- Books Get Your Hands Dirty on Clean Architecture Stratospheric
- Contribute Become an Author Writing Guide Author Workflow Author Payment
- Services Book me Advertise
- Categories Spring Boot Java Node Kotlin AWS Software Craft Simplify! Meta Book Reviews
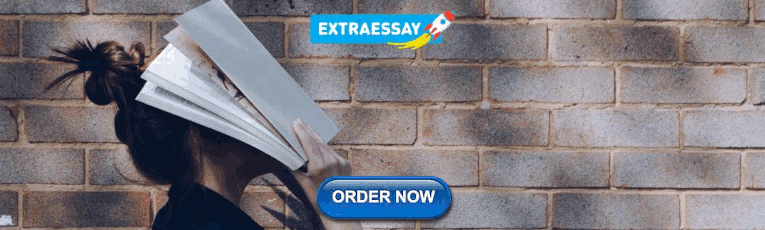
Creating and Analyzing Java Heap Dumps
- March 1, 2021
As Java developers, we are familiar with our applications throwing OutOfMemoryErrors or our server monitoring tools throwing alerts and complaining about high JVM memory utilization.
To investigate memory problems, the JVM Heap Memory is often the first place to look at.
To see this in action, we will first trigger an OutOfMemoryError and then capture a heap dump. We will next analyze this heap dump to identify the potential objects which could be the cause of the memory leak.
Example Code
What is a heap dump.
Whenever we create a Java object by creating an instance of a class, it is always placed in an area known as the heap. Classes of the Java runtime are also created in this heap.
The heap gets created when the JVM starts up. It expands or shrinks during runtime to accommodate the objects created or destroyed in our application.
When the heap becomes full, the garbage collection process is run to collect the objects that are not referenced anymore (i.e. they are not used anymore). More information on memory management can be found in the Oracle docs .
Heap dumps contain a snapshot of all the live objects that are being used by a running Java application on the Java heap. We can obtain detailed information for each object instance, such as the address, type, class name, or size, and whether the instance has references to other objects.
Heap dumps have two formats:
- the classic format, and
- the Portable Heap Dump (PHD) format.
PHD is the default format. The classic format is human-readable since it is in ASCII text, but the PHD format is binary and should be processed by appropriate tools for analysis.
Sample Program to Generate an OutOfMemoryError
To explain the analysis of a heap dump, we will use a simple Java program to generate an OutOfMemoryError :
We keep on allocating the memory by running a for loop until a point is reached, when JVM does not have enough memory to allocate, resulting in an OutOfMemoryError being thrown.
Finding the Root Cause of an OutOfMemoryError
We will now find the cause of this error by doing a heap dump analysis. This is done in two steps:
- Capture the heap dump
- Analyze the heap dump file to locate the suspected reason.
We can capture heap dump in multiple ways. Let us capture the heap dump for our example first with jmap and then by passing a VM argument in the command line.
Generating a Heap Dump on Demand with jmap
jmap is packaged with the JDK and extracts a heap dump to a specified file location.
To generate a heap dump with jmap , we first find the process ID of our running Java program with the jps tool to list down all the running Java processes on our machine:
Next, we run the jmap command to generate the heap dump file:
After running this command the heap dump file with extension hprof is created.
The option live is used to collect only the live objects that still have a reference in the running code. With the live option, a full GC is triggered to sweep away unreachable objects and then dump only the live objects.
Automatically Generating a Heap Dump on OutOfMemoryError s
This option is used to capture a heap dump at the point in time when an OutOfMemoryError occurred. This helps to diagnose the problem because we can see what objects were sitting in memory and what percentage of memory they were occupying right at the time of the OutOfMemoryError .
We will use this option for our example since it will give us more insight into the cause of the crash.
Let us run the program with the VM option HeapDumpOnOutOfMemoryError from the command line or our favorite IDE to generate the heap dump file:
After running our Java program with these VM arguments, we get this output:
As we can see from the output, the heap dump file with the name: hdump.hprof is created when the OutOfMemoryError occurs.
Other Methods of Generating Heap Dumps
Some of the other methods of generating a heap dump are:
jcmd : jcmd is used to send diagnostic command requests to the JVM. It is packaged as part of the JDK. It can be found in the \bin folder of a Java installation.
JVisualVM : Usually, analyzing heap dump takes more memory than the actual heap dump size. This could be problematic if we are trying to analyze a heap dump from a large server on a development machine. JVisualVM provides a live sampling of the Heap memory so it does not eat up the whole memory.
Analyzing the Heap Dump
What we are looking for in a Heap dump is:
- Objects with high memory usage
- Object graph to identify objects of not releasing memory
- Reachable and unreachable objects
Eclipse Memory Analyzer (MAT) is one of the best tools to analyze Java heap dumps. Let us understand the basic concepts of Java heap dump analysis with MAT by analyzing the heap dump file we generated earlier.
We will first start the Memory Analyzer Tool and open the heap dump file. In Eclipse MAT, two types of object sizes are reported:
