Create an Addition Game with React and JavaScript
- Post author By John Au-Yeung
- Post date January 29, 2021
- No Comments on Create an Addition Game with React and JavaScript

React is an easy to use JavaScript framework that lets us create front end apps.
In this article, we’ll look at how to create an addition game with React and JavaScript.
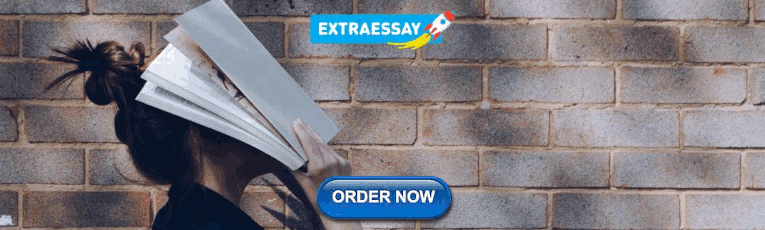
Create the Project
We can create the React project with Create React App.
To install it, we run:
with NPM to create our React project.
Create the Addition Game
To create the addition game, we write:
We have the num1 , num2 , sum , and score states created with the useState hook.
num1 and num2 are the parts of the addition question.
sum has the sum we enter.
score has the score.
Below that, we have the generateQuestion function to generate the num1 and num2 values.
setNum1 and setNum2 to set them.
Math.random creates a random number between 0 and 1.
Math.ceil rounds up to the nearest integer.
Then we have the submit function that checks the sum value against the sum of num1 and num2 .
Before we do the check, we call e.preventDefault() so we can do client-side submission.
Then we check if sum is a number bigger than or equal to 0.
And if it is, then we do the check.
If the answer matches the sum, then we increase the score with setScore .
We increase it by passing a callback that returns the original score plus 1.
Then we call generateQuestion again to generate the next question.
Below that, we have a form that has the onSubmit prop.
onSubmit runs when we click on the check button.
In the form, we display num1 and num2 .
And we have an input that takes the sum value and we set sum by call setSum in the onChange callback.
e.target.value has the inputted value.
Below that, we have the start game button which calls generateQuestion when we click it.
And finally, we show the score below that.
We can create an addition game easily with React and JavaScript.
Related Posts
Vue 3 is the latest version of the easy to use Vue JavaScript framework that…
React is an easy to use JavaScript framework that lets us create front end apps.…
By John Au-Yeung
Web developer specializing in React, Vue, and front end development.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.

JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript assignment, javascript assignment operators.
Assignment operators assign values to JavaScript variables.
Shift Assignment Operators
Bitwise assignment operators, logical assignment operators, the = operator.
The Simple Assignment Operator assigns a value to a variable.
Simple Assignment Examples
The += operator.
The Addition Assignment Operator adds a value to a variable.
Addition Assignment Examples
The -= operator.
The Subtraction Assignment Operator subtracts a value from a variable.
Subtraction Assignment Example
The *= operator.
The Multiplication Assignment Operator multiplies a variable.
Multiplication Assignment Example
The **= operator.
The Exponentiation Assignment Operator raises a variable to the power of the operand.
Exponentiation Assignment Example
The /= operator.
The Division Assignment Operator divides a variable.
Division Assignment Example
The %= operator.
The Remainder Assignment Operator assigns a remainder to a variable.
Remainder Assignment Example
Advertisement
The <<= Operator
The Left Shift Assignment Operator left shifts a variable.
Left Shift Assignment Example
The >>= operator.
The Right Shift Assignment Operator right shifts a variable (signed).
Right Shift Assignment Example
The >>>= operator.
The Unsigned Right Shift Assignment Operator right shifts a variable (unsigned).
Unsigned Right Shift Assignment Example
The &= operator.
The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
Bitwise AND Assignment Example
The |= operator.
The Bitwise OR Assignment Operator does a bitwise OR operation on two operands and assigns the result to the variable.
Bitwise OR Assignment Example
The ^= operator.
The Bitwise XOR Assignment Operator does a bitwise XOR operation on two operands and assigns the result to the variable.
Bitwise XOR Assignment Example
The &&= operator.
The Logical AND assignment operator is used between two values.
If the first value is true, the second value is assigned.
Logical AND Assignment Example
The &&= operator is an ES2020 feature .
The ||= Operator
The Logical OR assignment operator is used between two values.
If the first value is false, the second value is assigned.
Logical OR Assignment Example
The ||= operator is an ES2020 feature .
The ??= Operator
The Nullish coalescing assignment operator is used between two values.
If the first value is undefined or null, the second value is assigned.
Nullish Coalescing Assignment Example
The ??= operator is an ES2020 feature .
Test Yourself With Exercises
Use the correct assignment operator that will result in x being 15 (same as x = x + y ).
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
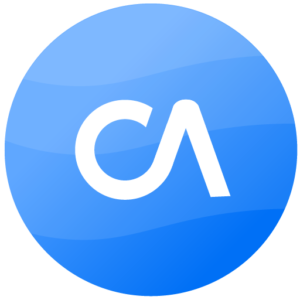
Text Similarity Checker Witth Javascript
Black sheep animation, animated diwali wishes | css animation tutorial, testimonial slider using javascript, math game with javascript.
Hello everyone. Welcome to today’s tutorial. In today’s tutorial, we will learn how to create a Math Game. To build this project, we need HTML, CSS and Javascript.
Math game is a beginner-friendly javascript tutorial. If you are looking for more tutorials to improve your javascript skills, you can check out this playlist here. This playlist consists of more than 100 javascript projects.
The difficulty level of these projects varies from easy to complex. Therefore it makes this playlist suitable for all kinds of javascript learners. So whether you are a javascript beginner or an expert, this playlist has something for you.
Let us take a look at how this project works. Initially, we display a start screen. When the user clicks the start button, we present them with the game screen. The game screen consists of a mathematical equation with a blank space.
The game goal is for the user to fill in the blank with the appropriate number or operator to complete the equation. Once the user fills in their answer, they click on submit. On clicking the submit button, we display a result screen. We include the result along with a restart button on the result screen.
Video Tutorial:
If you are interested to learn by watching a video tutorial rather than reading this blog post you can watch the video down below. Also if you like the video, subscribe to my youtube channel where I post new tips, tricks and tutorials related to web development every alternate day.
Project Structure:
Before we start coding let us take a look at the project folder structure. We create a project folder called – ‘Math Game’. Within this folder, we have three files. These files are index.html, style.css and script.js.
We begin with the HTML code. First, copy the code below and paste it into your HTML document.
Next, we style our project with CSS. For this once again copy the code provided to you below and paste it into your stylesheet.
Javascript:
Finally, we implement the functionality of our project. For this we use Javascript. Copy the code below and paste it into your script file.
That’s it for this tutorial. If you face any issues, you can download the source code by clicking on the ‘Download Code’ button below. You can post your queries and suggestions in the comments below. Happy Coding!
- javascript game
- javascript project
- javascript tutorial
- math game javascript
- maths game javascript
Barcode Generator | Javascript Project With Source Code
Dynamic color changer, fruit fall game javascript, leave a reply cancel reply.
Save my name, email, and website in this browser for the next time I comment.
Please enter an answer in digits: three × one =
Most Popular
Shake on invalid input | html, css & javascript.
- Privacy Policy
Coding Artist is dedicated to providing you quick and simple yet efficient coding tutorials. We provide best tutorials on HTML, CSS and Javascript. Let's learn, code and grow !
Contact us: [email protected]
20+ JavaScript Games with Source Code for Beginners
By Faraz - December 27, 2023
Explore 20+ JavaScript games with source code, perfect for beginners. Code, play, and learn game development in a fun and interactive way.
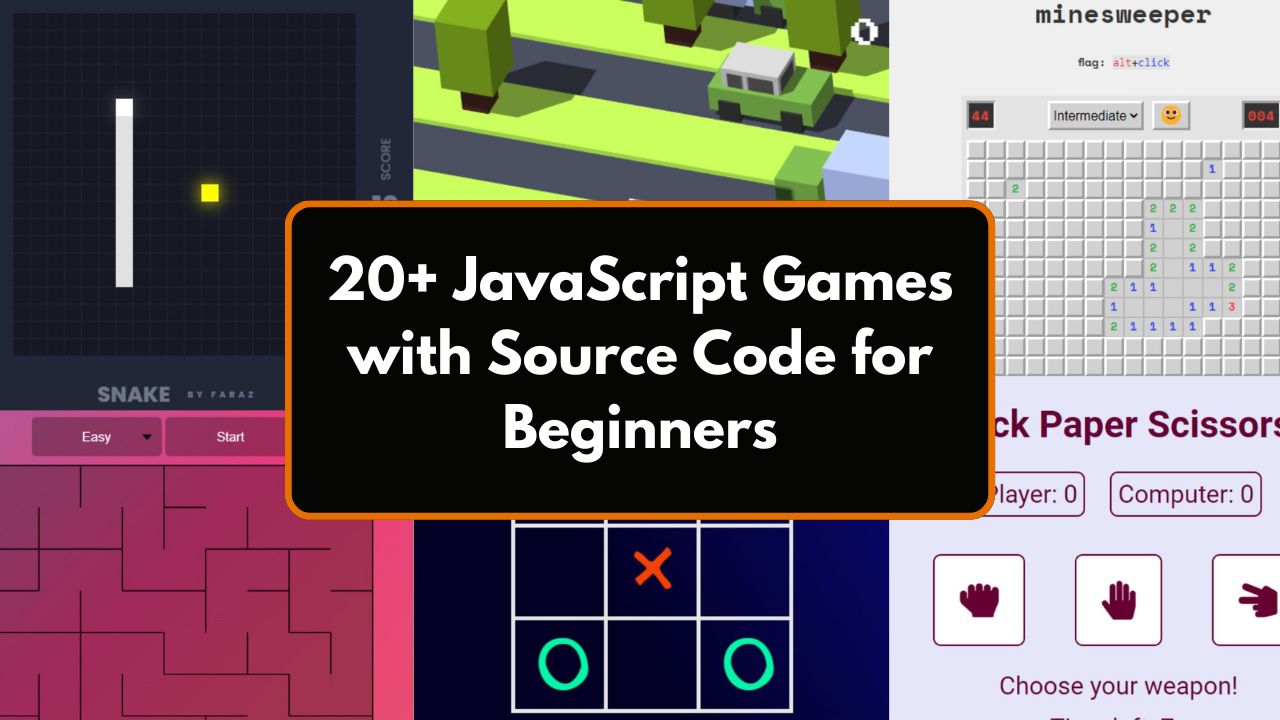
Table of Contents
Game 1: connect four game, game 2: candy crush, game 3: speed typing game, game 4: archery, game 5: flappy bird, game 6: maze game, game 7: hangman, game 8: crossy road, game 9: 2048 game, game 10: tilting maze game, game 11: tetris game, game 12: minesweeper game, game 13: ping pong game, game 14: flip card memory game, game 15: rock paper scissors, game 16: tic tac toe, game 17: snake game, game 18: fruit slicer, game 19: quiz, game 20: tower blocks, game 21: slot game, frequently asked questions (faqs), introduction.
Learning JavaScript can be both exciting and challenging, especially for beginners. One effective way to grasp the language's fundamentals is through practical projects like games. In this article, we will explore 20+ JavaScript games with source code, providing a hands-on approach for those venturing into the world of programming.
List of 20+ JavaScript Games for Beginners
Now, let's dive into the exciting world of JavaScript games suitable for beginners. We'll categorize these games based on complexity, providing a diverse range of projects to explore.
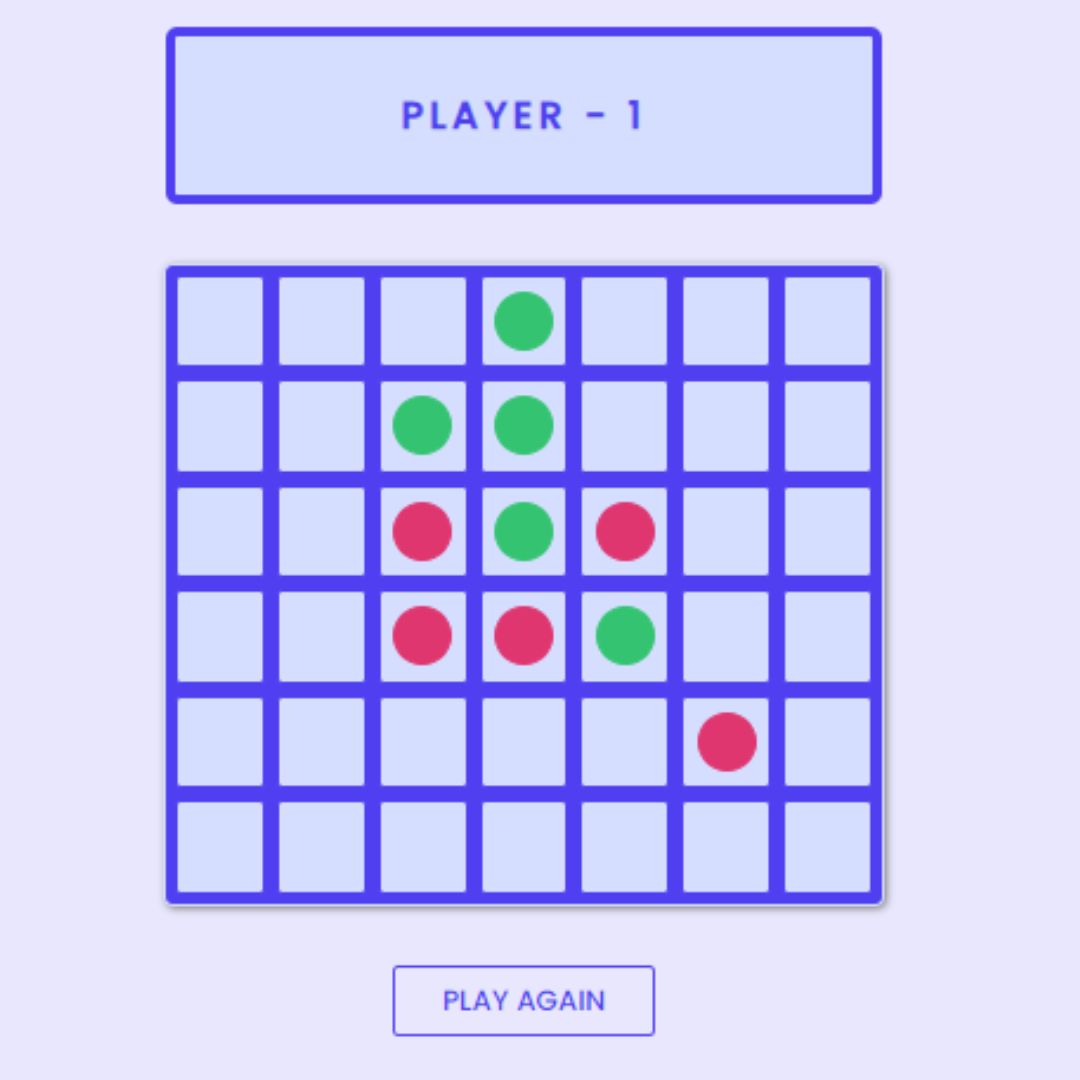
Connect Four is a classic two-player strategy game where the objective is to connect four of your own discs in a row. The game is played on a vertical grid, and players take turns dropping colored discs from the top. The first player to achieve a horizontal, vertical, or diagonal line of four discs wins.
Benefits: Teaches grid-based logic, win conditions, and turn-based gameplay.
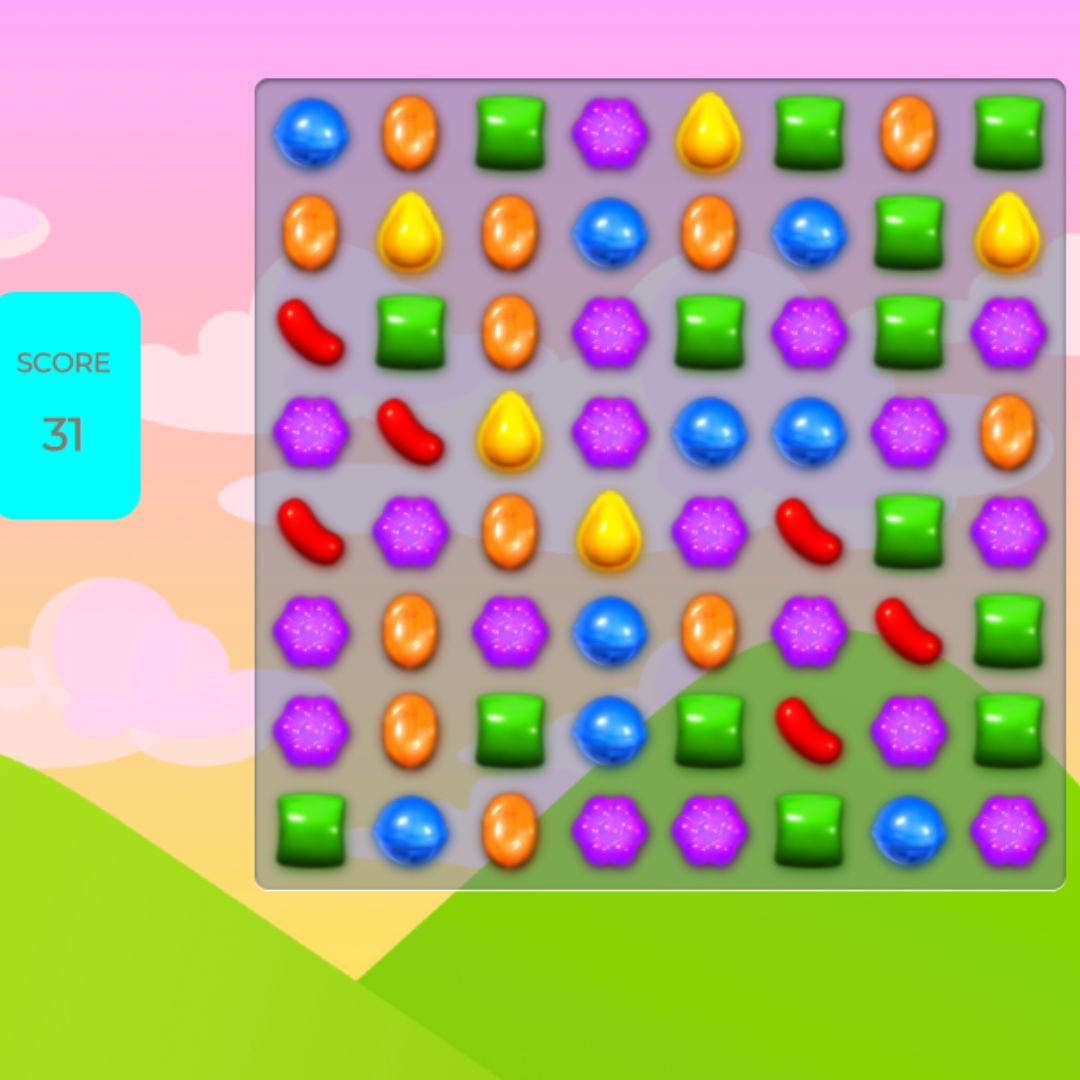
Candy Crush is a wildly popular match-three puzzle game that has captivated millions of players worldwide. In this visually enticing and addictive game, players match colorful candies to clear levels and progress through a sugary-sweet adventure. The primary goal is to achieve specific objectives within a limited number of moves, creating a delightful blend of strategy and visual appeal.
Benefits: Teaches match-three mechanics, cascading effects, and level progression. Covers UI elements like score display, level goals, and special candy effects. Provides insights into game balancing and designing engaging levels with increasing difficulty.
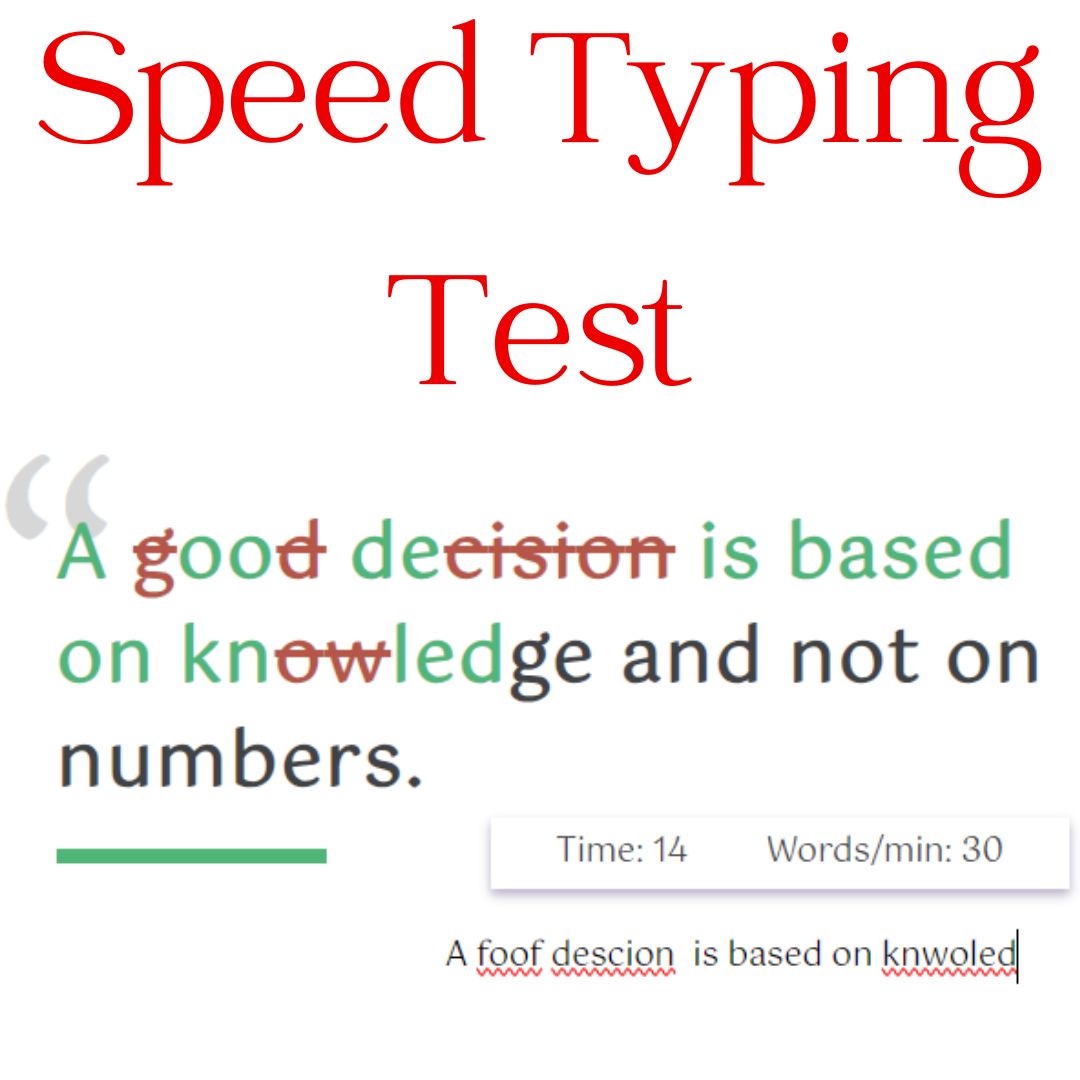
A Speed Typing Game is an engaging and educational way to improve typing skills while having fun. The game challenges players to type a given set of words or sentences as quickly and accurately as possible. Speed, accuracy, and word recognition are key factors, making it an excellent choice for those looking to enhance their typing proficiency.
Benefits: Enhances typing speed and accuracy. Improves cognitive skills, concentration, and reflexes.
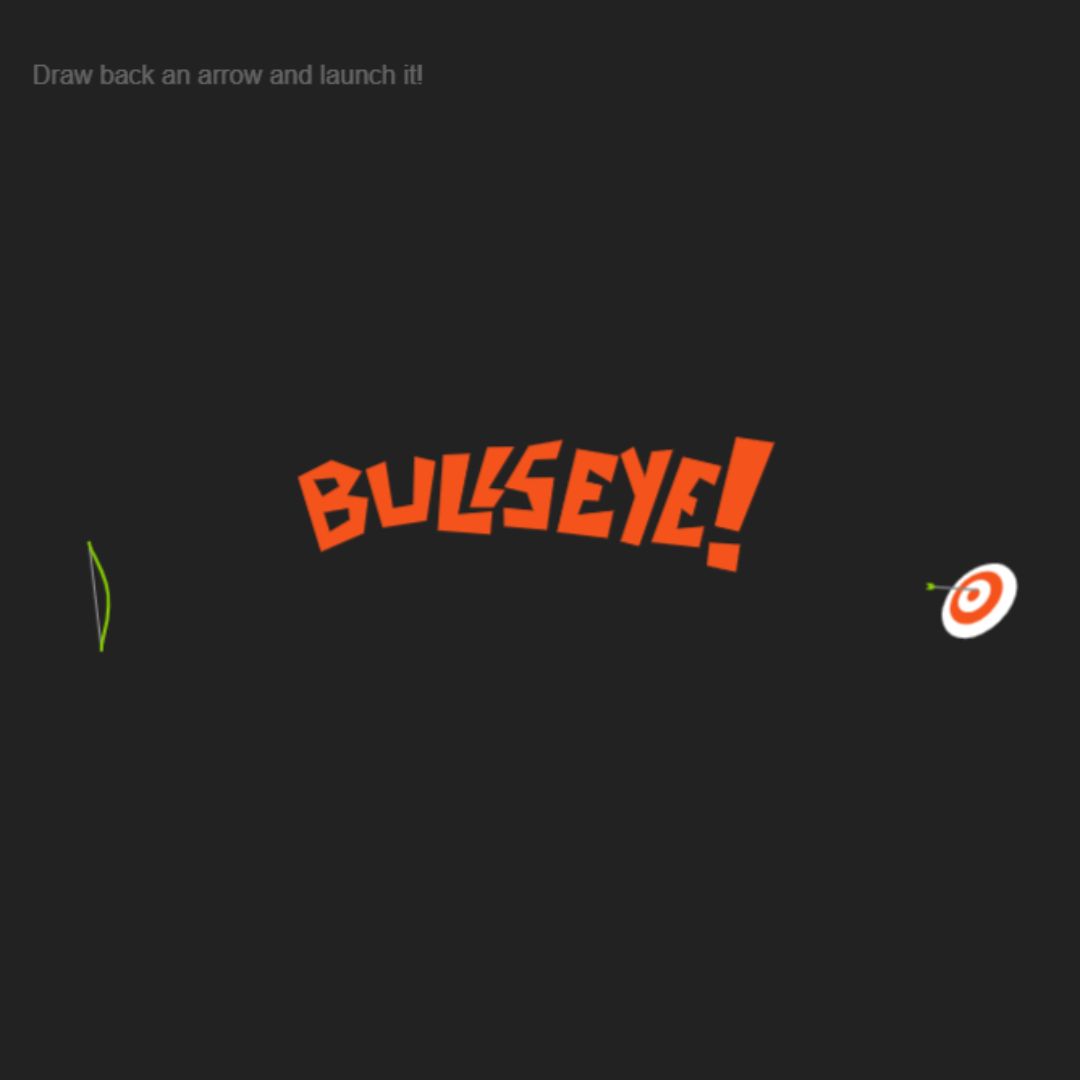
An Archery Game offers players the immersive experience of virtual archery, combining precision, concentration, and skill. Whether set in a realistic archery range or a fantastical world, this game challenges players to hit targets with accuracy and consistency, making it a thrilling and rewarding gaming experience.
Benefits: Improves focus, concentration, and patience as players strive for accuracy. Teaches basic physics concepts related to projectile motion.
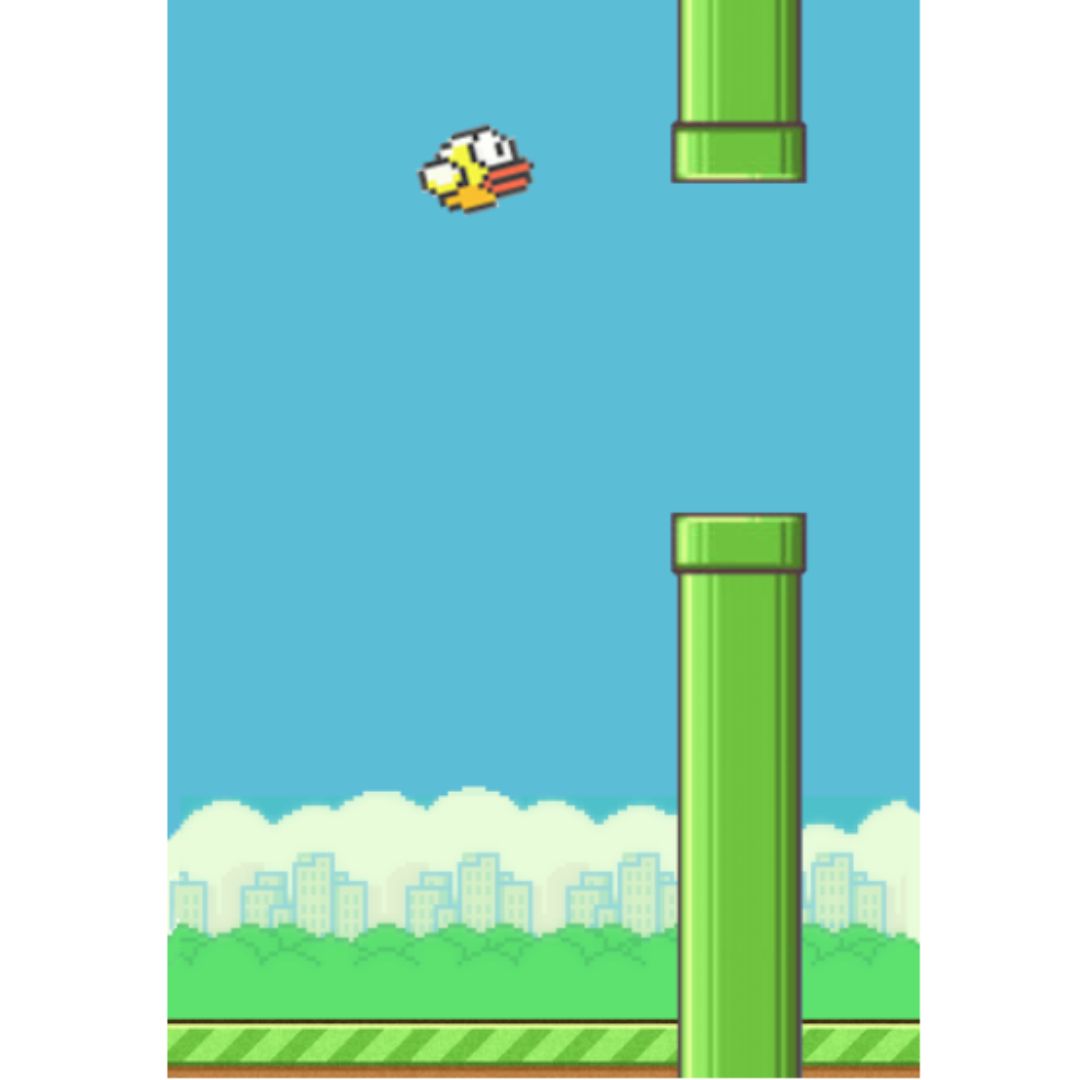
Flappy Bird is a simple yet notoriously addictive mobile game that gained immense popularity for its minimalist design and challenging gameplay. Created by Dong Nguyen, the game features a small bird navigating through a series of pipes. The objective is to guide the bird safely through the gaps in the pipes, testing the player's reflexes and precision.
