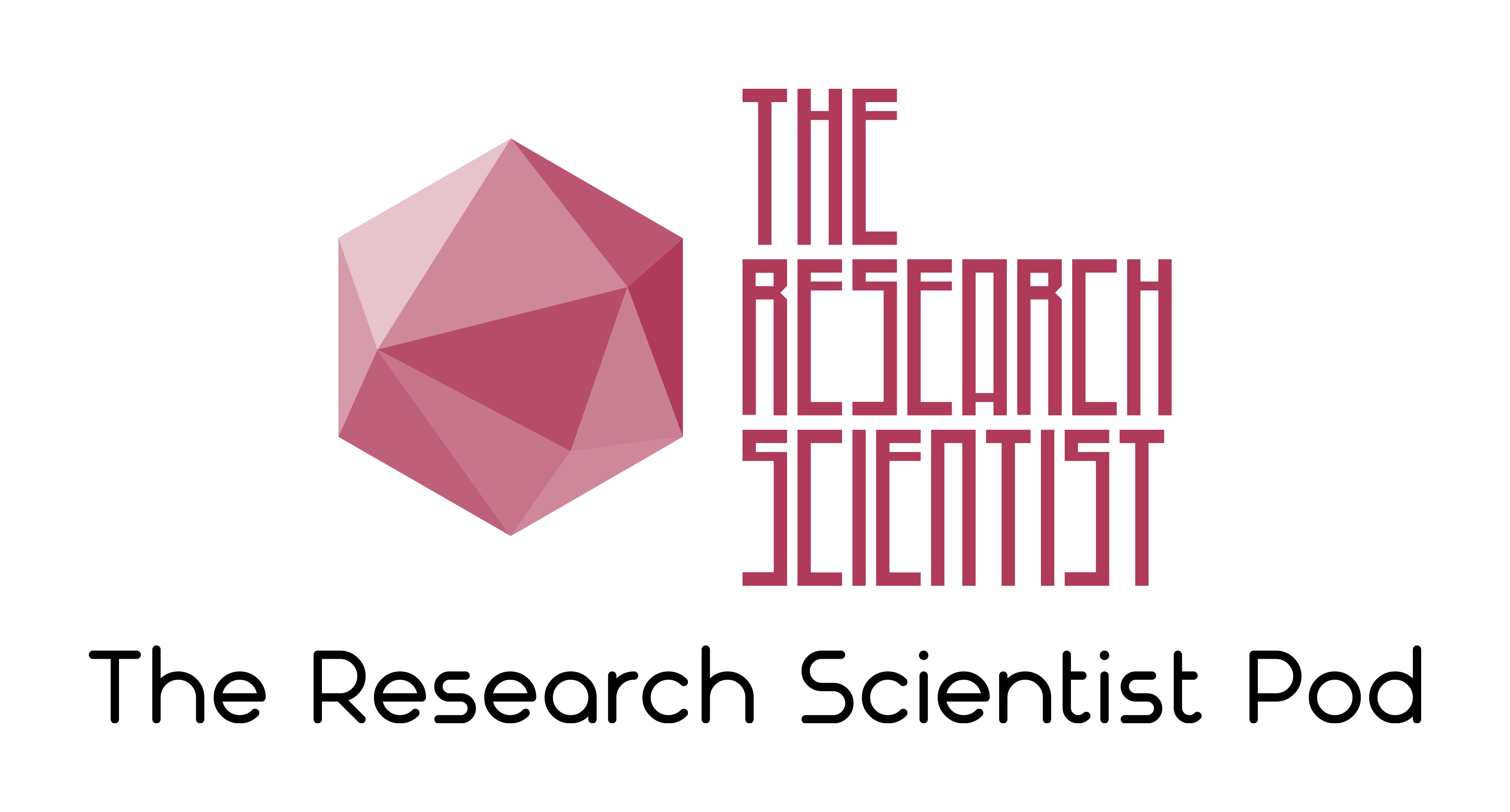
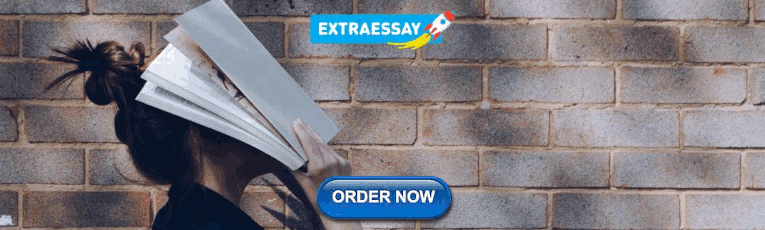
Python UnboundLocalError: local variable referenced before assignment
by Suf | Programming , Python , Tips
If you try to reference a local variable before assigning a value to it within the body of a function, you will encounter the UnboundLocalError: local variable referenced before assignment.
The preferable way to solve this error is to pass parameters to your function, for example:
Alternatively, you can declare the variable as global to access it while inside a function. For example,
This tutorial will go through the error in detail and how to solve it with code examples .
Table of contents
What is scope in python, unboundlocalerror: local variable referenced before assignment, solution #1: passing parameters to the function, solution #2: use global keyword, solution #1: include else statement, solution #2: use global keyword.
Scope refers to a variable being only available inside the region where it was created. A variable created inside a function belongs to the local scope of that function, and we can only use that variable inside that function.
A variable created in the main body of the Python code is a global variable and belongs to the global scope. Global variables are available within any scope, global and local.
UnboundLocalError occurs when we try to modify a variable defined as local before creating it. If we only need to read a variable within a function, we can do so without using the global keyword. Consider the following example that demonstrates a variable var created with global scope and accessed from test_func :
If we try to assign a value to var within test_func , the Python interpreter will raise the UnboundLocalError:
This error occurs because when we make an assignment to a variable in a scope, that variable becomes local to that scope and overrides any variable with the same name in the global or outer scope.
var +=1 is similar to var = var + 1 , therefore the Python interpreter should first read var , perform the addition and assign the value back to var .
var is a variable local to test_func , so the variable is read or referenced before we have assigned it. As a result, the Python interpreter raises the UnboundLocalError.
Example #1: Accessing a Local Variable
Let’s look at an example where we define a global variable number. We will use the increment_func to increase the numerical value of number by 1.
Let’s run the code to see what happens:
The error occurs because we tried to read a local variable before assigning a value to it.
We can solve this error by passing a parameter to increment_func . This solution is the preferred approach. Typically Python developers avoid declaring global variables unless they are necessary. Let’s look at the revised code:
We have assigned a value to number and passed it to the increment_func , which will resolve the UnboundLocalError. Let’s run the code to see the result:
We successfully printed the value to the console.
We also can solve this error by using the global keyword. The global statement tells the Python interpreter that inside increment_func , the variable number is a global variable even if we assign to it in increment_func . Let’s look at the revised code:
Let’s run the code to see the result:
Example #2: Function with if-elif statements
Let’s look at an example where we collect a score from a player of a game to rank their level of expertise. The variable we will use is called score and the calculate_level function takes in score as a parameter and returns a string containing the player’s level .
In the above code, we have a series of if-elif statements for assigning a string to the level variable. Let’s run the code to see what happens:
The error occurs because we input a score equal to 40 . The conditional statements in the function do not account for a value below 55 , therefore when we call the calculate_level function, Python will attempt to return level without any value assigned to it.
We can solve this error by completing the set of conditions with an else statement. The else statement will provide an assignment to level for all scores lower than 55 . Let’s look at the revised code:
In the above code, all scores below 55 are given the beginner level. Let’s run the code to see what happens:
We can also create a global variable level and then use the global keyword inside calculate_level . Using the global keyword will ensure that the variable is available in the local scope of the calculate_level function. Let’s look at the revised code.
In the above code, we put the global statement inside the function and at the beginning. Note that the “default” value of level is beginner and we do not include the else statement in the function. Let’s run the code to see the result:
Congratulations on reading to the end of this tutorial! The UnboundLocalError: local variable referenced before assignment occurs when you try to reference a local variable before assigning a value to it. Preferably, you can solve this error by passing parameters to your function. Alternatively, you can use the global keyword.
