ErrorDuringImport : A tuple value for exc_info parameter is deprecated, use an exception instance.
re : More strict rules are now applied for numerical group references and group names in regular expressions. Only sequence of ASCII digits is now accepted as a numerical reference. The group name in bytes patterns and replacement strings can now only contain ASCII letters and digits and underscore. (Contributed by Serhiy Storchaka in gh-91760 .)
sre_compile , sre_constants and sre_parse modules.
ssl options and protocols:
ssl.SSLContext without protocol argument is deprecated.
ssl.SSLContext : set_npn_protocols() and selected_npn_protocol() are deprecated: use ALPN instead.
ssl.OP_NO_SSL* options
ssl.OP_NO_TLS* options
ssl.PROTOCOL_SSLv3
ssl.PROTOCOL_TLS
ssl.PROTOCOL_TLSv1
ssl.PROTOCOL_TLSv1_1
ssl.PROTOCOL_TLSv1_2
ssl.TLSVersion.SSLv3
ssl.TLSVersion.TLSv1
ssl.TLSVersion.TLSv1_1
sysconfig.is_python_build() check_home parameter is deprecated and ignored.
threading methods:
threading.Condition.notifyAll() : use notify_all() .
threading.Event.isSet() : use is_set() .
threading.Thread.isDaemon() , threading.Thread.setDaemon() : use threading.Thread.daemon attribute.
threading.Thread.getName() , threading.Thread.setName() : use threading.Thread.name attribute.
threading.currentThread() : use threading.current_thread() .
threading.activeCount() : use threading.active_count() .
typing.Text ( gh-92332 ).
unittest.IsolatedAsyncioTestCase : it is deprecated to return a value that is not None from a test case.
urllib.parse deprecated functions: urlparse() instead
splitattr()
splithost()
splitnport()
splitpasswd()
splitport()
splitquery()
splittype()
splituser()
splitvalue()
urllib.request : URLopener and FancyURLopener style of invoking requests is deprecated. Use newer urlopen() functions and methods.
wsgiref : SimpleHandler.stdout.write() should not do partial writes.
zipimport.zipimporter.load_module() is deprecated: use exec_module() instead.
CPython Bytecode Changes ¶
The oparg of YIELD_VALUE is now 1 if the yield is part of a yield-from or await, and 0 otherwise. The oparg of RESUME was changed to add a bit indicating whether the except-depth is 1, which is needed to optimize closing of generators. (Contributed by Irit Katriel in gh-111354 .)
C API Changes ¶
You no longer have to define the PY_SSIZE_T_CLEAN macro before including Python.h when using # formats in format codes . APIs accepting the format codes always use Py_ssize_t for # formats. (Contributed by Inada Naoki in gh-104922 .)
The keywords parameter of PyArg_ParseTupleAndKeywords() and PyArg_VaParseTupleAndKeywords() now has type char * const * in C and const char * const * in C++, instead of char * * . It makes these functions compatible with arguments of type const char * const * , const char * * or char * const * in C++ and char * const * in C without an explicit type cast. This can be overridden with the PY_CXX_CONST macro. (Contributed by Serhiy Storchaka in gh-65210 .)
Add PyImport_AddModuleRef() : similar to PyImport_AddModule() , but return a strong reference instead of a borrowed reference . (Contributed by Victor Stinner in gh-105922 .)
Add PyWeakref_GetRef() function: similar to PyWeakref_GetObject() but returns a strong reference , or NULL if the referent is no longer live. (Contributed by Victor Stinner in gh-105927 .)
Add PyObject_GetOptionalAttr() and PyObject_GetOptionalAttrString() , variants of PyObject_GetAttr() and PyObject_GetAttrString() which don’t raise AttributeError if the attribute is not found. These variants are more convenient and faster if the missing attribute should not be treated as a failure. (Contributed by Serhiy Storchaka in gh-106521 .)
Add PyMapping_GetOptionalItem() and PyMapping_GetOptionalItemString() : variants of PyObject_GetItem() and PyMapping_GetItemString() which don’t raise KeyError if the key is not found. These variants are more convenient and faster if the missing key should not be treated as a failure. (Contributed by Serhiy Storchaka in gh-106307 .)
Add fixed variants of functions which silently ignore errors:
PyObject_HasAttrWithError() replaces PyObject_HasAttr() .
PyObject_HasAttrStringWithError() replaces PyObject_HasAttrString() .
PyMapping_HasKeyWithError() replaces PyMapping_HasKey() .
PyMapping_HasKeyStringWithError() replaces PyMapping_HasKeyString() .
New functions return not only 1 for true and 0 for false, but also -1 for error.
(Contributed by Serhiy Storchaka in gh-108511 .)
If Python is built in debug mode or with assertions , PyTuple_SET_ITEM() and PyList_SET_ITEM() now check the index argument with an assertion. (Contributed by Victor Stinner in gh-106168 .)
Add PyModule_Add() function: similar to PyModule_AddObjectRef() and PyModule_AddObject() but always steals a reference to the value. (Contributed by Serhiy Storchaka in gh-86493 .)
Add PyDict_GetItemRef() and PyDict_GetItemStringRef() functions: similar to PyDict_GetItemWithError() but returning a strong reference instead of a borrowed reference . Moreover, these functions return -1 on error and so checking PyErr_Occurred() is not needed. (Contributed by Victor Stinner in gh-106004 .)
Added PyDict_SetDefaultRef() , which is similar to PyDict_SetDefault() but returns a strong reference instead of a borrowed reference . This function returns -1 on error, 0 on insertion, and 1 if the key was already present in the dictionary. (Contributed by Sam Gross in gh-112066 .)
Add PyDict_ContainsString() function: same as PyDict_Contains() , but key is specified as a const char * UTF-8 encoded bytes string, rather than a PyObject * . (Contributed by Victor Stinner in gh-108314 .)
Added PyList_GetItemRef() function: similar to PyList_GetItem() but returns a strong reference instead of a borrowed reference .
Add Py_IsFinalizing() function: check if the main Python interpreter is shutting down . (Contributed by Victor Stinner in gh-108014 .)
Add PyLong_AsInt() function: similar to PyLong_AsLong() , but store the result in a C int instead of a C long . Previously, it was known as the private function _PyLong_AsInt() (with an underscore prefix). (Contributed by Victor Stinner in gh-108014 .)
Python built with configure --with-trace-refs (tracing references) now supports the Limited API . (Contributed by Victor Stinner in gh-108634 .)
Add PyObject_VisitManagedDict() and PyObject_ClearManagedDict() functions which must be called by the traverse and clear functions of a type using Py_TPFLAGS_MANAGED_DICT flag. The pythoncapi-compat project can be used to get these functions on Python 3.11 and 3.12. (Contributed by Victor Stinner in gh-107073 .)
Add PyUnicode_EqualToUTF8AndSize() and PyUnicode_EqualToUTF8() functions: compare Unicode object with a const char * UTF-8 encoded string and return true ( 1 ) if they are equal, or false ( 0 ) otherwise. These functions do not raise exceptions. (Contributed by Serhiy Storchaka in gh-110289 .)
