Home » PHP Tutorial » PHP Null Coalescing Operator
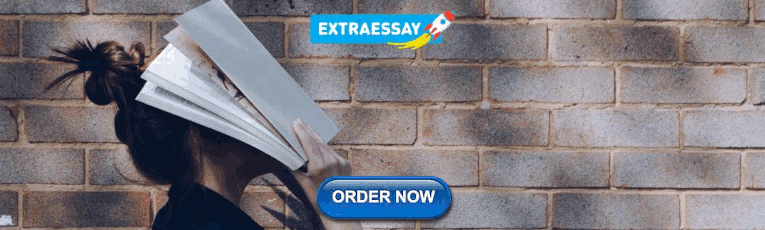
PHP Null Coalescing Operator
Summary : in this tutorial, you’ll learn about the PHP Null coalescing operator to assign a value to a variable if the variable doesn’t exist or null.
Introduction to the PHP null coalescing operator
When working with forms , you often need to check if a variable exists in the $_GET or $_POST by using the ternary operator in conjunction with the isset() construct. For example:
This example assigns the $_POST['name'] to the $name variable if $_POST['name'] exists and not null. Otherwise, it assigns the '' to the $name variable.
To make it more convenient, PHP 7.0 added support for a null coalescing operator that is syntactic sugar of a ternary operator and isset() :
In this example, the ?? is the null coalescing operator. It accepts two operands. If the first operand is null or doesn’t exist, the null coalescing operator returns the second operand. Otherwise, it returns the first one.
In the above example, if the variable name doesn’t exist in the $_POST array or it is null, the ?? operator will assign the string 'John' to the $name variable. See the following example:
As you can see clearly from the output, the ?? operator is like a gate that doesn’t allow null to pass through.
Stacking the PHP Null coalescing operators
PHP allows you to stack the null coalescing operators. For example:
In this example, since the $fullname , $first , and $last doesn’t exist, the $name will take the 'John' value.
PHP null coalescing assignment operator
The following example uses the null coalesing operator to assign the 0 to $counter if it is null or doesn’t exist:
The above statement repeats the variable $counter twice. To make it more concise, PHP 7.4 introduced the null coalescing assignment operator ( ??= ):
In this example, the ??= is the null coalescing assignment operator. It assigns the right operand to the left if the left operand is null. Otherwise, the coalescing assignment operator will do nothing.
It’s equivalent to the following:
In practice, you’ll use the null coalescing assignment operator to assign a default value to a variable if it is null.
- The null coalescing operator ( ?? ) is a syntactic sugar of the ternary operator and isset() .
- The null coalescing assignment operator assigns the right operand to the left one if the left operand is null.
Null Coalescing Operator in PHP: Embracing Graceful Defaults
Introduction.
The null coalescing operator introduced in PHP 7 provides a clean and succinct way to use a default value when a variable is null.
Getting Started with ‘??’
In PHP, the null coalescing operator (??) is a syntactic sugar construct that simplifies the task of checking for the existence and value of a variable. Prior to its introduction in PHP 7, developers would often utilize the isset() function combined with a ternary conditional to achieve a similar result. Let’s see this with a basic code example:
With the null coalescing operator, this simplifies to:
This approach immediately streamlines the code, making it more readable and easier to maintain.
Advancing with Null Coalescing
The operator is not only limited to simple variable checks but can be used with multiple operands, evaluating from left to right until it finds a non-null value. Let’s dive deeper with an advanced example:
This progresses through the $_GET, $_SESSION arrays, and ends with a literal if all else fails.
It also works seamlessly with functions and method calls. For instance:
In this scenario, if getUserFromDatabase($id) returns null, ‘fallback’ will be used.
Working with Complex Structures
The null coalescing operator is versatile, and can be directly applied to multi-dimensional arrays and even JSON objects. Consider the following example:
Let’s take it a step further with objects:
This code elegantly attempts to retrieve a profile picture, defaulting if nothing is returned.
Combining with Other PHP 7 Features
PHP 7 introduces other syntactic improvements such as the spaceship operator (<=>) for comparisons. Combine these with the null coalescing operator for powerful expressions. For example:
Real-World Scenarios
In real-world applications, embracing the null coalescing operator means focusing on the business logic rather than boilerplate checks. It particularly shines in scenarios involving configuration, forms, APIs, and conditional displays. The operator often becomes indispensable in modern PHP development workflows.
For instance, when processing API input:
Or, setting up a GUI with language fallbacks:
Good Practices and Pitfalls
While highly practical, there are scenarios where the null coalescing operator isn’t suitable. It should not replace proper validation and sanitization of user data, nor should it be overused in complex conditions where clearer logic may be needed for maintenance purposes. As with any tool in programming, using it judiciously is key.
Performance Implications
Performance-wise, the null coalescing operator is a favorable choice. Typically, it’s faster than combining isset() with a ternary operator due to reduced opcode generation. Let’s validate with a timed test:
You’re likely to notice slight but consistent speed advantages using the null coalescing operator.
The null coalescing operator in PHP streamlines default value assignment and contributes to cleaner code. As PHP continues to evolve, adopting such features not only leverages language efficiency but also enhances developer expressiveness and intent. In essence, the ?? operator fine-tunes the syntax dance that we perform every day as PHP developers.
Next Article: Why should you use PHP for web development?
Previous Article: Using 'never' return type in PHP (PHP 8.1+)
Series: Basic PHP Tutorials
Related Articles
- Using cursor-based pagination in Laravel + Eloquent
- Pandas DataFrame.value_counts() method: Explained with examples
- Constructor Property Promotion in PHP: Tutorial & Examples
- Understanding mixed types in PHP (5 examples)
- Union Types in PHP: A practical guide (5 examples)
- PHP: How to implement type checking in a function (PHP 8+)
- Symfony + Doctrine: Implementing cursor-based pagination
- Laravel + Eloquent: How to Group Data by Multiple Columns
- PHP: How to convert CSV data to HTML tables
- Nullable (Optional) Types in PHP: A practical guide (5 examples)
- Explore Attributes (Annotations) in Modern PHP (5 examples)
- An introduction to WeakMap in PHP (6 examples)
Search tutorials, examples, and resources
- PHP programming
- Symfony & Doctrine
- Laravel & Eloquent
- Tailwind CSS
- Sequelize.js
- Mongoose.js
In PHP 7.0, we got new null coalescing operator ( ?? ) which is a syntactic sugar of using isset() for check a value for null. PHP 7.4 has brought new syntactic sugar to assign default value to a variable if it is null.
Lets say, we have a variable called $student_name which should contain student name in it. But for some reason, sometimes student name may can't be found (null value), so we need to assign default value to it before using it. In PHP <7.4 , we would do something like this:
In line 5, we are checking if $student_name has been set, if not we are assigning 'Default Name' to it.
In PHP 7.4, we can use Null Coalescing Assignment Operator (??=) to do the same:
Above, Null Coalescing Assignment Operator checks if $student_name is null, if so it assign string(12) "Default Name" to it.
Best benefit of Null Coalescing Assignment Operator is when used for array element. lets say, we have an variable for a blog post which is array
Write comment about this article:
- Web Development
What Is the Null Coalescing Assignment Operator in PHP?
- Daniyal Hamid
- 29 Nov, 2021
Starting with PHP 7.4+, you can use the null coalescing assignment operator ( ??= ). It is a shorthand to assign a value to a variable if it hasn't been set already. Consider the following examples, which are all equivalent:
The null coalescing assignment operator only assigns the value if $x is null or not set. For example:
This post was published 29 Nov, 2021 by Daniyal Hamid . Daniyal currently works as the Head of Engineering in Germany and has 20+ years of experience in software engineering, design and marketing. Please show your love and support by sharing this post .
The PHP null coalescing operator (??) explained
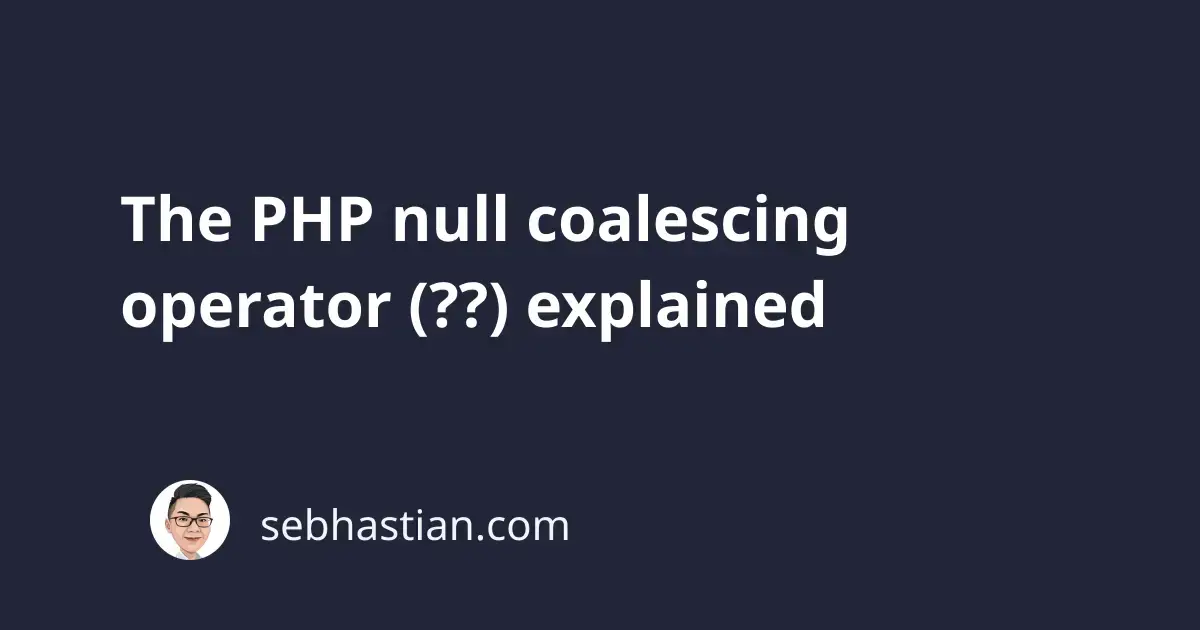
The operator returns the first operand when the value is not null. Otherwise, it returns the second operand.
