Copy assignment operator
(C++11) | ||||
(C++11) | ||||
(C++11) |
General topics | ||||
statement |
loop | ||||
loop (C++11) |
loop | ||||
loop |
statement | ||||
statement |
(C++11) | ||||
Literals | ||||
(C++11) | ||||
(C++11) |
expression |
pointer | ||||
(C++11) | ||||
(C++11) |
(C++11) | ||||
A copy assignment operator of class T is a non-template non-static member function with the name operator = that takes exactly one parameter of type T , T & , const T & , volatile T & , or const volatile T & . A type with a public copy assignment operator is CopyAssignable .
Syntax Explanation Implicitly-declared copy assignment operator Deleted implicitly-declared copy assignment operator Trivial copy assignment operator Implicitly-defined copy assignment operator Notes Copy and swap Example |
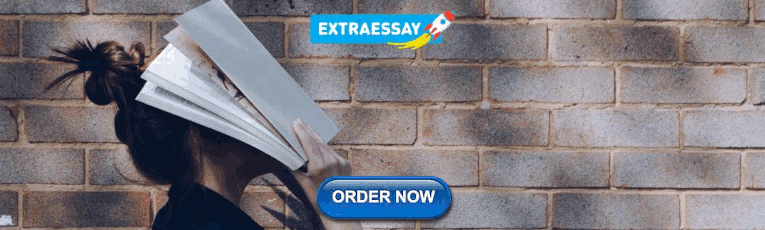
[ edit ] Syntax
class_name class_name ( class_name ) | (1) | (since C++11) | |||||||
class_name class_name ( const class_name ) | (2) | (since C++11) | |||||||
class_name class_name ( const class_name ) = default; | (3) | (since C++11) | |||||||
class_name class_name ( const class_name ) = delete; | (4) | (since C++11) | |||||||
[ edit ] Explanation
- Typical declaration of a copy assignment operator when copy-and-swap idiom can be used
- Typical declaration of a copy assignment operator when copy-and-swap idiom cannot be used
- Forcing a copy assignment operator to be generated by the compiler
- Avoiding implicit copy assignment
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type ( struct , class , or union ), the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B& or const volatile B &
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M& or const volatile M &
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) . (Note that due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument)
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( const T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default .
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Deleted implicitly-declared copy assignment operator
The implicitly-declared or defaulted copy assignment operator for class T is defined as deleted in any of the following is true:
- T has a non-static data member that is const
- T has a non-static data member of a reference type.
- T has a non-static data member that cannot be copy-assigned (has deleted, inaccessible, or ambiguous copy assignment operator)
- T has direct or virtual base class that cannot be copy-assigned (has deleted, inaccessible, or ambiguous move assignment operator)
- T has a user-declared move constructor
- T has a user-declared move assignment operator
[ edit ] Trivial copy assignment operator
The implicitly-declared copy assignment operator for class T is trivial if all of the following is true:
- T has no virtual member functions
- T has no virtual base classes
- The copy assignment operator selected for every direct base of T is trivial
- The copy assignment operator selected for every non-static class type (or array of class type) memeber of T is trivial
A trivial copy assignment operator makes a copy of the object representation as if by std:: memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is not deleted or trivial, it is defined (that is, a function body is generated and compiled) by the compiler. For union types, the implicitly-defined copy assignment copies the object representation (as by std:: memmove ). For non-union class types ( class and struct ), the operator performs member-wise copy assignment of the object's bases and non-static members, in their initialization order, using, using built-in assignment for the scalars and copy assignment operator for class types.
The generation of the implicitly-defined copy assignment operator is deprecated (since C++11) if T has a user-declared destructor or user-declared copy constructor.
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either prvalue such as a nameless temporary or xvalue such as the result of std:: move ), and selects the copy assignment if the argument is lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
[ edit ] Copy and swap
Copy assignment operator can be expressed in terms of copy constructor, destructor, and the swap() member function, if one is provided:
T & T :: operator = ( T arg ) { // copy/move constructor is called to construct arg swap ( arg ) ; // resources exchanged between *this and arg return * this ; } // destructor is called to release the resources formerly held by *this
For non-throwing swap(), this form provides strong exception guarantee . For rvalue arguments, this form automatically invokes the move constructor, and is sometimes referred to as "unifying assignment operator" (as in, both copy and move).
