Destructuring assignment
The two most used data structures in JavaScript are Object and Array .
- Objects allow us to create a single entity that stores data items by key.
- Arrays allow us to gather data items into an ordered list.
However, when we pass these to a function, we may not need all of it. The function might only require certain elements or properties.
Destructuring assignment is a special syntax that allows us to “unpack” arrays or objects into a bunch of variables, as sometimes that’s more convenient.
Destructuring also works well with complex functions that have a lot of parameters, default values, and so on. Soon we’ll see that.
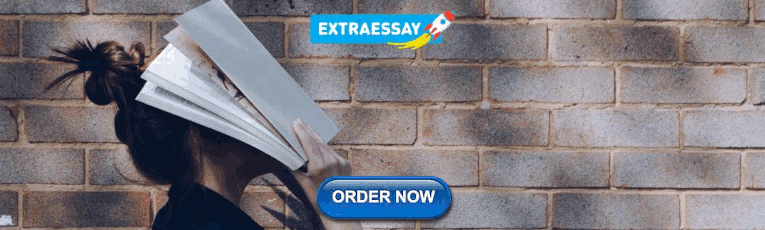
Array destructuring
Here’s an example of how an array is destructured into variables:
Now we can work with variables instead of array members.
It looks great when combined with split or other array-returning methods:
As you can see, the syntax is simple. There are several peculiar details though. Let’s see more examples to understand it better.
It’s called “destructuring assignment,” because it “destructurizes” by copying items into variables. However, the array itself is not modified.
It’s just a shorter way to write:
Unwanted elements of the array can also be thrown away via an extra comma:
In the code above, the second element of the array is skipped, the third one is assigned to title , and the rest of the array items are also skipped (as there are no variables for them).
…Actually, we can use it with any iterable, not only arrays:
That works, because internally a destructuring assignment works by iterating over the right value. It’s a kind of syntax sugar for calling for..of over the value to the right of = and assigning the values.
We can use any “assignables” on the left side.
For instance, an object property:
In the previous chapter, we saw the Object.entries(obj) method.
We can use it with destructuring to loop over the keys-and-values of an object:
The similar code for a Map is simpler, as it’s iterable:
There’s a well-known trick for swapping values of two variables using a destructuring assignment:
Here we create a temporary array of two variables and immediately destructure it in swapped order.
We can swap more than two variables this way.
The rest ‘…’
Usually, if the array is longer than the list at the left, the “extra” items are omitted.
For example, here only two items are taken, and the rest is just ignored:
If we’d like also to gather all that follows – we can add one more parameter that gets “the rest” using three dots "..." :
The value of rest is the array of the remaining array elements.
We can use any other variable name in place of rest , just make sure it has three dots before it and goes last in the destructuring assignment.
Default values
If the array is shorter than the list of variables on the left, there will be no errors. Absent values are considered undefined:
If we want a “default” value to replace the missing one, we can provide it using = :
Default values can be more complex expressions or even function calls. They are evaluated only if the value is not provided.
For instance, here we use the prompt function for two defaults:
Please note: the prompt will run only for the missing value ( surname ).
Object destructuring
The destructuring assignment also works with objects.
The basic syntax is:
We should have an existing object on the right side, that we want to split into variables. The left side contains an object-like “pattern” for corresponding properties. In the simplest case, that’s a list of variable names in {...} .
For instance:
Properties options.title , options.width and options.height are assigned to the corresponding variables.
The order does not matter. This works too:
The pattern on the left side may be more complex and specify the mapping between properties and variables.
If we want to assign a property to a variable with another name, for instance, make options.width go into the variable named w , then we can set the variable name using a colon:
The colon shows “what : goes where”. In the example above the property width goes to w , property height goes to h , and title is assigned to the same name.
For potentially missing properties we can set default values using "=" , like this:
Just like with arrays or function parameters, default values can be any expressions or even function calls. They will be evaluated if the value is not provided.
In the code below prompt asks for width , but not for title :
We also can combine both the colon and equality:
If we have a complex object with many properties, we can extract only what we need:
The rest pattern “…”
What if the object has more properties than we have variables? Can we take some and then assign the “rest” somewhere?
We can use the rest pattern, just like we did with arrays. It’s not supported by some older browsers (IE, use Babel to polyfill it), but works in modern ones.
It looks like this:
In the examples above variables were declared right in the assignment: let {…} = {…} . Of course, we could use existing variables too, without let . But there’s a catch.
This won’t work:
The problem is that JavaScript treats {...} in the main code flow (not inside another expression) as a code block. Such code blocks can be used to group statements, like this:
So here JavaScript assumes that we have a code block, that’s why there’s an error. We want destructuring instead.
To show JavaScript that it’s not a code block, we can wrap the expression in parentheses (...) :
Nested destructuring
If an object or an array contains other nested objects and arrays, we can use more complex left-side patterns to extract deeper portions.
In the code below options has another object in the property size and an array in the property items . The pattern on the left side of the assignment has the same structure to extract values from them:
All properties of options object except extra which is absent in the left part, are assigned to corresponding variables:
Finally, we have width , height , item1 , item2 and title from the default value.
Note that there are no variables for size and items , as we take their content instead.
Smart function parameters
There are times when a function has many parameters, most of which are optional. That’s especially true for user interfaces. Imagine a function that creates a menu. It may have a width, a height, a title, an item list and so on.
Here’s a bad way to write such a function:
In real-life, the problem is how to remember the order of arguments. Usually, IDEs try to help us, especially if the code is well-documented, but still… Another problem is how to call a function when most parameters are ok by default.
That’s ugly. And becomes unreadable when we deal with more parameters.
Destructuring comes to the rescue!
We can pass parameters as an object, and the function immediately destructurizes them into variables:
We can also use more complex destructuring with nested objects and colon mappings:
The full syntax is the same as for a destructuring assignment:
Then, for an object of parameters, there will be a variable varName for the property incomingProperty , with defaultValue by default.
Please note that such destructuring assumes that showMenu() does have an argument. If we want all values by default, then we should specify an empty object:
We can fix this by making {} the default value for the whole object of parameters:
In the code above, the whole arguments object is {} by default, so there’s always something to destructurize.
Destructuring assignment allows for instantly mapping an object or array onto many variables.
The full object syntax:
This means that property prop should go into the variable varName and, if no such property exists, then the default value should be used.
Object properties that have no mapping are copied to the rest object.
The full array syntax:
The first item goes to item1 ; the second goes into item2 , and all the rest makes the array rest .
It’s possible to extract data from nested arrays/objects, for that the left side must have the same structure as the right one.
We have an object:
Write the destructuring assignment that reads:
- name property into the variable name .
- years property into the variable age .
- isAdmin property into the variable isAdmin (false, if no such property)
Here’s an example of the values after your assignment:
The maximal salary
There is a salaries object:
Create the function topSalary(salaries) that returns the name of the top-paid person.
- If salaries is empty, it should return null .
- If there are multiple top-paid persons, return any of them.
P.S. Use Object.entries and destructuring to iterate over key/value pairs.
Open a sandbox with tests.
Open the solution with tests in a sandbox.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
- Skip to main content
- Select language
- Skip to search
- Destructuring assignment
Unpacking values from a regular expression match
Es2015 version, invalid javascript identifier as a property name.
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables.
Description
The object and array literal expressions provide an easy way to create ad hoc packages of data.
The destructuring assignment uses similar syntax, but on the left-hand side of the assignment to define what values to unpack from the sourced variable.
This capability is similar to features present in languages such as Perl and Python.
Array destructuring
Basic variable assignment, assignment separate from declaration.
A variable can be assigned its value via destructuring separate from the variable's declaration.
Default values
A variable can be assigned a default, in the case that the value unpacked from the array is undefined .
Swapping variables
Two variables values can be swapped in one destructuring expression.
Without destructuring assignment, swapping two values requires a temporary variable (or, in some low-level languages, the XOR-swap trick ).
Parsing an array returned from a function
It's always been possible to return an array from a function. Destructuring can make working with an array return value more concise.
In this example, f() returns the values [1, 2] as its output, which can be parsed in a single line with destructuring.
Ignoring some returned values
You can ignore return values that you're not interested in:
You can also ignore all returned values:
Assigning the rest of an array to a variable
When destructuring an array, you can unpack and assign the remaining part of it to a variable using the rest pattern:
Note that a SyntaxError will be thrown if a trailing comma is used on the left-hand side with a rest element:
When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to unpack the parts out of this array easily, ignoring the full match if it is not needed.
Object destructuring
Basic assignment, assignment without declaration.
A variable can be assigned its value with destructuring separate from its declaration.
The ( .. ) around the assignment statement is required syntax when using object literal destructuring assignment without a declaration.
{a, b} = {a: 1, b: 2} is not valid stand-alone syntax, as the {a, b} on the left-hand side is considered a block and not an object literal.
However, ({a, b} = {a: 1, b: 2}) is valid, as is var {a, b} = {a: 1, b: 2}
NOTE: Your ( ..) expression needs to be preceded by a semicolon or it may be used to execute a function on the previous line.
Assigning to new variable names
A property can be unpacked from an object and assigned to a variable with a different name than the object property.