Add PyThreadState_GetUnchecked() function: similar to PyThreadState_Get() , but don’t kill the process with a fatal error if it is NULL. The caller is responsible to check if the result is NULL. Previously, the function was private and known as _PyThreadState_UncheckedGet() . (Contributed by Victor Stinner in gh-108867 .)
Add PySys_AuditTuple() function: similar to PySys_Audit() , but pass event arguments as a Python tuple object. (Contributed by Victor Stinner in gh-85283 .)
PyArg_ParseTupleAndKeywords() now supports non-ASCII keyword parameter names. (Contributed by Serhiy Storchaka in gh-110815 .)
Add PyMem_RawMalloc() , PyMem_RawCalloc() , PyMem_RawRealloc() and PyMem_RawFree() to the limited C API (version 3.13). (Contributed by Victor Stinner in gh-85283 .)
Add PySys_Audit() and PySys_AuditTuple() functions to the limited C API. (Contributed by Victor Stinner in gh-85283 .)
Add PyErr_FormatUnraisable() function: similar to PyErr_WriteUnraisable() , but allow customizing the warning message. (Contributed by Serhiy Storchaka in gh-108082 .)
Add PyList_Extend() and PyList_Clear() functions: similar to Python list.extend() and list.clear() methods. (Contributed by Victor Stinner in gh-111138 .)
Add PyDict_Pop() and PyDict_PopString() functions: remove a key from a dictionary and optionally return the removed value. This is similar to dict.pop() , but without the default value and not raising KeyError if the key is missing. (Contributed by Stefan Behnel and Victor Stinner in gh-111262 .)
Add Py_HashPointer() function to hash a pointer. (Contributed by Victor Stinner in gh-111545 .)
Add PyObject_GenericHash() function that implements the default hashing function of a Python object. (Contributed by Serhiy Storchaka in gh-113024 .)
Add PyTime C API:
PyTime_t type.
PyTime_MIN and PyTime_MAX constants.
Add functions:
PyTime_AsSecondsDouble() .
PyTime_Monotonic() .
PyTime_MonotonicRaw() .
PyTime_PerfCounter() .
PyTime_PerfCounterRaw() .
PyTime_Time() .
PyTime_TimeRaw() .
(Contributed by Victor Stinner and Petr Viktorin in gh-110850 .)
Add PyLong_AsNativeBytes() , PyLong_FromNativeBytes() and PyLong_FromUnsignedNativeBytes() functions to simplify converting between native integer types and Python int objects. (Contributed by Steve Dower in gh-111140 .)
Add PyType_GetFullyQualifiedName() function to get the type’s fully qualified name. Equivalent to f"{type.__module__}.{type.__qualname__}" , or type.__qualname__ if type.__module__ is not a string or is equal to "builtins" . (Contributed by Victor Stinner in gh-111696 .)
Add PyType_GetModuleName() function to get the type’s module name. Equivalent to getting the type.__module__ attribute. (Contributed by Eric Snow and Victor Stinner in gh-111696 .)
Add support for %T , %#T , %N and %#N formats to PyUnicode_FromFormat() : format the fully qualified name of an object type and of a type: call PyType_GetModuleName() . See PEP 737 for more information. (Contributed by Victor Stinner in gh-111696 .)
Add Py_GetConstant() and Py_GetConstantBorrowed() functions to get constants. For example, Py_GetConstant(Py_CONSTANT_ZERO) returns a strong reference to the constant zero. (Contributed by Victor Stinner in gh-115754 .)
Add PyType_GetModuleByDef() to the limited C API (Contributed by Victor Stinner in gh-116936 .)
Add two new functions to the C-API, PyRefTracer_SetTracer() and PyRefTracer_GetTracer() , that allows to track object creation and destruction the same way the tracemalloc module does. (Contributed by Pablo Galindo in gh-93502 .)
Build Changes ¶
The configure option --with-system-libmpdec now defaults to yes . The bundled copy of libmpdecimal will be removed in Python 3.15.
Autoconf 2.71 and aclocal 1.16.4 are now required to regenerate the configure script. (Contributed by Christian Heimes in gh-89886 .)
SQLite 3.15.2 or newer is required to build the sqlite3 extension module. (Contributed by Erlend Aasland in gh-105875 .)
Python built with configure --with-trace-refs (tracing references) is now ABI compatible with the Python release build and debug build . (Contributed by Victor Stinner in gh-108634 .)
Building CPython now requires a compiler with support for the C11 atomic library, GCC built-in atomic functions, or MSVC interlocked intrinsics.
The errno , fcntl , grp , md5 , pwd , resource , termios , winsound , _ctypes_test , _multiprocessing.posixshmem , _scproxy , _stat , _statistics , _testconsole , _testimportmultiple and _uuid C extensions are now built with the limited C API . (Contributed by Victor Stinner in gh-85283 .)
wasm32-wasi is now a PEP 11 tier 2 platform. (Contributed by Brett Cannon in gh-115192 .)
wasm32-emscripten is no longer a PEP 11 supported platform. (Contributed by Brett Cannon in gh-115192 .)
Python now bundles the mimalloc library . It is licensed under the MIT license; see mimalloc license . The bundled mimalloc has custom changes, see gh-113141 for details. (Contributed by Dino Viehland in gh-109914 .)
Porting to Python 3.13 ¶
This section lists previously described changes and other bugfixes that may require changes to your code.
Changes in the Python API ¶
An OSError is now raised by getpass.getuser() for any failure to retrieve a username, instead of ImportError on non-Unix platforms or KeyError on Unix platforms where the password database is empty.
The threading module now expects the _thread module to have an _is_main_interpreter attribute. It is a function with no arguments that returns True if the current interpreter is the main interpreter.
Any library or application that provides a custom _thread module must provide _is_main_interpreter() , just like the module’s other “private” attributes. (See gh-112826 .)
mailbox.Maildir now ignores files with a leading dot. (Contributed by Zackery Spytz in gh-65559 .)
pathlib.Path.glob() and rglob() now return both files and directories if a pattern that ends with “ ** ” is given, rather than directories only. Users may add a trailing slash to match only directories.
The value of the mode attribute of gzip.GzipFile was changed from integer ( 1 or 2 ) to string ( 'rb' or 'wb' ). The value of the mode attribute of the readable file-like object returned by zipfile.ZipFile.open() was changed from 'r' to 'rb' . (Contributed by Serhiy Storchaka in gh-115961 .)
Calling locals() in an optimized scope now produces an independent snapshot on each call, and hence no longer implicitly updates previously returned references. Obtaining the legacy CPython behaviour now requires explicit calls to update the initially returned dictionary with the results of subsequent calls to locals() . (Changed as part of PEP 667 .)
Calling locals() from a comprehension at module or class scope (including via exec or eval ) once more behaves as if the comprehension were running as an independent nested function (i.e. the local variables from the containing scope are not included). In Python 3.12, this had changed to include the local variables from the containing scope when implementing PEP 709 . (Changed as part of PEP 667 .)
Accessing FrameType.f_locals in an optimized scope now returns a write-through proxy rather than a snapshot that gets updated at ill-specified times. If a snapshot is desired, it must be created explicitly with dict or the proxy’s .copy() method. (Changed as part of PEP 667 .)