Benefits: Covers side-scrolling mechanics, collision detection, and score tracking.
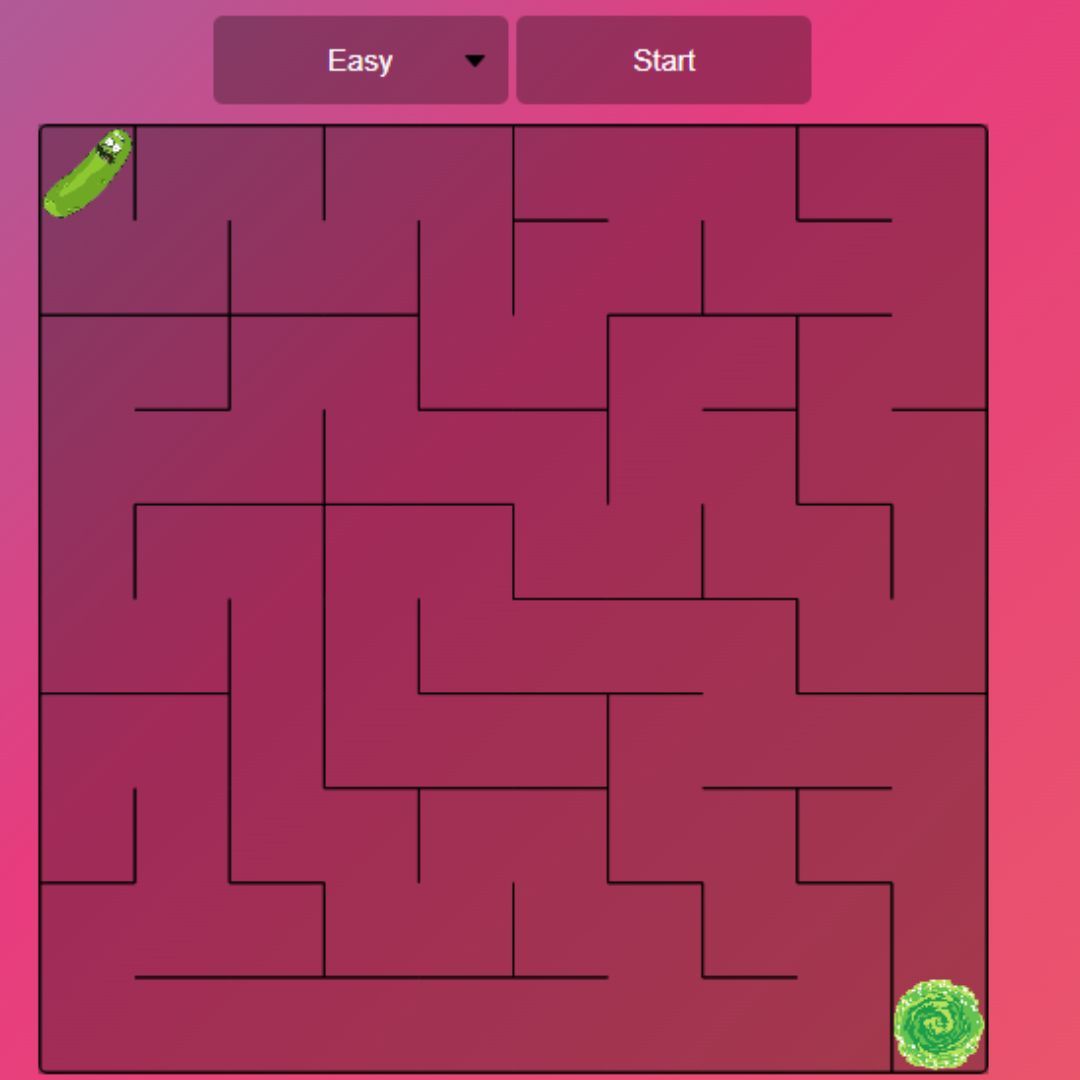
Players navigate through a maze, typically from a starting point to a goal, facing challenges such as dead ends and branching paths. The objective is to find the exit within the shortest possible time.
Benefits: Enhances problem-solving skills and spatial awareness. Improves memory as players mentally map out the maze. Additionally, it serves as an entertaining way to engage with puzzles and strategic thinking.
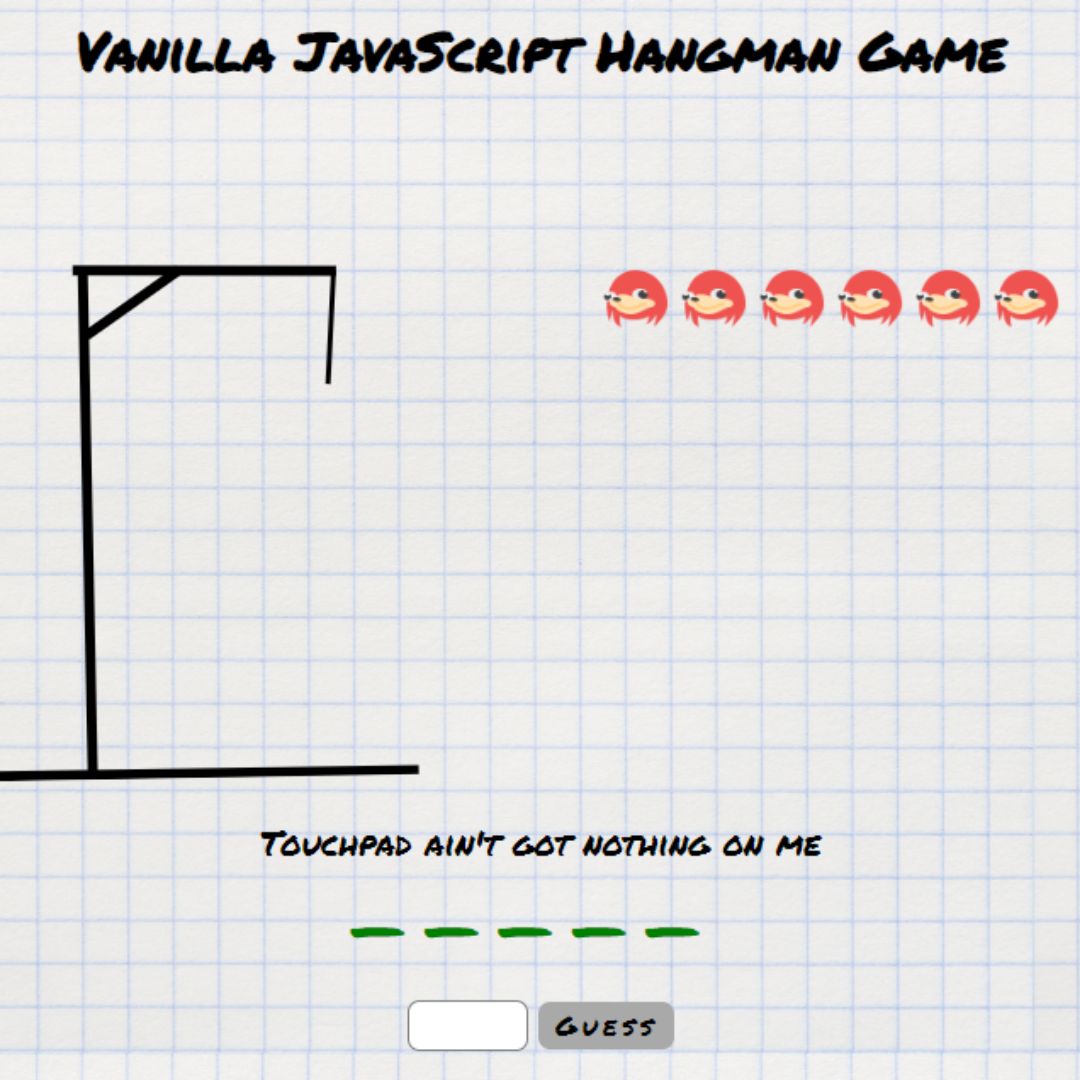
Word-guessing game where players try to guess a hidden word by suggesting letters.
Benefits: Covers word manipulation, user input validation, and game state management.
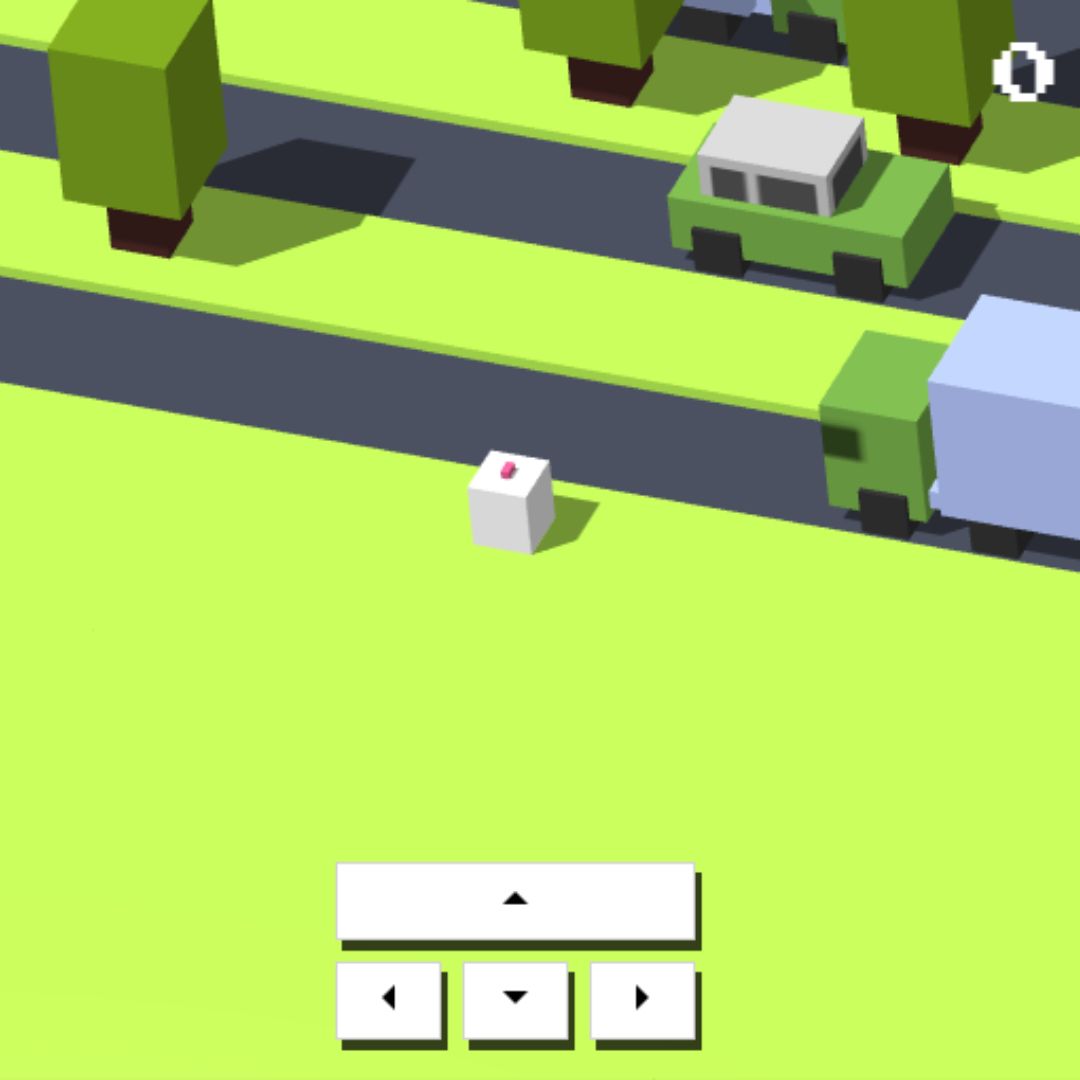
Modeled after Crossy Road, this game challenges players to guide a character through a series of obstacles, such as roads and rivers, with the goal of reaching the other side.
Benefits: Enhances quick decision-making and reflexes. Teaches pattern recognition as players identify safe moments to advance. Offers a casual yet engaging experience with endless gameplay. Additionally, it introduces risk assessment and coordination as players navigate dynamic environments.
.jpg)
A sliding puzzle game where players combine matching numbered tiles by moving them in four directions. When two tiles with the same number collide, they merge into a new tile with the sum of their values. The objective is to reach the elusive "2048" tile.
Benefits: Enhances strategic thinking and planning. Improves numerical and pattern recognition skills. Teaches spatial reasoning and decision-making. Provides a challenging yet rewarding experience, promoting perseverance and logical reasoning. Additionally, it offers insights into algorithmic problem-solving.
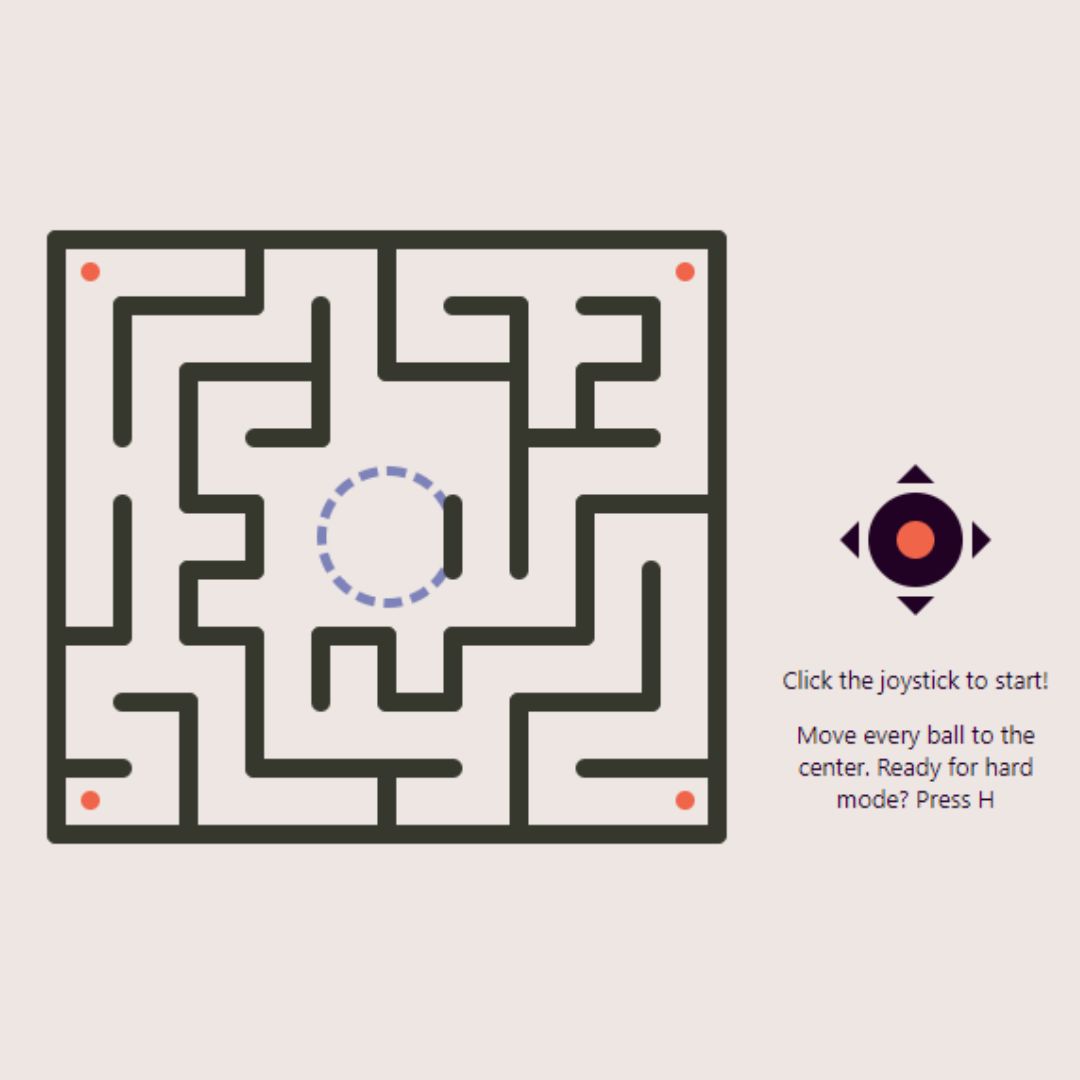
In this game, players control a ball or object within a maze by tilting their device or using arrow keys. The goal is to navigate through the maze, avoiding obstacles and reaching the destination.
Benefits: Enhances fine motor skills as players tilt or control the movement. Improves coordination and balance. Teaches spatial orientation and problem-solving as players navigate through dynamic environments. Provides an interactive and immersive experience, combining physical movement with gameplay.
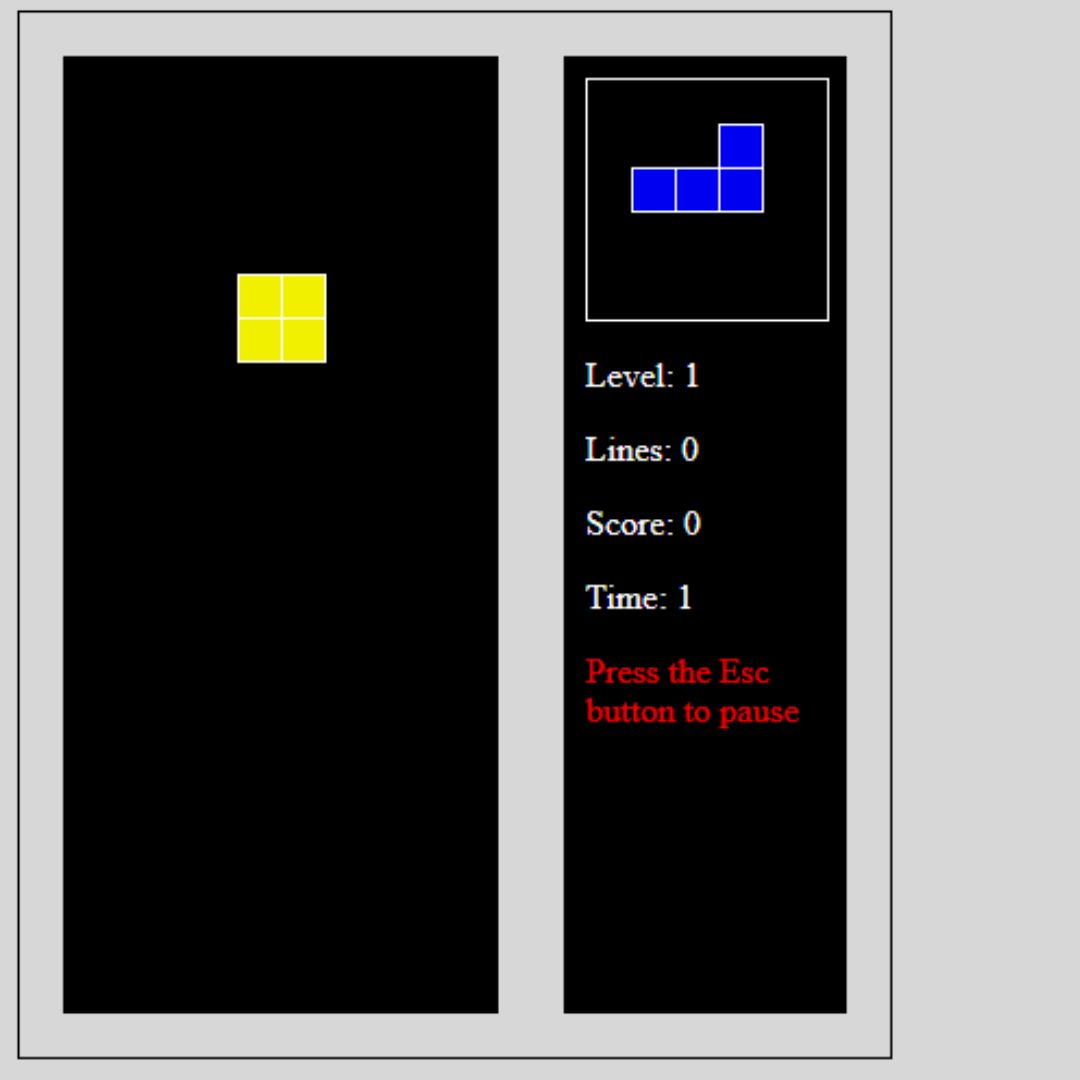
Tetris is a classic puzzle game where players manipulate falling geometric shapes (tetrominoes) to create complete horizontal lines. When a line is formed, it disappears, and the player earns points. The goal is to prevent the blocks from reaching the top of the screen.
Benefits: Enhances spatial awareness and pattern recognition. Improves hand-eye coordination and quick decision-making. Teaches strategic planning as players must efficiently arrange and rotate falling shapes. Provides a challenging and addictive experience, promoting focus and mental agility.
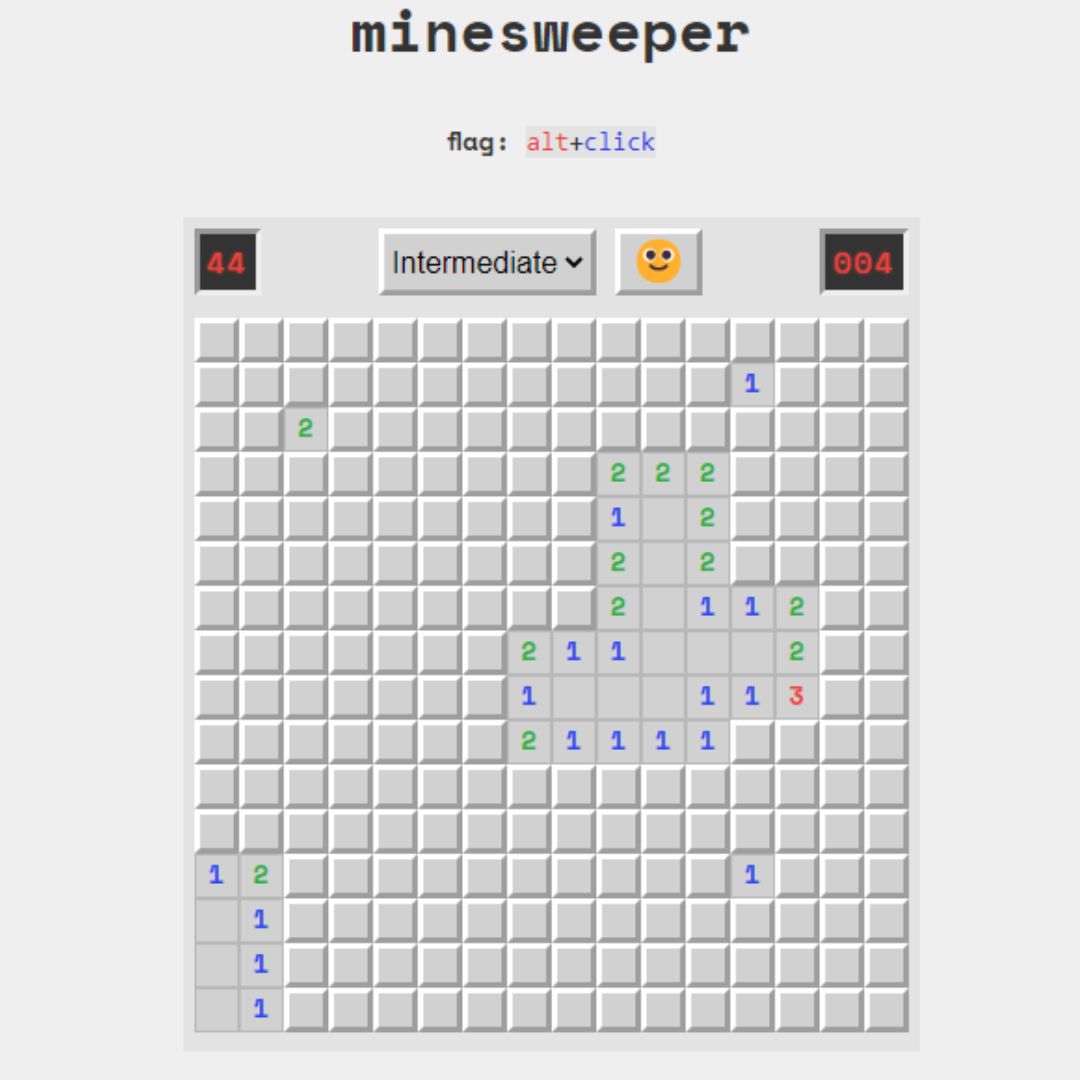
A logic-based puzzle game where players reveal hidden mines on a grid by strategically clicking on tiles. Numbers on the revealed tiles indicate the proximity of mines, and the goal is to clear the entire grid without triggering any mines.
Benefits: Enhances logical reasoning and deductive skills. Improves risk assessment and decision-making. Teaches systematic problem-solving as players uncover safe tiles. Provides a mentally stimulating experience that requires attention to detail and concentration.
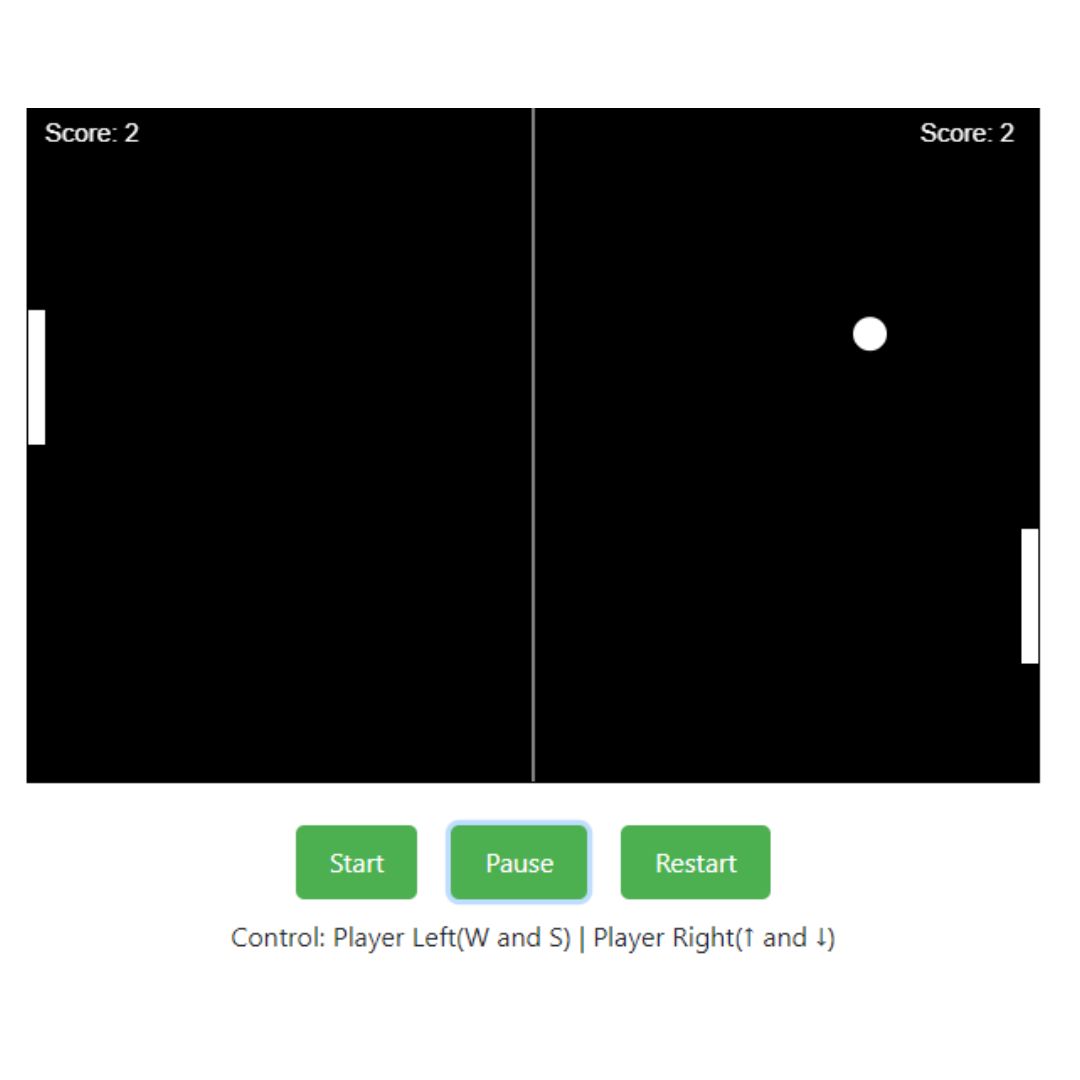
A virtual adaptation of the classic table tennis game, where players control paddles on either side of the screen to hit a ball back and forth. The objective is to score points by making the opponent miss the ball.
Benefits: Enhances hand-eye coordination and reflexes. Improves focus and concentration as players track the fast-moving ball. Teaches strategic thinking and prediction of ball trajectories. Provides a competitive and engaging multiplayer experience, fostering friendly competition and sportsmanship.