A variable can be assigned a default, in the case that the value unpacked from the object is undefined .
Setting a function parameter's default value
Es5 version, nested object and array destructuring, for of iteration and destructuring, unpacking fields from objects passed as function parameter.
This unpacks the id , displayName and firstName from the user object and prints them.
Computed object property names and destructuring
Computed property names, like on object literals , can be used with destructuring.
Rest in Object Destructuring
The Rest/Spread Properties for ECMAScript proposal (stage 3) adds the rest syntax to destructuring. Rest properties collect the remaining own enumerable property keys that are not already picked off by the destructuring pattern.
Destructuring can be used with property names that are not valid JavaScript identifiers by providing an alternative identifer that is valid.
Specifications
Specification | Status | Comment |
---|---|---|
Standard | Initial definition. | |
Draft | ||
Draft | Stage 3 draft. |
Browser compatibility
Feature | Chrome | Edge | Firefox (Gecko) | Internet Explorer | Opera | Safari |
---|---|---|---|---|---|---|
Basic support | 49.0 | 14 | (1.8.1) | No support | (Yes) | 7.1 |
Computed property names | 49.0 | 14 | (34) | No support | (Yes) | No support |
Rest in arrays | 49.0 | 12 | (34) | ? | ? | ? |
Rest in objects | 60.0 | No support | (55) | No support | No support | No support |
Feature | Android | Chrome for Android | Edge | Firefox Mobile (Gecko) | IE Mobile | Opera Mobile | Safari Mobile | Chrome for Android |
---|---|---|---|---|---|---|---|---|
Basic support | No support | 49.0 | (Yes) | 1.0 (1.0) | No support | No support | 8 | 49.0 |
Computed property names | No support | 49.0 | (Yes) | 34.0 (34) | No support | No support | No support | 49.0 |
Rest in arrays | No support | 49.0 | (Yes) | 34.0 (34) | ? | ? | ? | 49.0 |
Rest in objects | No support | No support | No support | 55.0 (55) | No support | No support | No support | No support |
[1] Requires "Enable experimental Javascript features" to be enabled under `about:flags`
Firefox-specific notes
- Firefox provided a non-standard language extension in JS1.7 for destructuring. This extension has been removed in Gecko 40 (Firefox 40 / Thunderbird 40 / SeaMonkey 2.37). See bug 1083498 .
- Starting with Gecko 41 (Firefox 41 / Thunderbird 41 / SeaMonkey 2.38) and to comply with the ES2015 specification, parenthesized destructuring patterns, like ([a, b]) = [1, 2] or ({a, b}) = { a: 1, b: 2 } , are now considered invalid and will throw a SyntaxError . See Jeff Walden's blog post and bug 1146136 for more details.
- Assignment operators
- "ES6 in Depth: Destructuring" on hacks.mozilla.org
Document Tags and Contributors
- Destructuring
- ECMAScript 2015
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Arithmetic operators
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
How to use destructuring assignment in JavaScript
Destructuring assignment is a powerful feature in JavaScript that allows you to extract values from arrays or properties from objects and assign them to variables in a concise and expressive way.
Destructuring assignment can be useful for:
- Reducing the amount of code and improving readability.
- Assigning default values to variables in case the source value is undefined.
- Renaming the variables that you assign from the source.
- Swapping the values of two variables without using a temporary variable.
- Extracting values from nested arrays or objects.
Array destructuring
Array destructuring in JavaScript is a syntax that allows you to extract individual elements from an array and assign them to distinct variables. Enclose the variables you want to assign values to within square brackets [] on the left-hand side of the assignment operator = , and place the array you want to destructure on the right-hand side.
Object destructuring
Object destructuring in JavaScript is a syntax that allows you to extract individual properties from an object and assign them to distinct variables in a single line of code, significantly reducing the boilerplate code needed compared to traditional methods like dot notation or bracket notation.
You might also like
A Guide to Variable Assignment and Mutation in JavaScript
Share this article
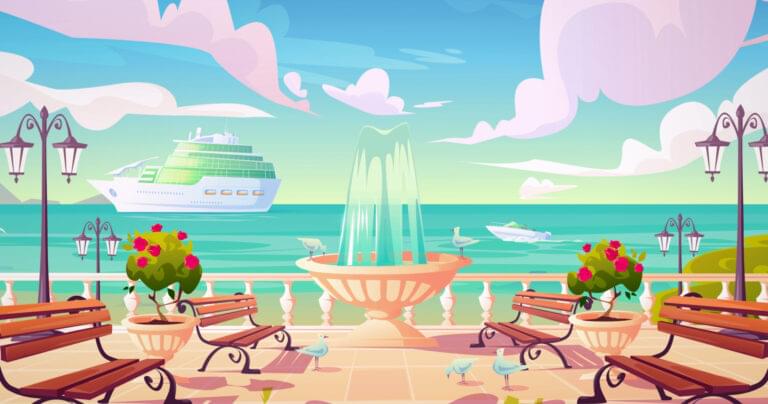
Variable Assignment
Variable reassignment, variable assignment by reference, copying by reference, the spread operator to the rescue, are mutations bad, frequently asked questions (faqs) about javascript variable assignment and mutation.
Mutations are something you hear about fairly often in the world of JavaScript, but what exactly are they, and are they as evil as they’re made out to be?
In this article, we’re going to cover the concepts of variable assignment and mutation and see why — together — they can be a real pain for developers. We’ll look at how to manage them to avoid problems, how to use as few as possible, and how to keep your code predictable.
If you’d like to explore this topic in greater detail, or get up to speed with modern JavaScript, check out the first chapter of my new book Learn to Code with JavaScript for free.
Let’s start by going back to the very basics of value types …
Every value in JavaScript is either a primitive value or an object. There are seven different primitive data types:
- numbers, such as 3 , 0 , -4 , 0.625
- strings, such as 'Hello' , "World" , `Hi` , ''
- Booleans, true and false
- symbols — a unique token that’s guaranteed never to clash with another symbol
- BigInt — for dealing with large integer values
Anything that isn’t a primitive value is an object , including arrays, dates, regular expressions and, of course, object literals. Functions are a special type of object. They are definitely objects, since they have properties and methods, but they’re also able to be called.
Variable assignment is one of the first things you learn in coding. For example, this is how we would assign the number 3 to the variable bears :
A common metaphor for variables is one of boxes with labels that have values placed inside them. The example above would be portrayed as a box containing the label “bears” with the value of 3 placed inside.
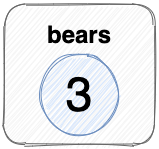
An alternative way of thinking about what happens is as a reference, that maps the label bears to the value of 3 :
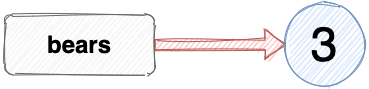
If I assign the number 3 to another variable, it’s referencing the same value as bears:
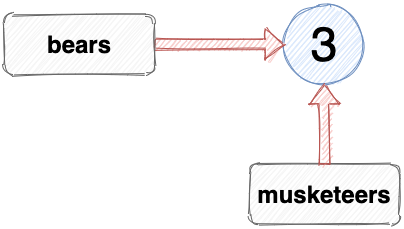
The variables bears and musketeers both reference the same primitive value of 3. We can verify this using the strict equality operator, === :
The equality operator returns true if both variables are referencing the same value.
Some gotchas when working with objects
The previous examples showed primitive values being assigned to variables. The same process is used when assigning objects:
This assignment means that the variable ghostbusters references an object:
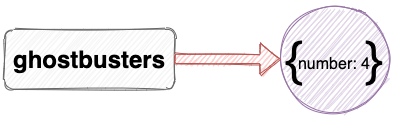
A big difference when assigning objects to variables, however, is that if you assign another object literal to another variable, it will reference a completely different object — even if both object literals look exactly the same! For example, the assignment below looks like the variable tmnt (Teenage Mutant Ninja Turtles) references the same object as the variable ghostbusters :
Even though the variables ghostbusters and tmnt look like they reference the same object, they actually both reference a completely different object, as we can see if we check with the strict equality operator:
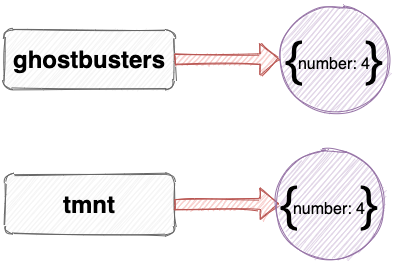
When the const keyword was introduced in ES6, many people mistakenly believed that constants had been introduced to JavaScript, but this wasn’t the case. The name of this keyword is a little misleading.
Any variable declared with const can’t be reassigned to another value. This goes for primitive values and objects. For example, the variable bears was declared using const in the previous section, so it can’t have another value assigned to it. If we try to assign the number 2 to the variable bears , we get an error:
The reference to the number 3 is fixed and the bears variable can’t be reassigned another value.
