- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
- C Exercises - Practice Questions with Solutions for C Programming
- C Programming Interview Questions (2024)
- C | Storage Classes and Type Qualifiers | Question 7
- C | Storage Classes and Type Qualifiers | Question 3
- C | Storage Classes and Type Qualifiers | Question 8
- Top 25 C Projects with Source Code in 2023
- C | Storage Classes and Type Qualifiers | Question 19
- C++ Exercises - C++ Practice Set with Solutions
- Top | MCQs on Dynamic Programming with Answers | Question 19
- C++ Programming Multiple Choice Questions
- Data Structures & C Programming - GATE CSE Previous Year Questions
- Class 8 NCERT Solutions - Chapter 16 Playing with Numbers - Exercise 16.1
- QA - Placement Quizzes | SP Contest 2 | Question 9
- Monotype Solutions Interview Experience
- QA - Placement Quizzes | SP Contest 2 | Question 3
- GATE | Quiz for Sudo GATE 2021 | Question 18
- QA - Placement Quizzes | SP Contest 2 | Question 8
- Mentor Graphics Interview Experience| Set 6 (On-Campus for Freshers)
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Bitwise Operators in C
- std::sort() in C++ STL
- What is Memory Leak? How can we avoid?
- Substring in C++
- Segmentation Fault in C/C++
- Socket Programming in C
- For Versus While
- Data Types in C
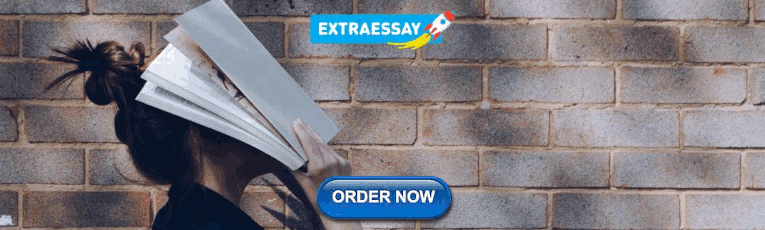
C Exercises – Practice Questions with Solutions for C Programming
The best way to learn C programming language is by hands-on practice. This C Exercise page contains the top 30 C exercise questions with solutions that are designed for both beginners and advanced programmers. It covers all major concepts like arrays, pointers, for-loop, and many more.
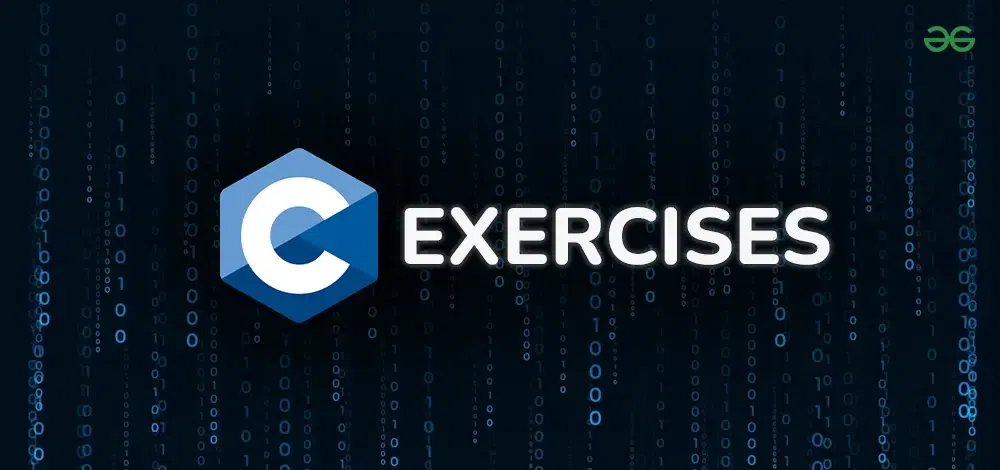
So, Keep it Up! Solve topic-wise C exercise questions to strengthen your weak topics.
C Programming Exercises
The following are the top 30 programming exercises with solutions to help you practice online and improve your coding efficiency in the C language. You can solve these questions online in GeeksforGeeks IDE.
Q1: Write a Program to Print “Hello World!” on the Console.
In this problem, you have to write a simple program that prints “Hello World!” on the console screen.
For Example,
Click here to view the solution.
Q2: write a program to find the sum of two numbers entered by the user..
In this problem, you have to write a program that adds two numbers and prints their sum on the console screen.
Q3: Write a Program to find the size of int, float, double, and char.
In this problem, you have to write a program to print the size of the variable.
Q4: Write a Program to Swap the values of two variables.
In this problem, you have to write a program that swaps the values of two variables that are entered by the user.
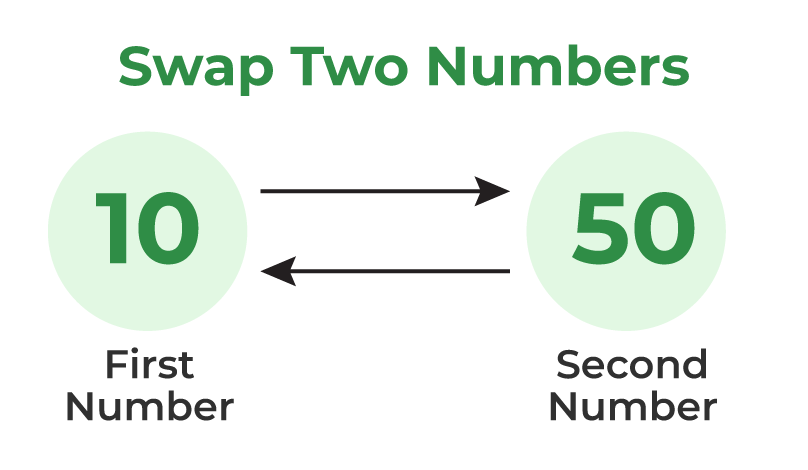
Swap two numbers
Q5: Write a Program to calculate Compound Interest.
In this problem, you have to write a program that takes principal, time, and rate as user input and calculates the compound interest.
Q6: Write a Program to check if the given number is Even or Odd.
In this problem, you have to write a program to check whether the given number is even or odd.
Q7: Write a Program to find the largest number among three numbers.
In this problem, you have to write a program to take three numbers from the user as input and print the largest number among them.
Q8: Write a Program to make a simple calculator.
In this problem, you have to write a program to make a simple calculator that accepts two operands and an operator to perform the calculation and prints the result.
Q9: Write a Program to find the factorial of a given number.
In this problem, you have to write a program to calculate the factorial (product of all the natural numbers less than or equal to the given number n) of a number entered by the user.
Q10: Write a Program to Convert Binary to Decimal.
In this problem, you have to write a program to convert the given binary number entered by the user into an equivalent decimal number.
Q11: Write a Program to print the Fibonacci series using recursion.
In this problem, you have to write a program to print the Fibonacci series(the sequence where each number is the sum of the previous two numbers of the sequence) till the number entered by the user using recursion.
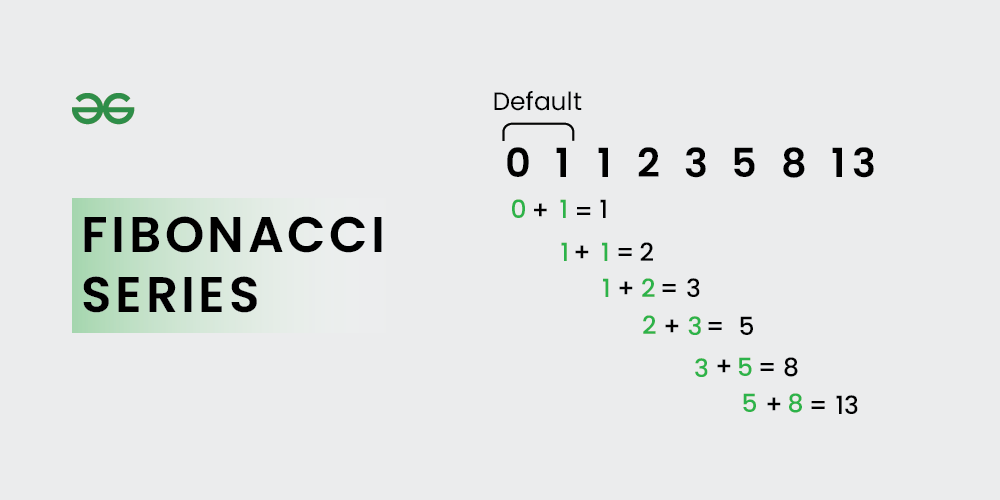
Fibonacci Series
Q12: Write a Program to Calculate the Sum of Natural Numbers using recursion.
In this problem, you have to write a program to calculate the sum of natural numbers up to a given number n.
Q13: Write a Program to find the maximum and minimum of an Array.
In this problem, you have to write a program to find the maximum and the minimum element of the array of size N given by the user.
Q14: Write a Program to Reverse an Array.
In this problem, you have to write a program to reverse an array of size n entered by the user. Reversing an array means changing the order of elements so that the first element becomes the last element and the second element becomes the second last element and so on.
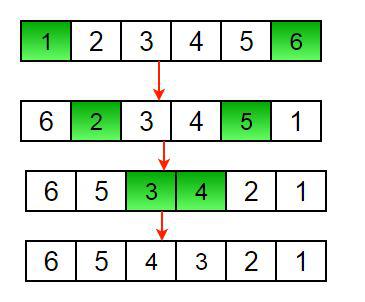
Reverse an array
Q15: Write a Program to rotate the array to the left.
In this problem, you have to write a program that takes an array arr[] of size N from the user and rotates the array to the left (counter-clockwise direction) by D steps, where D is a positive integer.
Q16: Write a Program to remove duplicates from the Sorted array.
In this problem, you have to write a program that takes a sorted array arr[] of size N from the user and removes the duplicate elements from the array.