[ edit ] Example
- Graphics and multimedia
- Language Features
- Unix/Linux programming
- Source Code
- Standard Library
- Tips and Tricks
- Tools and Libraries
- Windows API
- Copy constructors, assignment operators,
Copy constructors, assignment operators, and exception safe assignment

MyClass& other ); MyClass( MyClass& other ); MyClass( MyClass& other ); MyClass( MyClass& other ); |
MyClass* other ); |
MyClass { x; c; std::string s; }; |
MyClass& other ) : x( other.x ), c( other.c ), s( other.s ) {} |
); |
print_me_bad( std::string& s ) { std::cout << s << std::endl; } print_me_good( std::string& s ) { std::cout << s << std::endl; } std::string hello( ); print_me_bad( hello ); print_me_bad( std::string( ) ); print_me_bad( ); print_me_good( hello ); print_me_good( std::string( ) ); print_me_good( ); |
, ); |
=( MyClass& other ) { x = other.x; c = other.c; s = other.s; * ; } |
< T > MyArray { size_t numElements; T* pElements; : size_t count() { numElements; } MyArray& =( MyArray& rhs ); }; |
<> MyArray<T>:: =( MyArray& rhs ) { ( != &rhs ) { [] pElements; pElements = T[ rhs.numElements ]; ( size_t i = 0; i < rhs.numElements; ++i ) pElements[ i ] = rhs.pElements[ i ]; numElements = rhs.numElements; } * ; } |
<> MyArray<T>:: =( MyArray& rhs ) { MyArray tmp( rhs ); std::swap( numElements, tmp.numElements ); std::swap( pElements, tmp.pElements ); * ; } |
< T > swap( T& one, T& two ) { T tmp( one ); one = two; two = tmp; } |
<> MyArray<T>:: =( MyArray tmp ) { std::swap( numElements, tmp.numElements ); std::swap( pElements, tmp.pElements ); * ; } |
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Assignment operators
- 8 contributors
expression assignment-operator expression
assignment-operator : one of = *= /= %= += -= <<= >>= &= ^= |=
Assignment operators store a value in the object specified by the left operand. There are two kinds of assignment operations:
simple assignment , in which the value of the second operand is stored in the object specified by the first operand.
compound assignment , in which an arithmetic, shift, or bitwise operation is performed before storing the result.
All assignment operators in the following table except the = operator are compound assignment operators.
Assignment operators table
Operator | Meaning |
---|---|
Store the value of the second operand in the object specified by the first operand (simple assignment). | |
Multiply the value of the first operand by the value of the second operand; store the result in the object specified by the first operand. | |
Divide the value of the first operand by the value of the second operand; store the result in the object specified by the first operand. | |
Take modulus of the first operand specified by the value of the second operand; store the result in the object specified by the first operand. | |
Add the value of the second operand to the value of the first operand; store the result in the object specified by the first operand. | |
Subtract the value of the second operand from the value of the first operand; store the result in the object specified by the first operand. | |
Shift the value of the first operand left the number of bits specified by the value of the second operand; store the result in the object specified by the first operand. | |
Shift the value of the first operand right the number of bits specified by the value of the second operand; store the result in the object specified by the first operand. | |
Obtain the bitwise AND of the first and second operands; store the result in the object specified by the first operand. | |
Obtain the bitwise exclusive OR of the first and second operands; store the result in the object specified by the first operand. | |
Obtain the bitwise inclusive OR of the first and second operands; store the result in the object specified by the first operand. |
Operator keywords
Three of the compound assignment operators have keyword equivalents. They are:
Operator | Equivalent |
---|---|
C++ specifies these operator keywords as alternative spellings for the compound assignment operators. In C, the alternative spellings are provided as macros in the <iso646.h> header. In C++, the alternative spellings are keywords; use of <iso646.h> or the C++ equivalent <ciso646> is deprecated. In Microsoft C++, the /permissive- or /Za compiler option is required to enable the alternative spelling.
Simple assignment
The simple assignment operator ( = ) causes the value of the second operand to be stored in the object specified by the first operand. If both objects are of arithmetic types, the right operand is converted to the type of the left, before storing the value.
Objects of const and volatile types can be assigned to l-values of types that are only volatile , or that aren't const or volatile .