- Shallow heap size : The shallow heap of an object is its size in the memory
- Retained heap size : Retained heap is the amount of memory that will be freed when an object is garbage collected.
Overview Section in MAT
After opening the heap dump, we will see an overview of the application’s memory usage. The piechart shows the biggest objects by retained size in the overview tab as shown here:
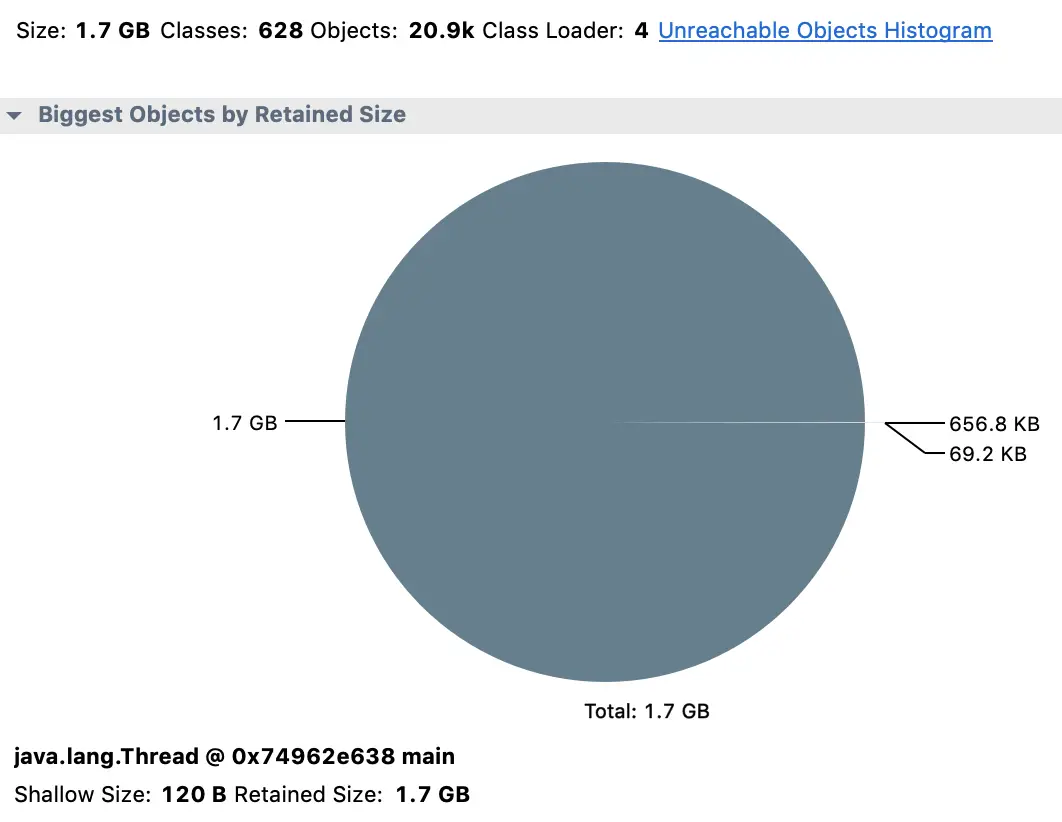
For our application, this information in the overview means if we could dispose of a particular instance of java.lang.Thread we will save 1.7 GB, and almost all of the memory used in this application.
Histogram View
While that might look promising, java.lang.Thread is unlikely to be the real problem here. To get a better insight into what objects currently exist, we will use the Histogram view:
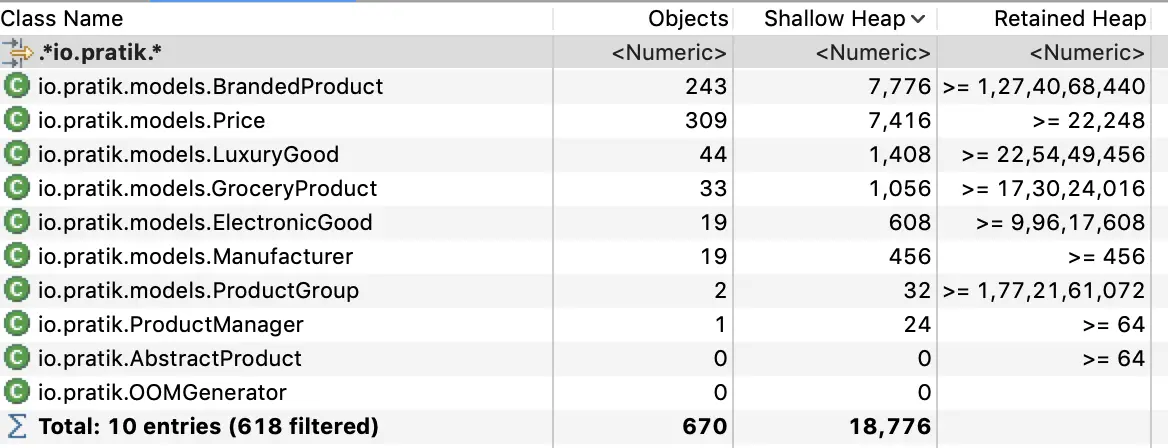
We have filtered the histogram with a regular expression “io.pratik.* " to show only the classes that match the pattern. With this view, we can see the number of live objects: for example, 243 BrandedProduct objects, and 309 Price Objects are alive in the system. We can also see the amount of memory each object is using.
There are two calculations, Shallow Heap and Retained Heap. A shallow heap is the amount of memory consumed by one object. An Object requires 32 (or 64 bits, depending on the architecture) for each reference. Primitives such as integers and longs require 4 or 8 bytes, etc… While this can be interesting, the more useful metric is the Retained Heap.
Retained Heap Size
The retained heap size is computed by adding the size of all the objects in the retained set. A retained set of X is the set of objects which would be removed by the Garbage Collector when X is collected.
The retained heap can be calculated in two different ways, using the quick approximation or the precise retained size:
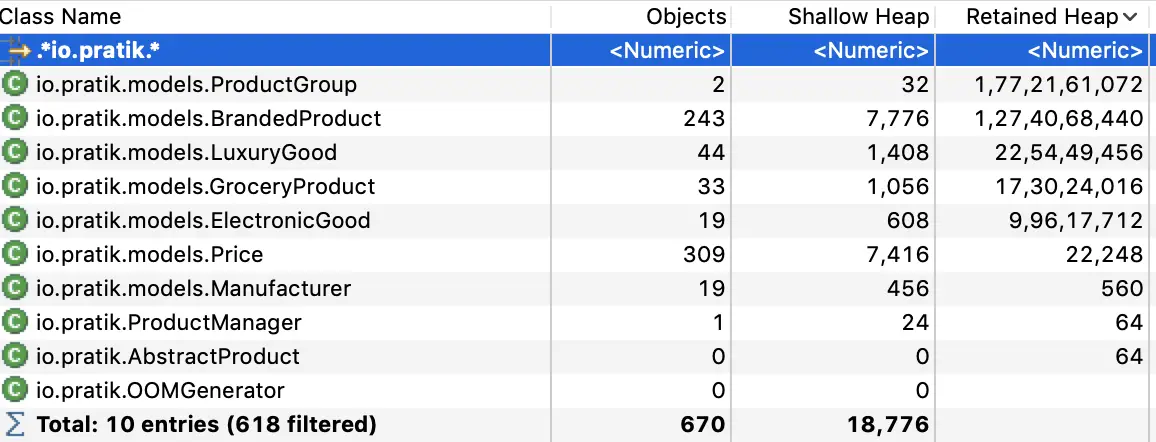
By calculating the Retained Heap we can now see that io.pratik.ProductGroup is holding the majority of the memory, even though it is only 32 bytes (shallow heap size) by itself. By finding a way to free up this object, we can certainly get our memory problem under control.
Dominator Tree
The dominator tree is used to identify the retained heap. It is produced by the complex object graph generated at runtime and helps to identify the largest memory graphs. An Object X is said to dominate an Object Y if every path from the Root to Y must pass through X.
Looking at the dominator tree for our example, we can see which objects are retained in the memory.
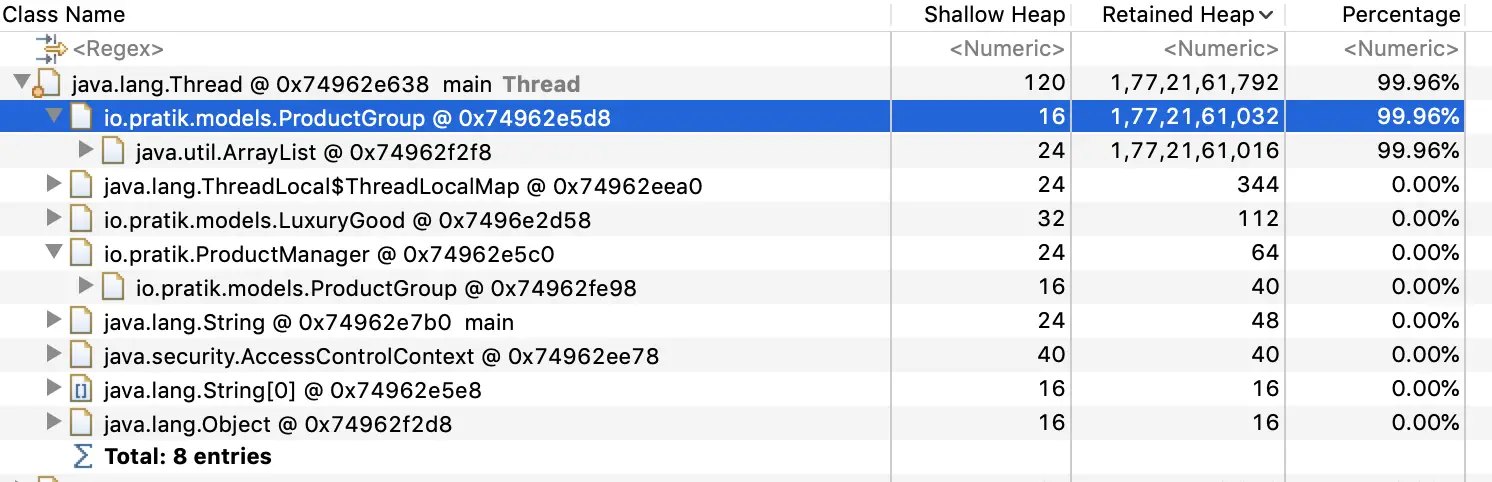
We can see that the ProductGroup object holds the memory instead of the Thread object. We can probably fix the memory problem by releasing objects contained in this object.
Leak Suspects Report
We can also generate a “Leak Suspects Report” to find a suspected big object or set of objects. This report presents the findings on an HTML page and is also saved in a zip file next to the heap dump file.
Due to its smaller size, it is preferable to share the “Leak Suspects Report” report with teams specialized in performing analysis tasks instead of the raw heap dump file.
The report has a pie chart, which gives the size of the suspected objects:
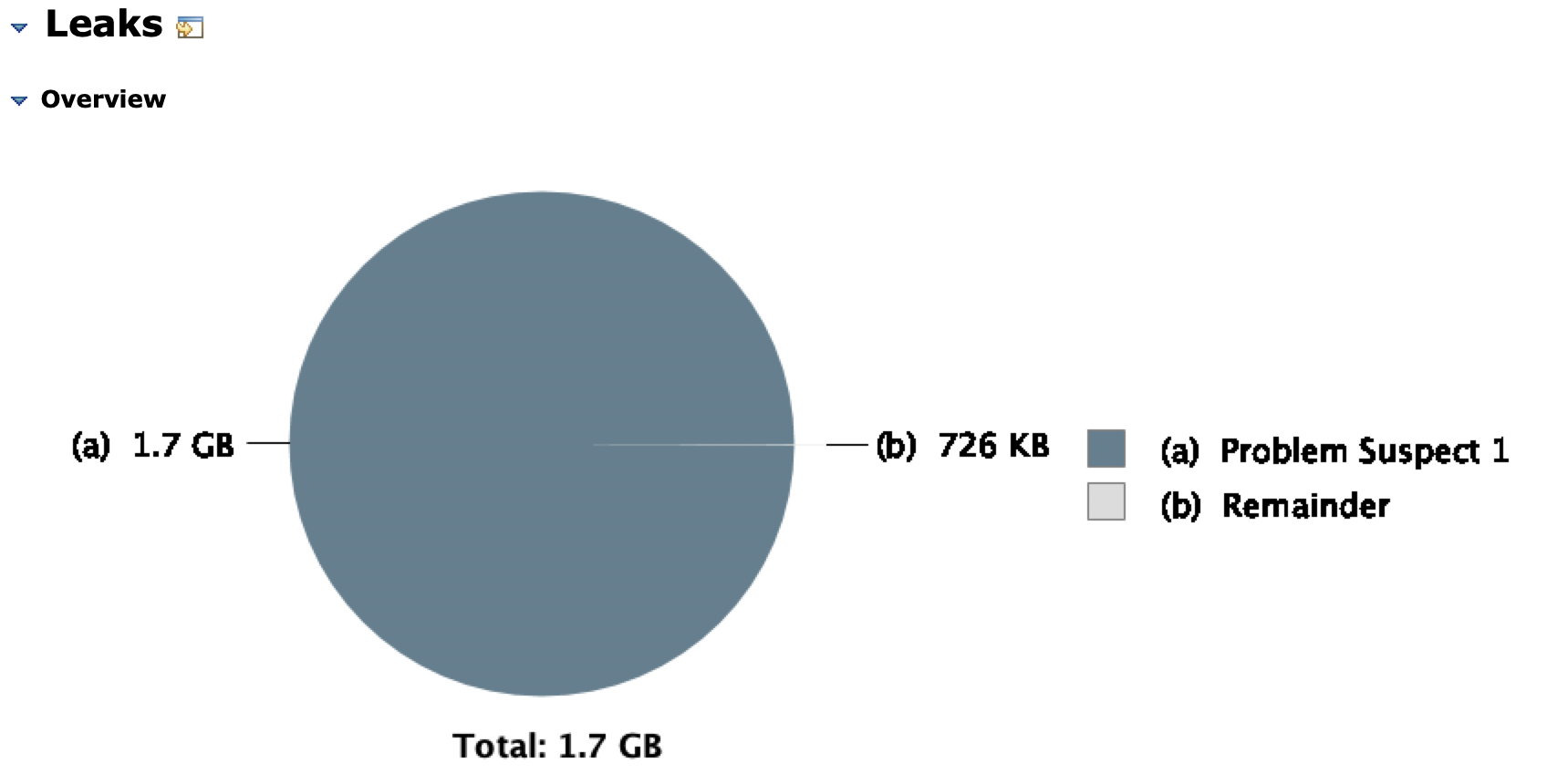
For our example, we have one suspect labeled as “Problem Suspect 1” which is further described with a short description:
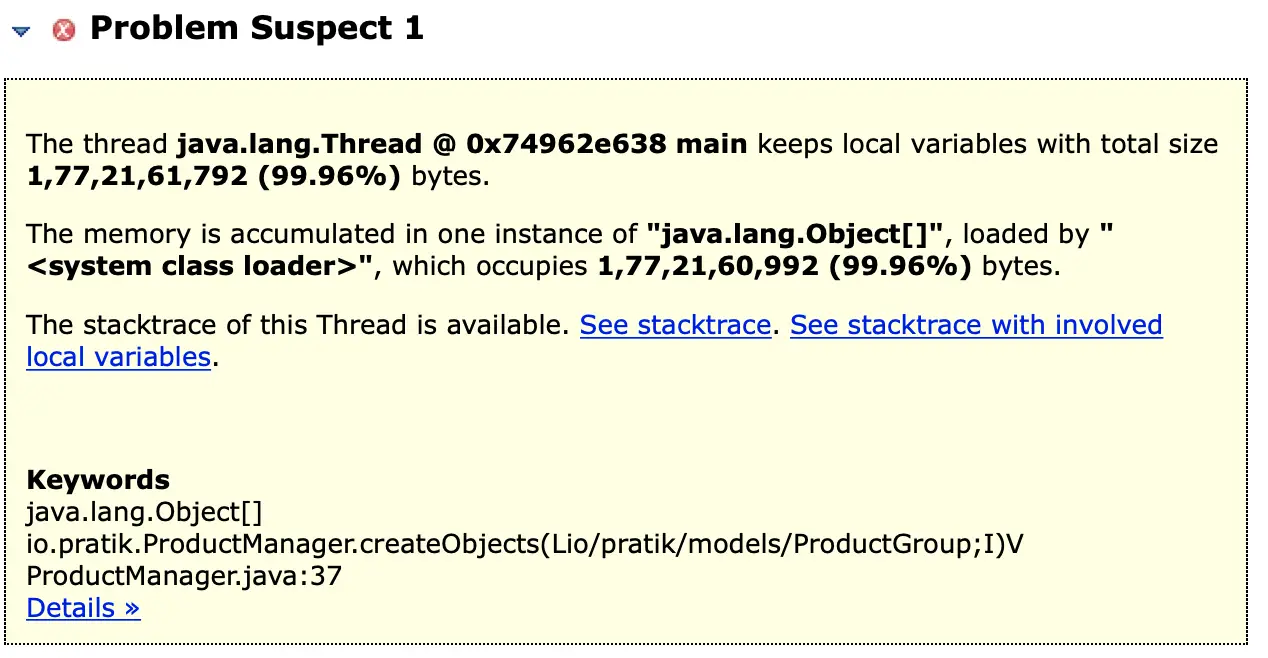
Apart from the summary, this report also contains detailed information about the suspects which is accessed by following the “details” link at the bottom of the report:
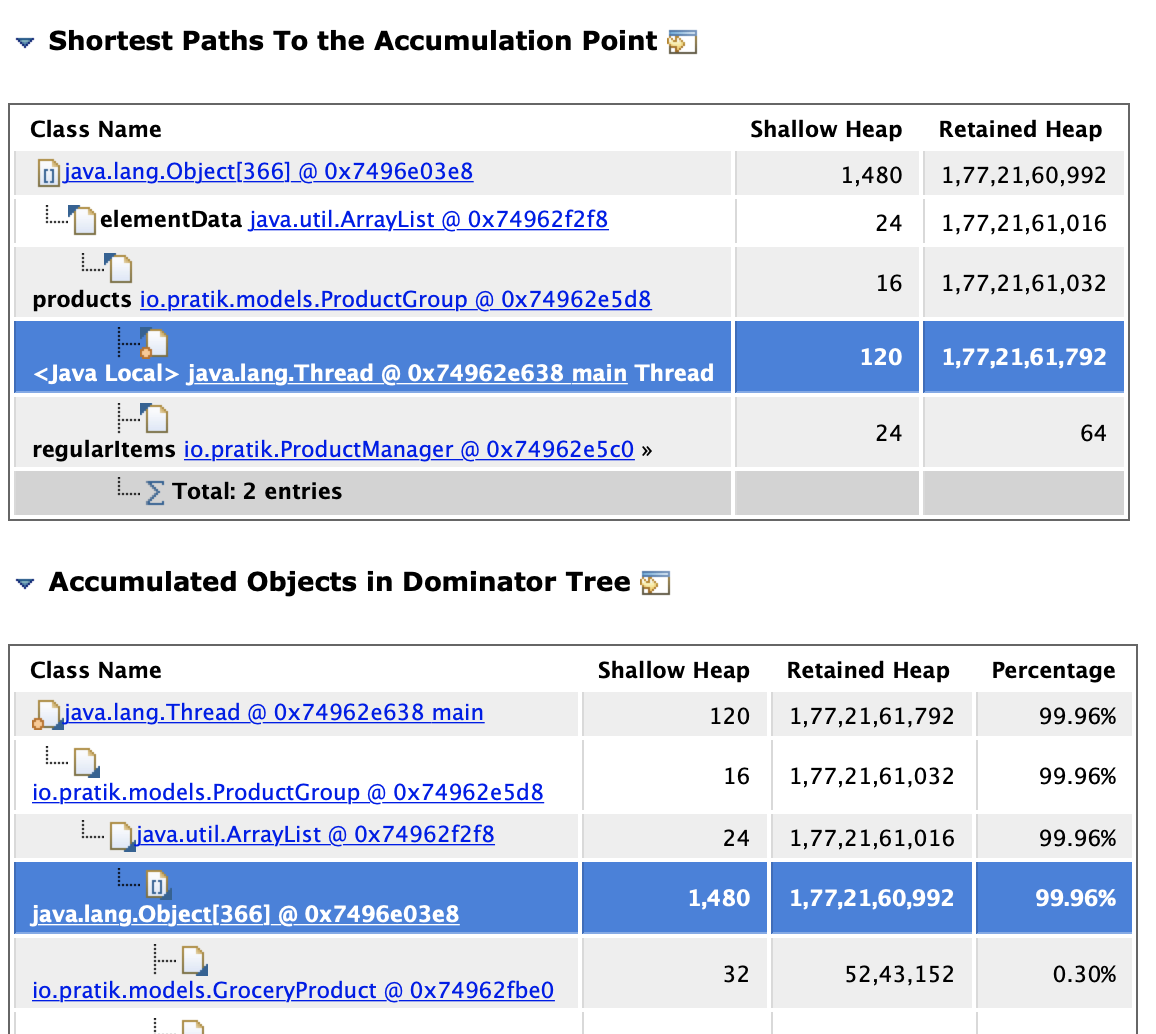
The detailed information is comprised of :
Shortest paths from GC root to the accumulation point : Here we can see all the classes and fields through which the reference chain is going, which gives a good understanding of how the objects are held. In this report, we can see the reference chain going from the Thread to the ProductGroup object.
Accumulated Objects in Dominator Tree : This gives some information about the content which is accumulated which is a collection of GroceryProduct objects here.
In this post, we introduced the heap dump, which is a snapshot of a Java application’s object memory graph at runtime. To illustrate, we captured the heap dump from a program that threw an OutOfMemoryError at runtime.
We then looked at some of the basic concepts of heap dump analysis with Eclipse Memory Analyzer: large objects, GC roots, shallow vs. retained heap, and dominator tree, all of which together will help us to identify the root cause of specific memory issues.
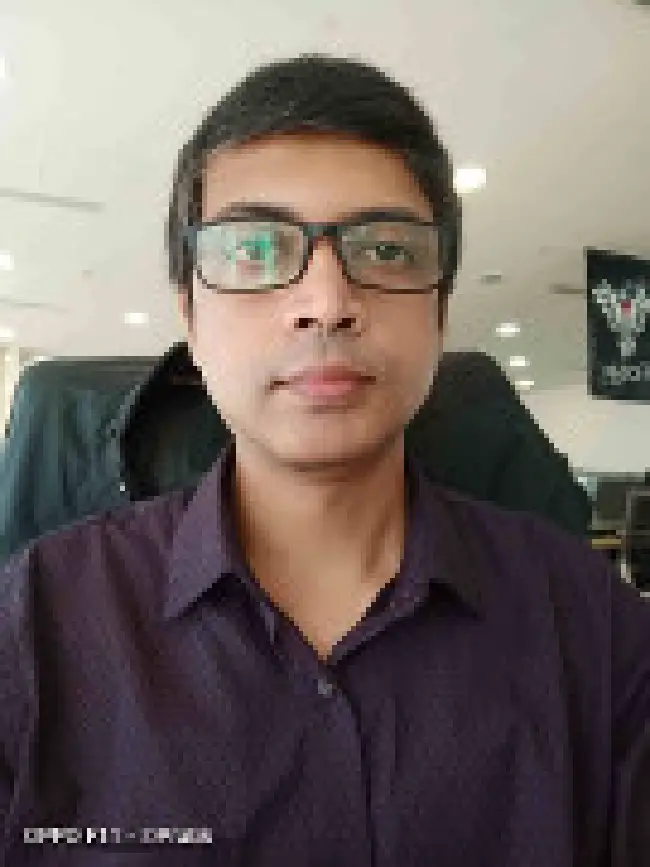
Software Engineer, Consultant and Architect with current expertise in Enterprise and Cloud Architecture, serverless technologies, Microservices, and Devops.
Recent Posts
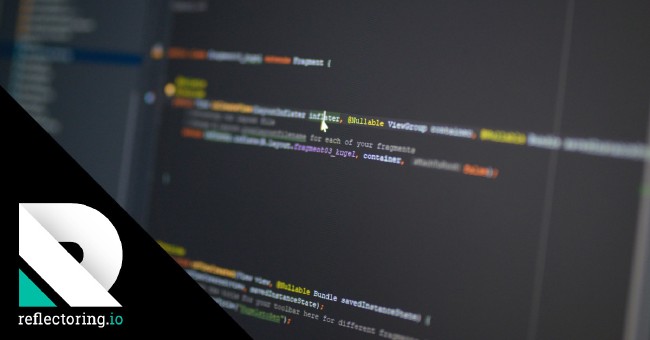
Inheritance, Polymorphism, and Encapsulation in Kotlin
- Ezra Kanake
- May 12, 2024
In the realm of object-oriented programming (OOP), Kotlin stands out as an expressive language that seamlessly integrates modern features with a concise syntax.
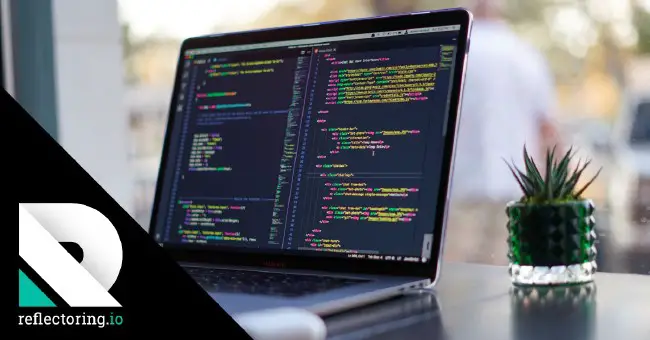
- Spring Boot
Publisher-Subscriber Pattern Using AWS SNS and SQS in Spring Boot