Stacking the null coalescing operands in PHP
Null coalescing assignment operator in php.
And that’s how you assign a variable value using the null coalescing operator.
Take your skills to the next level ⚡️
PHP's double question mark, or the null coalescing operator
The null coalescing operator (??), or the double question mark
The null coalescing operator , or double question mark, was introduced in PHP 7.0 and is a handy shortcut that helps you write cleaner and more readable code. It’s represented by two question marks ?? .
Let’s say you want to get a value from a variable, but if that variable is not set or is null , you want to use a default value instead. You can use the ?? operator to do this in one step.
For example:
This line of code will set $name to $_GET['name'] if it’s set and not null. Otherwise, it will set $name to “Unknown”.
You can also chain them together like this:
This will check $foo first, then $bar , and use “baz” if neither are set and not null.
The null coalescing assignment operator (??=), or the double question mark equals
PHP 7.4 introduced a new shortcut, ??= (double question mark equals), also called the null coalescing assignment operator . This is used when you want to set a variable to a new value only if it’s currently not set or null.
It’s hard to make up a good example, but here’s a simplified one from this very blog’s codebase:
This will set $to to a new DateTime instance only if it’s not already set (or null in that case).
Be the first to comment!
Get help or share something of value with other readers!
- Summarize and talk to YouTube videos. Bypass ads, sponsors, chit-chat, and get to the point. Try Nobinge →
- Monitor the health of your apps: downtimes, certificates, broken links, and more. 20% off the first 3 months using the promo code CROZAT . Try Oh Dear for free →
- Keep the customers coming; monitor your Google rankings. 30% off your first month using the promo code WELCOME30 Try Wincher for free →
- Receive Slack Notifications from your Laravel App with a 10-minute Setup → Karan Datwani • 2d ago
- Freelance Web Dev Hub: Free Community for Web Developers → Ash Allen • 1w ago
- Laravel Exchange Rates v7.6.0 released! → Ash Allen • 1w ago
- Top 10 PHP Features You Can Use in 2024 → Karan Datwani • 1w ago
- Craft emails with Vue and Tailwind using Inertia Mailable → Yannick Decat • 1w ago
Null Coalescing Operator
The null coalescing operator ( ?? ) in PHP is a shorthand way of checking if a value is "null", meaning it's either NULL or undefined , and if it is, providing a default value in its place. It can be used as a cleaner and more concise alternative to the ternary operator or conditional statement.
The result of $a ?? $b is:
- if $a is defined, then $a ,
- if $a isn't defined, then $b .
Using the null coalescing operator
The basic syntax of the null coalescing operator is as follows:
In this example, $someVariable is checked for a value. If it has a value that is not "null", it is assigned to $data . If $someVariable is "null", the default value "Default value" is assigned to $data .
The most common use case for this operator is to set a default value for a variable. For example:
If the $user variable has a value, we can assume the user is logged in. Otherwise, we'll refer to the user as "Anonymous" .
Create a variable called $email . Its value should be $userEmail or a default value of false . Use the null coalescing operator.
Key takeaways
- The null coalescing operator is written with the ?? characters.
- PHP checks for a value on the left. If the value is not null or undefined , the value on the left will be returned.
- Otherwise, the value on the right of the operator is returned.
Please read this before commenting
PHP 7.4: Null Coalescing Assignment
With PHP 7.4 upcoming, it’s time to start exploring some of the new features that will be arriving alongside it. Here we cover the enhancements around the null coalescing operator , namely the introduction of the null coalescing assignment operator. (Sometimes referred to as the “null coalesce equal operator”)
In PHP 7 this was originally released, allowing a developer to simplify an isset() check combined with a ternary operator. For example, before PHP 7, we might have this code:
['username'] = (isset($data['username']) ? $data['username'] : 'guest'); |
When PHP 7 was released, we got the ability to instead write this as:
['username'] = $data['username'] ?? 'guest'; |
Now, however, when PHP 7.4 gets released, this can be simplified even further into:
['username'] ??= 'guest'; |
One case where this doesn’t work is if you’re looking to assign a value to a different variable, so you’d be unable to use this new option. As such, while this is welcomed there might be a few limited use cases.
So for example, this code from before PHP 7 could only be optimised once using the null coalescing operator, and not the assignment operator:
= (isset($_SESSION['username']) ? $_SESSION['username'] : 'guest'); |
= $_SESSION['username'] ?? 'guest'; |
01 Jan 2020
- development
- PHP History
- Install PHP
- Hello World
- PHP Constant
- Predefined Constants
- PHP Comments
- Parameters and Arguments
- Anonymous Functions
- Variable Function
- Arrow Functions
- Variadic Functions
- Named Arguments
- Callable Vs Callback
- Variable Scope
- If Condition
- If-else Block
- Break Statement
- Operator Precedence
- PHP Arithmetic Operators
- Assignment Operators
- PHP Bitwise Operators
- PHP Comparison Operators
- PHP Increment and Decrement Operator
- PHP Logical Operators
- PHP String Operators
- Array Operators
- Conditional Operators
- Ternary Operator
- PHP Enumerable
- PHP NOT Operator
- PHP OR Operator
- PHP Spaceship Operator
- AND Operator
- Exclusive OR
- Spread Operator
- Elvis Operator
Null Coalescing Operator
- PHP Data Types
- PHP Type Juggling
- PHP Type Casting
- PHP strict_types
- Type Hinting
- PHP Boolean Type
- PHP Iterable
- PHP Resource
- Associative Arrays
- Multidimensional Array
- Programming
- PHP Tutorial
The PHP null coalescing operator, denoted by ?? , is a convenient feature introduced in PHP 7 that simplifies the way developers handle the default values for variables that may be null.
Null Coalescing Operator Syntax
How does the null coalescing operator work, null coalescing operator examples, wrapping up.
It provides a concise and readable syntax for dealing with potentially null values without the need for verbose ternary expressions.
Let’s move into its syntax.
The syntax of the PHP null coalescing operator ( ?? ) is quite straightforward. It is used to handle situations where a variable may be null or not set, providing a default value if the variable is null. The basic syntax is as follows:
- $variable : The variable you want to check for null.
- $value : The value to use if $variable is not null.
- $default : The default value to use if $variable is null.
The following section provides an overview of how the null coalescing operator works. Let’s proceed.
This operation occurs in a single line, making the code more readable and concise compared to traditional conditional statements.
Here’s an example that shows you how it works:
The operator checks if the variable on the left side ( $variable in this case) is set and is not null. If it is set and not null, the expression evaluates to the value of $variable . If $variable is not set or is null, the expression evaluates to the value of $default .
This operator is especially useful when dealing with user input, configuration settings, or other scenarios where a variable might be null, and you want to provide a default value in case it is.
By moving into the section below, you will learn more about the null coalescing operator in PHP through examples.
Here is a basic example:
In this example, we are trying to retrieve the value of the username parameter from the $_GET array. If the username parameter exists and is not null, it will be assigned to the $username variable. However, if the username parameter is null or does not exist, the value ‘Guest’ will be assigned to $username . This is because of the null coalescing operator.
The null coalescing operator can also be chained together to provide default values for multiple variables. For example:
In this example, we are trying to retrieve the value of the username parameter from the $_GET array. If it is null or does not exist, we try to retrieve the value from the $_POST array. If this value is also null or does not exist, the value ‘Guest’ is assigned to $username .