If you have if-elif statements in your code where you assign a value to a local variable and do not account for all outcomes, you may encounter this error. In which case, you must include an else statement to account for the missing outcome.
For further reading on Python code blocks and structure, go to the article: How to Solve Python IndentationError: unindent does not match any outer indentation level .
Go to the online courses page on Python to learn more about Python for data science and machine learning.
Have fun and happy researching!
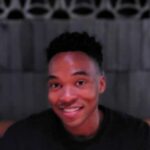
Suf is a senior advisor in data science with deep expertise in Natural Language Processing, Complex Networks, and Anomaly Detection. Formerly a postdoctoral research fellow, he applied advanced physics techniques to tackle real-world, data-heavy industry challenges. Before that, he was a particle physicist at the ATLAS Experiment of the Large Hadron Collider. Now, he’s focused on bringing more fun and curiosity to the world of science and research online.
- Suf https://researchdatapod.com/author/soofyserial/ Black-Scholes Option Pricing in Python
- Suf https://researchdatapod.com/author/soofyserial/ How to Solve Python TypeError: Object of type dict_items is not JSON serializable
- Suf https://researchdatapod.com/author/soofyserial/ How to Solve Python ValueError: input contains nan, infinity or a value too large for dtype('float64')
- Suf https://researchdatapod.com/author/soofyserial/ How to Solve Python ModuleNotFoundError: no module named ‘parso’
Buy Me a Coffee
- Python Course
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
UnboundLocalError Local variable Referenced Before Assignment in Python
Handling errors is an integral part of writing robust and reliable Python code. One common stumbling block that developers often encounter is the “UnboundLocalError” raised within a try-except block. This error can be perplexing for those unfamiliar with its nuances but fear not – in this article, we will delve into the intricacies of the UnboundLocalError and provide a comprehensive guide on how to effectively use try-except statements to resolve it.
What is UnboundLocalError Local variable Referenced Before Assignment in Python?
The UnboundLocalError occurs when a local variable is referenced before it has been assigned a value within a function or method. This error typically surfaces when utilizing try-except blocks to handle exceptions, creating a puzzle for developers trying to comprehend its origins and find a solution.
Why does UnboundLocalError: Local variable Referenced Before Assignment Occur?
below, are the reasons of occurring “Unboundlocalerror: Try Except Statements” in Python :
Variable Assignment Inside Try Block
Reassigning a global variable inside except block.
- Accessing a Variable Defined Inside an If Block
In the below code, example_function attempts to execute some_operation within a try-except block. If an exception occurs, it prints an error message. However, if no exception occurs, it prints the value of the variable result outside the try block, leading to an UnboundLocalError since result might not be defined if an exception was caught.
In below code , modify_global function attempts to increment the global variable global_var within a try block, but it raises an UnboundLocalError. This error occurs because the function treats global_var as a local variable due to the assignment operation within the try block.
Solution for UnboundLocalError Local variable Referenced Before Assignment
Below, are the approaches to solve “Unboundlocalerror: Try Except Statements”.
Initialize Variables Outside the Try Block
Avoid reassignment of global variables.
In modification to the example_function is correct. Initializing the variable result before the try block ensures that it exists even if an exception occurs within the try block. This helps prevent UnboundLocalError when trying to access result in the print statement outside the try block.
Below, code calculates a new value ( local_var ) based on the global variable and then prints both the local and global variables separately. It demonstrates that the global variable is accessed directly without being reassigned within the function.
In conclusion , To fix “UnboundLocalError” related to try-except statements, ensure that variables used within the try block are initialized before the try block starts. This can be achieved by declaring the variables with default values or assigning them None outside the try block. Additionally, when modifying global variables within a try block, use the `global` keyword to explicitly declare them.
Similar Reads
- Python Programs
- Python Errors
Please Login to comment...
- How to Watch NFL on NFL+ in 2024: A Complete Guide
- Best Smartwatches in 2024: Top Picks for Every Need
- Top Budgeting Apps in 2024
- 10 Best Parental Control App in 2024
- GeeksforGeeks Practice - Leading Online Coding Platform
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
"UnboundLocalError: local variable 'os' referenced before assignment"
OS: macOS Sonoma 14.0 (on an M2 chip) PsychoPy version: 2023.2.3 Standard Standalone? (y/n) y What are you trying to achieve?: Upgrade an experiment from 2021.1.4 to 2023.2.3
Context: Our lab computers were updated and 2021.1.4 was no longer working (tasks would not start). I think it had something to do with losing support for python 2.X, but I decided to just move forward and upgrade versions. Note: I didn’t go straight to 2023.2.3, but tried some other versions along the way.
What did you try to make it work?: Installed v2023.2.3, updated “use version” to 2023.2.3 Other details: window backend = pyglet, audio library = ptb (but experiment has no sound anyway), keyboard backend = PsychToolbox
What specifically went wrong when you tried that?: I got an: "UnboundLocalError: local variable 'os' referenced before assignment" …referring to line 301 in the generated python script which uses os.chdir(_thisDir) early on in the “run” fuction. According to posts on this forum, an UnboundLocalError is usually due to a problematic conditions file. However, an error with the os package in the run function seems to be a deeper problem. Have I totally corrupted the experiment by changing versions?
(somewhat redacted) error output:
Seems like it could be something like this scoping issue , but I have no idea how the py code got this way.
What happens if use version is blank?

Update: if I set useVersion back to v2022.1.0 (one of the previous versions this experiment passed through), the error does not occur and I am able to run the experiment normally. However, this version is on the list of versions to avoid … so I’d rather avoid it if possible.
Hi, I came into the same problem today. And I’m completely new with Psychopy, so you may not find my solution useful given that you may have already tried it. But for other users as new as me, we should put code component before the other stimuli component (e.g., text, image). Psychopy needs to run the code before it runs the stimuli.
I think this will depend on whether you need the results of that code component to display other components in that routine. There are other situations where a code component would need to come at the end of a routine.
My friend was having the same issue as you. We have been able to solve it. The problem is exactly the scoping issue you were referring to.
The problem is this: In the version 2023.2.3, when you build the project, generated code already imports os in global scope. However, unlike some of the older versions (2021, 2022) all the code related to the routines are under a function run(). At the beginning of this run() function, os module is used with the function os.chdir(). Crucial part is that, this call is being made before any other routine. The problem arises when in one of your routines, you write the statement “import os”. When that happens, the scope issue arises because os is already defined globally, and you are defining it locally, but you already tried to access it before you define it locally. So the exact situation in stackoverflow post happens.
Why wasn’t it happening in older versions? Because in those versions, when you build the project, all the code related to your routines are still under the global scope, unlike newer versions where its under the local scope of run() function.
Related Topics
Topic | Replies | Views | Activity | |
---|---|---|---|---|
Builder | 10 | 4329 | February 9, 2024 | |
Coding | 5 | 628 | February 29, 2024 | |
Builder | 4 | 331 | March 27, 2024 | |
Builder | 8 | 229 | March 11, 2024 | |
Builder | 2 | 263 | April 22, 2024 |
【Python】成功解决python报错:UnboundLocalError: local variable ‘xxx‘ referenced before assignment

成功解决python报错:UnboundLocalError: local variable ‘xxx’ referenced before assignment。在Python中, UnboundLocalError 是一种特定的 NameError ,它会在尝试引用一个还未被赋值的局部变量时发生。Python解释器需要知道变量的类型和作用域,因此,在局部作用域内引用一个未被赋值的变量时,就会抛出这个错误。
🧑 博主简介:现任阿里巴巴嵌入式技术专家,15年工作经验,深耕嵌入式+人工智能领域,精通嵌入式领域开发、技术管理、简历招聘面试。CSDN优质创作者,提供产品测评、学习辅导、简历面试辅导、毕设辅导、项目开发、C/C++/Java/Python/Linux/AI等方面的服务,如有需要请站内私信或者联系任意文章底部的的VX名片(ID: gylzbk )
💬 博主粉丝群介绍:① 群内高中生、本科生、研究生、博士生遍布,可互相学习,交流困惑。② 热榜top10的常客也在群里,也有数不清的万粉大佬,可以交流写作技巧,上榜经验,涨粉秘籍。③ 群内也有职场精英,大厂大佬,可交流技术、面试、找工作的经验。④ 进群免费赠送写作秘籍一份,助你由写作小白晋升为创作大佬。⑤ 进群赠送CSDN评论防封脚本,送真活跃粉丝,助你提升文章热度。有兴趣的加文末联系方式,备注自己的CSDN昵称,拉你进群,互相学习共同进步。
【Python】解决Python报错:
1. 什么是unboundlocalerror?, 2. 常见的场景和原因, 方法一:全局变量, 方法二:函数参数, 方法三:局部变量初始化, 方法四:结合条件语句.
