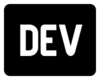
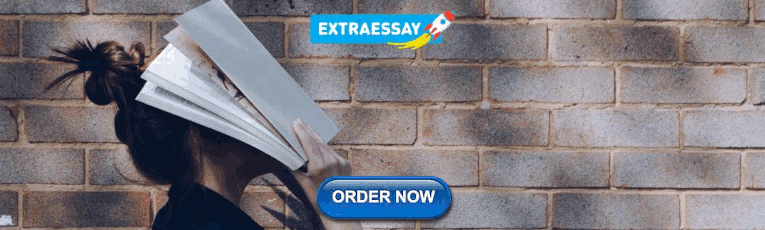
DEV Community

Posted on Jun 1, 2023
How to Avoid Unchecked Casts in Java Programs
Unchecked cast refers to the process of converting a variable of one data type to another data type without checks by the Java compiler.
This operation is unchecked because the compiler does not verify if the operation is valid or safe. Unchecked casts can lead to runtime errors, such as ClassCastException, when the program tries to assign an object to a variable of an incompatible type.
Hence, it is important to avoid unchecked casts in Java programs to prevent potential errors and ensure the program's reliability.
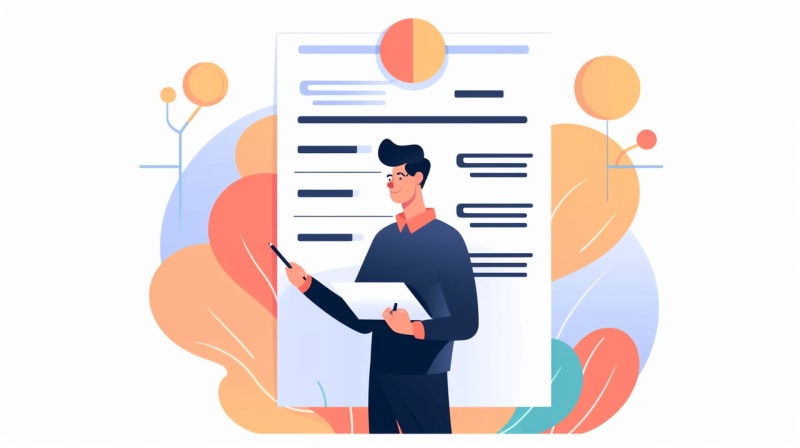
Consequences of Unchecked Casts
In Java programs, unchecked casts can lead to several issues. The most common problem is a ClassCastException at runtime, which occurs when we try to cast an object to a wrong type. This can cause the program to crash or behave unexpectedly.
Unchecked casts also violate the type safety of the Java language, which can lead to bugs that are difficult to detect and debug. Additionally, unchecked casts can make the code less readable and maintainable, as they hide the true type of objects and dependencies between components.
Therefore, it is important to avoid unchecked casts and use other mechanisms, such as generics or polymorphism, to ensure type safety and code quality in Java programs.
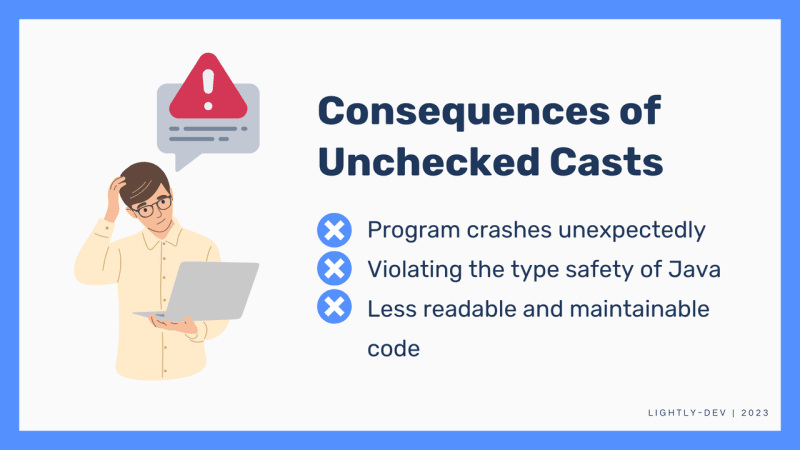
How Unchecked Casts Occur
Unchecked casts in Java programs occur when an object of one type is assigned to a reference of another type without proper type checking. This can happen when a programmer assumes that a reference to a superclass is actually a reference to its subclass and tries to cast it into that subclass. If the assumption is incorrect, the cast will result in a ClassCastException at runtime.
Unchecked casts can also occur when dealing with raw types, which are generic types without any type parameters specified. In such cases, the compiler cannot perform type checking and the programmer must ensure that the proper type conversions are made. Failing to do so can result in unchecked casts and potential runtime errors.
Why unchecked casts are problematic
In Java, unchecked casts allow a programmer to cast any object reference to any other reference type without providing any type information at compile-time. While this flexibility may seem useful, it can lead to serious run-time errors. If the object being casted is not actually of the type specified, a ClassCastException will occur at run-time.
Unchecked casts can cause difficult-to-debug errors in large and complex codebases, as it may not be immediately clear where the error originated. Additionally, unchecked casts can undermine Java's type system, creating code that is harder to read, maintain, and reason about. As a result, avoiding unchecked casts should be a priority when writing Java programs.
Examples of Unchecked Casts in Java
Unchecked casts are a common source of Java program errors. Here are some examples of unchecked casts:
This cast statement above can result in a class cast exception if the object referred to by obj is not a List.
In this case, the cast could fail at runtime if the array contains objects of a type other than String.
Finally, this cast could fail if the object referred to by obj is not a Map.
Using Generics to Avoid Unchecked Casts in Java
In Java, Generics is a powerful feature that allows you to write classes and methods that are parameterized by one or more types. Generics are a way of making your code more type-safe and reusable. With generics, you can define classes and methods that work on a variety of types, without having to write separate code for each type.
Using generics in Java programs has several advantages. It enables type safety at compile-time, which can prevent ClassCastException errors at runtime. With generics, the compiler can detect type mismatches and prevent them from happening, which leads to more robust and reliable code. It also allows for code reuse without sacrificing type safety and improve performance by avoiding unnecessary casting and allowing for more efficient code generation.
Generics allow Java developers to create classes and methods that can work with different data types. For example, a List can be defined to hold any type of object using generics. Here's an example:
In this example, we create a List that holds String objects. We can add String objects to the list and iterate over them using a for-each loop. The use of generics allows us to ensure type safety and avoid unchecked casts. Another example is the Map interface, which can be used to map keys to values of any data type using generics.
Using the instanceof operator to Avoid Unchecked Casts in Java
The instanceof operator is a built-in operator in Java that is used to check whether an object is an instance of a particular class or interface. The operator returns a boolean value - true if the object is an instance of the specified class or interface, and false otherwise.
The instanceof operator is defined as follows:
where object is the object that is being checked, and class/interface is the class or interface that is being tested against.