The null coalescing operator is particularly useful when dealing with arrays or objects. Here’s an example:
In this example, we are trying to retrieve the value of the age key from the $person array. However, this key has a value of null. Using the null coalescing operator, we can assign the value ‘Unknown’ to the $age variable instead of null.
Moreover, it also can work with functions. For example:
In this example, we are calling the getUserName() function, which returns null. Using the null coalescing operator, we assign the value ‘Guest’ to $username instead of null.
Let’s summarize it.
The PHP 7 null coalescing operator streamlines the handling of default values for variables that may be null. Its concise and readable syntax simplifies the code, eliminating the need for verbose ternary expressions.
The operator, denoted by ?? , efficiently deals with situations where a variable may be null or unset, providing a default value if needed. The basic syntax involves checking a variable ( $variable ) and using a default value ( $default ) if the variable is null.
The operator proves beneficial in scenarios such as user input, configuration settings, or any case where a variable might be null, requiring a default value. Its single-line execution enhances code readability and conciseness, offering an alternative to traditional conditional statements.
Further exploration of the null coalescing operator includes chaining for multiple variables and its application with arrays, objects, and functions. Examples demonstrate its versatility, whether handling array elements, object properties, or function returns.
Did you find this tutorial useful?
Your feedback helps us improve our tutorials.
- Migrating from PHP 5.6.x to PHP 7.0.x
New features
Scalar type declarations.
Scalar type declarations come in two flavours: coercive (default) and strict. The following types for parameters can now be enforced (either coercively or strictly): strings ( string ), integers ( int ), floating-point numbers ( float ), and booleans ( bool ). They augment the other types introduced in PHP 5: class names, interfaces, array and callable .
The above example will output:
To enable strict mode, a single declare directive must be placed at the top of the file. This means that the strictness of typing for scalars is configured on a per-file basis. This directive not only affects the type declarations of parameters, but also a function's return type (see return type declarations , built-in PHP functions, and functions from loaded extensions.
Full documentation and examples of scalar type declarations can be found in the type declaration reference.
Return type declarations
PHP 7 adds support for return type declarations . Similarly to argument type declarations , return type declarations specify the type of the value that will be returned from a function. The same types are available for return type declarations as are available for argument type declarations.
Full documentation and examples of return type declarations can be found in the return type declarations . reference.
Null coalescing operator
The null coalescing operator ( ?? ) has been added as syntactic sugar for the common case of needing to use a ternary in conjunction with isset() . It returns its first operand if it exists and is not null ; otherwise it returns its second operand.
Spaceship operator
The spaceship operator is used for comparing two expressions. It returns -1, 0 or 1 when $a is respectively less than, equal to, or greater than $b . Comparisons are performed according to PHP's usual type comparison rules .
Constant arrays using define()
Array constants can now be defined with define() . In PHP 5.6, they could only be defined with const .
Anonymous classes
Support for anonymous classes has been added via new class . These can be used in place of full class definitions for throwaway objects:
Full documentation can be found in the anonymous class reference .
Unicode codepoint escape syntax
This takes a Unicode codepoint in hexadecimal form, and outputs that codepoint in UTF-8 to a double-quoted string or a heredoc. Any valid codepoint is accepted, with leading 0's being optional.
Closure::call()
Closure::call() is a more performant, shorthand way of temporarily binding an object scope to a closure and invoking it.
Filtered unserialize()
This feature seeks to provide better security when unserializing objects on untrusted data. It prevents possible code injections by enabling the developer to whitelist classes that can be unserialized.
The new IntlChar class seeks to expose additional ICU functionality. The class itself defines a number of static methods and constants that can be used to manipulate unicode characters.
In order to use this class, the Intl extension must be installed.
Expectations
Expectations are a backwards compatible enhancement to the older assert() function. They allow for zero-cost assertions in production code, and provide the ability to throw custom exceptions when the assertion fails.
While the old API continues to be maintained for compatibility, assert() is now a language construct, allowing the first parameter to be an expression rather than just a string to be evaluated or a bool value to be tested.
Full details on this feature, including how to configure it in both development and production environments, can be found on the manual page of the assert() language construct.
Group use declarations
Classes, functions and constants being imported from the same namespace can now be grouped together in a single use statement.
Generator Return Expressions
This feature builds upon the generator functionality introduced into PHP 5.5. It enables for a return statement to be used within a generator to enable for a final expression to be returned (return by reference is not allowed). This value can be fetched using the new Generator::getReturn() method, which may only be used once the generator has finished yielding values.
Being able to explicitly return a final value from a generator is a handy ability to have. This is because it enables for a final value to be returned by a generator (from perhaps some form of coroutine computation) that can be specifically handled by the client code executing the generator. This is far simpler than forcing the client code to firstly check whether the final value has been yielded, and then if so, to handle that value specifically.
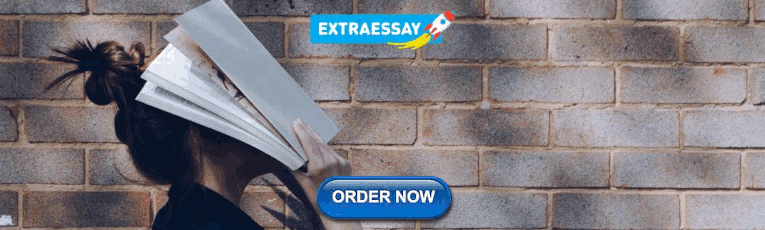
Generator delegation
Generators can now delegate to another generator, Traversable object or array automatically, without needing to write boilerplate in the outermost generator by using the yield from construct.
Integer division with intdiv()
The new intdiv() function performs an integer division of its operands and returns it.
Session options
session_start() now accepts an array of options that override the session configuration directives normally set in php.ini.
These options have also been expanded to support session.lazy_write , which is on by default and causes PHP to only overwrite any session file if the session data has changed, and read_and_close , which is an option that can only be passed to session_start() to indicate that the session data should be read and then the session should immediately be closed unchanged.
For example, to set session.cache_limiter to private and immediately close the session after reading it:
preg_replace_callback_array()
The new preg_replace_callback_array() function enables code to be written more cleanly when using the preg_replace_callback() function. Prior to PHP 7, callbacks that needed to be executed per regular expression required the callback function to be polluted with lots of branching.
Now, callbacks can be registered to each regular expression using an associative array, where the key is a regular expression and the value is a callback.
CSPRNG Functions
Two new functions have been added to generate cryptographically secure integers and strings in a cross platform way: random_bytes() and random_int() .
list() can always unpack objects implementing ArrayAccess
Previously, list() was not guaranteed to operate correctly with objects implementing ArrayAccess . This has been fixed.
Other Features
- Class member access on cloning has been added, e.g. (clone $foo)->bar() .
Improve This Page
User contributed notes 2 notes.