Assignment to objects of class type ( struct , union , and class types) is performed by a function named operator= . The default behavior of this operator function is to perform a member-wise copy assignment of the object's non-static data members and direct base classes; however, this behavior can be modified using overloaded operators. For more information, see Operator overloading . Class types can also have copy assignment and move assignment operators. For more information, see Copy constructors and copy assignment operators and Move constructors and move assignment operators .
An object of any unambiguously derived class from a given base class can be assigned to an object of the base class. The reverse isn't true because there's an implicit conversion from derived class to base class, but not from base class to derived class. For example:
Assignments to reference types behave as if the assignment were being made to the object to which the reference points.
For class-type objects, assignment is different from initialization. To illustrate how different assignment and initialization can be, consider the code
The preceding code shows an initializer; it calls the constructor for UserType2 that takes an argument of type UserType1 . Given the code
the assignment statement
can have one of the following effects:
Call the function operator= for UserType2 , provided operator= is provided with a UserType1 argument.
Call the explicit conversion function UserType1::operator UserType2 , if such a function exists.
Call a constructor UserType2::UserType2 , provided such a constructor exists, that takes a UserType1 argument and copies the result.
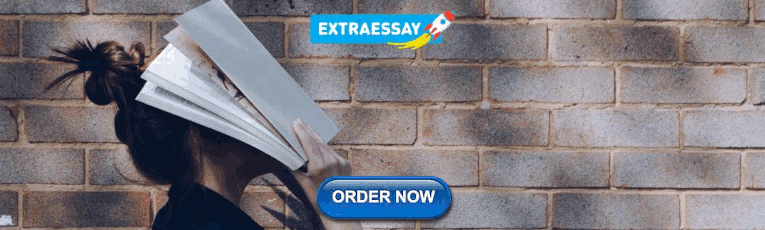
Compound assignment
The compound assignment operators are shown in the Assignment operators table . These operators have the form e1 op = e2 , where e1 is a non- const modifiable l-value and e2 is:
an arithmetic type
a pointer, if op is + or -
a type for which there exists a matching operator *op*= overload for the type of e1
The built-in e1 op = e2 form behaves as e1 = e1 op e2 , but e1 is evaluated only once.
Compound assignment to an enumerated type generates an error message. If the left operand is of a pointer type, the right operand must be of a pointer type, or it must be a constant expression that evaluates to 0. When the left operand is of an integral type, the right operand must not be of a pointer type.
Result of built-in assignment operators
The built-in assignment operators return the value of the object specified by the left operand after the assignment (and the arithmetic/logical operation in the case of compound assignment operators). The resultant type is the type of the left operand. The result of an assignment expression is always an l-value. These operators have right-to-left associativity. The left operand must be a modifiable l-value.
In ANSI C, the result of an assignment expression isn't an l-value. That means the legal C++ expression (a += b) += c isn't allowed in C.
Expressions with binary operators C++ built-in operators, precedence, and associativity C assignment operators
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
22.3 — Move constructors and move assignment
(Note: You may only get 4 outputs if your compiler elides the return value from function generateResource())
The move constructor and move assignment operator are simple. Instead of deep copying the source object (a) into the implicit object, we simply move (steal) the source object’s resources. This involves shallow copying the source pointer into the implicit object, then setting the source pointer to null.
The flow of the program is exactly the same as before. However, instead of calling the copy constructor and copy assignment operators, this program calls the move constructor and move assignment operators. Looking a little more deeply:
The compiler will create an implicit move constructor and move assignment operator if all of the following are true:
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
C++ Assignment Operator Overloading
Prerequisite: Operator Overloading
The assignment operator,”=”, is the operator used for Assignment. It copies the right value into the left value. Assignment Operators are predefined to operate only on built-in Data types.
- Assignment operator overloading is binary operator overloading.
- Overloading assignment operator in C++ copies all values of one object to another object.
- Only a non-static member function should be used to overload the assignment operator.
We can’t directly use the Assignment Operator on objects. The simple explanation for this is that the Assignment Operator is predefined to operate only on built-in Data types. As the class and objects are user-defined data types, so the compiler generates an error.
here, a and b are of type integer, which is a built-in data type. Assignment Operator can be used directly on built-in data types.
c1 and c2 are variables of type “class C”. Here compiler will generate an error as we are trying to use an Assignment Operator on user-defined data types.