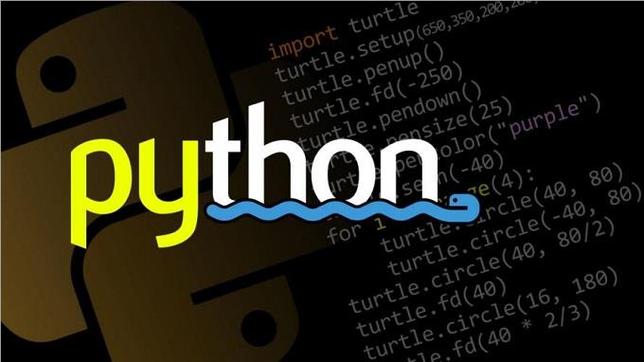
在Python编程中, UnboundLocalError: local variable 'xxx' referenced before assignment 是一个常见的错误,尤其是在写函数时可能会遇到。这篇技术博客将详细介绍 UnboundLocalError ,为什么会发生,以及如何解决这个错误。
在Python中, UnboundLocalError 是一种特定的 NameError ,它会在尝试引用一个还未被赋值的局部变量时发生。Python解释器需要知道变量的类型和作用域,因此,在局部作用域内引用一个未被赋值的变量时,就会抛出这个错误。
这是一个简单的代码示例来说明这个错误:
运行以上代码会抛出以下错误:
在这个例子中,Python解释器看到 print(x) 时,寻找局部作用域中的变量 x ,但这个变量在局部作用域内尚未被赋值(虽然在后面有赋值,解释器是从上到下执行代码的)。
理解错误的原因后,可以通过以下几种方式来解决 UnboundLocalError :
如果变量希望在函数内和函数外都使用,可以将其声明为全局变量:
通过在函数内使用 global 关键字,将 x 声明为全局变量,这样即使在函数内也能访问全局变量 x 。
通过将变量作为参数传递给函数,使得函数内可以访问并使用这个变量:
在这种情况下, x 是函数 my_function 的一个参数,无需在函数内部声明。
在使用变量之前,先初始化该局部变量:
确保在函数内部引用变量之前,该变量已经被赋值。
在复杂的逻辑中,特别是在涉及条件语句时,可以先在函数开始部分初始化变量,确保无论哪条路径都可以正确访问该变量:
在这个例子中,我们确保了变量 x 在函数内部任何地方都能被适当地引用。
- 命名冲突 :在全局变量和局部变量重名情况下,优先使用局部变量。如果不小心混用,容易引发错误。
- 提前规划变量作用域 :代码设计时,可以提前规划好变量应该属于哪个作用域,以减少变量冲突和未定义变量的情况。
UnboundLocalError: local variable 'xxx' referenced before assignment 错误是一个常见的初学者错误,但只要理解了Python的变量作用域规则和执行顺序,就可以轻松避开。通过合适的解决方法,如使用全局变量、函数参数、局部变量初始化或结合条件语句,可以高效且清晰地管理变量的使用。
希望这篇文章能帮助你理解和解决这个错误。如果有任何问题或其他建议,欢迎在评论中与我们讨论。Happy coding!
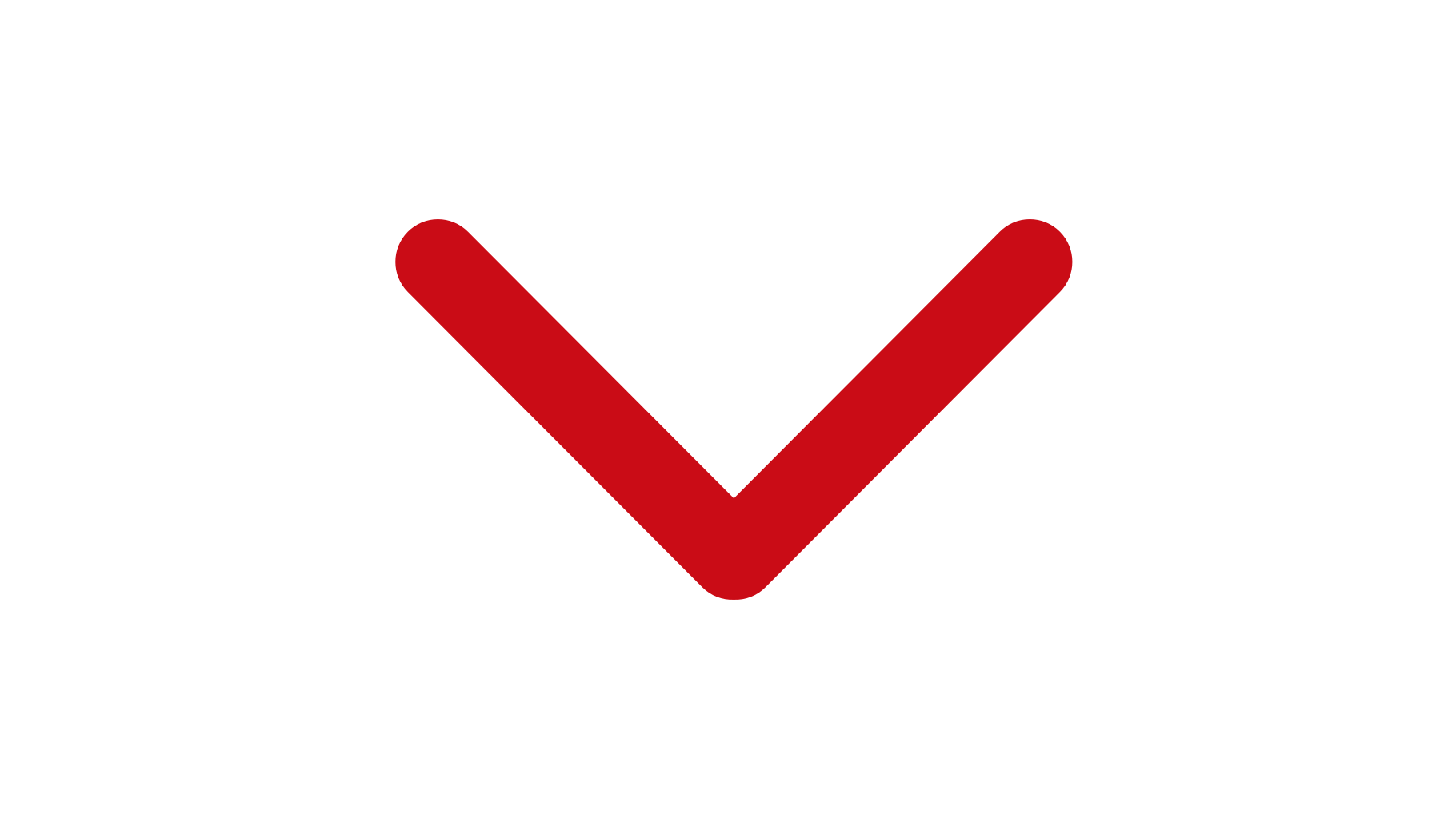
请填写红包祝福语或标题
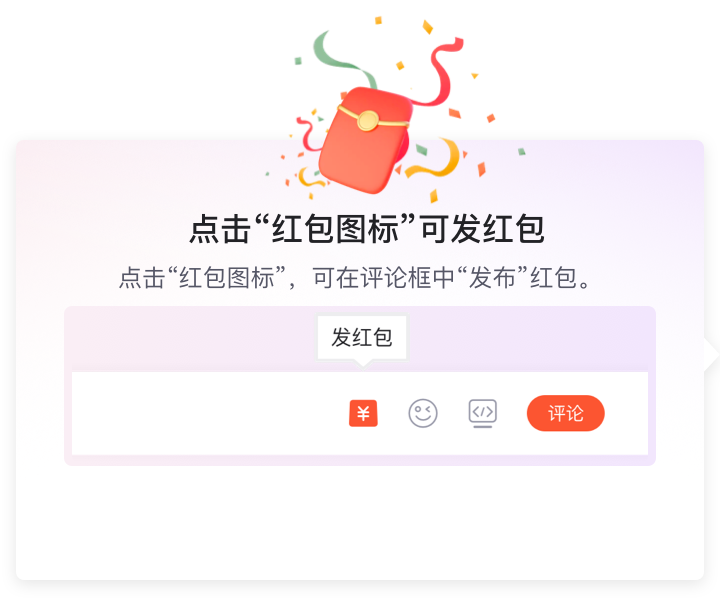
你的鼓励将是我创作的最大动力

您的余额不足,请更换扫码支付或 充值

1.余额是钱包充值的虚拟货币,按照1:1的比例进行支付金额的抵扣。 2.余额无法直接购买下载,可以购买VIP、付费专栏及课程。

[Python] UnboundLocalErrorとは?発生原因や対処法・回避方法を解説
Pythonで UnboundLocalError は、ローカル変数が参照される前に割り当てられていない場合に発生します。
通常、関数内でグローバル変数と同じ名前の変数を使用しようとすると、このエラーが発生します。
この問題を回避するには、 global キーワードを使用してグローバル変数を明示的に参照するか、変数名を変更してローカルスコープでの競合を避けることが推奨されます。
また、 nonlocal キーワードを使用して、ネストされた関数内で外側のスコープの変数を参照することも可能です。
- UnboundLocalErrorの基本的な理解
- エラーが発生する具体的なシナリオ
- グローバル変数やローカル変数の正しい使い方
- nonlocalキーワードの活用方法
- 大規模プロジェクトやデータサイエンスにおける変数管理の重要性
UnboundLocalErrorとは?
Pythonにおける UnboundLocalError は、ローカルスコープ内で変数が参照される際に、その変数が初期化されていない場合に発生するエラーです。
このエラーは、特に関数内で変数を使用する際に注意が必要です。
ローカル変数が定義されていない状態でアクセスしようとすると、Pythonはこのエラーを発生させます。
UnboundLocalErrorの定義
UnboundLocalError は、Pythonの組み込み例外の一つで、ローカルスコープ内で変数が参照されるが、その変数が初期化されていない場合に発生します。
具体的には、関数内で変数を使用する際に、その変数がローカルとして扱われるが、実際には値が設定されていない場合にこのエラーが発生します。
UnboundLocalErrorの基本的な例
以下は、 UnboundLocalError が発生する基本的な例です。
このコードを実行すると、 UnboundLocalError: local variable 'value' referenced before assignment というエラーメッセージが表示されます。
これは、 value が関数内で初期化される前に参照されているためです。
UnboundLocalError は、他のエラーといくつかの点で異なります。
以下の表に、主な違いを示します。
エラー名 | 説明 | 発生条件 |
---|---|---|
UnboundLocalError | ローカル変数が初期化されていない状態で参照された場合に発生 | 関数内でローカル変数を参照するが、初期化されていない |
NameError | 定義されていない変数を参照した場合に発生 | グローバルスコープで変数が未定義の場合 |
TypeError | 型が不適切な操作を行った場合に発生 | 例えば、数値と文字列を加算しようとした場合 |
このように、 UnboundLocalError は特にローカルスコープに関連するエラーであり、他のエラーとは異なる条件で発生します。
UnboundLocalErrorの発生原因
UnboundLocalError は、主に変数のスコープや初期化に関連する問題から発生します。
以下に、具体的な発生原因を詳しく解説します。
関数内で変数を参照する際、その変数がローカルスコープに存在しない場合、 UnboundLocalError が発生します。
Pythonは、関数内で変数が初期化される前にその変数を参照しようとすると、このエラーを投げます。
このコードでは、 number が初期化される前に参照されているため、エラーが発生します。
グローバル変数とローカル変数の混同
グローバル変数とローカル変数を混同することも、 UnboundLocalError の原因となります。
関数内で同名のローカル変数を定義すると、Pythonはその変数をローカルとして扱います。
これにより、グローバル変数が参照できなくなり、エラーが発生することがあります。
この場合、 value はローカル変数として扱われ、初期化される前に参照されるため、エラーが発生します。
関数内での変数の再定義
関数内で変数を再定義する場合も、 UnboundLocalError が発生することがあります。
特に、同じ関数内で変数を初期化する前にその変数を参照すると、エラーが発生します。
このコードでは、 counter が初期化される前に参照されているため、エラーが発生します。
関数のスコープと変数のライフタイム
Pythonでは、変数のスコープとライフタイムが重要です。
関数内で定義された変数は、その関数のスコープ内でのみ有効です。
関数が終了すると、ローカル変数は消失します。
このため、関数内で変数を参照する際には、必ず初期化されていることを確認する必要があります。
この場合、 temp は条件文内でのみ初期化されているため、条件が満たされない場合に参照されるとエラーが発生します。
スコープの理解は、 UnboundLocalError を回避するために非常に重要です。
UnboundLocalErrorの対処法
UnboundLocalError を回避するためには、変数のスコープや初期化に注意を払う必要があります。
以下に、具体的な対処法を解説します。
グローバル変数の正しい使用方法
グローバル変数を関数内で使用する場合、 global キーワードを使って明示的にその変数がグローバルであることを示すことが重要です。
これにより、ローカル変数として扱われることを防ぎます。
このコードでは、 global キーワードを使用することで、 value がグローバル変数として正しく参照され、エラーが発生しません。
関数内でローカル変数を使用する場合は、必ず初期化してから参照するようにしましょう。
これにより、 UnboundLocalError を回避できます。
このコードでは、 number が初期化されているため、エラーは発生しません。
関数の引数として変数を渡す
関数に変数を引数として渡すことで、ローカル変数の初期化を避けることができます。
これにより、関数内での変数のスコープを明確にし、エラーを防ぐことができます。
このように、引数を使用することで、 UnboundLocalError を回避できます。
nonlocalキーワードの使用
ネストされた関数内で外側の関数の変数を参照したい場合は、 nonlocal キーワードを使用します。
これにより、外側のスコープの変数をローカルとして扱うことができます。
このコードでは、 nonlocal を使用することで、 inner_function 内で outer_function の counter を正しく参照し、エラーを回避しています。
UnboundLocalErrorの回避方法
UnboundLocalError を回避するためには、コーディングスタイルや変数の管理方法に注意を払うことが重要です。
以下に、具体的な回避方法を解説します。
コーディングスタイルの改善
明確で一貫したコーディングスタイルを採用することで、変数のスコープや初期化の問題を減少させることができます。
以下のポイントに注意しましょう。
- 変数の初期化を必ず行う
- 変数のスコープを明確にする
- コメントを使って変数の役割を説明する
これにより、コードの可読性が向上し、エラーを未然に防ぐことができます。
変数名を一貫して使用することで、混乱を避けることができます。
特に、グローバル変数とローカル変数で同じ名前を使用しないようにしましょう。
以下のポイントを考慮してください。
- グローバル変数にはプレフィックスを付ける(例: global_value )
- ローカル変数には関数名や用途に基づいた名前を付ける(例: local_counter )
このようにすることで、変数のスコープを明確にし、 UnboundLocalError を回避できます。
関数の設計とスコープの管理
関数を設計する際には、変数のスコープを意識して管理することが重要です。
以下の点に注意しましょう。
- 変数は必要なスコープ内でのみ定義する
- 関数の引数を利用して外部のデータを渡す
- ネストされた関数を使用する場合は、 nonlocal や global を適切に使用する
これにより、変数のライフタイムを適切に管理し、エラーを防ぐことができます。
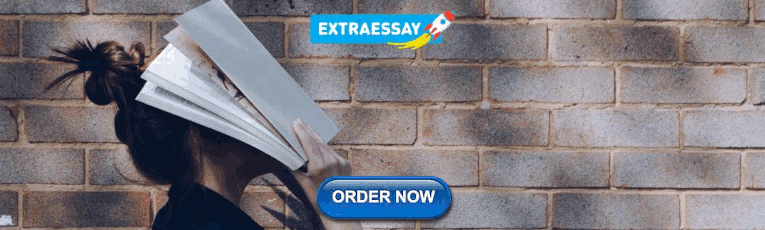
テストとデバッグの重要性
コードを書く際には、テストとデバッグを行うことが不可欠です。
以下の方法でエラーを早期に発見し、修正することができます。
- ユニットテストを作成して、関数の動作を確認する
- デバッグツールを使用して、変数のスコープや値を確認する
- エラーメッセージを注意深く読み、問題の原因を特定する
これにより、 UnboundLocalError を含むさまざまなエラーを未然に防ぐことができます。
テストとデバッグは、コードの品質を向上させるための重要なプロセスです。
UnboundLocalError を理解し、回避することは、さまざまなプロジェクトや分野で非常に重要です。
以下に、具体的な応用例を示します。
大規模プロジェクトでの変数管理
大規模なプロジェクトでは、複数の開発者が同じコードベースで作業するため、変数の管理が特に重要です。
以下のポイントに注意することで、 UnboundLocalError を回避できます。
- 明確な命名規則 : 変数名にプロジェクトのコンテキストを反映させることで、混乱を避ける。
- ドキュメンテーション : 変数の役割やスコープを文書化し、チーム全体で共有する。
- コードレビュー : 他の開発者によるレビューを通じて、潜在的なエラーを早期に発見する。
データサイエンスにおける変数のスコープ管理
データサイエンスのプロジェクトでは、データの前処理や分析を行う際に、変数のスコープを適切に管理することが重要です。
以下の方法でエラーを回避できます。
- 関数を利用したデータ処理 : データの前処理や分析を関数に分け、各関数内で変数を初期化する。
- 引数の活用 : データフレームや配列を関数の引数として渡し、スコープを明確にする。
- テストの実施 : 各関数の動作をテストし、エラーを早期に発見する。
Webアプリケーション開発でのエラー回避
Webアプリケーション開発では、ユーザーからの入力や外部APIとの連携が多いため、エラー処理が重要です。
- エラーハンドリング : 例外処理を適切に行い、 UnboundLocalError を含むエラーをキャッチする。
- スコープの明確化 : グローバル変数とローカル変数を明確に区別し、混同を避ける。
- ロギング : エラーが発生した際に、詳細なログを記録し、問題の特定を容易にする。
自動化スクリプトでのエラー防止
自動化スクリプトでは、エラーが発生すると処理が中断されるため、事前にエラーを防ぐことが重要です。
以下の方法で UnboundLocalError を回避できます。
- 変数の初期化 : スクリプトの冒頭で使用する変数を初期化し、参照エラーを防ぐ。
- 関数の利用 : 処理を関数に分け、各関数内で変数を管理することで、スコープを明確にする。
- テストの実施 : スクリプトを実行する前に、ユニットテストを行い、エラーを未然に防ぐ。
これらの応用例を通じて、 UnboundLocalError を理解し、適切に対処することが、さまざまなプロジェクトでの成功に繋がります。
UnboundLocalErrorが発生する具体的なシーンは?
UnboundLocalError は、関数内でローカル変数が初期化される前にその変数を参照しようとした場合に発生します。
ローカル変数は必ず初期化してから参照しましょう。
グローバル変数を使うべきか?
グローバル変数は便利ですが、使用する際には注意が必要です。
グローバル変数を使うべき場合は、以下のような状況です。
- 複数の関数で同じデータを共有する必要がある場合
- 状態を保持する必要がある場合
ただし、グローバル変数を多用すると、コードの可読性が低下し、バグの原因になることがあるため、必要最小限に留めることが推奨されます。
nonlocalキーワードの使い方は?
nonlocal キーワードは、ネストされた関数内で外側の関数の変数を参照する際に使用します。
以下のように使います。
例: nonlocal counter # 関数の外側で宣言されているcounter変数を参照
このように、 nonlocal を使うことで、外側のスコープの変数を正しく参照できます。
UnboundLocalError は、Pythonプログラミングにおいてよく見られるエラーであり、変数のスコープや初期化に関連しています。
この記事では、エラーの発生原因や対処法、回避方法、応用例について詳しく解説しました。
これらの知識を活用することで、エラーを未然に防ぎ、より良いコードを書くことができるでしょう。
ぜひ、実際のプロジェクトでこれらのポイントを意識してみてください。
当サイトはリンクフリーです。出典元を明記していただければ、ご自由に引用していただいて構いません。
- [Python] exceptionとは?発生原因や対処法・回避方法を解説
- [Python] EOFErrorとは?発生原因や対処法・回避方法を解説
- [Python] OSErrorとは?発生原因や対処法・回避方法を解説
- [Python] FileNotFoundErrorとは?発生原因や対処法・回避方法を解説
- [Python] MemoryErrorとは?発生原因や対処法・回避方法を解説
- [Python] TypeErrorとは?発生原因や対処法・回避方法を解説
- [Python] IsADirectoryErrorとは?発生原因や対処法・回避方法を解説
- [Python] SystemExitとは?発生原因や対処法・回避方法を解説
- [Python] AssertionErrorとは?発生原因や対処法・回避方法を解説
- [Python] ArithmeticErrorとは?発生原因や対処法・回避方法を解説
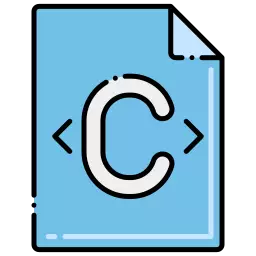
関連カテゴリーから探す
- exception (52)
- [Python] UnicodeDecodeErrorとは?発生原因や対処法・回避方法を解説
![unboundlocalerror local variable 'values' referenced before assignment shap [Python] RecursionErrorとは?発生原因や対処法・回避方法を解説](https://af-e.net/wp-content/uploads/2024/08/thumbnail-5840-300x158.png)
Access Comprehensive Templates to Optimize Workflow and Achieve Professional Goals lateralgdelphiteeter
Local Variable Referenced Before Assignment In Python Unboundlocalerror Local Variable K Means Refer

Unboundlocalerror local variable k_means referenced before assignment
Last updated: Apr 8, 2024 Reading time · 4 min
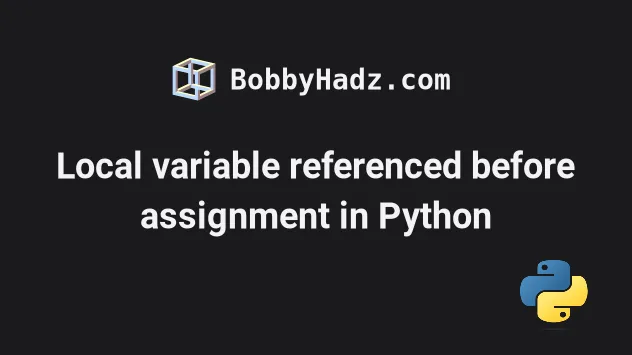
# Local variable referenced before assignment in Python
The Python “UnboundLocalError: Local variable referenced before assignment” occurs when we reference a local variable before assigning a value to it in a function.
To solve the error, mark the variable as global in the function definition, e.g. global my_var .
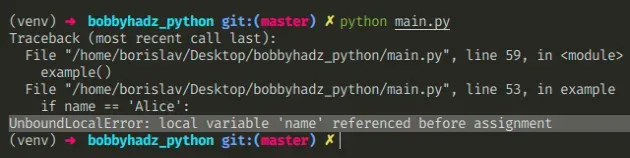
Here is an example of how the error occurs.
We assign a value to the name variable in the function.
This makes the name variable local to the function and the local name variable shadows the one in the global scope.
# Mark the variable as global to solve the error
To solve the error, mark the variable as global in your function definition.
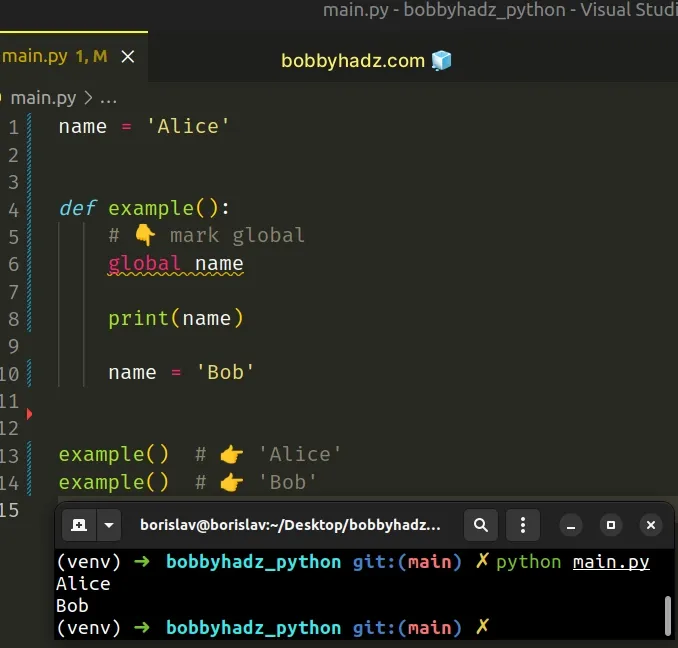
If a variable is assigned a value in a function’s body, it is a local variable unless explicitly declared as global .
# Local variables shadow global ones with the same name
You could reference the global name variable from inside the function but if you assign a value to the variable in the function’s body, the local variable shadows the global one.
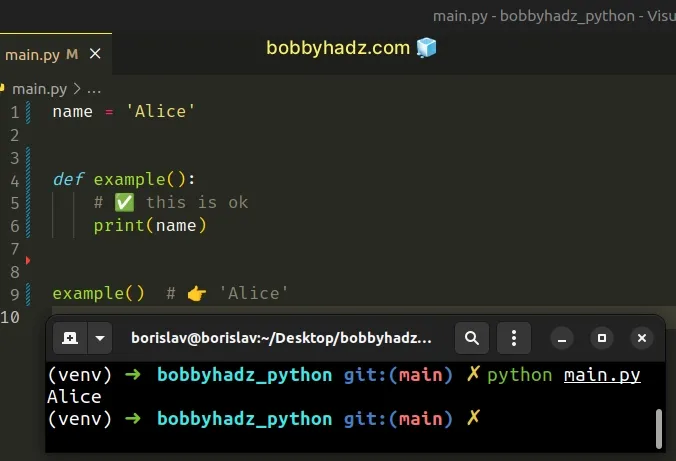
Accessing the name variable in the function is perfectly fine.
On the other hand, variables declared in a function cannot be accessed from the global scope.
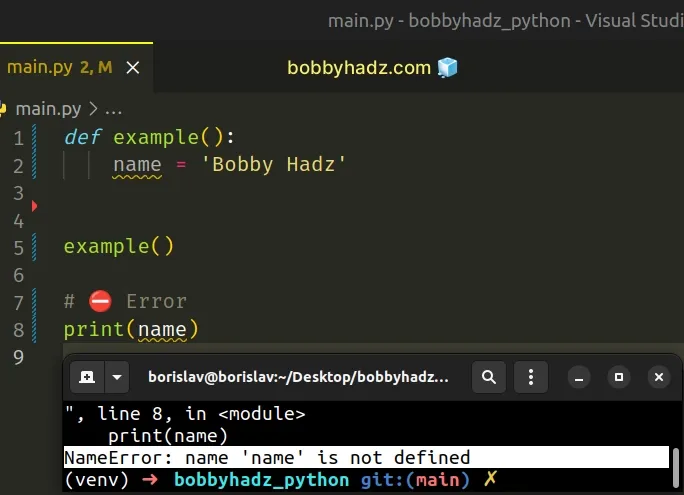
The name variable is declared in the function, so trying to access it from outside causes an error.
Make sure you don’t try to access the variable before using the global keyword, otherwise, you’d get the SyntaxError: name ‘X’ is used prior to global declaration error.
# Returning a value from the function instead
An alternative solution to using the global keyword is to return a value from the function and use the value to reassign the global variable.
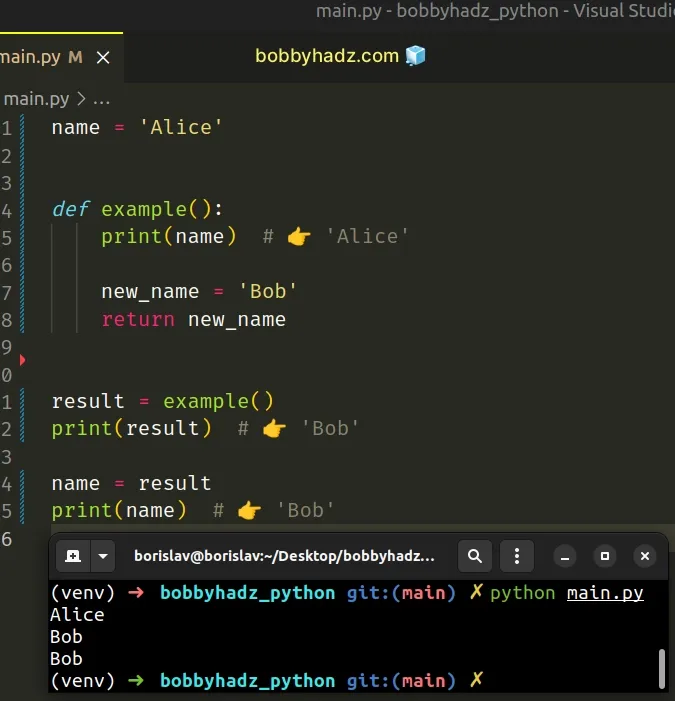
We simply return the value that we eventually use to assign to the name global variable.
# Passing the global variable as an argument to the function
You should also consider passing the global variable as an argument to the function.
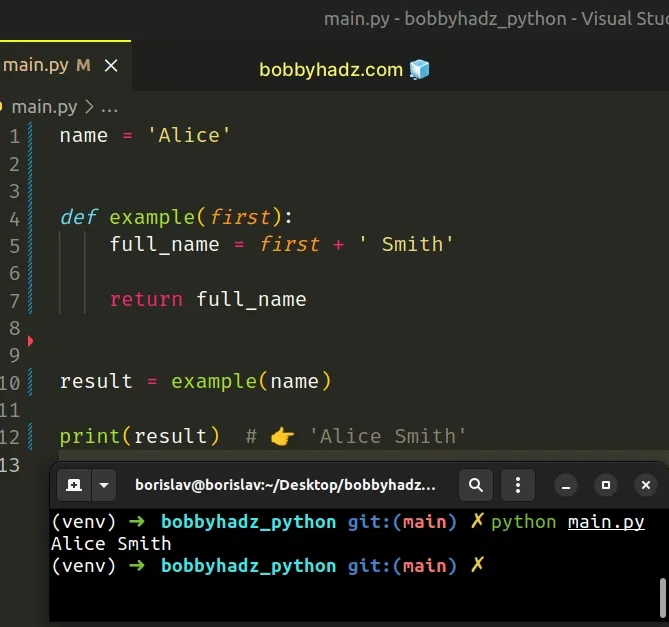
We passed the name global variable as an argument to the function.
When we assign a value to a variable in a scope, that variable becomes local to that scope and shadows variables with the same name in the outer scope.
If we assign a value to a variable in a function, the variable is assumed to be local unless explicitly declared as global .
# Assigning a value to a local variable from an outer scope
If you have a nested function and are trying to assign a value to the local variables from the outer function, use the nonlocal keyword.
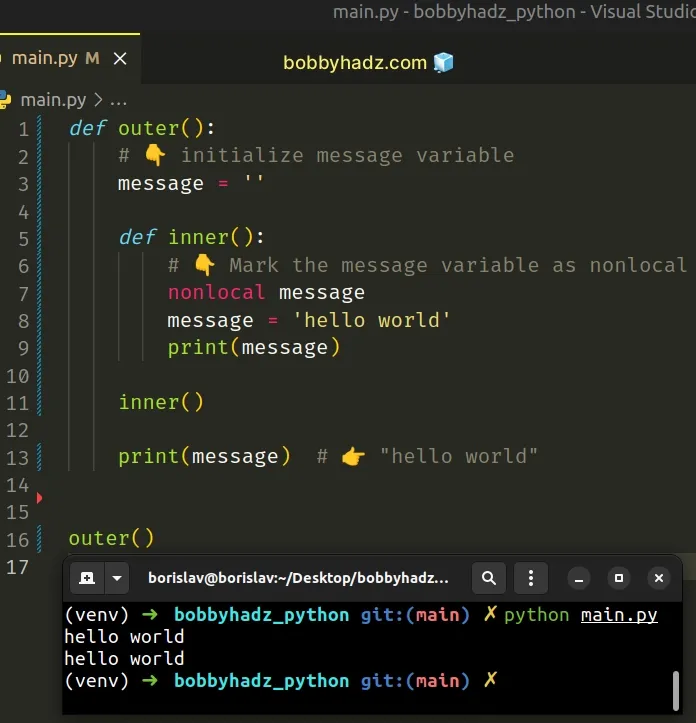
The nonlocal keyword allows us to work with the local variables of enclosing functions.
Note that we had to initialize the message variable in the outer function, but we were able to change its value in the inner function.
Had we not used the nonlocal statement, the call to the print() function would have returned an empty string.
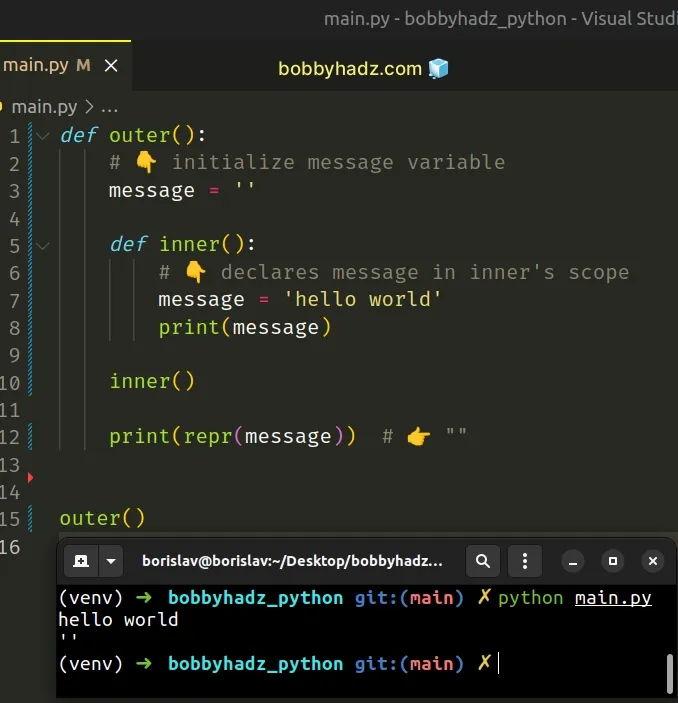
Printing the message variable on the last line of the function shows an empty string because the inner() function has its own scope.
Changing the value of the variable in the inner scope is not possible unless we use the nonlocal keyword.
Instead, the message variable in the inner function simply shadows the variable with the same name from the outer scope.
# Discussion
As shown in this section of the documentation, when you assign a value to a variable inside a function, the variable:
- Becomes local to the scope.
- Shadows any variables from the outer scope that have the same name.
The last line in the example function assigns a value to the name variable, marking it as a local variable and shadowing the name variable from the outer scope.
At the time the print(name) line runs, the name variable is not yet initialized, which causes the error.
The most intuitive way to solve the error is to use the global keyword.
The global keyword is used to indicate to Python that we are actually modifying the value of the name variable from the outer scope.
- If a variable is only referenced inside a function, it is implicitly global.
- If a variable is assigned a value inside a function’s body, it is assumed to be local, unless explicitly marked as global .
If you want to read more about why this error occurs, check out [this section] (this section) of the docs.
# Additional Resources
You can learn more about the related topics by checking out the following tutorials:
I wrote a book in which I share everything I know about how to become a better, more efficient programmer.
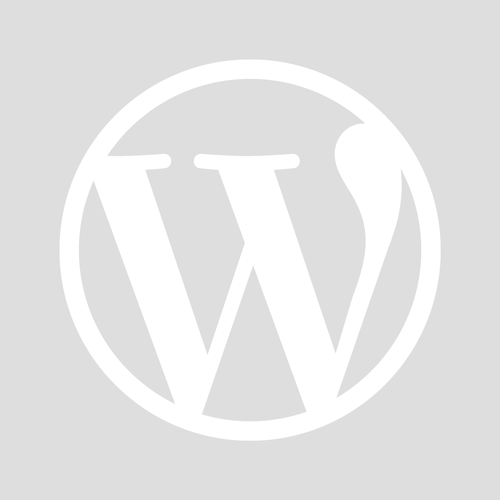
- Already have a WordPress.com account? Log in now.
- Subscribe Subscribed
- Copy shortlink
- Report this content
- View post in Reader
- Manage subscriptions
- Collapse this bar
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Streamlit error "UnboundLocalError: local variable 'dados' referenced before assignment"
I'm trying to plot some stock data using streamlit. I have a selectbox with two options: Home, Charts.
Home is the default page and is responsible to collect data from user, data as stock tickers, begin date and end date.
Charts is responsible to plot a line chart with those data.
My problem is in the Charts screen, because I can't read data altought I can read it in the Home page.
The error message is:
UnboundLocalError: local variable 'dados' referenced before assignment
My code is below:

- The traceback helps locate the failing line. Consider the line if submit: . Suppose there is no submit and so dados is not set. You try to use it anyway. – tdelaney Commented Aug 24, 2021 at 21:32
dados variable is defined inside choice == 'Home' block. So when you trying to use it inside choice == 'Charts': block with
code line it is not defined there. In order to use it in both blocks you should define it as a global variable / in scope containing both these methods.

- I literally used dados outside choice == 'Home' but I got the follow error: UnboundLocalError: local variable 'submit' referenced before assignment . Also I tried a few things with submit button, but nothing worked. – BeatrizGomes Commented Aug 24, 2021 at 21:55
- You are trying to USE it there, but it should be defined / initialized BEFORE that. – Prophet Commented Aug 24, 2021 at 21:57
- 2 I solved using st.session_state.dados insteado of dados = baixa_dados(...) . Thank you for the help! – BeatrizGomes Commented Aug 27, 2021 at 21:15
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged python pandas streamlit or ask your own question .
- The Overflow Blog
- Masked self-attention: How LLMs learn relationships between tokens
- Deedy Das: from coding at Meta, to search at Google, to investing with Anthropic
- Featured on Meta
- User activation: Learnings and opportunities
- Preventing unauthorized automated access to the network
- Feedback Requested: How do you use the tagged questions page?
Hot Network Questions
- How to write an Antagonist that is hot, manipulative, but has good reasoning for being the 'villain'?
- Which ancient philosopher compared thoughts to birds?
- What does "we are out"mean here?
- If a 'fire temple' was built in a gigantic city, with many huge perpetual flames inside, how could they keep smoke from bothering non-worshippers?
- Prove that of all triangles having same base and equal areas, the isosceles triangle has the least perimeter.
- Best way to replace a not yet faild drive
- When does derived tensor product commute with arbitrary products?
- What is the role of this suffix for an opamp?
- Java class subset of C++ std::list with efficient std::list::sort()
- How can I make a 2D FTL-lane map on a galaxy-wide scale?
- Why are METAR issued at 53 minutes of the hour?