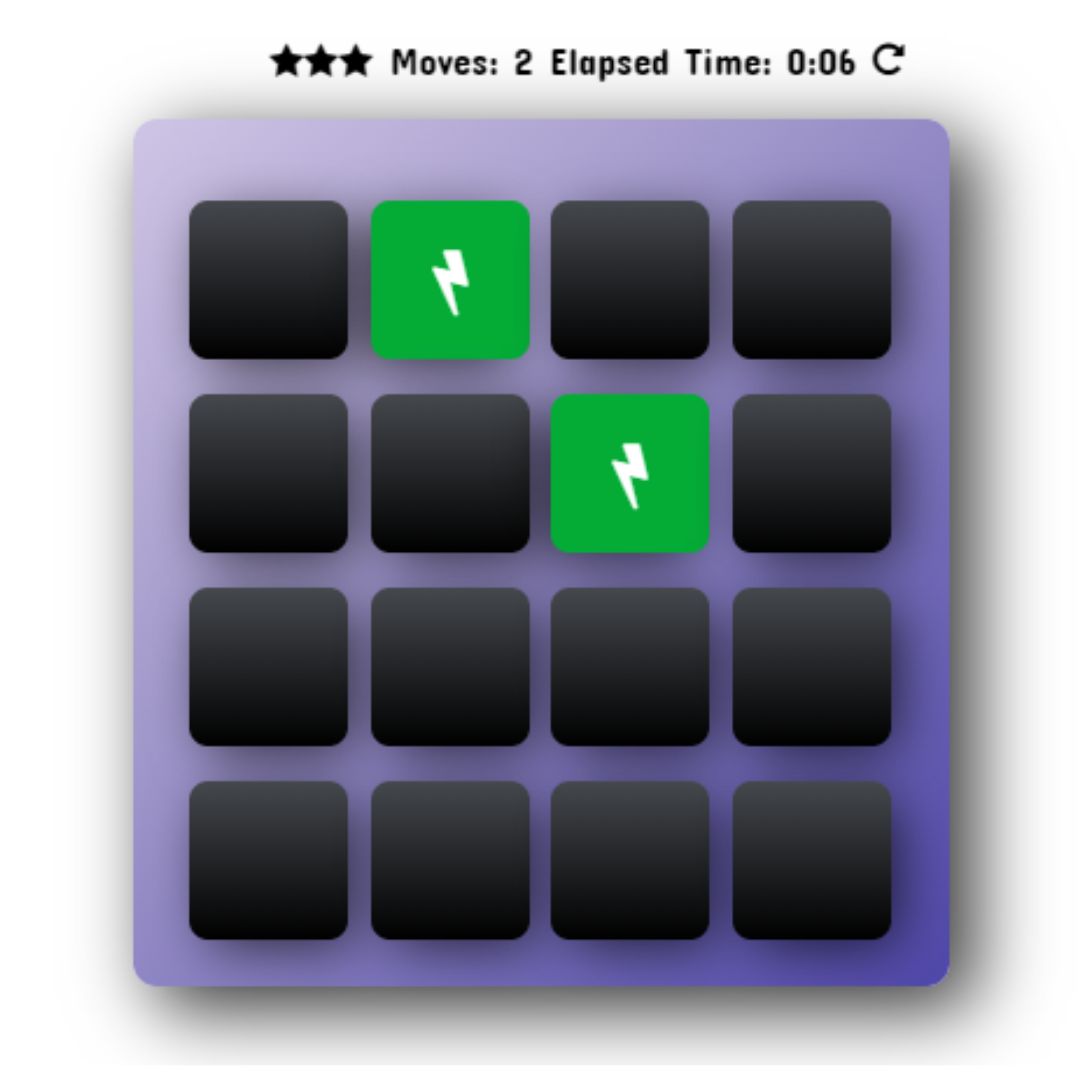
A memory-matching game where players flip cards to reveal images or symbols, aiming to find matching pairs by remembering the card positions.
Benefits: Enhances memory recall and concentration. Improves visual recognition and pattern matching skills. Teaches attention to detail and strategic thinking as players try to remember card locations. Provides an enjoyable and accessible cognitive exercise suitable for all ages.
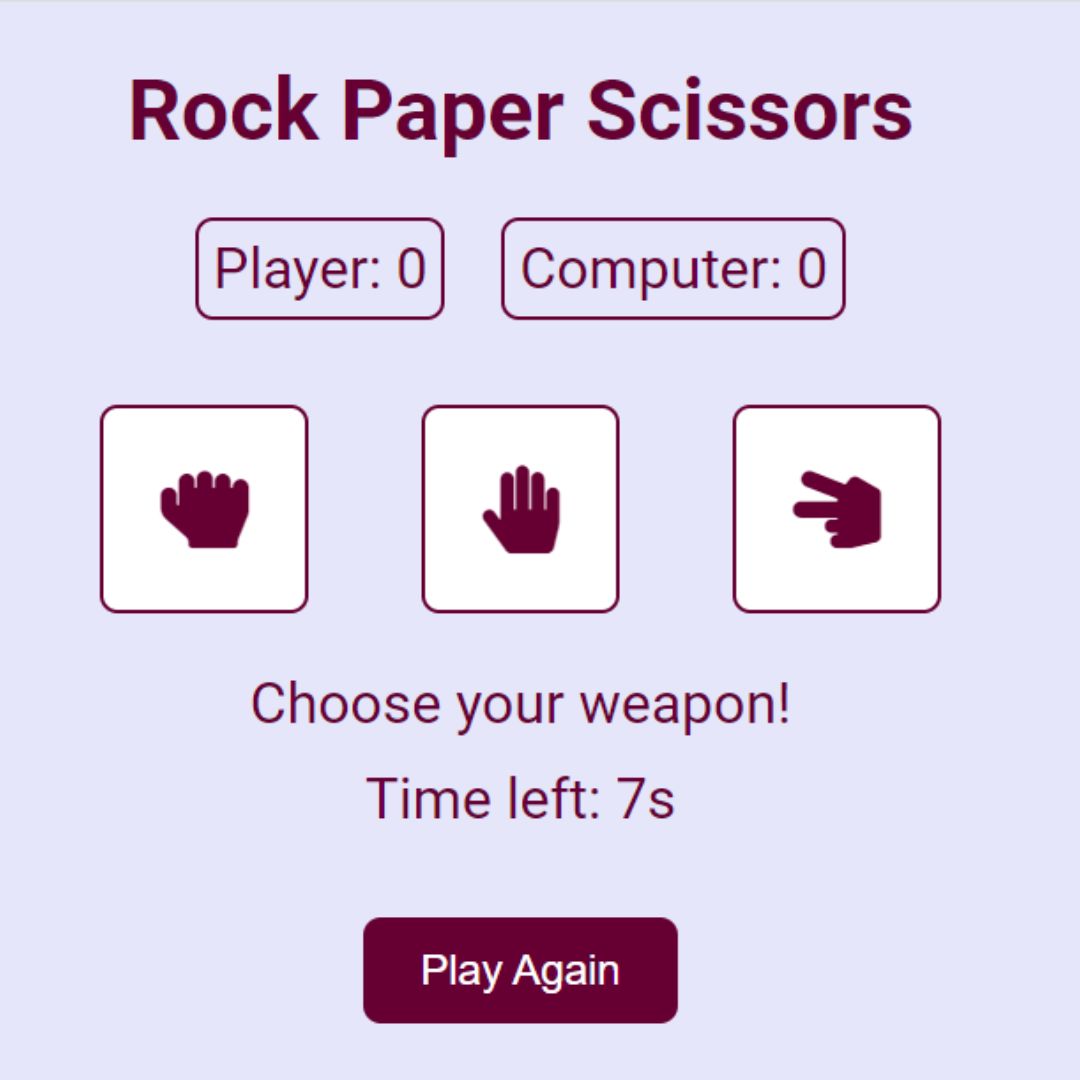
A simple hand game where two players simultaneously choose one of three shapes—rock, paper, or scissors. The winner is determined by the rules: rock crushes scissors, scissors cuts paper, and paper covers rock.
Benefits: Encourages quick decision-making and prediction. Enhances understanding of basic game theory and probability. Teaches the concept of strategic choices and the cyclic nature of outcomes. Provides a quick and accessible activity for fun and friendly competition.
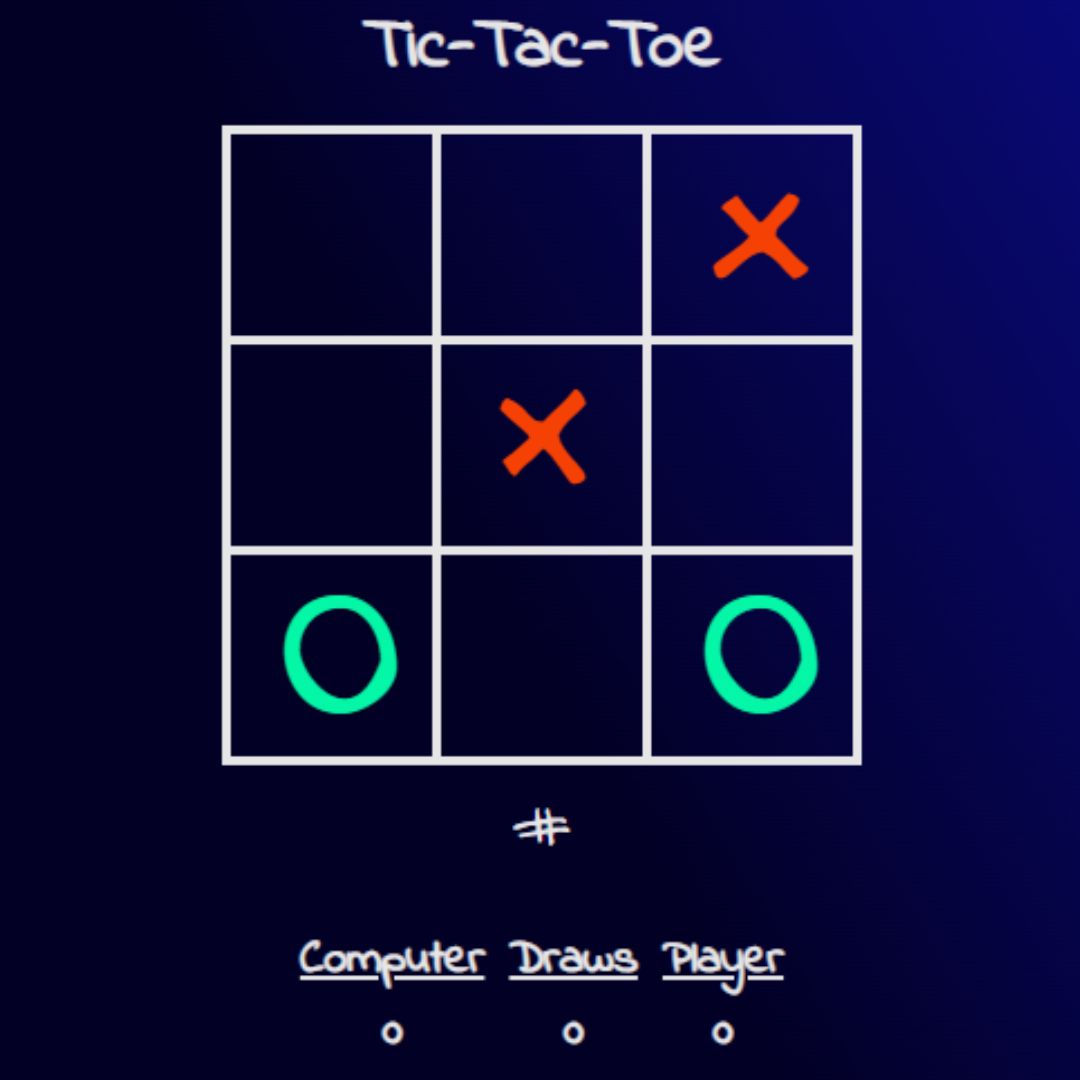
A classic two-player game played on a 3x3 grid where players take turns marking Xs and Os in an attempt to form a horizontal, vertical, or diagonal line of their symbol. The goal is to achieve three in a row.
Benefits: Teaches strategic thinking and planning. Enhances pattern recognition and spatial awareness. Promotes decision-making skills as players anticipate and block their opponent's moves.
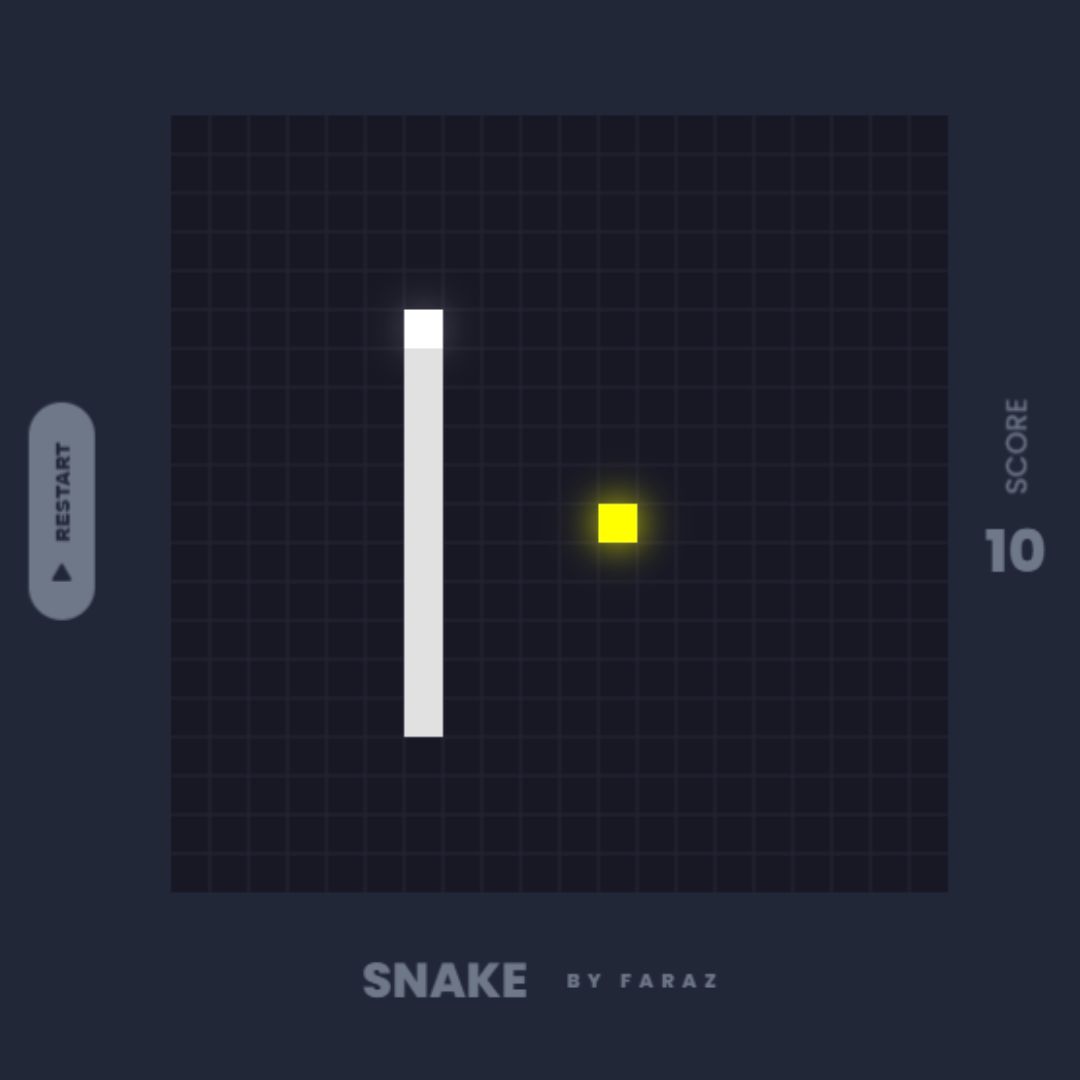
A classic arcade game where players control a snake that moves around the screen, eating food to grow longer. The challenge is to avoid collisions with the snake's own body and the boundaries of the playing area.
Benefits: Enhances hand-eye coordination and reflexes. Improves strategic planning as players navigate and plan the snake's path. Teaches the concept of continuous movement and growth within a confined space. Additionally, it introduces basic game mechanics such as scoring and collision detection.
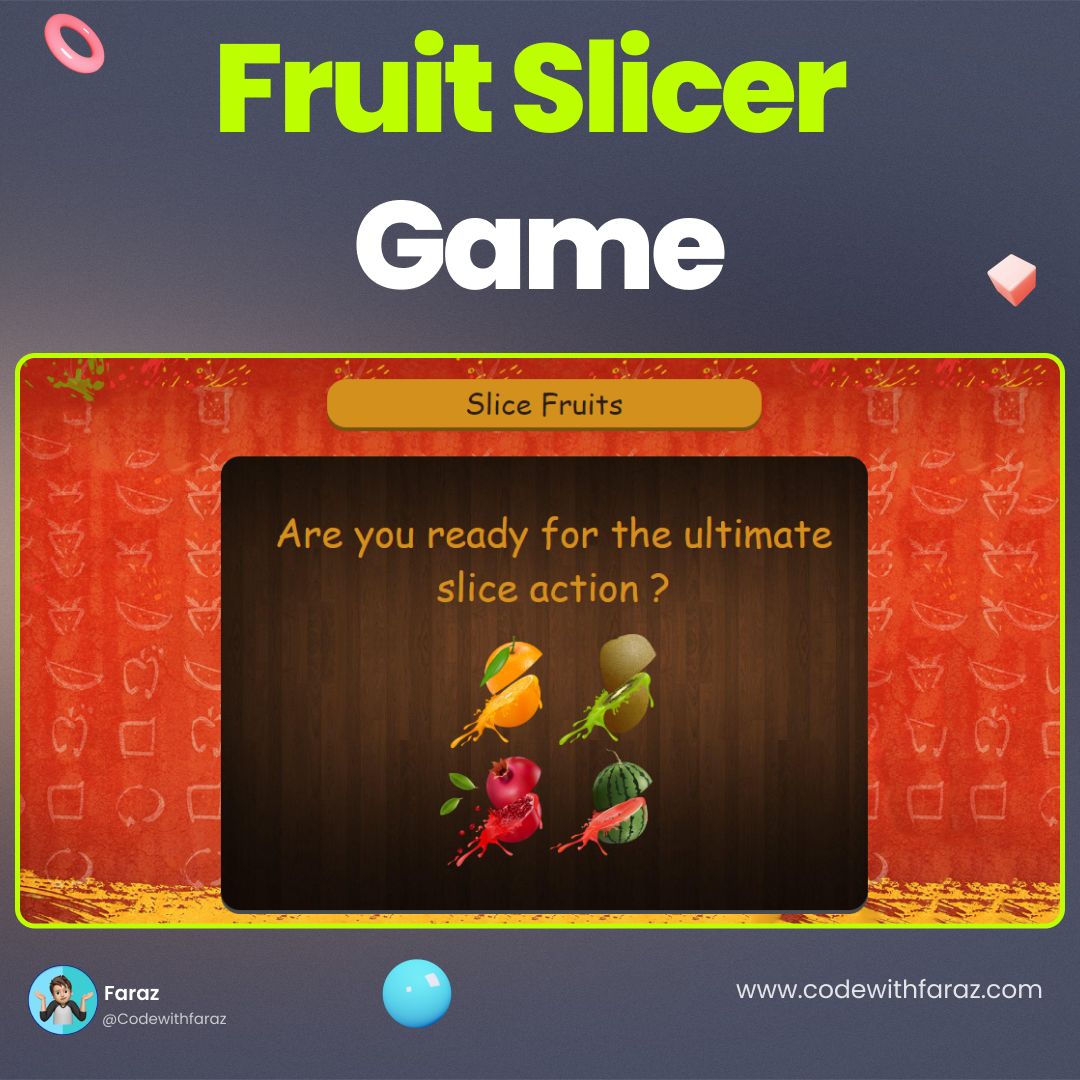
In this game, players swipe their finger across the screen to slice through falling fruits. The goal is to slice as many fruits as possible while avoiding slicing bombs or other obstacles.
Benefits: Enhances hand-eye coordination and reflexes. Improves focus and concentration as players react quickly to the movement of fruits. Teaches precision in finger movements for efficient slicing.
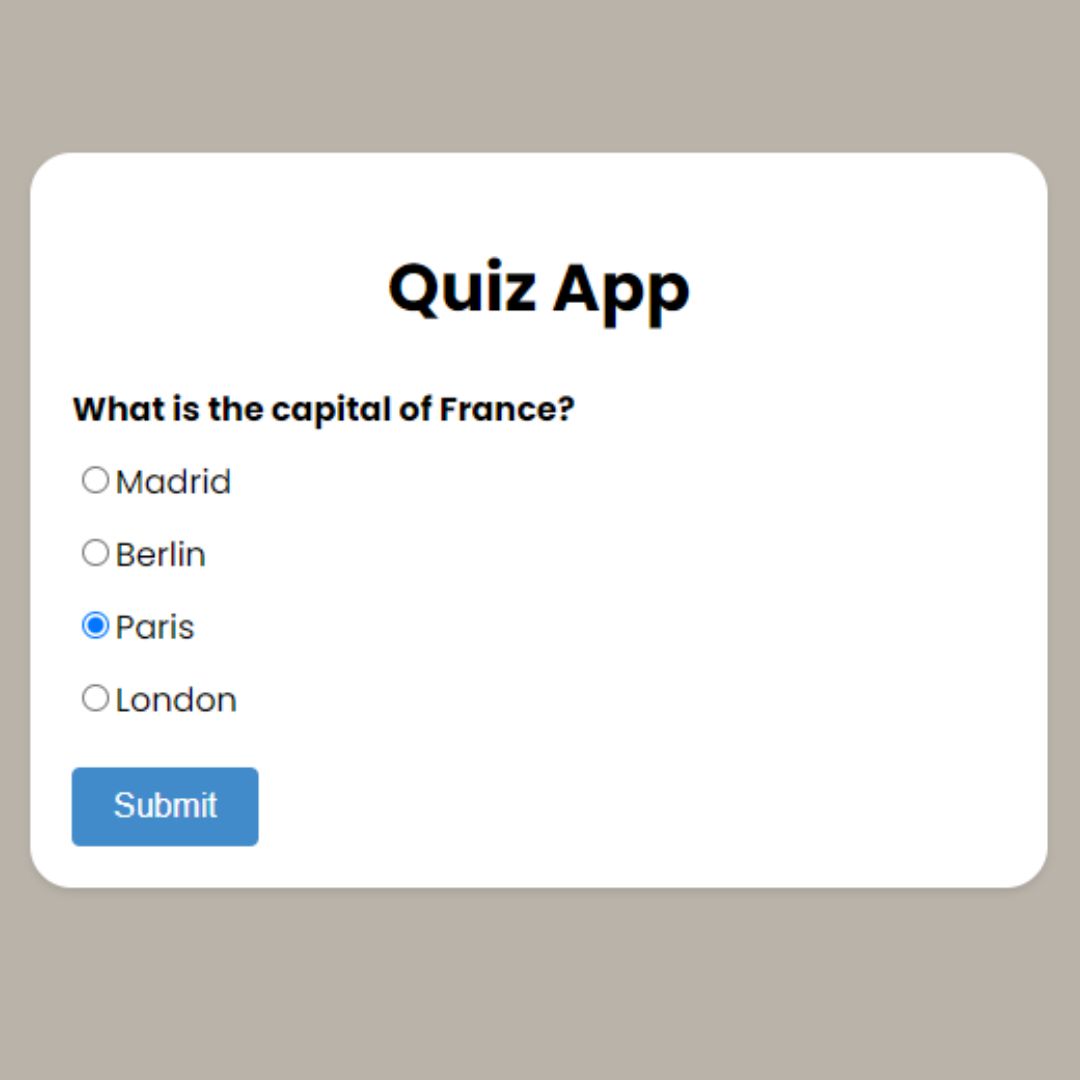
A knowledge-based game where players answer questions across various categories. Players typically earn points for correct answers and may face consequences for incorrect ones.
Benefits: Enhances general knowledge and retention of information. Encourages critical thinking and problem-solving. Teaches time management and decision-making under pressure in timed quizzes. Provides an interactive way to learn and reinforce facts across different subjects.
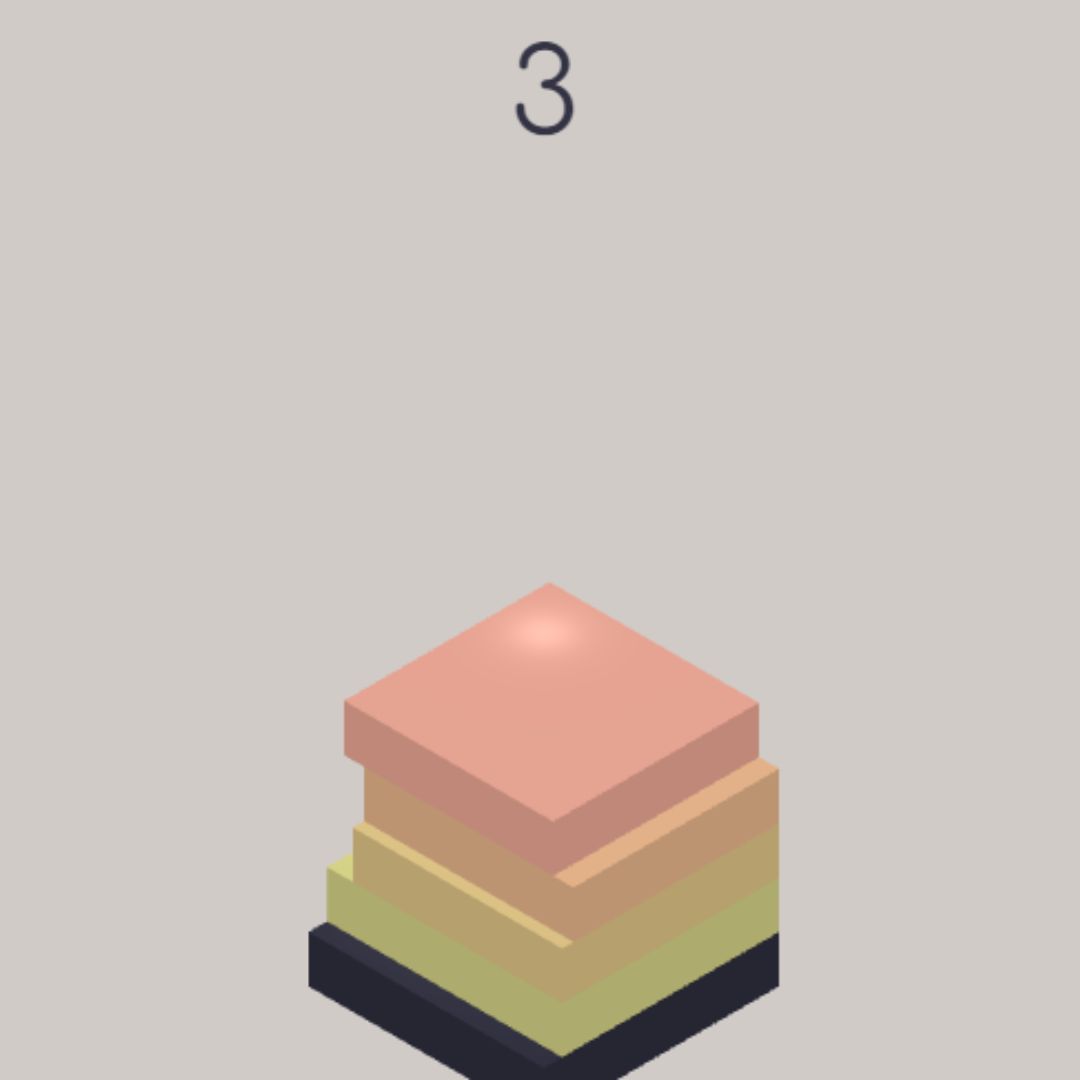
A stacking game where players must build a tower by precisely placing blocks on top of each other. The goal is to create the tallest tower possible without letting it collapse.
Benefits: Enhances fine motor skills and hand-eye coordination. Improves spatial awareness and balance as players carefully stack blocks. Teaches strategic decision-making as players assess the stability of the tower.
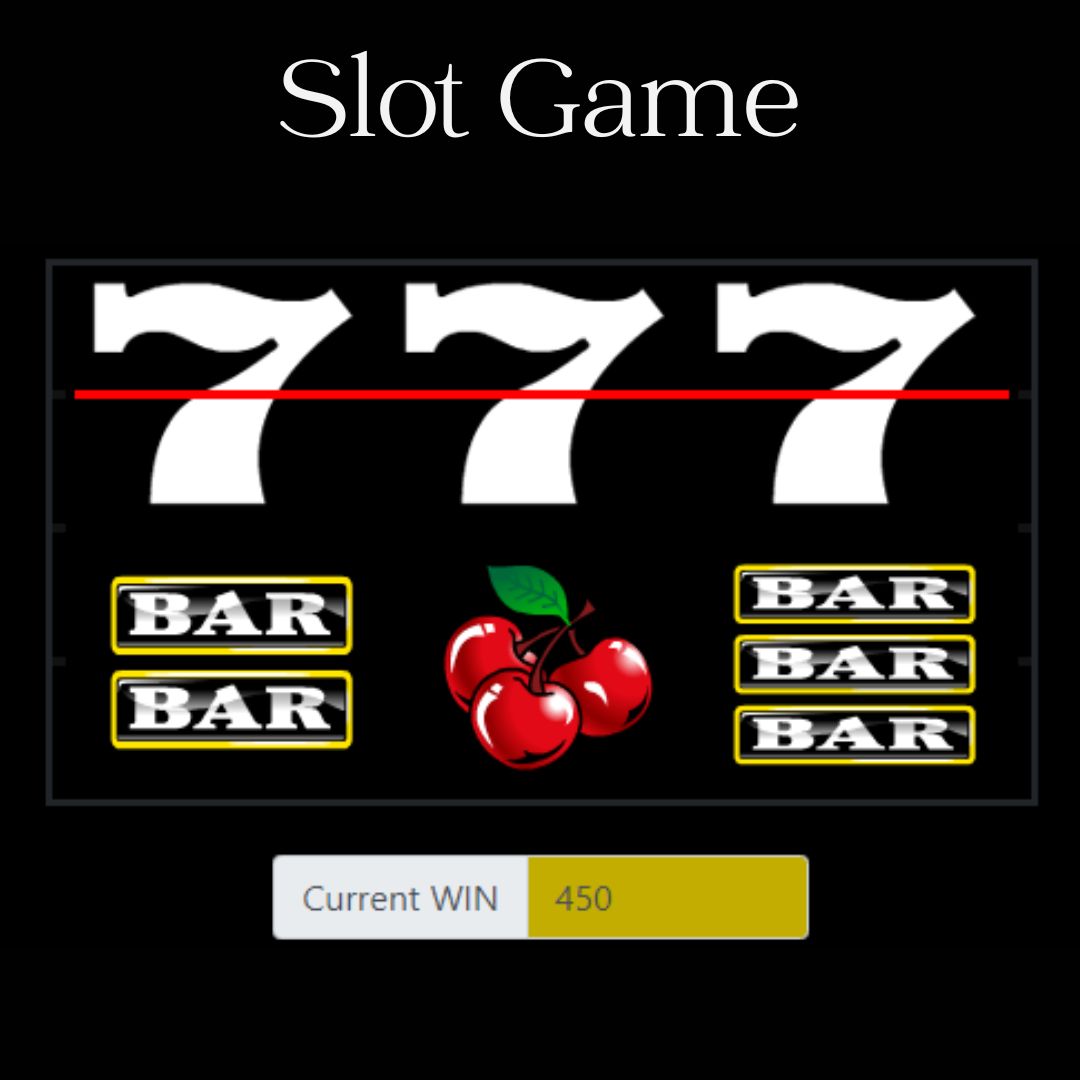
A casino-style game where players spin a set of reels containing various symbols. The objective is to align matching symbols across designated paylines to win rewards or trigger bonus features.