Changes in the C API ¶
Python.h no longer includes the <ieeefp.h> standard header. It was included for the finite() function which is now provided by the <math.h> header. It should now be included explicitly if needed. Remove also the HAVE_IEEEFP_H macro. (Contributed by Victor Stinner in gh-108765 .)
Python.h no longer includes these standard header files: <time.h> , <sys/select.h> and <sys/time.h> . If needed, they should now be included explicitly. For example, <time.h> provides the clock() and gmtime() functions, <sys/select.h> provides the select() function, and <sys/time.h> provides the futimes() , gettimeofday() and setitimer() functions. (Contributed by Victor Stinner in gh-108765 .)
On Windows, Python.h no longer includes the <stddef.h> standard header file. If needed, it should now be included explicitly. For example, it provides offsetof() function, and size_t and ptrdiff_t types. Including <stddef.h> explicitly was already needed by all other platforms, the HAVE_STDDEF_H macro is only defined on Windows. (Contributed by Victor Stinner in gh-108765 .)
If the Py_LIMITED_API macro is defined, Py_BUILD_CORE , Py_BUILD_CORE_BUILTIN and Py_BUILD_CORE_MODULE macros are now undefined by <Python.h> . (Contributed by Victor Stinner in gh-85283 .)
The old trashcan macros Py_TRASHCAN_SAFE_BEGIN and Py_TRASHCAN_SAFE_END were removed. They should be replaced by the new macros Py_TRASHCAN_BEGIN and Py_TRASHCAN_END .
A tp_dealloc function that has the old macros, such as:
should migrate to the new macros as follows:
Note that Py_TRASHCAN_BEGIN has a second argument which should be the deallocation function it is in. The new macros were added in Python 3.8 and the old macros were deprecated in Python 3.11. (Contributed by Irit Katriel in gh-105111 .)
Functions PyDict_GetItem() , PyDict_GetItemString() , PyMapping_HasKey() , PyMapping_HasKeyString() , PyObject_HasAttr() , PyObject_HasAttrString() , and PySys_GetObject() , which clear all errors which occurred when calling them, now report them using sys.unraisablehook() . You may replace them with other functions as recommended in the documentation. (Contributed by Serhiy Storchaka in gh-106672 .)
PyCode_GetFirstFree() is an unstable API now and has been renamed to PyUnstable_Code_GetFirstFree() . (Contributed by Bogdan Romanyuk in gh-115781 .)
Removed C APIs ¶
Remove many APIs (functions, macros, variables) with names prefixed by _Py or _PY (considered as private API). If your project is affected by one of these removals and you consider that the removed API should remain available, please open a new issue to request a public C API and add cc @vstinner to the issue to notify Victor Stinner. (Contributed by Victor Stinner in gh-106320 .)
Remove functions deprecated in Python 3.9:
PyEval_CallObject() , PyEval_CallObjectWithKeywords() : use PyObject_CallNoArgs() or PyObject_Call() instead. Warning: PyObject_Call() positional arguments must be a tuple and must not be NULL , keyword arguments must be a dict or NULL , whereas removed functions checked arguments type and accepted NULL positional and keyword arguments. To replace PyEval_CallObjectWithKeywords(func, NULL, kwargs) with PyObject_Call() , pass an empty tuple as positional arguments using PyTuple_New(0) .
PyEval_CallFunction() : use PyObject_CallFunction() instead.
PyEval_CallMethod() : use PyObject_CallMethod() instead.
PyCFunction_Call() : use PyObject_Call() instead.
(Contributed by Victor Stinner in gh-105107 .)
Remove old buffer protocols deprecated in Python 3.0. Use Buffer Protocol instead.
PyObject_CheckReadBuffer() : Use PyObject_CheckBuffer() to test if the object supports the buffer protocol. Note that PyObject_CheckBuffer() doesn’t guarantee that PyObject_GetBuffer() will succeed. To test if the object is actually readable, see the next example of PyObject_GetBuffer() .
PyObject_AsCharBuffer() , PyObject_AsReadBuffer() : PyObject_GetBuffer() and PyBuffer_Release() instead:
PyObject_AsWriteBuffer() : Use PyObject_GetBuffer() and PyBuffer_Release() instead:
(Contributed by Inada Naoki in gh-85275 .)
Remove the following old functions to configure the Python initialization, deprecated in Python 3.11:
PySys_AddWarnOptionUnicode() : use PyConfig.warnoptions instead.
PySys_AddWarnOption() : use PyConfig.warnoptions instead.
PySys_AddXOption() : use PyConfig.xoptions instead.
PySys_HasWarnOptions() : use PyConfig.xoptions instead.
PySys_SetPath() : set PyConfig.module_search_paths instead.
Py_SetPath() : set PyConfig.module_search_paths instead.
Py_SetStandardStreamEncoding() : set PyConfig.stdio_encoding instead, and set also maybe PyConfig.legacy_windows_stdio (on Windows).
_Py_SetProgramFullPath() : set PyConfig.executable instead.
Use the new PyConfig API of the Python Initialization Configuration instead ( PEP 587 ), added to Python 3.8. (Contributed by Victor Stinner in gh-105145 .)
Remove PyEval_ThreadsInitialized() function, deprecated in Python 3.9. Since Python 3.7, Py_Initialize() always creates the GIL: calling PyEval_InitThreads() does nothing and PyEval_ThreadsInitialized() always returned non-zero. (Contributed by Victor Stinner in gh-105182 .)
Remove PyEval_AcquireLock() and PyEval_ReleaseLock() functions, deprecated in Python 3.2. They didn’t update the current thread state. They can be replaced with:
PyEval_SaveThread() and PyEval_RestoreThread() ;
low-level PyEval_AcquireThread() and PyEval_RestoreThread() ;
or PyGILState_Ensure() and PyGILState_Release() .
(Contributed by Victor Stinner in gh-105182 .)
Remove private _PyObject_FastCall() function: use PyObject_Vectorcall() which is available since Python 3.8 ( PEP 590 ). (Contributed by Victor Stinner in gh-106023 .)
Remove cpython/pytime.h header file: it only contained private functions. (Contributed by Victor Stinner in gh-106316 .)
Remove _PyInterpreterState_Get() alias to PyInterpreterState_Get() which was kept for backward compatibility with Python 3.8. The pythoncapi-compat project can be used to get PyInterpreterState_Get() on Python 3.8 and older. (Contributed by Victor Stinner in gh-106320 .)
The PyModule_AddObject() function is now soft deprecated : PyModule_Add() or PyModule_AddObjectRef() functions should be used instead. (Contributed by Serhiy Storchaka in gh-86493 .)
Deprecated C APIs ¶
Deprecate the old Py_UNICODE and PY_UNICODE_TYPE types: use directly the wchar_t type instead. Since Python 3.3, Py_UNICODE and PY_UNICODE_TYPE are just aliases to wchar_t . (Contributed by Victor Stinner in gh-105156 .)
Deprecate old Python initialization functions:
PySys_ResetWarnOptions() : clear sys.warnoptions and warnings.filters instead.
Py_GetExecPrefix() : get sys.exec_prefix instead.
Py_GetPath() : get sys.path instead.