Q17: Write a Program to search elements in an array (using Binary Search).
In this problem, you have to write a program that takes an array arr[] of size N and a target value to be searched by the user. Search the target value using binary search if the target value is found print its index else print ‘element is not present in array ‘.
Q18: Write a Program to reverse a linked list.
In this problem, you have to write a program that takes a pointer to the head node of a linked list, you have to reverse the linked list and print the reversed linked list.
Q18: Write a Program to create a dynamic array in C.
In this problem, you have to write a program to create an array of size n dynamically then take n elements of an array one by one by the user. Print the array elements.
Q19: Write a Program to find the Transpose of a Matrix.
In this problem, you have to write a program to find the transpose of a matrix for a given matrix A with dimensions m x n and print the transposed matrix. The transpose of a matrix is formed by interchanging its rows with columns.
Q20: Write a Program to concatenate two strings.
In this problem, you have to write a program to read two strings str1 and str2 entered by the user and concatenate these two strings. Print the concatenated string.
Q21: Write a Program to check if the given string is a palindrome string or not.
In this problem, you have to write a program to read a string str entered by the user and check whether the string is palindrome or not. If the str is palindrome print ‘str is a palindrome’ else print ‘str is not a palindrome’. A string is said to be palindrome if the reverse of the string is the same as the string.
Q22: Write a program to print the first letter of each word.
In this problem, you have to write a simple program to read a string str entered by the user and print the first letter of each word in a string.
Q23: Write a program to reverse a string using recursion
In this problem, you have to write a program to read a string str entered by the user, and reverse that string means changing the order of characters in the string so that the last character becomes the first character of the string using recursion.
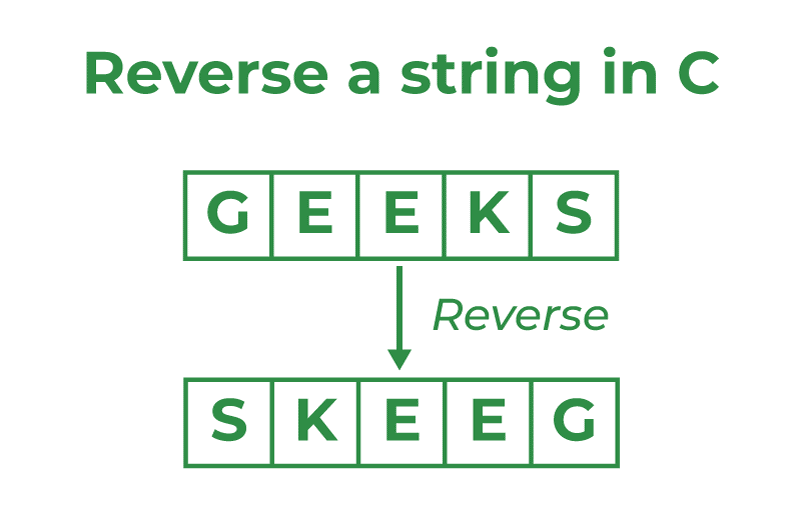
reverse a string
Q24: Write a program to Print Half half-pyramid pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print the half-pyramid pattern of numbers. Half pyramid pattern looks like a right-angle triangle of numbers having a hypotenuse on the right side.
Q25: Write a program to print Pascal’s triangle pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print Pascal’s triangle pattern. Pascal’s Triangle is a pattern in which the first row has a single number 1 all rows begin and end with the number 1. The numbers in between are obtained by adding the two numbers directly above them in the previous row.
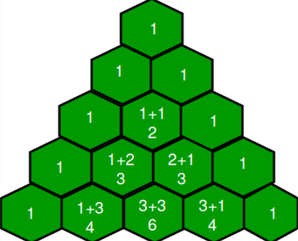
Pascal’s Triangle
Q26: Write a program to sort an array using Insertion Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending or descending order using insertion sort.
Q27: Write a program to sort an array using Quick Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending order using quick sort.
Q28: Write a program to sort an array of strings.
In this problem, you have to write a program that reads an array of strings in which all characters are of the same case entered by the user and sort them alphabetically.
Q29: Write a program to copy the contents of one file to another file.
In this problem, you have to write a program that takes user input to enter the filenames for reading and writing. Read the contents of one file and copy the content to another file. If the file specified for reading does not exist or cannot be opened, display an error message “Cannot open file: file_name” and terminate the program else print “Content copied to file_name”
Q30: Write a program to store information on students using structure.
In this problem, you have to write a program that stores information about students using structure. The program should create various structures, each representing a student’s record. Initialize the records with sample data having data members’ Names, Roll Numbers, Ages, and Total Marks. Print the information for each student.
We hope after completing these C exercises you have gained a better understanding of C concepts. Learning C language is made easier with this exercise sheet as it helps you practice all major C concepts. Solving these C exercise questions will take you a step closer to becoming a C programmer.
Frequently Asked Questions (FAQs)
Q1. what are some common mistakes to avoid while doing c programming exercises.
Some of the most common mistakes made by beginners doing C programming exercises can include missing semicolons, bad logic loops, uninitialized pointers, and forgotten memory frees etc.
Q2. What are the best practices for beginners starting with C programming exercises?
Best practices for beginners starting with C programming exercises: Start with easy codes Practice consistently Be creative Think before you code Learn from mistakes Repeat!
Q3. How do I debug common errors in C programming exercises?
You can use the following methods to debug a code in C programming exercises Read the error message carefully Read code line by line Try isolating the error code Look for Missing elements, loops, pointers, etc Check error online
Please Login to comment...
Similar reads.

Improve your Coding Skills with Practice
What kind of Experience do you want to share?
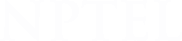
- Announcements
- Explore Courses
Problem solving through Programming In C
- BE/BTech in all disciplines
- BCA/MCA/M. Sc
- All IT Industries
65741 students have enrolled already!!
In association with

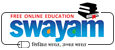
- Review Assignment
- Announcements
- About the Course
- Explore Courses
Problem Solving Through Programming In C - Unproctored Exam Test - Session 1
Dear Students, The questions for the Unproctored Programming Exam Test are available now in the course page. Please fine the questions under the course outline: Programming Exam 2022 (First Session) (10am - 11am). You can access the questions in the below link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=189 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=190 Best of Luck! -NPTEL Team
Problem Solving Through Programming In C - Unproctored Exam Test - Session 2
Dear Students, The questions for the Unproctored Programming Exam Test are available now in the course page. Please fine the questions under the course outline: Programming Exam 2022 (Second Session) (8pm - 9pm). You can access the questions in the below link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=191 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=192 Best of Luck! -NPTEL Team
Reminder 4 : Problem solving through Programming In C: Online Programming test (Jan 2022 Semester)
Dear Candidate Note: If you are registering and taking exam in Jan-Apr 2022 exam , it is mandatory to take this Non-proctored exam as the previous semester Non-proctored programming exam score will not be considered. The criteria for certification of this course " Problem solving through Programming In C " is different because of the online programming exam component. Please read the following carefully. Final score = 25% of Assignment score + 50% of Online exam score (Proctored) + 25% of Programming exam (unproctored). - Unproctored means, candidates will be taking the exam from college/home without NPTEL team present for monitoring the test- with access to all resources. - The discussion forum will be disabled during the exam duration (during both sessions) and re-enabled thereafter. How to take the online programming test 1. Go to: https://onlinecourses.nptel.ac.in/noc21_cs01/ 2. Log in using the same email id which you have been accessing the course (i.e. using to watch videos & submit Assignments) 3. There will be sections under the course outline "Programming Test (April 11)" that will have the online programming exam link. 4. You can click on this link and attempt the programming exam. Date: April 11 , 2022 (Monday) First Session: 10.00AM - 11.00AM (Duration of the session will be 1 hr) Second Session: 8.00PM - 9.00PM (Duration of the session will be 1 hr ) Note - You can take any one or both sessions too; the best score will be considered towards the final certification score. - You can take the exam from your college or home. - The exam has to be taken in pc or laptop. DO NOT TRY TO TAKE THE EXAM USING YOUR MOBILE PHONE. - The discussion forum will be disabled during the exam duration (during both sessions) and re-enabled thereafter. - If you encounter any issues during the exam, please write to [email protected] IMPORTANT: CERTIFICATION CRITERIA Candidates have to come to the exam center and take the theory test on April 23,2022. It is mandatory to take the Proctored in-person final exam to obtain a certificate. To pass the course and get a certificate: Assignment score >= 40/100 AND Programming exam score >= 40/100 AND Proctored exam score >= 40/100 OR Assignment score >= 10/25 AND Programming exam score >= 10/25 AND Proctored exam score >= 20/50 All 3 conditions have to be satisfied. All the best to the candidates! -NPTEL Team.
Reminder 3 : Problem solving through Programming In C: Online Programming test (Jan 2022 Semester)
Reminder 2 : problem solving through programming in c: online programming test (jan 2022 semester), reminder 1 : problem solving through programming in c: online programming test (jan 2022 semester), problem solving through programming in c: online programming test (jan 2022 semester), nptel: exam registration is open now for jan 2022 courses.
Dear Candidate,
Here is a golden opportunity for those who had previously enrolled in this course during the Jan 2021 semester, but could not participate in the exams or were absent/did not pass the exam for this course. This course is being reoffered in Jan 2022 and we are giving you another chance to write the exam in April 2022 and obtain a certificate based on NPTEL norms. Do not let go of this unique opportunity to earn a certificate from the IITs/IISc.
IMPORTANT instructions for learners - Please read this carefully
1. The exam date for this course: April 23, 2022
2. Certification exam registration URL is: CLICK HERE
Please fill the exam form using the same Enrolled email id & make fee payment via the form, as before.
3. Choose from the Cities where exam will be conducted: Exam Cities
4. You DO NOT have to re-enroll in the courses.
5. You DO NOT have to resubmit Assignments OR participate in the non-proctored
programming exams.