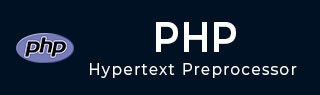
- PHP Tutorial
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook and Paypal Integration
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - Callbacks
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
The Null Coalescing operator is one of the many new features introduced in PHP 7. The word "coalescing" means uniting many things into one. This operator is used to replace the ternary operation in conjunction with the isset() function.
Ternary Operator in PHP
PHP has a ternary operator represented by the " ? " symbol. The ternary operator compares a Boolean expression and executes the first operand if it is true, otherwise it executes the second operand.
Let us use the ternary operator to check if a certain variable is set or not with the help of the isset() function, which returns true if declared and false if not.
It will produce the following output −
Now, let's remove the declaration of "x" and rerun the code −
The code will now produce the following output −
The Null Coalescing Operator
The Null Coalescing Operator is represented by the "??" symbol. It acts as a convenient shortcut to use a ternary in conjunction with isset(). It returns its first operand if it exists and is not null; otherwise it returns its second operand.
The first operand checks whether a certain variable is null or not (or is set or not). If it is not null, the first operand is returned, else the second operand is returned.
Take a look at the following example −
Now uncomment the first statement that sets $num to 10 and rerun the code −
It will now produce the following output −
A useful application of Null Coalescing operator is while checking whether a username has been provided by the client browser.
The following code reads the name variable from the URL. If indeed there is a value for the name parameter in the URL, a Welcome message for him is displayed. However, if not, the user is called Guest.
Assuming that this script "hello.php" is in the htdocs folder of the PHP server, enter http://localhost/hello.php?name=Amar in the URL, the browser will show the following message −
If http://localhost/hello.php is the URL, the browser will show the following message −
The Null coalescing operator is used as a replacement for the ternary operator’s specific case of checking isset() function. Hence, the following statements give similar results −
You can chain the "??" operators as shown below −
This will set the username to Guest if the variable $name is not set either by GET or by POST method.
PHP RFC: Null Coalescing Assignment Operator
- Version: 0.1.0
- Date: 2016-03-09
- Author: Midori Kocak, [email protected]
- Status: Implemented (in PHP 7.4)
- First Published at: http://wiki.php.net/rfc/null_coalesce_equal_operator
- Introduction
Combined assignment operators have been around since 1970's, appearing first in the C Programming Language. For example, $x = $x + 3 can be shortened to $x += 3 . With PHP being a web focused language, the ?? operator is often used to check something's existence like $username = $_GET['user'] ?? 'nobody'; However, because variable names are often much longer than $username, the use of ?? for self assignment creates repeated code, like $this->request->data['comments']['user_id'] = $this->request->data['comments']['user_id'] ?? ‘value’; . It is also intuitive to use combined assignment operator null coalesce checking for self assignment.
Despite ?? coalescing operator being a comparison operator, coalesce equal or ??= operator is an assignment operator. If the left parameter is null, assigns the value of the right paramater to the left one. If the value is not null, nothing is made.
The value of right-hand parameter is copied if the left-hand parameter is null.
- Proposed PHP Version(s)
This proposed for the next PHP 7.x.
- Patches and Tests
A pull request with a working implementation, targeting master, is here: https://github.com/php/php-src/pull/1795
As this is a language change, a 2/3 majority is required. A straight Yes/No vote is being held.
Voting started at 2016/03/24 16:08 and will be closed at 2016/04/02.
Approve Equal Null Coalesce Operator RFC and merge patch into master? | ||
---|---|---|
Real name | Yes | No |
(aharvey) | ||
(ajf) | ||
(brianlmoon) | ||
(colinodell) | ||
(daverandom) | ||
(davey) | ||
(dmitry) | ||
(dragoonis) | ||
(francois) | ||
(frozenfire) | ||
(galvao) | ||
(guilhermeblanco) | ||
(jwage) | ||
(klaussilveira) | ||
(krakjoe) | ||
(laruence) | ||
(lcobucci) | ||
(leigh) | ||
(levim) | ||
(lstrojny) | ||
(malukenho) | ||
(marcio) | ||
(mariano) | ||
(mbeccati) | ||
(mcmic) | ||
(mrook) | ||
(ocramius) | ||
(patrickallaert) | ||
(pauloelr) | ||
(pierrick) | ||
(pollita) | ||
(ralphschindler) | ||
(stas) | ||
(svpernova09) | ||
(toby) | ||
(tpunt) | ||
(trowski) | ||
(weierophinney) | ||
(yohgaki) | ||
(zeev) | ||
(zimt) | ||
This poll has been closed. |
Links to external references, discussions or RFCs
http://programmers.stackexchange.com/questions/134118/why-are-shortcuts-like-x-y-considered-good-practice
- Rejected Features
Keep this updated with features that were discussed on the mail lists.
- Show pagesource
- Old revisions
- Back to top
Table of Contents

Buckle up, fellow PHP enthusiast! We're loading up the rocket fuel for your coding adventures...
- Best Practices ,
PHP null coalescing operator
Hello everyone, I'm relatively new to PHP and I recently came across the term "null coalescing operator." I have heard people mention it, but I'm not entirely sure what it does and how it can be used in my code. To give you some context, I am working on a project where I need to handle situations where variables might be null. I've been using conditionals and ternary operators to check for null values, but I've heard that the null coalescing operator could simplify my code. So my question is, what exactly is the null coalescing operator in PHP? How does it work and what are some practical use cases for it? I would appreciate it if someone could explain it to me in a way that is beginner-friendly and provide some code examples to illustrate its usage. Thank you in advance for your help!
All Replies
Hey there! I've been using the null coalescing operator in my PHP projects for a while now, and I must say, it's a fantastic addition to the language. As the name suggests, it helps in tackling situations where you need to handle null values. Here's how it works: the null coalescing operator (`??`) allows you to assign a default value to a variable if it is null. It's a concise way to check if a variable is null and provide an alternative value in a single expression. For example, let's say you have a variable `$name` that might be null. Instead of using an if statement or ternary operator, you can use the null coalescing operator like this: $defaultName = "Guest"; $fullName = $name ?? $defaultName; In this case, if `$name` is not null, the value of `$name` will be assigned to `$fullName`. However, if `$name` is null, `$defaultName` will be assigned to `$fullName`. It's a neat shortcut that keeps your code clean and concise. Another use case is when working with arrays. Suppose you have an associative array `$data` that may or may not have a key called `'email'`. To assign a default email if the `'email'` key is missing or null, you can use the null coalescing operator like this: $defaultEmail = "[email protected]"; $email = $data['email'] ?? $defaultEmail; If `$data['email']` exists and is not null, `$email` will be assigned its value. Otherwise, the default email address will be assigned. I've found the null coalescing operator to be handy in scenarios where you want to provide fallback values without writing lengthy if-else blocks or ternary statements. It definitely simplifies the code and makes it more readable. I hope this helps you understand and use the null coalescing operator effectively in your PHP projects. Feel free to ask if you have any more questions!
Hey everyone, I just wanted to chime in and share my experience with the null coalescing operator in PHP. I've been using it extensively in my projects, and it has proven to be a real time-saver. One particular use case where the null coalescing operator shines is when dealing with form input data. Typically, you would handle form submissions by checking if each field has a value or is null before using it. With the null coalescing operator, you can simplify this process and handle default values elegantly. Let's say you have a form with various inputs, such as name, email, and phone number. To avoid errors when these fields are left blank, you can utilize the null coalescing operator to assign default values. php $name = $_POST['name'] ?? "Anonymous"; $email = $_POST['email'] ?? "[email protected]"; $phone = $_POST['phone'] ?? "N/A"; In this example, if the corresponding `$_POST` variable is not null, the value will be assigned to the respective variable. However, if the field is null (left blank in the form), the null coalescing operator will assign the provided default value instead. This approach saves you from writing verbose conditional statements to handle each form input individually. It condenses the code and makes it more readable. Not only does the null coalescing operator simplify handling null values, but it also allows for cleaner code in cases where API responses or database query results may contain null values. Instead of manually checking each value, you can leverage the operator and set appropriate defaults or fallback values as needed. Overall, the null coalescing operator in PHP is a powerful tool for gracefully handling null values and reducing code complexity. I highly recommend integrating it into your PHP projects for smoother data handling. If you have any questions or need further clarification, feel free to ask. Happy coding!
Hello all, I couldn't resist joining the discussion on the null coalescing operator in PHP. As a seasoned PHP developer, I can't stress enough how much it has improved my code readability and reduced boilerplate code. One practical use case I'd like to share is dealing with database results. We often fetch data from a database and assign it to variables, but sometimes certain fields may be null. That's where the null coalescing operator comes in handy. Imagine a scenario where you're fetching user records and want to display their names. Some users may not have specified their first name, so the database field would be null. Instead of writing lengthy if-else statements, the null coalescing operator provides an elegant solution. php $firstName = $user['first_name'] ?? "Unknown"; $lastName = $user['last_name'] ?? "Unknown"; With just a single line for each variable, we're able to handle null values effortlessly. If `first_name` or `last_name` is null, the corresponding variable will be assigned the default value of "Unknown". This not only streamlines the code but also improves legibility. It becomes much easier to track the intention of assigning default values to variables without bloating the codebase. Additionally, the null coalescing operator is a blessing when working with APIs. Sometimes APIs may have optional fields that could be null if not provided. By employing the null coalescing operator, you can quickly set fallback values to ensure your code continues to function seamlessly. To sum it up, the null coalescing operator is an essential tool that simplifies handling null values in PHP. Whether it's handling user input, database records, or API responses, this operator proves to be an elegant and efficient solution. If you have any questions or need further insights, feel free to ask. Happy coding, everyone!
More Topics Related to PHP
- What are the recommended PHP versions for different operating systems?
- Can I install PHP without root/administrator access?
- How can I verify the integrity of the PHP installation files?
- Are there any specific considerations when installing PHP on a shared hosting environment?
- Is it possible to install PHP alongside other programming languages like Python or Ruby?
More Topics Related to Code Examples
- What is the syntax for defining constants in PHP?
- How do I access elements of an array in PHP?
- How do I declare a null variable in PHP?
- What are resources used for in PHP?
More Topics Related to Variables
- How do I declare a variable in PHP?
- What is the difference between a constant and a variable in PHP?
- How do I handle type casting in PHP?
- Can a variable name start with a number in PHP?
- Are variables case-sensitive in PHP?
Popular Tags
- Best Practices
- Web Development
- Documentation
- Implementation