Benefits: Provides entertainment with a risk-free simulation of slot machine mechanics. Enhances pattern recognition as players look for winning combinations. Teaches probability and luck-based gameplay.
In conclusion, learning JavaScript through game development offers a unique and enjoyable experience for beginners. The curated list of 20+ JavaScript games with source code serves as a valuable resource to kickstart your coding adventure. By exploring and modifying source codes, aspiring programmers can gain practical insights and build a strong foundation in the language.
Q1: Can I modify the source code of these games?
A1: Absolutely! Modifying the source code is encouraged as it enhances your understanding and creativity.
Q2: Are these games suitable for absolute beginners?
A2: Yes, the list includes games of varying complexities, ensuring there's something for everyone, including beginners.
Q3: How can I use these games to improve my JavaScript skills?
A3: Study the source code, understand the logic, and experiment with modifications to deepen your understanding.
Q4: Is game development a good way to learn programming for beginners?
A4: Yes, game development provides a practical and engaging way to apply programming concepts.
Q5: Where can I find more resources for JavaScript game development?
A5: Explore online platforms, forums, and communities dedicated to game development for a wealth of additional resources.
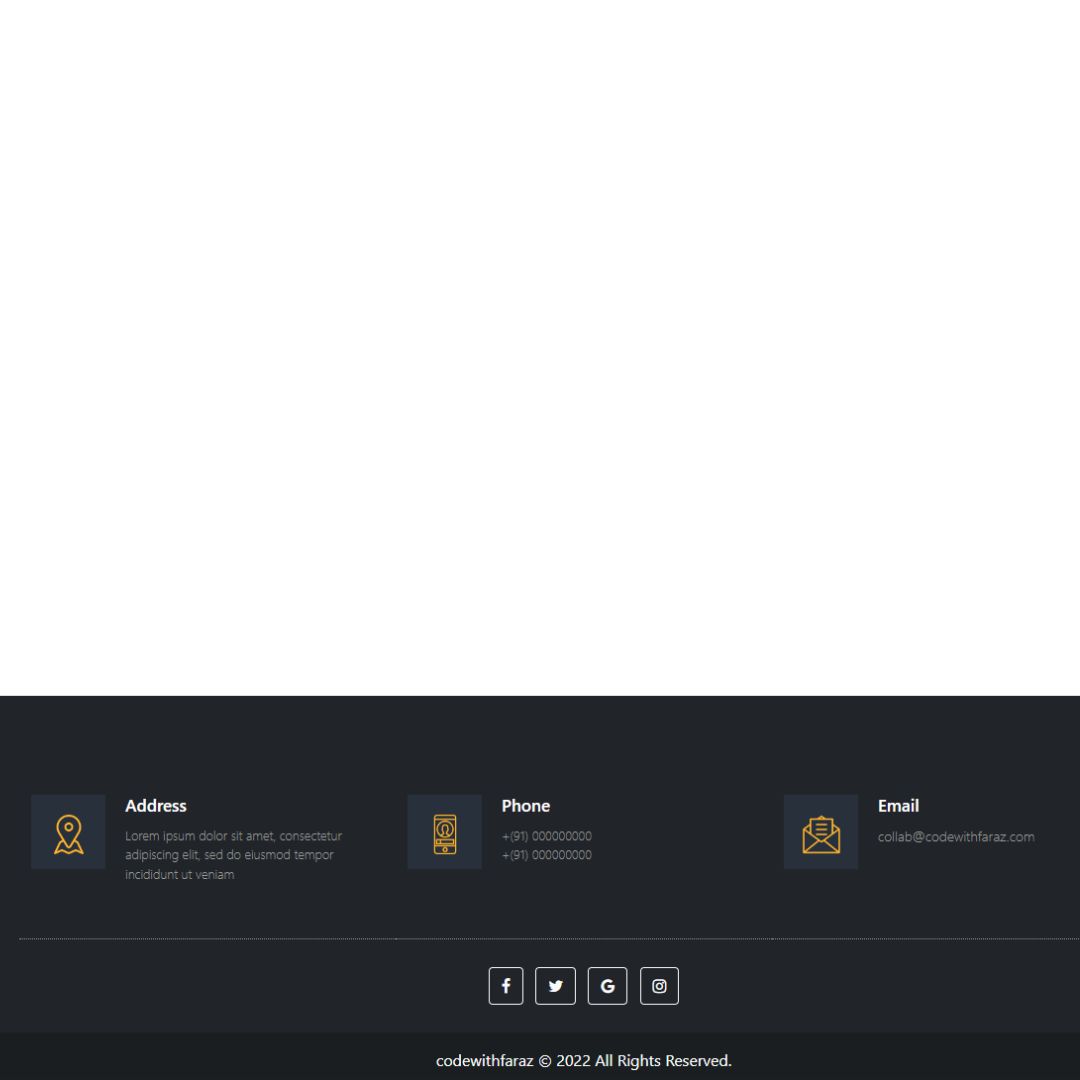
That’s a wrap!
I hope you enjoyed this article
Did you like it? Let me know in the comments below 🔥 and you can support me by buying me a coffee.
And don’t forget to sign up to our email newsletter so you can get useful content like this sent right to your inbox!
Thanks! Faraz 😊
Subscribe to my Newsletter
Get the latest posts delivered right to your inbox, latest post.
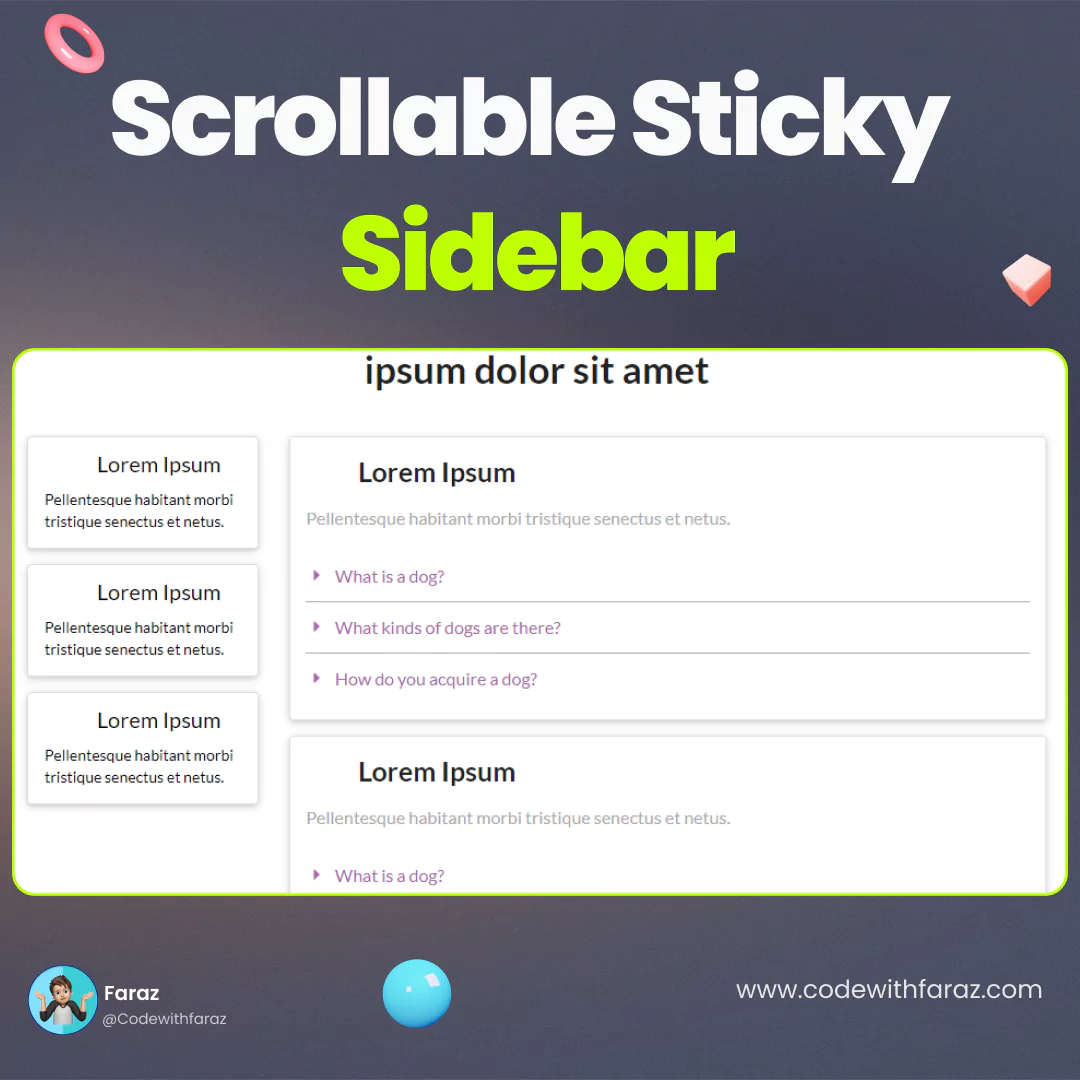
How to Create a Scrollable Sticky Sidebar with HTML, CSS, and JavaScript
Learn how to enhance your website's usability by implementing a scrollable sticky sidebar using HTML, CSS, and JavaScript. Follow our step-by-step guide for seamless integration.
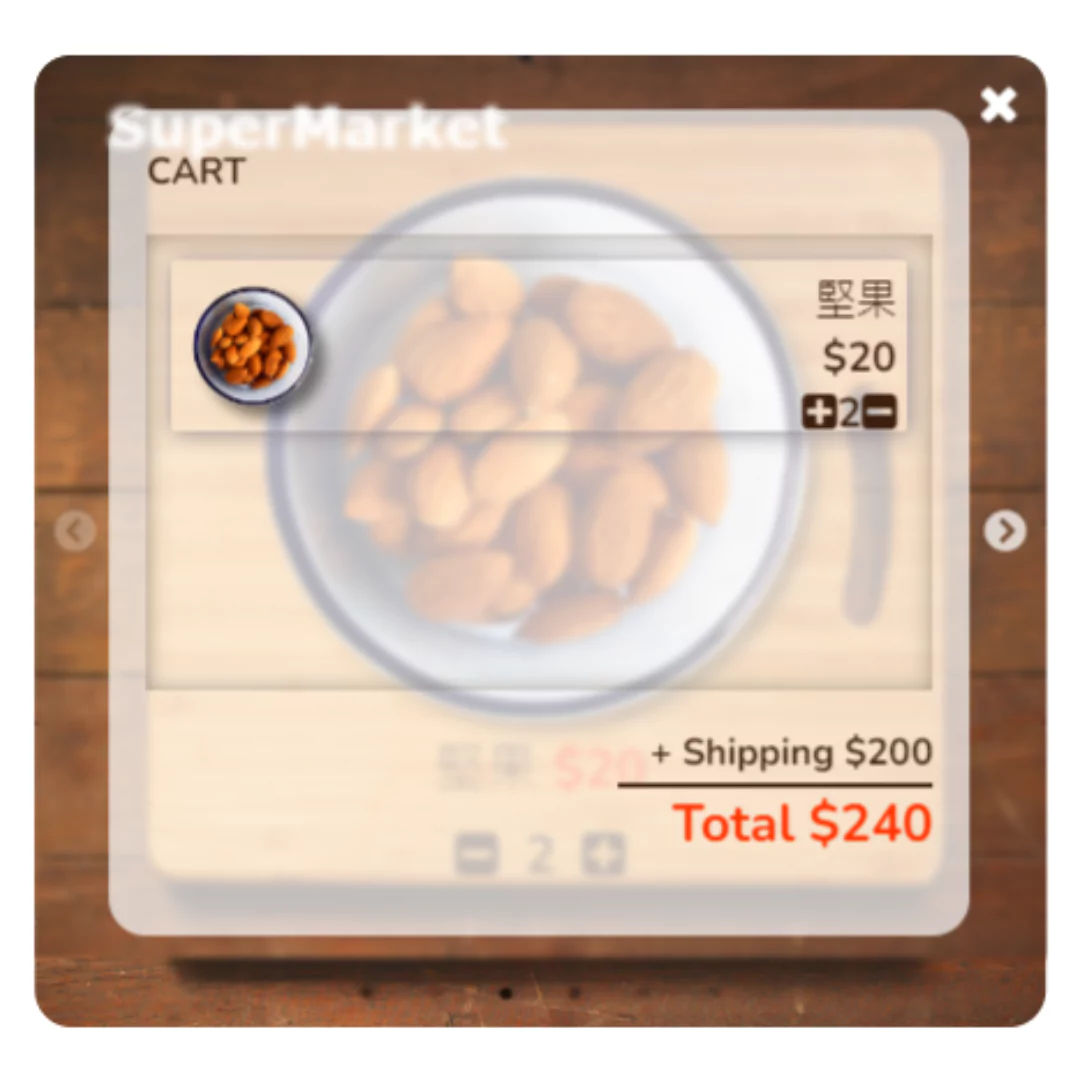
Creating a Shopping Cart Concept Using HTML, CSS, Vue, and Slick.js
May 18, 2024
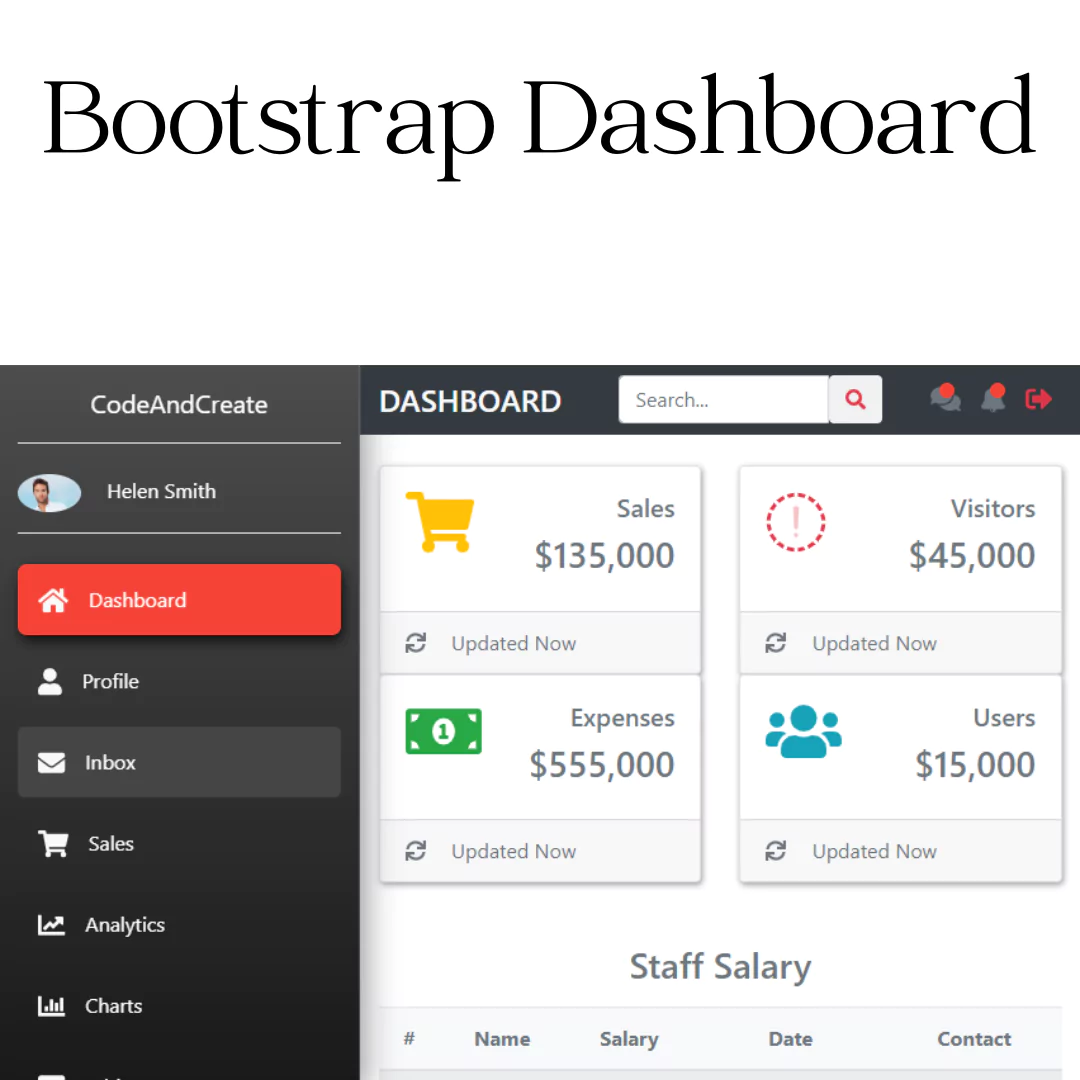
Creating a Responsive Bootstrap Dashboard: Source Code Included
May 14, 2024
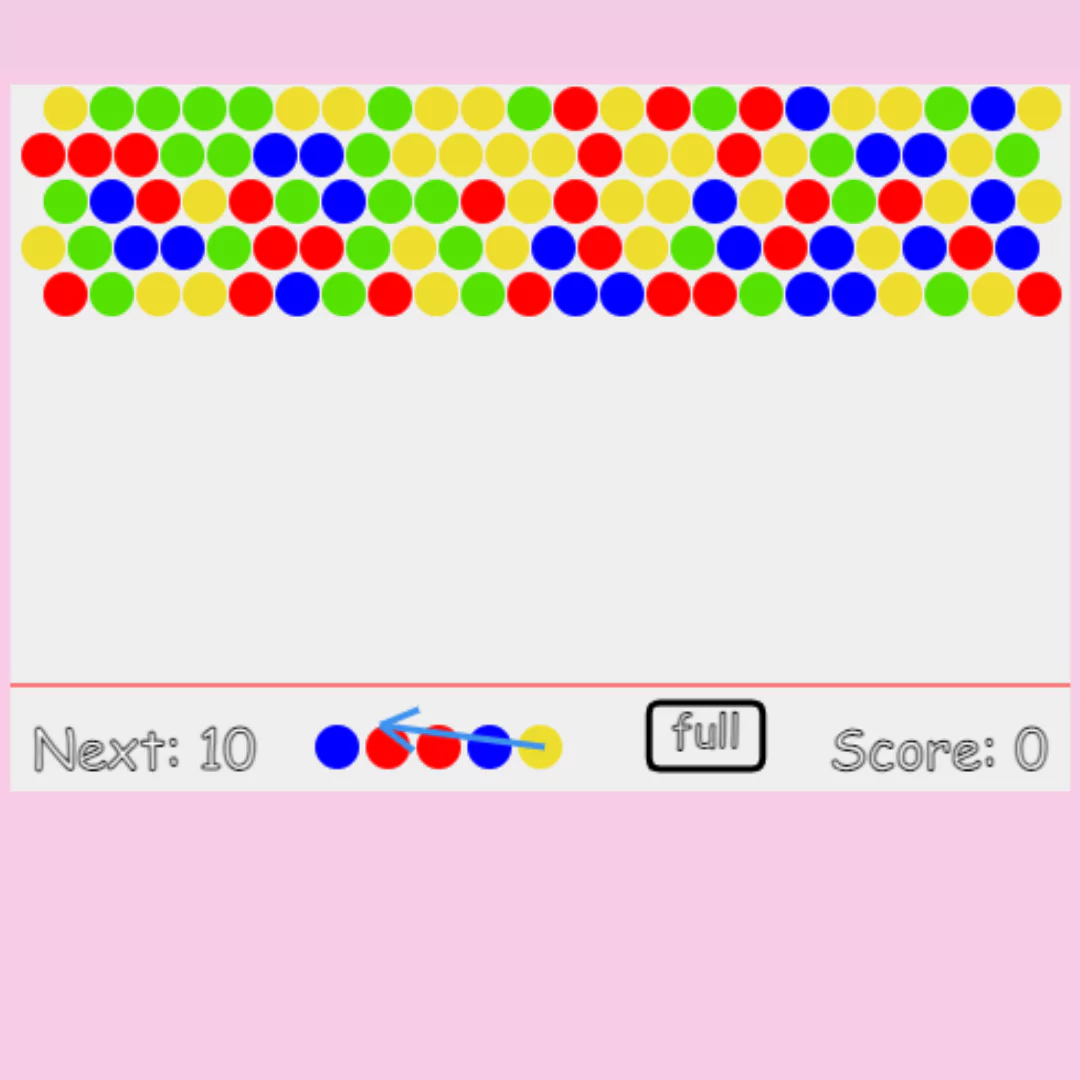
Create Your Own Bubble Shooter Game with HTML and JavaScript
May 01, 2024
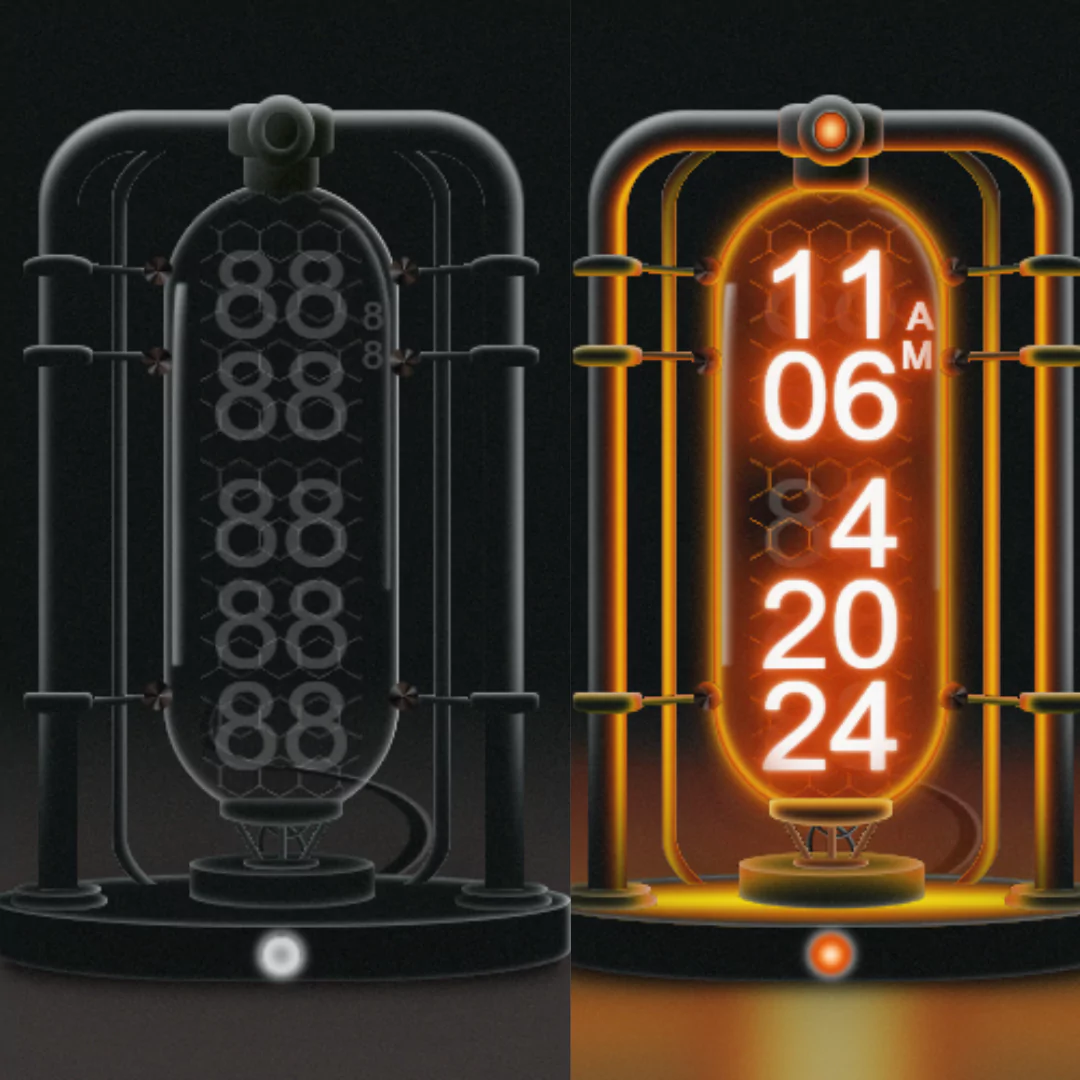
Build Your Own Nixie Tube Clock using HTML, CSS, and JavaScript (Source Code)
April 20, 2024
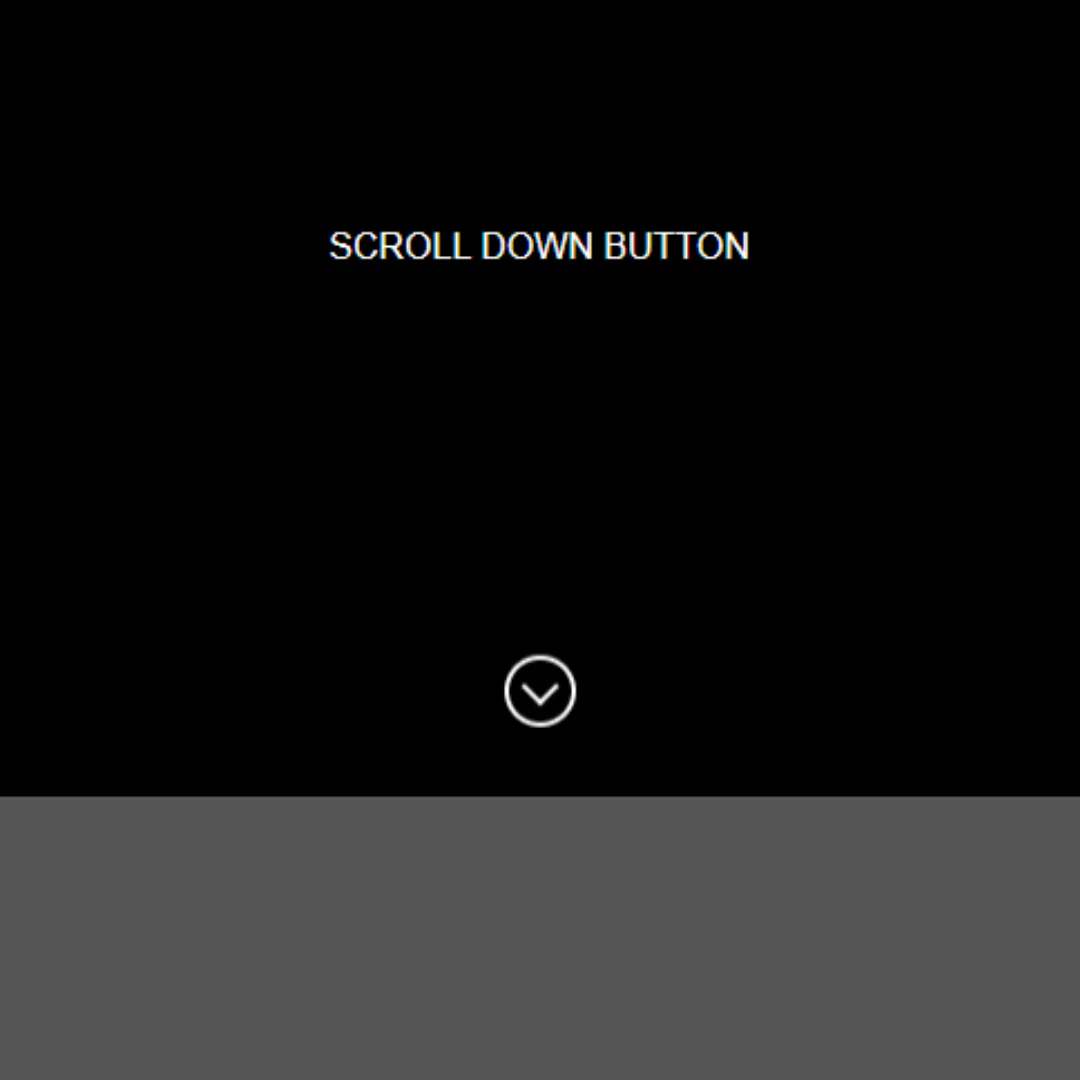
How to Create a Scroll Down Button: HTML, CSS, JavaScript Tutorial
Learn to add a sleek scroll down button to your website using HTML, CSS, and JavaScript. Step-by-step guide with code examples.