The above example can be done by implementing methods or functions inside the class, but we choose operator overloading instead. The reason for this is, operator overloading gives the functionality to use the operator directly which makes code easy to understand, and even code size decreases because of it. Also, operator overloading does not affect the normal working of the operator but provides extra functionality to it.
Now, if the user wants to use the assignment operator “=” to assign the value of the class variable to another class variable then the user has to redefine the meaning of the assignment operator “=”. Redefining the meaning of operators really does not change their original meaning, instead, they have been given additional meaning along with their existing ones.
Please Login to comment...
Similar reads.
- cpp-operator
- cpp-operator-overloading
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Class Assignment Operators
I made the following operator overloading test:
The assignment operator behaves as-expected, outputting the address of the other instance.
Now, how would I actually assign something from the other instance? For example, something like this:
- operator-overloading
- assignment-operator
- You don't need either, but it still looks odd that you have an assignment operator and a destructor, but no copy constructor. As per the Rule of Three, if you need either, you'll likely need all three. – sbi Commented Dec 22, 2010 at 14:54
- @sbi Of course. This is just some test code, though. – Maxpm Commented Dec 22, 2010 at 15:24
- Still, reflexes kick in when I see that. I also noted that you pass a std::string object per copy instead of const reference. You might want to read this . – sbi Commented Dec 22, 2010 at 15:32
5 Answers 5
The code you've shown would do it. No one would consider it to be a particularly good implementation, though.
This conforms to what is expected of an assignment operator:
BTW, you talk about "other class", but you have only one class, and multiple instances of that class.

- Ben, actually it would be better to provide a swap() member function and call that. Nevertheless, this is better than assigning. – sbi Commented Dec 22, 2010 at 14:21
The traditional canonical form of the assignment operator looks like this:
(you don't want to invoke the copy constructor for assignment, too) and it returns a reference to *this .
A naive implementation would assign each data member individually:
(Note that this is exactly what the compiler-generated assignment operator would do, so it's pretty useless to overload it. I take it that this is for exercising, though.)
A better approach would be to employ the Copy-And-Swap idiom . (If you find GMan's answer too overwhelming, try mine , which is less exhaustive. :) ) Note that C&S employs the copy constructor and destructor to do assignment and therefore requires the object to be passed per copy, as you had in your question:
- I know you know about copy-and-swap, why did you declare the parameter as a reference? – Ben Voigt Commented Dec 22, 2010 at 13:51
- @Ben: Thanks. I've added a note that, using c&s, the object should be copied. Old habits die hard, I guess. (Oh, and I'm not sure what's a "ninja edit", BTW.) – sbi Commented Dec 22, 2010 at 13:56
- In this case, it was a ninja edit because you made the changes Ben was suggesting as he was suggesting them. – Bill Commented Dec 22, 2010 at 18:00
almost all said, a few notes:
- check for self-assignment, i.e. if (&other != this) // assign
- look here for an excellent guide on operator overloading
- 1 If your assignment operator needs a check for self-assignment, chances are there's a better implementation. Good implementations (like Copy-And-Swap) don't need that test (which puts the burden of checking for the rare case on every assignment). – sbi Commented Dec 22, 2010 at 14:06
- 2 <shameless_plug> We also have an operator overloading FAQ here on SO now: stackoverflow.com/questions/4421706/operator-overloading . </shameless_plug> – sbi Commented Dec 22, 2010 at 14:06
- @sbi: thanks for the ref, I'll read it one day ;). The one I mention is short and easy for beginners, giving just bare essentials. I'll also read up the C&S one day, but as for self-test overhead - seems that C&S has an overhead of copying and in many cases memory allocation (if your class contains strings, vectors etc.), so it should have a "handle with care" label, isn't it? – davka Commented Dec 22, 2010 at 14:21
- 1 @davka: The one you linked to is questionable, though. Also, C&S has no overhead. I have explained why it doesn't. . In short: assignment is tearing down old state, and building up new state by copying data from another object. That's exactly what copy-constructor and destructor do, and C&S manages to employ them in the right order to be exception-safe. – sbi Commented Dec 22, 2010 at 14:26
- 1 @davka: When swapping, you allocate for the new data, copy the new data, swap old and new data, and deallocate the old data. When assigning, you deallocate the old data, allocate for the new data, and copy the data (and you pray allocation won't fail and catch you with your pants down). But swapping is supposed to be O(1) and non-throwing, so it doesn't factor into the runtime. (For example, with std::vector swapping will swap two pointers. Comparing to the O(N) of copying and the O(VeryLooong) of allocation, this is neglectable.) – sbi Commented Dec 22, 2010 at 16:30
Traditionnaly the assignment operator and the copy constructor are defined passing a const reference, and not with a copy by value mechanism.