New to LearnPHP.org Community?

Ready to learn even more PHP ? Take my free Beginner’s Guide to PHP video series at https://johnmorrisonline.com/learnphp
Get this source code along with 1000s of other lines of code (including for a CMS, Social Network and more) as a supporting listener of the show at https://johnmorrisonline.com/patreon
P.S. If you liked the show, give it a like and share with the communities and people you think will benefit. And, you can always find all my tutorials, source code and exclusive courses on Patreon .
Join 7,700 Other Freelancers Who've Built Thriving Freelance Businesses
Over 7,700 other freelancers have started thriving freelance businesses using the information on this blog. Are you next? Subscribe below to get notified whenever a new article is posted and create your own success story:
You might also like

Complete HTML Tutorial for Beginners
Description Are you interested in starting a career in web development? This beginner-friendly HTML tutorial is the perfect starting point for you. In this comprehensive

25 Common Upwork Proposal Mistakes to Avoid at All Costs
Your ability to write high-quality proposals will determine your success on Upwork. Period. It’s also no secret that creating a great proposal requires time, effort,

45 Proven Techniques to (Drastically) Improve Your Upwork Proposal Writing Skills
As a platform connecting talented freelancers with clients from all around the globe, Upwork offers incredible opportunities to showcase your skills and secure rewarding projects.