The instanceof operator can be useful in situations where we need to perform different operations based on the type of an object. It provides a way to check the type of an object at runtime, which can help prevent errors that can occur when performing unchecked casts.
Here are some examples of using the instanceof operator:
In this example, we use the instanceof operator to check whether the object obj is an instance of the String class. If it is, we perform an explicit cast to convert the object to a String and call the toUpperCase() method on it.
In this example, we use the instanceof operator to check whether the List object passed as a parameter is an instance of the ArrayList or LinkedList classes. If it is, we perform an explicit cast to convert the List to the appropriate class and perform different operations on it depending on its type.
Overall, using the instanceof operator can help us write more robust and flexible code. However, it should be used judiciously as it can also make code harder to read and understand.
Using Polymorphism to Avoid Unchecked Casts in Java
Polymorphism is a fundamental concept in object-oriented programming. It refers to the ability of an object or method to take on multiple forms. It allows us to write code that can work with objects of different classes as long as they inherit from a common superclass or implement a common interface. This helps to reduce code duplication and makes our programs more modular and extensible.
Some of the advantages of using polymorphism are:
- Code reusability: We can write code that can work with multiple objects without having to rewrite it for each specific class.
- Flexibility: Polymorphism allows us to write code that can adapt to different types of objects at runtime.
- Ease of maintenance: By using polymorphism, changes made to a superclass or interface are automatically propagated to all its subclasses.
Here are a few examples of how you can use polymorphism to avoid unchecked casts in Java:
Example 1: Shape Hierarchy
In this example, the abstract class Shape defines the common behavior draw(), which is implemented by the concrete classes Circle and Rectangle. By using the Shape reference type, we can invoke the draw() method on different objects without the need for unchecked casts.
Example 2: Polymorphic Method Parameter
In this example, the makeAnimalSound() method accepts an Animal parameter. We can pass different Animal objects, such as Dog or Cat, without the need for unchecked casts. The appropriate implementation of the makeSound() method will be invoked based on the dynamic type of the object.
By utilizing polymorphism in these examples, we achieve type safety and avoid unchecked casts, allowing for cleaner and more flexible code.
Tips to Avoid Unchecked Casts in Java Programs
Unchecked casts in Java programs can introduce runtime errors and compromise type safety. Fortunately, there are several techniques and best practices you can employ to avoid unchecked casts and ensure a more robust codebase. Here are some effective tips to help you write Java programs that are type-safe and free from unchecked cast exceptions.
- Use generic classes, interfaces, and methods to ensure that your code handles compatible types without relying on casting.
- Embrace polymorphism by utilizing abstract classes and interfaces, define common behavior and interact with objects through their common type.
- Check the type of an object using the instanceof operator. This allows you to verify that an object is of the expected type before proceeding with the cast.
- Favor composition over inheritance, where classes contain references to other classes as instance variables.
- Familiarize yourself with design patterns that promote type safety and avoid unchecked casts. Patterns such as Factory Method, Builder, and Strategy provide alternative approaches to object creation and behavior, often eliminating the need for explicit casting.
- Clearly define the contracts and preconditions for your methods. A well-defined contract helps ensure that the method is called with appropriate types, improving overall code safety.
- Refactor your code and improve its overall design. Look for opportunities to apply the aforementioned tips, such as utilizing generics, polymorphism, or design patterns.
Unchecked casts in Java programs can introduce runtime errors and undermine type safety. By adopting practices like using generics, leveraging polymorphism, checking types with instanceof, favoring composition over inheritance, reviewing design patterns, employing design by contract, and improving code design, you can avoid unchecked casts and enhance the robustness of your Java programs. Prioritizing type safety will result in more reliable code and a smoother development process.
Lightly IDE as a Programming Learning Platform
So, you want to learn a new programming language? Don't worry, it's not like climbing Mount Everest. With Lightly IDE, you'll feel like a coding pro in no time. With Lightly IDE , you don't need to be a coding wizard to start programming.
One of its standout features is its intuitive design, which makes it easy to use even if you're a technologically challenged unicorn. With just a few clicks, you can become a programming wizard in Lightly IDE. It's like magic, but with less wands and more code.
If you're looking to dip your toes into the world of programming or just want to pretend like you know what you're doing, Lightly IDE's online Java compiler is the perfect place to start. It's like a playground for programming geniuses in the making! Even if you're a total newbie, this platform will make you feel like a coding superstar in no time.
Read more: How to Avoid Unchecked Casts in Java Programs
Top comments (0)
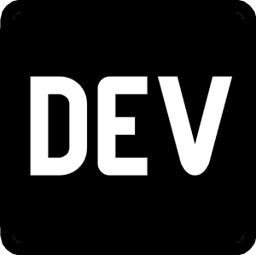
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
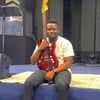
Spring Boot Annotations
Oloruntobi Ajayi - May 23
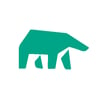
Why Every Business May Soon Need an AI Agent
MindsDB Team - May 23
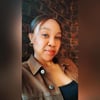
React Hooks?
Thatohatsi Matshidiso Tilodi - May 23
Introduction to Spring Boot
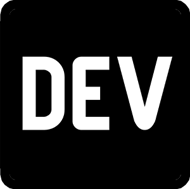
We're a place where coders share, stay up-to-date and grow their careers.
- Watch & Listen
- Oracle University
Next in the Series: Type Inference
Introducing Generics
Why use generics.
In a nutshell, generics enable types (classes and interfaces) to be parameters when defining classes, interfaces and methods. Much like the more familiar formal parameters used in method declarations, type parameters provide a way for you to re-use the same code with different inputs. The difference is that the inputs to formal parameters are values, while the inputs to type parameters are types.
Code that uses generics has many benefits over non-generic code:
Stronger type checks at compile time. A Java compiler applies strong type checking to generic code and issues errors if the code violates type safety. Fixing compile-time errors is easier than fixing runtime errors, which can be difficult to find.
Elimination of casts. The following code snippet without generics requires casting:
When re-written to use generics, the code does not require casting:
- Enabling programmers to implement generic algorithms. By using generics, programmers can implement generic algorithms that work on collections of different types, can be customized, and are type safe and easier to read.
Generic Types
A simple box class.
A generic type is a generic class or interface that is parameterized over types. The following Box class will be modified to demonstrate the concept.
Since its methods accept or return an Object , you are free to pass in whatever you want, provided that it is not one of the primitive types. There is no way to verify, at compile time, how the class is used. One part of the code may place an Integer in the box and expect to get objects of type Integer out of it, while another part of the code may mistakenly pass in a String , resulting in a runtime error.
A Generic Version of the Box Class
A generic class is defined with the following format:
The type parameter section, delimited by angle brackets ( <> ), follows the class name. It specifies the type parameters (also called type variables) T1 , T2 , ..., and Tn .
To update the Box class to use generics, you create a generic type declaration by changing the code " public class Box " to " public class Box<T> ". This introduces the type variable, T , that can be used anywhere inside the class.