- May 3, 2024
In an event-driven architecture where multiple microservices need to communicate with each other, the publisher-subscriber pattern provides an asynchronous communication model to achieve this.
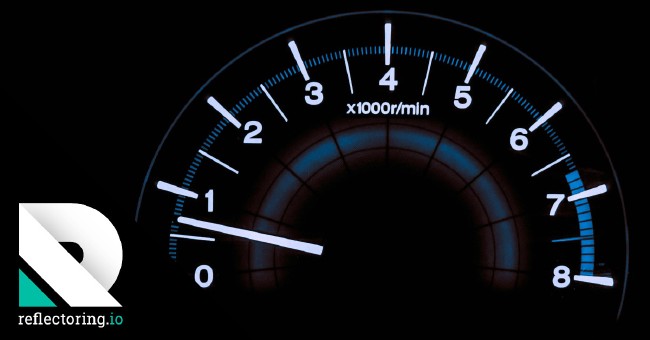
Optimizing Node.js Application Performance with Caching

- April 20, 2024
Endpoints or APIs that perform complex computations and handle large amounts of data face several performance and responsiveness challenges. This occurs because each request initiates a computation or data retrieval process from scratch, which can take time.
Andy Balaam's Blog
Four in the morning, still writing Free Software
How to analyse a .phd heap dump from an IBM JVM
Share on Mastodon
If you have been handed a .phd file which is a dump of the heap of an IBM Java virtual machine, you can analyse it using the Eclipse Memory Analyzer Tool (MAT), but you must install the IBM Monitoring and Diagnostic Tools first.
Download MAT from eclipse.org/mat/downloads.php . I suggest the Standalone version.
Unzip it and run the MemoryAnalyzer executable inside the zip. Add an argument to control how much memory it gets e.g. to give it 4GB:
Once it’s started, go to Help -> Install new software.
Next to “Work with” paste in the URL for the IBM Developer Toolkit update site: http://public.dhe.ibm.com/ibmdl/export/pub/software/websphere/runtimes/tools/dtfj/
Click Add…
Type in a name like “IBM Monitoring and Diagnostic Tools” and click OK.
In the list below, an item should appear called IBM Monitoring and Diagnostic Tools. Tick the box next to it, click Next, and follow the wizard to accept the license agreements and install the toolkit.
Restart Eclipse when prompted.
Choose File -> Open Heap Dump and choose your .phd file. It should open in MAT and allow you to figure out who is using all that memory.
9 thoughts on “How to analyse a .phd heap dump from an IBM JVM”
Very helpful guide.
Very nice buddy! Thank you!
If need any help on HEAPDUMP and JAVACORE for WebSphere products, please contact me!
https://www.linkedin.com/in/dougcardoso21/
Thanks Douglas!
Thanks for this… IBM product is garbage (no pun intended).
Thanks you !!
When I tried to update ini file to 4g, it did not open MAT. I needed to reset to what it was that is 1024m and then when I opened this phd file, it gave an error that Error opening heap dump is encountered. Does someone know what to do?
Very helpful , thanks.
Hi Poonam If you are on windows, type cmd in the search , go into command prompt. In the command prompt , change directory (cd) to the directory that MemoryAnalyser.exe is in. Then type MemoryAnalyzer -vmargs -Xmx4g and press enter.
- Pingback: ¿Cómo crear un volcado de almacenamiento dinámico compatible con OpenJ9 a través de API? – stack
Leave a Reply
Your email address will not be published. Required fields are marked *
Don't subscribe All new comments Replies to my comments Notify me of followup comments via e-mail. You can also subscribe without commenting.
This site uses Akismet to reduce spam. Learn how your comment data is processed .
Heap dump analysis using Eclipse Memory Analyzer Tool (MAT)
A heap dump is a snapshot of all the Java objects that exist in the heap space. The heap dump file is usually stored with .hprof extension.
In this post, we will see how you can take the heap dump of your running Java application and use Eclipse’s Memory Analyzer (MAT) to identify memory hotspots and possibility detect memory leak.
Why and When should I take the Heap dump?
You may need to take the Heap dump if your Java application is taking up more memory than you expected or your Java application crashed with OutOfMemoryError. Analyzing the heap dump will lead us to the root cause of the anomaly.
Using the heap dump we can find details like the memory usage per class, number of objects per class, etc. We can also go into fine details and find out the amount of memory retained by a single Java object in the application. These details can help us pinpoint the actual code that is causing the memory leak issues.
How do you analyze very large Heap dumps?
Usually analyzing heap dump takes even more memory than the actual heap dump size and this may be problematic if you are trying to analyze heap dump from a large server on your development machine. For instance, a server may have crashed with a heap dump of size 24 GB and your local machine may only have 16 GB of memory. Therefore, tools like MAT, Jhat won’t be able to load the heap dump file. In this case, you should either analyze the heap dump on the same server machine which doesn’t have memory constraint or use live memory sampling tools provided by VisualVM.
How to take a Heap dump of your running Java Application
There are several ways to take a heap dump. We will talk about the 3 easiest ways to do it.
Command-line interface to generate the Heap Dump
These steps are common for all operating systems including Windows, Linux, and macOS.
- Find the process id of your running Java application. You can use the jps tool to list down all the running Java processes on your local machine. The processes will be listed in the following format “<pid> <MainClass>”
The option live is important if you want to collect only the live objects i.e objects that still have a reference in the running code.
VisualVM to generate the Heap Dump
Visual VM makes it very easy to take a heap dump running on your local machine. The following steps can be used to generate heap dump using VisualVM
- Start Visual VM and connect your local Java Application to it.

- After clicking on the heap dump you will be redirected to a new tab from which you can find out the location of your heap dump.
JConsole to generate the Heap dump
- Connect your application to JConsole .
- Switch to MBeans tab and select com.sun.management > HotSpotDiagnostic > Operations > dumpHeap.

- The parameter p0 is the location and the name of the heap dump file. Ensure that you add the “.hprof” extension at the end of the file name.
- The parameter p1, if set to true, performs a GC before dumping the heap so that only live objects are present in the heap dump.
Which tools can be used to analyze the heap dump or open the .hprof file?
Once you have the heap dump the next step is to analyze it using a tool. There are multiple paid and equally good open source tools available to analyze the Heap dump. Memory Analyzer (MAT) is one of the best open-source tool that can be used as a plugin with Eclipse or as a standalone application if you don’t have Eclipse IDE installed. Apart from MAT, you can use Jhat, VisualVM. However, in this post, we will discuss the features provided with MAT.
Downloading the Memory Analyzer (MAT)
There are two ways to use the Memory Analyzer tool.
Integrating MAT plugin with Eclipse
- Open Eclipse IDE and select Help > Eclipse Marketplace.

- Restart Eclipse and the plugin is ready to be used.
Downloading the standalone version of Eclipse MAT
- Download and install the Java Development Kit .
- Download the install standalone MAT application from this link .