Py_GetPrefix() : get sys.prefix instead.
Py_GetProgramFullPath() : get sys.executable instead.
Py_GetProgramName() : get sys.executable instead.
Py_GetPythonHome() : get PyConfig.home or PYTHONHOME environment variable instead.
Functions scheduled for removal in Python 3.15. (Contributed by Victor Stinner in gh-105145 .)
Deprecate the PyImport_ImportModuleNoBlock() function which is just an alias to PyImport_ImportModule() since Python 3.3. Scheduled for removal in Python 3.15. (Contributed by Victor Stinner in gh-105396 .)
Deprecate the PyWeakref_GetObject() and PyWeakref_GET_OBJECT() functions, which return a borrowed reference : use the new PyWeakref_GetRef() function instead, it returns a strong reference . The pythoncapi-compat project can be used to get PyWeakref_GetRef() on Python 3.12 and older. (Contributed by Victor Stinner in gh-105927 .)
Creating immutable types ( Py_TPFLAGS_IMMUTABLETYPE ) with mutable bases using the C API.
Functions to configure the Python initialization, deprecated in Python 3.11:
PySys_SetArgvEx() : set PyConfig.argv instead.
PySys_SetArgv() : set PyConfig.argv instead.
Py_SetProgramName() : set PyConfig.program_name instead.
Py_SetPythonHome() : set PyConfig.home instead.
The Py_InitializeFromConfig() API should be used with PyConfig instead.
Global configuration variables:
Py_DebugFlag : use PyConfig.parser_debug
Py_VerboseFlag : use PyConfig.verbose
Py_QuietFlag : use PyConfig.quiet
Py_InteractiveFlag : use PyConfig.interactive
Py_InspectFlag : use PyConfig.inspect
Py_OptimizeFlag : use PyConfig.optimization_level
Py_NoSiteFlag : use PyConfig.site_import
Py_BytesWarningFlag : use PyConfig.bytes_warning
Py_FrozenFlag : use PyConfig.pathconfig_warnings
Py_IgnoreEnvironmentFlag : use PyConfig.use_environment
Py_DontWriteBytecodeFlag : use PyConfig.write_bytecode
Py_NoUserSiteDirectory : use PyConfig.user_site_directory
Py_UnbufferedStdioFlag : use PyConfig.buffered_stdio
Py_HashRandomizationFlag : use PyConfig.use_hash_seed and PyConfig.hash_seed
Py_IsolatedFlag : use PyConfig.isolated
Py_LegacyWindowsFSEncodingFlag : use PyPreConfig.legacy_windows_fs_encoding
Py_LegacyWindowsStdioFlag : use PyConfig.legacy_windows_stdio
Py_FileSystemDefaultEncoding : use PyConfig.filesystem_encoding
Py_HasFileSystemDefaultEncoding : use PyConfig.filesystem_encoding
Py_FileSystemDefaultEncodeErrors : use PyConfig.filesystem_errors
Py_UTF8Mode : use PyPreConfig.utf8_mode (see Py_PreInitialize() )
The bundled copy of libmpdecimal .
PyImport_ImportModuleNoBlock() : use PyImport_ImportModule() .
PyWeakref_GET_OBJECT() : use PyWeakref_GetRef() instead.
PyWeakref_GetObject() : use PyWeakref_GetRef() instead.
Py_UNICODE_WIDE type: use wchar_t instead.
Py_UNICODE type: use wchar_t instead.
Python initialization functions:
Py_TPFLAGS_HAVE_FINALIZE : no needed since Python 3.8.
PyErr_Fetch() : use PyErr_GetRaisedException() .
PyErr_NormalizeException() : use PyErr_GetRaisedException() .
PyErr_Restore() : use PyErr_SetRaisedException() .
PyModule_GetFilename() : use PyModule_GetFilenameObject() .
PyOS_AfterFork() : use PyOS_AfterFork_Child() .
PySlice_GetIndicesEx() .
PyUnicode_AsDecodedObject() .
PyUnicode_AsDecodedUnicode() .
PyUnicode_AsEncodedObject() .
PyUnicode_AsEncodedUnicode() .
PyUnicode_READY() : not needed since Python 3.12.
_PyErr_ChainExceptions() .
PyBytesObject.ob_shash member: call PyObject_Hash() instead.
PyDictObject.ma_version_tag member.
PyThread_create_key() : use PyThread_tss_alloc() .
PyThread_delete_key() : use PyThread_tss_free() .
PyThread_set_key_value() : use PyThread_tss_set() .
PyThread_get_key_value() : use PyThread_tss_get() .
PyThread_delete_key_value() : use PyThread_tss_delete() .
PyThread_ReInitTLS() : no longer needed.
Remove undocumented PY_TIMEOUT_MAX constant from the limited C API. (Contributed by Victor Stinner in gh-110014 .)
Regression Test Changes ¶
Python built with configure --with-pydebug now supports a -X presite=package.module command-line option. If used, it specifies a module that should be imported early in the lifecycle of the interpreter, before site.py is executed. (Contributed by Łukasz Langa in gh-110769 .)
Table of Contents
- Summary – Release Highlights
- A Better Interactive Interpreter
- Improved Error Messages
- Defined mutation semantics for locals()
- Incremental Garbage Collection
- Support For Mobile Platforms
- Experimental JIT Compiler
- Free-threaded CPython
- Other Language Changes
- New Modules
- unicodedata
- xml.etree.ElementTree
- Optimizations
- PEP 594: dead batteries (and other module removals)
- configparser
- Pending Removal in Python 3.14
- Pending Removal in Python 3.15
- Pending Removal in Python 3.16
- Pending Removal in Future Versions
- CPython Bytecode Changes
- New Features
- Build Changes
- Changes in the Python API
- Changes in the C API
- Removed C APIs
- Deprecated C APIs
- Regression Test Changes
Previous topic
What’s New in Python
What’s New In Python 3.12
- Report a Bug
- Show Source
- Python Basics
- Interview Questions
Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- Python Turtle Tutorial
- Python Pillow Tutorial
- Python Tkinter Tutorial
- Python Requests Tutorial
- Selenium Python Tutorial
- Pygal Tutorial
- OpenCV Tutorial in Python
- Python Automation Tutorial
- Pandas Tutorial
- PyGame Tutorial
- Python Web Scraping Tutorial
- NumPy Tutorial - Python Library
- Advanced Python Topics Tutorial
- Python Syntax
- Flask Tutorial
- Python - Data visualization tutorial
- Machine Learning with Python Tutorial
- Python Tkinter - Label
- Python Tkinter - Message
Python Tutorial | Learn Python Programming
This Programming Language Python Tutorial is very well suited for beginners and also for experienced programmers. This specially designed free Python tutorial will help you learn Python programming most efficiently, with all topics from basics to advanced (like Web-scraping, Django, Learning, etc.) with examples.
What is Python?
Python is a high-level, general-purpose, and very popular programming language. Python programming language (latest Python 3) is being used in web development, and Machine Learning applications, along with all cutting-edge technology in Software Industry. Python language is being used by almost all tech-giant companies like – Google, Amazon, Facebook, Instagram, Dropbox, Uber… etc.