The same applies to objects. If we try to assign a different object to the variable ghostbusters , we get the same error:
Variable reassignment using let
When the keyword let is used to declare a variable, it can be reassigned to reference a different value later on in our code. For example, we declared the variable musketeers using let , so we can change the value that musketeers references. If D’Artagnan joined the Musketeers, their number would increase to 4:
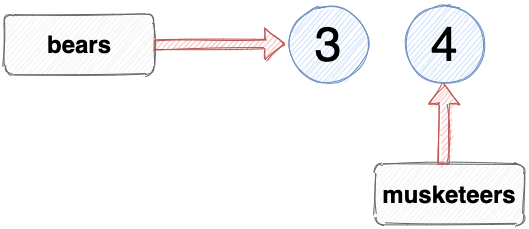
This can be done because let was used to declare the variable. We can alter the value that musketeers references as many times as we like.
The variable tmnt was also declared using let , so it can also be reassigned to reference another object (or a different type entirely if we like):
Note that the variable tmnt now references a completely different object ; we haven’t just changed the number property to 5.
In summary , if you declare a variable using const , its value can’t be reassigned and will always reference the same primitive value or object that it was originally assigned to. If you declare a variable using let , its value can be reassigned as many times as required later in the program.
Using const as often as possible is generally considered good practice, as it means that the value of variables remains constant and the code is more consistent and predictable, making it less prone to errors and bugs.
In native JavaScript, you can only assign values to variables. You can’t assign variables to reference another variable, even though it looks like you can. For example, the number of Stooges is the same as the number of Musketeers, so we can assign the variable stooges to reference the same value as the variable musketeers using the following:
This looks like the variable stooges is referencing the variable musketeers , as shown in the diagram below:
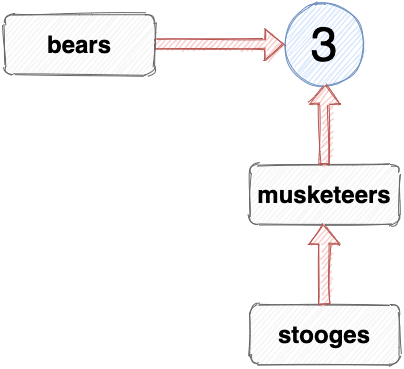
However, this is impossible in native JavaScript: a variable can only reference an actual value; it can’t reference another variable . What actually happens when you make an assignment like this is that the variable on the left of the assignment will reference the value the variable on the right references, so the variable stooges will reference the same value as the musketeers variable, which is the number 3. Once this assignment has been made, the stooges variable isn’t connected to the musketeers variable at all.
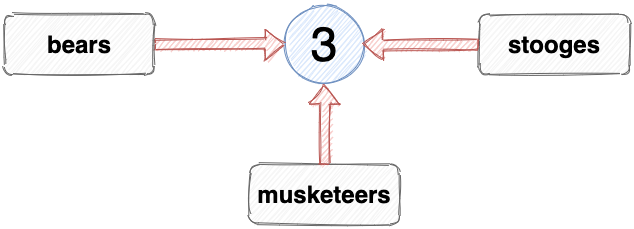
This means that if D’Artagnan joins the Musketeers and we set the value of the musketeers to 4, the value of stooges will remain as 3. In fact, because we declared the stooges variable using const , we can’t set it to any new value; it will always be 3.
In summary : if you declare a variable using const and set it to a primitive value, even via a reference to another variable, then its value can’t change. This is good for your code, as it means it will be more consistent and predictable.
A value is said to be mutable if it can be changed. That’s all there is to it: a mutation is the act of changing the properties of a value.
All primitive value in JavaScript are immutable : you can’t change their properties — ever. For example, if we assign the string "cake" to variable food , we can see that we can’t change any of its properties:
If we try to change the first letter to “f”, it looks like it has changed:
But if we take a look at the value of the variable, we see that nothing has actually changed:
The same thing happens if we try to change the length property:
Despite the return value implying that the length property has been changed, a quick check shows that it hasn’t:
Note that this has nothing to do with declaring the variable using const instead of let . If we had used let , we could set food to reference another string, but we can’t change any of its properties. It’s impossible to change any properties of primitive data types because they’re immutable .
Mutability and objects in JavaScript
Conversely, all objects in JavaScript are mutable, which means that their properties can be changed, even if they’re declared using const (remember let and const only control whether or not a variable can be reassigned and have nothing to do with mutability). For example, we can change the the first item of an array using the following code:
Note that this change still occurred, despite the fact that we declared the variable food using const . This shows that using const does not stop objects from being mutated .
We can also change the length property of an array, even if it has been declared using const :
Remember that when we assign variables to object literals, the variables will reference completely different objects, even if they look the same:
But if we assign a variable fantastic4 to another variable, they will both reference the same object:
This assigns the variable fantastic4 to reference the same object that the variable tmnt references, rather than a completely different object.
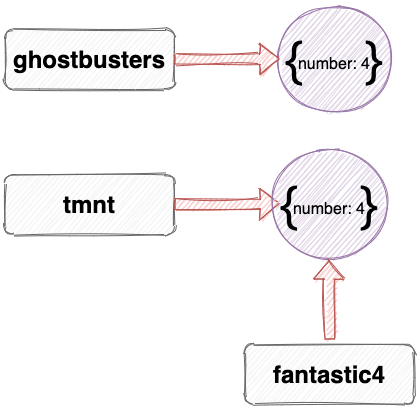
This is often referred to as copying by reference , because both variables are assigned to reference the same object.
This is important, because any mutations made to this object will be seen in both variables.
So, if Spider-Man joins The Fantastic Four, we might update the number value in the object:
This is a mutation, because we’ve changed the number property rather than setting fantastic4 to reference a new object.
This causes us a problem, because the number property of tmnt will also also change, possibly without us even realizing:
This is because both tmnt and fantastic4 are referencing the same object, so any mutations that are made to either tmnt or fantastic4 will affect both of them.
This highlights an important concept in JavaScript: when objects are copied by reference and subsequently mutated, the mutation will affect any other variables that reference that object. This can lead to unintended side effects and bugs that are difficult to track down.
So how do you make a copy of an object without creating a reference to the original object? The answer is to use the spread operator !
The spread operator was introduced for arrays and strings in ES2015 and for objects in ES2018. It allows you to easily make a shallow copy of an object without creating a reference to the original object.
The example below shows how we could set the variable fantastic4 to reference a copy of the tmnt object. This copy will be exactly the same as the tmnt object, but fantastic4 will reference a completely new object. This is done by placing the name of the variable to be copied inside an object literal with the spread operator in front of it:
What we’ve actually done here is assign the variable fantastic4 to a new object literal and then used the spread operator to copy all the enumerable properties of the object referenced by the tmnt variable. Because these properties are values, they’re copied into the fantastic4 object by value, rather than by reference.
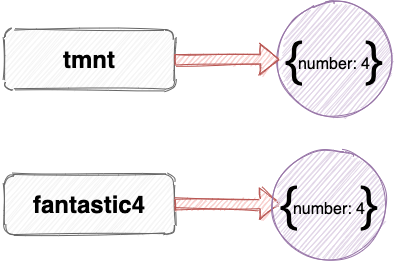
Now any changes that are made to either object won’t affect the other. For example, if we update the number property of the fantastic4 variable to 5, it won’t affect the tmnt variable:
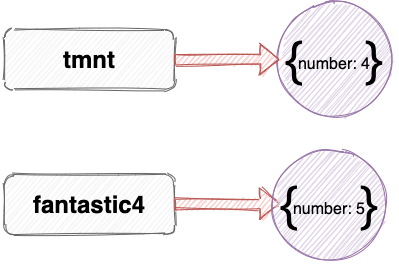
The spread operator also has a useful shortcut notation that can be used to make copies of an object and then make some changes to the new object in a single line of code.
For example, say we wanted to create an object to model the Teenage Mutant Ninja Turtles. We could create the first turtle object, and assign the variable leonardo to it:
The other turtles all have the same properties, except for the weapon and color properties, that are different for each turtle. It makes sense to make a copy of the object that leonardo references, using the spread operator, and then change the weapon and color properties, like so:
We can do this in one line by adding the properties we want to change after the reference to the spread object. Here’s the code to create new objects for the variables donatello and raphael :
Note that using the spread operator in this way only makes a shallow copy of an object. To make a deep copy, you’d have to do this recursively, or use a library. Personally, I’d advise that you try to keep your objects as shallow as possible.
In this article, we’ve covered the concepts of variable assignment and mutation and seen why — together — they can be a real pain for developers.
Mutations have a bad reputation, but they’re not necessarily bad in themselves. In fact, if you’re building a dynamic web app, it must change at some point. That’s literally the meaning of the word “dynamic”! This means that there will have to be some mutations somewhere in your code. Having said that, the fewer mutations there are, the more predictable your code will be, making it easier to maintain and less likely to develop any bugs.
A particularly toxic combination is copying by reference and mutations. This can lead to side effects and bugs that you don’t even realize have happened. If you mutate an object that’s referenced by another variable in your code, it can cause lots of problems that can be difficult to track down. The key is to try and minimize your use of mutations to the essential and keep track of which objects have been mutated.
In functional programming, a pure function is one that doesn’t cause any side effects, and mutations are one of the biggest causes of side effects.