With this change, the Box class becomes:
As you can see, all occurrences of Object are replaced by T . A type variable can be any non-primitive type you specify: any class type, any interface type, any array type, or even another type variable.
This same technique can be applied to create generic interfaces.
Type Parameter Naming Conventions
By convention, type parameter names are single, uppercase letters. This stands in sharp contrast to the variable naming conventions that you already know about, and with good reason: without this convention, it would be difficult to tell the difference between a type variable and an ordinary class or interface name.
The most commonly used type parameter names are:
E - Element (used extensively by the Java Collections Framework)
S, U, V etc. - 2nd, 3rd, 4th types
You will see these names used throughout the Java SE API and the rest of this section.
Invoking and Instantiating a Generic Type
To reference the generic Box class from within your code, you must perform a generic type invocation, which replaces T with some concrete value, such as Integer :
You can think of a generic type invocation as being similar to an ordinary method invocation, but instead of passing an argument to a method, you are passing a type argument — Integer in this case — to the Box class itself.
Type Parameter and Type Argument Terminology : Many developers use the terms "type parameter" and "type argument" interchangeably, but these terms are not the same. When coding, one provides type arguments in order to create a parameterized type. Therefore, the T in Foo<T> is a type parameter and the String in Foo<String> f is a type argument. This section observes this definition when using these terms.
Like any other variable declaration, this code does not actually create a new Box object. It simply declares that integerBox will hold a reference to a "Box of Integer", which is how Box<Integer> is read.
An invocation of a generic type is generally known as a parameterized type.
To instantiate this class, use the new keyword, as usual, but place <Integer> between the class name and the parenthesis:
The Diamond
In Java SE 7 and later, you can replace the type arguments required to invoke the constructor of a generic class with an empty set of type arguments ( <> ) as long as the compiler can determine, or infer, the type arguments from the context. This pair of angle brackets, <> , is informally called the diamond. For example, you can create an instance of Box<Integer> with the following statement:
For more information on diamond notation and type inference, see the Type Inference section of this tutorial.
Multiple Type Parameters
As mentioned previously, a generic class can have multiple type parameters. For example, the generic OrderedPair class, which implements the generic Pair interface:
The following statements create two instantiations of the OrderedPair class:
The code, new OrderedPair<String, Integer>() , instantiates K as a String and V as an Integer . Therefore, the parameter types of OrderedPair 's constructor are String and Integer , respectively. Due to autoboxing, it is valid to pass a String and an int to the class.
As mentioned in The Diamond section, because a Java compiler can infer the K and V types from the declaration OrderedPair<String, Integer> , these statements can be shortened using diamond notation:
To create a generic interface, follow the same conventions as for creating a generic class.
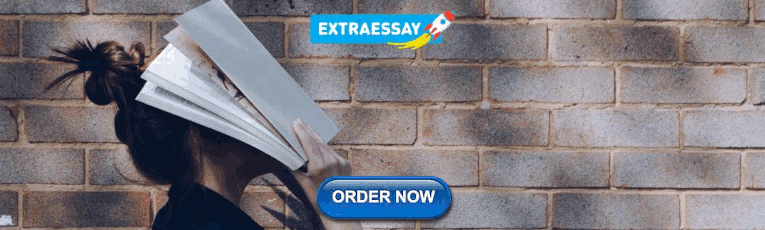
Parameterized Types
You can also substitute a type parameter (that is, K or V ) with a parameterized type, that is, List<String> . For example, using the OrderedPair<K, V> example:
A raw type is the name of a generic class or interface without any type arguments. For example, given the generic Box class:
To create a parameterized type of Box<T> , you supply an actual type argument for the formal type parameter T :
If the actual type argument is omitted, you create a raw type of Box<T> :
Therefore, Box is the raw type of the generic type Box<T> . However, a non-generic class or interface type is not a raw type.
Raw types show up in legacy code because lots of API classes (such as the Collections classes) were not generic prior to JDK 5.0. When using raw types, you essentially get pre-generics behavior — a Box gives you Objects. For backward compatibility, assigning a parameterized type to its raw type is allowed:
But if you assign a raw type to a parameterized type, you get a warning:
You also get a warning if you use a raw type to invoke generic methods defined in the corresponding generic type:
The warning shows that raw types bypass generic type checks, deferring the catch of unsafe code to runtime. Therefore, you should avoid using raw types.
The Type Erasure section has more information on how the Java compiler uses raw types.
Unchecked Error Messages
As mentioned previously, when mixing legacy code with generic code, you may encounter warning messages similar to the following:
This can happen when using an older API that operates on raw types, as shown in the following example:
The term "unchecked" means that the compiler does not have enough type information to perform all type checks necessary to ensure type safety. The "unchecked" warning is disabled, by default, though the compiler gives a hint. To see all "unchecked" warnings, recompile with -Xlint:unchecked .
Recompiling the previous example with -Xlint:unchecked reveals the following additional information:
To completely disable unchecked warnings, use the -Xlint:-unchecked flag. The @SuppressWarnings("unchecked") annotation suppresses unchecked warnings. If you are unfamiliar with the @SuppressWarnings syntax, see the section Annotations.
Generic Methods
Generic methods are methods that introduce their own type parameters. This is similar to declaring a generic type, but the type parameter's scope is limited to the method where it is declared. Static and non-static generic methods are allowed, as well as generic class constructors.
The syntax for a generic method includes a list of type parameters, inside angle brackets, which appears before the method's return type. For static generic methods, the type parameter section must appear before the method's return type.
The Util class includes a generic method, compare, which compares two Pair objects:
The complete syntax for invoking this method would be:
The type has been explicitly provided, as shown in bold. Generally, this can be left out and the compiler will infer the type that is needed:
This feature, known as type inference, allows you to invoke a generic method as an ordinary method, without specifying a type between angle brackets. This topic is further discussed in the following section, Type Inference.
Bounded Type Parameters
There may be times when you want to restrict the types that can be used as type arguments in a parameterized type. For example, a method that operates on numbers might only want to accept instances of Number or its subclasses. This is what bounded type parameters are for.
To declare a bounded type parameter, list the type parameter's name, followed by the extends keyword, followed by its upper bound, which in this example is Number . Note that, in this context, extends is used in a general sense to mean either " extends " (as in classes) or " implements " (as in interfaces).
By modifying our generic method to include this bounded type parameter, compilation will now fail, since our invocation of inspect still includes a String :
In addition to limiting the types you can use to instantiate a generic type, bounded type parameters allow you to invoke methods defined in the bounds:
The isEven() method invokes the intValue() method defined in the Integer class through n .
Multiple Bounds
The preceding example illustrates the use of a type parameter with a single bound, but a type parameter can have multiple bounds:
A type variable with multiple bounds is a subtype of all the types listed in the bound. If one of the bounds is a class, it must be specified first. For example:
If bound A is not specified first, you get a compile-time error:
Generic Methods and Bounded Type Parameters
Bounded type parameters are key to the implementation of generic algorithms. Consider the following method that counts the number of elements in an array T[] that are greater than a specified element elem .