Writing your first Python Program to Learn Python Programming
There are two ways you can execute your Python program:
- First, we write a program in a file and run it one time.
- Second, run a code line by line.
Here we provided the latest Python 3 version compiler where you can edit and compile your written code directly with just one click of the RUN Button. So test yourself with Python first exercises.
Let us now see what will you learn in this Python Tutorial, in detail:
The first and foremost step to get started with Python tutorial is to setup Python in your system.
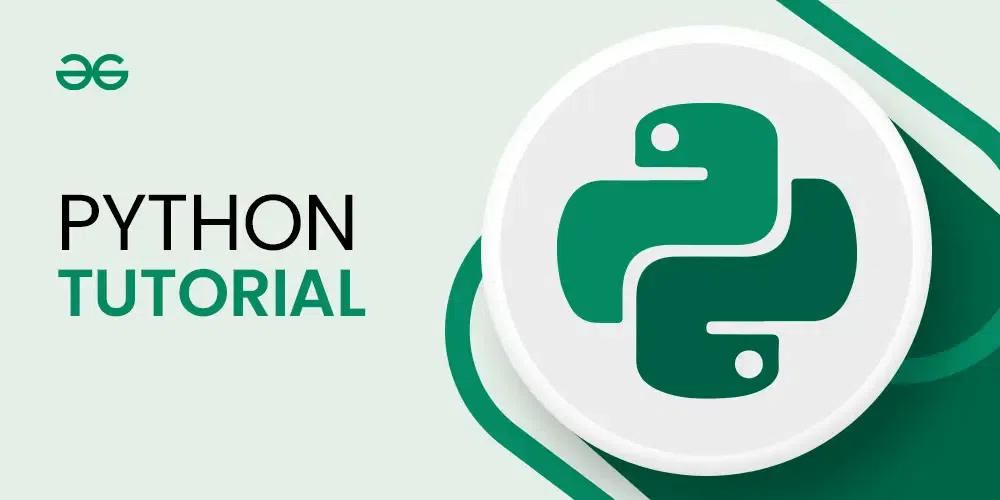
Below are the steps based your system requirements:
Setting up Python
- Download and Install Python 3 Latest Version
- How to set up Command Prompt for Python in Windows10
- Setup Python VS Code or PyCharm
- Creating Python Virtual Environment in Windows and Linux
Note: Python 3.13 is the latest version of Python, but Python 3.12 is the latest stable version.
Now let us deep dive into the basics and components to learn Python Programming:
Getting Started with Python Programming
Welcome to the Python tutorial section! Here, we’ll cover the essential elements you need to kickstart your journey in Python programming. From syntax and keywords to comments, variables, and indentation, we’ll explore the foundational concepts that underpin Python development.
- Learn Python Basics
- Keywords in Python
- Comments in Python
- Learn Python Variables
- Learn Python Data Types
- Indentation and why is it important in Python
Learn Python Input/Output
In this segment, we delve into the fundamental aspects of handling input and output operations in Python, crucial for interacting with users and processing data effectively. From mastering the versatile print() function to exploring advanced formatting techniques and efficient methods for receiving user input, this section equips you with the necessary skills to harness Python’s power in handling data streams seamlessly.
- Python print() function
- f-string in Python
- Print without newline in Python
- Python | end parameter in print()
- Python | sep parameter in print()
- Python | Output Formatting
- Taking Input in Python
- Taking Multiple Inputs from users in Python
Python Data Types
Python offers, enabling you to manipulate and manage data with precision and flexibility. Additionally, we’ll delve into the dynamic world of data conversion with casting, and then move on to explore the versatile collections Python provides, including lists, tuples, sets, dictionaries, and arrays.
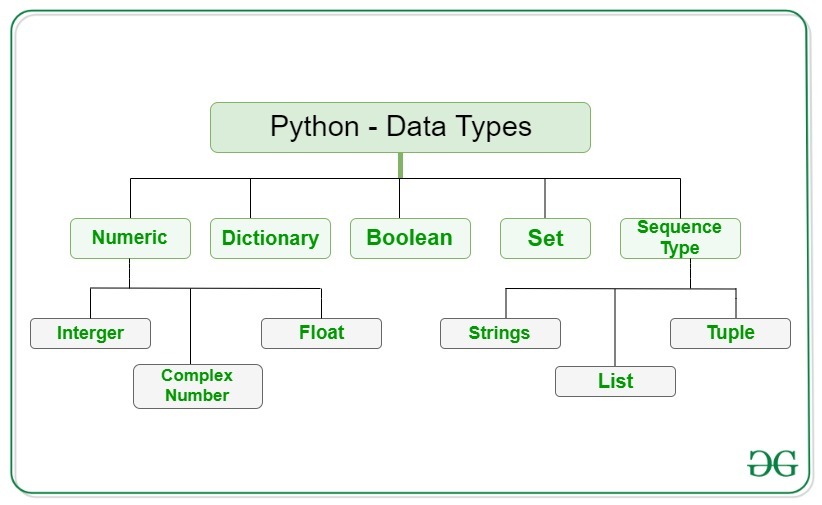
By the end of this section, you’ll not only grasp the essence of Python’s data types but also wield them proficiently to tackle a wide array of programming challenges with confidence.
- Python List
- Python Tuples
- Python Sets
- Python Dictionary
- Python Arrays
- Type Casting
Python Operators
From performing basic arithmetic operations to evaluating complex logical expressions, we’ll cover it all. We’ll delve into comparison operators for making decisions based on conditions, and then explore bitwise operators for low-level manipulation of binary data. Additionally, we’ll unravel the intricacies of assignment operators for efficient variable assignment and updating. Lastly, we’ll demystify membership and identity operators, such as in and is, enabling you to test for membership in collections and compare object identities with confidence.
- Arithmetic operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Membership & Identity Operators | Python “in”, and “is” operator
Python Conditional Statement
These statements are pivotal in programming, enabling dynamic decision-making and code branching. In this section of Python Tutorial, we’ll explore Python’s conditional logic, from basic if…else statements to nested conditions and the concise ternary operator. We’ll also introduce the powerful match case statement, new in Python 3.10. By the end, you’ll master these constructs, empowering you to write clear, efficient code that responds intelligently to various scenarios. Let’s dive in and unlock the potential of Python’s conditional statements.
- Python If else
- Nested if statement
- Python if-elif-else Ladder
- Python If Else on One Line
- Ternary Condition in Python
- Match Case Statement
Python Loops
Here, we’ll explore Python’s loop constructs, including the for and while loops, along with essential loop control statements like break, continue, and pass. Additionally, we’ll uncover the concise elegance of list and dictionary comprehensions for efficient data manipulation. By mastering these loop techniques, you’ll streamline your code for improved readability and performance.
- Loop control statements (break, continue, pass)
- Python List Comprehension
- Python Dictionary Comprehension
Python Functions
Functions are the backbone of organized and efficient code in Python. Here, we’ll explore their syntax, parameter handling, return values, and variable scope. From basic concepts to advanced techniques like closures and decorators. Along the way, we’ll also introduce versatile functions like range(), and powerful tools such as *args and **kwargs for flexible parameter handling. Additionally, we’ll delve into functional programming with map, filter, and lambda functions.