A golden rule is to avoid copying any objects by reference. If you want to copy another object, use the spread operator and then make any mutations immediately after making the copy.
Next up, we’ll look into array mutations in JavaScript .
Don’t forget to check out my new book Learn to Code with JavaScript if you want to get up to speed with modern JavaScript. You can read the first chapter for free. And please reach out on Twitter if you have any questions or comments!
What is the difference between variable assignment and mutation in JavaScript?
In JavaScript, variable assignment refers to the process of assigning a value to a variable. For example, let x = 5; Here, we are assigning the value 5 to the variable x. On the other hand, mutation refers to the process of changing the value of an existing variable. For example, if we later write x = 10; we are mutating the variable x by changing its value from 5 to 10.
How does JavaScript handle variable assignment and mutation differently for primitive and non-primitive data types?
JavaScript treats primitive data types (like numbers, strings, and booleans) and non-primitive data types (like objects and arrays) differently when it comes to variable assignment and mutation. For primitive data types, when you assign a variable, a copy of the value is created and stored in a new memory location. However, for non-primitive data types, when you assign a variable, both variables point to the same memory location. Therefore, if you mutate one variable, the change is reflected in all variables that point to that memory location.
What is the concept of pass-by-value and pass-by-reference in JavaScript?
Pass-by-value and pass-by-reference are two ways that JavaScript can pass variables to a function. When JavaScript passes a variable by value, it creates a copy of the variable’s value and passes that copy to the function. Any changes made to the variable inside the function do not affect the original variable. However, when JavaScript passes a variable by reference, it passes a reference to the variable’s memory location. Therefore, any changes made to the variable inside the function also affect the original variable.
How can I prevent mutation in JavaScript?
There are several ways to prevent mutation in JavaScript. One way is to use the Object.freeze() method, which prevents new properties from being added to an object, existing properties from being removed, and prevents changing the enumerability, configurability, or writability of existing properties. Another way is to use the const keyword when declaring a variable. This prevents reassignment of the variable, but it does not prevent mutation of the variable’s value if the value is an object or an array.
What is the difference between shallow copy and deep copy in JavaScript?
In JavaScript, a shallow copy of an object is a copy of the object where the values of the original object and the copy point to the same memory location for non-primitive data types. Therefore, if you mutate the copy, the original object is also mutated. On the other hand, a deep copy of an object is a copy of the object where the values of the original object and the copy do not point to the same memory location. Therefore, if you mutate the copy, the original object is not mutated.
How can I create a deep copy of an object in JavaScript?
One way to create a deep copy of an object in JavaScript is to use the JSON.parse() and JSON.stringify() methods. The JSON.stringify() method converts the object into a JSON string, and the JSON.parse() method converts the JSON string back into an object. This creates a new object that is a deep copy of the original object.
What is the MutationObserver API in JavaScript?
The MutationObserver API provides developers with a way to react to changes in a DOM. It is designed to provide a general, efficient, and robust API for reacting to changes in a document.
How does JavaScript handle variable assignment and mutation in the context of closures?
In JavaScript, a closure is a function that has access to its own scope, the scope of the outer function, and the global scope. When a variable is assigned or mutated inside a closure, it can affect the value of the variable in the outer scope, depending on whether the variable was declared in the closure’s scope or the outer scope.
What is the difference between var, let, and const in JavaScript?
In JavaScript, var, let, and const are used to declare variables. var is function scoped, and if it is declared outside a function, it is globally scoped. let and const are block scoped, meaning they exist only within the block they are declared in. The difference between let and const is that let allows reassignment, while const does not.
How does JavaScript handle variable assignment and mutation in the context of asynchronous programming?
In JavaScript, asynchronous programming allows multiple things to happen at the same time. When a variable is assigned or mutated in an asynchronous function, it can lead to unexpected results if other parts of the code are relying on the value of the variable. This is because the variable assignment or mutation may not have completed before the other parts of the code run. To handle this, JavaScript provides several features, such as promises and async/await, to help manage asynchronous code.
Darren loves building web apps and coding in JavaScript, Haskell and Ruby. He is the author of Learn to Code using JavaScript , JavaScript: Novice to Ninja and Jump Start Sinatra .He is also the creator of Nanny State , a tiny alternative to React. He can be found on Twitter @daz4126.
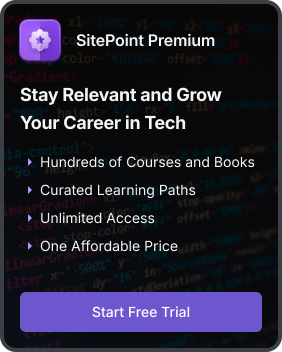
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
- Solve Coding Problems
- Share Your Experience
- JavaScript Tutorial
JavaScript Basics
- Introduction to JavaScript
- JavaScript Versions
- How to Add JavaScript in HTML Document?
- JavaScript Statements
- JavaScript Syntax
- JavaScript Output
- JavaScript Comments
JS Variables & Datatypes
- Variables and Datatypes in JavaScript
- Global and Local variables in JavaScript
- JavaScript Let
- JavaScript Const
- JavaScript var
JS Operators
- JavaScript Operators
- Operator precedence in JavaScript
- JavaScript Arithmetic Operators
- JavaScript Assignment Operators
- JavaScript Comparison Operators
- JavaScript Logical Operators
- JavaScript Bitwise Operators
- JavaScript Ternary Operator
- JavaScript Comma Operator
- JavaScript Unary Operators
- JavaScript Relational operators
- JavaScript String Operators
- JavaScript Loops
- 7 Loops of JavaScript
- JavaScript For Loop
- JavaScript While Loop
- JavaScript for-in Loop
- JavaScript for...of Loop
- JavaScript do...while Loop
JS Perfomance & Debugging
- JavaScript | Performance
- Debugging in JavaScript
- JavaScript Errors Throw and Try to Catch
- Objects in Javascript
- Introduction to Object Oriented Programming in JavaScript
- JavaScript Objects
- Creating objects in JavaScript
- JavaScript JSON Objects
- JavaScript Object Reference
JS Function
- Functions in JavaScript
- How to write a function in JavaScript ?
- JavaScript Function Call
- Different ways of writing functions in JavaScript
- Difference between Methods and Functions in JavaScript
- Explain the Different Function States in JavaScript
- JavaScript Function Complete Reference
JavaScript Arrays
- JavaScript Array Methods
- Best-Known JavaScript Array Methods
- What are the Important Array Methods of JavaScript ?
- JavaScript Array Reference
- JavaScript Strings
- JavaScript String Methods
- JavaScript String Reference
- JavaScript Numbers
- How numbers are stored in JavaScript ?
- How to create a Number object using JavaScript ?
- JavaScript Number Reference
- JavaScript Math Object
- What is the use of Math object in JavaScript ?
- JavaScript Math Reference
- JavaScript Map
- What is JavaScript Map and how to use it ?
- JavaScript Map Reference
- Sets in JavaScript
- How are elements ordered in a Set in JavaScript ?
- How to iterate over Set elements in JavaScript ?
- How to sort a set in JavaScript ?
- JavaScript Set Reference
- JavaScript Date
- JavaScript Promise
- JavaScript BigInt
- JavaScript Boolean
- JavaScript Proxy/Handler
- JavaScript WeakMap
- JavaScript WeakSet
- JavaScript Function Generator
- JavaScript JSON
- Arrow functions in JavaScript
- JavaScript this Keyword
- Strict mode in JavaScript
- Introduction to ES6
- JavaScript Hoisting
- Async and Await in JavaScript
JavaScript Exercises
- JavaScript Exercises, Practice Questions and Solutions
- Full Stack Development Course
An array in JavaScript is a data structure used to store multiple values in a single variable. It can hold various data types and allows for dynamic resizing. Elements are accessed by their index, starting from 0.
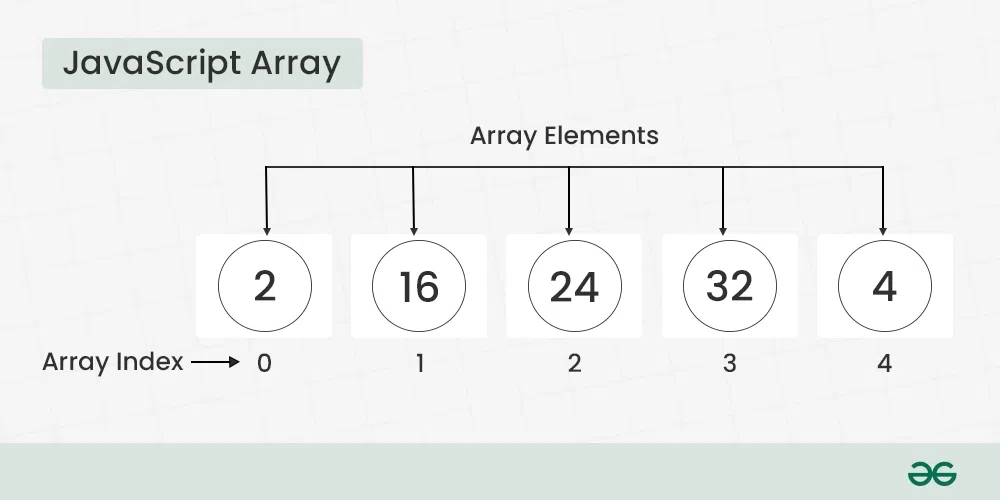
JavaScript Array
You have two ways to create JavaScript Arrays: using the Array constructor or the shorthand array literal syntax , which is just square brackets. Arrays are flexible in size, so they can grow or shrink as you add or remove elements.