Cheat Codes: 32 Upwork Proposal Hacks You Need to Know
Crafting an effective Upwork proposal is a crucial skill for freelancers seeking success on the platform. Your proposal serves as your first impression and can

Why Your Upwork Proposals Aren’t Getting Accepted (And How to Fix It)
As a freelancer on Upwork, you know that submitting a proposal is just the first step in the process of landing a job. But what

How to Make Your Upwork Proposal Unforgettable: Top Tips for Leaving a Lasting Impression
Welcome to the wonderful world of Upwork proposals! If you’re looking to land that dream project, then you’ll need to learn how to make a

JOHN MORRIS
I’m a 15-year veteran of freelance web development. I’ve worked with bestselling authors and average Joe’s next door. These days, I focus on helping other freelancers build their freelance business and their lifestyles.
Latest Shorts
Can I get a job just knowing HTML and CSS?
Is it still worth learning HTML and CSS in 2023?
How do I teach myself HTML?
Where do I write my HTML code?
What is the starting code for HTML?
Which software is best for HTML?
Latest Posts
Success stories, ready to add your name here.

Tim Covello
75 SEO and website clients now. My income went from sub zero to over 6K just last month. Tracking 10K for next month. Seriously, you changed my life.

Michael Phoenix
By the way, just hit 95K for the year. I can’t thank you enough for everything you’ve taught me. You’ve changed my life. Thank you!

Stephanie Korski
I started this 3 days ago, following John’s suggestions, and I gained the Upwork Rising Talent badge in less than 2 days. I have a call with my first potential client tomorrow. Thanks, John!

Jithin Veedu
John is the man! I followed his steps and I am flooded with interviews in a week. I got into two Talent clouds. The very next day, I got an invitation from the talent specialists from Upwork and a lot more. I wanna shout out, he is the best in this. Thanks John for helping me out!

Divyendra Singh Jadoun
After viewing John’s course, I made an Upwork account and it got approved the same day. Amazingly, I got my first job the very same day, I couldn’t believe it, I thought maybe I got it by coincidence. Anyways I completed the job and received my first earnings. Then, after two days, I got another job and within a week I got 3 jobs and completed them successfully. All the things he says seem to be minute but have a very great impact on your freelancing career.

I’ve been in an existential crisis for the last week about what the heck I’m doing as a business owner. Even though I’ve been a business for about a year, I’m constantly trying to think of how to prune and refine services. This was very personable and enjoyable to watch. Usually, business courses like this are dry and hard to get through…. repeating the same things over and over again. This was a breath of fresh air. THANK YOU.

Waqas Abdul Majeed
I’ve definitely learnt so much in 2.5 hours than I’d learn watching different videos online on Youtube and reading tons of articles on the web. John has a natural way of teaching, where he is passionately diving in the topics and he makes it very easy to grasp — someone who wants you to really start running your business well by learning about the right tools and implement them in your online business. I will definitely share with many of the people I know who have been struggling for so long, I did find my answers and I’m sure will do too.

Scott Plude
I have been following John Morris for several years now. His instruction ranges from beginner to advanced, to CEO-level guidance. I have referred friends and clients to John, and have encouraged my own daughter to pay attention to what he says. All of his teachings create wealth for me (and happiness for my clients!) I can’t speak highly enough about John, his name is well known in my home.

John is a fantastic and patient tutor, who is not just able to share knowledge and communicate it very effectively – but able to support one in applying it. However, I believe that John has a very rare ability to go further than just imparting knowledge and showing one how to apply it. He is able to innately provoke one’s curiosity when explaining and demonstrating concepts, to the extent that one can explore and unravel their own learning journey. Thanks very much John!

Misrab Mohamed
John has been the most important person in my freelance career ever since I started. Without him, I would have taken 10 or 20 years more to reach the position I am at now (Level 2 seller on Fiverr and Top Rated on Upwork).
© 2021 IDEA ENGINE LLC. All rights reserved
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Php Null coalescing operator
I am trying to understand how the null coalescing operator does really work. So, I tested many examples after reading the documentation in php.net and some posts on stackoverflow.
However, I can't understand this code:
since it's equivalent to (from php.net#null-coalescing )
and since isset(false) generates a fatal error .
Could, please, someone explain to me?
- null-coalescing-operator

- Being 'equivalent to' does not mean the same as. – Nigel Ren Commented May 17, 2018 at 13:52
- from the docs : "In particular, this operator does not emit a notice if the left-hand side value does not exist, just like isset(). This is especially useful on array keys." – Kaddath Commented May 17, 2018 at 13:55
- Like it says, it's syntactic sugar for a common use-case of using a ternary operator in conjunction with isset . If that's not what you're doing, this isn't the operator you need. The docs could possibly be worded a little better, in fairness. – iainn Commented May 17, 2018 at 13:55
- Note that it is named the " Null Coalescing Operator", not the "False Coalescing Operator". – Sammitch Commented May 17, 2018 at 18:06
Null coalescing operator returns its first operand if it exists and is not NULL;
Otherwise it returns its second operand.
In your case first operand is false so it is assigned to the variable. For e.g; if you initialize null to first operand then it will assign second operands value as shown.