- Python Function syntax
- Arguments and Return Values in Python Function
- Python Function Global and Local Scope Variables
- Use of pass Statement in Function
- Return statemen in Python Function
- Python range() function
- *args and **kwargs in Python Function
- Python closures
- Python ‘Self’ as Default Argument
- Decorators in Python
- Map Function
- Filter Function
- Reduce Function
- Lambda Function
Python OOPs Concepts
In this segment, we’ll explore the core principles of object-oriented programming (OOP) in Python. From encapsulation to inheritance, polymorphism, abstract classes, and iterators, we’ll cover the essential concepts that empower you to build modular, reusable, and scalable code.
- Python Classes and Objects
- Polymorphism
- Inheritance
- Encapsulation
Python Exception Handling
In this section of Python Tutorial, we’ll explore how Python deals with unexpected errors, enabling you to write robust and fault-tolerant code. We’ll cover file handling, including reading from and writing to files, before diving into exception handling with try and except blocks. You’ll also learn about user-defined exceptions and Python’s built-in exception types.
- Python File Handling
- Python Read Files
- Python Write/Create Files
- Exception handling
- User defined Exception
- Built-in Exception
- Try and Except in Python
Python Packages or Libraries
The biggest strength of Python is a huge collection of standard libraries which can be used for the following:
- Built-in Modules in Python
- Python DSA Libraries
- Machine Learning
- Python GUI Libraries
- Web Scraping Pakages
- Game Development Packages
- Web Frameworks like, Django , Flask
- Image processing (like OpenCV , Pillow )
Python Collections
Here, we’ll explore key data structures provided by Python’s collections module. From counting occurrences with Counters to efficient queue operations with Deque, we’ll cover it all. By mastering these collections, you’ll streamline your data management tasks in Python.
- OrderedDict
- Defaultdict
Python Database Handling
In this section you will learn how to access and work with MySQL and MongoDB databases
- Python MongoDB Tutorial
- Python MySQL Tutorial
Python vs. Other Programming Languages
Here’s a comparison of Python with the programming languages C, C++, and Java in a table format:
Let us now begin learning about various important steps required in this Python Tutorial.
Learn More About Python with Different Applications :
Python is a versatile and widely-used programming language with a vast ecosystem. Here are some areas where Python is commonly used:
- Web Development : Python is used to build web applications using frameworks like Django, Flask, and Pyramid. These frameworks provide tools and libraries for handling web requests, managing databases, and more.
- Data Science and Machine Learning : Python is popular in data science and machine learning due to libraries like NumPy, pandas, Matplotlib, and scikit-learn. These libraries provide tools for data manipulation, analysis, visualization, and machine learning algorithms.
- Artificial Intelligence and Natural Language Processing : Python is widely used in AI and NLP applications. Libraries like TensorFlow, Keras, PyTorch, and NLTK provide tools for building and training neural networks, processing natural language, and more.
- Game Development : Python can be used for game development using libraries like Pygame and Panda3D. These libraries provide tools for creating 2D and 3D games, handling graphics, and more.
- Desktop Applications : Python can be used to build desktop applications using libraries like Tkinter, PyQt, and wxPython. These libraries provide tools for creating graphical user interfaces (GUIs), handling user input, and more.
- Scripting and Automation : Python is commonly used for scripting and automation tasks due to its simplicity and readability. It can be used to automate repetitive tasks, manage files and directories, and more.
- Web Scraping and Crawling : Python is widely used for web scraping and crawling using libraries like BeautifulSoup and Scrapy. These libraries provide tools for extracting data from websites, parsing HTML and XML, and more.
- Education and Research : Python is commonly used in education and research due to its simplicity and readability. Many universities and research institutions use Python for teaching programming and conducting research in various fields.
- Community and Ecosystem : Python has a large and active community, which contributes to its ecosystem. There are many third-party libraries and frameworks available for various purposes, making Python a versatile language for many applications.
- Cross-Platform : Python is a cross-platform language, which means that Python code can run on different operating systems without modification. This makes it easy to develop and deploy Python applications on different platforms.
To achieve a solid understanding of Python, it’s very important to engage with Python quizzes and MCQs. These quizzes can enhance your ability to solve similar questions and improve your problem-solving skills.
Here are some quiz articles related to Python Tutorial:
- Python MCQs
- Python Sets Quiz
- Python List Quiz
- Python String Quiz
- Python Tuple Quiz
- Python Dictionary Quiz
Python Latest & Upcoming Features
Python recently release Python 3.12 in October 2023 and here in this section we have mentioned all the features that Python 3.12 offer. Along with this we have also mentioned the lasted trends.
- Security Fix: A critical security patch addressing potential vulnerabilities (details not publicly disclosed).
- SBOM (Software Bill of Materials) Documents: Availability of SBOM documents for CPython, improving transparency in the software supply chain.
Expected Upcoming Features of Python 3.13
- Pattern Matching (PEP 635): A powerful new syntax for pattern matching, potentially similar to features found in languages like Ruby. This could significantly improve code readability and maintainability.
- Union Typing Enhancements (PEP 647): Extending type annotations for unions, allowing for more precise type definitions and improved static type checking.
- Improved Exception Groups (PEP 653): A new mechanism for grouping related exceptions, making error handling more organized and user-friendly.
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?
NumPy 1.26.0 Release Notes #
The NumPy 1.26.0 release is a continuation of the 1.25.x release cycle with the addition of Python 3.12.0 support. Python 3.12 dropped distutils, consequently supporting it required finding a replacement for the setup.py/distutils based build system NumPy was using. We have chosen to use the Meson build system instead, and this is the first NumPy release supporting it. This is also the first release that supports Cython 3.0 in addition to retaining 0.29.X compatibility. Supporting those two upgrades was a large project, over 100 files have been touched in this release. The changelog doesn’t capture the full extent of the work, special thanks to Ralf Gommers, Sayed Adel, Stéfan van der Walt, and Matti Picus who did much of the work in the main development branch.
The highlights of this release are:
Python 3.12.0 support.
Cython 3.0.0 compatibility.
Use of the Meson build system
Updated SIMD support
f2py fixes, meson and bind(x) support
Support for the updated Accelerate BLAS/LAPACK library
The Python versions supported in this release are 3.9-3.12.
New Features #
Array api v2022.12 support in numpy.array_api #.
numpy.array_api now full supports the v2022.12 version of the array API standard. Note that this does not yet include the optional fft extension in the standard.
( gh-23789 )
Support for the updated Accelerate BLAS/LAPACK library #
Support for the updated Accelerate BLAS/LAPACK library, including ILP64 (64-bit integer) support, in macOS 13.3 has been added. This brings arm64 support, and significant performance improvements of up to 10x for commonly used linear algebra operations. When Accelerate is selected at build time, the 13.3+ version will automatically be used if available.
( gh-24053 )
meson backend for f2py #
f2py in compile mode (i.e. f2py -c ) now accepts the --backend meson option. This is the default option for Python 3.12 on-wards. Older versions will still default to --backend distutils .
To support this in realistic use-cases, in compile mode f2py takes a --dep flag one or many times which maps to dependency() calls in the meson backend, and does nothing in the distutils backend.