EDIT: I corrected because I had put code that didnt return the TestClass& (c.f. @sbi 's answer)
- 1 The new common practice actually does pass the RHS by value. It's called the copy-and-swap idiom . – Ben Voigt Commented Dec 22, 2010 at 13:50
- And just an instictive automatic repulsion about RHS by value... ( without having looked a single second at the thourough SO subject about copy and swap idiom)... RHS by value while using polymorphism has meant such a hundred of bugs in my career... it will take me hours to be convinced to using RHS by value ;-) – Stephane Rolland Commented Dec 22, 2010 at 14:12
You are correct about how to copy the contents from the other class. Simple objects can just be assigned using operator= .
However, be wary of cases where TestClass contains pointer members -- if you just assign the pointer using operator= , then both objects will have pointers pointing to the same memory, which may not be what you want. You may instead need to make sure you allocate some new memory and copy the pointed-to data into it so both objects have their own copy of the data. Remember you also need to properly deallocate the memory already pointed to by the assigned-to object before allocating a new block for the copied data.
By the way, you should probably declare your operator= like this:
This is the general convention used when overloading operator= . The return statement allows chaining of assignments (like a = b = c ) and passing the parameter by const reference avoids copying Other on its way into the function call.
- 1 The new common practice actually does pass the RHS by value. It's called the copy-and-swap idiom . – Ben Voigt Commented Dec 22, 2010 at 13:51
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c++ class operators operator-overloading assignment-operator or ask your own question .
- The Overflow Blog
- How to build open source apps in a highly regulated industry
- Community Products Roadmap Update, July 2024
- Featured on Meta
- We spent a sprint addressing your requests — here’s how it went
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
- Policy: Generative AI (e.g., ChatGPT) is banned
- What makes a homepage useful for logged-in users
- The [lib] tag is being burninated
Hot Network Questions
- Con permiso to enter your own house?
- What’s the highest salary the greedy king can arrange for himself?
- Can LP be solved using the previous solution during branching?
- Plausible reasons for the usage of Flying Ships
- Don't make noise. OR Don't make a noise
- Understanding symbol of a latching push switch
- Why do I see low voltage in a repaired underground cable?
- Staying in USA longer than 3 months
- What does a letter "R" means in a helipad?
- How to handle a missing author on an ECCV paper submission after the deadline?
- Need help implementing a 16x13bit ROM
- Word split between two lines is not found in man pages search
- Can someone explain the Trump immunity ruling?
- Wait a minute, this *is* 1 across!
- Are there examples of triple entendres in English?
- Travel to Mexico from India, do I need to pay a fee?
- Is there a way to do artificial gravity testing of spacecraft on the ground in KSP?
- Evil God Challenge: What if an evil god is just trolling humanity and that explains why there's good in the world?
- Where can I place a fire near my bed to gain the benefits but not suffer from the smoke?
- Bypass flow and apex triggers when executing DML on batch apex
- Do Christians believe that Jews and Muslims go to hell?
- Position where last x halfmoves are determined
- Why didn't Jimmy Neutron realize immediately when he read the note on the refrigerator that the note is phony, as the note says "son or daughter..."?
- exploded pie chart, circumscribing arc, and text labels
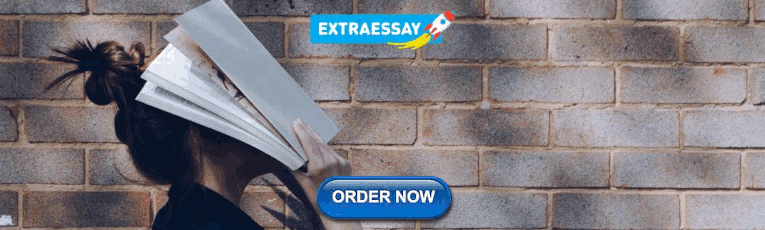
IMAGES
VIDEO
COMMENTS
23. Yes, default operator= is a shallow copy. By the way, the actual difference between shallow copy and deep copy becomes visible when the class has pointers as member fields. In the absence of pointers, there is no difference (to the best of my knowledge)! To know the difference between them, see these topics (on stackoverflow itself): What ...
the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial. A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD types) are trivially copy-assignable.