The implementation of the method is straightforward, but it does not compile because the greater than operator ( > ) applies only to primitive types such as short , int , double , long , float , byte , and char . You cannot use the > operator to compare objects. To fix the problem, use a type parameter bounded by the Comparable<T> interface:
The resulting code will be:
Generics, Inheritance, and Subtypes
As you already know, it is possible to assign an object of one type to an object of another type provided that the types are compatible. For example, you can assign an Integer to an Object , since Object is one of Integer 's supertypes:
In object-oriented terminology, this is called an "is a" relationship. Since an Integer is a kind of Object, the assignment is allowed. But Integer is also a kind of Number , so the following code is valid as well:
The same is also true with generics. You can perform a generic type invocation, passing Number as its type argument, and any subsequent invocation of add will be allowed if the argument is compatible with Number :
Now consider the following method:
What type of argument does it accept? By looking at its signature, you can see that it accepts a single argument whose type is Box<Number> . But what does that mean? Are you allowed to pass in Box<Integer> or Box<Double> , as you might expect? The answer is "no", because Box<Integer> and Box<Double> are not subtypes of Box<Number> .
This is a common misunderstanding when it comes to programming with generics, but it is an important concept to learn. Box<Integer> is not a subtype of Box<Number> even though Integer is a subtype of Number .
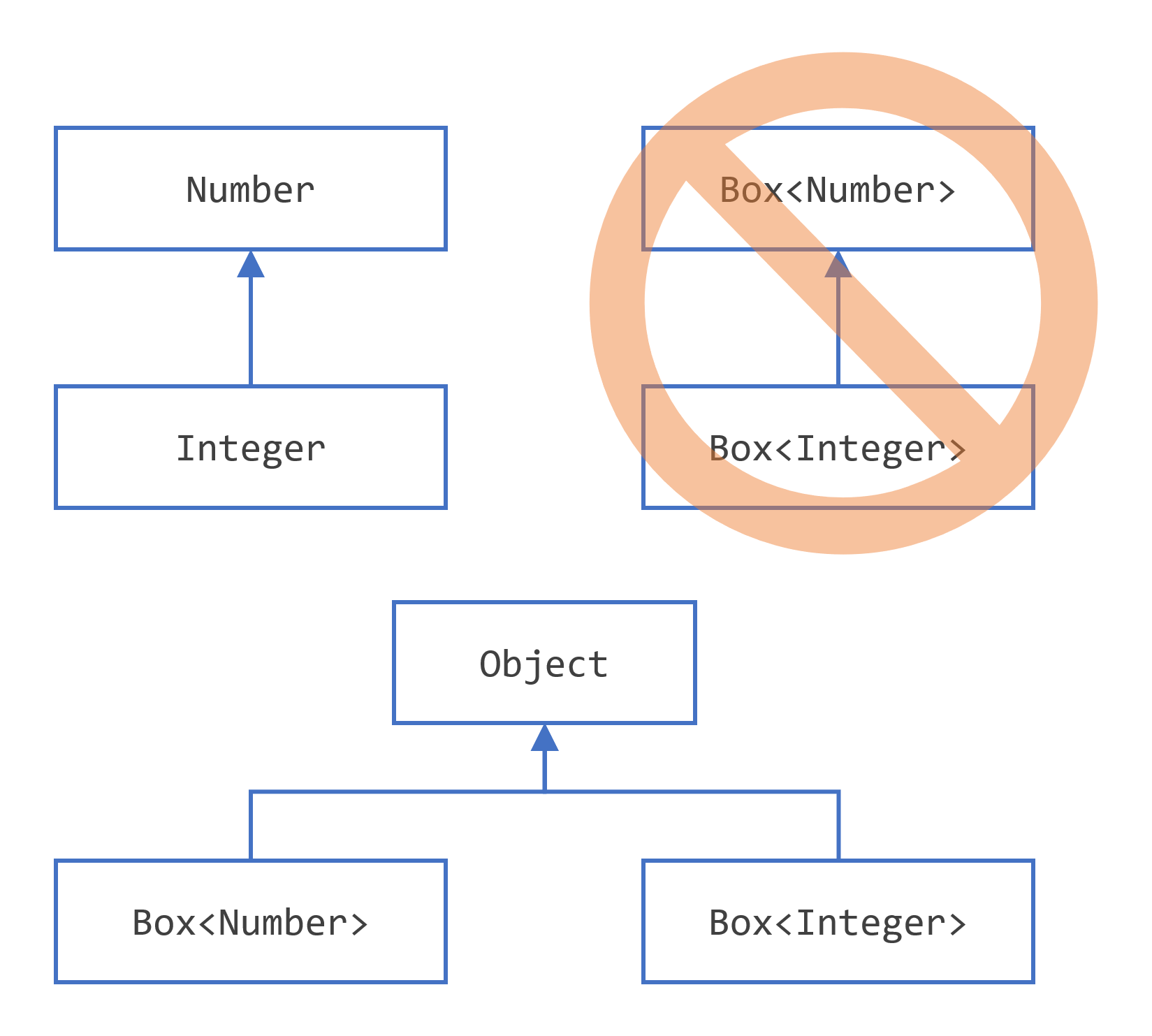
Note: Given two concrete types A and B , for example, Number and Integer , MyClass<A> has no relationship to MyClass<B> , regardless of whether or not A and B are related. The common parent of MyClass<A> and MyClass<B> is Object .
For information on how to create a subtype-like relationship between two generic classes when the type parameters are related, see the section Wildcards and Subtyping .
Generic Classes and Subtyping
You can subtype a generic class or interface by extending or implementing it. The relationship between the type parameters of one class or interface and the type parameters of another are determined by the extends and implements clauses.
Using the Collections classes as an example, ArrayList<E> implements List<E> , and List<E> extends Collection<E> . So ArrayList<String> is a subtype of List<String> , which is a subtype of Collection<String> . So long as you do not vary the type argument, the subtyping relationship is preserved between the types.