There are no changes for users of f2py only as a code generator, i.e. without -c .
( gh-24532 )
bind(c) support for f2py #
Both functions and subroutines can be annotated with bind(c) . f2py will handle both the correct type mapping, and preserve the unique label for other C interfaces.
Note: bind(c, name = 'routine_name_other_than_fortran_routine') is not honored by the f2py bindings by design, since bind(c) with the name is meant to guarantee only the same name in C and Fortran , not in Python and Fortran .
( gh-24555 )
Improvements #
Iso_c_binding support for f2py #.
Previously, users would have to define their own custom f2cmap file to use type mappings defined by the Fortran2003 iso_c_binding intrinsic module. These type maps are now natively supported by f2py
Build system changes #
In this release, NumPy has switched to Meson as the build system and meson-python as the build backend. Installing NumPy or building a wheel can be done with standard tools like pip and pypa/build . The following are supported:
Regular installs: pip install numpy or (in a cloned repo) pip install .
Building a wheel: python -m build (preferred), or pip wheel .
Editable installs: pip install -e . --no-build-isolation
Development builds through the custom CLI implemented with spin : spin build .
All the regular pip and pypa/build flags (e.g., --no-build-isolation ) should work as expected.
NumPy-specific build customization #
Many of the NumPy-specific ways of customizing builds have changed. The NPY_* environment variables which control BLAS/LAPACK, SIMD, threading, and other such options are no longer supported, nor is a site.cfg file to select BLAS and LAPACK. Instead, there are command-line flags that can be passed to the build via pip / build ’s config-settings interface. These flags are all listed in the meson_options.txt file in the root of the repo. Detailed documented will be available before the final 1.26.0 release; for now please see the SciPy “building from source” docs since most build customization works in an almost identical way in SciPy as it does in NumPy.
Build dependencies #
While the runtime dependencies of NumPy have not changed, the build dependencies have. Because we temporarily vendor Meson and meson-python, there are several new dependencies - please see the [build-system] section of pyproject.toml for details.
Troubleshooting #
This build system change is quite large. In case of unexpected issues, it is still possible to use a setup.py -based build as a temporary workaround (on Python 3.9-3.11, not 3.12), by copying pyproject.toml.setuppy to pyproject.toml . However, please open an issue with details on the NumPy issue tracker. We aim to phase out setup.py builds as soon as possible, and therefore would like to see all potential blockers surfaced early on in the 1.26.0 release cycle.
Contributors #
A total of 20 people contributed to this release. People with a “+” by their names contributed a patch for the first time.
Albert Steppi +
Bas van Beek
Charles Harris
Developer-Ecosystem-Engineering
Filipe Laíns +
Jake Vanderplas
Liang Yan +
Marten van Kerkwijk
Matti Picus
Melissa Weber Mendonça
Namami Shanker
Nathan Goldbaum
Ralf Gommers
Rohit Goswami
Sebastian Berg
Stefan van der Walt
Tyler Reddy
Warren Weckesser
Pull requests merged #
A total of 59 pull requests were merged for this release.
#24305 : MAINT: Prepare 1.26.x branch for development
#24308 : MAINT: Massive update of files from main for numpy 1.26
#24322 : CI: fix wheel builds on the 1.26.x branch
#24326 : BLD: update openblas to newer version
#24327 : TYP: Trim down the _NestedSequence.__getitem__ signature
#24328 : BUG: fix choose refcount leak
#24337 : TST: fix running the test suite in builds without BLAS/LAPACK
#24338 : BUG: random: Fix generation of nan by dirichlet.
#24340 : MAINT: Dependabot updates from main
#24342 : MAINT: Add back NPY_RUN_MYPY_IN_TESTSUITE=1
#24353 : MAINT: Update extbuild.py from main.
#24356 : TST: fix distutils tests for deprecations in recent setuptools…
#24375 : MAINT: Update cibuildwheel to version 2.15.0
#24381 : MAINT: Fix codespaces setup.sh script
#24403 : ENH: Vendor meson for multi-target build support
#24404 : BLD: vendor meson-python to make the Windows builds with SIMD…
#24405 : BLD, SIMD: The meson CPU dispatcher implementation
#24406 : MAINT: Remove versioneer
#24409 : REL: Prepare for the NumPy 1.26.0b1 release.
#24453 : MAINT: Pin upper version of sphinx.
#24455 : ENH: Add prefix to _ALIGN Macro
#24456 : BUG: cleanup warnings [skip azp][skip circle][skip travis][skip…
#24460 : MAINT: Upgrade to spin 0.5
#24495 : BUG: asv dev has been removed, use asv run .
#24496 : BUG: Fix meson build failure due to unchanged inplace auto-generated…
#24521 : BUG: fix issue with git-version script, needs a shebang to run
#24522 : BUG: Use a default assignment for git_hash [skip ci]
#24524 : BUG: fix NPY_cast_info error handling in choose
#24526 : BUG: Fix common block handling in f2py
#24541 : CI,TYP: Bump mypy to 1.4.1
#24542 : BUG: Fix assumed length f2py regression
#24544 : MAINT: Harmonize fortranobject
#24545 : TYP: add kind argument to numpy.isin type specification
#24561 : BUG: fix comparisons between masked and unmasked structured arrays
#24590 : CI: Exclude import libraries from list of DLLs on Cygwin.
#24591 : BLD: fix _umath_linalg dependencies
#24594 : MAINT: Stop testing on ppc64le.
#24602 : BLD: meson-cpu: fix SIMD support on platforms with no features
#24606 : BUG: Change Cython binding directive to “False”.
#24613 : ENH: Adopt new macOS Accelerate BLAS/LAPACK Interfaces, including…
#24614 : DOC: Update building docs to use Meson
#24615 : TYP: Add the missing casting keyword to np.clip
#24616 : TST: convert cython test from setup.py to meson
#24617 : MAINT: Fixup fromnumeric.pyi
#24622 : BUG, ENH: Fix iso_c_binding type maps and fix bind(c) …
#24629 : TYP: Allow binary_repr to accept any object implementing…
#24630 : TYP: Explicitly declare dtype and generic hashable
#24637 : ENH: Refactor the typing “reveal” tests using typing.assert_type
#24638 : MAINT: Bump actions/checkout from 3.6.0 to 4.0.0
#24647 : ENH: meson backend for f2py
#24648 : MAINT: Refactor partial load Workaround for Clang
#24653 : REL: Prepare for the NumPy 1.26.0rc1 release.