Table of Content
- What is Array in JavaScript?
Basic Terminologies of JavaScript Array
Declaration of an array, basic operations on javascript arrays, difference between javascript arrays and objects, when to use javascript arrays and objects, recognizing a javascript array, javascript array complete reference, javascript array examples, javascript cheatsheet.
- Array: A data structure in JavaScript that allows you to store multiple values in a single variable.
- Array Element: Each value within an array is called an element. Elements are accessed by their index.
- Array Index: A numeric representation that indicates the position of an element in the array. JavaScript arrays are zero-indexed, meaning the first element is at index 0.
- Array Length: The number of elements in an array. It can be retrieved using the length property.
There are basically two ways to declare an array i.e. Array Literal and Array Constructor.
1. Creating an Array using Array Literal
Creating an array using array literal involves using square brackets [] to define and initialize the array. This method is concise and widely preferred for its simplicity.
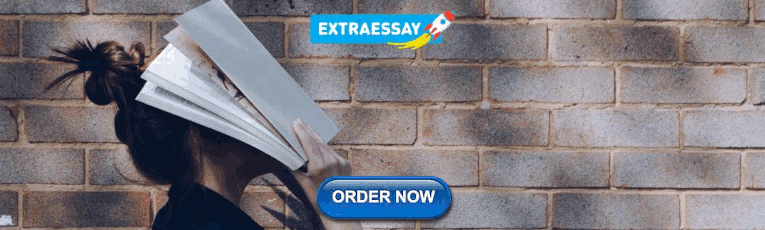
2. Creating an Array using Array Constructor (JavaScript new Keyword)
The “Array Constructor” refers to a method of creating arrays by invoking the Array constructor function. This approach allows for dynamic initialization and can be used to create arrays with a specified length or elements.
Note: Both the above methods do exactly the same. Use the array literal method for efficiency, readability, and speed.
1. Accessing Elements of an Array
Any element in the array can be accessed using the index number. The index in the arrays starts with 0.
2. Accessing the First Element of an Array
The array indexing starts from 0, so we can access first element of array using the index number.
3. Accessing the Last Element of an Array
We can access the last array element using [array.length – 1] index number.
4. Modifying the Array Elements
Elements in an array can be modified by assigning a new value to their corresponding index.
5. Adding Elements to the Array
Elements can be added to the array using methods like push() and unshift().
6. Removing Elements from an Array
Remove elements using methods like pop(), shift(), or splice().
7. Array Length
Get the length of an array using the length property.
8. Increase and Decrease the Array Length
We can increase and decrease the array length using the JavaScript length property.
9. Iterating Through Array Elements
We can iterate array and access array elements using for and forEach loop.
Example: It is an example of for loop.
Example: It is the example of Array.forEach() loop.
10. Array Concatenation
Combine two or more arrays using the concat() method. Ir returns new array conaining joined arrays elements.
11. Conversion of an Array to String
We have a builtin method toString() to converts an array to a string.
12. Check the Type of an Arrays
The JavaScript typeof operator is used ot check the type of an array. It returns “object” for arrays.
- JavaScript arrays use indexes as numbers.
- objects use indexes as names..
- Arrays are used when we want element names to be numeric.
- Objects are used when we want element names to be strings.
There are two methods by which we can recognize a JavaScript array:
- By using Array.isArray() method
- By using instanceof method
Below is an example showing both approaches:
Note: A common error is faced while writing the arrays:
The above two statements are not the same.
Output: This statement creates an array with an element ” [5] “.
We have a complete list of Javascript Array, to check those please go through this JavaScript Array Reference article. It contains a detailed description and examples of all Array Properties and Methods.
JavaScript Array examples contain a list of questions that are majorly asked in interviews. Please check this article JavaScript Array Examples for more detail.
We have a Cheat Sheet on Javascript where we have covered all the important topics of Javascript to check those please go through Javascript Cheat Sheet-A Basic guide to JavaScript .
Please Login to comment...
Similar reads.
- javascript-array
- Web Technologies
- OpenAI launches ChatGPT Edu for universities: Here is what it is and how it works
- NVIDIA Launches AI Assistants With GeForce RTX AI PCs
- How to Download WhatsApp Status in iPhone
- 10 Best ChatGPT Prompts for Increasing Productivity in 2024
- 10 Best Free Reverse Phone Number Lookup
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
JavaScript Arrays - How to Create an Array in JavaScript
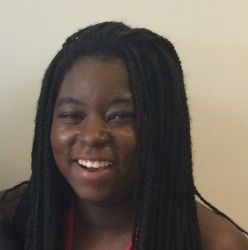
An array is a type of data structure where you can store an ordered list of elements.
In this article, I will show you 3 ways you can create an array using JavaScript. I will also show you how to create an array from a string using the split() method.
How to create an array in JavaScript using the assignment operator
The most common way to create an array in JavaScript would be to assign that array to a variable like this:
If we console.log the array, then it will show us all 4 elements listed in the array.

How to create an array in JavaScript using the new operator and Array constructor
Another way to create an array is to use the new keyword with the Array constructor.
Here is the basic syntax:
If a number parameter is passed into the parenthesis, that will set the length for the new array.
In this example, we are creating an array with a length of 3 empty slots.

If we use the length property on the new array, then it will return the number 3.
But if we try to access any elements of the array, it will come back undefined because all of those slots are currently empty.
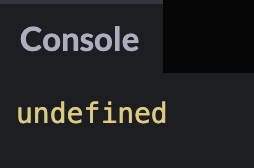
We can modify our example to take in multiple parameters and create an array of food items.
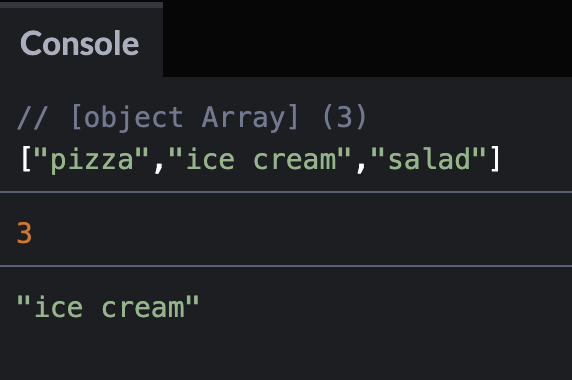
How to create an array in JavaScript using Array.of()
Another way to create an array is to use the Array.of() method. This method takes in any number of arguments and creates a new array instance.
We can modify our earlier food example to use the Array.of() method like this.

This method is really similar to using the Array constructor. The key difference is that if you pass in a single number using Array.of() it will return an array with that number in it. But the Array constructor creates x number of empty slots for that number.
In this example it would return an array with the number 4 in it.

But if I changed this example to use the Array constructor, then it would return an array of 4 empty slots.

How to create an array from a string using the split() method
Here is the syntax for the JavaScript split() method.
The optional separator is a type of pattern that tells the computer where each split should happen.
The optional limit parameter is a positive number that tells the computer how many substrings should be in the returned array value.
In this example, I have the string "I love freeCodeCamp" . If I were to use the split() method without the separator, then the return value would be an array of the entire string.

If I wanted to change it so the string is split up into individual characters, then I would need to add a separator. The separator would be an empty string.

Notice how the spaces are considered characters in the return value.
If I wanted to change it so the string is split up into individual words, then the separator would be an empty string with a space.

In this article I showed you three ways to create an array using the assignment operator, Array constructor, and Array.of() method.
If a number parameter is passed into the parenthesis, that will set the length for the new array with that number of empty slots.
For example, this code will create an array with a length of 3 empty slots.
We can also pass in multiple parameters like this:
You can also take a string and create an array using the split() method
I hope you enjoyed this article on JavaScript arrays.
I am a musician and a programmer.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
JS Reference
Html events, html objects, other references, javascript object.assign(), description.
The Object.assign() method copies properties from one or more source objects to a target object.
Related Methods:
Object.assign() copies properties from a source object to a target object.
Object.create() creates an object from an existing object.
Object.fromEntries() creates an object from a list of keys/values.
Parameter | Description |
Required. An existing object. | |
Required. One or more sources. |
Return Value
Type | Description |
Object | The target object. |
Advertisement
Browser Support
Object.assign() is an ECMAScript6 (ES6) feature.
ES6 (JavaScript 2015) is supported in all modern browsers since June 2017:
Chrome 51 | Edge 15 | Firefox 54 | Safari 10 | Opera 38 |
May 2016 | Apr 2017 | Jun 2017 | Sep 2016 | Jun 2016 |
Object.assign() is not supported in Internet Explorer.
Object Tutorials
JavaScript Objects
JavaScript Object Definition
JavaScript Object Methods
JavaScript Object Properties

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Version 1.90 is now available! Read about the new features and fixes from May.
Snippets in Visual Studio Code
Code snippets are templates that make it easier to enter repeating code patterns, such as loops or conditional-statements.
In Visual Studio Code, snippets appear in IntelliSense ( ⌃Space (Windows, Linux Ctrl+Space ) ) mixed with other suggestions, as well as in a dedicated snippet picker ( Insert Snippet in the Command Palette). There is also support for tab-completion: Enable it with "editor.tabCompletion": "on" , type a snippet prefix (trigger text), and press Tab to insert a snippet.
The snippet syntax follows the TextMate snippet syntax with the exceptions of 'interpolated shell code' and the use of \u ; both are not supported.
Built-in snippets
VS Code has built-in snippets for a number of languages such as: JavaScript, TypeScript, Markdown, and PHP.
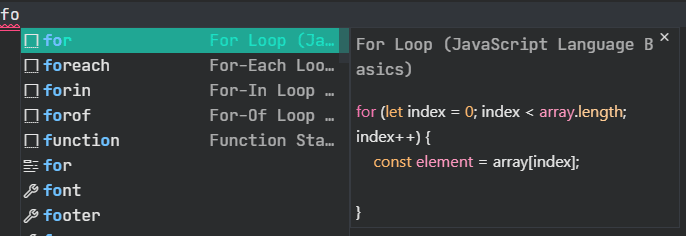
You can see the available snippets for a language by running the Insert Snippet command in the Command Palette to get a list of the snippets for the language of the current file. However, keep in mind that this list also includes user snippets that you have defined, and any snippets provided by extensions you have installed.
Install snippets from the Marketplace
Many extensions on the VS Code Marketplace include snippets. You can search for extensions that contains snippets in the Extensions view ( ⇧⌘X (Windows, Linux Ctrl+Shift+X ) ) using the @category:"snippets" filter.
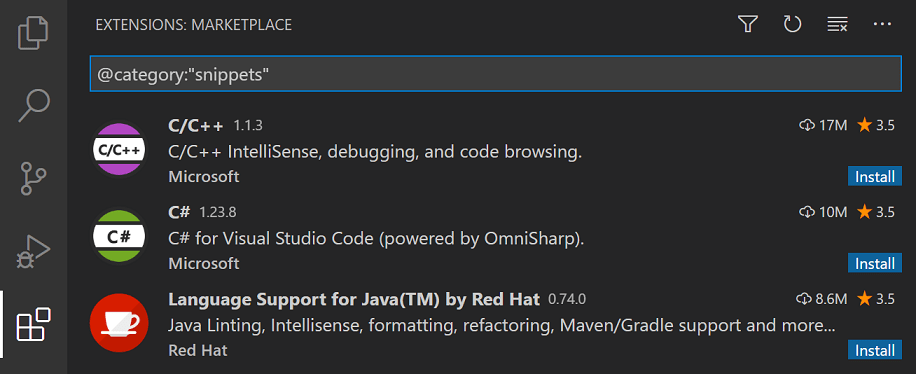
If you find an extension you want to use, install it, then restart VS Code and the new snippets will be available.
Create your own snippets
You can easily define your own snippets without any extension. To create or edit your own snippets, select Configure User Snippets under File > Preferences , and then select the language (by language identifier ) for which the snippets should appear, or the New Global Snippets file option if they should appear for all languages. VS Code manages the creation and refreshing of the underlying snippets file(s) for you.
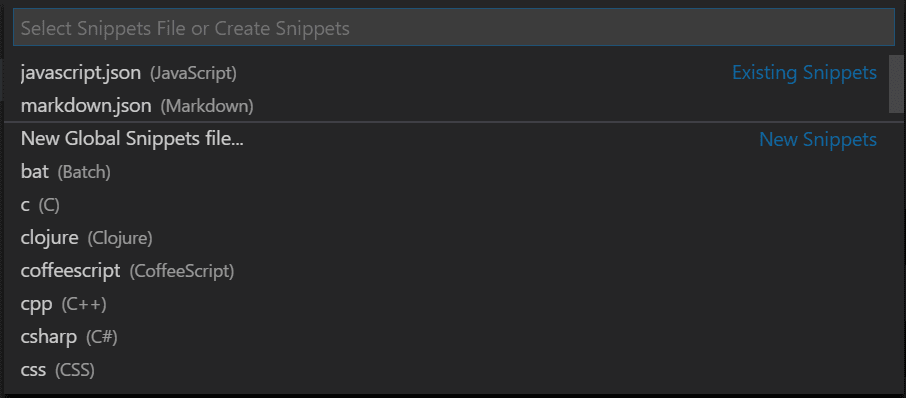
Snippets files are written in JSON, support C-style comments, and can define an unlimited number of snippets. Snippets support most TextMate syntax for dynamic behavior, intelligently format whitespace based on the insertion context, and allow easy multiline editing.
Below is an example of a for loop snippet for JavaScript:
In the example above:
- "For Loop" is the snippet name. It is displayed via IntelliSense if no description is provided.
- prefix defines one or more trigger words that display the snippet in IntelliSense. Substring matching is performed on prefixes, so in this case, "fc" could match "for-const".
- body is one or more lines of content, which will be joined as multiple lines upon insertion. Newlines and embedded tabs will be formatted according to the context in which the snippet is inserted.
- description is an optional description of the snippet displayed by IntelliSense.
Additionally, the body of the example above has three placeholders (listed in order of traversal): ${1:array} , ${2:element} , and $0 . You can quickly jump to the next placeholder with Tab , at which point you may edit the placeholder or jump to the next one. The string after the colon : (if any) is the default text, for example element in ${2:element} . Placeholder traversal order is ascending by number, starting from one; zero is an optional special case that always comes last, and exits snippet mode with the cursor at the specified position.
File template snippets
You can add the isFileTemplate attribute to your snippet's definition if the snippet is intended to populate or replace a file's contents. File template snippets are displayed in a dropdown when you run the Snippets: Populate File from Snippet command in a new or existing file.
Snippet scope
Snippets are scoped so that only relevant snippets are suggested. Snippets can be scoped by either:
- the language(s) to which snippets are scoped (possibly all)
- the project(s) to which snippets are scoped (probably all)
Language snippet scope
Every snippet is scoped to one, several, or all ("global") languages based on whether it is defined in:
- a language snippet file
- a global snippet file
Single-language user-defined snippets are defined in a specific language's snippet file (for example javascript.json ), which you can access by language identifier through Snippets: Configure User Snippets . A snippet is only accessible when editing the language for which it is defined.
Multi-language and global user-defined snippets are all defined in "global" snippet files (JSON with the file suffix .code-snippets ), which is also accessible through Snippets: Configure User Snippets . In a global snippets file, a snippet definition may have an additional scope property that takes one or more language identifiers , which makes the snippet available only for those specified languages. If no scope property is given, then the global snippet is available in all languages.
Most user-defined snippets are scoped to a single language, and so are defined in a language-specific snippet file.
Project snippet scope
You can also have a global snippets file (JSON with file suffix .code-snippets ) scoped to your project. Project-folder snippets are created with the New Snippets file for ' '... option in the Snippets: Configure User Snippets dropdown menu and are located at the root of the project in a .vscode folder. Project snippet files are useful for sharing snippets with all users working in that project. Project-folder snippets are similar to global snippets and can be scoped to specific languages through the scope property.
Snippet syntax
The body of a snippet can use special constructs to control cursors and the text being inserted. The following are supported features and their syntaxes:
With tabstops, you can make the editor cursor move inside a snippet. Use $1 , $2 to specify cursor locations. The number is the order in which tabstops will be visited, whereas $0 denotes the final cursor position. Multiple occurrences of the same tabstop are linked and updated in sync.
Placeholders
Placeholders are tabstops with values, like ${1:foo} . The placeholder text will be inserted and selected such that it can be easily changed. Placeholders can be nested, like ${1:another ${2:placeholder}} .
Placeholders can have choices as values. The syntax is a comma-separated enumeration of values, enclosed with the pipe-character, for example ${1|one,two,three|} . When the snippet is inserted and the placeholder selected, choices will prompt the user to pick one of the values.
With $name or ${name:default} , you can insert the value of a variable. When a variable isn't set, its default or the empty string is inserted. When a variable is unknown (that is, its name isn't defined) the name of the variable is inserted and it is transformed into a placeholder.
The following variables can be used:
- TM_SELECTED_TEXT The currently selected text or the empty string
- TM_CURRENT_LINE The contents of the current line
- TM_CURRENT_WORD The contents of the word under cursor or the empty string
- TM_LINE_INDEX The zero-index based line number
- TM_LINE_NUMBER The one-index based line number
- TM_FILENAME The filename of the current document
- TM_FILENAME_BASE The filename of the current document without its extensions
- TM_DIRECTORY The directory of the current document
- TM_FILEPATH The full file path of the current document
- RELATIVE_FILEPATH The relative (to the opened workspace or folder) file path of the current document
- CLIPBOARD The contents of your clipboard
- WORKSPACE_NAME The name of the opened workspace or folder
- WORKSPACE_FOLDER The path of the opened workspace or folder
- CURSOR_INDEX The zero-index based cursor number
- CURSOR_NUMBER The one-index based cursor number
For inserting the current date and time:
- CURRENT_YEAR The current year
- CURRENT_YEAR_SHORT The current year's last two digits
- CURRENT_MONTH The month as two digits (example '02')
- CURRENT_MONTH_NAME The full name of the month (example 'July')
- CURRENT_MONTH_NAME_SHORT The short name of the month (example 'Jul')
- CURRENT_DATE The day of the month as two digits (example '08')
- CURRENT_DAY_NAME The name of day (example 'Monday')
- CURRENT_DAY_NAME_SHORT The short name of the day (example 'Mon')
- CURRENT_HOUR The current hour in 24-hour clock format
- CURRENT_MINUTE The current minute as two digits
- CURRENT_SECOND The current second as two digits
- CURRENT_SECONDS_UNIX The number of seconds since the Unix epoch
- CURRENT_TIMEZONE_OFFSET The current UTC time zone offset as +HH:MM or -HH:MM (example -07:00 ).
For inserting random values:
- RANDOM 6 random Base-10 digits
- RANDOM_HEX 6 random Base-16 digits
- UUID A Version 4 UUID
For inserting line or block comments, honoring the current language:
- BLOCK_COMMENT_START Example output: in PHP /* or in HTML <!--
- BLOCK_COMMENT_END Example output: in PHP */ or in HTML -->
- LINE_COMMENT Example output: in PHP //
The snippet below inserts /* Hello World */ in JavaScript files and <!-- Hello World --> in HTML files:
Variable transforms
Transformations allow you to modify the value of a variable before it is inserted. The definition of a transformation consists of three parts:
- A regular expression that is matched against the value of a variable, or the empty string when the variable cannot be resolved.
- A "format string" that allows to reference matching groups from the regular expression. The format string allows for conditional inserts and simple modifications.
- Options that are passed to the regular expression.
The following example inserts the name of the current file without its ending, so from foo.txt it makes foo .
Placeholder-Transform
Like a Variable-Transform, a transformation of a placeholder allows changing the inserted text for the placeholder when moving to the next tab stop. The inserted text is matched with the regular expression and the match or matches - depending on the options - are replaced with the specified replacement format text. Every occurrence of a placeholder can define its own transformation independently using the value of the first placeholder. The format for Placeholder-Transforms is the same as for Variable-Transforms.
Transform examples
The examples are shown within double quotes, as they would appear inside a snippet body, to illustrate the need to double escape certain characters. Sample transformations and the resulting output for the filename example-123.456-TEST.js .
Example | Output | Explanation |
---|---|---|
Replace the first with | ||
Replace each or with | ||
Change to all uppercase | ||
Remove non-alphanumeric characters |
Below is the EBNF ( extended Backus-Naur form ) for snippets. With \ (backslash), you can escape $ , } , and \ . Within choice elements, the backslash also escapes comma and pipe characters. Only the characters required to be escaped can be escaped, so $ should not be escaped within these constructs and neither $ or } should be escaped inside choice constructs.
Using TextMate snippets
You can also use existing TextMate snippets (.tmSnippets) with VS Code. See the Using TextMate Snippets topic in our Extension API section to learn more.
Assign keybindings to snippets
You can create custom keybindings to insert specific snippets. Open keybindings.json ( Preferences: Open Keyboard Shortcuts File ), which defines all your keybindings, and add a keybinding passing "snippet" as an extra argument:
The keybinding will invoke the Insert Snippet command but instead of prompting you to select a snippet, it will insert the provided snippet. You define the custom keybinding as usual with a keyboard shortcut, command ID, and optional when clause context for when the keyboard shortcut is enabled.
Also, instead of using the snippet argument value to define your snippet inline, you can reference an existing snippet by using the langId and name arguments. The langId argument selects the language for which the snippet denoted by name is inserted, e.g the sample below selects the myFavSnippet that's available for csharp -files.
- Command Line - VS Code has a rich command-line interface to open or diff files and install extensions.
- Extension API - Learn about other ways to extend VS Code.
- Snippet Guide - You can package snippets for use in VS Code.
Common questions
What if i want to use existing textmate snippets from a .tmsnippet file.
You can easily package TextMate snippets files for use in VS Code. See Using TextMate Snippets in our Extension API documentation.
How do I have a snippet place a variable in the pasted script?
To have a variable in the pasted script, you need to escape the '$' of the $variable name so that it isn't parsed by the snippet expansion phase.
This results in the pasted snippet as:
Can I remove snippets from IntelliSense?
Yes, you can hide specific snippets from showing in IntelliSense (completion list) by selecting the Hide from IntelliSense button to the right of snippet items in the Insert Snippet command dropdown.
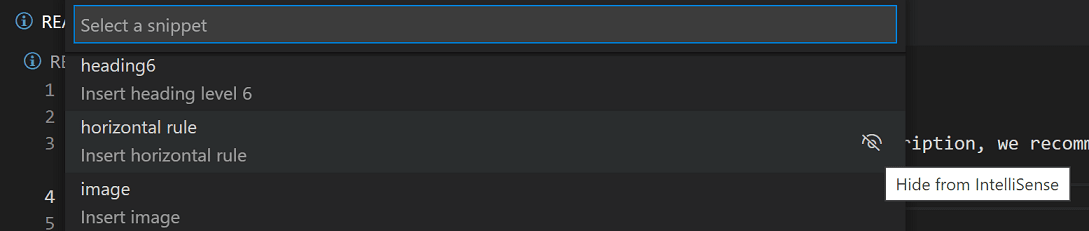
You can still select the snippet with the Insert Snippet command but the hidden snippet won't be displayed in IntelliSense.
- Stack Overflow Public questions & answers
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Talent Build your employer brand
- Advertising Reach developers & technologists worldwide
- Labs The future of collective knowledge sharing
- About the company
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Setting environment variable from command stored in array
I have a list of commands which I dynamically read in my script, each command is stored in a line. Some lines contain commands like the following
line="env_var=\"xxx\" myexe -flag1 -flag2"
I'm trying to run all lines (including these) from my script.
Then for each line I do
cmd=($line)
"${cmd[@]}"
However this fails with
env_var="xxx": command not found
I suspect the issue is that in my array declaration the part env_var="xxx" is seen as 1 single word, and somehow I need to put syntatic equals and quotes, but I can't seem to do it. Any help is greatly appreciated. Thanks! p.s. I tried eval $line and that works but would like to avoid that.

- 4 You have to decide what you would like your file format to be and treat it accordingly. If you want to take in shell statements, then you need to eval them. If you want to take in lists of commands and arguments, then you can do that, but know that env_var="xxx" will no longer be treated as an assignment because that's shell syntax and when you treat it as a command name you will instead get env_var="xxx": command not found – that other guy May 31 at 22:55
- Note that env_var=\"xxx\" and then $line , if it would work, it will set env_var to "xxx" including " , not to xxx . – KamilCuk Jun 1 at 15:24
- 2 If you could reliably parse line into an array, you wouldn't need arrays in the first place. – chepner Jun 1 at 20:14
2 Answers 2
You can use env to parse environment assignments and xargs to parse quotes.
Read also https://mywiki.wooledge.org/BashFAQ/050 man xargs and man env . Chec your scripts with shellcheck.

- If not eval , the command xargs env will execute in near same way. – F. Hauri - Give Up GitHub Jun 1 at 16:08
- Something like line="env_var=\"xxx\" bash -c 'echo \$env_var \$@' -- -flag1 -flag2";declare -a cmd="($line)";declare -n tmpvar="${cmd[0]%=*}";tmpvar="${cmd[0]#*=}" "${cmd[@]:1}" seem closer to the request! ( This output: xxx -flag1 -flag2 ;) – F. Hauri - Give Up GitHub Jun 1 at 16:11
It's not clear why you're trying to avoid eval as what you're trying to do is what eval exists to do but you should do eval "$line" , i.e. quoted, not eval $line unquoted for all the usual reasons you don't remove quotes unless you need to (see https://mywiki.wooledge.org/Quotes ).
See https://mywiki.wooledge.org/BashFAQ/048 for best practices when using eval and see https://mywiki.wooledge.org/BashFAQ/050 for more information about storing commands in variables and note it says:
In bash , the only ways to generate, manipulate, or store code more complex than a simple command at runtime involve storing the code's plain text in a variable, file, stream, or function, and then using eval or sh to evaluate the stored code
i.e. that using eval is 1 of only 2 ways (the other being to run a shell on it) to do what you're trying to do robustly.
The negative to using eval (or calling a shell to execute the code in your variable) is that it executes what you tell it to and so if you tell it to execute bad code then bad things will happen, but that's the same problem as telling the shell to execute a script, the only difference being that code stored in a variable is often created dynamically, possibly including input from a user or some other source outside of your control, while that's extremely rare for code stored in a script. So as long as the commands in your input file are completely under your control, you treat then with the same rigor as any script you write, and you just want a way to execute them as if you had created a script for each - then eval is your friend.
Using a command with flags ( date ) as in the OPs sample input and adding a mix of single and double quotes plus a globbing char ( * ), potential variable expansion ( $bar ), command expansion ( $(date) ), and a complex command in a sample input file to really test that only the correct expansions, etc. happen in the script:
which, as desired, is exactly the same output (aside from current time of course) as if we just executed the input file:
If you want to avoid eval for some reason then instead of using eval we could have done it the other way that https://mywiki.wooledge.org/BashFAQ/050 recommends which is to pass your command to a shell to execute:
and also got the desired output but it's not obvious why you'd want to do that. There I'm using $SHELL to run the currently executing shell defined by your shebang, e.g. bash , rather than plain sh in case your file of commands uses bash syntax.
The difference between the 2 approaches is that eval 'command' executes in your current shell and so is more efficient but can affect the value of the variables in your current shell while bash -c 'command' executes in a subshell and so it's variable changes are local to that subshell:
You can, of course, manually put the call to eval in a subshell if you like:
If we try the above input file with xargs env as in the currently accepted answer instead of eval or "$SHELL" -c as above:
and even if we simplify the input to just this one line with no command substitution:
then it still fails:
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged linux bash shell or ask your own question .
- The Overflow Blog
- Introducing Staging Ground: The private space to get feedback on questions...
- How to prevent your new chatbot from giving away company secrets
- Featured on Meta
- The [tax] tag is being burninated
- The return of Staging Ground to Stack Overflow
- The 2024 Developer Survey Is Live
- Policy: Generative AI (e.g., ChatGPT) is banned
Hot Network Questions
- How did ALT + F4 become the shortcut for closing?
- Why does White castle into Black's attack in the King's Indian, and then run away afterwards?
- chmod responds cannot access
- Can a Kerr black hole become super-extremal?
- A phrase that means you are indifferent towards the things you are familiar with?
- What does 年頃 mean in terms of marrigeable age and adulthood?
- Accelerating Expansion of Universe - Why Not Caused by Radiation?
- Connecting to very old Linux OS with ssh
- Implicit function equation f(x) + log(f(x)) = x
- Is it common to email researchers to "sell" your work?
- Why are spherical shapes so common in the universe?
- Linear regression: interpret coefficient in terms of percentage change when the outcome variable is a count number
- Estimating Probability Density for Sample
- Is bike tyre pressure info deliberately hard to read?
- Is it legal to deposit a check that says pay to the order of cash
- What caused localized cracking and peeling of wall paint and how should I go about fixing it?
- A trigonometric equation: how hard could it be?
- Review not needed after review
- Is a weak version of the three sets Lemma provable in ZF?
- Which program is used in this shot of the movie "The Wrong Woman"
- Can we find the equivalent resistance just by using series and parallel combinations?
- Descartes Statement in Second Meditation is illogical?
- Do you have an expression saying "when you are very hungry, bread is also delicious for you" or similar one?
- Could a delayed choice Aharonov-Bohm experiment be used for FTL information transfer?
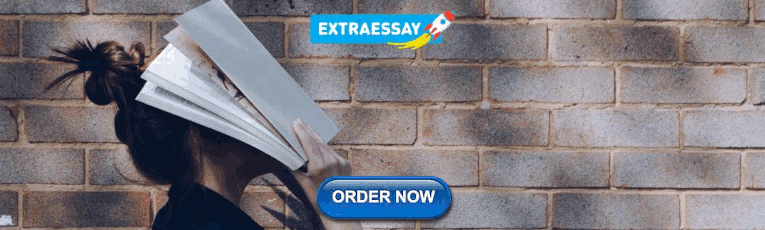
COMMENTS
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables. ... The rest property of array destructuring assignment can be another array or object binding pattern. The inner destructuring destructures from the array created after ...
1. When you assign a value to a variable, the variable has to be on the left of the assignment operator (the equals sign "="). This way has the variable on the left: largest = array[i]; It puts the array [i] value into the largest variable, and so it works. This way is backwards: array[i] = largest; It puts the largest value into the array [i ...
The destructuring assignment is a cool feature that came along with ES6. Destructuring is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables. That is, we can extract data from arrays and objects and assign them to variables. Why
It's called "destructuring assignment," because it "destructurizes" by copying items into variables. However, the array itself is not modified. It's just a shorter way to write: // let [firstName, surname] = arr; let firstName = arr [0]; let surname = arr [1]; Ignore elements using commas.
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, ... Assigning the rest of an array to a variable. When destructuring an array, you can unpack and assign the remaining part of it to a variable using the rest pattern:
Creating an Array. Using an array literal is the easiest way to create a JavaScript Array. Syntax: const array_name = [ item1, item2, ... ]; It is a common practice to declare arrays with the const keyword. Learn more about const with arrays in the chapter: JS Array Const.
Array destructuring in JavaScript is a syntax that allows you to extract individual elements from an array and assign them to distinct variables. Enclose the variables you want to assign values to within square brackets [] on the left-hand side of the assignment operator = , and place the array you want to destructure on the right-hand side.
In JavaScript, variable assignment refers to the process of assigning a value to a variable. For example, let x = 5; Here, we are assigning the value 5 to the variable x. On the other hand ...
The variables on the left side of the assignment operator are assigned to the value of the corresponding elements of the array on the right. You can skip array elements and go to the next ones by typing more than one comma between each variable name.
An array in JavaScript is also an object and variables only hold a reference to an object, not the object itself. Thus both variables have a reference to the same object. So changing made through one variable reflected in other as well.
Array: A data structure in JavaScript that allows you to store multiple values in a single variable. Array Element: Each value within an array is called an element. Elements are accessed by their index. Array Index: A numeric representation that indicates the position of an element in the array.
Another way to create an array is to use the new keyword with the Array constructor. Here is the basic syntax: new Array(); If a number parameter is passed into the parenthesis, that will set the length for the new array. In this example, we are creating an array with a length of 3 empty slots. new Array(3)
All JavaScript variables must be identified with unique names. These unique names are called identifiers. Identifiers can be short names (like x and y) or more descriptive names (age, sum, totalVolume). The general rules for constructing names for variables (unique identifiers) are: Names can contain letters, digits, underscores, and dollar signs.
Arrays. In the final article of this module, we'll look at arrays — a neat way of storing a list of data items under a single variable name. Here we look at why this is useful, then explore how to create an array, retrieve, add, and remove items stored in an array, and more besides.
Related Methods: Object.assign () copies properties from a source object to a target object. Object.create () creates an object from an existing object. Object.fromEntries () creates an object from a list of keys/values.
Sorry about the confusing question, but I specifically want to assign to multiple variables from an array. The split function will return an array. Btw eval is evil ;)
Here is an example: var a = 'quux'; a.foo = 'bar'; document.writeln(a.foo); This will output undefined: a holds a primitive value, which gets promoted to an object when assigning the property foo. But this new object is immediately discarded, so the value of foo is lost. Think of it like this: var a = 'quux';
This chapter documents all of JavaScript's standard, built-in objects, including their methods and properties. The term "global objects" (or standard built-in objects) here is not to be confused with the global object. Here, "global objects" refer to objects in the global scope. The global object itself can be accessed using the this operator ...
VS Code has built-in snippets for a number of languages such as: JavaScript, TypeScript, Markdown, and PHP. You can see the available snippets for a language by running the Insert Snippet command in the Command Palette to get a list of the snippets for the language of the current file. However, keep in mind that this list also includes user ...
how do I assign the values from the array in to these variables such that for the first time. var1 =1, var2=2, var3=3, var4=4, var5=5, var6=6. and the second time. var1 =7, var2 = 8, var3 = 9, var4 = 10, var5 = 11, var6 = 12. and so on. the length of the array is variables, but it will always be in a multiple of 6
The function passed to forEach is executed once for every item in the array, with the array item passed as the argument to the function. Unassigned values are not iterated in a forEach loop.. Note that the elements of an array that are omitted when the array is defined are not listed when iterating by forEach, but are listed when undefined has been manually assigned to the element:
The negative to using eval (or calling a shell to execute the code in your variable) is that it executes what you tell it to and so if you tell it to execute bad code then bad things will happen, but that's the same problem as telling the shell to execute a script, the only difference being that code stored in a variable is often created ...
JavaScript (JS) is a lightweight interpreted (or just-in-time compiled) programming language with first-class functions. While it is most well-known as the scripting language for Web pages, many non-browser environments also use it, such as Node.js, Apache CouchDB and Adobe Acrobat. JavaScript is a prototype-based, multi-paradigm, single-threaded, dynamic language, supporting object-oriented ...
JavaScript is a prototype-based language — an object's behaviors are specified by its own properties and its prototype's properties. However, with the addition of classes, the creation of hierarchies of objects and the inheritance of properties and their values are much more in line with other object-oriented languages such as Java. In this section, we will demonstrate how objects can be ...
Functions are one of the fundamental building blocks in JavaScript. A function in JavaScript is similar to a procedure—a set of statements that performs a task or calculates a value, but for a procedure to qualify as a function, it should take some input and return an output where there is some obvious relationship between the input and the output. To use a function, you must define it ...