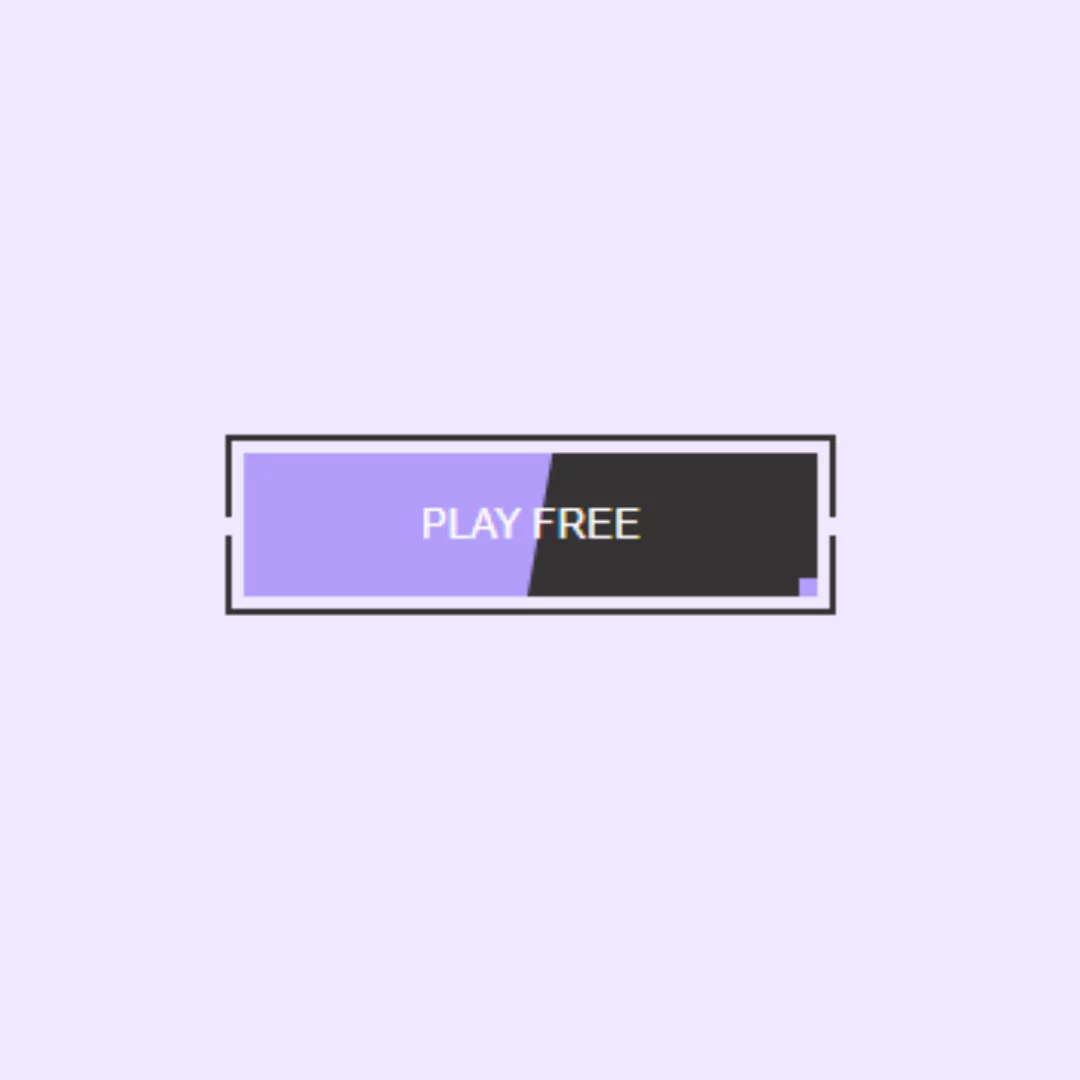
How to Create a Trending Animated Button Using HTML and CSS
March 15, 2024
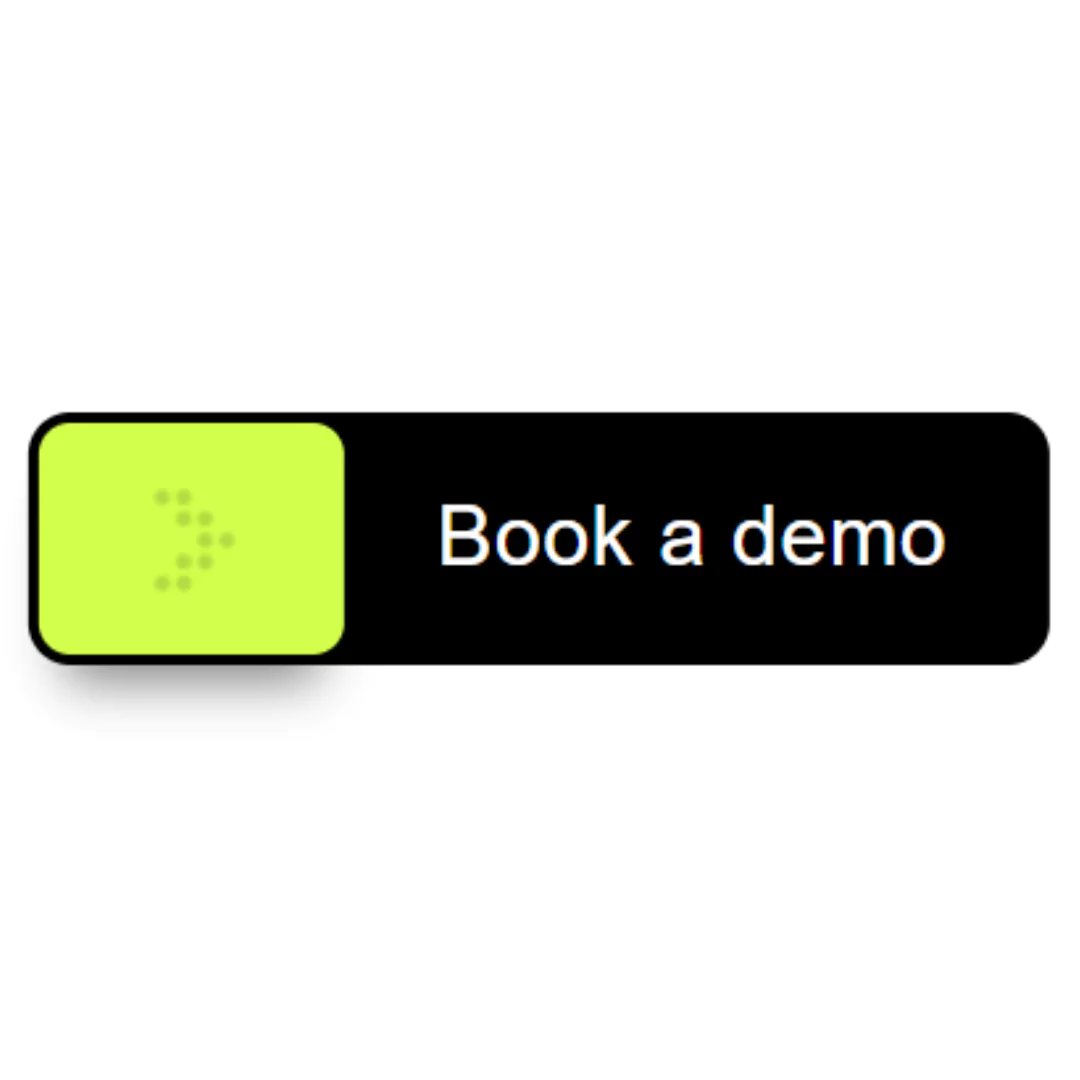
Create Interactive Booking Button with mask-image using HTML and CSS (Source Code)
March 10, 2024
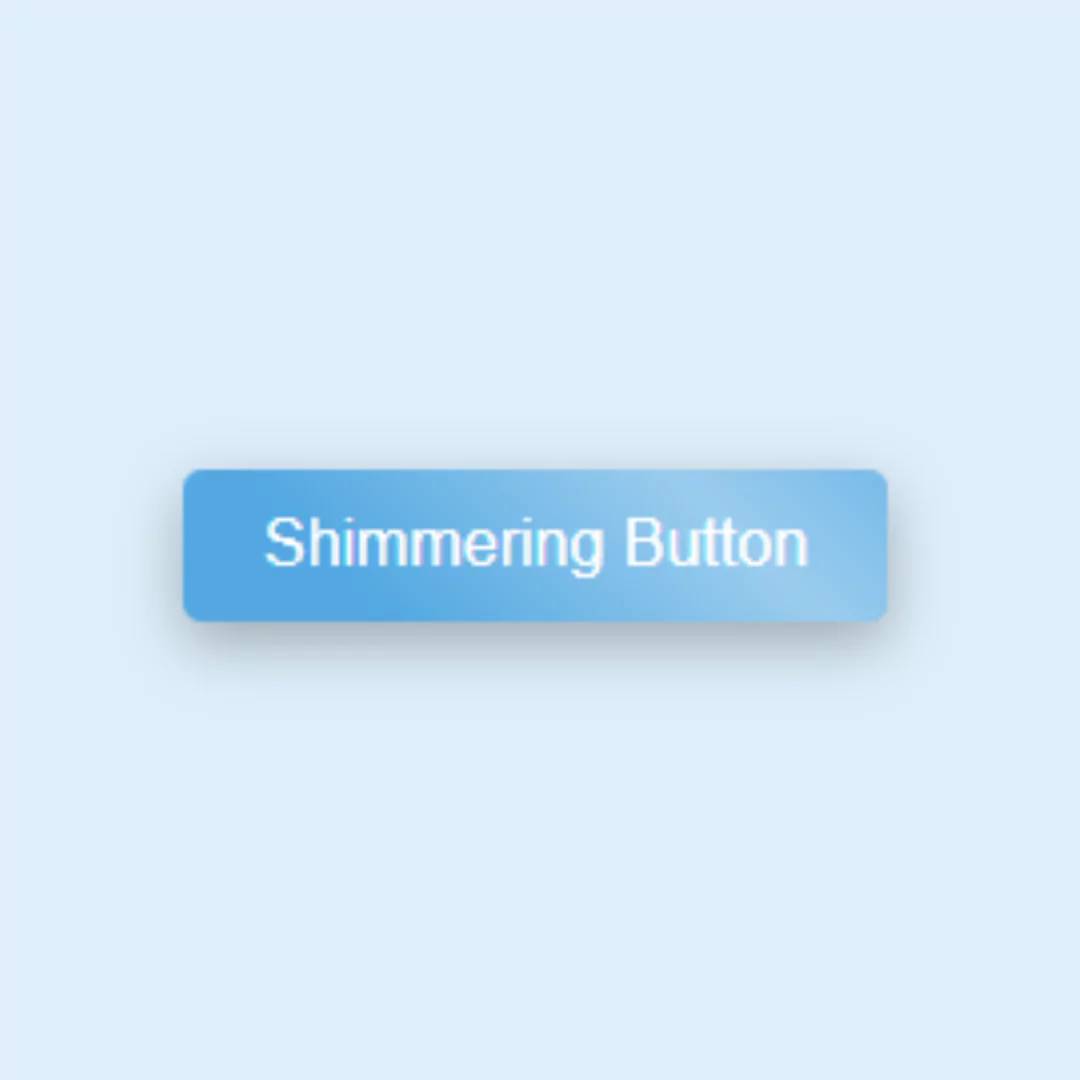
Create Shimmering Effect Button: HTML & CSS Tutorial (Source Code)
March 07, 2024
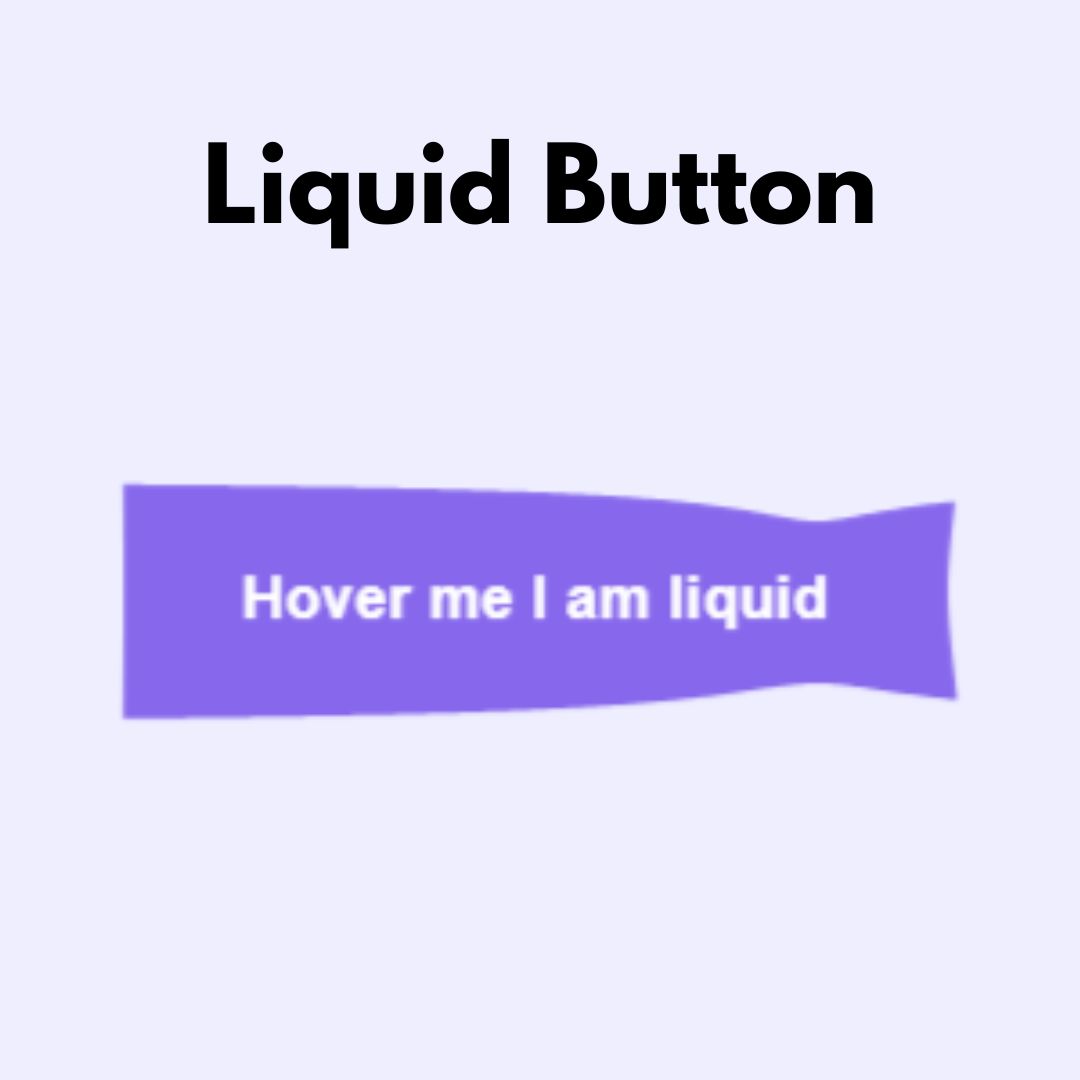
How to Create a Liquid Button with HTML, CSS, and JavaScript (Source Code)
March 01, 2024
Learn how to develop a bubble shooter game using HTML and JavaScript with our easy-to-follow tutorial. Perfect for beginners in game development.
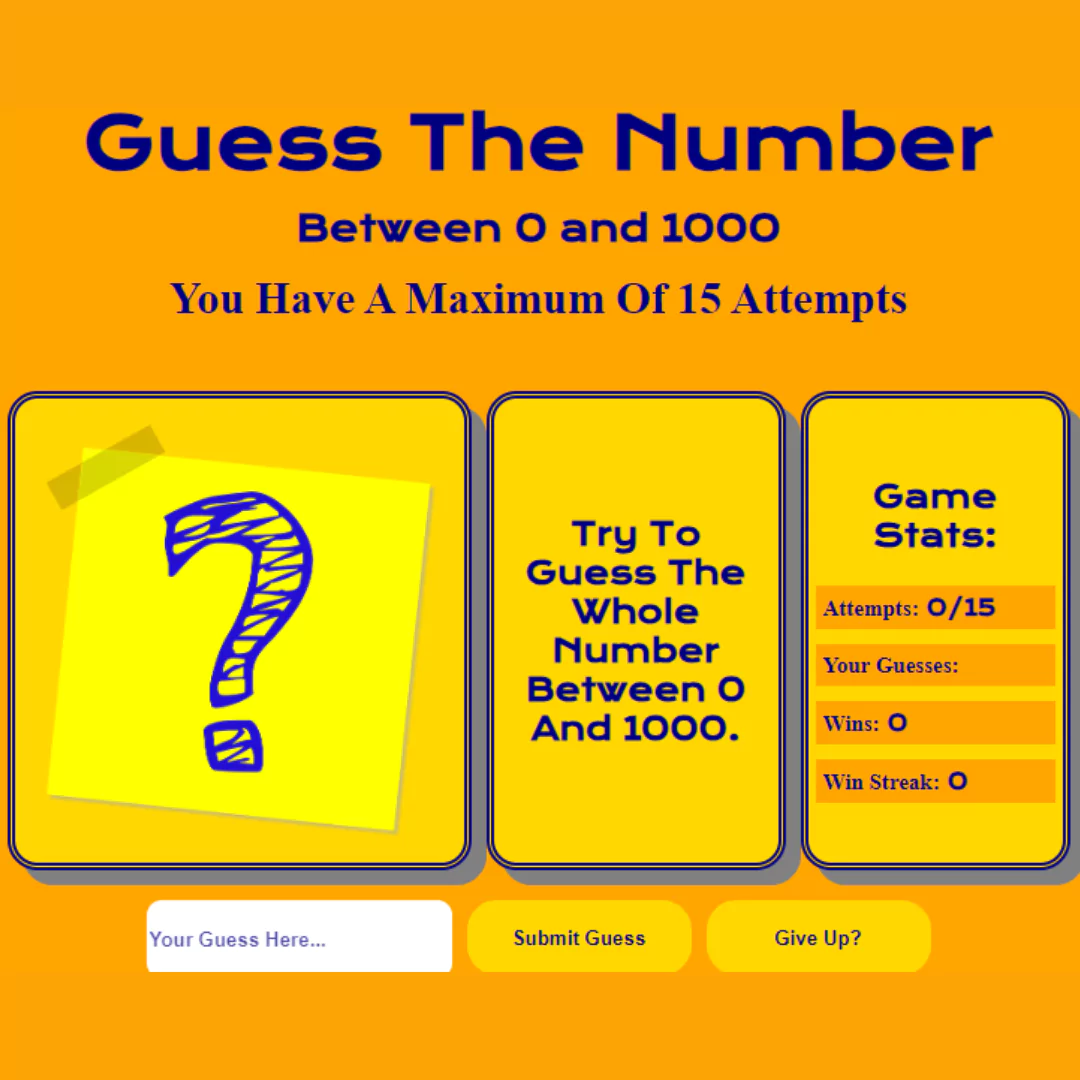
Build a Number Guessing Game using HTML, CSS, and JavaScript | Source Code
April 01, 2024
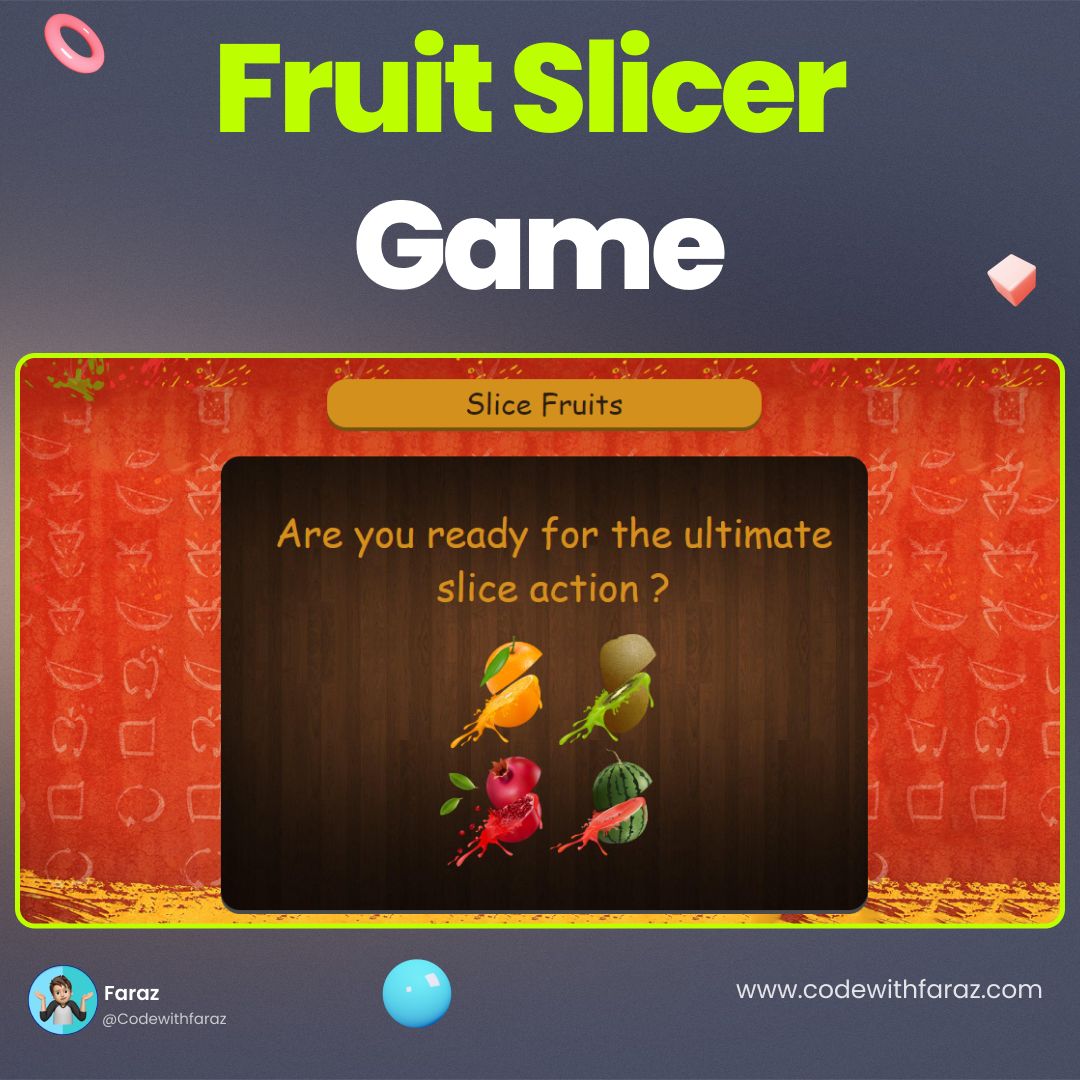
Building a Fruit Slicer Game with HTML, CSS, and JavaScript (Source Code)
December 25, 2023
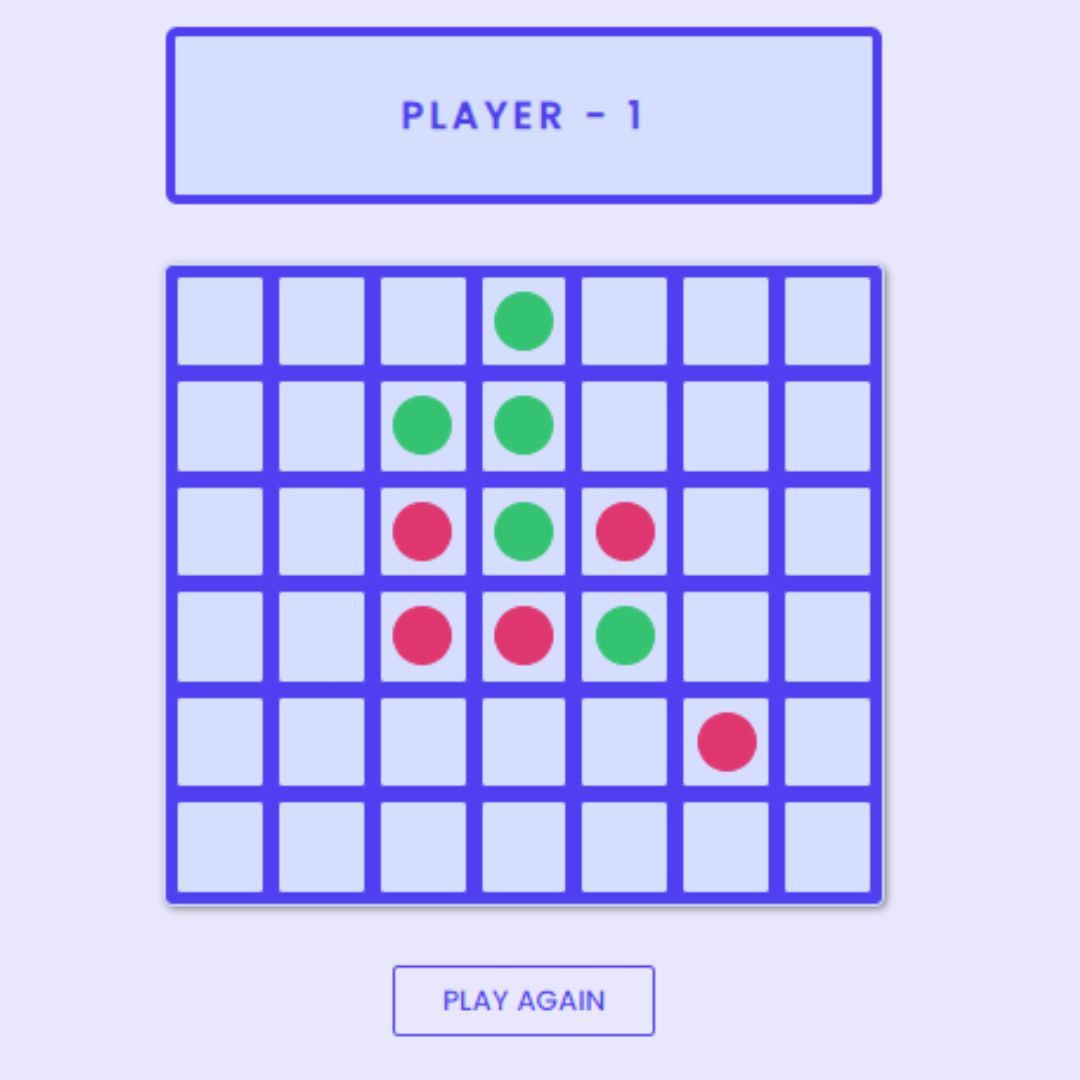
Create Connect Four Game Using HTML, CSS, and JavaScript (Source Code)
December 07, 2023
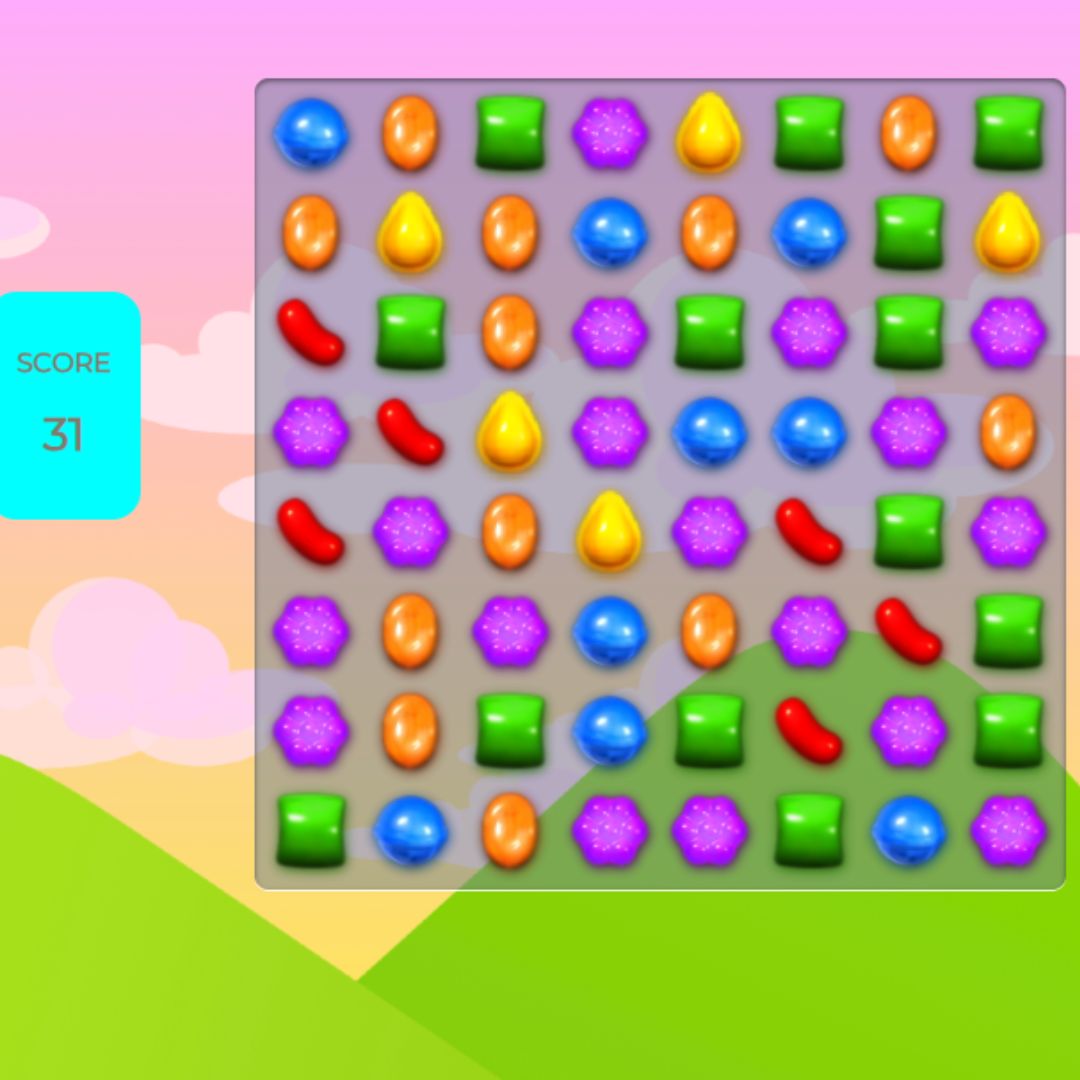
Creating a Candy Crush Clone: HTML, CSS, and JavaScript Tutorial (Source Code)
November 17, 2023
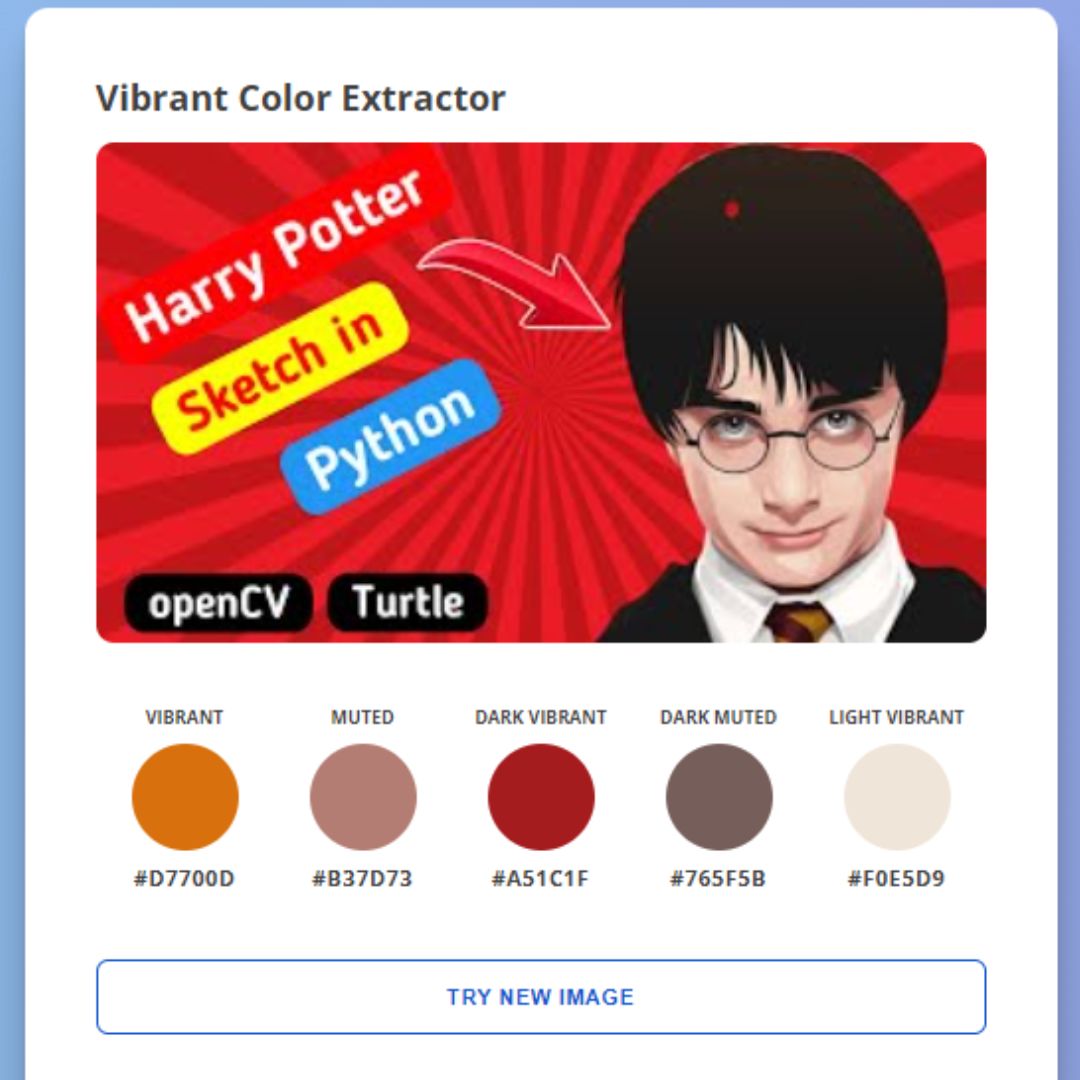
Create Image Color Extractor Tool using HTML, CSS, JavaScript, and Vibrant.js
Master the art of color picking with Vibrant.js. This tutorial guides you through building a custom color extractor tool using HTML, CSS, and JavaScript.
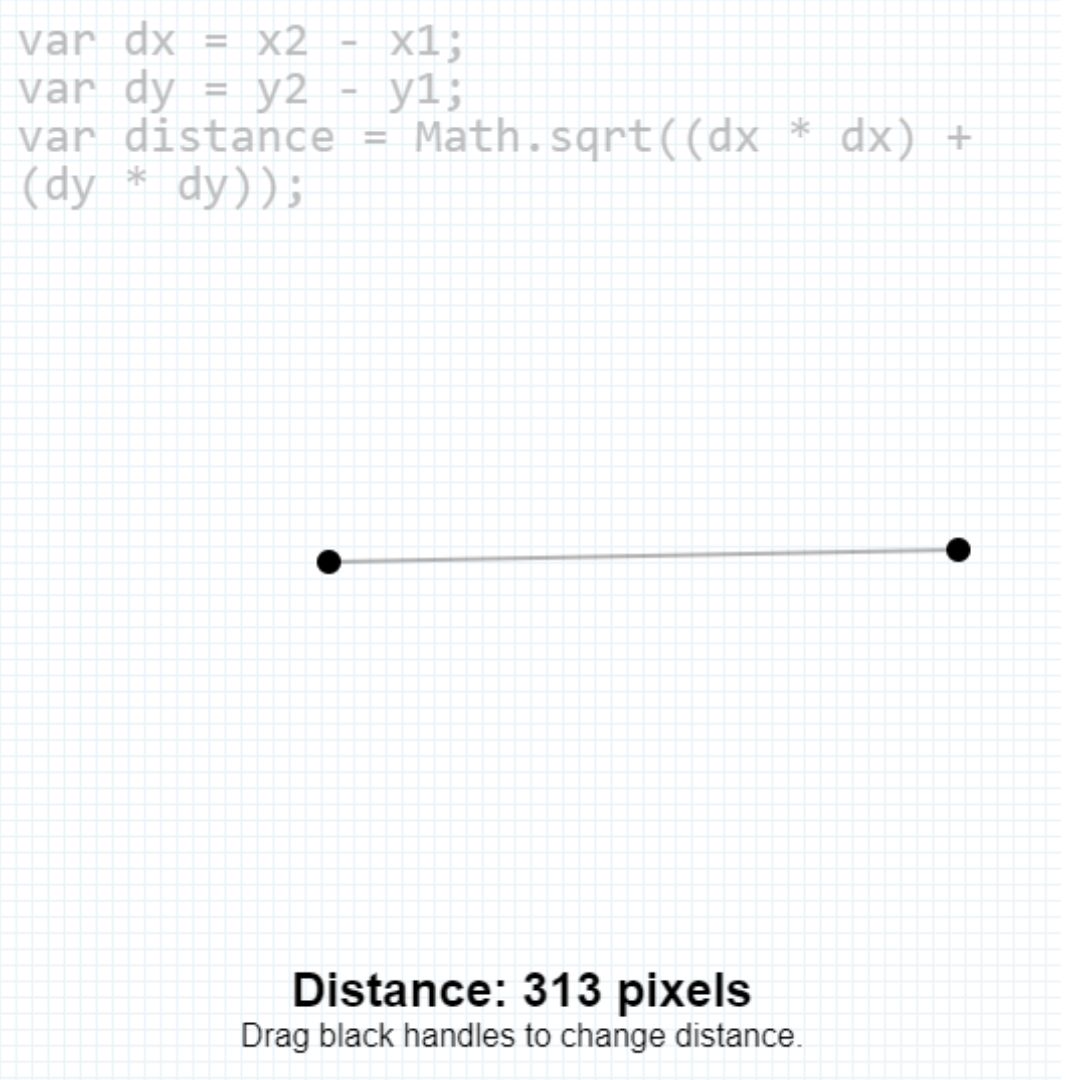
Build a Responsive Screen Distance Measure with HTML, CSS, and JavaScript
January 04, 2024
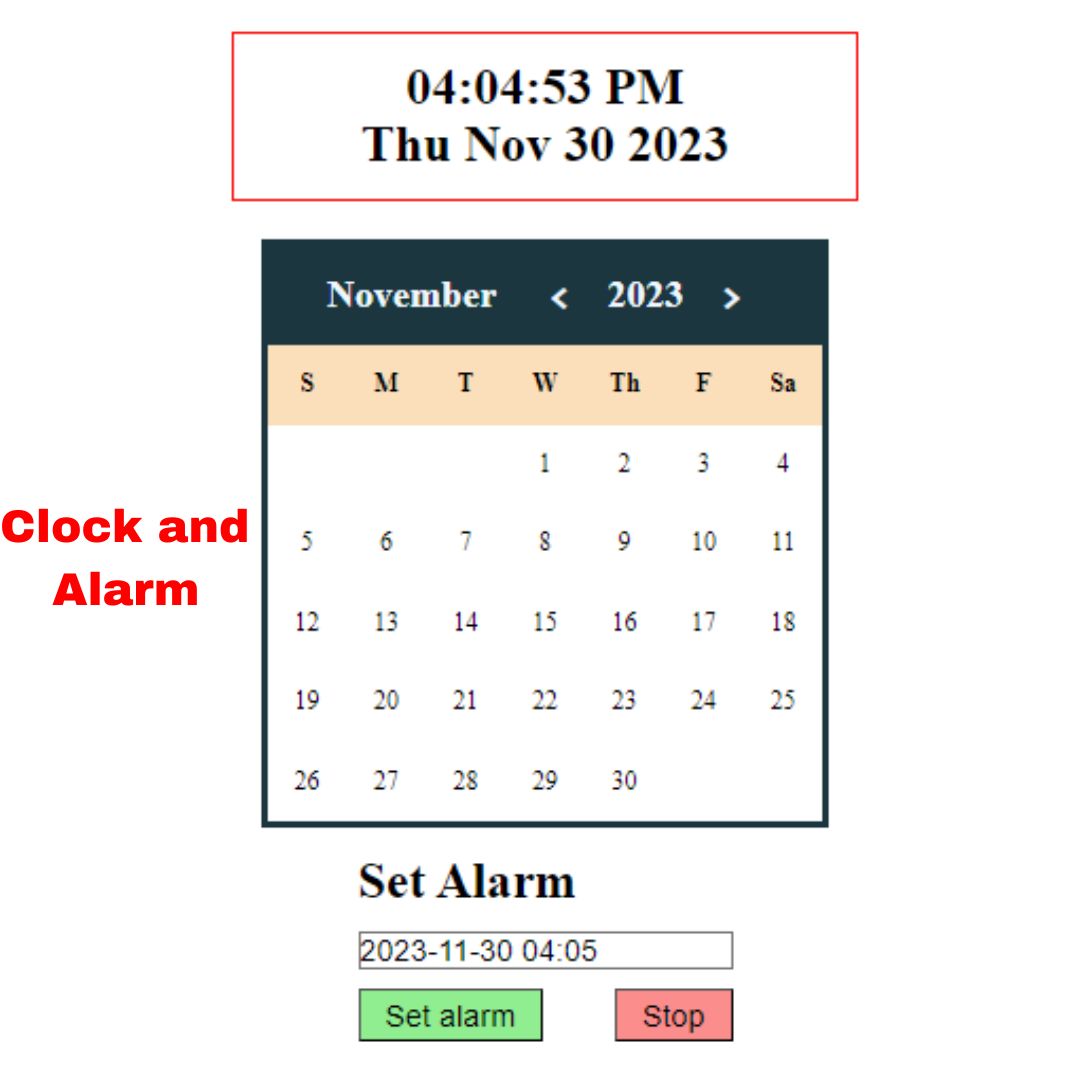
Crafting Custom Alarm and Clock Interfaces using HTML, CSS, and JavaScript
November 30, 2023
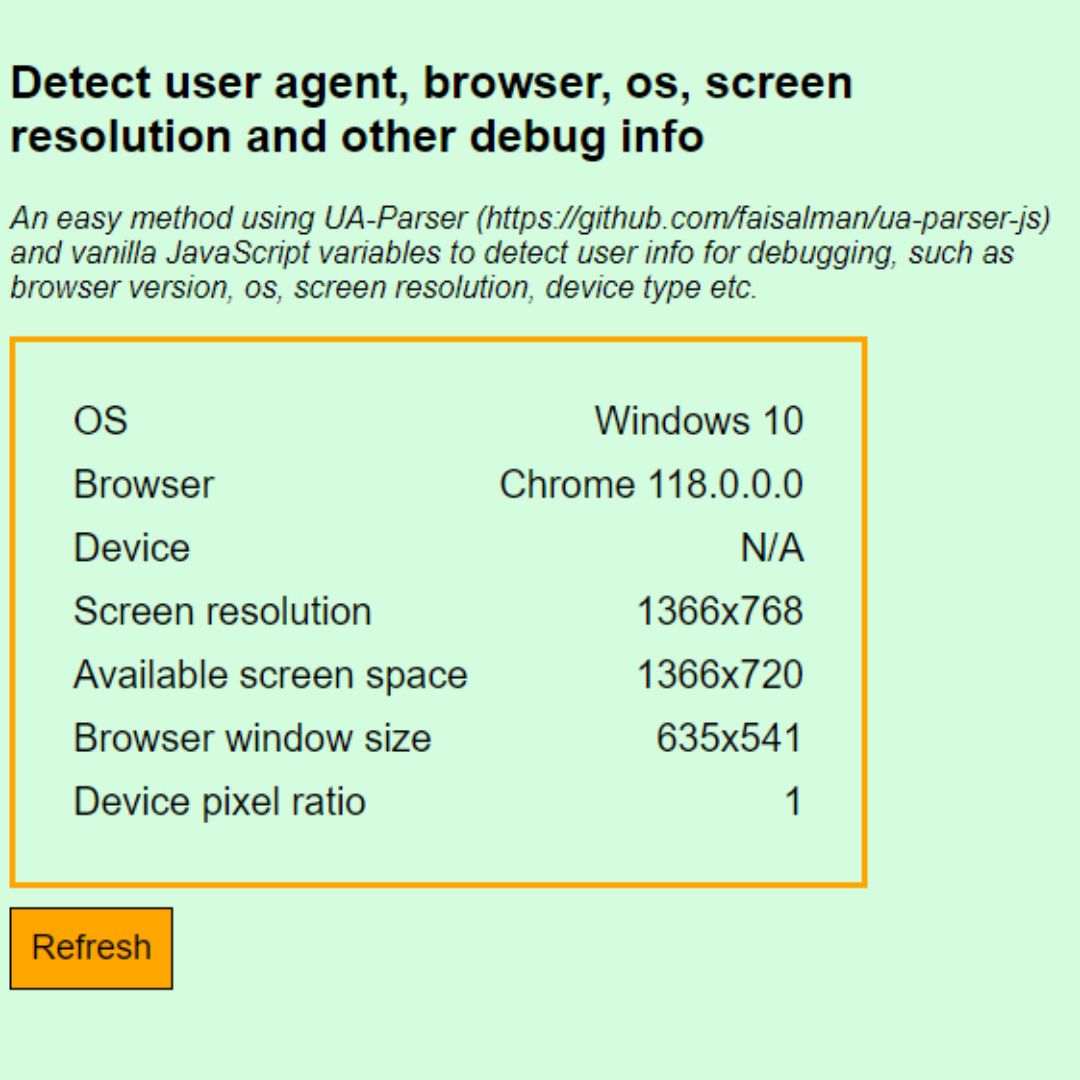
Detect User's Browser, Screen Resolution, OS, and More with JavaScript using UAParser.js Library
October 30, 2023
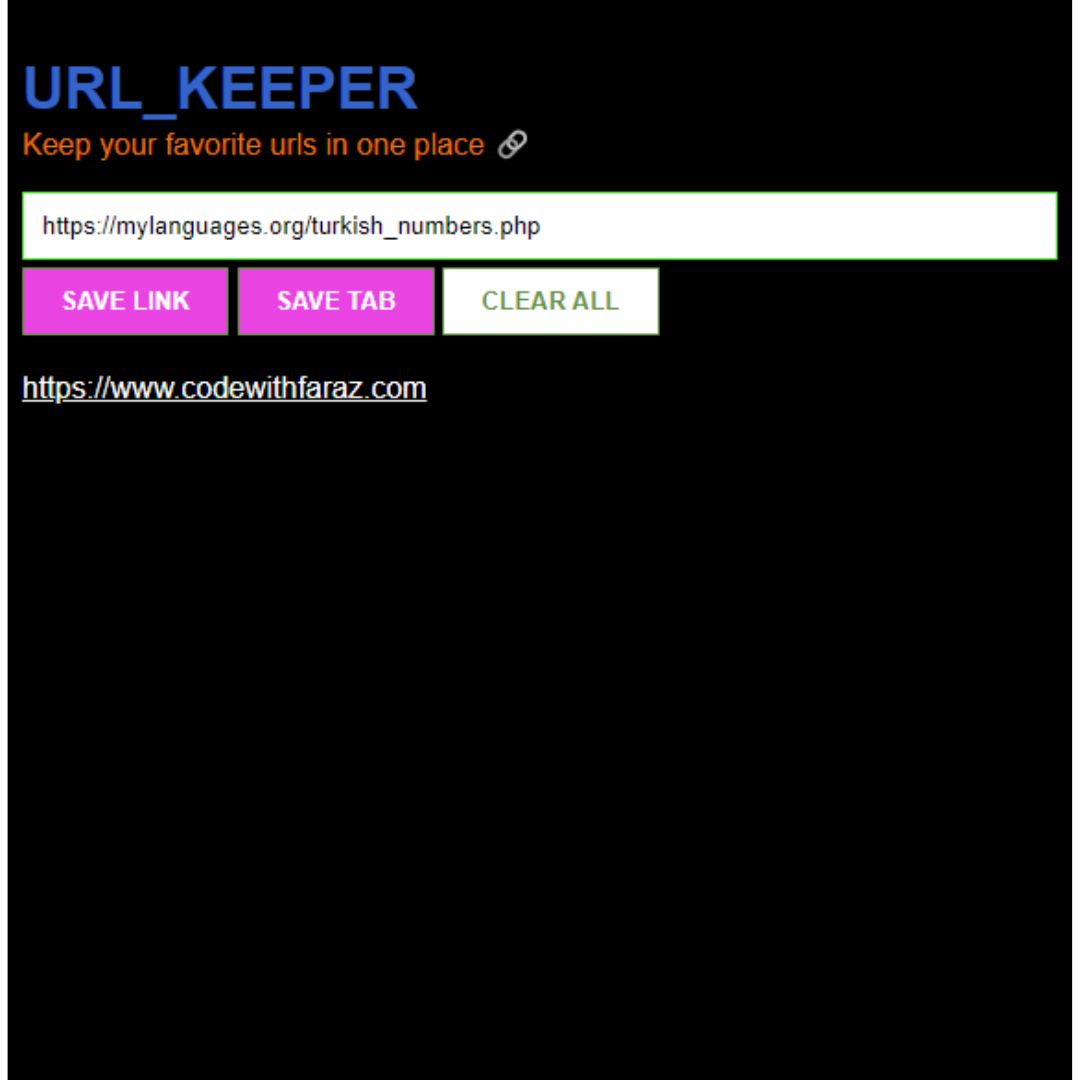
URL Keeper with HTML, CSS, and JavaScript (Source Code)
October 26, 2023
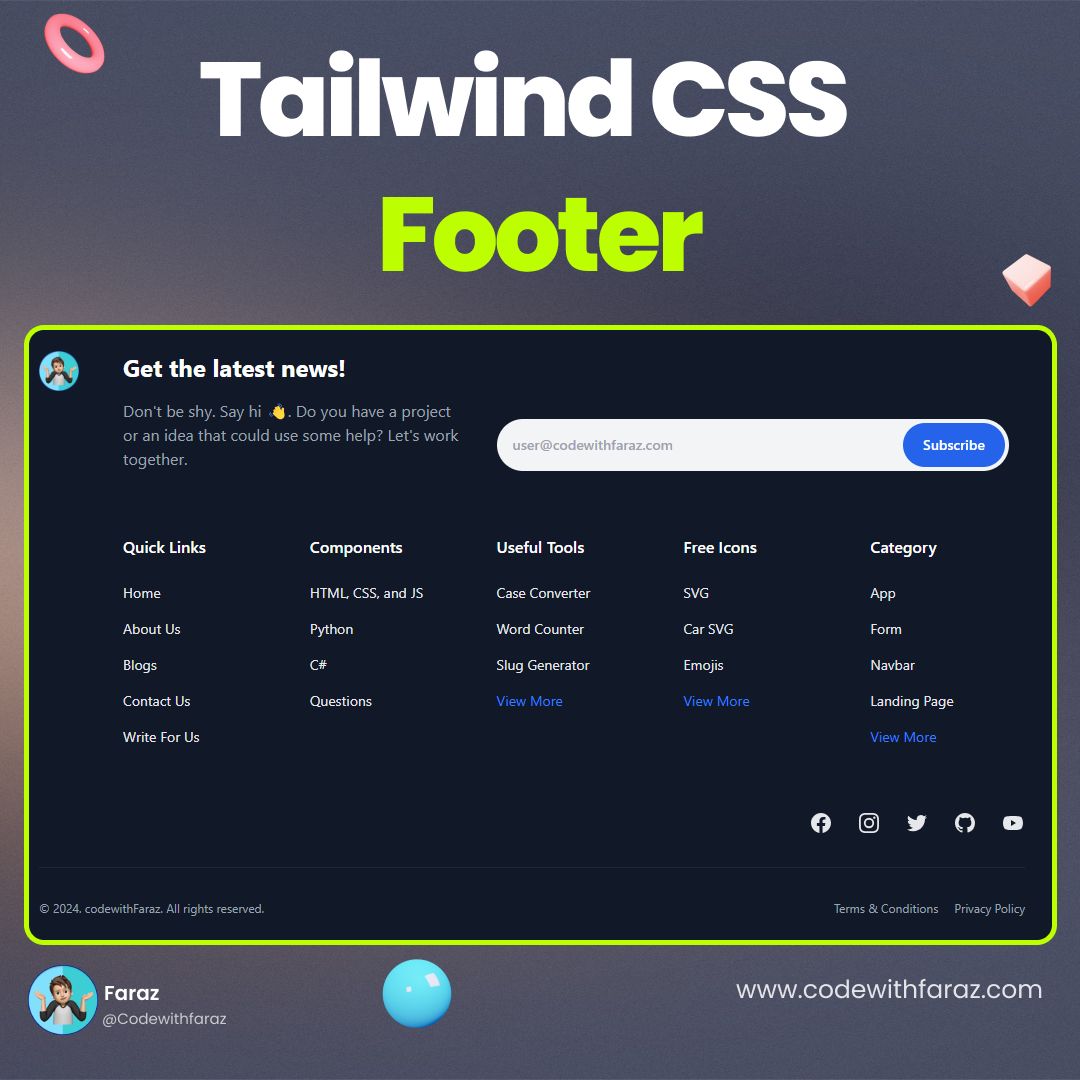
Creating a Responsive Footer with Tailwind CSS (Source Code)
Learn how to design a modern footer for your website using Tailwind CSS with our detailed tutorial. Perfect for beginners in web development.
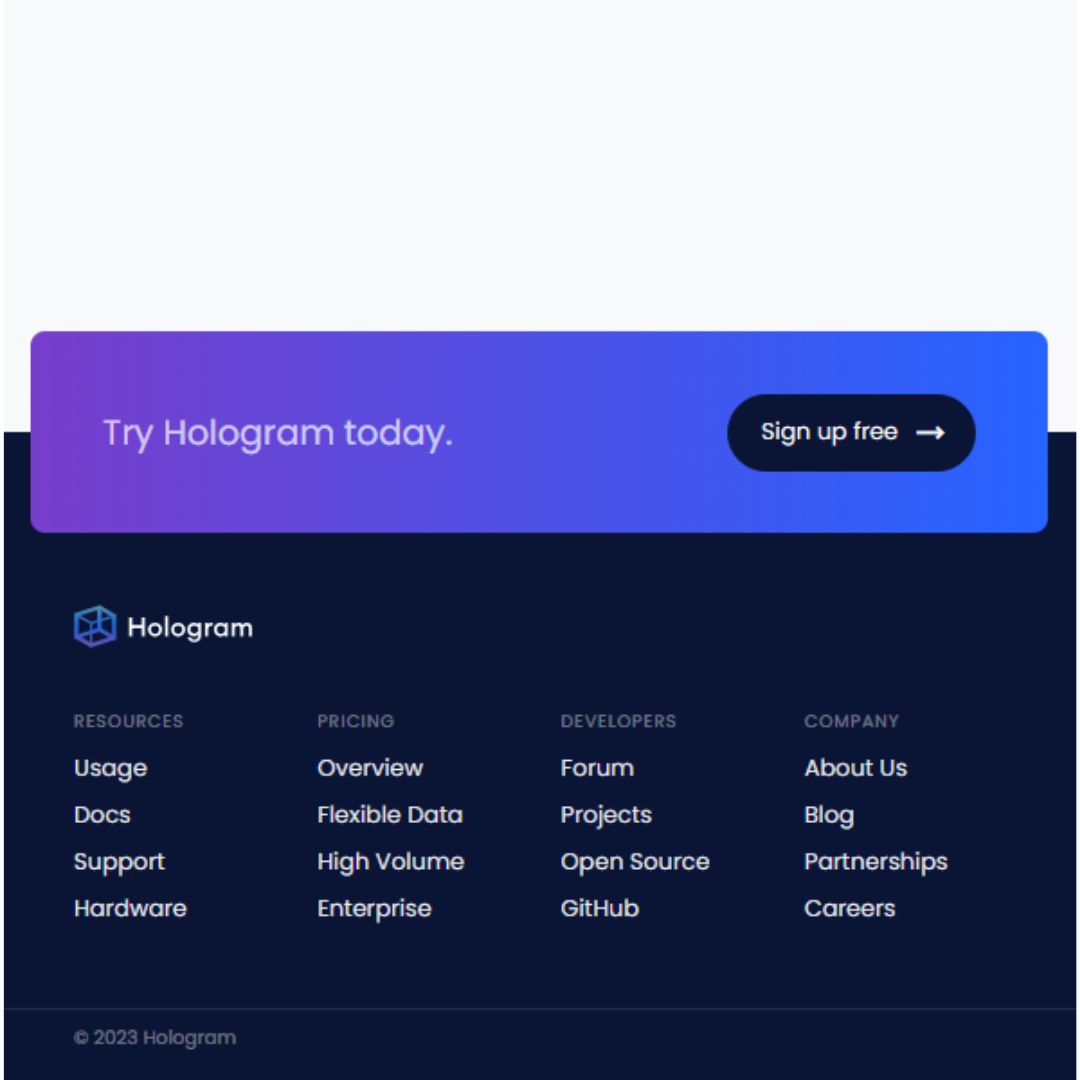
Crafting a Responsive HTML and CSS Footer (Source Code)
November 11, 2023
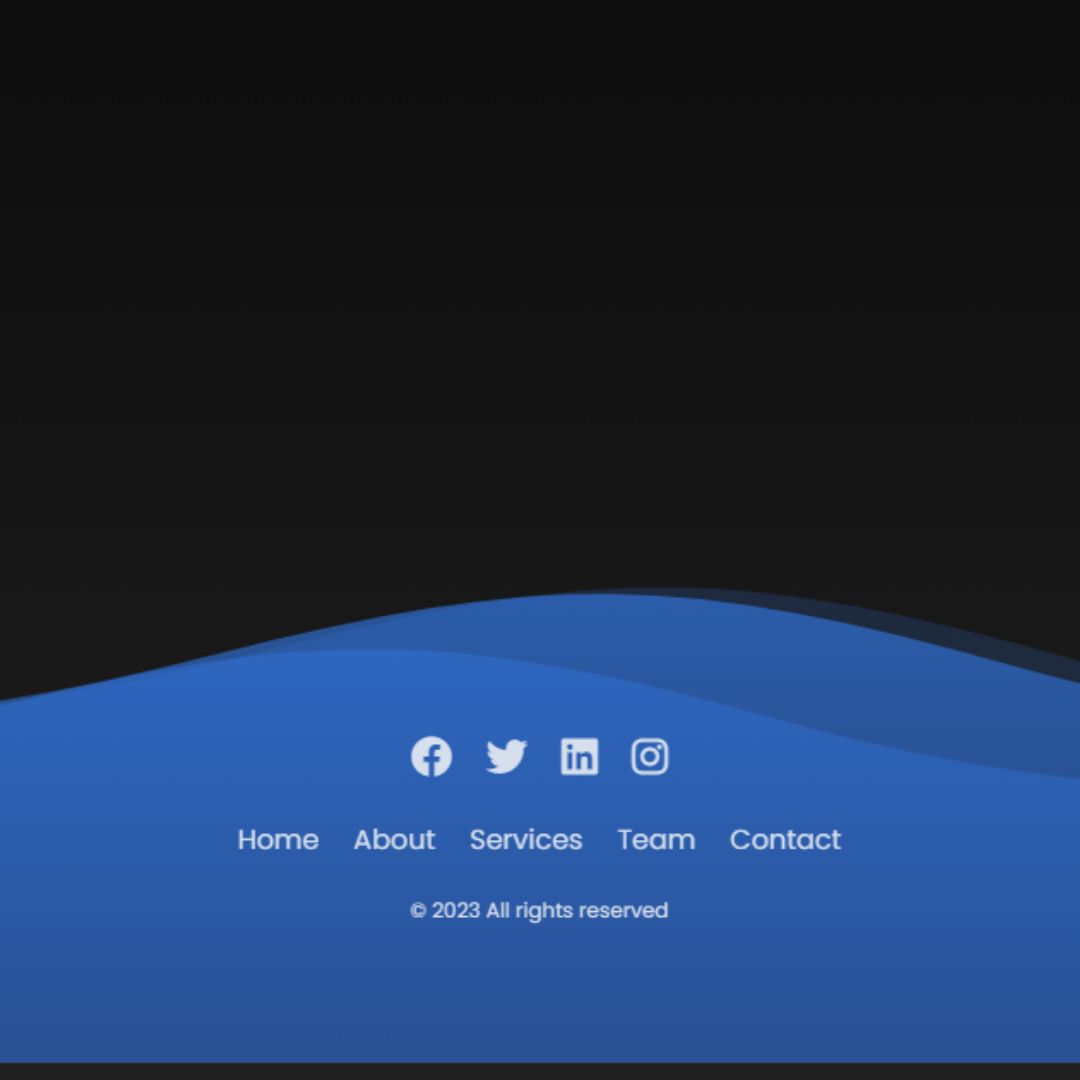
Create an Animated Footer with HTML and CSS (Source Code)
October 17, 2023
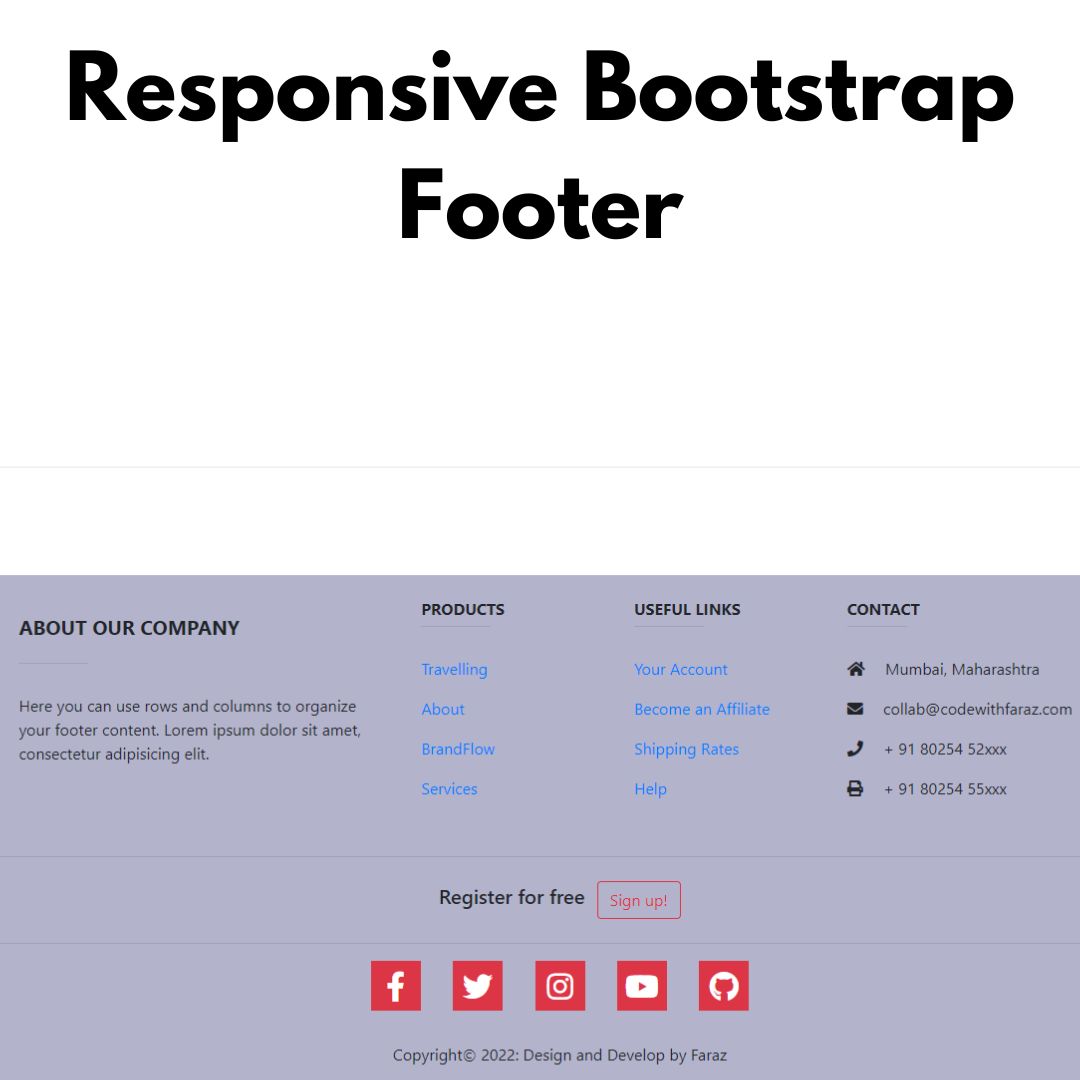
Bootstrap Footer Template for Every Website Style
March 08, 2023
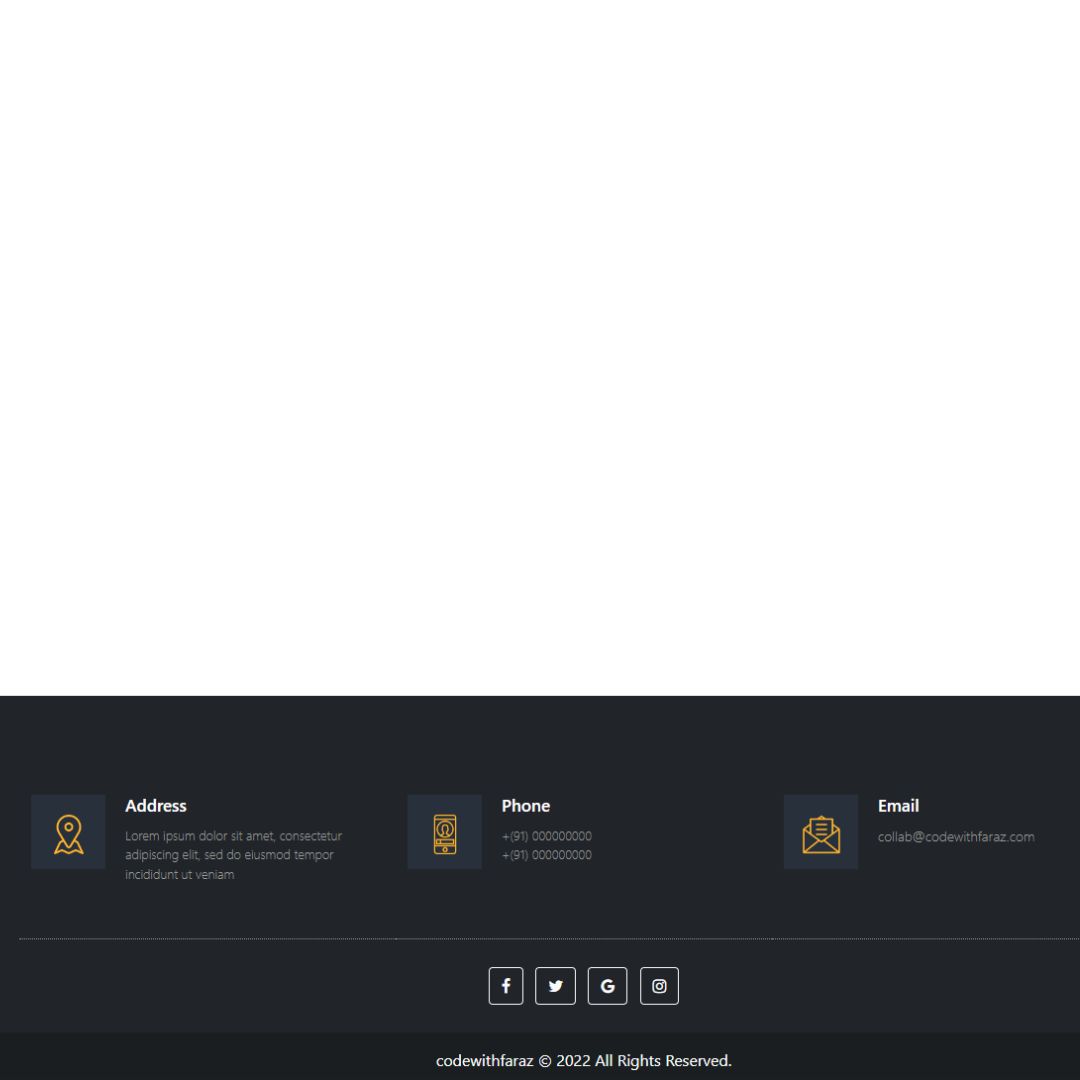
How to Create a Responsive Footer for Your Website with Bootstrap 5
August 19, 2022
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
Assignment (=)
The assignment ( = ) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
A valid assignment target, including an identifier or a property accessor . It can also be a destructuring assignment pattern .
An expression specifying the value to be assigned to x .
Return value
The value of y .
Thrown in strict mode if assigning to an identifier that is not declared in the scope.
Thrown in strict mode if assigning to a property that is not modifiable .
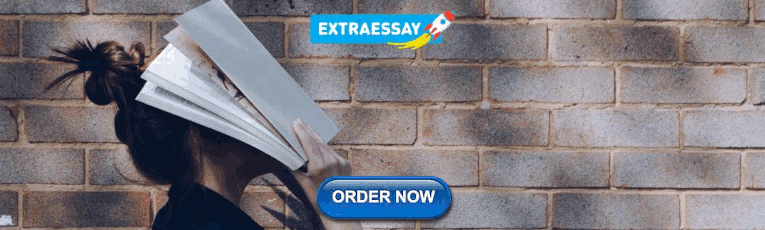
Description
The assignment operator is completely different from the equals ( = ) sign used as syntactic separators in other locations, which include:
- Initializers of var , let , and const declarations
- Default values of destructuring
- Default parameters
- Initializers of class fields
All these places accept an assignment expression on the right-hand side of the = , so if you have multiple equals signs chained together:
This is equivalent to:
Which means y must be a pre-existing variable, and x is a newly declared const variable. y is assigned the value 5 , and x is initialized with the value of the y = 5 expression, which is also 5 . If y is not a pre-existing variable, a global variable y is implicitly created in non-strict mode , or a ReferenceError is thrown in strict mode. To declare two variables within the same declaration, use:
Simple assignment and chaining
Value of assignment expressions.
The assignment expression itself evaluates to the value of the right-hand side, so you can log the value and assign to a variable at the same time.
Unqualified identifier assignment
The global object sits at the top of the scope chain. When attempting to resolve a name to a value, the scope chain is searched. This means that properties on the global object are conveniently visible from every scope, without having to qualify the names with globalThis. or window. or global. .
Because the global object has a String property ( Object.hasOwn(globalThis, "String") ), you can use the following code:
So the global object will ultimately be searched for unqualified identifiers. You don't have to type globalThis.String ; you can just type the unqualified String . To make this feature more conceptually consistent, assignment to unqualified identifiers will assume you want to create a property with that name on the global object (with globalThis. omitted), if there is no variable of the same name declared in the scope chain.
In strict mode , assignment to an unqualified identifier in strict mode will result in a ReferenceError , to avoid the accidental creation of properties on the global object.
Note that the implication of the above is that, contrary to popular misinformation, JavaScript does not have implicit or undeclared variables. It just conflates the global object with the global scope and allows omitting the global object qualifier during property creation.
Assignment with destructuring
The left-hand side of can also be an assignment pattern. This allows assigning to multiple variables at once.
For more information, see Destructuring assignment .
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators in the JS guide
- Destructuring assignment

JAVASCRIPT ASSIGNMENT OPERATORS
In this tutorial, you will learn about all the different assignment operators in javascript and how to use them in javascript.
Assignment Operators
In javascript, there are 16 different assignment operators that are used to assign value to the variable. It is shorthand of other operators which is recommended to use.
The assignment operators are used to assign value based on the right operand to its left operand.
The left operand must be a variable while the right operand may be a variable, number, boolean, string, expression, object, or combination of any other.
One of the most basic assignment operators is equal = , which is used to directly assign a value.
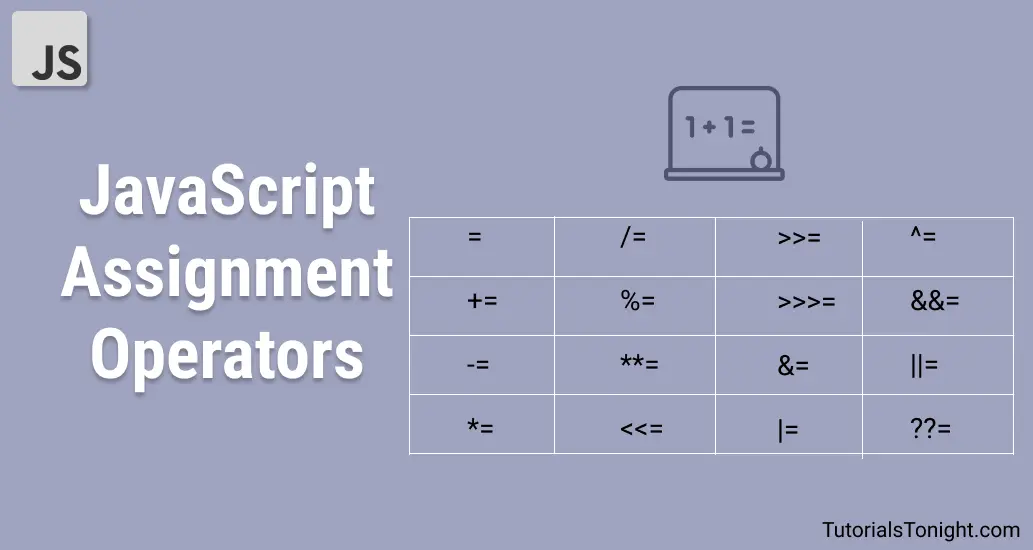
Assignment Operators List
Here is the list of all assignment operators in JavaScript:
In the following table if variable a is not defined then assume it to be 10.
Assignment operator
The assignment operator = is the simplest value assigning operator which assigns a given value to a variable.
The assignment operators support chaining, which means you can assign a single value in multiple variables in a single line.
Addition assignment operator
The addition assignment operator += is used to add the value of the right operand to the value of the left operand and assigns the result to the left operand.
On the basis of the data type of variable, the addition assignment operator may add or concatenate the variables.
Subtraction assignment operator
The subtraction assignment operator -= subtracts the value of the right operand from the value of the left operand and assigns the result to the left operand.
If the value can not be subtracted then it results in a NaN .
Multiplication assignment operator
The multiplication assignment operator *= assigns the result to the left operand after multiplying values of the left and right operand.
Division assignment operator
The division assignment operator /= divides the value of the left operand by the value of the right operand and assigns the result to the left operand.
Remainder assignment operator
The remainder assignment operator %= assigns the remainder to the left operand after dividing the value of the left operand by the value of the right operand.
Exponentiation assignment operator
The exponential assignment operator **= assigns the result of exponentiation to the left operand after exponentiating the value of the left operand by the value of the right operand.
Left shift assignment
The left shift assignment operator <<= assigns the result of the left shift to the left operand after shifting the value of the left operand by the value of the right operand.
Right shift assignment
The right shift assignment operator >>= assigns the result of the right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Unsigned right shift assignment
The unsigned right shift assignment operator >>>= assigns the result of the unsigned right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Bitwise AND assignment
The bitwise AND assignment operator &= assigns the result of bitwise AND to the left operand after ANDing the value of the left operand by the value of the right operand.
Bitwise OR assignment
The bitwise OR assignment operator |= assigns the result of bitwise OR to the left operand after ORing the value of left operand by the value of the right operand.
Bitwise XOR assignment
The bitwise XOR assignment operator ^= assigns the result of bitwise XOR to the left operand after XORing the value of the left operand by the value of the right operand.
Logical AND assignment
The logical AND assignment operator &&= assigns value to left operand only when it is truthy .
Note : A truthy value is a value that is considered true when encountered in a boolean context.
Logical OR assignment
The logical OR assignment operator ||= assigns value to left operand only when it is falsy .
Note : A falsy value is a value that is considered false when encountered in a boolean context.
Logical nullish assignment
The logical nullish assignment operator ??= assigns value to left operand only when it is nullish ( null or undefined ).
.png)
Discover more from daily.dev
Personalized news feed, dev communities and search, much better than what’s out there. Maybe ;)
Introduction to Game Development with Javascript
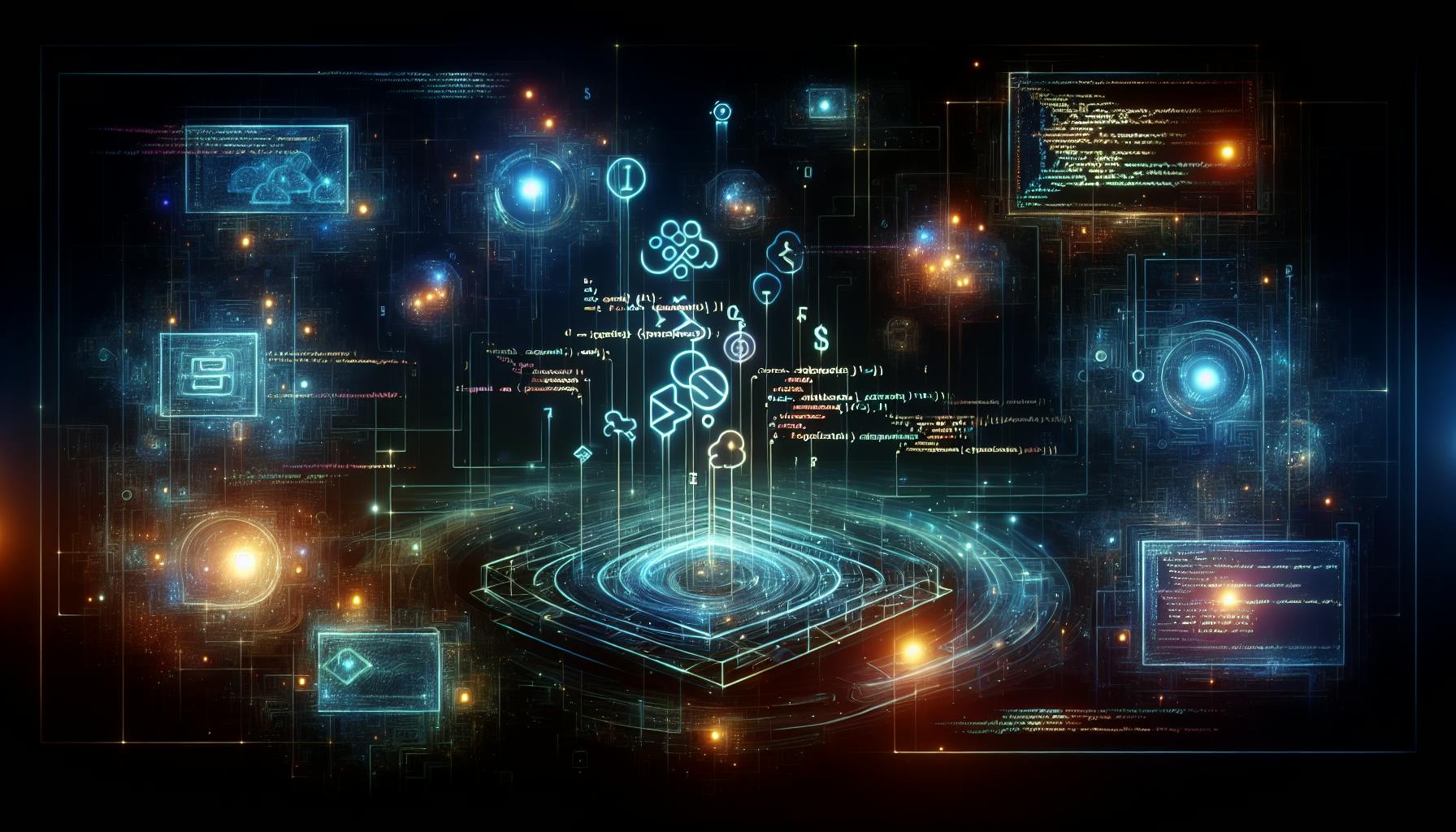
Learn how to create web and mobile games with JavaScript. Explore development tools, core technologies, optimization techniques, deployment options, and resources to enhance your game development skills.
Quick Start Guide to Game Development with JavaScript
- JavaScript is a versatile language perfect for creating web and mobile games.
- Development Tools: You'll need an editor like Visual Studio Code , a browser for testing, and Node.js.
- Libraries and Frameworks: Phaser, Pixi.js, and Three.js are great for building games.
- Core Technologies: Games are built using HTML, CSS, and JavaScript, with Canvas and WebGL for graphics.
- Building a Game: Plan your game, develop its components, and implement game loops and UI.
- Optimization: Ensure smooth gameplay and responsive controls. Use tools to minimize code and load assets efficiently.
- Deployment: You can monetize your game through ads, in-game purchases, or subscriptions.
- Learning Resources: Books, online courses, and communities like HTML5 Game Devs Forum can help you improve.
- Next Steps: Keep creating games to refine your skills. Experiment with different genres and technologies.
Creating games with JavaScript is accessible and fun, offering a wide range of tools and libraries to get started. Whether you're interested in 2D or 3D game development , this guide provides a foundation for your journey.
Setting Up a Development Environment
To make games with JavaScript, you'll need:
- A program like Visual Studio Code to write your code
- A web browser like Chrome or Firefox to see your game
- Node.js to help with some technical stuff
- A game-making tool like Phaser 3, Pixi.js, or Three.js
It's also good to know some basics about:
- How to program with JavaScript
- HTML and CSS for showing your game
- How to use Git to keep track of your work
With these basics, you're ready to start making fun games that work in web browsers!
Building Games with JavaScript
Making games with JavaScript is becoming a go-to for many because it's easy to start and you can make games that run in web browsers. Here's a simple guide to get you going.
Core Web Technologies
When making games with JavaScript, you use some basic web tech:
And you'll also use some special tools JavaScript offers for games:
- Canvas for drawing 2D stuff
- WebGL for making 3D graphics
- Web Audio for sound effects and music
- Tools for handling game controls and more
Game Engines and Frameworks
There are tools that make game making easier:
PixiJS - Good for 2D games, helps with pictures and animations BabylonJS - For 3D games, includes things like physics and sound Phaser - A tool for 2D games with lots of features melonJS - Simple to use for 2D games Three.js - Makes 3D games look amazing
Pick one based on what you need for your game and how much you know.
Developing Your First Game
Before you start making your game, plan it out:
- Game Concept - A simple idea you can make
- Story and Rules - How to win, score points, and what players do
- Technical Specs - What devices it will work on and what you need to make it
- Development Roadmap - A plan with goals and tasks
Then, make the game piece by piece:
- Initialization - Set up the game with objects, sounds, and everything it needs
- Update Loop - Make the game react to what the player does
- Render Loop - Draw everything on the screen
- Interactions - Make things like enemy movement, collision detection, and point & shoot game mechanics work
- UI Screens - Create menus and game info displays
Stick with it, and you'll end up with a game you can play in your web browser!
Optimizing and Deploying Your Game
Polishing for users.
Here are some ways to make your game better for people who play it:
Make things move smoothly: Adding smooth movements makes your game feel more complete. Use JavaScript's requestAnimationFrame() for fluid animations. Adding fade effects to menus can make changes feel smoother.
Make menus and UI easy to use: Your game's menus should be simple to get around, with clear directions on what to do next. Let players move between options easily. Don't forget to include instructions and helpful hints.
Make the game harder gradually: Start easy and slowly make the game harder. Use tutorials to teach the basics, then let players try out what they've learned. Test your game with real users to make sure it's not too easy or too hard.
Make controls quick to respond: Game controls should work right away. Get rid of any lag between when a player does something and when the game responds. Include support for gamepads and touch screens, not just keyboards and mice.
Keep the look consistent: Use the same style for your game's visuals. Adding custom cursors and icons can make your game look more polished.
Optimization and Testing
To make your game run better:
Load everything before starting: Load all images, sounds, and data before the game starts to avoid delays. Show a loading bar while everything is getting ready.
Make your code smaller: Use tools like UglifyJS to make your code take up less space by removing extra spaces and comments.
Draw things together: Drawing lots of things at once can slow the game down. Use sprite sheets and WebGL to draw multiple items at the same time.
Use CSS for animations: For animated menus and UI, CSS can be faster than using Canvas.
Before you launch, test everything:
- Check all parts of the game on different browsers and devices yourself.
- Fix any problems, then check everything again.
- Have other people test the game and tell you what they think.
Deployment and Monetization
You can put your JavaScript games on websites or use tools like Cordova to make them into mobile apps.
Ways to make money with your game:
- Selling things in the game
- Showing ads
- Charging for the game
- Selling related merchandise
To get the word out, share your game on social media, forums, and through emails. Letting players create their own content and mods can help build a community around your game.
sbb-itb-bfaad5b
Continuing your learning journey, helpful resources.
Here are some great places to learn more about making games with JavaScript:
- Learn Javascript for Game Development by Ivan Kuckir - This book is a good starting point that teaches you JavaScript through game projects.
- HTML5 Game Development by Example by Makzan - Focuses on creating different types of HTML5 games.
- Learn Phaser by Pablo Farias Navarro - A detailed guide on using the Phaser game engine.
- Online Courses:
- Udemy - Offers a variety of game development courses focusing on JavaScript and tools like Phaser.
- Frontend Masters - Provides advanced lessons on topics like WebGL and Web Audio.
- Communities:
- HTML5 Game Devs Forum - A place to meet other developers working on browser games.
- /r/gamedev subreddit - A general game development community with some JavaScript discussions.
- HTML5 Game Development slack channel - A spot to chat with developers who are into HTML5 games.
- Newsletters: HTML5 Weekly, Gamedev.js Weekly - These newsletters share news and links about HTML5 and JavaScript game development.
Staying updated will help you create even better games. Follow game developers on Twitter, read blogs, and try out new APIs and libraries.
Next Game Projects
The best way to get better is by making more games. Here are some ideas for your next projects:
- Clone a simple classic game: Try making a version of Breakout, Snake, or Space Invaders. Focus on the basic game play.
- Add power ups and abilities: Work on an action game that includes special moves, temporary power boosts, and weapon upgrades.
- Build multiplayer features: Create games that let two or more people play together online.
- Explore new inputs: Try using device sensors, voice commands, or computer vision for new ways to control games.
- Procedural generation: Use code to automatically create random levels so your game stays interesting.
- Make mobile adaptations: Make sure your game works well on phones by supporting touch events and optimizing for mobile performance.
The more games you make, the more you'll learn. Break big ideas into smaller, doable tasks. Try new things and keep improving your skills. Showing off a collection of games you've made is a great way to demonstrate what you can do.
Most importantly, keep enjoying the process! Making games is fun, so choose projects that excite you.
Making games with JavaScript is really cool because you can do a lot with it. Here's what you should remember:
JavaScript is Versatile for Games
- JavaScript is great for making things happen on websites, which makes it perfect for games.
- There are lots of tools and helpers, like Phaser or Three.js, that make making games easier.
- JavaScript games can be played on both computers and phones.
Essential Skills to Build
- Start by getting good at JavaScript basics—things like making variables and functions.
- Know how the web works with HTML, CSS, and how to draw with Canvas and WebGL.
- Get to know a game-making tool really well.
- Work on game stuff like making characters move, figuring out when things bump into each other, and setting up game menus.
Room for Innovation
- Adding ways to play with friends online can make your games stand out.
- Exploring new ways to control games, like using phone sensors, can be really fun.
- JavaScript lets you try new game ideas quickly.
Helpful Resources for Growth
- There are lots of places to learn from, like forums, books, and online courses.
- Begin with easy projects, like making your own version of classic games, then try bigger things.
- Looking into automatic level creation and making your game work well on phones are good next steps.
- Keep up with new tools, game-making tips, and what's working well for others.
The world of making games with JavaScript is always growing. Whether you're just playing around or thinking about a career, now's a great time to start learning and making your own games. With a love for figuring things out and making cool stuff, you can create almost anything!
Related Questions
Can i use javascript for game development.
Yes, you can definitely use JavaScript to make both web-based and mobile games. It works well with other web tech, making it a good choice for browser games or mobile apps. Plus, it's easier for beginners to get started with JavaScript than with more complex languages.
Is JavaScript or C++ better for game development?
C++ is used for big, fancy 3D games because it's really powerful. But JavaScript is great for simpler 2D and mobile games. It's easier to play around with ideas in JavaScript, but for big 3D games, C++ might be better.
Can you make a 3D game with JavaScript?
Yes, you can make 3D games with JavaScript using engines like Babylon.js. These tools give you everything you need, like lights, shapes, and animations, so you don't have to build them from scratch.
Is JavaScript better than Python for game development?
If you're making a game for the web, JavaScript is usually the way to go because it works directly in browsers. Python is good for making games that you download and play on your computer. The best choice depends on where your game will be played.
Related posts
- Mozilla JavaScript: A Comprehensive Guide
- Tools JS Newcomers Should Learn
- Modern JavaScript Essentials for Developers
- Type JS Essentials for Developers
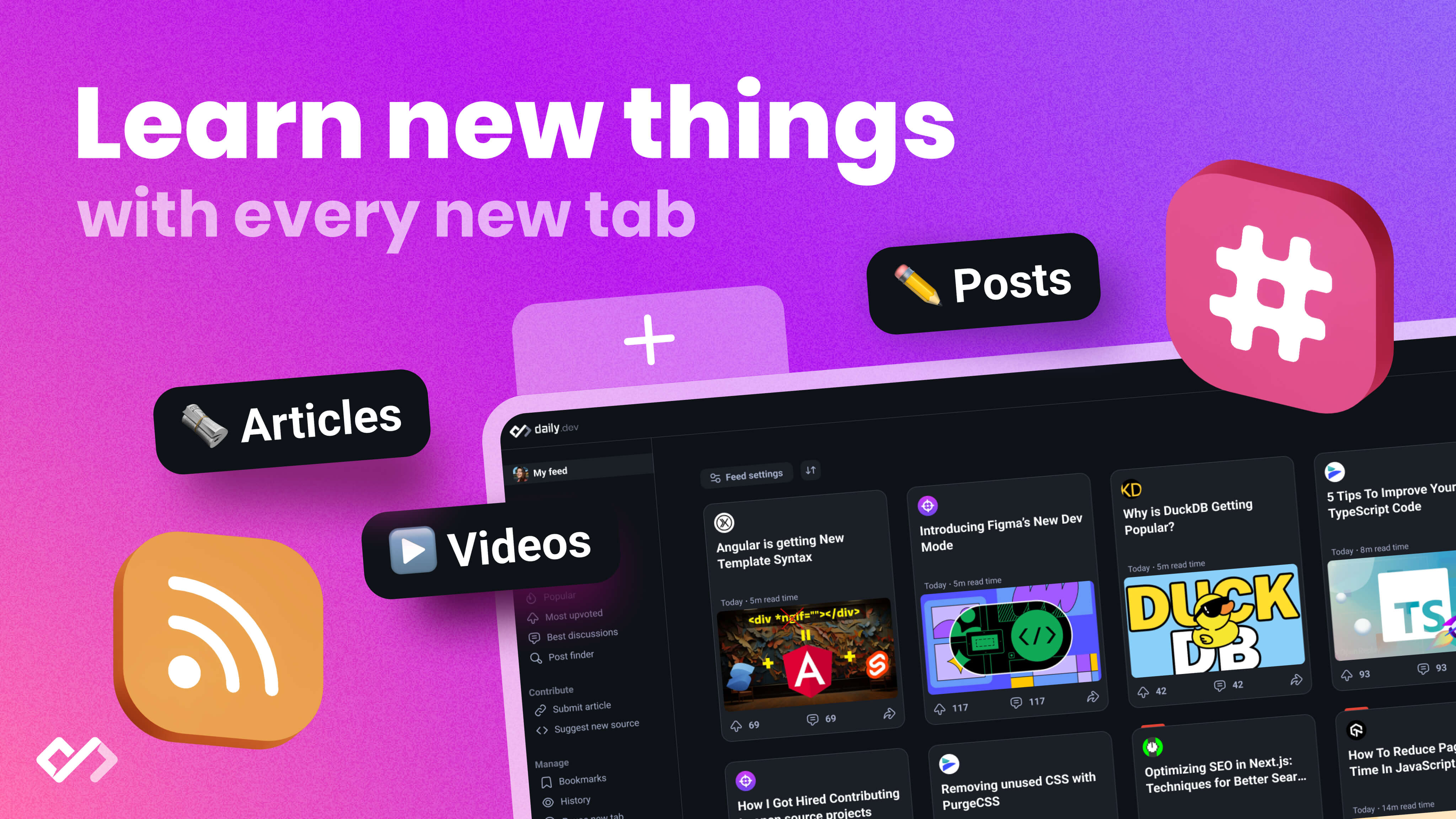
Why not level up your reading with daily.dev?

JavaScript Demo: Expressions - Addition assignment operator
Explore your training options in 10 minutes Get Started
- Graduate Stories
- Partner Spotlights
- Bootcamp Prep
- Bootcamp Admissions
- University Bootcamps
- Coding Tools
- Software Engineering
- Web Development
- Data Science
- Tech Guides
- Tech Resources
- Career Advice
- Online Learning
- Internships
- Apprenticeships
- Tech Salaries
- Associate Degree
- Bachelor's Degree
- Master's Degree
- University Admissions
- Best Schools
- Certifications
- Bootcamp Financing
- Higher Ed Financing
- Scholarships
- Financial Aid
- Best Coding Bootcamps
- Best Online Bootcamps
- Best Web Design Bootcamps
- Best Data Science Bootcamps
- Best Technology Sales Bootcamps
- Best Data Analytics Bootcamps
- Best Cybersecurity Bootcamps
- Best Digital Marketing Bootcamps
- Los Angeles
- San Francisco
- Browse All Locations
- Digital Marketing
- Machine Learning
- See All Subjects
- Bootcamps 101
- Full-Stack Development
- Career Changes
- View all Career Discussions
- Mobile App Development
- Cybersecurity
- Product Management
- UX/UI Design
- What is a Coding Bootcamp?
- Are Coding Bootcamps Worth It?
- How to Choose a Coding Bootcamp
- Best Online Coding Bootcamps and Courses
- Best Free Bootcamps and Coding Training
- Coding Bootcamp vs. Community College
- Coding Bootcamp vs. Self-Learning
- Bootcamps vs. Certifications: Compared
- What Is a Coding Bootcamp Job Guarantee?
- How to Pay for Coding Bootcamp
- Ultimate Guide to Coding Bootcamp Loans
- Best Coding Bootcamp Scholarships and Grants
- Education Stipends for Coding Bootcamps
- Get Your Coding Bootcamp Sponsored by Your Employer
- GI Bill and Coding Bootcamps
- Tech Intevriews
- Our Enterprise Solution
- Connect With Us
- Publication
- Reskill America
- Partner With Us
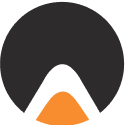
- Resource Center
- Bachelor’s Degree
- Master’s Degree
How to Use the JavaScript += Operator
The JavaScript += operator adds two values together and assigns the result to a variable. This operator is called the addition assignment operator. It is more convenient than the regular variable = X + Y syntax.
A plus sign and an equals sign together? Is that a typo? In JavaScript, a plus and an equals sign side-by-side has its own meaning. It is the JavaScript addition assignment operator.
Find your bootcamp match
In this tutorial, we’re going to talk about what the JavaScript += operator is and how it works. We’ll walk through an example of this operator in action to help you learn how to use it.
What is the JavaScript += Operator?
The JavaScript += operator adds the value on the right of the operator to the variable on the left. The resultant value is then assigned to the variable on the left. This operator is called the addition assignment operator.
Let’s take a look at the syntax for this operator:
We have declared a JavaScript variable called “welcome” whose value is “Hello there, “. Then, we have added “Sophie. to this value. The addition assignment operator adds these two values and then assigns the result to the “welcome” variable.
Our code returns:
This operator has two uses. It is used to add two numbers together. It is also used to add the values of two strings together.
The assignment operator is another way of saying:
The addition assignment operator is a way to make your code easier to read. A += sign is much clearer than writing “variable = x + y” to add two values and assign the result to a variable.
You will often see the addition assignment operator in loops with a counter that tracks how many times the loop has executed.
JavaScript += Operator: Adding Numbers
The addition assignment operator allows you to add two numbers together. Let’s create a program which counts how many times “The Count of Monte Cristo” appears in a list. This list contains the results of a book club’s “Book of the Year” poll.
We’ll start by defining a JavaScript array containing the names of books. We shall also declare a variable to track how many times the book for which we are searching appears:
Next, we’ll write a JavaScript for loop which loops through this list and counts how many times “The Count of Monte Cristo” appears:
This for loop iterates through the “books” list. For each book in the list, our program checks if the title is equal to “The Count of Monte Cristo”. If it is, we use the addition assignment operator to increment the value of “count” by 1. Otherwise, nothing happens.
Once our loop has run, our program prints out how many times the book has appeared in our list. Let’s try out our code:
Our code has counted how many times the book appears in the list.
+= Operator JavaScript: Strings
The JavaScript += operator can merge two strings together. This operator is more convenient than the long-form “variable = x + y” syntax.
For instance, say you have a user’s forename and the surname in two strings. You could use the += operator to merge these values into one string.
Let’s create a program that checks for any cake that begins with “B” in a list. If that cake begins with a “B”, it should be added to a new string. Otherwise, nothing should happen.
We’ll start by defining a list and a string:
The variable “start_with_b” will contain all the cakes that start with “B”. Initially, its value is “| ”.
Next, we’ll create a for loop to iterate through every cake and check if each cake starts with “B”:
We use the JavaScript startsWith() method to check if each cake in our list starts with “B”.
If a cake starts with “B”, our if statement runs. Inside the if statement we declare a variable called “message”. This adds “ | ” to the end of every cake name. We do this so that they will appear separately in our string.
Next, we use the assignment operator to add the contents of “message” to the end of the “start_with_b” variable.

"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Let’s run our code:
Our code returns a list of all cakes that begin with “B”.
The alternative to merge two strings is to use the concatenation operator or the concat() method. To learn more about these methods, check out our JavaScript string concatenation guide .
The addition assignment (+=) operator adds a value to another value and assigns the resultant value to a variable. It is often used to add values to the end of a string or to add numerical values together.
Do you want to learn more about JavaScript? Check out our complete How to Learn JavaScript guide for expert learning tips and guidance on top online books and courses.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication .
What's Next?

Get matched with top bootcamps
Ask a question to our community, take our careers quiz.
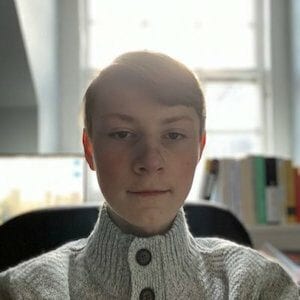
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
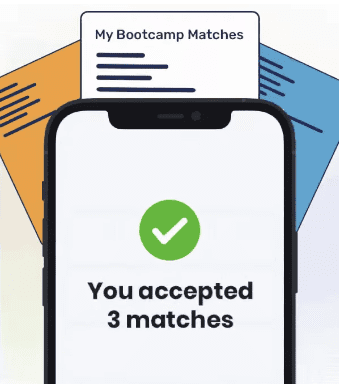
Learn Basic JavaScript by Building a Role Playing Game - Step 83
Sorry, your code does not pass. Don’t give up.
You should use compound assignment to add one to currentWeapon .
function buyWeapon() { if (gold >= 30) { gold -= 30;
The challenge seed code and/or your solution exceeded the maximum length we can port over from the challenge.
You will need to take an additional step here so the code you wrote presents in an easy to read format.
Please copy/paste all the editor code showing in the challenge from where you just linked.
Your browser information:
User Agent is: Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/121.0.0.0 Safari/537.36
Challenge Information:
Hi! On the new line you have to add 1 to currentWeapon using compound assignment, which is +=
- Skip to main content
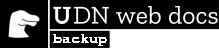
- Addition assignment (+=)
The addition assignment operator ( += ) adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible.
The source for this interactive example is stored in a GitHub repository. If you'd like to contribute to the interactive examples project, please clone https://github.com/mdn/interactive-examples and send us a pull request.
Using addition assignment
Specifications, browser compatibility.
- Assignment operators in the JS guide
- Addition operator
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Using promises
- Iterators and generators
- Meta programming
- JavaScript modules
- Client-side JavaScript frameworks
- Client-side web APIs
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- AggregateError
- ArrayBuffer
- AsyncFunction
- BigInt64Array
- BigUint64Array
- FinalizationRegistry
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- ReferenceError
- SharedArrayBuffer
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Addition (+)
- Assignment (=)
- Bitwise AND (&)
- Bitwise AND assignment (&=)
- Bitwise NOT (~)
- Bitwise OR (|)
- Bitwise OR assignment (|=)
- Bitwise XOR (^)
- Bitwise XOR assignment (^=)
- Comma operator (,)
- Conditional (ternary) operator
- Decrement (--)
- Destructuring assignment
- Division (/)
- Division assignment (/=)
- Equality (==)
- Exponentiation (**)
- Exponentiation assignment (**=)
- Function expression
- Greater than (>)
- Greater than or equal (>=)
- Grouping operator ( )
- Increment (++)
- Inequality (!=)
- Left shift (<<)
- Left shift assignment (<<=)
- Less than (<)
- Less than or equal (<=)
- Logical AND (&&)
- Logical AND assignment (&&=)
- Logical NOT (!)
- Logical OR (||)
- Logical OR assignment (||=)
- Logical nullish assignment (??=)
- Multiplication (*)
- Multiplication assignment (*=)
- Nullish coalescing operator (??)
- Object initializer
- Operator precedence
- Optional chaining (?.)
- Pipeline operator (|>)
- Property accessors
- Remainder (%)
- Remainder assignment (%=)
- Right shift (>>)
- Right shift assignment (>>=)
- Spread syntax (...)
- Strict equality (===)
- Strict inequality (!==)
- Subtraction (-)
- Subtraction assignment (-=)
- Unary negation (-)
- Unary plus (+)
- Unsigned right shift (>>>)
- Unsigned right shift assignment (>>>=)
- async function expression
- class expression
- delete operator
- function* expression
- in operator
- new operator
- void operator
- async function
- for await...of
- function declaration
- import.meta
- try...catch
- Arrow function expressions
- Default parameters
- Method definitions
- Rest parameters
- The arguments object
- Private class fields
- Public class fields
- constructor
- Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration "x" before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the "delete" operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: "x" is not iterable
- TypeError: More arguments needed
- TypeError: Reduce of empty array with no initial value
- TypeError: X.prototype.y called on incompatible type
- TypeError: can't access dead object
- TypeError: can't access property "x" of "y"
- TypeError: can't assign to property "x" on "y": not an object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cannot use "in" operator to search for "x" in "y"
- TypeError: cyclic object value
- TypeError: invalid "instanceof" operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
Design a typing speed test game using JavaScript
- Self-Typing Text Effect using CSS & JavaScript
- Typing Speed Tester using React
- Simple Tic-Tac-Toe Game using JavaScript
- Create a Reflex Game using JavaScript
- Create a Simon Game using HTML CSS & JavaScript
- Rock, Paper and Scissor Game using Javascript
- Design Hit the Mouse Game using HTML, CSS and Vanilla Javascript
- Crack-The-Code Game using JavaScript
- Random String Generator using JavaScript
- Building a Dice Game using JavaScript
- Rock Paper Scissor Game using Tailwind CSS & JavaScript
- How to Design Digital Clock using JavaScript ?
- Design a Digital Clock in Neumorphism Style using JavaScript
- Create a Minesweeper Game using HTML CSS & JavaScript
- Design a Survey Form using HTML CSS and JavaScript
- Create a Bingo Game Using JavaScript
- Create a snake game using HTML, CSS and JavaScript
- How to detect network speed using JavaScript?
- How to test Typing Speed using Python?
A typing test is designed to find how fast one types in a given amount of time. We will be designing a typing game using JavaScript that presents a simple typing challenge and finds the performance of typing by calculating the Characters Per Minute (CPM), Words Per Minute (WPM) and the accuracy of the typed characters. The game shows a series of quotes that have to be typed in a specified time limit, as fast as possible. A higher typing speed would show a higher WPM value. Incorrectly typed characters would be marked accordingly during typing. We will create the HTML layout first, style it using CSS and then write the logic in JavaScript. The HTML Layout: The HTML layout defines the element structure that would be shown on the page. This includes:
- Header Portion: This section shows the statistics of the current typing session. This includes the display of the time left, number of errors, accuracy, WPM and CPM.
- Quote Section: This section shows the current text that has to be typed in the input area.
- Input Area: This section contains the input area where the text has to be typed.
- Restart Button: This is the restart button which would be shown once the time runs out and the game finishes.
- Code:
Note: Each of the portions is filled with dummy data to make styling easier. The HTML code of the above is as follows. The CSS Styling: CSS is used to style the different portions and make it more visually appealing.
- The header portion is displayed using the flex layout.
- Adequate padding and margin are given to each element.
- The text size of each element is such that it is easily readable by the user when playing the game.
- Two additional classes are defined to denote the letters that are typed correctly or incorrectly. These classes would be dynamically added or removed when required.
The result of the HTML layout and CSS styling would look like this:
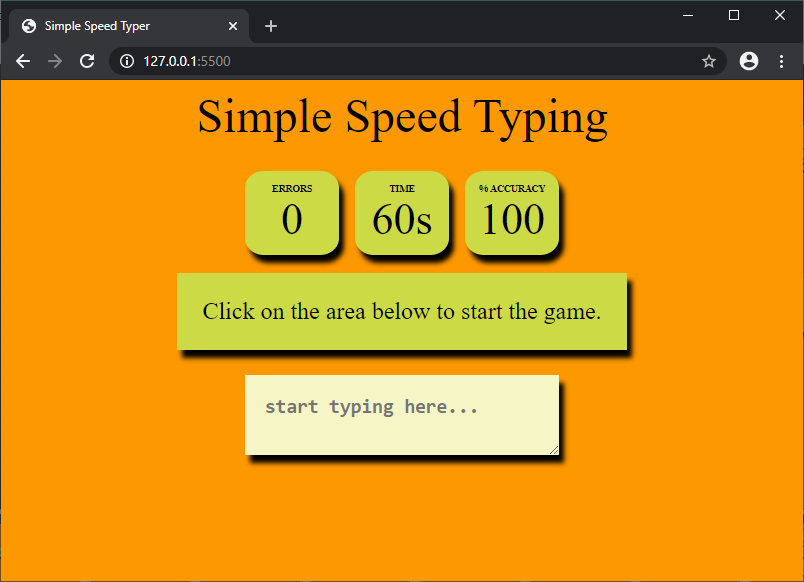
Main Logic of the game: The main logic of the game is defined in a JavaScript file. There are several functions that work together to run the game. Step 1: Selecting all the elements and defining variables The required elements in the HTML layout are first selected using the querySelector() method. They are assigned variable names so that they could be easily accessed and modified. Other variables that would be accessed throughout the program are also defined in the beginning.
Step 2: Preparing the text to be displayed A function updateQuote() is defined which handles the following things:
- Getting the text Quotes have been used as the text that has to be typed to play the game. Each quote is taken one by one from a predefined array. A variable keeps track of the current quote index and increments it whenever a new one is requested.
- Splitting the characters into elements Each of the characters in the text is separated into a series of <span> elements. This makes it possible to individually change the color of each character depending upon if it has been correctly typed by the user. These elements are appended to a variable quote_text.
Step 3: Getting the currently typed text by the user A function processCurrentText() is defined which will be invoked whenever the user types or changes anything in the input box. It is hence used with the oninput event handler of the input box. This function handles the following things:
- Getting the current value of the input box The value property of the input area is used to get the current text typed by the user. This is split into an array of characters to compare with the quote text. This is stored in curr_input_array.
- Coloring the characters of the quote text The characters of the displayed quote are colored ‘red’ or ‘green’ depending on whether it has been typed correctly. This is done by selecting the span elements of the quote we have created earlier and looping through them. The element has then applied the classes created above depending on if it matches the typed text.
- Calculating the errors and accuracy Every time the user makes a mistake during typing, the errors variable is incremented. This is used to calculate the accuracy value by dividing the number of correctly typed characters with the total number of characters typed by the user.
- Moving to next quote When the length of the input text matches the quote text length, the updateQuote() function is called which changes the quote and clears the input area. The number of total errors is also updated to be used for the next quote.
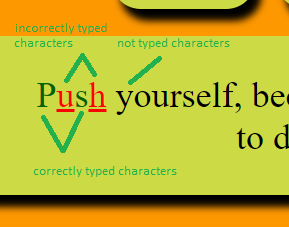
Coloring of the characters based its correctness
Step 4: Starting a new game A function startGame() is defined which will be invoked when the user focuses on the input box. It is hence used with the onfocus event handler of the input box. This function handles the following things:
- Reset all values All the values are reset to their default ones before the starting of a new game. We create a different function named resetValues() which handles this.
- Update the quote text A new quote text is made ready and displayed by calling the updateQuote() function.
- Creating a new timer A timer keeps track of the number of seconds left and displays it to the user. It is created using the setInterval() method which repeatedly calls the updateTimer() function defined below. Before creating a new timer, the previous timer instance is cleared using clearInterval().
Step 5: Updating the timer A function updateTimer() is defined which will be invoked every second to keep track of the time. This function handles the following things:
- Update the time values All the variables that keep track of the time are updated. The timeLeft value is decremented, the timeElapsed value is incremented, and the timer text is updated to the current time left.
- Finishing the game This portion is triggered when the time limit is reached. It calls the finishGame() function defined below which finishes the game.
Step 6: Finishing the game A function finishGame() is defined which will be invoked when the game has to be finished. This function handles the following things:
- Deleting the timer The timer instance created before is removed.
- Displaying the restart game text and button The quoted text displayed to the user is changed to one that indicates that the game is over. The ‘Restart’ button is also displayed by setting the display property to ‘block’.
- The Characters Per Minute (CPM) is calculated by dividing the total number of characters typed with the time elapsed and then multiplying the result with 60. It is rounded off to prevent decimal points.
- The Words Per Minute (WPM) is calculated by dividing the CPM by 5 and then multiplying the result with 60. The 5 denotes the average number of characters per word. It is rounded off to prevent decimal points.
Final Demonstration The game is now ready to be played in any browser.
Source Code: https://github.com/sayantanm19/js-simple-typing-game
Please Login to comment...
Similar reads.
- JavaScript-Misc
- Technical Scripter 2019
- Technical Scripter
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Harinadh93/Match-Game-React-Assignment
Folders and files, repository files navigation.
In this project, let's build a Match Game by applying the concepts we have learned till now.
Refer to the video below:
Design files.
- Extra Small (Size < 576px) and Small (Size >= 576px)
- Medium (Size >= 768px), Large (Size >= 992px) and Extra Large (Size >= 1200px) - Match Game
- Medium (Size >= 768px), Large (Size >= 992px) and Extra Large (Size >= 1200px) - Scorecard
Set Up Instructions
- Download dependencies by running npm install
- Start up the app using npm start
Completion Instructions
The app must have the following functionalities
- Score should be 0 and time should be 60 sec
- The image to be matched should have the src attribute value as the value of the key imageUrl from the first object in imagesList provided
- The Fruits tab should be active and the thumbnails with FRUIT as their category should be displayed
The timer should start running backwards from the 60 sec
When a tab is clicked, then the thumbnails in the corresponding category should be displayed
When a thumbnail is clicked, if that is matched with the image to be matched,
- Score is incremented by one
- The new image to be matched should be generated randomly among the value of the key imageUrl from imagesList provided
When a thumbnail is clicked, if it is not matched with the image to be matched,
- The game should end, and the Scorecard view should be displayed
- The score and time values should be reset to 0 and 60 sec respectively
- The image to be matched should reset to the value of the key imageUrl from the first object in imagesList provided
- The active tab should reset to Fruits , and the thumbnails with FRUIT as their category should be displayed
When the timer reached 0 sec, then the game should end, and the Scorecard view should be displayed
The App is provided with tabsList . It consists of a list of tabItem objects with the following properties in each tabItem object
The App is provided with imagesList . It consists of a list of imageItem objects with the following properties in each imageItem object
Important Note
The following instructions are required for the tests to pass
- The image to be matched in the app should have the alt as match
- The thumbnail images in the app should have the alt as thumbnail
- https://assets.ccbp.in/frontend/react-js/match-game-bg.png
- https://assets.ccbp.in/frontend/react-js/match-game-score-card-lg-bg.png
- https://assets.ccbp.in/frontend/react-js/match-game-score-card-sm-bg.png
- https://assets.ccbp.in/frontend/react-js/match-game-website-logo.png alt should be website logo
- https://assets.ccbp.in/frontend/react-js/match-game-timer-img.png alt should be timer
- https://assets.ccbp.in/frontend/react-js/match-game-play-again-img.png alt should be reset
- https://assets.ccbp.in/frontend/react-js/match-game-trophy.png alt should be trophy
Things to Keep in Mind All components you implement should go in the src/components directory. Don't change the component folder names as those are the files being imported into the tests. Do not remove the pre-filled code Want to quickly review some of the concepts you’ve been learning? Take a look at the Cheat Sheets.
- JavaScript 72.9%
- How it works
- Homework answers
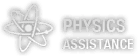
Answer to Question #306573 in Python for Aswad
Difficult Addition
Arjun is trying to add two numbers. Since he has learned addition recently, an addition which requires a carry is difficult for him. Your task is to print Easy if the addition does not involve a carry, Otherwise print Hard.
The first line of input contains two space separated integers A and B.
If the addition does not involve a carry,print Easy, otherwise print Hard.
Explanation
When calculating 229 + 390, we have a carry from the tens digit to the hundreds digit, so the answer is Hard.
Sample Input1
123456789 9876543218
Sample Output1
Sample Input2
Sample Output2
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Leave a comment
Ask your question, related questions.
- 1. question: Cards GameThere are M people from 1 to M . Andy has N cards with her. Starting with person
- 2. question: Difficult AdditionArjun is trying to add two numbers. since he has learned addition recent
- 3. Question 3Rules:1. Play a simple Black Jack game.2. The player will get two cards first.3. If the pl
- 4. Machine Problem 2Create a Python script that will generate random n integers from a given start and
- 5. Machine problem 3In the game Rock Paper Scissors, two players simultaneously choose one of the three
- 6. Machine Problem 1Number Guessing GameIn the number guessing game, the computer will randomly select
- 7. Using Python class Person, write a program that will generate the output below:Hello, my name is Bob
- Programming
- Engineering
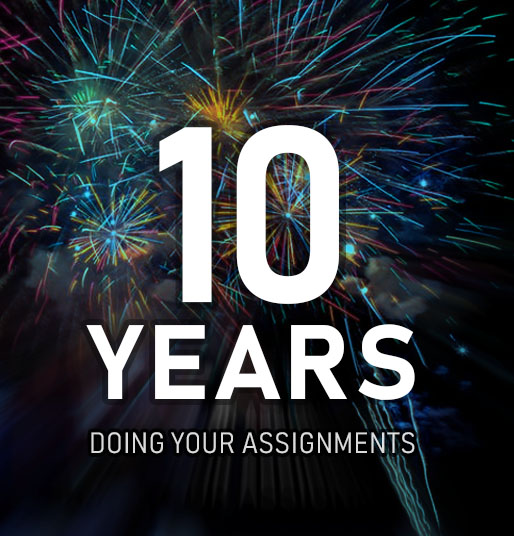
Who Can Help Me with My Assignment
There are three certainties in this world: Death, Taxes and Homework Assignments. No matter where you study, and no matter…
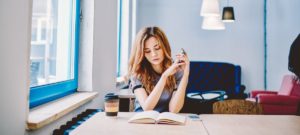
How to Finish Assignments When You Can’t
Crunch time is coming, deadlines need to be met, essays need to be submitted, and tests should be studied for.…
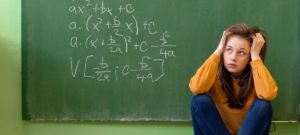
How to Effectively Study for a Math Test
Numbers and figures are an essential part of our world, necessary for almost everything we do every day. As important…
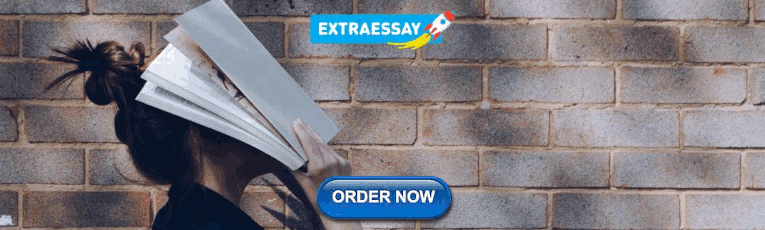
IMAGES
VIDEO
COMMENTS
5. Created a simple math (addition/subtraction) game to practice my JavaScript. Any feedback on the JavaScript appreciated. Can play in: CodePen. Instructions: A math equation will show up and simply press the corresponding number on your keyboard to answer. Speed game so no need to press enter or anything, but also means no deleting/going back ...
Spread the love Related Posts Create an Addition Game Program with Vue 3 and JavaScriptVue 3 is the latest version of the easy to use Vue JavaScript framework that… Create a Counter App with React and JavaScriptReact is an easy to use JavaScript framework that lets us create front end apps.… Create a Digital Clock […]
Create a function that takes two numbers as arguments and returns their sum. Examples addition(3, 2) 5 addition(-3, -6) -9 addition(7, 3) 10 Notes Don't forget to return the result. If you get stuck on a challenge, find help in the Resources tab. If you're really stuck, unlock solutions in the Solutions tab.
We do JavaScript tasks of any level of difficulty - including Boolean objects, pop-ups, terms and conditions, HTML controls, languages, and even games and quizzes for your JavaScript assignments. Assignment Expert is the best option in the market to deal with any JavaScript project within a short time and for less money.
Use the correct assignment operator that will result in x being 15 (same as x = x + y ). Start the Exercise. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Just put a URL to it here and we'll add it, in the order you have them, before the JavaScript in the Pen itself. If the script you link to has the file extension of a preprocessor, we'll attempt to process it before applying.
This playlist consists of more than 100 javascript projects. The difficulty level of these projects varies from easy to complex. Therefore it makes this playlist suitable for all kinds of javascript learners. So whether you are a javascript beginner or an expert, this playlist has something for you. Let us take a look at how this project works.
Code, play, and learn game development in a fun and interactive way. Join us on Telegram. Read Also Creating a Text-Based Drawing App with HTML, CSS, and JavaScript. Table of Contents. Game 1: Connect Four Game. Game 2: Candy Crush. Game 3: Speed Typing Game. Game 4: Archery. Game 5: Flappy Bird.
The addition assignment (+=) operator performs addition (which is either numeric addition or string concatenation) on the two operands and assigns the result to the left operand. ... JavaScript. Learn to run scripts in the browser. Accessibility. Learn to make the web accessible to all. Plus Plus. Overview. A customized MDN experience. AI Help ...
Assignment (=) The assignment ( =) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
javascript has 16 different assignment operators that are used to assign value to the variable. It is shorthand of other operators which is recommended to use. Tutorials . HTML5; ... The addition assignment operator += is used to add the value of the right operand to the value of the left operand and assigns the result to the left operand.
Assignment Operators. Assignment operators are used to assign values to variables in JavaScript. Syntax: data=value. Example: // Lets take some variablesx=10y=20x=y // Here, x is equal to 20y=x // Here, y is equal to 10.
Core Technologies: Games are built using HTML, CSS, and JavaScript, with Canvas and WebGL for graphics. Building a Game: Plan your game, develop its components, and implement game loops and UI. Optimization: Ensure smooth gameplay and responsive controls. Use tools to minimize code and load assets efficiently.
JavaScript Addition assignment operator (+=) adds a value to a variable, The Addition Assignment (+ =) Sums up left and right operand values and then assigns the result to the left operand. The two major operations that can be performed using this operator are the addition of numbers and the concatenation of strings. Syntax: a += b.
let a = 2; let b = 'hello'; console.log((a += 3)); // Addition // Expected output: 5 console.log((b += ' world')); // Concatenation // Expected output: "hello world ...
The JavaScript += operator adds the value on the right of the operator to the variable on the left. The resultant value is then assigned to the variable on the left. This operator is called the addition assignment operator. Let's take a look at the syntax for this operator: console. log (welcome += "Sophie."
Hi! On the new line you have to add 1 to currentWeapon using compound assignment, which is +=
The addition assignment operator ( +=) adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. JavaScript Demo: Expressions - Addition assignment operator. 9.
Answer to Question #296146 in HTML/JavaScript Web Application for chethan. the goal of this code is to get output using with Classes. return inputString[currentLine++]; // Create your Super Class Race and Sub Class Car here class Car {. constructor ( playerName, nitro, speed) {. this. playerName = playerName;
Main Logic of the game: The main logic of the game is defined in a JavaScript file. There are several functions that work together to run the game. Step 1: Selecting all the elements and defining variables. The required elements in the HTML layout are first selected using the querySelector () method.
Functionality to be added. The app must have the following functionalities. Initially, Score should be 0 and time should be 60 sec; The image to be matched should have the src attribute value as the value of the key imageUrl from the first object in imagesList provided; The Fruits tab should be active and the thumbnails with FRUIT as their category should be displayed
javascript. The assignment is to add the following: if player hits 21 or goes over 21 the game is over and the appropriate message is displayed ("Player Wins! or Player Busted") If player stands at under 21, the dealer hits and will continue to hit as long as dealer score is less than player score
Question #306573. Difficult Addition. Arjun is trying to add two numbers. Since he has learned addition recently, an addition which requires a carry is difficult for him. Your task is to print Easy if the addition does not involve a carry, Otherwise print Hard. Input. The first line of input contains two space separated integers A and B. Output.