#24659 : BLD: allow specifying the long double format to avoid the runtime…
#24665 : BLD: fix bug in random.mtrand extension, don’t link libnpyrandom
#24675 : BLD: build wheels for 32-bit Python on Windows, using MSVC
#24700 : BLD: fix issue with compiler selection during cross compilation
#24701 : BUG: Fix data stmt handling for complex values in f2py
#24707 : TYP: Add annotations for the py3.12 buffer protocol
#24718 : DOC: fix a few doc build issues on 1.26.x and update spin docs …
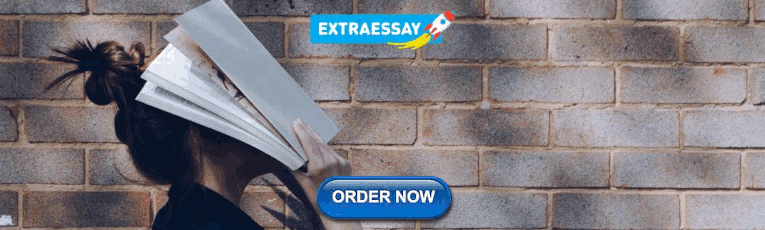
COMMENTS
Use numpy.meshgrid () to make arrays of indexes that you can use to index into both your original array and the array of values for the third dimension. import numpy as np. import scipy as sp. import scipy.stats.distributions. a = np.zeros((2,3,4)) z = sp.stats.distributions.randint.rvs(0, 4, size=(2,3))
Element Assignment in NumPy Arrays. We can assign new values to an element of a NumPy array using the = operator, just like regular python lists. A few examples are below (note that this is all one code block, which means that the element assignments are carried forward from step to step). array([0.12, 0.94, 0.66, 0.73, 0.83])
ndarrays. #. ndarrays can be indexed using the standard Python x[obj] syntax, where x is the array and obj the selection. There are different kinds of indexing available depending on obj : basic indexing, advanced indexing and field access. Most of the following examples show the use of indexing when referencing data in an array.
NumPy's np.flip() function allows you to flip, or reverse, the contents of an array along an axis. When using np.flip(), specify the array you would like to reverse and the axis. If you don't specify the axis, NumPy will reverse the contents along all of the axes of your input array. Reversing a 1D array.
This article explains how to get and set values, such as individual elements or subarrays (e.g., rows or columns), in a NumPy array ( ndarray) using various indexing. See the following articles for information on deleting, concatenating, and adding to ndarray. The NumPy version used in this article is as follows.
delete (arr, obj [, axis]) Return a new array with sub-arrays along an axis deleted. insert (arr, obj, values [, axis]) Insert values along the given axis before the given indices. append (arr, values [, axis]) Append values to the end of an array. resize (a, new_shape) Return a new array with the specified shape.
What is a Python Numpy Array? You already read in the introduction that NumPy arrays are a bit like Python lists, but still very much different at the same time. ... # Import the NumPy library and give it an alias of `np` import numpy as np # Initialize a 3x4 array of ones and assign it to the variable `x` x = np.ones((3,4)) # Print the shape ...
The NumPy slicing syntax follows that of the standard Python list; to access a slice of an array x, use this: x[start:stop:step] If any of these are unspecified, they default to the values start=0, stop= size of dimension, step=1 . We'll take a look at accessing sub-arrays in one dimension and in multiple dimensions.
Getting into Shape: Intro to NumPy Arrays. The fundamental object of NumPy is its ndarray (or numpy.array), an n-dimensional array that is also present in some form in array-oriented languages such as Fortran 90, R, and MATLAB, as well as predecessors APL and J. Let's start things off by forming a 3-dimensional array with 36 elements:
Introducing Numpy Arrays. In the 2nd part of this book, we will study the numerical methods by using Python. We will use array/matrix a lot later in the book. Therefore, here we are going to introduce the most common way to handle arrays in Python using the Numpy module. Numpy is probably the most fundamental numerical computing module in Python.
Exercise 2: Create a 5X2 integer array from a range between 100 to 200 such that the difference between each element is 10. Expected Output: [120 130] [140 150] [160 170] [180 190]] Exercise 3: Following is the provided numPy array. Return array of items by taking the third column from all rows. Expected Output:
When copy=False and a copy is made for other reasons, the result is the same as if copy=True, with some exceptions for 'A', see the Notes section.The default order is 'K'. subok bool, optional. If True, then sub-classes will be passed-through, otherwise the returned array will be forced to be a base-class array (default).
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
Assign value to array (Python, Numpy) 0. How can I use numpy array elements as indices to assign values for another numpy array. Hot Network Questions I got hit with a spell carrying "Personal fireball thrower". How long will I throw fireballs everywhere?
101 Practice exercises with pandas. 1. Import numpy as np and see the version. Difficulty Level: L1. Q. Import numpy as np and print the version number. 2. How to create a 1D array? Difficulty Level: L1. Q. Create a 1D array of numbers from 0 to 9.
We can perform a modulus operation in NumPy arrays using the % operator or the mod() function. This operation calculates the remainder of element-wise division between two arrays. Let's see an example. import numpy as np. first_array = np.array([9, 10, 20]) second_array = np.array([2, 5, 7]) # using the % operator.
Notice when you perform operations with two arrays of the same dtype: uint32, the resulting array is the same type.When you perform operations with different dtype, NumPy will assign a new type that satisfies all of the array elements involved in the computation, here uint32 and int32 can both be represented in as int64.. The default NumPy behavior is to create arrays in either 32 or 64-bit ...
Numpy arrays have a copy method which you can use for just this purpose. So if you write. b = a.copy() then Python will first actually make a copy of the array - that is, it sets aside a new region of memory, let's say at address 0x123904381, then goes to memory address 0x123674283 and copies all the values of the array from the latter section ...
$ python numpy.py Traceback (most recent call last): File "/home/numpy.py", line 1, in <module> import numpy as np; np.array ... User-defined functions: Assignment to a function's __code__ attribute where the new code object's type does not match the function's type, is deprecated. The different types are: plain function, generator, async ...
Python has a dynamic approach when it comes to types: every element in a list can have a different type. But numpy works with matrices where all elements have the same type. Therefore assigning a float to an int matrix, will convert the row first to int s. This will construct an array: >>> arr = np.array([[3, -4, 4], [1, -2, 2]],dtype=np.float)
This Python Tutorial will help you learn Python programming language most efficiently from basics to advanced (like Web-scraping, Django, Learning, etc.) ... Python Arrays; Type Casting; Python Operators. ... Python is popular in data science and machine learning due to libraries like NumPy, pandas, Matplotlib, and scikit-learn. These libraries ...
Assigning to rows seems to work easily, but I couldn't find an example of assigning an array to a column of another array. python; numpy; Share. Improve this question. Follow edited Jan 8, 2022 at 10:59. mkrieger1. 21.5k ... Python, Numpy: Cannot assign the values of a numpy array to a column of a matrix. 1.
This often is used for a name or label field. Using NumPy indexing and broadcasting with arrays of Python strings of unknown length, which may or may not have data defined for every value. For the first use case, NumPy provides the fixed-width numpy.void , numpy.str_ and numpy.bytes_ data types. For the second use case, numpy provides numpy ...
The NumPy 1.26.0 release is a continuation of the 1.25.x release cycle with the addition of Python 3.12.0 support. Python 3.12 dropped distutils, consequently supporting it required finding a replacement for the setup.py/distutils based build system NumPy was using. ... Array API v2022.12 support in numpy.array_api # ... #24522: BUG: Use a ...
Found the answer for why the first dimension works but not the second. List multiplication makes a shallow copy. When you assign to an index, it does a proper change, but access does not, so when you do a[x][y] = 2, it's accessing, not assigning, for the xth index - only the yth access is actually changed.This page helped me explain with diagrams that are probably better than what I tried ...