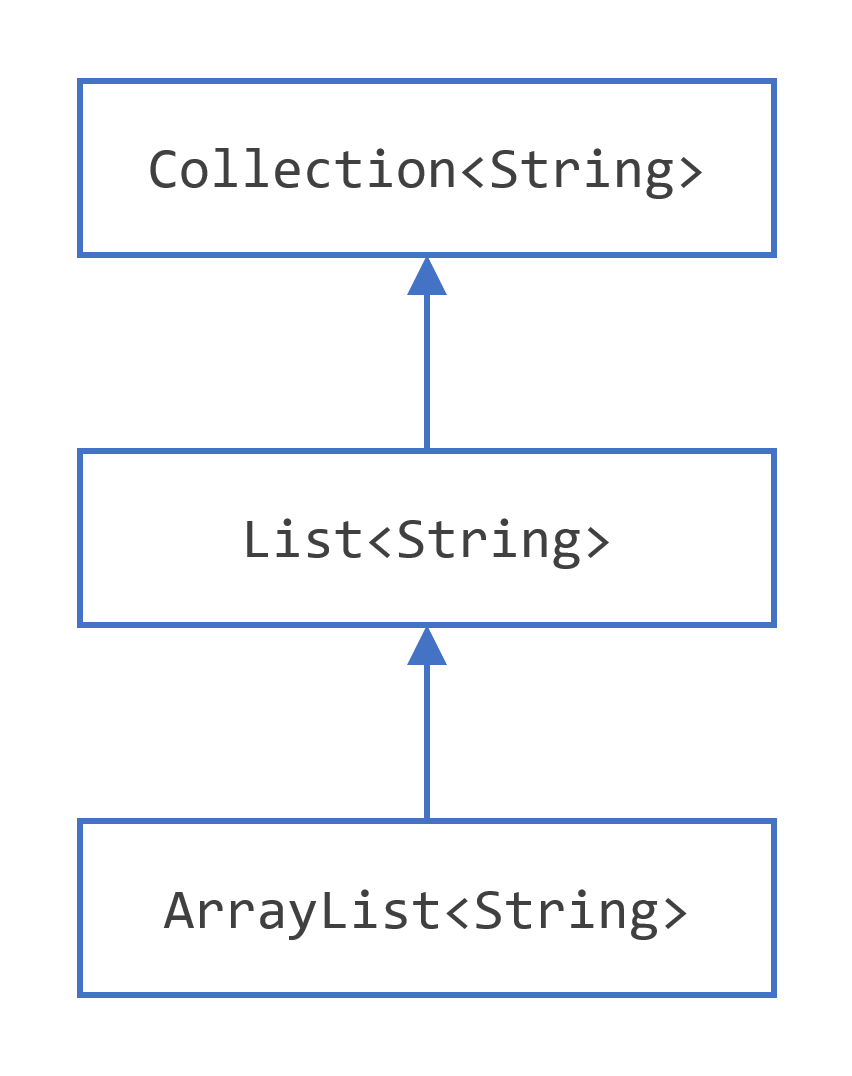
Now imagine we want to define our own list interface, PayloadList , that associates an optional value of generic type P with each element. Its declaration might look like:
The following parameterizations of PayloadList are subtypes of List<String> :
- PayloadList<String,String>
- PayloadList<String,Integer>
- PayloadList<String,Exception>
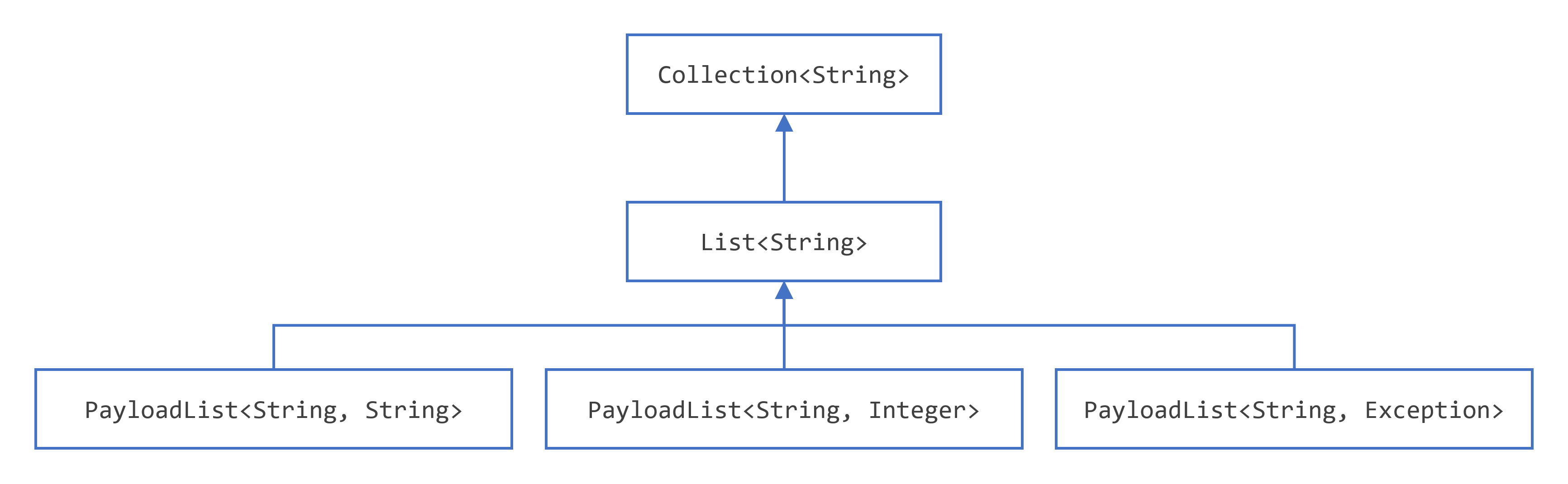
In this tutorial
Last update: September 14, 2021
Generics unchecked assignment

SCJP 1.4 - SCJP 6 - SCWCD 5 - OCEEJBD 6 - OCEJPAD 6 How To Ask Questions How To Answer Questions
Technicalities - Under The Hood Of The Compiler
Under the hood of the compiler, compiler messages, what is an "unchecked" warning, what is the -xlint:unchecked compiler option, what is the suppresswarnings annotation, how can i avoid "unchecked cast" warnings, is it possible to eliminate all "unchecked" warnings, why do i get an "unchecked" warning although there is no type information missing, heap pollution, type erasure, what is type erasure, what is reification, what is a bridge method, under which circumstances is a bridge method generated, why does the compiler add casts when it translates generics, how does type erasure work when a type parameter has several bounds, what is a reifiable type, what is the type erasure of a parameterized type, what is the type erasure of a type parameter, what is the type erasure of a generic method, is generic code faster or slower than non-generic code, how do i compile generics for use with jdk <= 1.4, type system, how do parameterized types fit into the java type system, how do instantiations of a generic type relate to instantiations of other generic types that have the same type argument, how do unbounded wildcard instantiations of a generic type relate to other instantiations of the same generic type, how do wildcard instantiations with an upper bound relate to other instantiations of the same generic type, how do wildcard instantiations with a lower bound relate to other instantiations of the same generic type, which super-subtype relationships exist among instantiations of generic types, which super-subset relationships exist among wildcards.
- A wildcard with an upper bound A denotes a superset of another wildcard with an upper bound B, if A denotes a super set of B.
- A wildcard with a lower bound A denotes a superset of another wildcard with a lower bound B, if A denotes a sub set of B.
Does "extends" always mean "inheritance"?