Output: can't use default assignment operator. The compiler doesn't create default assignment operator in the following cases: 1. Class has a non-static data member of a const type or a reference type. 2. Class has a non-static data member of a type that has an inaccessible copy assignment operator. 3.
21.12 — Overloading the assignment operator. Alex November 27, 2023. The copy assignment operator (operator=) is used to copy values from one object to another already existing object. As of C++11, C++ also supports "Move assignment". We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
The copy assignment operator selected for every non-static class type (or array of class type) memeber of T is trivial. A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD types) are trivially copy-assignable.
Note that none of the following constructors, despite the fact that. they could do the same thing as a copy constructor, are copy. constructors: 1. 2. MyClass( MyClass* other ); MyClass( const MyClass* other ); or my personal favorite way to create an infinite loop in C++: MyClass( MyClass other );
Use an assignment operator operator= that returns a reference to the class type and takes one parameter that's passed by const reference—for example ClassName& operator=(const ClassName& x);. Use the copy constructor. If you don't declare a copy constructor, the compiler generates a member-wise copy constructor for you.
Definition. A defaulted comparison operator function is a non-template comparison operator function (i.e. <=>, ==, !=, <, >, <=, or >=) satisfying all following conditions: . It is a non-static member or friend of some class C.; It is defined as defaulted in C or in a context where C is complete.; It has two parameters of type const C & or two parameters of type C, where the implicit object ...
C++ compiler implicitly provides a copy constructor, if no copy constructor is defined in the class. A bitwise copy gets created, if the Assignment operator is not overloaded. Consider the following C++ program. Explanation: Here, t2 = t1; calls the assignment operator, same as t2.operator= (t1); and Test t3 = t1; calls the copy constructor ...
In situations like these, you'll need to override C++'s default behavior by providing your own copy constructors and assignment operators. The Rule of Three There's a well-established C++ principle called the "rule of three" that almost always identifies the spots where you'll need to write your own copy constructor and assignment operator.
The built-in assignment operators return the value of the object specified by the left operand after the assignment (and the arithmetic/logical operation in the case of compound assignment operators). The resultant type is the type of the left operand. The result of an assignment expression is always an l-value.
The move assignment operator is called whenever it is selected by overload resolution, e.g. when an object appears on the left-hand side of an assignment expression, where the right-hand side is an rvalue of the same or implicitly convertible type.. Move assignment operators typically "steal" the resources held by the argument (e.g. pointers to dynamically-allocated objects, file descriptors ...
C++11 defines two new functions in service of move semantics: a move constructor, and a move assignment operator. Whereas the goal of the copy constructor and copy assignment is to make a copy of one object to another, the goal of the move constructor and move assignment is to move ownership of the resources from one object to another (which is typically much less expensive than making a copy).
In those situations where copy assignment cannot benefit from resource reuse (it does not manage a heap-allocated array and does not have a (possibly transitive) member that does, such as a member std::vector or std::string), there is a popular convenient shorthand: the copy-and-swap assignment operator, which takes its parameter by value (thus working as both copy- and move-assignment ...
The copy assignment operator, often just called the "assignment operator", is a special case of assignment operator where the source (right-hand side) and destination (left-hand side) are of the same class type. It is one of the special member functions, which means that a default version of it is generated automatically by the compiler if the ...
1) Do not allow assignment of one object to other object. We can create our own dummy assignment operator and make it private. 2) Write your own assignment operator that does deep copy. Same is true for Copy Constructor. Following is an example of overloading assignment operator for the above class. #include<iostream>.
The assignment operator,"=", is the operator used for Assignment. It copies the right value into the left value. Assignment Operators are predefined to operate only on built-in Data types. Assignment operator overloading is binary operator overloading. Overloading assignment operator in C++ copies all values of one object to another object.
The traditional canonical form of the assignment operator looks like this: TestClass& operator=(const TestClass& Other); (you don't want to invoke the copy constructor for assignment, too) and it returns a reference to *this.. A naive implementation would assign each data member individually: