- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- Compiler Design Tutorial
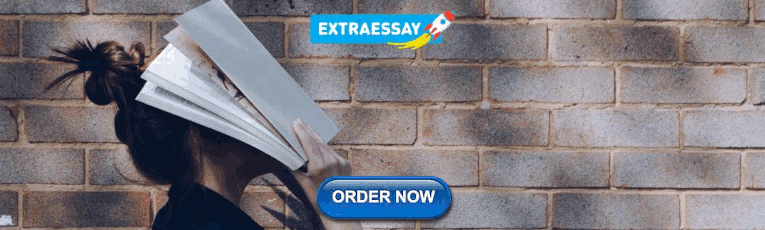
Introduction
- Introduction of Compiler Design
- Compiler construction tools
- Phases of a Compiler
- Symbol Table in Compiler
- Error detection and Recovery in Compiler
- Error Handling in Compiler Design
- Language Processors: Assembler, Compiler and Interpreter
- Generation of Programming Languages
Lexical Analysis
- Introduction of Lexical Analysis
- Flex (Fast Lexical Analyzer Generator )
- Introduction of Finite Automata
- Ambiguous Grammar
Syntax Analysis
- Introduction to Syntax Analysis in Compiler Design
- Why FIRST and FOLLOW in Compiler Design?
- FIRST Set in Syntax Analysis
- FOLLOW Set in Syntax Analysis
- Classification of Context Free Grammars
- Parsing | Set 1 (Introduction, Ambiguity and Parsers)
- Classification of Top Down Parsers
- Bottom-up or Shift Reduce Parsers | Set 2
- Shift Reduce Parser in Compiler
- SLR Parser (with Examples)
- CLR Parser (with Examples)
- Construction of LL(1) Parsing Table
- LALR Parser (with Examples)
Syntax Directed Translation
- Syntax Directed Translation in Compiler Design
- S - attributed and L - attributed SDTs in Syntax directed translation
- Parse Tree in Compiler Design
- Parse Tree and Syntax Tree
- Code Generation and Optimization
- Code Optimization in Compiler Design
- Intermediate Code Generation in Compiler Design
- Issues in the design of a code generator
- Three address code in Compiler
- Data flow analysis in Compiler
- Compiler Design | Detection of a Loop in Three Address Code
- Introduction of Object Code in Compiler Design
Runtime Environments
- Static and Dynamic Scoping
- Runtime Environments in Compiler Design
- Loader in C/C++
Compiler Design LMN
- Last Minute Notes - Compiler Design
Compiler Design GATE PYQ's and MCQs
- Lexical analysis
- Parsing and Syntax directed translation
- Compiler Design - GATE CSE Previous Year Questions
Last Minute Notes – Compiler Design
See Last Minute Notes on all subjects here .
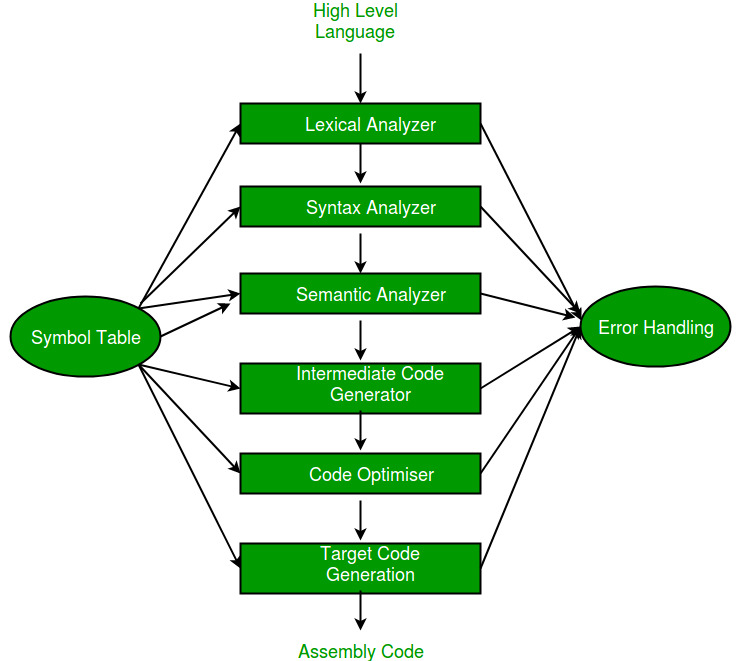
Symbol Table : It is a data structure being used and maintained by the compiler, consists all the identifier’s name along with their types. It helps the compiler to function smoothly by finding the identifiers quickly.
- Lexical Analysis : Lexical analyzer reads a source program character by character to produce tokens. Tokens can be identifiers, keywords, operators, separators etc.
- Syntax Analysis : Syntax analyzer is also known as parser. It constructs the parse tree. It takes all the tokens one by one and uses Context Free Grammar to construct the parse tree.
- Semantic Analyzer : It verifies the parse tree, whether it’s meaningful or not. It furthermore produces a verified parse tree.
- Intermediate Code Generator : It generates intermediate code, that is a form which can be readily executed by machine We have many popular intermediate codes.
- Code Optimizer : It transforms the code so that it consumes fewer resources and produces more speed.
- Target Code Generator : The main purpose of Target Code generator is to write a code that the machine can understand. The output is dependent on the type of assembler.
Error handling : The tasks of the Error Handling process are to detect each error, report it to the user, and then make some recover strategy and implement them to handle error. An Error is the blank entries in the symbol table. There are two types of error : Run-Time Error : A run-time error is an error which takes place during the execution of a program, and usually happens because of adverse system parameters or invalid input data. Compile-Time Error : Compile-time errors rises at compile time, before execution of the program.
- Lexical : This includes misspellings of identifiers, keywords or operators.
- Syntactical : missing semicolon or unbalanced parenthesis.
- Semantical : incompatible value assignment or type mismatches between operator and operand.
- Logical : code not reachable, infinite loop.
Left Recursion : The grammar : A -> Aa | a is left recursive. Top down parsing techniques cannot handle left recursive grammar so we convert left recursion into right recursion. Left recursion elimination : A -> Aa | a ⇒ A -> aA’ A’ -> aA’ | a
Left Factoring : If a grammar has common prefixes in r.h.s of nonterminal then suh grammar needs to be left factored by eliminating common prefixes as follows : A -> ab1 | ac2 ⇒ A -> A -> aA’ A’ -> A -> b1 | c2
FIRST(A) is a set of the terminal symbols which occur as first symbols in string derived from A
FOLLOW(A) is the set of terminals which occur immediately after the nonterminal A in the strings derived from the starting symbol.
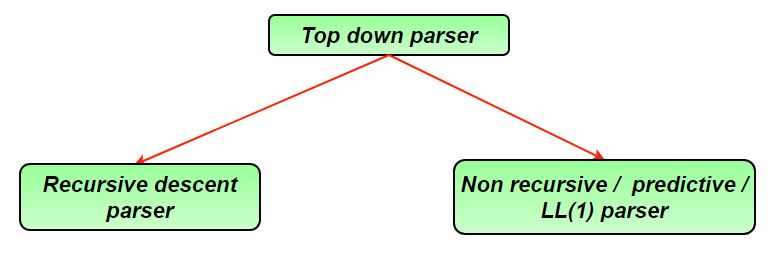
LL(1) Parser : LL(1) grammar is unambiguous, left factored and non left recursive. To check whether a grammar is LL(1) or not : 1. If A -> B1 | C2 ⇒ { FIRST(B1) ∩ FIRST(C2 ) = φ } 2. If A -> B | ∈ ⇒ { FIRST(B) ∩ FOLLOW(A) = φ }
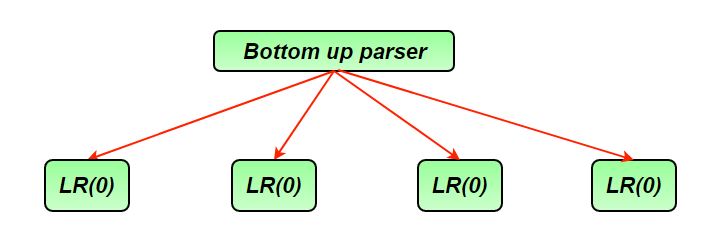
LR(0) Parser : Closure() and goto() functions are used to create canonical collection of LR items. Conflicts in LR(0) parser : 1. Shift Reduce (SR) conflict : when the same state in DFA contains both shift and reduce items. A -> B . xC (shifting) B -> a. (reduced) 2. Reduced Reduced (RR) conflict : two reductions in same state of DFA A -> a. (reduced) B -> b. (reduced)
SLR Parser : It is powerful than LR(0). Ever LR(0) is SLR but every SLR need not be LR(0). Conflicts in SLR 1. SR conflict : A -> B . xC (shifting) B -> a. (reduced) if FOLLOW(B) ∩ {x} ≠ φ 2. RR conflict : A -> a. (reduced) B -> b. (reduced) if FOLLOW(A) ∩ FOLLOW(B) ≠ φ
CLR Parser : It is same as SLR parser except that the reduced entries in CLR parsing table go only in the FOLLOW of the l.h.s nonterminal.
LALR Parser : It is constructed from CLR parser, if two states having same productions but may contain different lookaheads, those two states of CLR are combined into single state in LALR. Every LALR grammar is CLR but every CLR grammar need not be LALR. Parsers Comparison : LR(0) ⊂ SLR ⊂ LALR ⊂ CLR LL(1) ⊂ LALR ⊂ CLR If number of states LR(0) = n1, number of states SLR = n2, number of states LALR = n3, number of states CLR = n4 then, n1 = n2 = n3 <= n4
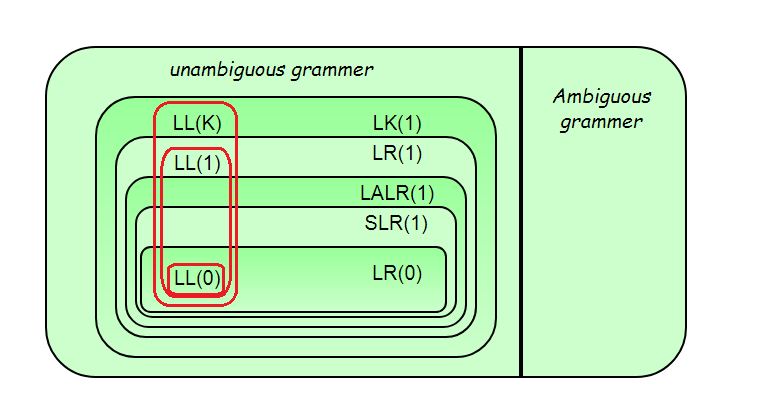
Syntax Directed Translation : Syntax Directed Translation are augmented rules to the grammar that facilitate semantic analysis. Eg – S -> AB {print (*)} A -> a {print (1)} B -> b {print (2)} Synthesized Attribute : attribute whose value is evaluated in terms of attribute values of its children. Inherited Attribute : attribute whose value is evaluated in terms of attribute values of siblings or parents.
S-attributed SDT : If an SDT uses only synthesized attributes, it is called as S-attributed SDT. S-attributed SDTs are evaluated in bottom-up parsing, as the values of the parent nodes depend upon the values of the child nodes. L-attributed SDT : If an SDT uses either synthesized attributes or inherited attributes with a restriction that it can inherit values from left siblings only, it is called as L-attributed SDT. Attributes in L-attributed SDTs are evaluated by depth-first and left-to-right parsing manner.
Activation Record : Information needed by a single execution of a procedure is managed using a contiguous block of storage called activation record. An activation record is allocated when a procedure is entered and it is deallocated when that procedure is exited.
Intermediate Code : They are machine independent codes. Syntax trees, postfix notation, 3-address codes can be used to represent intermediate code.
Three address code : 1. Quadruples (4 fields : operator, operand1, operand2, result) 2. Triplets (3 fields : operator, operand1, operand2) 3. Indirect triples
Code Optimization :
Types of machine independent optimizations – 1. Loop optimizations :
- Code motion : reduce the evaluation frequency of expression.
- Loop unrolling : to execute less number of iterations
- Loop jamming : combine body of two loops whenever they are sharing same index.
2. Constant folding : replacing the value of constants during compilation 3. Constant propagation : replacing the value of an expression during compile time. 4. Strength reduction : replacing costly operators by simple operators.
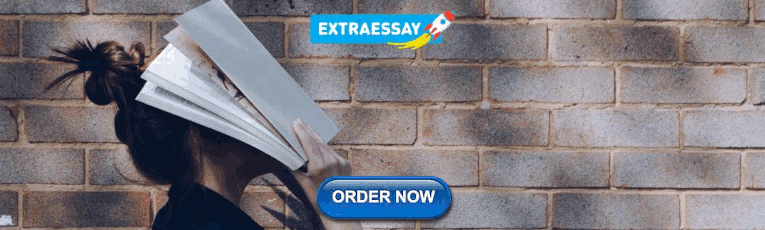
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?
Compiler Design
- Lesson Plan
Assignment Questions

Explain SEmantic Analyzer and Intermediate code generator in terms of compiler design ... please answer
Important Short Questions and Answers: Principles of Compiler Design - Intermediate Code Generation - Computer Science Engineering (CSE) PDF Download
1. What are the benefits of using machine-independent intermediate form?
· Retargeting is facilitated; a compiler for a different machine can be created by attaching a back end for the new machine to an existing front end.
· A machine-independent code optimizer can be applied to the intermediate representation.
2. List the three kinds of intermediate representation.
The three kinds of intermediate representations are i. Syntax trees ii. Postfix notation iii. Three address code
3. How can you generate three-address code?
The three-address code is generated using semantic rules that are similar to those for constructing syntax trees for generating postfix notation.
4. What is a syntax tree? Draw the syntax tree for the assignment statement a := b * -c + b * -c.
· A syntax tree depicts the natural hierarchical structure of a source program.
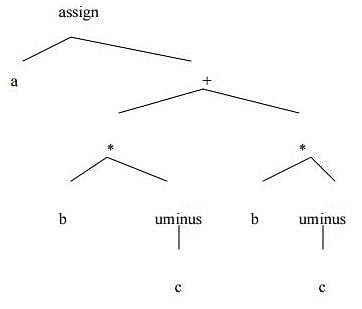
5. What is postfix notation?
A Postfix notation is a linearized representation of a syntax tree. It is a list of nodes of the tree in which a node appears immediately after its children.
6. What is the usage of syntax directed definition. Syntax trees for assignment statement are produced by the syntax directed definition.
7. Why “Three address code” is named so?
The reason for the term “Three address code” is that each usually contains three addresses, two for operands and one for the result.
8. Define three-address code.
· Three-address code is a sequence of statements of the general form x := y op z
where x, y and z are names, constants, or compiler-generated temporaries; op stands for any operator, such as fixed or floating-point arithmetic operator, or a logical operator on boolean-valued data.
· Three-address code is a linearized representation of a syntax tree or a dag in which explicit names correspond to the interior nodes of the graph.
9. State quadruple
A quadruple is a record structure with four fields, which we call op, arg1, arg2 and result.
10. What is called an abstract or syntax tree?
A tree in which each leaf represents an operand and each interior node an operator is called as abstract or syntax tree.
11. Construct Three address code for the following
position := initial + rate * 60
Ans: temp1 := inttoreal(60) temp2 := id3 * temp1 temp3 := id2 + temp2 id1 := temp3
12. What are triples?
· The fields arg1,and arg2 for the arguments of op, are either pointers to the symbol table or pointers into the triple structure then the three fields used in the intermediate code format are called triples.
· In other words the intermediate code format is known as triples.
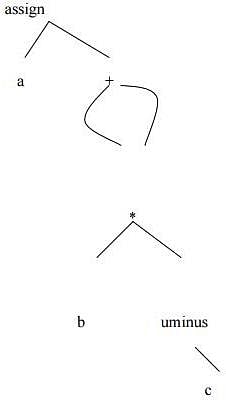
14. List the types of three address statements. The types of three address statements are a. Assignment statements b. Assignment Instructions c. Copy statements d. Unconditional Jumps e. Conditional jumps f. Indexed assignments g. Address and pointer assignments h. Procedure calls and return
15. What are the various methods of implementing three-address statements? i. Quadruples ii. Triples iii. Indirect triples
16. What is meant by declaration? The process of declaring keywords, procedures, functions, variables, and statements with proper syntax is called declaration.
17. How semantic rules are defined?
The semantic rules are defined by the following ways a. mktable(previous) b. enter(table,name,type,offset) c. addwith(table,width) d. enterproc(table,name,newtable)
18. What are the two primary purposes of Boolean Expressions?
· They are used to compute logical values
· They are used as conditional expressions in statements that alter the flow of control, such as if-then, if-then-else, or while-do statements.
19. Define Boolean Expression.
Expressions which are composed of the Boolean operators (and, or, and not) applied to elements that are Boolean variables or relational expressions are known as Boolean expressions
20. What are the two methods to represent the value of a Boolean expression? i.The first method is to encode true and false numerically and to evaluate a Boolean expression analogously to an arithmetic expression. ii. The second principal method of implementing Boolean expression is by flow of control that is representing the value of a Boolean expression by a position reached in a program.
21. What do you mean by viable prefixes. Nov/Dec 2004
Viable prefixes are the set of prefixes of right sentinels forms that can appear on the stack of shift/reduce parser are called viable prefixes. It is always possible to add terminal symbols to the end of the viable prefix to obtain a right sentential form.
22. What is meant by Shot-Circuit or jumping code?
We can also translate a Boolean expression into three-address code without generating code for any of the Boolean operators and without having the code necessarily evaluate the entire expression. This style of evaluation is sometimes called “short-circuit” or “jumping” code.
23. What is known as calling sequence?
A sequence of actions taken on entry to and exit from each procedure is known as calling sequence.
What is the intermediate code representation for the expression a or b and not c? (Or) Translate a or b and not c into three address code.
Three-address sequence is
t1 := not c
t2 := b and t1
t3 := a or t2
25. Translate the conditional statement if a<b then 1 else 0 into three address code. Three-address sequence is 100: if a < b goto 103 101: t := 0 102: goto 104 103: t := 1 104:
26. Explain the following functions:
i) makelist( i ) ii) merge( p1,p2 ) iii) backpatch( p,i ) i. makelist( i ) creates a new list containing only I, an index into the array of quadruples; makelist returns a pointer to the list it has made. ii. merge( p1,p2 ) concatenates the lists pointed to by p1 and p2 , and returns a pointer to the concatenated list. iii. backpatch( p,i ) inserts i as the target label for each of the statements on the list pointed to by p .
27. Define back patching. May/June 2007 & Nov/Dec 2007
Back patching is the activity of filling up unspecified information of labels using appropriate semantic actions in during the code generation process.
28. What are the methods of representing a syntax tree?
i. Each node is represented as a record with a field for its operator and additional fields for pointers to its children.
ii. Nodes are allocated from an array of records and the index or position of the node serves as the pointer to the node
29. Give the syntax directed definition for if-else statement. Ans:
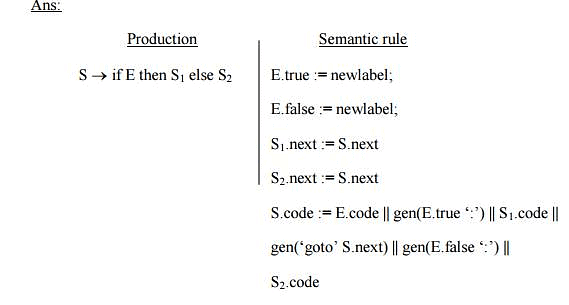
Top Courses for Computer Science Engineering (CSE)
Faqs on important short questions and answers: principles of compiler design - intermediate code generation - computer science engineering (cse), viva questions, important short questions and answers: principles of compiler design - intermediate code generation - computer science engineering (cse), extra questions, semester notes, past year papers, important questions, previous year questions with solutions, video lectures, practice quizzes, shortcuts and tricks, mock tests for examination, objective type questions, study material, sample paper.
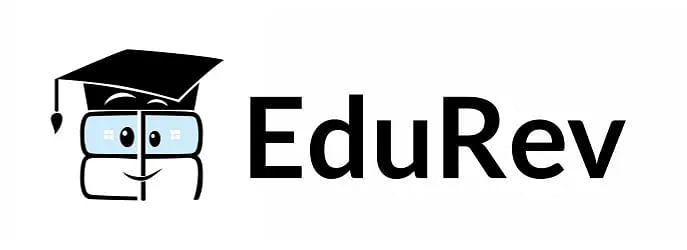
Important Short Questions and Answers: Principles of Compiler Design - Intermediate Code Generation Free PDF Download
Importance of important short questions and answers: principles of compiler design - intermediate code generation, important short questions and answers: principles of compiler design - intermediate code generation notes, important short questions and answers: principles of compiler design - intermediate code generation computer science engineering (cse), study important short questions and answers: principles of compiler design - intermediate code generation on the app, welcome back, create your account for free.

Forgot Password
Unattempted tests, change country, practice & revise.
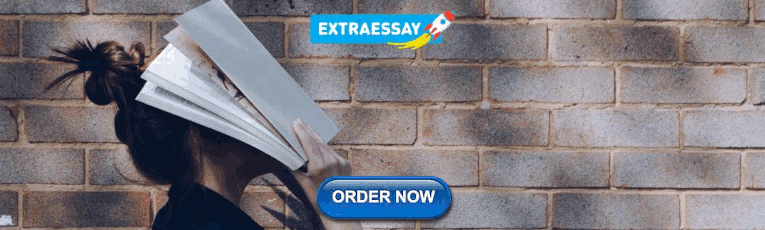
IMAGES
VIDEO
COMMENTS
from Introduction to Compiler Design Third Edition Torben Æ. Mogensen Last update: December 7, 2023 Introduction This document provides solutions for selected exercises from "Introduction to Compiler Design", third edition. Most of the exercises are numbered the same in the second edition.
Construct the DAG for the following basic blocks [L3, 10M] 8. Explain the simple code generator and generate target code sequence for the following statement d:=(a-b)+(a-c)+(a-c) [L2, 10M] 9. Write short notes on i)Simple code generator [L6, 5+5M] ii) Register allocation. 10. Explain the following terms.
CS 3501 Compiler Design Question Bank with answer Unit 1-4 - Free download as PDF File (.pdf), Text File (.txt) or read online for free.
a. Assignment b. Unconditional jump (goto) c. Array statement (2D and 3D) d. Boolean expression e. If-then-else statement f. While, do-while statement g. Switch case statement UNIT 4 1. Write the definition of symbol table and procedure to store the names in symbol table. 2. What are the data structures used in symbol table? 3.
Last Minute Notes - Compiler Design. See Last Minute Notes on all subjects here. Phases of Compiler: Symbol Table : It is a data structure being used and maintained by the compiler, consists all the identifier's name along with their types. It helps the compiler to function smoothly by finding the identifiers quickly.
Short Answer Questions 1. Define Compiler and what are the phases of compiler. ... Assignments statements. (ii) Case statements. 7. Write three codes for x:=A[y, z] with detail explanation. ... What is stack allocation in compiler design. Long Answer Questions 1. Explain principle sources of optimization in details.
LONG questions: Explain the various phases of a compiler with an illustrative example Define Regular expression. Explain the properties of Regular expressions. Differentiate between top down and bottom up parsing techniques. Construct an FA equivalent to the regular expression (0+1)*(00+11)(0+1)* Explain the various phases of a compiler in detail.
15-411 Compiler DesignLabs & Assignments. 15-411 Compiler Design. Labs & Assignments. The lab and assignment schedule is tentative! The labs are the heart of this course and count for 70% of your grade. Much of what you learn in this course will be through completing these labs. Labs can be done individually or in pairs.
Assignment_1_Compiler Design - Free download as PDF File (.pdf), Text File (.txt) or read online for free. ... The correct answers are provided for each question along with short explanations for some answers. The quiz covers fundamental concepts and phases of compiler design. This document contains a 12 question multiple choice quiz about ...
C311.2 Evaluating the role of tokens in analysis phase of compiler. C311.3 Evaluating the role of Parser in a compiler. C311.4 Summarize the semantic action taken by the compiler during semantic phase of the compiler. C311.5 Analyse the method of finding code generation and code optimization techniques in compilation.
Compiler Design Important Questions — 1. What is a compiler? A compiler is a program that reads a program written in one language -the source language and translates it into an equivalent ...
The section contains Compiler Design multiple choice questions and answers on code optimization, elimination of induction variables, eliminating global common subexpressions, loop optimization, unrolling and jamming. Code Optimization - 1. Code Optimization - 2. Loop Optimization - 1.
Assignment Questions. Explain the role of assembler, compiler, loader and linker in the language processing system. Explain the role of Lexical Analysis. Show the output of each phase in compiler for the following statement. Explain about input buffering in detail. Design a transition diagram for identifier and the keyword "else".
Assignment [Unit-1] Sno Question CO Bloom Level 1 Explain in detail the process of compilation for the statement a =b+c* 1 L 2 Write subset construction algorithm to convert NFA into DFA. 1 L 3 Write the algorithm for moving forward pointer in "input buffering" scheme. 1 L 4 Design a DFA for the following regular expression: (x+y)*xyy using ...
Assignment_4_Compiler Design - Free download as PDF File (.pdf), Text File (.txt) or read online for free. This document contains an 11 question multiple choice quiz on the topic of compiler design. The questions cover topics such as the definition of terminals and non-terminals in a language, ambiguous and unambiguous grammars, regular expressions and regular grammars, left factoring and left ...
CD Important questions with answers; Compiler Design U2 - it is very helpful for revision; Compiler Design U5 - it is very helpful for revision; 156AN - Design AND Analysis OF Algorithms ... We assume that the values of operands (y and z) are not changed from assignment of variable a to variable c. Here, if the condition statement is true, then ...
CS 3501 Compiler Design Question Bank with answer Unit 1 - Free download as PDF File (.pdf), Text File (.txt) or read online for free.
Full syllabus notes, lecture and questions for Important Short Questions and Answers: Principles of Compiler Design - Intermediate Code Generation - Computer Science Engineering (CSE) - Computer Science Engineering (CSE) - Plus excerises question with solution to help you revise complete syllabus - Best notes, free PDF download
Compiler Design Question 12 Detailed Solution. Download Solution PDF. In second production T → T * U here T is generating T*U left recursively so * is left associative. In third production P → Q + P, Here P is generating Q + P right recursively so + is right associative. Hence option 2 is the correct answer.
This document lists over 400 compiler design lab viva questions and answers. It begins by defining a compiler as a program that translates a program written in one language into an equivalent program in another language. It then covers topics like the two parts of compilation, phases of analysis and synthesis, classifications of compilers, symbol tables, compiler construction tools, regular ...
Crypto - Assignment 2 Questions; PP - Crypto Assignment 2 Answers; HJ - Crypto Assignment 2 Answers; Sem 6 - .NET Syllabus - PSNM; ... Compiler Design Assignment-PIET. 1 Explain lexical analysis phase of a compiler and, for a statement given below, write output of all phases (except of an optimization phase) of a complier. Assume a, b and c of ...
Compiler Design Mcq Questions and Answers - Free download as PDF File (.pdf), Text File (.txt) or read online for free. This document contains 10 multiple choice questions about compiler design topics like types of compilers, advantages of compilers over interpreters, symbol table implementations, parser types, parsing processes, and compiler optimization techniques.