Exception handling, can i use generic or parameterized types in exception handling, why are generic exception and error types illegal, can i use a type parameter in exception handling, can i use a type parameter in a catch clause, can i use a type parameter in in a throws clause, can i throw an object whose type is a type parameter, static context, how do i refer to static members of a parameterized type, how do i refer to a (non-static) inner class of a generic type, how do i refer to an interface type nested into a generic type, how do i refer to an enum type nested into a generic type, can i import a particular parameterized type, why are generic enum types illegal, type argument inference, what is type argument inference, is there a correspondence between type inference for method invocation and type inference for instance creation, what is the "diamond" operator, what is type argument inference for instance creation expressions, why does the type inference for an instance creation expression fail, what is type argument inference for generic methods, why doesn't type argument inference fail when i provide inconsistent method arguments, why do temporary variables matter in case of invocation of generic methods, wildcard capture, what is the capture of a wildcard, what is the capture of an unbounded wildcard compatible to, is the capture of a bounded wildcard compatible to the bound, wildcard instantiations, which methods and fields are accessible/inaccessible through a reference variable of a wildcard type, which methods that use the type parameter in the argument or return type are accessible in an unbounded wildcard parameterized type, which methods that use the type parameter in the argument or return type are accessible in an upper bound wildcard parameterized type, which methods that use the type parameter in the argument or return type are accessible in a lower bound wildcard parameterized type, which methods that use the type parameter as type argument of a parameterized argument or return type are accessible in a wildcard parameterized type, which methods that use the type parameter as upper wildcard bound in a parameterized argument or return type are accessible in a wildcard parameterized type, which methods that use the type parameter as lower wildcard bound in a parameterized argument or return type are accessible in a wildcard parameterized type, in a wildcard parameterized type, can i read and write fields whose type is the type parameter, cast and instanceof, which types can or must not appear as target type in an instanceof expression, overloading and overriding, what is method overriding, what is method overloading, what is the @override annotation, what is a method signature, what is a subsignature, what are override-equivalent signatures, when does a method override its supertype's method.
class Super { public void method( String arg) { ... } } class Sub extends Super { public void method( String arg) { ... } // overrides }
class Super { public void printCollection( Collection <?> c) { ... } } class Sub extends Super { public void printCollection( Collection c) { ... } // overrides }
- the subtype method's return type must be substitutable for the supertype method's return type, and
- the throws clauses of both methods must not be in conflict.
class Super { public void method(String arg) { ... } } class Sub extends Super { public int method(String arg) { ... } // error } error: method(String) in Sub cannot override method(String) in Super; attempting to use incompatible return type found : int required: void public int method(String arg) { ... } ^
- both type are void
- both types are the same primitive type
- R Sub is identical to R Super
- R Sub is a subtype of R Super
- R Sub is a raw type that is identical to the erasure of R Super
- R Sub is a raw type that is a subtype of the erasure of R Super
class Super { public Super copy() { ... } public List<String> getList() { ... } public Map getLinks() { ... } } class Sub extends Super { public Sub copy() { ... } // overrides public ArrayList<String> getList() { ... } // overrides public Map<String,File> getLinks() { ... } // overrides }
class Super { public Class<?> getType() { ... } public Map<String,File> getLinks() { ... } } class Sub extends Super { public Class getType() { ... } // overrides public Map getLinks() { ... } // overrides with unchecked warning }
warning: getLinks() in Sub overrides getLinks() in Super; return type requires unchecked conversion found : java.util.Map required: java.util.Map<java.lang.String,java.io.File> public Map getLinks() { ... } ^
class Super { public void method() { ... } } class Sub extends Super { public void method() throws UserException { ... } // error } error: method() in Sub cannot override method() in Super; overridden method does not throw UserException public void method() throws UserException { ... } ^
class Super { public void method() throws IOException, InterruptedException { ... } } class Sub extends Super { public void method() throws FileNotFoundException { ... } // overrides }
What are covariant return types?
What are substitutable return types, can a method of a non-generic subtype override a method of a generic supertype, can a method of a generic subtype override a method of a generic supertype, can a generic method override a generic one, can a non-generic method override a generic one, can a generic method override a non-generic one, what is overload resolution.
- Unchecked Cast in Java
- Java Howtos
What Is the Unchecked Cast Warning in Java
Understanding unchecked cast warnings in java, preventing unchecked cast warnings, best practices for preventing unchecked cast warnings in java.
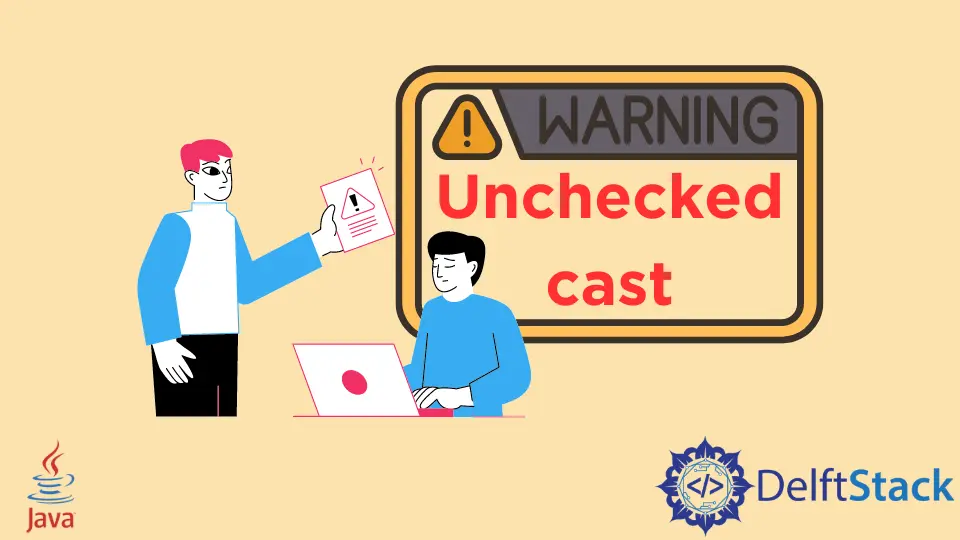
Java is a programming language that enforces type safety, which means that we should always specify the type of data that we are going to store or use and cannot store incompatible types in them.
Discover how to prevent unchecked cast warnings in Java. Explore the causes, solutions, and best practices for ensuring type safety and reliability in your code.
An unchecked cast warning in Java occurs when the compiler cannot ensure type safety during a casting operation. It warns the developer about potential runtime errors, such as ClassCastException , that may occur due to type mismatches.
Unchecked cast warnings typically arise when casting from a generic type to a specific type or when casting to a parameterized type without proper type checking. Addressing these warnings is crucial to ensure code reliability and prevent unexpected runtime errors.
In Java programming, unchecked cast warnings are common occurrences that indicate potential type safety issues in your code. Let’s delve into two significant causes of unchecked cast warnings:
Understanding these causes is crucial for maintaining code quality and preventing unexpected runtime errors. Let’s explore solutions to address unchecked cast warnings in Java code.
In Java programming, unchecked cast warnings signify potential type safety issues that can lead to runtime errors if not addressed properly. Direct casting from raw types and casting without type checking are common scenarios where unchecked cast warnings occur.