6. If you do enroll to Jan 2022 course, we will take the best average assignment scores/non-proctored programming exam score across the two semesters
Our suggestion:
- Please check once if you have >= 40/100 in average assignment score and also participate in the non-proctored programming exams that will be conducted during this semester in the course to become eligible for the e-certificate, wherever applicable.
- If not, please submit Assignments again in the Jan 2022 course & and also participate in the non-proctored programming exams to become eligible for the e-certificate.
- You can also submit Assignments again and participate in the non-proctored programming exams if you want to better your previous scores.
RECOMMENDATION: Please enroll to the Jan 2022 course and brush up your lessons for the exam.
7. Exam fees:
If you register for the exam and pay before March 14, 2022, 10:00 AM, Exam fees will be Rs. 1000/- per exam .
If you register for exam before March 14, 2022, 10:00 AM and have not paid or if you register between March 14, 2022, 10:00 AM & March 18, 2022, 10:00 AM, Exam fees will be Rs. 1500/- per exam
8. 50% fee waiver for the following categories:
Students belonging to the SC/ST category: please select Yes for the SC/ST option and upload the correct Community certificate.
Students belonging to the PwD category with more than 40% disability: please select Yes for the option and upload the relevant Disability certificate.
9. Last date for exam registration: March 18, 2022 10:00 AM (Friday).
10. Mode of payment: Online payment - debit card/credit card/net banking.
11. HALL TICKET:
The hall ticket will be available for download tentatively by 2 weeks prior to the exam date . We will confirm the same through an announcement once it is published.
12. FOR CANDIDATES WHO WOULD LIKE TO WRITE MORE THAN 1 COURSE EXAM:- you can add or delete courses and pay separately – till the date when the exam form closes. Same day of exam – you can write exams for 2 courses in the 2 sessions. Same exam center will be allocated for both the sessions.
13. Data changes:
Last date for data changes: March 18, 2022 10:00 AM :
All the fields in the Exam form except for the following ones can be changed until the form closes.
The following 6 fields can be changed ONLY when there are NO courses in the course cart. And you will be able to edit the following fields only if you: -
REMOVE unpaid courses from the cart And/or - CANCEL paid courses
1. Do you come under the SC/ST category? *
2. SC/ST Proof
3. Are you a person with disabilities? *
4. Are you a person with disabilities above 40%?
5. Disabilities Proof
6. What is your role ?
Note: Once you remove or cancel a course, you will be able to edit these fields immediately.
But, for cancelled courses, refund of fees will be initiated only after 2 weeks.
14. LAST DATE FOR CANCELLING EXAMS and getting a refund: March 18, 2022 10:00 AM
15. Click here to view Timeline and Guideline : Guideline
Domain Certification
Domain Certification helps learners to gain expertise in a specific Area/Domain. This can be helpful for learners who wish to work in a particular area as part of their job or research or for those appearing for some competitive exam or becoming job ready or specialising in an area of study.
Every domain will comprise Core courses and Elective courses. Once a learner completes the requisite courses per the mentioned criteria, you will receive a Domain Certificate showcasing your scores and the domain of expertise. Kindly refer to the following link for the list of courses available under each domain: https://nptel.ac.in/noc/Domain/discipline.html
Outside India Candidates
Candidates who are residing outside India may also fill the exam form and pay the fees. Mode of exam and other details will be communicated to you separately.
Thanks & Regards,
Reminder 4: Problem solving through Programming In C: Online Programming test (July 2021 Semester)
Dear Candidate Note: If you are registering and taking exam in July-Dec 2021 exam , it is mandatory to take this Non-proctored exam as the previous semester Non-proctored programming exam score will not be considered. The criteria for certification of this course " Problem solving through Programming In C " is different because of the online programming exam component. Please read the following carefully. Final score = 25% of Assignment score + 50% of Online exam score (Proctored) + 25% of Programming exam (unproctored). - Unproctored means, candidates will be taking the exam from college/home without NPTEL team present for monitoring the test- with access to all resources. - The discussion forum will be disabled during the exam duration (during both sessions) and re-enabled thereafter. How to take the online programming test 1. Go to: https://onlinecourses.nptel.ac.in/noc21_cs01/ 2. Log in using the same email id which you have been accessing the course (i.e. using to watch videos & submit Assignments) 3. There will be sections under the course outline "Programming Test (October 17)" that will have the online programming exam link. 4. You can click on this link and attempt the programming exam. Date: October 17, 2021 (Sunday) First Session: 10.00AM - 11.00AM (Duration of the session will be 1 hr) Second Session: 8.00PM - 9.00PM (Duration of the session will be 1 hr ) Note - You can take any one or both sessions too; the best score will be considered towards the final certification score. - You can take the exam from your college or home. - The exam has to be taken in pc or laptop. DO NOT TRY TO TAKE THE EXAM USING YOUR MOBILE PHONE. - The discussion forum will be disabled during the exam duration (during both sessions) and re-enabled thereafter. - If you encounter any issues during the exam, please write to [email protected] IMPORTANT: CERTIFICATION CRITERIA Candidates have to come to the exam center and take the theory test on October 23,2021. It is mandatory to take the Proctored in-person final exam to obtain a certificate. To pass the course and get a certificate: Assignment score >= 40/100 AND Programming exam score >= 40/100 AND Proctored exam score >= 40/100 OR Assignment score >= 10/25 AND Programming exam score >= 10/25 AND Proctored exam score >= 20/50 All 3 conditions have to be satisfied. All the best to the candidates! -NPTEL Team.
Problem solving through Programming In C - Unproctored Exam Test - Session 2
Dear Students, The questions for the Unproctored Programming Exam Test are available now in the course page. Please fine the questions under the course outline Programming Exam Oct 2021 (Second Session) : (8.00PM - 9.00PM). You can access the questions in the below link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=185 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=184 Best of Luck! -NPTEL Team
Problem solving through Programming In C - Unproctored Exam Test - Session 1
Dear Students, The questions for the Unproctored Programming Exam Test are available now in the course page. Please fine the questions under the course outline Programming Exam Oct 2021 (First Session): (10.00AM - 11.00AM). You can access the questions in the below link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=181 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=183 Best of Luck! -NPTEL Team
Reminder 3: Problem solving through Programming In C: Online Programming test (July 2021 Semester)
Reminder 1: problem solving through programming in c: online programming test (july 2021 semester), reminder 2: problem solving through programming in c: online programming test (july 2021 semester), thank you for learning with nptel.
Dear Learner, Thank you for taking the course with NPTEL!! Hope you enjoyed the journey with us. The results for this course have been published and we are closing this course now. You will still have access to the contents and assignments of this course, if you click on the course name from the "Mycourses" tab on swayam.gov.in. The discussion forum is being closed though and you cannot ask questions here. For any further queries please write to [email protected] . - Team NPTEL
Problem solving through Programming In C: Result Published!
- Hard copies of certificates will not be dispatched.
- The duration shown in the certificate will be based on the timeline of offering of the course in 2021, irrespective of which Assignment score that will be considered.
Feedback for Problem solving through Programming In C
Dear student, We are glad that you have attended the NPTEL online certification course. We hope you found the NPTEL Online course useful and have started using NPTEL extensively. In this regard, we would like to have feedback from you regarding our course and whether there are any improvements, you would like to suggest. We are enclosing an online feedback form and would request you to spare some of your valuable time to input your observations. Your esteemed input will help us in serving you better. The link to give your feedback is: https://docs.google.com/forms/d/1DUKdDzK6b24hpJSBTnuurZSfFwDAy30TMwpNM1vIt48/viewform We thank you for your valuable time and feedback. Thanks & Regards, -NPTEL Team
Problem solving through Programming In C : Online Programming test (July 2021 Semester)
Problem solving through programming in c: open now for exam registration july 2021.