Loading Heap dump file in Eclipse MAT
We will be analyzing the heap dump generated by this Java application . The memory leak in the application is discussed in depth in this tutorial . And the screenshots posted below are from the MAT plugin used with Eclipse IDE.
The steps to load the heap dump are as follows.
- Open Eclipse IDE or the standalone MAT Tool.
- From the toolbar, Select Files > Open File from the dropdown menu.
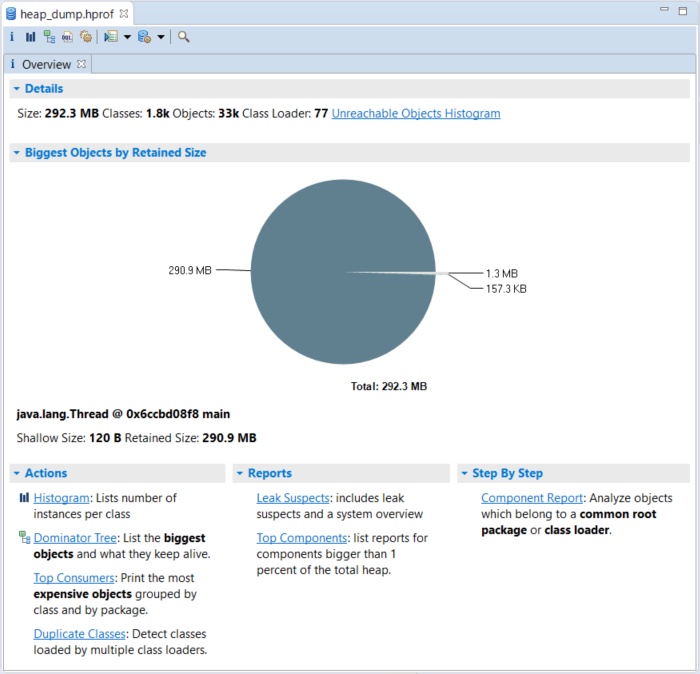
We will go through some of the important tools like Histogram, Dominator Tree and Leak Suspect report which can be used to identify memory leaks.
Histogram lists all the different classes loaded in your Java Application at the time of heap dump. It also lists the number of objects per class along with the shallow and retained heap size. Using the histogram, it is hard to identify which object is taking the most memory. However, we can easily identify which class type holds the largest amount of memory. For instance, in the screenshot below byte array holds the largest amount of memory. But, we cannot identify which object actually holds that byte array.
Shallow Heap v/s Retained Heap
Shallow Heap is the size of the object itself. For instance, in the screenshot below byte array itself holds the largest amount of memory. Retained Heap is the size of the object itself as well as the size of all the objects retained in it. For instance, in the screenshot below the DogShelter object itself holds a size of 16 bytes. However, it has a retained heap size of more than 305Mb which means it likely holds the byte array which contributes to the very large retained heap size.

Finally, from the Histogram, we infer that the problem suspect is byte[] which is retained by the object of class DogShelter or Dog.
Dominator Tree
The dominator tree of the Java objects allows you to easily identify object holding the largest chunk of memory. For instance, we can see from the snipped below that the Main Thread object holds the largest memory. On collapsing the main thread tree we can see that the instance of class DogShelter holds a hashmap holding over 300Mb of memory.
Dominotart tree is useful when you have a single object that is eating up a large amount of memory. The Dominator tree wouldn’t make much sense if multiple small objects are leading to a memory leak. In that case, it would be better to use the Histogram to find out the instances of classes that consume the most amount of memory.
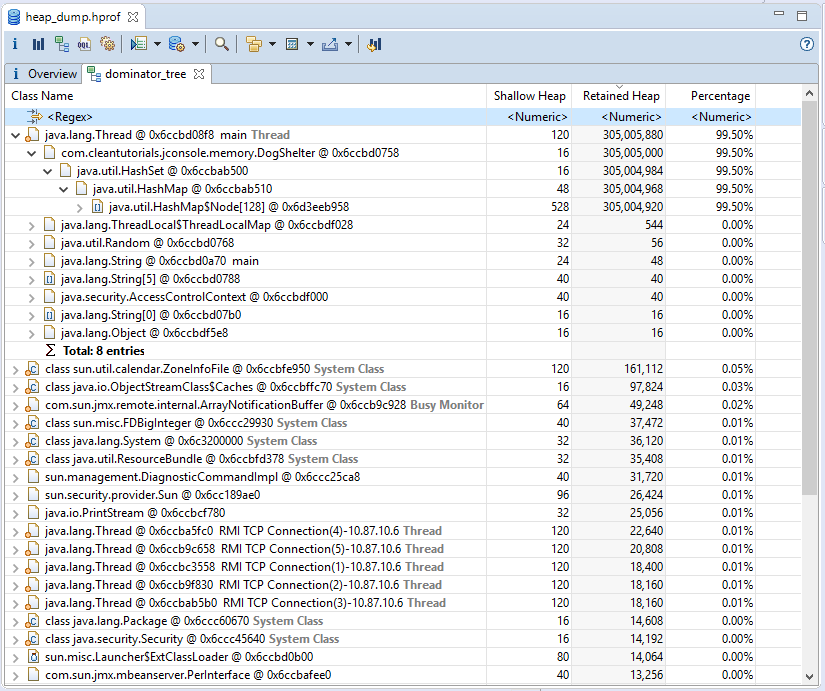
From the Dominator Tree, we infer that the problem suspect is the DogShelter class.
Duplicate Classes
The duplicate class tab will list down the classes that are loaded multiple times. If you are using ClassLoaders in your code you can use the Duplicate Classes to ensure that the code is functioning properly and classes are not loaded multiple times.
Leak Suspect
Finally, the Leak suspect report runs a leak suspect query that analyzes the Heap dump and tries to find the memory leak. For non-trivial memory leaks, the Leak suspect query may not be able to identify the memory leak and it’s up to the developer with the knowledge of the program to pinpoint the leak using the tools discussed above.
Since we had a very trivial memory leak, the inference that we derived manually using Histogram and Dominator Tree is the same as the inference from the leak suspect report as seen below.
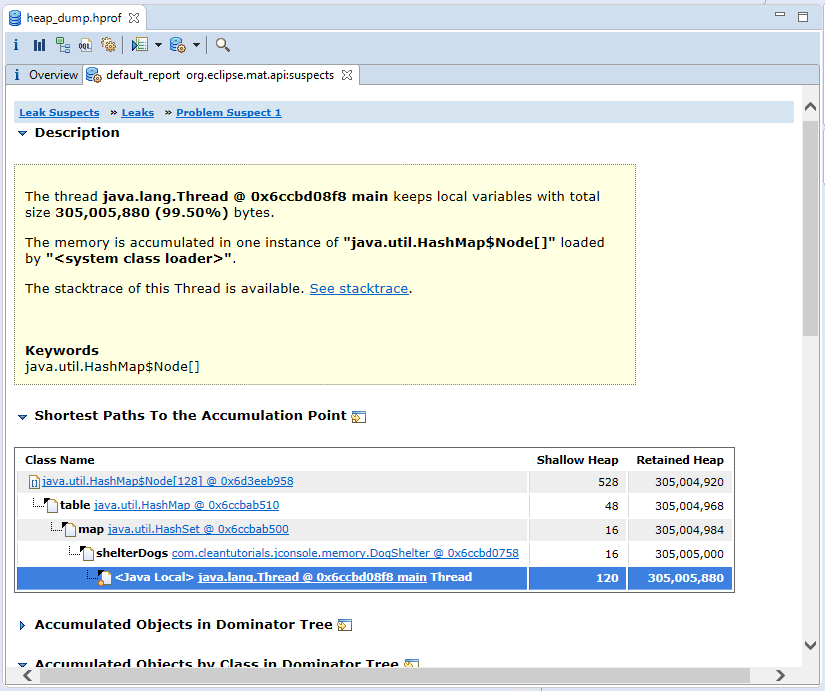
- Manage Cookies
- Working Groups
- Marketplace
- Planet Eclipse
- Report a Bug
- Mailing Lists
- Documentation
- Getting Started / Support
- How to Contribute
- IDE and Tools
- Newcomer Forum
Participate
Eclipse IDE
Breadcrumbs
- Eclipse Wiki
MemoryAnalyzer
Notice: this wiki is now read only and edits are no longer possible. please see: https://gitlab.eclipse.org/eclipsefdn/helpdesk/-/wikis/wiki-shutdown-plan for the plan..
- View source
- 2.1 Installation
- 2.2 Basic Tutorials
- 2.3 Further Reading
- 3.1 HPROF dumps from Sun Virtual Machines
- 3.2 System Dumps and Heap Dumps from IBM Virtual Machines
- 3.3 What if the Heap Dump is NOT Written on OutOfMemoryError?
- 4 Extending Memory Analyzer
The Eclipse Memory Analyzer tool (MAT) is a fast and feature-rich heap dump analyzer that helps you find memory leaks and analyze high memory consumption issues.
With Memory Analyzer one can easily
- find the biggest objects, as MAT provides reasonable accumulated size (retained size)
- explore the object graph, both inbound and outbound references
- compute paths from the garbage collector roots to interesting objects
- find memory waste, like redundant String objects, empty collection objects, etc...
Getting Started
Installation.
See the download page for installation instructions.
Basic Tutorials
Both the Basic Tutorial chapter in the MAT documentation and the Eclipse Memory Analyzer Tutorial by Lars Vogel are a good first reading, if you are just starting with MAT.
Further Reading
Check MemoryAnalyzer/Learning Material . You will find there a collection of presentations and web articles on Memory Analyzer, which are also a good resource for learning. These pages Querying Heap Objects (OQL) OQL Syntax MemoryAnalyzer/OQL also explain some of the ways to use Object Query Language (OQL)
Getting a Heap Dump
Hprof dumps from sun virtual machines.
The Memory Analyzer can work with HPROF binary formatted heap dumps . Those heap dumps are written by Sun HotSpot and any VM derived from HotSpot. Depending on your scenario, your OS platform and your JDK version, you have different options to acquire a heap dump.
Non-interactive
If you run your application with the VM flag -XX:+HeapDumpOnOutOfMemoryError a heap dump is written on the first Out Of Memory Error. There is no overhead involved unless a OOM actually occurs. This flag is a must for production systems as it is often the only way to further analyze the problem.
As per this article , the heap dump will be generated in the "current directory" of the JVM by default. It can be explicitly redirected with -XX:HeapDumpPath= for example -XX:HeapDumpPath=/disk2/dumps . Note that the dump file can be huge, up to Gigabytes, so ensure that the target file system has enough space.
Interactive
As a developer, you want to trigger a heap dump on demand. On Windows, use JDK 6 and JConsole . On Linux and Mac OS X , you can also use jmap that comes with JDK 5.
- tutorial here
Via Java VM parameters:
- -XX:+HeapDumpOnOutOfMemoryError writes heap dump on OutOfMemoryError (recommended)
- -XX:+HeapDumpOnCtrlBreak writes heap dump together with thread dump on CTRL+BREAK
- -agentlib:hprof=heap=dump,format=b combines the above two settings (old way; not recommended as the VM frequently dies after CTRL+BREAK with strange errors)
- Sun (Linux, Solaris; not on Windows) JMap Java 5 : jmap -heap:format=b <pid>
- Sun (Linux, Solaris; Windows see link) JMap Java 6 : jmap.exe -dump:format=b,file=HeapDump.hprof <pid>
- Sun (Linus, Solaris) JMap with Core Dump File: jmap -dump:format=b,file=HeapDump.hprof /path/to/bin/java core_dump_file
- Sun JConsole: Launch jconsole.exe and invoke operation dumpHeap() on HotSpotDiagnostic MBean
- SAP JVMMon: Launch jvmmon.exe and call menu for dumping the heap
Heap dump will be written to the working directory.
System Dumps and Heap Dumps from IBM Virtual Machines
Memory Analyzer may read memory-related information from IBM system dumps and from Portable Heap Dump (PHD) files with the IBM DTFJ feature installed. Once installed, then File > Open Heap Dump should give the following options for the file types:
- All known formats
- HPROF binary heap dumps
- IBM 1.4.2 SDFF
- IBM Javadumps
- IBM SDK for Java (J9) system dumps
- IBM SDK for Java Portable Heap Dumps
For a comparison of dump types, see Debugging from dumps . System dumps are simply operating system core dumps; therefore, they are a superset of portable heap dumps. System dumps are far superior than PHDs, particularly for more accurate GC roots, thread-based analysis, and unlike PHDs, system dumps contain memory contents like HPROFs. Older versions of IBM Java (e.g. < 5.0SR12, < 6.0SR9) require running jextract on the operating system core dump which produced a zip file that contained the core dump, XML or SDFF file, and shared libraries. The IBM DTFJ feature still supports reading these jextracted zips; however, newer versions of IBM Java do not require jextract for use in MAT since DTFJ is able to directly read each supported operating system's core dump format. Simply ensure that the operating system core dump file ends with the .dmp suffix for visibility in the MAT Open Heap Dump selection. It is also common to zip core dumps because they are so large and compress very well. If a core dump is compressed with .zip , the IBM DTFJ feature in MAT is able to decompress the ZIP file and read the core from inside (just like a jextracted zip). The only significant downsides to system dumps over PHDs is that they are much larger, they usually take longer to produce, they may be useless if they are manually taken in the middle of an exclusive event that manipulates the underlying Java heap such as a garbage collection, and they sometimes require operating system configuration ( Linux , AIX ) to ensure non-truncation.