Understanding how to prevent these warnings is crucial for maintaining code integrity and preventing unexpected runtime errors. In this section, we’ll explore effective techniques to prevent unchecked cast warnings in Java by addressing direct casting from raw types and casting without proper type checking.
Code Example:
The code example provided illustrates two common scenarios in Java where unchecked cast warnings can arise: direct casting from raw types and casting without proper type checking.
In the first scenario, a raw ArrayList named rawList is instantiated, and a String ( Hello ) is added to it. Initially, an attempt is made to directly cast rawList to a parameterized type List<String> .
Such direct casting from a raw type can trigger unchecked cast warnings as it bypasses type safety checks. To address this, we adopt a safer approach by creating a new ArrayList , stringList1 .
By passing rawList as a parameter to its constructor, we ensure that stringList1 maintains the correct generic type. This action effectively prevents unchecked cast warnings, ensuring type safety throughout the code.
In the second scenario, an Object ( obj ) is assigned the value World . There is an initial attempt to cast obj directly to List<String> without performing proper type checking.
Such casting without type checking can lead to unchecked cast warnings as it lacks verification of type compatibility. To mitigate this risk, we instantiate a new ArrayList ( stringList2 ) and add obj after performing necessary type checking and casting.
By ensuring that the object being added to stringList2 is indeed a String type, we maintain type safety and avoid unchecked cast warnings.
By employing these techniques, developers can effectively prevent unchecked cast warnings in their Java code, thereby enhancing type safety and reducing the likelihood of unexpected runtime errors.
The code will produce the following output:

By following the demonstrated techniques, developers can effectively prevent unchecked cast warnings in Java code, ensuring type safety and reducing the risk of unexpected runtime errors. Understanding and implementing these practices are essential for maintaining code reliability and integrity in Java applications.
Use Generics Consistently
Utilize parameterized types (generics) consistently throughout your code to ensure type safety and prevent unchecked cast warnings.
Perform Type Checking Before Casting
Always perform proper type checking before casting objects to parameterized types to ensure compatibility and prevent unchecked cast warnings.
Avoid Raw Types
Minimize the use of raw types and prefer parameterized types whenever possible to maintain type safety and prevent unchecked cast warnings.
Consider Type Inference
Leverage type inference where applicable to automatically determine generic types and reduce the likelihood of unchecked cast warnings.
Review and Test Code
Regularly review and test your code to identify and address any instances of unchecked cast warnings, ensuring robustness and reliability.
Unchecked cast warnings in Java signal potential type safety issues arising from direct casting from raw types and casting without proper type checking. The causes include direct casting from raw types and casting without type checking.
Solutions involve creating new parameterized types instead of directly casting from raw types and performing type checking before casting. Best practices include using generics consistently, avoiding raw types, considering type inference, and regularly reviewing and testing code.
By implementing these strategies, developers can ensure type safety and prevent unchecked cast warnings in Java programs.
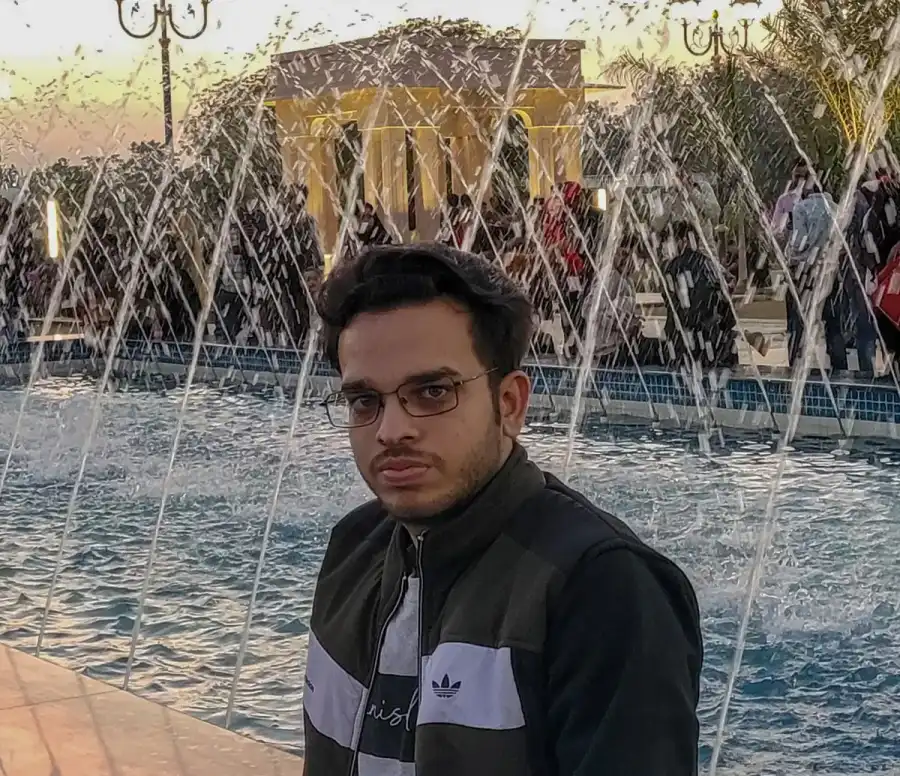
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
Non-generic Vs Generic Collection in Java
- Garbage Collection in Java
- Need of Concurrent Collections in java
- Iterator vs Collection in Java
- Java Collection Exercise
- Collections.nCopies() in Java
- Collections enumeration() method in Java with Examples
- Collection vs Collections in Java with Example
- Why We Need Collection Framework in Java?
- Generic For Loop in Java
- Java.util.Collections.frequency() in Java
- Java Convenience Factory Methods for Collections
- Collections.sort() in Java with Examples
- How to Compare two Collections in Java?
- C++ STL vs Java Collections Framework
- Collection contains() method in Java with Examples
- Convert an Iterable to Collection in Java
- Templates in C++ vs Generics in Java
- Collection Interface in Java with Examples
- Collections in Java
We will be discussing differences later prior let us understand what is generic Collection and non-generic Collection, and most importantly dealing with the implementation part as during implementation one can only really get the real understanding of the concept, henceforth the differences between them.
Generics are basically the errors appearing are compile-time than at run-time. there are certain advantages of generics over non-generic are as follows:
- Code Reuse: With help of Generics, one needs to write a method/class/interface only once and use it for any type whereas, in non-generics, the code needs to be written again and again whenever needed.
- Type Safety: Generics make errors to appear compile time than at run time (It’s always better to know problems in your code at compile time rather than making your code fail at run time).
Example: To create an ArrayList that store name of students and if by mistake programmer adds an integer object instead of string, the compiler allows it. But, when this data is retrieved from ArrayList, it causes problems at runtime for Non-generic ArrayList
Implementation:
Output:
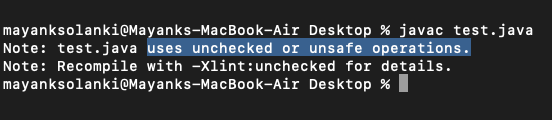
How generics solve this problem?