- Showing that a ball is a convex set
- Lilypond Orchestral score: how to show duplicated tempo marking for violins?
- Does this work for page turns in a busy violin part?
- Matter made of neutral charges does not radiate?
- In a shell script, how do i wait for a volume to be available?
- How to format units inside math environment?
- Will a Palm tree in Mars be approximately 2.5 times taller than the same tree on Earth?
- What happens if parents refuse to name their newborn child?
- Can one freely add content to a quotation in square brackets?
- The meaning of the recursive type μt.t
- What is the origin of the many extra mnemonics in Manx Software Systems’ 8086 assembler?
- Writing horizontally right-to-left
- Are file attachments in email safe for transferring financial documents?
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[Bug] UnboundLocalError: local variable 'output_shape' referenced before assignment #22
acorderob commented Apr 4, 2023 • edited Loading
I get sometimes this error, trying to generate an image (even with no lycoris loras). Commit: |
The text was updated successfully, but these errors were encountered: |
acorderob commented Apr 4, 2023
Trying to debug the problem. The check for the module class in that function is failing, so the output_shape is never assigned. fails, but the variable has an object of that class! I don't know enough python to know what could be happening. |
Sorry, something went wrong.
KohakuBlueleaf commented Apr 5, 2023
I have checked and this can only happend when you have other lora extension that will modify the loaded lora or modify the load lora function. I have added a check for this, but the only solution is you may need to disable other lora function. |
acorderob commented Apr 5, 2023
The only other extension that seems to do something to loras would be "stable-diffusion-webui-composable-lora", but i have it disabled. I'll try removing it, just in case. |
KohakuBlueleaf commented Apr 6, 2023
It looks like it is some bug in webui (but I don't know how it happend) |
No branches or pull requests
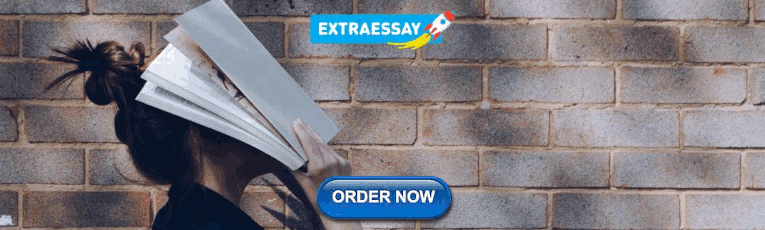
IMAGES
VIDEO
COMMENTS
order = convert_ordering (order, values) UnboundLocalError: local variable 'values' referenced before assignment. The text was updated successfully, but these errors were encountered: 👍 29. berkistayn, micqu, elleros, giemmecci, indiana-nikel-teck, JohnPaulR, chrislill, courentin, Scusemua, shadigoodarzi, and 19 more reacted with thumbs up ...
55 # out_names = shap_exp.output_names 56---> 57 order = convert_ordering(order, values) 58 59. UnboundLocalError: local variable 'values' referenced before assignment. and here is my code which produced the aforementioned error: ``print('summary plot of shap values - normal') shap.plots.beeswarm(shap_values, show=False)
Output. Hangup (SIGHUP) Traceback (most recent call last): File "Solution.py", line 7, in <module> example_function() File "Solution.py", line 4, in example_function x += 1 # Trying to modify global variable 'x' without declaring it as global UnboundLocalError: local variable 'x' referenced before assignment Solution for Local variable Referenced Before Assignment in Python
File "weird.py", line 5, in main. print f(3) UnboundLocalError: local variable 'f' referenced before assignment. Python sees the f is used as a local variable in [f for f in [1, 2, 3]], and decides that it is also a local variable in f(3). You could add a global f statement: def f(x): return x. def main():
seems to be a issue with this change in k -diffusion. Skip noise addition in DPM++ 2M SDE if eta is 0 crowsonkb/k-diffusion@d911c4b. issue seems to be at all samplers from DPM++ 2M SDE and DPM++ 3M SDE upping steps to over 100 also works as workaround.
Use a list comprehension to group every n, 3 in this case, values together, then map them to the values in table. The original function iterates through each group of 3, matches it to table , and adds the value to protein .
UnboundLocalError: local variable referenced before assignment. Example #1: Accessing a Local Variable. Solution #1: Passing Parameters to the Function. Solution #2: Use Global Keyword. Example #2: Function with if-elif statements. Solution #1: Include else statement. Solution #2: Use global keyword. Summary.
Avoid Reassignment of Global Variables. Below, code calculates a new value (local_var) based on the global variable and then prints both the local and global variables separately.It demonstrates that the global variable is accessed directly without being reassigned within the function.
UndboundLocalError: local variable referenced before assignment. MarcelloSilvestre February 29, 2024, 12:17pm 1. Hello all, I'm using PsychoPy 2023.2.3. Win 10 x64bits. I am having a few issues in my experiment, some of the errors I never saw in older versions of Psychopy. What I'm trying to do?
Steps fix: Please add an additional elif line for dictionaries and create a cohorts object. Steps to reproduce: Simply pass a dictionary to shap.plots.bar () The text was updated successfully, but these errors were encountered: Collaborator.
while True: tries = tries + 1. You can check if a variable is defined using. try: tries. except NameError: tries = 0. but I absolutely recommend against it. There is always a way to make sure a variable is defined and code which is uncertain if a variable is defined is badly designed.
The problem arises when in one of your routines, you write the statement "import os". When that happens, the scope issue arises because os is already defined globally, and you are defining it locally, but you already tried to access it before you define it locally. So the exact situation in stackoverflow post happens.
问题背景. 在Python编程中,UnboundLocalError: local variable 'xxx' referenced before assignment 是一个常见的错误,尤其是在写函数时可能会遇到。 这篇技术博客将详细介绍UnboundLocalError,为什么会发生,以及如何解决这个错误。. 1. 什么是UnboundLocalError? 在Python中,UnboundLocalError是一种特定的NameError,它会在尝试 ...
UnboundLocalError: local variable 'a' referenced before assignment The text was updated successfully, but these errors were encountered: 👍 17 callensm, bendesign55, nulladdict, rohanbanerjee, balovbohdan, drewszurko, hellotuitu, rribani, jeromesteve202, egmaziero, and 7 more reacted with thumbs up emoji
このコードを実行すると、UnboundLocalError: local variable 'value' referenced before assignmentというエラーメッセージが表示されます。 これは、valueが関数内で初期化される前に参照されているためです。 他のエラーとの違い. UnboundLocalErrorは、他のエラーといくつかの点で異なります。
This is happening because request.method == 'POST' is True (first condition passes) but form.is_valid() is False (second nested condition fails), which means the final return after the else is run but ctxt is not defined.. Perhaps you intended that final return to be indented as a part of the else clause?
UnboundLocalError: local variable 'mask_sharp' referenced before assignment #81. Open SuperMaximus1984 opened this issue Jul 27, 2023 · 3 comments Open ... sigmaY=1, borderType = cv2.BORDER_DEFAULT) UnboundLocalError: local variable 'mask_sharp' referenced before assignment ...
name = 'Alice' def example (first): full_name = first + ' Smith' return full_name result = example (name) print (result) # 👉️ 'Alice Smith'. We passed the name global variable as an argument to the function. When we assign a value to a variable in a scope, that variable becomes local to that scope and shadows variables with the same name in the outer scope.
UnboundLocalError: local variable 'decoded_samples' referenced before assignment; What should have happened? no errror throwhn and image beeign generated. Commit where the problem happens. 35c45df. What platforms do you use to access UI ? Windows. What browsers do you use to access the UI ? Google Chrome. Command Line Arguments. No response
dados variable is defined inside choice == 'Home' block. So when you trying to use it inside choice == 'Charts': block with. st.line_chart(dados) code line it is not defined there. In order to use it in both blocks you should define it as a global variable / in scope containing both these methods.
UnboundLocalError: local variable 'output_shape' referenced before assignment. The text was updated successfully, but these errors were encountered: All reactions. Copy link Author. acorderob commented Apr 4, 2023. Trying to debug the problem. The check for the module class in that function is failing, so the output_shape is never assigned.