In recent versions of IBM Java (> 6.0.1), by default, when an OutOfMemoryError is thrown, IBM Java produces a system dump, PHD, javacore, and Snap file on the first occurrence for that process (although often the core dump is suppressed by the default 0 core ulimit on operating systems such as Linux). For the next three occurrences, it produces only a PHD, javacore, and Snap. If you only plan to use system dumps, and you've configured your operating system correctly as per the links above (particularly core and file ulimits), then you may disable PHD generation with -Xdump:heap:none. For versions of IBM Java older than 6.0.1, you may switch from PHDs to system dumps using -Xdump:system:events=systhrow,filter=java/lang/OutOfMemoryError,request=exclusive+prepwalk -Xdump:heap:none
In addition to an OutOfMemoryError, system dumps may be produced using operating system tools (e.g. gcore in gdb for Linux, gencore for AIX, Task Manager for Windows, SVCDUMP for z/OS, etc.), using the IBM Java APIs , using the various options of -Xdump , using Java Surgery , and more.
Versions of IBM Java older than IBM JDK 1.4.2 SR12, 5.0 SR8a and 6.0 SR2 are known to produce inaccurate GC root information.
What if the Heap Dump is NOT Written on OutOfMemoryError?
Heap dumps are not written on OutOfMemoryError for the following reasons:
- Application creates and throws OutOfMemoryError on its own
- Another resource like threads per process is exhausted
- C heap is exhausted
As for the C heap, the best way to see that you won't get a heap dump is if it happens in C code (eArray.cpp in the example below):
C heap problems may arise for different reasons, e.g. out of swap space situations, process limits exhaustion or just address space limitations, e.g. heavy fragmentation or just the depletion of it on machines with limited address space like 32 bit machines. The hs_err-file will help you with more information on this type of error. Java heap dumps wouldn't be of any help, anyways.
Also please note that a heap dump is written only on the first OutOfMemoryError. If the application chooses to catch it and continues to run, the next OutOfMemoryError will never cause a heap dump to be written!
Extending Memory Analyzer
Memory Analyzer is extensible, so new queries and dump formats can be added. Please see MemoryAnalyzer/Extending_Memory_Analyzer for details.
- Tools Project
- Memory Analyzer
This page was last modified 07:12, 28 December 2022 by Erik Brangs . Based on work by Andrew Johnson , Kevin Grigorenko and Krum Tsvetkov and others .
Back to the top
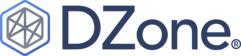
- Manage Email Subscriptions
- How to Post to DZone
- Article Submission Guidelines
- Manage My Drafts
Data Engineering: Work with DBs? Build data pipelines? Or maybe you're exploring AI-driven data capabilities? We want to hear your insights.
Modern API Management : Dive into APIs’ growing influence across domains, prevalent paradigms, microservices, the role AI plays, and more.
Programming in Python: Dive into the Python ecosystem to learn about popular libraries, tools, modules, and more.
PostgreSQL: Learn about the open-source RDBMS' advanced capabilities, core components, common commands and functions, and general DBA tasks.
- Java Thread Dump Analysis
- Problems With Nested CompletableFuture in Java
- Achieving Inheritance in NoSQL Databases With Java Using Eclipse JNoSQL
- Mastering Exception Handling in Java CompletableFuture: Insights and Examples
- Is RBAC Still Relevant in 2024?
- Spring Microservice Application Resilience: The Role of @Transactional in Preventing Connection Leaks
- SQL Convertor for Easy Migration from Presto, Trino, ClickHouse, and Hive to Apache Doris
- AWS To Azure Migration
Java Heap Dump Analyzer
Learn about heap and garbage collection in java applications and how to take the heap dump of an application and analyze it in eclipse memory analyzer..
Join the DZone community and get the full member experience.
Identifying the reason for an OutOfMemory error in Java applications with a larger heap size is a nightmare for a developer, because most of the OutOfMemory situations may not be identified during the testing phase. It may occur only in production after running for a long time. The purpose of this article is to explain the use of a heap analyzer tool to identify a memory leakage in larger enterprise Java applications, which use a larger size of heaps. Before going to the details, we will discuss the following points:
Garbage Collection
Outofmemory, what is heap.
The space used by the Java Runtime to allocate memory to Objects and JRE Classes is called Heap. The heap space can be configured using the following JVM arguments:
- -Xmx<size> — Setting maximum Java heap size
- -Xms<size> — Setting initial Java heap size
The maximum heap size that can be configured in a 32 bit JVM is 2GB. If any application requires more than 2 GB, it should run on 64 bit JVM. 64MB is the maximum heap size by default.
One of the advantages of Java over C++ is automatic memory management. In C++, memory can be released manually, but it will happen automatically in Java using a process called garbage collection. Garbage collection will free up the memory of an object that doesn’t have any reference; it will destroy unused objects. The garbage collection process can be tuned for different applications based on the object creation characteristics of the application. This can be achieved through a number of JVM arguments. Following are a few JVM arguments which can be used to tune the garbage collection process:
GC Execution has a direct relationship with the size of the Heap.
- Larger Heap size will increase the GC execution time, but decrease the number of GC executions.
- Smaller Heap Size will increase the number of GC executions, but decrease the GC execution time
A java.lang.OutOfMemoryError will occur when the application tries to add more objects into the heap and there is no space left. This will happen when the maximum heap size set in the start of the application is filled with objects and the garbage collector is not able to free up the memory because the all objects in heap still have some references. This may happen because of two reasons:
The application may need more memory to run; the currently allocated heap size is not enough to accommodate the objects generated during the runtime.
Due to a coding error in the application which is keeping the references of unwanted objects.
The solution for the first reason is to increase the heap size. The solution for the second is to analyze the code flow and heap dump to identify the unwanted objects in heap. To analyze the application heap, we need to take the heap dump and open it in a memory analyzing tool.
In this article, we will discuss on how to take the heap dump of an application running on Oracle Java and analyze it in Eclipse Memory Analyzer .
How to Take Heap Dump
Heap dump can be taken in two ways:
- A JVM argument can be added to generate heap dump whenever an OutOfMemoryError occurs.
The -XX:+HeapDumpOnOutOfMemoryError option can be added to generate a heap dump on OutOfMemoryError. By default, the heap dump is created in a file called java_pid pid .hprof in the working directory of the VM, but we can set an alternative path using the JVM option -XX:HeapDumpPath=path .
- Using a jmap tool available with JDK. The following command can be executed from the command line:
jmap -dump:format=b,file=heap.bin <pid>
"<pid>" can be replaced with the process id of the application.
Eclipse Memory Analyzer
Eclipse Memory Analyzer can download from eclipse.org .
Unzip the downloaded file and double click "MemoryAnalyzer" to start the application.
Execute a Java program which is continuously running.