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged php php-7.1 null-coalescing-operator or ask your own question .
- The Overflow Blog
- Where developers feel AI coding tools are working—and where they’re missing...
- He sold his first company for billions. Now he’s building a better developer...
- Featured on Meta
- User activation: Learnings and opportunities
- Preventing unauthorized automated access to the network
- Should low-scoring meta questions no longer be hidden on the Meta.SO home...
- Announcing the new Staging Ground Reviewer Stats Widget
Hot Network Questions
- I want a smooth orthogonalization process
- How to make a wall of unicode symbols useful?
- Is a private third party allowed to take things to court?
- What causes, and how to avoid, finger numbness?
- tan 3θ = cot(−θ)
- What evidence exists for the historical name of Kuwohi Mountain (formerly Clingmans Dome)?
- Expected value of a matrix = matrix of expected value?
- Place some or all of the White Chess pieces on a chessboard in such a way that none of them can legally move
- How can I draw a wedge of a cylinder?
- A scrambled word which can't be guessed without all of its letters
- Is this a balanced way to implement the "sparks" spell from Skyrim into D&D?
- What exactly do I buy when I buy an index-following ETF?
- How do I avoid getting depressed after receiving edits?
- Why does the Rdson limit from a MOSFET’s SOA graph not match its output characteristics curve?
- Is it common in modern classical music for timpani to play chromatic passages?
- is it okay to mock a database when writing unit test?
- What is the mechanical equivalent of an electronic AND gate?
- Player sprite becomes smaller during attack animation (Java)
- Story from the mid-20th century about a biochemist who finds youth algae
- Is there any language which distinguishes between “un” as in “not” and “un” as in “inverse”?
- Image localisation and georeference
- Is the Earth still capable of massive volcanism, like the kind that caused the formation of the Siberian Traps?
- Alien Weekends!
- Easily unload gravel from pickup truck
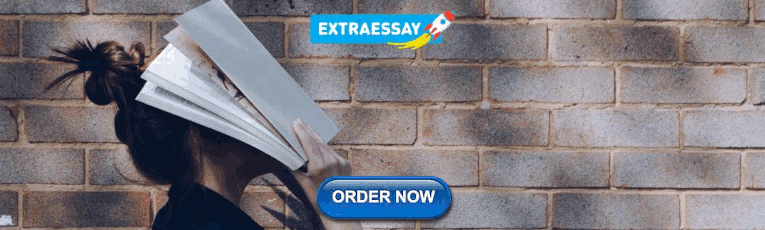
IMAGES
VIDEO
COMMENTS
Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers; Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand; OverflowAI GenAI features for Teams; OverflowAPI Train & fine-tune LLMs; Labs The future of collective knowledge sharing; About the company Visit the blog
Summary: in this tutorial, you'll learn about the PHP Null coalescing operator to assign a value to a variable if the variable doesn't exist or null.. Introduction to the PHP null coalescing operator. When working with forms, you often need to check if a variable exists in the $_GET or $_POST by using the ternary operator in conjunction with the isset() construct.
Assignment Operators. The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". ... An exception to the usual assignment by value behaviour within PHP occurs with object s, ... null coalescing operator; Improve This Page. Learn How To Improve This Page • Submit a Pull Request • Report a Bug
The null coalescing operator introduced in PHP 7 provides a clean and succinct way to use a default value when a variable is null. ... The null coalescing operator in PHP streamlines default value assignment and contributes to cleaner code. As PHP continues to evolve, adopting such features not only leverages language efficiency but also ...
In PHP 7.0, we got new null coalescing operator (??) which is a syntactic sugar of using isset() for check a value for null. PHP 7.4 has brought new syntactic sugar to assign default value to a variable if it is null.
Starting with PHP 7.4+, you can use the null coalescing assignment operator (??=).It is a shorthand to assign a value to a variable if it hasn't been set already.
The PHP null coalescing operator is a new syntax introduced in PHP 7. The operator returns the first operand if it exists and not null. Otherwise, it returns the second operand. It's a nice syntactic sugar that allows you to shorten the code for checking variable values using the isset() function. Now you've learned how the null coalescing ...
The null coalescing operator (??), or the double question mark. The null coalescing operator, or double question mark, was introduced in PHP 7.0 and is a handy shortcut that helps you write cleaner and more readable code. It's represented by two question marks ??. Let's say you want to get a value from a variable, but if that variable is ...
Null Coalescing Operator. The null coalescing operator (??) in PHP is a shorthand way of checking if a value is "null", meaning it's either NULL or undefined, and if it is, providing a default value in its place.It can be used as a cleaner and more concise alternative to the ternary operator or conditional statement.
Here we cover the enhancements around the null coalescing operator, namely the introduction of the null coalescing assignment operator. (Sometimes referred to as the "null coalesce equal operator") Basics. In PHP 7 this was originally released, allowing a developer to simplify an isset() check combined with a ternary operator. For example ...
Null Coalescing Operator. The PHP null coalescing operator, denoted by ??, is a convenient feature introduced in PHP 7 that simplifies the way developers handle the default values for variables that may be null. It provides a concise and readable syntax for dealing with potentially null values without the need for verbose ternary expressions.
This feature builds upon the generator functionality introduced into PHP 5.5. It enables for a return statement to be used within a generator to enable for a final expression to be returned (return by reference is not allowed). This value can be fetched using the new Generator::getReturn() method, which may only be used once the generator has ...
The Null Coalescing operator is one of the many new features introduced in PHP 7. The word "coalescing" means uniting many things into one. This operator is used to replace the ternary operation in conjunction with the isset() function. Ternary Operator in PHP. PHP has a ternary operator represented by the "?" symbol. The ternary operator ...
PHP RFC: Null Coalescing Assignment Operator. Version: 0.1.0. Date: 2016-03-09. Author: Midori Kocak, [email protected]. Status: Implemented (in PHP 7.4) ... It is also intuitive to use combined assignment operator null coalesce checking for self assignment. Proposal.
By employing the null coalescing operator, you can quickly set fallback values to ensure your code continues to function seamlessly. To sum it up, the null coalescing operator is an essential tool that simplifies handling null values in PHP. Whether it's handling user input, database records, or API responses, this operator proves to be an ...
PHP 7's Null Coalescing Operator: How and Why You Should Use It. By John Morris ; August 2, 2016; PHP Code Snippets; null coalescing operator, php, php7; PHP 7's null coalescing operator is handy shortcut along the lines of the ternary operator. Here's what it is, how to use it and why:
2. Null coalescing operator returns its first operand if it exists and is not NULL; Otherwise it returns its second operand. In your case first operand is false so it is assigned to the variable. For e.g; if you initialize null to first operand then it will assign second operands value as shown.