Dear Candidate, Here is a golden opportunity for those who had previously enrolled in this course during the Jan 2021 semester, but could not participate in the exams or were absent/did not pass the exam for this course. This course is being reoffered in July 2021 and we are giving you another chance to write the exam in Sep/Oct 2021 and obtain a certificate based on NPTEL norms. Do not let go of this unique opportunity to earn a certificate from the IITs/IISc. IMPORTANT instructions for learners - Please read this carefully 1. The exam date for this course: October 23, 2021 2. Certification exam registration URL is: https://examform.nptel.ac.in/ Please fill the exam form using the same Enrolled email id & make fee payment via the form, as before. 3. Choose from the Cities where exam will be conducted: Exam Cities 4. You DO NOT have to re-enroll in the courses. 5. You DO NOT have to resubmit Assignments OR participate in the non-proctored programming exams. 6. If you do enroll to July 2021 course, we will take the best average assignment scores/non-proctored programming exam score across the two semesters Our suggestion: - Please check once if you have >= 40/100 in average assignment score and also participate in the non-proctored programming exams that will be conducted during this semester in the course to become eligible for the e-certificate, wherever applicable. - If not, please submit Assignments again in the July 2021 course & and also participate in the non-proctored programming exams to become eligible for the e-certificate. - You can also submit Assignments again and participate in the non-proctored programming exams if you want to better your previous scores. RECOMMENDATION: Please enroll to the July 2021 course and brush up your lessons for the exam. 7. Exam fees: If you register for the exam and pay before Sep 13, 2021, 10:00 AM , Exam fees will be Rs. 1000/- per exam . If you register for exam before Sep 13, 2021, 10:00 AM and have not paid or if you register between Sep 13, 2021, 10:00 AM & Sep 17, 2021, 5:00 PM , Exam fees will be Rs. 1500/- per exam 8. 50% fee waiver for the following categories: Students belonging to the SC/ST category: please select Yes for the SC/ST option and upload the correct Community certificate. Students belonging to the PwD category with more than 40% disability: please select Yes for the option and upload the relevant Disability certificate. 9. Last date for exam registration: Sep 17, 2021, 5:00 PM (Friday). 10. Mode of payment: Online payment - debit card/credit card/net banking. 11. HALL TICKET: The hall ticket will be available for download tentatively by 2 weeks prior to the exam date . We will confirm the same through an announcement once it is published. 12. FOR CANDIDATES WHO WOULD LIKE TO WRITE MORE THAN 1 COURSE EXAM:- you can add or delete courses and pay separately – till the date when the exam form closes. Same day of exam – you can write exams for 2 courses in the 2 sessions. Same exam center will be allocated for both the sessions. 13. Data changes: Last date for data changes: Sep 17, 2021, 5:00 PM: All the fields in the Exam form except for the following ones can be changed until the form closes. The following 6 fields can be changed ONLY when there are NO courses in the course cart. And you will be able to edit the following fields only if you: - REMOVE unpaid courses from the cart And/or - CANCEL paid courses 1. Do you come under the SC/ST category? * 2. SC/ST Proof 3. Are you a person with disabilities? * 4. Are you a person with disabilities above 40%? 5. Disabilities Proof 6. What is your role ? Note: Once you remove or cancel a course, you will be able to edit these fields immediately. But, for cancelled courses, refund of fees will be initiated only after 2 weeks. 14. LAST DATE FOR CANCELLING EXAMS and getting a refund: Sep 17, 2021, 5:00 PM 15. Click here to view Timeline and Guideline : Guideline Domain Certification Domain Certification helps learners to gain expertise in a specific Area/Domain. This can be helpful for learners who wish to work in a particular area as part of their job or research or for those appearing for some competitive exam or becoming job ready or specialising in an area of study. Every domain will comprise Core courses and Elective courses. Once a learner completes the requisite courses per the mentioned criteria, you will receive a Domain Certificate showcasing your scores and the domain of expertise. Kindly refer to the following link for the list of courses available under each domain: https://nptel.ac.in/noc/Domain/discipline.html Thanks & Regards, NPTEL TEAM
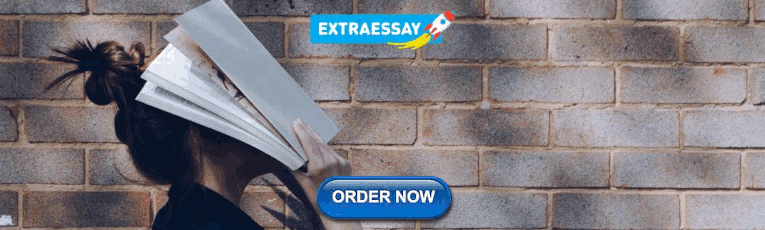
Problem solving through Programming In C - Session I - Program II Change of score
Those who have answered the program -II of Session-I correctly regardless of whichever printf statement ("Total Repeated elements" or "Total repeated elements" ) you have used may notice that their score is shown as 50% (for this particular program only). You do not need to worry about it. It will be treated as full marks (100%) while calculating the final score. This is mainly done to incorporate both the printf statement. Thanks
Dear Students, The questions for the Unproctored Programming Exam Test are available now in the course page. Please fine the questions under the course outline Programming Exam Jan 2021 (First Session): (10.00AM - 11.00AM). You can access the questions in the below link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=181 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=183 Best of Luck! -NPTEL Team
Dear Students, The questions for the Unproctored Programming Exam Test are available now in the course page. Please fine the questions under the course outline Programming Exam Jan 2021 (Second Session) : (8.00PM - 9.00PM). You can access the questions in the below link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=185 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=184 Best of Luck! -NPTEL Team
April 2021 NPTEL Exams have been postponed!
Dear learner Taking the current covid situation into consideration, the NPTEL exams scheduled to be conducted on 24/25 April stand postponed until further notice. We will keep you informed of the potential dates for the exams as the situation improves and we finalize the same. Thanks and Regards, NPTEL TEAM.
Reminder 2: Problem solving through Programming In C : Online Programming test (Jan 2021 Semester) - April 18,2021!!
Dear Learner Unproctored Programming exam for this course will be as per schedule. Date: April 18, 2021 (Sunday) First Session: 10.00AM - 11.00AM (Duration of the session will be 1 hr) Second Session: 8.00PM - 9.00PM (Duration of the session will be 1 hr ) Please check previous announcement for more details. -NPTEL Team
Problem solving through Programming In C - Week 12 Question no. 10 Correction
Week 12 Question no. 10 in the statement if((x == sizeof(int) + 2*sizeof(char)) one extra bracket is printed after the if statement. Kindly ignore the typo and answer accordingly. The correct statement will be if(x == sizeof(int) + 2*sizeof(char))
Problem solving through Programming In C - Solution for Week 12 Assignment
Dear Learners,
Detailed Solution for Assignment 12 is available now in the Course Outline section. Please go through the solution and in case of any doubt post your queries in the forum.
-NPTEL Team
Problem solving through Programming In C : Assignment 6 question no 6
Dear Students, There is a mistake in assignment 6 question no 6. Hence the question is not considered for evaluation. The re-evaluation has been done. The updated score is displayed under Progress tab. Sorry for the inconvenience. -NPTEL Team
Problem solving through Programming In C - Solution for Week 11 Assignment
Detailed Solution for Assignment 11 is available now in the Course Outline section. Please go through the solution and in case of any doubt post your queries in the forum.
Problem solving through Programming In C - Week 12 Feedback Form
Thank you for enrolling to this NPTEL course and we hope you have gone through the contents for this week and also attempted the assignment.
We value your feedback and wish to know how you found the videos and the questions asked - whether they were easy, difficult, as per your expectations, etc
We shall use this to make the course better and we can also know from the feedback which concepts need more explanation, etc.
Please do spare some time to give your feedback - comprises just 5 questions - should not take more than a minute, but makes a lot of difference for us as we know what the Learners feel.
Here is the link to the form: https://docs.google.com/forms/d/e/1FAIpQLScnQOFwbrWSE19GlYdIR1qcDTVrDoHNVkrnGyIALvrXv7Yrqg/viewform
Exam Format - April 24,2021
Dear Candidate, ****This is applicable only for the exam registered candidates**** Type of exam will be available in the list: Click Here You will have to appear at the allotted exam center and produce your Hall ticket and Government Photo Identification Card (Example: Driving License, Passport, PAN card, Voter ID, Aadhaar-ID with your Name, date of birth, photograph and signature) for verification and take the exam in person. You can find the final allotted exam center details in the hall ticket. The hall ticket is yet to be released . We will notify the same through email and SMS. Type of exam: Computer based exam (Please check in the above list corresponding to your course name) The questions will be on the computer and the answers will have to be entered on the computer; type of questions may include multiple choice questions, fill in the blanks, essay-type answers, etc. Type of exam: Paper and pen Exam (Please check in the above list corresponding to your course name) The questions will be on the computer. You will have to write your answers on sheets of paper and submit the answer sheets. Papers will be sent to the faculty for evaluation. On-Screen Calculator Demo Link: Kindly use the below link to get an idea of how the On-screen calculator will work during the exam. https://tcsion.com/ OnlineAssessment/ ScientificCalculator/ Calculator.html NOTE: Physical calculators are not allowed inside the exam hall. -NPTEL Team
Problem solving through Programming In C - Week 12 is live now!!
Dear Students
The lecture videos for Week 12 have been uploaded for the course Problem solving through Programming In C . The lectures can be accessed using the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=94&lesson=95
The other lectures of this week are accessible from the navigation bar to the left. Please remember to login into the website to view contents (if you aren't logged in already).
Assignment for Week 12 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=94&assessment=175 Programming Assignment for Week 11 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=168 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=176 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=177 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=178 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=179
The assignment has to be submitted on or before Wednesday, 2021-04-14, 23:59 IST. The programming assignment has to be submitted on or before Thursday, 2021-04-15, 23:59 IST.
As we have done so far, please use the discussion forums if you have any questions on this module.
--NPTEL Team
Problem solving through Programming In C - Week 11 Feedback Form
Problem solving through programming in c - solution for week 10 assignment.
Detailed Solution for Assignment 10 is available now in the Course Outline section. Please go through the solution and in case of any doubt post your queries in the forum.
Problem solving through Programming In C - Solution for Week 9 Assignment
Detailed Solution for Assignment 9 is available now in the Course Outline section. Please go through the solution and in case of any doubt post your queries in the forum.
Problem solving through Programming In C - Week 10 Feedback Form
Problem solving through programming in c - week 11 is live now.
The lecture videos for Week 11 have been uploaded for the course Problem solving through Programming In C . The lectures can be accessed using the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=87&lesson=88
Assignment for Week 11 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=87&assessment=174 Programming Assignment for Week 11 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=163 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=164 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=165 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=166 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=167
The assignment has to be submitted on or before Wednesday, 2021-04-07, 23:59 IST. The programming assignment has to be submitted on or before Thursday, 2021-04-08, 23:59 IST.
Problem solving through Programming In C - Week 9 Feedback Form
Problem solving through programming in c - solution for week 8 assignment.
Detailed Solution for Assignment 8 is available now in the Course Outline section. Please go through the solution and in case of any doubt post your queries in the forum.
Problem solving through Programming In C - Week 10 is live now!!
The lecture videos for Week 10 have been uploaded for the course Problem solving through Programming In C . The lectures can be accessed using the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=80&lesson=81
Assignment for Week 10 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=80&assessment=162 Programming Assignment for Week 10 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=169 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=170 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=171 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=172 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=173
The assignment has to be submitted on or before Wednesday, 2021-03-31, 23:59 IST. The programming assignment has to be submitted on or before Thursday, 2021-04-01, 23:59 IST.
Problem solving through Programming In C - Week 9 is live now!!
The lecture videos for Week 9 have been uploaded for the course Problem solving through Programming In C . The lectures can be accessed using the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=73&lesson=74
Assignment for Week 9 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=73&assessment=156 Programming Assignment for Week 9 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=157 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=158 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=159 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=160 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=161
The assignment has to be submitted on or before Wednesday, 2021-03-24, 23:59 IST. The programming assignment has to be submitted on or before Thursday, 2021-03-25, 23:59 IST.
Problem solving through Programming In C - Week 8 Feedback Form
Reminder 1: problem solving through programming in c : online programming test (postponed to april 18,2021).
Dear Candidate The criteria for certification of this course " Problem solving through Programming In C " is different because of the online programming exam component. Please read the following carefully. Final score = 25% of Assignment score + 50% of Online exam score (Proctored) + 25% of Programming exam (unproctored). - Unproctored means, candidates will be taking the exam from college/home without NPTEL team present for monitoring the test- with access to all resources. - The discussion forum will be disabled during the exam duration (during both sessions) and re-enabled thereafter. How to take the online programming test 1. Go to: https://onlinecourses.nptel.ac.in/noc21_cs01/ 2. Log in using the same email id which you have been accessing the course (i.e. using to watch videos & submit Assignments) 3. There will be sections under the course outline "Programming Test (April 18)" that will have the online programming exam link. 4. You can click on this link and attempt the programming exam. Date: April 18, 2021 (Sunday) First Session: 10.00AM - 11.00AM (Duration of the session will be 1 hr) Second Session: 8.00PM - 9.00PM (Duration of the session will be 1 hr ) Note - You can take any one or both sessions too; the best score will be considered towards the final certification score. - You can take the exam from your college or home. - The exam has to be taken in pc or laptop. DO NOT TRY TO TAKE THE EXAM USING YOUR MOBILE PHONE. - The discussion forum will be disabled during the exam duration (during both sessions) and re-enabled thereafter. - If you encounter any issues during the exam, please write to [email protected] IMPORTANT: CERTIFICATION CRITERIA Candidates have to come to the exam center and take the theory test on April 24,2021. It is mandatory to take the Proctored in-person final exam to obtain a certificate. To pass the course and get a certificate: Assignment score >= 40/100 AND Programming exam score >= 40/100 AND Proctored exam score >= 40/100 OR Assignment score >= 10/25 AND Programming exam score >= 10/25 AND Proctored exam score >= 20/50 All 3 conditions have to be satisfied. All the best to the candidates! -NPTEL Team.
Problem solving through Programming In C - Solution for Week 7 Assignment
Detailed Solution for Assignment 7 is available now in the Course Outline section. Please go through the solution and in case of any doubt post your queries in the forum.
Problem solving through Programming In C - Week 8 is live now!!
The lecture videos for Week 8 have been uploaded for the course Problem solving through Programming In C . The lectures can be accessed using the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=66&lesson=67
Assignment for Week 8 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=66&assessment=150 Programming Assignment for Week 8 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=151 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=152 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=153 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=154 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=155
The assignment has to be submitted on or before Wednesday, 2021-03-17, 23:59 IST. The programming assignment has to be submitted on or before Thursday, 2021-03-18, 23:59 IST.
Problem solving through Programming In C - Week 6 Feedback Form
Problem solving through programming in c - solution for week 6 assignment.
Detailed Solution for Assignment 6 is available now in the Course Outline section. Please go through the solution and in case of any doubt post your queries in the forum.
Problem solving through Programming In C - Week 7 Feedback Form
Problem solving through programming in c : online programming test.
Dear Candidate The criteria for certification of this course " Problem solving through Programming In C " is different because of the online programming exam component. Please read the following carefully. Final score = 25% of Assignment score + 50% of Online exam score (Proctored) + 25% of Programming exam (unproctored). - Unproctored means, candidates will be taking the exam from college/home without NPTEL team present for monitoring the test- with access to all resources. - The discussion forum will be disabled during the exam duration (during both sessions) and re-enabled thereafter. How to take the online programming test 1. Go to: https://onlinecourses.nptel.ac.in/noc21_cs01/ 2. Log in using the same email id which you have been accessing the course (i.e. using to watch videos & submit Assignments) 3. There will be sections under the course outline "Programming Test (March 16)" that will have the online programming exam link. 4. You can click on this link and attempt the programming exam. Date: March 16, 2021 (Tuesday) First Session: 10.00AM - 11.00AM (Duration of the session will be 1 hr) Second Session: 8.00PM - 9.00PM (Duration of the session will be 1 hr ) Note - You can take any one or both sessions too; the best score will be considered towards the final certification score. - You can take the exam from your college or home. - The exam has to be taken in pc or laptop. DO NOT TRY TO TAKE THE EXAM USING YOUR MOBILE PHONE. - The discussion forum will be disabled during the exam duration (during both sessions) and re-enabled thereafter. - If you encounter any issues during the exam, please write to [email protected] IMPORTANT: CERTIFICATION CRITERIA Candidates have to come to the exam center and take the theory test on April 24,2021. It is mandatory to take the Proctored in-person final exam to obtain a certificate. To pass the course and get a certificate: Assignment score >= 40/100 AND Programming exam score >= 40/100 AND Proctored exam score >= 40/100 OR Assignment score >= 10/25 AND Programming exam score >= 10/25 AND Proctored exam score >= 20/50 All 3 conditions have to be satisfied. All the best to the candidates! -NPTEL Team.
Problem solving through Programming In C - Week 7 is live now!!
The lecture videos for Week 7 have been uploaded for the course Problem solving through Programming In C . The lectures can be accessed using the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=59&lesson=60
Assignment for Week 7 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=59&assessment=144 Programming Assignment for Week 7 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=145 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=146 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=147 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=148 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=149
The assignment has to be submitted on or before Wednesday, 2021-03-10, 23:59 IST. The programming assignment has to be submitted on or before Thursday, 2021-03-11, 23:59 IST.
Problem solving through Programming In C - Solution for Week 5 Assignment
Detailed Solution for Assignment 5 is available now in the Course Outline section. Please go through the solution and in case of any doubt post your queries in the forum.
Problem solving through Programming In C - Week 5 Feedback Form
Problem solving through programming in c - week 6 is live now.
The lecture videos for Week 6 have been uploaded for the course Problem solving through Programming In C . The lectures can be accessed using the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=52&lesson=53
Assignment for Week 6 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=52&assessment=138 Programming Assignment for Week 6 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=139 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=140 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=141 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=142 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=143
The assignment has to be submitted on or before Wednesday, 2021-03-03, 23:59 IST. The programming assignment has to be submitted on or before Thursday, 2021-03-04, 23:59 IST.
Problem solving through Programming In C - Solution for Week 4 Assignment
Detailed Solution for Assignment 4 is available now in the Course Outline section. Please go through the solution and in case of any doubt post your queries in the forum.
Problem solving through Programming In C - Week 4 Feedback Form
Problem solving through programming in c - solution for week 3 assignment.
Detailed Solution for Assignment 3 is available now in the Course Outline section. Please go through the solution and in case of any doubt post your queries in the forum.
Problem solving through Programming In C - Week 5 is live now!!
The lecture videos for Week 5 have been uploaded for the course Problem solving through Programming In C . The lectures can be accessed using the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=45&lesson=46
Assignment for Week 5 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=45&assessment=127 Programming Assignment for Week 5 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=133 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=134
The assignment has to be submitted on or before Wednesday, 2021-02-24, 23:59 IST. The programming assignment has to be submitted on or before Thursday, 2021-02-25, 23:59 IST.
Problem solving through Programming In C - Solution for Week 2 Assignment
Detailed Solution for Assignment 2 is available now in the Course Outline section. Please go through the solution and in case of any doubt post your queries in the forum.
Problem solving through Programming In C - Week 4 is live now!!
The lecture videos for Week 4 have been uploaded for the course Problem solving through Programming In C . The lectures can be accessed using the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=38&lesson=39
Assignment for Week 4 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=38&assessment=126 Programming Assignment for Week 4 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=128 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=129 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=130 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=131
The assignment has to be submitted on or before Wednesday, 2021-02-17, 23:59 IST. The programming assignment has to be submitted on or before Thursday, 2021-02-18, 23:59 IST.
Problem solving through Programming In C - Week 3 Feedback Form
Problem solving through programming in c - solution for week 1 assignment.
Detailed Solution for Assignment 1 is available now in the Course Outline section. Please go through the solution and in case of any doubt post your queries in the forum.
Problem solving through Programming In C - Assignment 2 Due date Extended!!
Dear Learners, Assignment 2 has been released already and the due date for the assignment has been extended Due date of assignment 2 is Sunday, 07-02-2021, 23:59 IST. Please note that there will not be any extension for the upcoming assignments. Note: Please check the due date of the assignments in the announcement and assignment page if you see any mismatch write to us immediately. Thanks & Regards, -NPTEL Team
Problem solving through Programming In C - Week 3 is live now!!
The lecture videos for Week 3 have been uploaded for the course Problem solving through Programming In C . The lectures can be accessed using the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=31&lesson=32
Assignment for Week 3 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=31&assessment=121 Programming Assignment for Week 3 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=122 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=123 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=124 https://onlinecourses.nptel.ac.in/noc21_cs01/progassignment?name=125
The assignment has to be submitted on or before Wednesday, 2021-02-10, 23:59 IST. The programming assignment has to be submitted on or before Thursday, 2021-02-11, 23:59 IST.
Problem solving through Programming In C - Week 2 Feedback Form
Problem solving through programming in c - week 1 feedback form, problem solving through programming in c - week 2 is live now.
The lecture videos for Week 2 have been uploaded for the course Problem solving through Programming In C . The lectures can be accessed using the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=24&lesson=25
Assignment for Week 2 is also uploaded and can be accessed from the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=24&assessment=120
The assignment has to be submitted on or before Wednesday, 2021-02-03, 23:59 IST.
Problem solving through Programming In C - Download video links are available now!!
The download video links for the course Problem solving through Programming In C are available now in the course outline. Please check the download video link: https://nptel.ac.in/courses/106/105/106105171/
Problem solving through Programming In C - Week 1 assignment is live now!!
The assignment for Week 1 for the course Problem solving through Programming In C is made available early for viewing to get an idea about the assignments but the actual start date of the course remains unchanged.
Assignment 1 can be accessed using the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=17&assessment=119
The other Assignment 1 is accessible from the navigation bar to the left under Week 1. Please remember to login into the website to view contents (if you aren't logged in already).
The assignment has to be submitted on or before Wednesday, 2021-02-03, 23:59 IST.
Please use the discussion forums if you have any questions on this module.
Happy Learning!
Some Corrections in Video lectures of Week-1 - Problem Solving through Programming in C
Dear Learners, At 11.25 mins of the Lecture 3: Flow Chart and Pseudocode , in the flow chart of printing average of 3 numbers the final print statement should be “ Print AVG ” instead of “ Print SUM ” as we are finding the Average of 3 numbers. At 7.12 mins of the Lecture 3: Flow Chart and Pseudocode , Regarding the symbols used to represent the flow chart, generally oblong symbol is used to represent START and END of a flow chart and a parallelogram symbol is used to represent INPUT and OUTPUT, and the Circle symbol is used as a connector, when we need to represent the continuation of flow chart when there is shortage of space. At 18.52 mins of the Lecture 3: Flow Chart and Pseudocode , in the flow chart of printing the average of N numbers: after reading the number and storing it in N we should store in another variable say M. So after the statement “ Read the value of N ” there will be another statement which will be “ M=N ” in a rectangular box. And after the decision box instead of AVG=SUM/N it will be AVG=SUM/M , as the value of N is becoming zero at the end of the decision loop. At 28.07mins of the Lecture 3: Flow Chart and Pseudocode : in the flow chart for finding Max of N positive integer please follow the flow chart drawn in blue colour. Please ignore the corrections made. -NPTEL Team
Problem solving through Programming In C - Assignment-0-RELEASED
We welcome you all to this course. The assignment 0 for the course Problem solving through Programming In C has been released. This assignment is based on a prerequisite of the course. Kindly note that marks obtained in this assignment will not be considered for the final assessment. You can find the assignment under Week 0 unit on the left-hand side of your screen You can submit the assignment multiple times before the due date. All the best !!
The extended due date of Assignment 0: Monday, 2021-01-25, 23:59 IST.
NPTEL: Exam Registration is open now for Jan 2021 courses!
Dear Learner, Here is the much-awaited announcement on registering for the Jan 2021 NPTEL course certification exam. 1. The registration for the certification exam is open only to those learners who have enrolled in the course. 2. If you want to register for the exam for this course, login here using the same email id which you had used to enroll to the course in Swayam portal. Please note that Assignments submitted through the exam registered email id ALONE will be taken into consideration towards final consolidated score & certification. 3 . Date of exam: April 24, 2021 Certification exam registration URL is: https://examform.nptel.ac. in/ Choose from the Cities where exam will be conducted: Exam Cities 4. Exam fees: If you register for the exam and pay before Mar 8, 2021, 10:00 AM, Exam fees will be Rs. 1000/- per exam . If you register for exam before Mar 8, 2021 , 10:00 AM and have not paid or if you register between Mar 8, 2021, 10:00 AM & Mar 12, 2021, 5:00 PM, Exam fees will be Rs. 1500/- per exam 5. 50% fee waiver for the following categories: Students belonging to the SC/ST category: please select Yes for the SC/ST option and upload the correct Community certificate. Students belonging to the PwD category with more than 40% disability: please select Yes for the option and upload the relevant Disability certificate. 6. Last date for exam registration: Mar 12, 2021 5:00 PM (Friday). 7. Mode of payment: Online payment - debit card/credit card/net banking. 8. HALL TICKET: The hall ticket will be available for download tentatively by 2 weeks prior to the exam date . We will confirm the same through an announcement once it is published. 9. FOR CANDIDATES WHO WOULD LIKE TO WRITE MORE THAN 1 COURSE EXAM:- you can add or delete courses and pay separately – till the date when the exam form closes. Same day of exam – you can write exams for 2 courses in the 2 sessions. Same exam center will be allocated for both the sessions. 10. Data changes: Last date for data changes: Mar 12, 2021, 5:00 PM: All the fields in the Exam form except for the following ones can be changed until the form closes. The following 6 fields can be changed ONLY when there are NO courses in the course cart. And you will be able to edit the following fields only if you: - REMOVE unpaid courses from the cart And/or - CANCEL paid courses 1. Do you come under the SC/ST category? * 2. SC/ST Proof 3. Are you a person with disabilities? * 4. Are you a person with disabilities above 40%? 5. Disabilities Proof 6. What is your role ? Note: Once you remove or cancel a course, you will be able to edit these fields immediately. But, for cancelled courses, refund of fees will be initiated only after 2 weeks. 11. LAST DATE FOR CANCELLING EXAMS and getting a refund: Mar 12, 2021, 5:00 PM 12. Click here to view Timeline and Guideline : Guideline Thanks & Regards, NPTEL TEAM
Week 1 videos are live now!! - Problem solving through Programming In C
The videos for Week 1 for the course Six Sigma is made available early for viewing to get an idea about the content but the actual start date remains unchanged.
The lectures can be accessed using the following link: https://onlinecourses.nptel.ac.in/noc21_cs01/unit?unit=17&lesson=18
Welcome to NPTEL Online Course - Jan 2021!!
- Every week, about 2.5 to 4 hours of videos containing content by the Course instructor will be released along with an assignment based on this. Please watch the lectures, follow the course regularly and submit all assessments and assignments before the due date. Your regular participation is vital for learning and doing well in the course. This will be done week on week through the duration of the course.
- Please do the assignments yourself and even if you take help, kindly try to learn from it. These assignments will help you prepare for the final exams. Plagiarism and violating the Honor code will be taken very seriously if detected during the submission of assignments.
- The announcement group - will only have messages from course instructors and teaching assistants - regarding the lessons, assignments, exam registration, hall tickets etc.
- The discussion forum (Ask a question tab on the portal) - is for everyone to ask questions and interact.Anyone who knows the answers can reply to anyone's post and the course instructor/TA will also respond to your queries.
- Please make maximum use of this feature as this will help you learn much better.
- If you have any questions regarding the exam, registration, hall tickets, results, queries related to the technical content in the lectures, any doubts in the assignments, etc can be posted in the forum section
- The course is free to enroll and learn from. But if you want a certificate, you have to register and write the proctored exam conducted by us in person at any of the designated exam centres.
- The exam is optional for a fee of Rs 1000/- (Rupees one thousand only).
- Date and Time of Exams: April 24,2021 Morning session 9am to 12 noon; Afternoon Session 2pm to 5pm.
- Registration url: Announcements will be made when the registration form is open for registrations.
- The online registration form has to be filled and the certification exam fee needs to be paid. More details will be made available when the exam registration form is published. If there are any changes, it will be mentioned then.
- Please check the form for more details on the cities where the exams will be held, the conditions you agree to when you fill the form etc.
- Once again, thanks for your interest in our online courses and certification. Happy learning.
NPTEL : Keep in touch with us via Social Media
Dear Learner You already must know NPTEL is providing course certificates to those who complete the course successfully, with the learning happening right at your home or where you are. But NPTEL also keeps bringing out new initiatives and courses - which we would like to keep you posted on. Click the below links to like and follow us on Social Media for instant Updates: Facebook: https://www.facebook.com/NPTELNoc Twitter: https://twitter.com/nptelindia Linkedin: https://www.linkedin.com/in/nptel-india-085866ba/ Instagram: https://www.instagram.com/swayam_nptel/ Like and Follow us on Social Media. Let's create a better future by learning and growing together. -NPTEL Team.
A project of

In association with

Problem Solving Through Programming In C Week 9
Session: JULY-DEC 2023
Course Name: Problem Solving Through Programming In C
Course Link: Click Here
These are Problem Solving Through Programming In C Assignment 9 Answers
Q1. What is the worst case complexity of selection sort? a) O(nlogn) b) O(logn) c) O(n) d) O(n 2 )
Answer: d) O(n 2 )
Q2. What is the best case and worst case complexity of ordered linear search? a) O(nlogn), O(logn) b) O(logn), O(nlogn) c) O(n), O(1) d) O(1), O(n)
Answer: d) O(1), O(n)
Q3. Given an array arr = {12, 34, 47, 62, 85, 92, 95, 99,105} and key = 34; what are the mid values (corresponding array elements) generated in the first and second iterations? a) 85 and 12 b) 85 and 34 c) 62 and 34 d) 62 and 47
Answer: b) 85 and 34
Q4. When the Binary search is best applied to an array? a) For very large size array b) When the array is sorted c) When the array elements are mixed data type d) When the array is unsorted
Answer: b) When the array is sorted
Q5. Consider the array A[ ]= {5,4,9,1,3} apply the insertion sort to sort the array. Consider the cost associated with each sort is 25 rupees, what is the total cost of the insertion sort for sorting the entire array? a) 25 b) 50 c) 75 d) 100
Answer: c) 75
Q6. Select the code snippet which performs unordered linear search iteratively?
Q7. What will be the output? a) 3 5 b) 3 0 c) 5 0 d) 5 3
Answer: d) 5 3
Q8. Consider an array of elements arr[5]= {5,4,3,2,1}, what are the steps of insertions done while doing insertion sort in the array.

Q9. What will be the output of the following C code? a) 0 b) 1 c) 01 d) None of the above
Answer: b) 1
Q10. What will be the output? a) 10 10 b) 10 50 c) 50 50 d) Compilation error
Answer: d) Compilation error
Question 1 Write a program to print all the locations at which a particular element (taken as input) is found in a list and also print the total number of times it occurs in the list. The location starts from 1. For example if there are 4 elements in the array 5 6 5 7 If the element to search is 5 then the output will be 5 is present at location 1 5 is present at location 3 5 is present 2 times in the array.
Question 2 Write a C program to search a given element from a 1D array and display the position at which it is found by using linear search function. The index location starts from 1.
Question 3 Write a C program to search a given number from a sorted 1D array and display the position at which it is found using binary search algorithm. The index location starts from 1.
Question 4 Write a C program to reverse an array by swapping the elements and without using any new array.
More Weeks of Problem Solving Through Programming In C: Click here
More Nptel Courses: Click here
Session: JAN-APR 2023
Q1. What is the worst case complexity of selection sort? a) O(nlogn) b) O(logn) c) O(n) d) O(n^2)
Answer: d) O(n^2)
Q2. What is the error in the following program? a) Variable ‘a’ should be replaced by ‘si’ b) Nothing will print c) Error due to multiple declaration of si d) No error
Answer: c) Error due to multiple declaration of si
Q3. When the Binary search is best applied to an array? a) For very large size array b) When the array is sorted c) When the array elements are mixed data type d) When the array is unsorted
Q4. Select the code snippet which performs unordered linear search iteratively? a) int unorderedLinear Search(int arr[], int size, int data) { int index; for(int i = 0; i < size; i++) { if(arr[i] { } } = data) index = i; break; } return index : } b) int unordered Linear Search(int arr[], int size, int data) { int index; for(int i = 0; i < size; i++) { if(arr[i] == data) { break; } } return index : } c) int unorderedLinear Search(int arr[], int size, int data) { int index; for(int i = 0; i <= size; i++) { } if(arr[i] = data) { index = i; continue; } return index : } d) None of these
Answer: a) int unorderedLinear Search(int arr[], int size, int data) { int index; for(int i = 0; i < size; i++) { if(arr[i] { } } = data) index = i; break; } return index : }
Q5. What is the best case and worst case complexity of ordered linear search? a) O(nlogn), O(logn) b) O(logn), O(nlogn) c) O(n), O(1) d) O(1), O(n)
Q6. Which of the following is a disadvantage of linear search? a) Requires more space b) Greater time complexities compared to other searching algorithms c) Not easy to understand d) All of the mentioned
Answer: b) Greater time complexities compared to other searching algorithms
Q7. Given an array arr = {45, 77, 89, 91, 94, 98,100} and key = 100; what are the mid values (corresponding array elements) generated in the first and second iterations? a) 91 and 98 b) 91 and 100 c) 89 and 94 d) 94 and 98
Answer: a) 91 and 98
Q8. Select the appropriate pseudocode that performs selection sort a) int min; for(int j=0; j<arr.length-1; j++) { min = j; for(int k=j+1; k<=arr.length-1; k++) { if(arr[k] < arr[min]) min = k; } int temp = arr[min]; arr[min] = arr[j]; arr[j] = temp; } b) int min; for(int j=0; j<arr.length-1; j++) { min =j; for(int k=j+1; k<=arr.length; k++) { if(arr[k] < arr[min]) min = k; } int temp = arr[min]; arr[min] = arr[j]; arr[j] = temp; c) int min; for(int j=0; j arr[min]) min = k; } int temp = arr[min]; arr[min] = arr[j]; arr[j] = temp : } d) int min; for(int j=0; j arr[min]) min = k; } int temp = arr[min]; arr[min] = arr[j]; arr[j] = temp : }
Answer: a) int min; for(int j=0; j<arr.length-1; j++) { min = j; for(int k=j+1; k<=arr.length-1; k++) { if(arr[k] < arr[min]) min = k; } int temp = arr[min]; arr[min] = arr[j]; arr[j] = temp; }
Q9. Consider the array A[ ]= {5,4.9,1,3} apply the insertion sort to sort the array. Consider the cost associated with each sort is 25 rupees, what is the total cost of the insertion sort when element 1 reaches the first position of the array? a) 25 b) 50 c) 75 d) 100
Answer: b) 50
Q10. Find the output of the following C program a) 5,4 b) 5,5 c) 4,4 d) 3,4
Answer: c) 4,4
Problem Solving Through Programming In C Programming Assignment
Write a program to print all the locations at which a particular element (taken as input) is found in a list and also print the total number of times it occurs in the list. The location starts from 1. For example there are 4 elements in the array 5 6 5 7 The element to search is 5 then the output will be: 5 is present at location 1 5 is present at location 3 5 is present 2 times in the array.
Write a C program to marge two given sorted arrays (sorted in ascending order). The code for input and output is already written. You have to write the merge function so that the merged array is displayed in ascending order.
Write a C program to search a given number from a sorted 1D array and display the position at which it is found using binary search algorithm. The index location starts from 1.
Write a C program to reverse an array by swapping the elements and without using any new array.
More Weeks of Problem Solving Through Programming In C: Click Here
More Nptel courses: https://progiez.com/nptel
Session: JULY-DEC 2022
Course Name: Problem Solving Through Programming In C NPTEL
Link of course: Click Here
Q1. What is the best case complexity of ordered linear search and worst case complexity of selection sort respectively? a) 0(1), O(n²) b) O(logn), O(1). c) O(n), O(logn) d) O(n²), O(nlogn)
Answer: a) 0(1), O(n²)
Q2. Which of the following is/are correct? I.Binary search is applied when elements are sorted. II.Linear search can be applied when elements are in random order. III. Binary search can be categorized into divide and conquer rule. a) I & II b) Only I c) I and III d) I,II & III
Answer: d) I,II & III
Q3. What is the recurrence relation for the linear search recursive algorithm? a) T(n-2)+c b) 2T(n-1)+c c) T(n-1)+c d) T(n+1)+c
Answer: c) T(n-1)+c
Q4. Given an array arr = {20, 45, 77, 89, 91, 94, 98,100} and key = 45; what are the mid values (corresponding array elements) generated in the first and second iterations? a) 91 and 98 b) 89 and 45 c) 89 and 77 d) 91 and 94
Answer: b) 89 and 45
Q5. Consider an array of elements A[7]= {10,4,7,23,67,12,5}, what will be the resultant array A after third pass of insertion sort. a) 67,12,10,5,4,7,23 b) 4,7,10,23,67,12,5 c) 4,5,7,67,10,12,23 d) 10,7,4,67,23,12,5
Answer: b) 4,7,10,23,67,12,5
Answer: (a)
Q7. Which of the following input will give worst case time complexity for selection sort to sort an array in ascending order? I. 1,2,3, 4, 5, 6, 7, 8 II. 8,7,6, 5, 4, 3, 2, 1, III. 8,7,5,6,3,2,1,4 a) I b) II c) II and III d) I,II and III
Answer: b) II
Q8. Consider the array A[] {5,4,9,1,3} apply the insertion sort to sort the array. Consider the cost associated with each sort is 25 rupees, what is the total cost of the insertion sort for sorting the entire array? a) 25 b) 50 c) 75 d) 100
Q9. A sorting technique is called stable if : a) It takes O(nlog n)time b) It maintains the relative order of occurrence of non-distinct elements c) It uses divide and conquer paradigm d) It takes O(n) space
Answer: b) It maintains the relative order of occurrence of non-distinct elements
Q10. The average case occurs in the Linear Search Algorithm when a) The item to be searched is in some where middle of the Array b) The item to be searched is not in the array c) The item to be searched is in the last of the array d) The item to be searched is either in the last or not in the array
Answer: a) The item to be searched is in some where middle of the Array
Programming Assignment
Question 1 Write a program to print all the locations at which a particular element (taken as input) is found in a list and also print the total number of times it occurs in the list. The location starts from 1. For example if there are 4 elements in the array 5 6 5 7 If the element to search is 5, then the output will be: 5 is present at location 1 5 is present at location 3 5 is present 2 times in the array.
More NPTEL Solution: https://progiez.com/nptel

- Wednesday, May 8, 2024
Problem Solving Through Programming In C Week12 Assignment July 2023
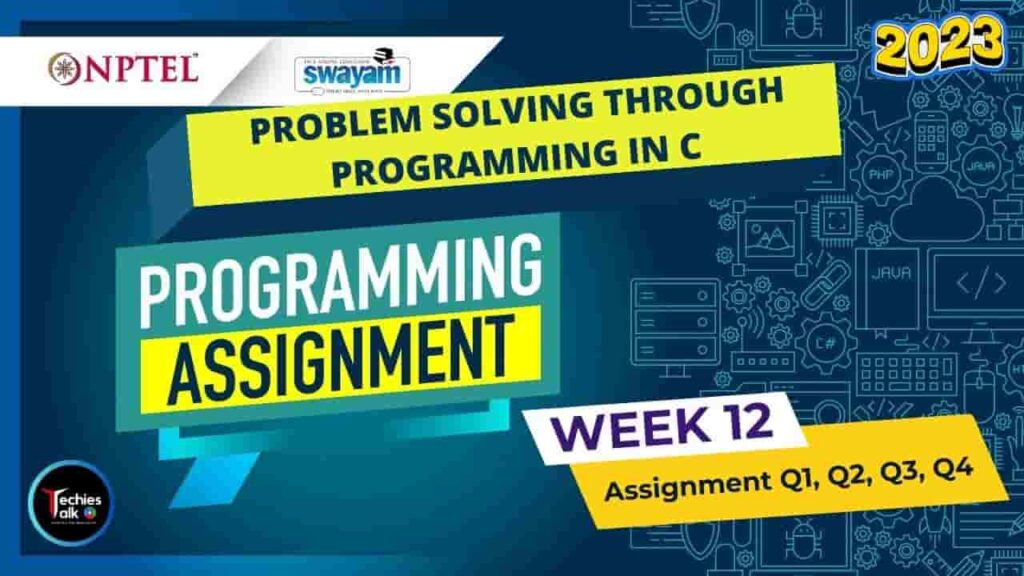
NPTEL Problem Solving Through Programming In C Week12 All Programming Assignment Solutions | Swayam July 2023. With the growth of Information and Communication Technology, there is a need to develop large and complex software.