Take heap dump using jmap:
jmap -dump:format=b,file=heap.bin 6920

In Linux, to identify the process id, use ps –ef | grep java .
Open the heap dump in Eclipse Memory Analyzer using the option File --> Open Heap Dump.
First, it will prompt you to create a leak suspect report. The user can create it or skip it.
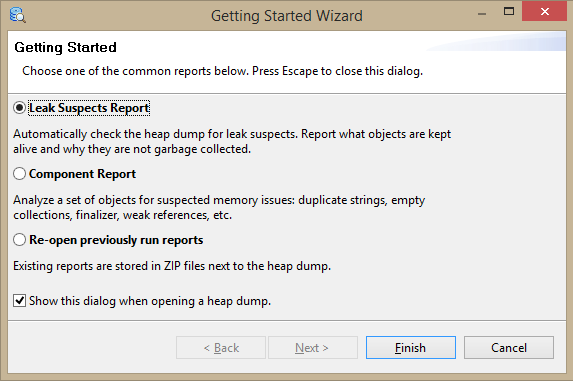
The "overview" tab of the memory analyzer will show the total size of the heap and a pie chart of object size.
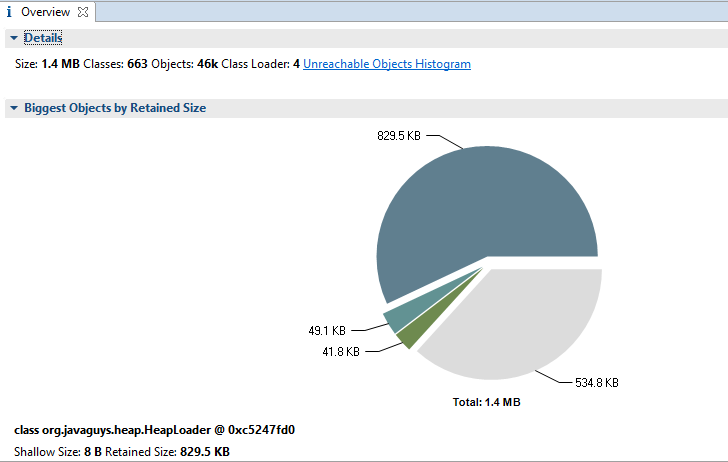
Click on the highest value in the pie chart and select List Objects --> with outgoing references.
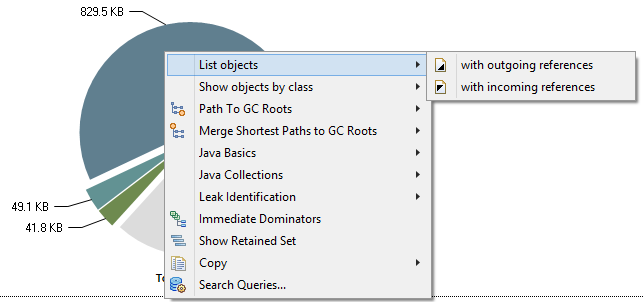
It will open a new tab, " List Objects ," and expand the tree structure of the object.
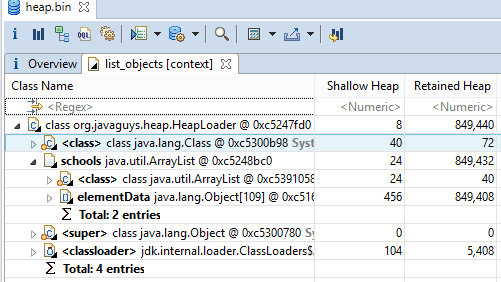
The user can expand and find the objects available in heap. In this example, the HeapLoader object consist of a list of School objects, and school objects consist of a list of students.
School List in HeapLoader
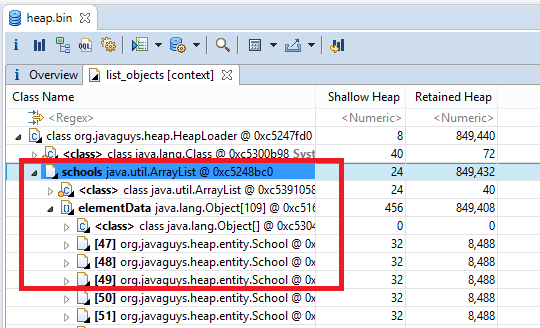
Each school object in List and its attributes
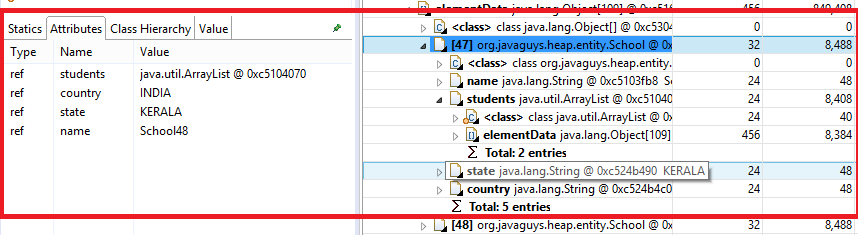
Student object in school and its attribute
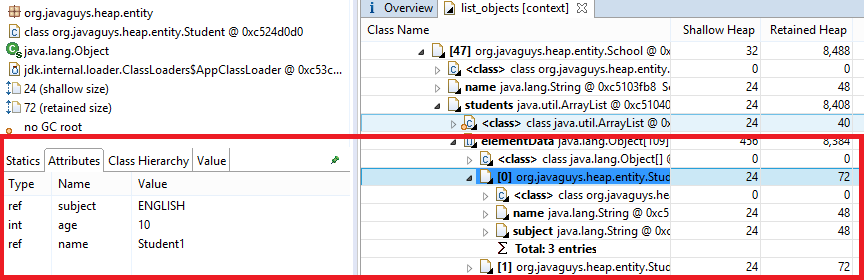
There is an option available in Eclipse Memory Analyzer to Acquire Heap Dump if the application is running on the same machine. Select File --> Acquire Heap Dump to show all Java applications available on the machine. Select the process, browse the path where you want the heap to save. and click "finish."
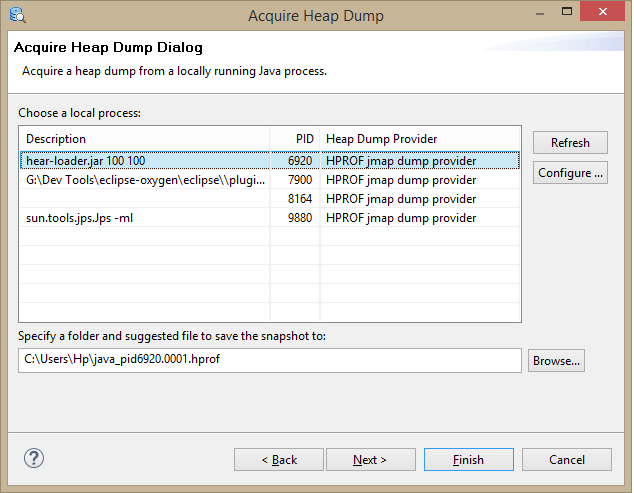
These are the basic steps to analyze a heap dump. There are more options available in Memory Analyzer, which will give more insight into the heap dump. Eclipse Heap Analyzer can be used for the dumps generated from an application running on Oracle Java.
Sample Application for Testing
The sample application used in the above example consists of two objects, School and Student. Details about the sample application are available here .
https://www.oracle.com/technetwork/articles/java/vmoptions-jsp-140102.html
https://www.eclipse.org/mat/downloads.php
Published at DZone with permission of Sudeep C M . See the original article here.
Opinions expressed by DZone contributors are their own.
Partner Resources
- About DZone
- Send feedback
- Community research
- Advertise with DZone
CONTRIBUTE ON DZONE
- Become a Contributor
- Core Program
- Visit the Writers' Zone
- Terms of Service
- Privacy Policy
- 3343 Perimeter Hill Drive
- Nashville, TN 37211
- [email protected]
Let's be friends:
- Manage Cookies
- Documentation
- Marketplace
- Planet Eclipse
- Report a Bug
- Mailing Lists
- Getting Started / Support
- How to Contribute
- IDE and Tools
- Newcomer Forum
Participate
Eclipse IDE
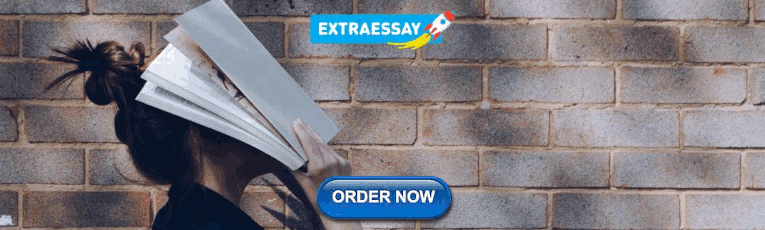
Breadcrumbs
Memory analyzer (mat).
- Download the latest version as RCP application.
- Read our Blog for background information.
- Post your questions to the Forum .
- 8 May 2024, Memory Analyzer moved to Github The Memory Analyzer code repository has moved from the Eclipse Git servers to Github - https://github.com/eclipse-mat/mat . With this move, the project also switched from using the Eclipse Bugzilla to using Github Issues on the MAT Github project. We are really happy about the move, however, it might take some time to update all places pointing to the old repository at Eclipse. Please bear with us while we do the transition and give us feedback if you see places we've missed to update!
- 6 December 2023, Memory Analyzer version 1.15.0 released Memory Analyzer 1.15.0 Release is now available for download . Check the New and Noteworthy page for an overview of new features and fixes, including security fixes. Further details are available in the release record .
Back to the top
JAVA & ANDROID HEAP
Dump analyzer.
- Auto Memory Leak Detection
- Tips to Reduce Memory 30-70%
- No Download/No Installation
Deep Learning
- Brilliant UI
- Report in seconds
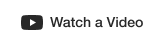
Upload Heap Dump File
Tip: For quick results compress (*.zip, *.gz) and upload heap dump file
1. POST HTTP request to API end point https://heaphero.io/analyze-hd-api?apiKey={Add Your API_KEY}
2. The body of the HTTP request should contain the Heap dump
3. HTTP response will be sent back in JSON format
Tip: For quick results compress (*.zip & *.gz) and paste http(s) or S3 presigned URL (More details)
I accept the terms of service
- Uploading heap dump...
Download and Install on your Local Machine
Start your 14 day free trial today
Ready to move ahead? Buy Now
What is Remote Location?
Companies trusted and collaborated with us.
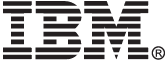
How Much Memory Your Application Wastes?
Due to inefficient programming, modern applications waste 30% to 70% of memory. HeapHero is the industry's first tool to detect the amount of wasted memory. It reports what lines of source code originating the memory wastage and solutions to fix them.
Android Memory Leak
Android mobile applications can also suffer from memory leaks, which can be attributed to poor programming practices. Memory leaks in mobile apps bare direct consumer impact and dissatisfaction. Memory leak slows down the application's responsiveness, makes it hang or crashes the application entirely. It will leave an unpleasant and negative user experience.
Java Memory Leak
A Memory leak is a type of resource drain that occurs when an application allocates memory and does not release after finish using it. This allocated memory can not be used for any other purpose and it remains wasted. As a consequence, Java applications will exhibit one or more of these non-desirable behaviors: poor response time, long JVM pauses, application hang, or even crash.
OutOfMemoryError
One common indication of a memory problem is the java.lang.OutOfMemoryError. This error is typically thrown when there is insufficient space to create a new object in the Java heap. There are 8 flavors of OutOfMemoryError. Each flavor of OutOfMemoryError has different causes and solutions.
Memory Regression
Sometimes the latest version of an application might be consuming more memory than the previous version. You need to analyze what are the large objects residing in the memory? Where is it being created? Where is it being held up? Answers to all these questions can be found in Heap Hero's Heap Analysis Report.

Memory Hogs
Wrong data structure choice, created but unused data structure, overallocated and underutilized data structure, suboptimal data type usage (i.e., using 'long' instead of 'int'), data duplication - all these can easily waste 30 - 70% of your memory. Heap Hero's intelligence report helps eliminate these memory hogs.
Universal Memory Analyzer
Hprof viewer and analyzer.
HPROF is a simple command-line tool that captures CPU/Heap profiles to identify performance bottlenecks in applications. By default, this tool writes the captured profiles to a file with '.hprof ' extension. HPROF file may contain CPU usage, heap allocation statistics, heap dump, thread stack traces and monitor states. It can be either in binary or text format. Heap Hero is a powerful tool to view and analyze HPROF files.
Universal Memory Dump Analysis
Heap Hero, a universal tool that will parse and analyze heaps dumps written in any language that runs on the JVM. It will convert Java, Scala, Jython, JRuby heap dumps to useful information to optimize your memory usage.
Reliably and quickly fix your memory problems through a precision single-page view of your heap dumps. View intuitive data display of heap histogram, largest objects, and memory leak suspects with a concisely brilliant interface.
Free Service
Our award-winning heap dump analysis tool is offered as a free service. Our tools help you to fix memory leaks, OutOfMemoryError, memory regression, memory hogs and any memory-related problems. All this power at your fingertip for free.
Android Memory Analysis
Android is the world's largest mobile platform. Heap Hero can parse and analyze the heap dumps generated from any Android devices. Heap Hero's deep learning algorithms can report memory leak suspects and objects wasting memory.
Online Heap Dump Analysis Tool
Heap Hero is the world's first and the only cloud-based heap dump analysis tool. Registration, download, or installation is not required to use the tool. Just upload your application's heap dumps & review the beautiful reports instantly.
Android Memory Optimizer
Heap Hero has built the industry's first and only REST API to analyze heap dumps. Stop manually uploading and analyzing heap dumps. Instead, analyze heap dumps from all your JVMs and Android devices in a programmatic manner through HEAP HERO's REST API .
Our award-winning deep learning algorithms have the intelligence to detect memory leaks and isolate the objects that are causing the memory leaks. Save time spent on diagnosing the memory leaks. It's all done automatically.
Collaboration
Heap Dump files consume a lot of disk space, making it very hard to share and collaborate with the team. HeapHero provides shareable URL links to heap dump analysis reports, making it a breeze to share and collaborate heap dump analysis with your fellow engineers.
Heap Dump Analysis Tool Beauty to the Beast
Analyzing heap dump doesn't have to be a tedious job. it can be fun, and it can be 'wow'.
- Online Tool
- Android Memory Analyzer
- Memory Leak Detection Tool
- Heap Analyzer
- HPROF Viewer & Analyzer
Optimize Memory
OutOfMemory Error
Detect Memory Leak
Our Services
We have optimized hundreds of open source and enterprise applications. Please take advantage of our battle-fought experience. We can either come on-site or provide remote consulting services.
Our easy to understand, fun-filled, on-site training programs are a preferred choice for several enterprises to transform thier engineers into performance experts.
Scaling in AWS
Are you looking to port your application to the AWS cloud? Are you following the AWS best practices? Are looking to lower your AWS bills? We are here to help you.
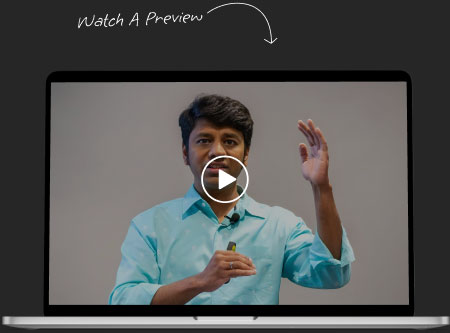
Learn JVM Performance and Troubleshooting
- Become a world class JVM performance expert
- Troubleshoot production performance problems in a fraction of time
Instructor: Ram Lakshmanan, Architect of GCeasy
What's included:
9 hours of video series with case studies and real life examples
3 months yCrash tool subscription
e-books and study material to complete this course
LinkedIn shareable certificate
1 year course subscription
Attended by engineers from all over the world from the premier brands

Check Out Our Other Products
Universal Garbage collection log analysis tool. Tune and troubleshoot memory and GC problems.
Automatically captures & analyzes GC Logs, thread dumps, heap dumps & several more artifacts to identify root cause.
Machine learning algorithms aided tool to analyze the thread dumps, core dumps, and also hs_err_pid dumps.
Top Analyzer
Parses Unix/Linux/solaris,..etc 'top' command output & generates an intuitive report to optimize the performance.
Simulates performance problems like Memory Leak, OutOfMemoryError, CPU spike, StackOverflowError etc.
Frequently Asked Questions
Java GC Tuning is made to appear as rocket science, but it's a common sense!
How to capture Java heap dump?
Heap hero isn't able to parse my heap dumps, how to generate an android heap dump, can the tool parse both java and android heap dumps, can i look at generated heap dump reports, can i install this tool locally.
If there are questions that you are looking for, please contact us at [email protected]
Want to try HeapHero?
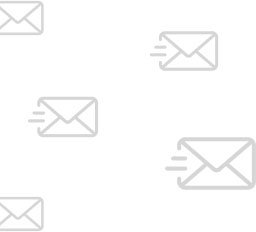
Subscribe to our newsletter
Congratulations.
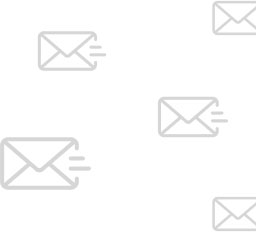
Acquiring Heap Dumps
HPROF Binary Heap Dumps
Get Heap Dump on an OutOfMemoryError
One can get a HPROF binary heap dump on an OutOfMemoryError for Sun JVM (1.4.2_12 or higher and 1.5.0_07 or higher), Oracle JVMs, OpenJDK JVMs, HP-UX JVM (1.4.2_11 or higher) and SAP JVM (since 1.5.0) by setting the following JVM parameter:
-XX:+HeapDumpOnOutOfMemoryError
By default, the heap dump is written to the work directory. This can be controlled using the following option to specify a directory or file name. -XX:HeapDumpPath=/dumpPath/ -XX:HeapDumpPath=./java_pidPIDNNN.hprof
Interactively Trigger a Heap Dump
To get heap dump on demand one can add the following parameter to the JVM and press CTRL + BREAK in the preferred moment:
-XX:+HeapDumpOnCtrlBreak
This is only available between Java 1.4.2 and Java 6.
HPROF agent
To use the HPROF agent to generate a dump on the end of execution, or on SIGQUIT signal use the following JVM parameter:
-agentlib:hprof=heap=dump,format=b
This was removed in Java 9 and later.
Alternatively, other tools can be used to acquire a heap dump:
- jmap -dump:format=b,file= <filename.hprof> <pid>
- jcmd <pid> GC.heap_dump <filename.hprof>
- JConsole (see sample usage in Basic Tutorial )
- JVisualVM This was available with a Java 7 or Java 8, but is now available from a separate download site .
- Memory Analyzer (see bottom of page )
System Dumps and Heap Dumps from IBM Virtual Machines
- All known formats
- HPROF binary heap dumps
- IBM 1.4.2 SDFF 1
- IBM Javadumps
- IBM SDK for Java (J9) system dumps
- IBM SDK for Java Portable Heap Dumps
Older versions of IBM Java (e.g. < 5.0SR12, < 6.0SR9) require running jextract on the operating system core dump which produced a zip file that contained the core dump, XML or SDFF file, and shared libraries. The IBM DTFJ feature still supports reading these jextracted zips although IBM DTFJ feature version 1.12.29003.201808011034 and later cannot read IBM Java 1.4.2 SDFF files, so MAT cannot read them either. Dumps from newer versions of IBM Java do not require jextract for use in MAT since DTFJ is able to directly read each supported operating system's core dump format. Simply ensure that the operating system core dump file ends with the .dmp suffix for visibility in the MAT Open Heap Dump selection. It is also common to zip core dumps because they are so large and compress very well. If a core dump is compressed with .zip , the IBM DTFJ feature in MAT is able to decompress the ZIP file and read the core from inside (just like a jextracted zip). The only significant downsides to system dumps over PHDs is that they are much larger, they usually take longer to produce, they may be useless if they are manually taken in the middle of an exclusive event that manipulates the underlying Java heap such as a garbage collection, and they sometimes require operating system configuration ( Linux , AIX ) to ensure non-truncation.
In recent versions of IBM Java (> 6.0.1), by default, when an OutOfMemoryError is thrown, IBM Java produces a system dump, PHD, javacore, and Snap file on the first occurrence for that process (although often the core dump is suppressed by the default 0 core ulimit on operating systems such as Linux). For the next three occurrences, it produces only a PHD, javacore, and Snap. If you only plan to use system dumps, and you've configured your operating system correctly as per the links above (particularly core and file ulimits), then you may disable PHD generation with -Xdump:heap:none . For versions of IBM Java older than 6.0.1, you may switch from PHDs to system dumps using -Xdump:system:events=systhrow,filter=java/lang/OutOfMemoryError,request=exclusive+prepwalk -Xdump:heap:none
In addition to an OutOfMemoryError, system dumps may be produced using operating system tools (e.g. gcore in gdb for Linux, gencore for AIX, Task Manager for Windows, SVCDUMP for z/OS, etc.), using the IBM and OpenJ9 Java APIs , using the various options of -Xdump , using Java Surgery , and more.
Versions of IBM Java older than IBM JDK 1.4.2 SR12, 5.0 SR8a and 6.0 SR2 are known to produce inaccurate GC root information.
Acquire Heap Dump from Memory Analyzer
If the Java process from which the heap dump is to be acquired is on the same machine as the Memory Analyzer, it is possible to acquire a heap dump directly from the Memory Analyzer. Dumps acquired this way are directly parsed and opened in the tool.
Acquiring the heap dump is VM specific. Memory Analyzer comes with several so called heap dump providers - for OpenJDK, Oracle and Sun based VMs (needs a OpenJDK, Oracle or Sun JDK with jmap) and for IBM VMs (needs an IBM JDK or JRE). Also extension points are provided for adopters to plug-in their own heap dump providers.
To trigger a heap dump from Memory Analyzer open the File > Acquire Heap Dump... menu item. Try Acquire Heap Dump now.
Depending on the concrete execution environment the pre-installed heap dump providers may work with their default settings and in this case a list of running Java processes should appear: To make selection easier, the order of the Java processes can be altered by clicking on the column titles for pid or Heap Dump Provider .
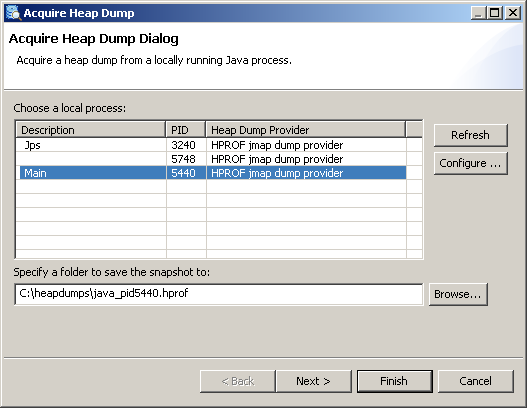
One can now select from which process a heap dump should be acquired, provide a preferred location for the heap dump and press Finish to acquire the dump. Some of the heap dump providers may allow (or require) additional parameters (e.g. type of the heap dump) to be set. This can be done by using Next button to get to the Heap Dump Provider Arguments page of the wizard.
Configuring the Heap Dump Providers
If the process list is empty try to configure the available heap dump providers. To do this press Configure... , select a matching provider from the list and click on it. You can see then what are the required settings and specify them. Next will then apply any changed settings, and refresh the JVM list if any settings have been changed. Prev will return to the current JVM list without applying any changed settings. To then apply the changed settings reenter and exit the Configure Heap Dump Providers... page as follows: Configure... > Next
If a process is selected before pressing Configure... then the corresponding dump provider will be selected on entering the Configure Heap Dump Providers... page.
If a path to a jcmd executable is provided then this command will be used to generate a list of running JVMs and to generate the dumps.
System dumps can be processed using jextract which compressed the dump and also adds extra system information so that the dump could be moved to another machine.
Portable Heap Dump (PHD) files generated with the Heap option can be compressed using the gzip compressor to reduce the file size.
HPROF files can be compressed using the Gzip compressor to reduce the file size. A compressed file may take longer to parse in Memory Analyzer, and running queries and reports and reading fields from objects may take longer.
Multiple snapshots in one heap dump
Memory Analyzer 1.2 and earlier handled this situation by choosing the first heap dump snapshot found unless another was selected via an environment variable or MAT DTFJ configuration option.
Memory Analyzer 1.3 handles this situation by detecting the multiple dumps, then presenting a dialog for the user to select the required snapshot.
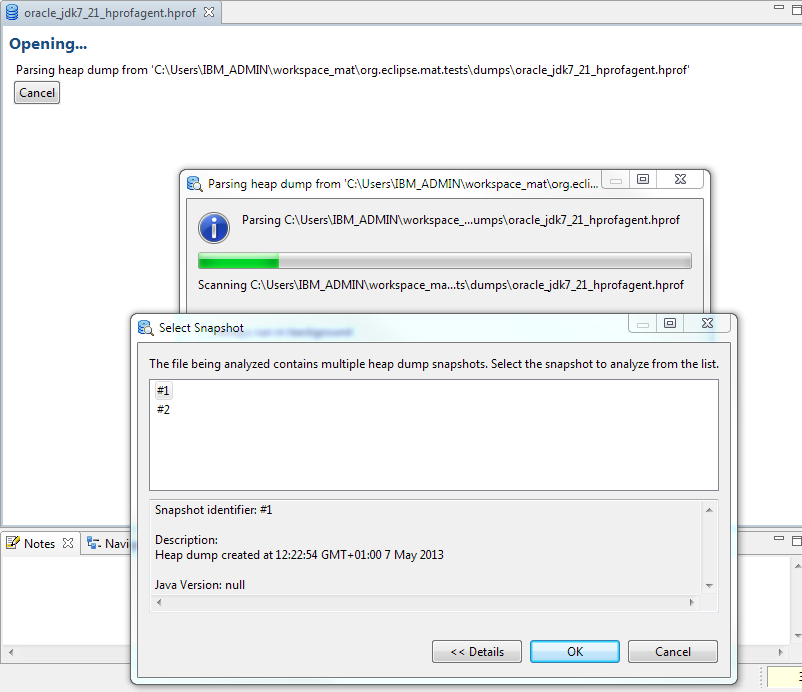
The index files generated have a component in the file name from the snapshot identifier, so the index files from each snapshot can be distinguished. This means that multiple snapshots from one heap dump file can be examined in Memory Analyzer simultaneously. The heap dump history for the file remembers the last snapshot selected for that file, though when the snapshot is reopened via the history the index file is also shown in the history. To open another snapshot in the dump, close the first snapshot, then reopen the heap dump file using the File menu and another snapshot can be chosen to be parsed. The first snapshot can then be reopened using the index file in the history, and both snapshots can be viewed at once.
The following table shows the availability of VM options and tools on the various platforms:
- Java Software
- Java SE Downloads
- Java SE 8 Documentation
Java VisualVM - Browsing a Heap Dump
You can use Java VisualVM to browse the contents of a heap dump file and quickly see the allocated objects in the heap. Heap dumps are displayed in the heap dump sub-tab in the main window. You can open binary format heap dump files ( .hprof ) saved on your local system or use Java VisualVM to take heap dumps of running applications.
A heap dump is a snapshot of all the objects in the Java Virtual Machine (JVM) heap at a certain point in time. The JVM software allocates memory for objects from the heap for all class instances and arrays. The garbage collector reclaims the heap memory when an object is no longer needed and there are no references to the object. By examining the heap you can locate where objects are created and find the references to those objects in the source. If the JVM software is failing to remove unneeded objects from the heap, Java VisualVM can help you locate the nearest garbage collecting root for the object.
Opening a Heap Dump File
If you have a heap dump file saved on your local system, you can open the file in Java VisualVM by choosing File > Load from the main menu. Java VisualVM can open heap dumps saved in the .hprof file format. When you open a saved heap dump, the heap dump opens as a tab in the main window.
Taking a Heap Dump
You can use Java VisualVM to take a heap dump of a local running application. When you use Java VisualVM to take a heap dump, the file is only temporary until you explicitly save it. If you do not save the file, the file will be deleted when the application terminates.
You can take a heap dump by doing either of the following:
- Right-click the application in the Applications window and choose Heap Dump.
- Click Heap Dump in the Monitor tab of the application.
Heap dumps for a local application open as sub-tabs in the application's tab. The heap dump also appears as a time-stamped heap dump node under the application node in the Applications window. To save a heap dump to your local system, right-click the heap dump in the Applications window and choose Save As.
Browsing a Heap Dump
Java VisualVM enables you to visually browse heap dumps in the following views:
Summary View
When you open a heap dump, Java VisualVM displays the Summary view by default. The Summary view displays the running environment where the heap dump was taken and other system properties.

Classes View
The Classes view displays a list of classes and the number and percentage of instances referenced by that class. You can view a list of the instances of a specific class by right-clicking the name and choosing Show in Instances View.
You can sort how results are displayed by clicking a column heading. You can use the filter below the list to filter the classes by name or limit the displayed results to sub-classes of a class by right-clicking a class name and choosing Show Only Subclasses.

Instances View
The Instance view displays object instances for a selected class. When you select an instance from the Instance pane, Java VisualVM displays the fields of that class and references to that class in the respective panes. In the References pane, you can right-click an item and choose Show Nearest GC Root to display the nearest garbage collection root object.


Memory Analyzer – Standalone
Eclipse memory analyzer – standalone installation.
In this tutorial let’s see how to
- Download and start working on Eclipse Memory Analyzer – Standalone version.
- Open a java heap dump created out of sun/oracle jdk (*.hprof) and a heap dump created out of IBM jdk (*.phd) files.
Search for “eclipse memory analyzer” and download “Windows (x86_64)” version (if the windows machine has 64 bit jdk) from https://eclipse.org/mat/downloads.php

Save file and unzip it.

Launch MemoryAnalyzer.exe

If the default java version is 1.7 or greater, MemoryAnalyzer will start without any issues.

Now, we are all set to open a heap dump (*.hprof) generated out of sun/oracle jdk. But before opening lets increase the Max Java heap size argument in “MemoryAnalyzer.ini”. (If needed).
-vmargs -Xmx1024m
Navigate to File -> Open Heap Dump . Select the hprof file.

Once we select the hprof file, it may take 15-20 minutes depending on the heap dump size and CPU of the local machine, to complete analyzing and open the report as shown below.

To Open a IBM JVM Heap dump – (Portable Heap Dump (phd) format)
IBM heap dumps are generated in *.phd file format. To open *.phd heap dumps, we need to install IBM Diagnostic tool framework for java (dtfj), from the below URL.
http://public.dhe.ibm.com/ibmdl/export/pub/software/websphere/runtimes/tools/dtfj/
In Eclipse Memory Analyzer Window, Navigate to Help -> Install New Software and provide the dtfj url and press Enter.

Click Next twice, Accept the terms of the license agreements and then click Finish. IBM diagnostic tool framework will start installing. This may take 5-10 minutes. Once the installation is completed, press “Yes” to restart eclipse.

Once eclipse is restarted, we can now see *.phd files under known formats. To check this, navigate to File -> Open Heap Dump. Select the phd file.

Now the phd file will be loaded and analyzed. This step may take 15-20 minutes depending on the heap dump size.

Some general errors we may face during the initial use and solutions for them are provided below.
The above errors occur when Memory analyser was invoked with java 1.6. They disappear when Java 1.7 is used.
Heap Space.
Sometimes while parsing heap dumps, it fails in-between with the error heap space.
In such scenarios, increase the Xmx value in MemoryAnalyzer.ini and try.
Share this:
Select Your Language
- Single-page
Language and Page Formatting Options
Chapter 3. diagnosing performance issues, 3.1. enabling garbage collection logging.
Examining garbage collection logs can be useful when attempting to troubleshoot Java performance issues, especially those related to memory usage.
Other than some additional disk I/O activity for writing the log files, enabling garbage collection logging does not significantly affect server performance.
Garbage collection logging is already enabled by default for a standalone JBoss EAP server running on OpenJDK or Oracle JDK. For a JBoss EAP managed domain, garbage collection logging can be enabled for the host controller, process controller, or individual JBoss EAP servers.
Get the correct JVM options for enabling garbage collection logging for your JDK. Replace the path in the options below to where you want the log to be created.
The Red Hat Customer Portal has a JVM Options Configuration Tool that can help you generate optimal JVM settings.
For OpenJDK or Oracle JDK:
For IBM JDK:
Apply the garbage collection JVM options to your JBoss EAP server.
See the JBoss EAP Configuration Guide for instructions on how to apply JVM options to a standalone server or servers in a managed domain .
3.2. Java Heap Dumps
A Java heap dump is a snapshot of a JVM heap created at a certain point in time. Creating and analyzing heap dumps can be useful for diagnosing and troubleshooting issues with Java applications.
Depending on which JDK you are using, there are different ways of creating and analyzing a Java heap dump for a JBoss EAP process. This section covers common methods for Oracle JDK, OpenJDK, and IBM JDK.
3.2.1. Creating a Heap Dump
3.2.1.1. openjdk and oracle jdk.
Create an On-Demand Heap Dump
You can use the jcmd command to create an on-demand heap dump for JBoss EAP running on OpenJDK or Oracle JDK.
- Determine the process ID of the JVM that you want to create a heap dump from.
Create the heap dump with the following command:
This creates a heap dump file in the HPROF format, usually located in EAP_HOME or EAP_HOME /bin . Alternatively, you can specify a file path to another directory.
Create a Heap Dump Automatically on OutOfMemoryError
You can use the -XX:+HeapDumpOnOutOfMemoryError JVM option to automatically create a heap dump when an OutOfMemoryError exception is thrown.
This creates a heap dump file in the HPROF format, usually located in EAP_HOME or EAP_HOME /bin . Alternatively, you can set a custom path for the heap dump using -XX:HeapDumpPath= /path/ . If you specify a file name using -XX:HeapDumpPath , for example, -XX:HeapDumpPath= /path/filename.hprof , the heap dumps will overwrite each other.
3.2.1.2. IBM JDK
When using the IBM JDK, heap dumps are automatically generated when an OutOfMemoryError is thrown.
Heap dumps from the IBM JDK are saved in the /tmp/ directory as a portable heap dump (PHD) formatted file.
3.2.2. Analyzing a Heap Dump
Heap Dump Analysis Tools
There are many tools that can analyze heap dump files and help identify issues. Red Hat Support recommends using the Eclipse Memory Analyzer tool (MAT) , which can analyze heap dumps formatted in either HPROF or PHD formats.
For information on using Eclipse MAT, see the Eclipse MAT documentation .
Heap Dump Analysis Tips
Sometimes the cause of the heap performance issues are obvious, but other times you may need an understanding of your application’s code and the specific circumstances that cause issues like an OutOfMemoryError . This can help to identify whether an issue is a memory leak, or if the heap is just not large enough.
Some suggestions for identifying memory usage issues include:
- If a single object is not found to be consuming too much memory, try grouping by class to see if many small objects are consuming a lot of memory.
- Check if the biggest usage of memory is a thread. A good indicator of this is if the OutOfMemoryError -triggered heap dump is much smaller than the specified Xmx maximum heap size.
- A technique to make memory leaks more detectable is to temporarily double the normal maximum heap size. When an OutOfMemoryError occurs, the size of the objects related to the memory leak will be about half the size of the heap.
When the source of a memory issue is identified, you can view the paths from garbage collection roots to see what is keeping the objects alive.
3.3. Identifying High CPU Utilization by Java Threads
For customers using JBoss EAP on Red Hat Enterprise Linux or Solaris, the JVMPeg lab tool on the Red Hat Customer Portal helps collect and analyze Java thread information to identify high CPU utilization. Follow the instructions for using the JVMPeg lab tool instead of using the following procedure.
For OpenJDK and Oracle JDK environments, Java thread diagnostic information is available using the jstack utility.
Identify the process ID of the Java process that is utilizing a high percentage of the CPU.
It can also be useful to obtain per-thread CPU data on high-usage processes. This can be done using the top -H command on Red Hat Enterprise Linux systems.
Using the jstack utility, create a stack dump of the Java process. For example, on Linux and Solaris:
You might need to create multiple dumps at intervals to see any changes or trends over a period of time.
- Analyze the stack dumps. You can use a tool such as the Thread Dump Analyzer (TDA) .
Quick Links
- Subscriptions
- Support Cases
- Customer Service
- Product Documentation
- Contact Customer Portal
- Customer Portal FAQ
- Log-in Assistance
- Trust Red Hat
- Browser Support Policy
- Accessibility
- Awards and Recognition
Related Sites
- developers.redhat.com
- connect.redhat.com
- cloud.redhat.com
Systems Status
- Red Hat Subscription Value
- About Red Hat
- Red Hat Jobs
Red Hat legal and privacy links
- Contact Red Hat
- Red Hat Blog
- Diversity, equity, and inclusion
- Cool Stuff Store
- Red Hat Summit
- Privacy statement
- Terms of use
- All policies and guidelines
- Digital accessibility
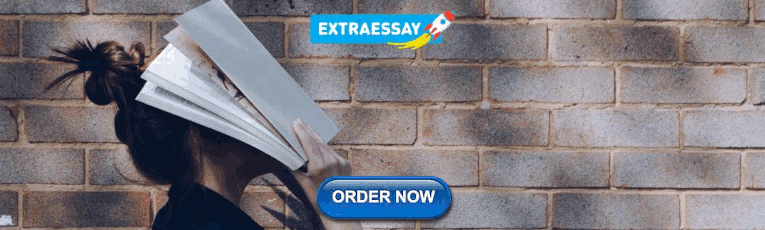
IMAGES
VIDEO
COMMENTS
According to this question, it is necessary to install DTJF on Eclipse Memory Analyzer. This link in the question says: Memory Analyzer can also read memory-related information from IBM system dumps and from Portable Heap Dump (PHD) files. For this purpose one just has to install the IBM DTFJ feature into Memory Analyzer version 0.8 or later.
the Portable Heap Dump (PHD) format. PHD is the default format. The classic format is human-readable since it is in ASCII text, but the PHD format is binary and should be processed by appropriate tools for analysis. ... We will first start the Memory Analyzer Tool and open the heap dump file. In Eclipse MAT, two types of object sizes are ...
In the list below, an item should appear called IBM Monitoring and Diagnostic Tools. Tick the box next to it, click Next, and follow the wizard to accept the license agreements and install the toolkit. Restart Eclipse when prompted. Choose File -> Open Heap Dump and choose your .phd file. It should open in MAT and allow you to figure out who is ...
In the list below, an item should appear called IBM Monitoring and Diagnostic Tools. Tick the box next to it, click Next, and follow the wizard to accept the license agreements and install the toolkit. Restart Eclipse when prompted. Choose File -> Open Heap Dump and choose your .phd file. It should open in MAT and allow you to figure out who is ...
And the screenshots posted below are from the MAT plugin used with Eclipse IDE. The steps to load the heap dump are as follows. Open Eclipse IDE or the standalone MAT Tool. From the toolbar, Select Files > Open File from the dropdown menu. Open the heap dump file with the extension .hprof and you should see the overview page as shown below.
General structure. The following structure comprises the header section of a PHD file: A UTF string indicating that the file is a portable heap dump; An int containing the PHD version number; An int containing flags:. 1 indicates that the word length is 64-bit.; 2 indicates that all the objects in the dump are hashed. This flag is set for heap dumps that use 16-bit hash codes.
General structure. The following structure comprises the header section of a PHD file: A UTF string indicating that the file is a portable heap dump; An int containing the PHD version number; An int containing flags:. 1 indicates that the word length is 64-bit.; 2 indicates that all the objects in the dump are hashed. This flag is set for heap dumps that use 16-bit hash codes.
System Dumps and Heap Dumps from IBM Virtual Machines. Memory Analyzer may read memory-related information from IBM system dumps and from Portable Heap Dump (PHD) files with the IBM DTFJ feature installed. Once installed, then File > Open Heap Dump should give the following options for the file types: . All known formats
Eclipse Memory Analyzer can download from eclipse.org. Unzip the downloaded file and double click "MemoryAnalyzer" to start the application. Execute a Java program which is continuously running ...
The Eclipse Memory Analyzer is a fast and feature-rich Java heap analyzer that helps you find memory leaks and reduce memory consumption.. Use the Memory Analyzer to analyze productive heap dumps with hundreds of millions of objects, quickly calculate the retained sizes of objects, see who is preventing the Garbage Collector from collecting objects, run a report to automatically extract leak ...
Heap dumps are usually stored in binary format hprof files. We can open and analyze these files using tools like jhat or JVisualVM. Also, for Eclipse users, it's very common to use MAT. In the next sections, we'll go through multiple tools and approaches to generate a heap dump, and we'll show the main differences between them. 2. JDK Tools
How to capture Java heap dump? There are 7 options to capture heap dumps. jmap tool under <JAVA_HOME>\bin folder is an effective option. It can be invoked using the command: jmap -dump:format=b,file=<heap-dump-file-path> <process-id>.
Acquire Heap Dump from Memory Analyzer. If the Java process from which the heap dump is to be acquired is on the same machine as the Memory Analyzer, it is possible to acquire a heap dump directly from the Memory Analyzer. ... Portable Heap Dump (PHD) files generated with the Heap option can be compressed using the gzip compressor to reduce the ...
You can use Java VisualVM to browse the contents of a heap dump file and quickly see the allocated objects in the heap. Heap dumps are displayed in the heap dump sub-tab in the main window. You can open binary format heap dump files ( .hprof) saved on your local system or use Java VisualVM to take heap dumps of running applications.
Eclipse Memory Analyzer - Standalone Installation In this tutorial let's see how to Download and start working on Eclipse Memory Analyzer - Standalone version. Open a java heap dump created out of sun/oracle jdk (*.hprof) and a heap dump created out of IBM jdk (*.phd) files. Step 1: Search for "eclipse memory analyzer" and download…
Gather all the .phd files and transfer them to your problem determination machine for analysis.; Many tools are available to analyze heap dumps that include Rational® Application Developer 6.0. WebSphere Application Server serviceability released a technology preview called Memory Dump Diagnostic For Java™.
A dump is data queried from a storage medium and stored somewhere for further analysis.The Java Virtual Machine (JVM) helps to manage memory in Java, and in the case of errors, we can get a dump file from the JVM to diagnose errors.. In this tutorial, we'll explore three common Java dump files - heap dump, thread dump, and core dump - and understand their use cases.
Heap dumps from the IBM JDK are saved in the /tmp/ directory as a portable heap dump (PHD) formatted file. 3.2.2. Analyzing a Heap Dump. Heap Dump Analysis Tools. There are many tools that can analyze heap dump files and help identify issues. Red Hat Support recommends using the Eclipse Memory Analyzer tool ...
Summary. IBM HeapAnalyzer is a graphical tool for discovering possible Java heap leaks. Steps. Note: IBM HeapAnalyzer has no new development and therefore, in general, we recommend using the Eclipse Memory Analyzer Tool (MAT)instead which is open source, has active development, and has a similar feature set (leak suspects, etc.). Download.
If you want to analyze heap dump using ParseHeapDump.sh script then you need to pass the name of the report you want to generate. For example, if you want to see "Leak Suspect Report" then you can run it like following: ParseHeapDump.sh path/to/dump.hprof org.eclipse.mat.api:suspects It will then create a .zip file with dump's name.
2. This happens due to that the default heap size is smaller than needed by the dump size to be loaded, to resolve this, you need to set the VM args Xms, and XmX with the right values, below is what worked for me: "<JAVA_PATH>\Java.exe" -Xms256m -Xmx6144m -jar <HEAP_ANALYSER_NAME>.jar.