If this list was made Generic, then it would take only String objects and threw Compile Time Error in any other case.
Now moving forward, Individual Type Casting is not needed.
If Generics is not needed, then, in the above example every time the data is to be retrieved from ArrayList, it needs to be typecasted. Typecasting at every retrieval operation is a big headache. This can be avoided if somehow it is already known that the list only holds string data.
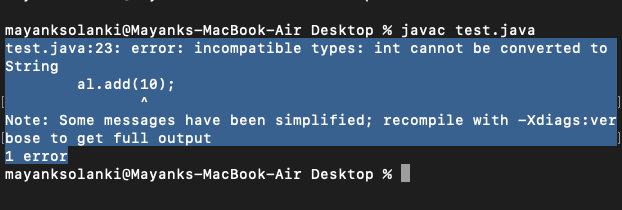
Geek , now you should be wondering how generics solve this problem?
If this list was made Generic, then it would take only String objects and would return only String objects while retrieval. And hence individual typecasting won’t be required. The above statement is justified
Note: With the help of generics, while one can implement algorithms Implementing generic algorithms, one can have t hat work on different types of objects and at the same they are type-safe too.
Do remember that there are some points, which will describe the difference between Generics and Non-Generic which are tabulated below in order to get a crisp understanding between them.
Please Login to comment...
Similar reads.
- Java-Collections
- Technical Scripter 2018
- Difference Between
- Technical Scripter
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
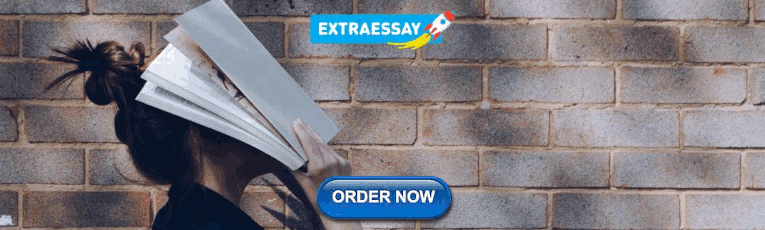
IMAGES
VIDEO
COMMENTS
Map<Integer, String> map = a.getMap(); gets you a warning now: "Unchecked assignment: 'java.util.Map to java.util.Map<java.lang.Integer, java.lang.String>'. Even though the signature of getMap is totally independent of T, and the code is unambiguous regarding the types the Map contains. I know that I can get rid of the warning by reimplementing ...
I'm using the following code to find a one-dimensional list of unique objects in an n-dimensional list (credits to someone on StackOverflow a while ago for the approach): public static <T> List<T> getUniqueObjectsInArray(List<T> array) {. Integer dimension = getDimensions(array); return getUniqueObjectsInArray(array, dimension);
5.2. Checking Type Conversion Before Using the Raw Type Collection. The warning message " unchecked conversion " implies that we should check the conversion before the assignment. To check the type conversion, we can go through the raw type collection and cast every element to our parameterized type.
The "unchecked cast" is a compile-time warning . Simply put, we'll see this warning when casting a raw type to a parameterized type without type checking. An example can explain it straightforwardly. Let's say we have a simple method to return a raw type Map: public class UncheckedCast {. public static Map getRawMap() {.
Unchecked assignment: java.util.List to java.util.List<String> ... Now it all works, and I don't have to fight those generic types when using the mock method (or, for that matter, ...
Unchecked cast refers to the process of converting a variable of one data type to another data type without checks by the Java compiler. This operation is unchecked because the compiler does not verify if the operation is valid or safe. Unchecked casts can lead to runtime errors, such as ClassCastException, when the program tries to assign an ...
transient Object[] elementData; Notice ArrayList uses Object as the element type. As our generic type isn't known until runtime, Object is used as the superclass of any type. It's worth noting that nearly all the operations in ArrayList can use this generic array, as they don't need to provide a strongly typed array to the outside world (except for one method, toArray).
Code that uses generics has many benefits over non-generic code: ... WarningDemo.java:4: warning: [unchecked] unchecked conversion found : Box required: Box<java.lang.Integer> bi = createBox(); ^ 1 warning ... this is called an "is a" relationship. Since an Integer is a kind of Object, the assignment is allowed. But Integer is also a kind of ...
When you cast an object to ArrayList<Vehicle>, the Java runtime environment can only ensure that the cast to ArrayList<something> succeeds, but due to backwards compatibility (Java 1.4 from 2002), the cast will succeed, no matter if the arraylist contains Integer or String or Vehicle objects. Therefore, in this situation, the compiler warning ...
The most generic way of using generics is. This causes "Unchecked assignment: 'java.util.Set' to 'java.util.Collection <?
When source code is compiled for use in Java 5.0 that was developed before Java 5.0 and uses classes that are generic in Java 5.0, then "unchecked" warnings are inevitable. For instance, if "legacy ... - The third new-expression demonstrates that the lefthand side of the assignment is indeed ignored (in Java 7). The compiler again infers ...
The code example provided illustrates two common scenarios in Java where unchecked cast warnings can arise: direct casting from raw types and casting without proper type checking.. In the first scenario, a raw ArrayList named rawList is instantiated, and a String (Hello) is added to it.Initially, an attempt is made to directly cast rawList to a parameterized type List<String>.
It is an annotation to suppress compile warnings about unchecked generic operations (not exceptions), such as casts. It essentially implies that the programmer did not wish to be notified about these which he is already aware of when compiling a particular bit of code. ... warning: [unchecked] unchecked cast found : java.lang.Object required ...
The more experience you acquire with generics, the fewer warnings you'll get, but don't expect newly written code to compile cleanly. Many unchecked warnings are easy to eliminate. For example, suppose you accidentally write this declaration: Set<Lark> exaltation = new HashSet(); The compiler will gently remind you what you did wrong:
An unchecked cast warning in Java occurs when the compiler cannot verify that a cast is safe at compile time. This can happen when you are casting an object to a type that is not a supertype or subtype of the object's actual type. To address an unchecked cast warning, you can either suppress the warning using the @SuppressWarnings("unchecked ...
Unchecked casts are a common source of Java program errors. Here are some examples of unchecked casts: List names = (List) obj; // cast Object to List. This cast statement above can result in a ...
I don't put this in as an answer because those are Java basics. You obviously have to put in the type for the definition as well: new List<String>[arrayLength] - Smutje
Do remember that there are some points, which will describe the difference between Generics and Non-Generic which are tabulated below in order to get a crisp understanding between them. Base. Non-generic Collection. Generic Collection. Syntax. ArrayList list = new ArrayList (); ArrayList<ReferenceType> list = new ArrayList<ReferenceType> ();
Of course that's an unchecked assignment. You seem to be implementing a heterogeneous map. Java (and any other strongly-typed language) has no way to express the value type of your map in a statically-type-safe way. That is because the element types are only known at runtime.