ABOUT THE COURSE:
- Formulate simple algorithms for arithmetic and logical problems
- Translate the algorithms to programs (in C language)
- Test and execute the programs and correct syntax and logical errors
- Implement conditional branching, iteration and recursion
- Decompose a problem into functions and synthesize a complete program using divide and conquer approach
- Use arrays, pointers and structures to formulate algorithms and programs
- Apply programming to solve matrix addition and multiplication problems and searching and sorting problems
- Apply programming to solve simple numerical method problems, namely rot finding of function, differentiation of function and simple integration
COURSE LAYOUT
- Week 1 : Introduction to Problem Solving through programs, Flowcharts/Pseudo codes, the compilation process, Syntax and Semantic errors, Variables and Data Types
- Week 2 : Arithmetic expressions, Relational Operations, Logical expressions; Introduction to Conditional Branching
- Week 3 : Conditional Branching and Iterative Loops
- Week 4 : Arranging things : Arrays
- Week 5 : 2-D arrays, Character Arrays and Strings
- Week 6 : Basic Algorithms including Numerical Algorithms
- Week 7 : Functions and Parameter Passing by Value
- Week 8 : Passing Arrays to Functions, Call by Reference
- Week 9 : Recursion
- Week 10 : Structures and Pointers
- Week 11 : Self-Referential Structures and Introduction to Lists
- Week 12 : Advanced Topics
Once again, thanks for your interest in our online courses and certification. Happy learning!
Problem Solving through Programming In C July 2023
Question : 1 Write a program in C to find the factorial of a given number using pointers .
Question : 2 Write a C program to print the Record of the Student Merit wise . Here a structure variable is defined which contains student rollno, name and score .
Question : 3 Write a C program to store n elements using Dynamic Memory Allocation – calloc() and find the Largest element .
Question : 4 Write a C program to find the sum of two 1D integer arrays ‘A’ and ‘B’ of same size and store the result in another array ‘C’ , where the size of the array and the elements of the array are taken as input.
[Week 1] NPTEL Problem Solving Through Programming In C Assignment Answers 2024
Nptel problem solving through programming in c week 1 assignment answers 2024.
1. CPU comprises of
a) ALU- Arithmetic and Logic Unit b) Registers c) Control unit d) All of the above
2. Input device/s of a computer
a) Printer b) Speaker c) Joystick d) Monitor
3. Choose the correct statements from the following i) In high-level language, testing and debugging a program is difficult than assembly language. ii) C programs are highly portable on any type of operating system platform. iii) A flowchart is a visual representation of the sequence of steps for solving a problem. iv) The role of a compiler is to translate source program statements to decimal codes.
a) (i) and (ii) b) (ii) and (iii) c) (i), (ii), and (iii) d) (ii), (iii), and (iv)
4. How many bits are there in a byte?
a) 2 b) 4 c) 8 d) 16
![[Week 1] NPTEL Problem Solving Through Programming In C Assignment Answers 2024 1 Screenshot 2024 01 20 201419](https://gecmunger.in/wp-content/uploads/2024/01/Screenshot-2024-01-20-201419.png)
a) 4 b) 8 c) 16 d) 20
![[Week 1] NPTEL Problem Solving Through Programming In C Assignment Answers 2024 2 Screenshot 2024 01 20 201541](https://gecmunger.in/wp-content/uploads/2024/01/Screenshot-2024-01-20-201541.png)
a) 21 b) 28 c) 30 d) 40
![[Week 1] NPTEL Problem Solving Through Programming In C Assignment Answers 2024 3 Screenshot 2024 01 20 202144](https://gecmunger.in/wp-content/uploads/2024/01/Screenshot-2024-01-20-202144.png)
a) a=4,b=6 b) a=6,b=4 c) a=10,b=2 d) a=2,b=10
8. The program which translates high level program into its equivalent machine language program is called
a) a translator b) a language processor c) a converter d) None of the above
9. An interpreter reads the source code of a program
a) one line at a time b) two line at a time c) complete program in one stroke d) None of these
10. Flowchart and algorithms are used for
a) Easy testing and debugging b) Better programming c) Efficient coding d) All of these
Share your love
Related posts, [week 1] nptel programming in modern c++ assignment answers 2024, [week 1] nptel forests and their management assignment answers 2024, [week 1] nptel social networks assignment answers 2024.
![[Week 1 to 8] NPTEL Data Science For Engineers Assignment Answers 2023 7 [Week 1] NPTEL Data Science For Engineers Assignment Answers 2023](https://gecmunger.in/wp-content/uploads/2023/07/Data-Science-For-Engineers-1024x576.png)
[Week 1 to 8] NPTEL Data Science For Engineers Assignment Answers 2023
![[Week 1] NPTEL Ecology And Environment Assignment Answers 2023 8 NPTEL Ecology And Environment Assignment Answers](https://gecmunger.in/wp-content/uploads/2023/08/Nptel-Ecology-And-Environment-Answers-1024x576.png)
[Week 1] NPTEL Ecology And Environment Assignment Answers 2023
[week 1] nptel blockchain and its applications assignment answers 2024, leave a comment cancel reply.
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
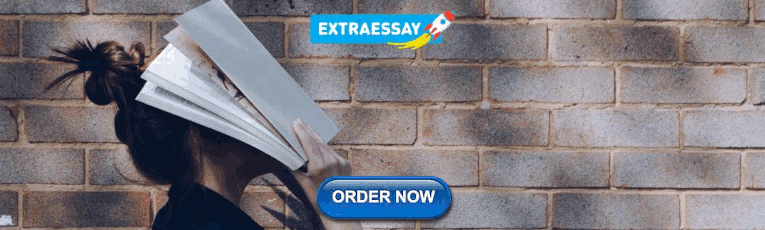
IMAGES
VIDEO
COMMENTS
Learners enrolled: 29073. ABOUT THE COURSE : This course is aimed at enabling the students to. Formulate simple algorithms for arithmetic and logical problems. Translate the algorithms to programs (in C language) Test and execute the programs and correct syntax and logical errors. Implement conditional branching, iteration and recursion.
Q2: Write a Program to find the Sum of two numbers entered by the user. In this problem, you have to write a program that adds two numbers and prints their sum on the console screen. For Example, Input: Enter two numbers A and B : 5 2. Output: Sum of A and B is: 7.
Courses. Computer Science and Engineering. NOC:Problem Solving through Programming in C (Video) Syllabus. Co-ordinated by : IIT Kharagpur. Available from : 2017-12-21. Lec : 1.
Neilblaze/NPTEL-Problem-solving-through-Programming-In-C-Solutions This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository. master
This course is aimed at enabling the students toFormulate simple algorithms for arithmetic and logical problems.Translate the algorithms to programs (in C la...
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
Problem solving through Programming In C. ABOUT THE COURSE This course is aimed at enabling the students to. ·formulate simple algorithms for arithmetic and logical problems·translate the algorithms to programs (in C language)·test and execute the programs and correct syntax and logical errors·implement conditional branching, iteration and ...
Problem Solving Through Programming In C - Assignment-12. Solution Released Dear Participants, The Assignment-12 of Week- 12 Solution for the course "Problem Solving Through Programming In C" has been released in the portal. Please go through the solution and in case of any doubt post your queries in the forum.
NPTEL | Problem Solving Through Programming In C | Week 4 : Assignment 4 | Answers with Proof | Jan 2024 Quiz SolutionsJoin Telegram Group of this course, NP...
03/07/2020 Problem solving through Programming In C - - Unit 14 - Week 12 https://onlinecourses.nptel.ac.in/noc20_cs06/unit?unit=13&assessment=159 3/7
C is a general-purpose, imperative computer programming language, supporting structured programming, lexical variable scope and recursion, while a static type system prevents many unintended operations. C was originally developed by Dennis Ritchie between 1969 and 1973 at Bell Labs.
Problem Solving through Programming In C Jan 2024. Program-01 : Write a C Program to find Largest Element of an Integer Array. Here the number of elements in the array 'n' and the elements of the array is read from the test data. Use the printf statement given below to print the largest element. printf ("Largest element = %d", largest);
COURSE LAYOUT. Week 1 : Introduction to Problem Solving through programs, Flowcharts/Pseudo codes, the compilation process, Syntax and Semantic errors, Variables and Data Types Week 2 : Arithmetic expressions, Relational Operations, Logical expressions; Introduction to Conditional Branching Week 3 : Conditional Branching and Iterative Loops Week 4 : Arranging things : Arrays
The criteria for certification of this course " Problem solving through Programming In C " is different because of the online programming exam component. Please read the following carefully. Final score = 25% of Assignment score + 50% of Online exam score (Proctored) + 25% of Programming exam (unproctored).
Problem Solving Through Programming In C Programming Assignment Question 1 Write a program to print all the locations at which a particular element (taken as input) is found in a list and also print the total number of times it occurs in the list.
COURSE LAYOUT. Week 1 : Introduction to Problem Solving through programs, Flowcharts/Pseudo codes, the compilation process, Syntax and Semantic errors, Variables and Data Types. Week 2 : Arithmetic expressions, Relational Operations, Logical expressions; Introduction to Conditional Branching. Week 3 : Conditional Branching and Iterative Loops.
NPTEL Problem Solving Through Programming In C Week 1 Assignment Answers 2024. 1. CPU comprises of. 2. Input device/s of a computer. 3. Choose the correct statements from the following. i) In high-level language, testing and debugging a program is difficult than assembly language.